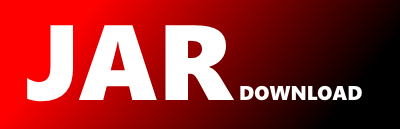
com.alogient.cameleon.sdk.content.service.impl.CultureServiceImpl Maven / Gradle / Ivy
The newest version!
package com.alogient.cameleon.sdk.content.service.impl;
import com.alogient.cameleon.sdk.common.dao.model.Culture;
import com.alogient.cameleon.sdk.content.dao.CultureDao;
import com.alogient.cameleon.sdk.content.service.CultureService;
import com.alogient.cameleon.sdk.content.vo.CultureVo;
import java.util.Collection;
import java.util.Collections;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Set;
public class CultureServiceImpl implements CultureService {
/**
* Culture data access object
*/
private CultureDao cultureDao;
/**
* @param cultureDao the cultureDao to set
*/
public void setCultureDao(CultureDao cultureDao) {
this.cultureDao = cultureDao;
}
/**
* {@inheritDoc}
*/
@Override
public Collection getCultures() {
List cultures = cultureDao.getCultures();
// If the dao returns null, don't crash
if (cultures == null) {
return Collections.emptySet();
}
Set cultureVos = new LinkedHashSet(cultures.size());
for (Culture culture : cultures) {
cultureVos.add(new CultureVo(culture));
}
return cultureVos;
}
/**
* {@inheritDoc}
*/
@Override
public CultureVo getDefaultCulture() {
// FIXME Reimplement to use as DB flag (when flag available)
Culture defaultCulture = cultureDao.getCulture(1);
if (defaultCulture != null) {
return new CultureVo(defaultCulture);
} else {
// We migth consider good practice to throw an exception in this case
return null;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy