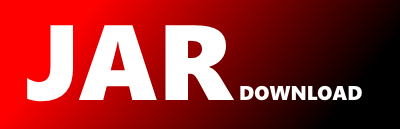
com.alogient.cameleon.sdk.content.service.PageService Maven / Gradle / Ivy
The newest version!
package com.alogient.cameleon.sdk.content.service;
import java.util.List;
import java.util.Map;
import org.springframework.transaction.annotation.Propagation;
import org.springframework.transaction.annotation.Transactional;
import com.alogient.cameleon.sdk.content.model.AbstractCameleonModel;
import com.alogient.cameleon.sdk.content.util.exception.PageNotFoundException;
import com.alogient.cameleon.sdk.content.vo.CultureVo;
/**
* User: J?r?me Mirc Date: 6-Aug-2009 Time: 10:30:54 AM This Intererface defines a list of methods to manage a page
*/
@Transactional(propagation = Propagation.REQUIRES_NEW, readOnly = true)
public interface PageService {
/**
* Verify if a page exists for a culture and a navId
*
* @param cultureVo culture of the page
* @param pageId id of the page
* @param isLiveMode true if the user is viewing the site in live mode
* @return true if the page exists, false otherwise
*/
boolean exist(CultureVo cultureVo, Integer pageId, Boolean isLiveMode);
/**
* Get the page from page id
*
* @param The model class
* @param pageId id of the page
* @param isLiveMode true if the user is viewing the site in live model
* @param isSecurityAware Should the security information be embeded into the menu
* @param modelClass The model class
* @return a page
* @throws PageNotFoundException thrown if the page is not found
*/
T getPage(Integer pageId, Boolean isLiveMode, Boolean isSecurityAware, Class modelClass) throws PageNotFoundException;
/**
* Get the page from NavId and culture
*
* @param The model class
* @param cultureVo culture of the page
* @param navId id of the navigation
* @param isLiveMode true if the user is viewing the site in live mode
* @param isSecurityAware Should the security information be embeded into the menu
* @param modelClass The model class
* @return a page
* @throws PageNotFoundException thrown if the page is not found
*/
T getPage(CultureVo cultureVo, Integer navId, Boolean isLiveMode, Boolean isSecurityAware, Class modelClass) throws PageNotFoundException;
/**
* Get a list of sub pages from a specific node
*
* @param The model class
* @param pageId id of the navigation
* @param isLiveMode true if the user is viewing the site in live mode
* @param isSecurityAware Should the security information be embeded into the menu
* @param modelClass The model class
* @return a list of sub pages
* @throws com.alogient.cameleon.sdk.content.util.exception.PageNotFoundException thrown if the page is not found
*/
List getSubPages(Integer pageId, Boolean isLiveMode, Boolean isSecurityAware, Class modelClass) throws PageNotFoundException;
/**
* Get a list of sub pages from a specific node
*
* @param pageId id of the navigation
* @param isLiveMode true if the user is viewing the site in live mode
* @param isSecurityAware Should the security information be embeded into the menu
* @param modelClasses The model classes
* @return a list of sub pages
* @throws com.alogient.cameleon.sdk.content.util.exception.PageNotFoundException thrown if the page is not found
*/
List getSubPages(Integer pageId, Boolean isLiveMode, Boolean isSecurityAware, Class extends AbstractCameleonModel>... modelClasses)
throws PageNotFoundException;
/**
* Get a list of sub pages from a specific node
*
* @param The model class
* @param cultureVo culture of the page
* @param navId id of the navigation
* @param isLiveMode true if the user is viewing the site in live mode
* @param isSecurityAware Should the security information be embeded into the menu
* @param modelClass The model class
* @return a list of sub pages
* @throws com.alogient.cameleon.sdk.content.util.exception.PageNotFoundException thrown if the page is not found
*/
List getSubPages(CultureVo cultureVo, Integer navId, Boolean isLiveMode, Boolean isSecurityAware, Class modelClass)
throws PageNotFoundException;
/**
* Get a list of sub pages from a specific node
*
* @param The model class
* @param pageId id of the navigation
* @param isLiveMode true if the user is viewing the site in live mode
* @param isSecurityAware Should the security information be embeded into the menu
* @param modelClass The model class
* @param modelClasses The model classes
* @return a list of sub pages
* @throws com.alogient.cameleon.sdk.content.util.exception.PageNotFoundException thrown if the page is not found
*/
List getSubPages(Class modelClass, Integer pageId, Boolean isLiveMode, Boolean isSecurityAware, Class extends T>... modelClasses)
throws PageNotFoundException;
/**
* Get a list of sub pages from a specific node
*
* @param cultureVo culture of the page
* @param navId id of the navigation
* @param isLiveMode true if the user is viewing the site in live mode
* @param isSecurityAware Should the security information be embeded into the menu
* @param modelClasses The model classes
* @return a list of sub pages
* @throws com.alogient.cameleon.sdk.content.util.exception.PageNotFoundException thrown if the page is not found
*/
List getSubPages(CultureVo cultureVo, Integer navId, Boolean isLiveMode, Boolean isSecurityAware, Class extends AbstractCameleonModel>... modelClasses)
throws PageNotFoundException;
/**
* Get a list of pages corresponding to a certain template
*
* @param cultureVo culture of the page
* @param isLiveMode true if the user is viewing the site in live mode
* @param isSecurityAware Should the security information be embeded into the menu
* @param modelClass The model class
* @param The target class
* @return a list of pages
*/
List getPages(CultureVo cultureVo, Boolean isLiveMode, Boolean isSecurityAware, Class modelClass);
/**
* Get a list of pages corresponding to a certain template
*
* @param cultureVo culture of the page
* @param multiAssociationNavIds the nav ids of the requested pages
* @param isLiveMode true if the user is viewing the site in live mode
* @param isSecurityAware Should the security information be embeded into the menu
* @param modelClass The model class
* @param The target class
* @return a list of pages
*/
List getPages(CultureVo cultureVo, String multiAssociationNavIds, Boolean isLiveMode, Boolean isSecurityAware, Class modelClass);
/**
* Returns the first page that can be found of a certain template
*
* @param The model class
* @param templateName the template name
* @param cultureVo the culture vo
* @param isLiveMode should we look in preview or live
* @param isSecurityAware should we append the security information
* @param modelClass the model calss
* @return the model (if a page could be found)
* @throws PageNotFoundException if no page could be found
*/
T getFirstTemplatePage(String templateName, CultureVo cultureVo, Boolean isLiveMode, Boolean isSecurityAware, Class modelClass)
throws PageNotFoundException;
/**
* Creates a new page (nav)
*
* @param parentNavId The parent under which to create the page
* @param templateName The template the page corresponds to
* @param cultureVo The culture (default) to create the page in
* @param pageName The page name
* @param author The page author (creator)
* @return The created navId
*/
Integer createPage(Integer parentNavId, String templateName, CultureVo cultureVo, String pageName, String author);
/**
* Updates a page
*
* @param navId the nav id to update
* @param cultureVo the culture to update it in
* @param values the values to set
* @param pageName the page name to set
* @param author the author of the modification
*/
void updatePage(Integer navId, CultureVo cultureVo, Map values, String pageName, String author);
/**
* Approves a page
*
* @param navId the navId of the page to approve
* @param cultureVo the culture to approve it in
* @param author the approval author
*/
void approvePage(Integer navId, CultureVo cultureVo, String author);
/**
* Changes the visibility of a page
*
* @param navId the navId to alter
* @param cultureVo the culture to alter
* @param isLive is this a change to live or preview status
* @param isVisible is this a change to visible or hidden
* @param author the change author
*/
void changePageVisibility(Integer navId, CultureVo cultureVo, boolean isLive, boolean isVisible, String author);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy