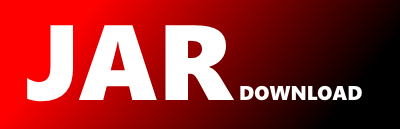
com.alogient.cameleon.sdk.content.service.impl.ImageServiceImpl Maven / Gradle / Ivy
The newest version!
package com.alogient.cameleon.sdk.content.service.impl;
import static com.alogient.cameleon.sdk.content.util.InternalCameleonConfig.CAMELEON_MEDIA_URL;
import static com.alogient.cameleon.sdk.content.util.InternalCameleonConfig.IMAGE_STORE_LOCATION;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.Date;
import org.apache.commons.io.IOUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.alogient.cameleon.sdk.content.service.ImageService;
import com.alogient.cameleon.sdk.content.util.InternalCameleonConfig;
import com.alogient.cameleon.sdk.content.vo.media.ImageEntry;
import com.springsource.insight.annotation.InsightOperation;
/**
* Service used to manage images
*/
public class ImageServiceImpl implements ImageService {
public static final Logger LOGGER = LoggerFactory.getLogger(ImageServiceImpl.class);
private static final String SEPARATOR = "_";
/**
* Default constructor
*/
public ImageServiceImpl() {
}
@Override
@InsightOperation
public byte[] getImage(ImageEntry imageEntry) throws IOException {
byte[] imageData = null;
File localeImageFile = getLocaleImageFile(imageEntry);
//
if (isImageAvailableOnLocal(imageEntry)) {
if (LOGGER.isDebugEnabled()) {
LOGGER.debug("File " + localeImageFile.getAbsolutePath() + " is loaded from the local.");
}
imageData = getLocalImage(imageEntry);
}
// Not available on local. Get it from the remote
if (imageData == null) {
imageData = getRemoteImage(imageEntry);
if (imageData != null) {
// Open the file, then read it in.
OutputStream outputStream = null;
try {
outputStream = new FileOutputStream(localeImageFile);
IOUtils.write(imageData, outputStream);
return imageData;
} finally {
IOUtils.closeQuietly(outputStream);
}
}
if (LOGGER.isDebugEnabled()) {
LOGGER.debug("File " + localeImageFile.getAbsolutePath() + " is loaded from the remote.");
}
}
return imageData;
}
@Override
public boolean isImageAvailableOnLocal(ImageEntry imageEntry) {
return getLocaleImageFile(imageEntry).exists();
}
@Override
public Date getLastModifyTime(ImageEntry imageEntry) {
try {
File f = getLocaleImageFile(imageEntry);
URL url = getRemoteImageURL(imageEntry);
HttpURLConnection uc = (HttpURLConnection) url.openConnection();
try {
uc.setIfModifiedSince(f.lastModified());
} catch (IllegalStateException e) {
// already connected
}
// The
if (uc.getResponseCode() == 304) {
return new Date(f.lastModified());
}
Date fileDate = new Date(f.lastModified());
Date urlDate = new Date(uc.getLastModified());
if (urlDate.after(fileDate)) {
boolean isDeleted = f.delete();
if (!isDeleted && LOGGER.isErrorEnabled()) {
LOGGER.error("Unable to delete the image :" + f.getAbsolutePath());
}
return new Date(uc.getLastModified());
}
return new Date(f.lastModified());
} catch (IOException e) {
return null;
}
}
@Override
public void save(ImageEntry imageEntry, InputStream imageStream) throws IOException {
// Read in the image data.
byte[] imageData = IOUtils.toByteArray(imageStream);
// Create a unique name for the file in the image directory and
// write the image data into it.
File newFile = getLocaleImageFile(imageEntry);
OutputStream outStream = new FileOutputStream(newFile);
try {
IOUtils.write(imageData, outStream);
} catch (IOException e) {
IOUtils.closeQuietly(outStream);
}
}
/**
* Create a unique name for the file in the image directory and write the image data into it.
*
* @param imageEntry image entry
* @return the created image
*/
private File getLocaleImageFile(ImageEntry imageEntry) {
String tmpFolder = System.getProperty("java.io.tmpdir");
// create the folder if the temp file does not exist.
File tmpImgFolder = new File(InternalCameleonConfig.getString(IMAGE_STORE_LOCATION, tmpFolder));
if (!tmpImgFolder.exists()) {
if (tmpImgFolder.mkdirs()) {
if (LOGGER.isWarnEnabled()) {
LOGGER.warn("Images folder " + InternalCameleonConfig.getString(IMAGE_STORE_LOCATION, tmpFolder) + " has been created.");
}
} else {
if (LOGGER.isErrorEnabled()) {
LOGGER.error("Images folder " + InternalCameleonConfig.getString(IMAGE_STORE_LOCATION, tmpFolder) + " has not been created.");
}
}
}
return new File(InternalCameleonConfig.getString(IMAGE_STORE_LOCATION, tmpFolder), getUniqueImageName(imageEntry));
}
/**
* @param imageEntry the image entry
* @return remote image
* @throws java.net.MalformedURLException if the URL is malformed
*/
private URL getRemoteImageURL(ImageEntry imageEntry) throws MalformedURLException {
return new URL(InternalCameleonConfig.getString(CAMELEON_MEDIA_URL, "/") + getUniqueImageName(imageEntry));
}
/**
* Get unique file name
*
* @param imageEntry the image entry
* @return the unique image name
*/
private String getUniqueImageName(ImageEntry imageEntry) {
StringBuffer sb = new StringBuffer();
if (!imageEntry.isLive()) {
sb.append("Preview");
sb.append(SEPARATOR);
}
sb.append(imageEntry.getNavId());
sb.append(SEPARATOR);
sb.append(imageEntry.getImageElementPartId());
sb.append(SEPARATOR);
sb.append(imageEntry.getCultureCode());
sb.append(SEPARATOR);
sb.append(imageEntry.getImageType().getType());
sb.append(SEPARATOR);
sb.append(imageEntry.getFileName());
return sb.toString();
}
/**
* Retrieve the image from the Cameleon Media
*
* @param imageEntry the image entry
* @return the image as byte array
*/
private byte[] getRemoteImage(ImageEntry imageEntry) {
try {
URL url = getRemoteImageURL(imageEntry);
HttpURLConnection uc = (HttpURLConnection) url.openConnection();
try {
return IOUtils.toByteArray(uc.getInputStream());
} catch (Exception e) {
LOGGER.error("Failed to load remote image. \n\r" + " CAMELEON_MEDIA_URL = " + url.toString() + "\n\r" + "Image Entry = " + imageEntry.toString(), e);
} finally {
IOUtils.closeQuietly(uc.getInputStream());
uc.disconnect();
}
} catch (IOException e) {
LOGGER.error("Failed to load remote image", e);
}
return null;
}
/**
* Retrieve image from local
*
* @param imageEntry the image entry
* @return the image as byte array
* @throws IOException if the image could not be read from the local
*/
@SuppressWarnings({ "ResultOfMethodCallIgnored" })
private byte[] getLocalImage(ImageEntry imageEntry) throws IOException {
return IOUtils.toByteArray(new FileInputStream(getLocaleImageFile(imageEntry)));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy