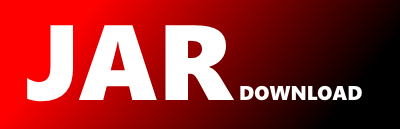
com.alogient.cameleon.sdk.content.dao.model.Template Maven / Gradle / Ivy
The newest version!
package com.alogient.cameleon.sdk.content.dao.model;
import java.io.Serializable;
import java.util.Set;
import javax.persistence.Basic;
import javax.persistence.CascadeType;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.FetchType;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
import javax.persistence.NamedNativeQueries;
import javax.persistence.NamedNativeQuery;
import javax.persistence.OneToMany;
import javax.persistence.OrderBy;
import javax.persistence.Table;
@Entity
@Table(name = "Template")
@NamedNativeQueries( { @NamedNativeQuery(name = "getTemplate", query = "SELECT " + "t.* " + "FROM " + "Template AS t " + "WHERE " + "t.TemplateName = :templateName", resultClass = Template.class) })
public class Template implements Serializable {
/**
* Serial version unique identifier
*/
private static final long serialVersionUID = -3621096640068284425L;
/**
* Primary key
*/
private Integer templateId;
/**
* Unique name for the template
*/
private String templateName;
/**
* Client side uri of the template
*/
private String templateUri;
/**
* FIXME Identify what this field is and add a correct comment
*/
private Boolean isListView;
/**
* The parts of this template
*/
private Set parts;
/**
* @return the templateId
*/
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
@Column(name = "TemplateID", nullable = false)
public Integer getTemplateId() {
return templateId;
}
/**
* @param templateId the templateId to set
*/
public void setTemplateId(Integer templateId) {
this.templateId = templateId;
}
/**
* @return the templateName
*/
@Column(name = "TemplateName", nullable = false, length = 256)
@Basic(optional = false)
public String getTemplateName() {
return templateName;
}
/**
* @param templateName the templateName to set
*/
public void setTemplateName(String templateName) {
this.templateName = templateName;
}
/**
* @return the templateUri
*/
@Column(name = "TemplateUrl", nullable = true, length = 1024)
@Basic(optional = true)
public String getTemplateUri() {
return templateUri;
}
/**
* @param templateUri the templateUri to set
*/
public void setTemplateUri(String templateUri) {
this.templateUri = templateUri;
}
/**
* @return the isListView
*/
@Column(name = "IsListView", nullable = false)
@Basic(optional = true)
public Boolean getIsListView() {
return isListView;
}
/**
* @param isListView the isListView to set
*/
public void setIsListView(Boolean isListView) {
this.isListView = isListView;
}
/**
* @return the parts
*/
@OneToMany(fetch = FetchType.LAZY, mappedBy = "template", cascade = CascadeType.ALL)
@OrderBy("partOrder ASC")
public Set getParts() {
return parts;
}
/**
* @param parts the parts to set
*/
public void setParts(Set parts) {
this.parts = parts;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy