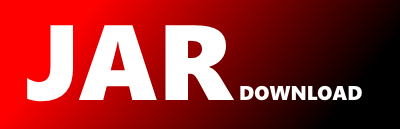
com.alogient.cameleon.sdk.content.model.AbstractCameleonModel Maven / Gradle / Ivy
The newest version!
package com.alogient.cameleon.sdk.content.model;
import java.io.Serializable;
import java.util.Date;
import java.util.HashSet;
import java.util.Set;
/**
* Base class for all the models returned by the SDK
*
* @author alord
*/
public class AbstractCameleonModel implements Serializable {
/**
* Serial version unique identifier
*/
private static final long serialVersionUID = 8543763446189089174L;
/**
* The primary for this item
*/
private Integer pageId;
/**
* The page name
*/
private String pageName;
/**
* The associated navigation node
*/
private Integer navId;
/**
* When was this page created
*/
private Date creationDate;
/**
* When was this page last update
*/
private Date lastModified;
/**
* The model culture code
*/
private String cultureCode;
private Set roles = new HashSet();
/**
* @return the pageId
*/
public Integer getPageId() {
return pageId;
}
/**
* @param pageId the pageId to set
*/
public void setPageId(Integer pageId) {
this.pageId = pageId;
}
/**
* @return the pageName
*/
public String getPageName() {
return pageName;
}
/**
* @param pageName the pageName to set
*/
public void setPageName(String pageName) {
this.pageName = pageName;
}
/**
* @return the navId
*/
public Integer getNavId() {
return navId;
}
/**
* @param navId the navId to set
*/
public void setNavId(Integer navId) {
this.navId = navId;
}
/**
* @return the creationDate
*/
public Date getCreationDate() {
return creationDate;
}
/**
* @param creationDate the creationDate to set
*/
public void setCreationDate(Date creationDate) {
this.creationDate = creationDate;
}
/**
* @return the lastModified
*/
public Date getLastModified() {
return lastModified;
}
/**
* @param lastModified the lastModified to set
*/
public void setLastModified(Date lastModified) {
this.lastModified = lastModified;
}
/**
* @return the cultureCode
*/
public String getCultureCode() {
return cultureCode;
}
/**
* @param cultureCode the cultureCode to set
*/
public void setCultureCode(String cultureCode) {
this.cultureCode = cultureCode;
}
/**
* Adds a role
*
* @param role The role to add
*/
public void addRole(String role) {
roles.add(role);
}
/**
* Checks this entry is available
*
* @param authRoles The roles to check for
* @return true if it is available
*/
public boolean isAuthorized(Set authRoles) {
if (roles == null || roles.size() == 0) {
return true;
} else {
for (String role : authRoles) {
if (roles.contains(role)) {
return true;
}
}
}
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy