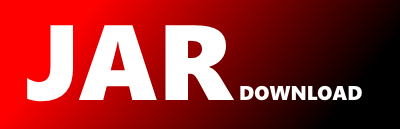
com.alogient.cameleon.sdk.content.dao.model.Video Maven / Gradle / Ivy
package com.alogient.cameleon.sdk.content.dao.model;
import java.io.Serializable;
import java.util.Set;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.FetchType;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
import javax.persistence.OneToMany;
import javax.persistence.Table;
/**
* @author alord
*/
@Entity
@Table(name = "Video")
public class Video implements Serializable {
/**
* Serial version unique identifier
*/
private static final long serialVersionUID = -7391016329307843431L;
/**
* This entitie's primary key
*/
private Integer videoId;
/**
* Is this video external or internal
*/
private Boolean isExternal;
/**
* The actual videos
*/
private Set videos;
/**
* @return the videoId
*/
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
@Column(name = "ID", nullable = false)
public Integer getVideoId() {
return videoId;
}
/**
* @param videoId the videoId to set
*/
public void setVideoId(Integer videoId) {
this.videoId = videoId;
}
/**
* @return the isExternal
*/
@Column(name = "ExternalSource", nullable = false)
public Boolean getIsExternal() {
return isExternal;
}
/**
* @param isExternal the isExternal to set
*/
public void setIsExternal(Boolean isExternal) {
this.isExternal = isExternal;
}
/**
* @return the videos
*/
@OneToMany(targetEntity = VideoCulture.class, mappedBy = "video", fetch = FetchType.LAZY)
public Set getVideos() {
return videos;
}
/**
* @param videos the videos to set
*/
public void setVideos(Set videos) {
this.videos = videos;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy