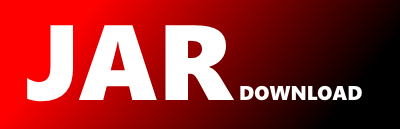
com.alogient.cameleon.sdk.content.util.InternalCameleonConfig Maven / Gradle / Ivy
The newest version!
package com.alogient.cameleon.sdk.content.util;
import java.io.File;
import java.util.Enumeration;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
import javax.servlet.ServletContext;
import org.apache.commons.configuration.PropertiesConfiguration;
import org.apache.commons.lang.StringUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* This config cache is used to manage the static configs.
*/
public class InternalCameleonConfig {
private static final Logger LOGGER = LoggerFactory.getLogger(InternalCameleonConfig.class);
public static final String CONFIGURATION = "configuration";
public static final String MEDIA_EXTENSION = "Media";
public static final String MEDIA_FREE_EXTENSION = "MediaFree";
public static final String CAMELEON_CMS_URL = "CAMELEON_CMS_URL";
public static final String CAMELEON_CMS_FORM_MEDIA_PATH = "CAMELEON_CMS_FORM_MEDIA_PATH";
public static final String CAMELEON_MEDIA_URL = "CAMELEON_MEDIA_URL";
public static final String CAMELEON_CMS_MEDIA_PATH = "CAMELEON_CMS_MEDIA_PATH";
public static final String CAMELEON_MEDIA_FREE_URL = "CAMELEON_MEDIA_FREE_URL";
public static final String IMAGE_STORE_LOCATION = "IMAGE_STORE_LOCATION";
public static final String LIVE_REFRESH_TIME = "LIVE_REFRESH_TIME";
public static final String PREVIEW_REFRESH_TIME = "PREVIEW_REFRESH_TIME";
public static final String REFRESH_TIME_ENABLED = "REFRESH_TIME_ENABLED";
public static final String SMTP_SERVER = "SMTP_SERVER";
public static final String HTTP_SERVER = "HTTP_SERVER";
public static final String HTTPS_SERVER = "HTTPS_SERVER";
public static final String PREVIEW_HTTP_SERVER = "PREVIEW_HTTP_SERVER";
public static final String PREVIEW_HTTPS_SERVER = "PREVIEW_HTTPS_SERVER";
public static final String MENU_CACHE_REFRESH_TIME = "cameleon.menu_cache.refresh_time";
public static final String INDEX_REFRESH_TIME = "cameleon.index.refresh_time";
public static final String URL_MENU_GENERATOR_CLASS = "cameleon.url.generator_class";
private static Map configs = new HashMap();
private static InternalCameleonConfig instance = new InternalCameleonConfig();
private static boolean isDevMode = false;
/**
* @return the unique instance of this class
*/
public static InternalCameleonConfig getInstance() {
return instance;
}
/**
* Get a config.
*
* @param key a key of the config
* @param defaultValue default value if the key doesn't exist
* @return return value
*/
public static String getString(String key, String defaultValue) {
if (configs.get(key) == null)
return defaultValue;
return (String) configs.get(key);
}
/**
* Get a config.
*
* @param key a key of the config
* @param defaultValue default value
* @return return value
*/
public static Integer getInteger(String key, Integer defaultValue) {
if (configs.get(key) == null) {
return defaultValue;
}
if (configs.get(key) instanceof Integer) {
return (Integer) configs.get(key);
} else if (configs.get(key) instanceof String) {
return new Integer((String) configs.get(key));
} else {
return defaultValue;
}
}
/**
* Get a config.
*
* @param key a key of the config
* @param defaultValue default value
* @return return value
*/
public static Boolean getBoolean(String key, Boolean defaultValue) {
if (configs.get(key) == null) {
return defaultValue;
}
if (configs.get(key) instanceof Boolean) {
return (Boolean) configs.get(key);
} else if (configs.get(key) instanceof String) {
return new Boolean((String) configs.get(key));
} else {
return defaultValue;
}
}
/**
* Set a config
*
* @param key the key of the config
* @param value the value of the config
*/
public static void addConfig(String key, Object value) {
configs.put(key, value);
}
/**
* @return the isDevMode
*/
public static boolean isDevMode() {
return isDevMode;
}
/**
* @param isDevMode the isDevMode to set
*/
public static void setDevMode(boolean isDevMode) {
InternalCameleonConfig.isDevMode = isDevMode;
}
/**
* Set the cameleon media URL
*
* @param cameleonCmsUrlParam the cameleon based URL
*/
public void setCameleonCmsUrl(String cameleonCmsUrlParam) {
String cameleonCmsUrl = cameleonCmsUrlParam;
if (!cameleonCmsUrl.endsWith("/")) {
cameleonCmsUrl += "/";
}
String cameleonMediaUrl = cameleonCmsUrl + MEDIA_EXTENSION + "/";
String cameleonMediaFreeUrl = cameleonCmsUrl + MEDIA_FREE_EXTENSION + "/";
InternalCameleonConfig.addConfig(CAMELEON_MEDIA_URL, cameleonMediaUrl);
InternalCameleonConfig.addConfig(CAMELEON_MEDIA_FREE_URL, cameleonMediaFreeUrl);
}
/**
* Set the cameleon form media path
*
* @param cameleonCmsFormMediaPath the cameleon form media path
*/
public void setCameleonCmsFormMediaPath(String cameleonCmsFormMediaPath) {
if (!cameleonCmsFormMediaPath.endsWith(File.separator)) {
InternalCameleonConfig.addConfig(CAMELEON_CMS_FORM_MEDIA_PATH, cameleonCmsFormMediaPath + File.separator);
} else {
InternalCameleonConfig.addConfig(CAMELEON_CMS_FORM_MEDIA_PATH, cameleonCmsFormMediaPath);
}
}
/**
* Set the cameleon media path
*
* @param cameleonCmsMediaPath the cameleon media path
*/
public void setCameleonCmsMediaPath(String cameleonCmsMediaPath) {
if (!cameleonCmsMediaPath.endsWith(File.separator)) {
InternalCameleonConfig.addConfig(CAMELEON_CMS_MEDIA_PATH, cameleonCmsMediaPath + File.separator);
} else {
InternalCameleonConfig.addConfig(CAMELEON_CMS_MEDIA_PATH, cameleonCmsMediaPath);
}
}
/**
* Set the image store location
*
* @param imageStoreLocation set the image store location
*/
public void setImageStoreLocation(String imageStoreLocation) {
InternalCameleonConfig.addConfig(IMAGE_STORE_LOCATION, imageStoreLocation);
}
/**
* Set the time to refresh the content from the CMS in live mode
*
* @param liveRefreshTime the time to refresh the content
*/
public void setLiveRefreshTime(String liveRefreshTime) {
InternalCameleonConfig.addConfig(LIVE_REFRESH_TIME, Integer.parseInt(liveRefreshTime));
}
/**
* Set the time to refresh the content from the CMS in preview mode
*
* @param previewRefreshTime the time to refresh the content
*/
public void setPreviewRefreshTime(String previewRefreshTime) {
InternalCameleonConfig.addConfig(PREVIEW_REFRESH_TIME, Integer.parseInt(previewRefreshTime));
}
/**
* Set the flag to indicate if the refresh content is activate
*
* @param enable true if the refresh is enabled
*/
public void setRefreshTimeEnabled(String enable) {
InternalCameleonConfig.addConfig(REFRESH_TIME_ENABLED, Boolean.parseBoolean(enable));
}
/**
* @param smtpServer Set the smtp server
*/
public void setSmtpServer(String smtpServer) {
InternalCameleonConfig.addConfig(SMTP_SERVER, smtpServer);
}
/**
* Initialize the cache
*
* @param servletContext the servlet context
*/
public void init(ServletContext servletContext, PropertiesConfiguration configuration) {
if (StringUtils.isNotBlank(servletContext.getInitParameter(CONFIGURATION)) && "development".equals(servletContext.getInitParameter(CONFIGURATION))) {
InternalCameleonConfig.setDevMode(true);
}
try {
setCameleonCmsUrl(servletContext.getInitParameter(CAMELEON_CMS_URL));
} catch (Exception e) {
if (LOGGER.isErrorEnabled()) {
LOGGER.error("No CAMELEON_CMS_URL parameter has been put in the web.xml.");
}
}
try {
setCameleonCmsFormMediaPath(servletContext.getInitParameter(CAMELEON_CMS_FORM_MEDIA_PATH));
} catch (Exception e) {
if (LOGGER.isWarnEnabled()) {
LOGGER.warn("No REFRESH_TIME_ENABLED parameter has been put in the web.xml");
}
}
try {
setCameleonCmsMediaPath(servletContext.getInitParameter(CAMELEON_CMS_MEDIA_PATH));
} catch (Exception e) {
if (LOGGER.isWarnEnabled()) {
LOGGER.warn("No REFRESH_TIME_ENABLED parameter has been put in the web.xml");
}
}
try {
setImageStoreLocation(servletContext.getInitParameter(IMAGE_STORE_LOCATION));
} catch (Exception e) {
if (LOGGER.isErrorEnabled()) {
LOGGER.error("No IMAGE_STORE_LOCATION parameter has been put in the web.xml.");
}
}
try {
setLiveRefreshTime(servletContext.getInitParameter(LIVE_REFRESH_TIME));
} catch (Exception e) {
if (LOGGER.isWarnEnabled()) {
LOGGER.warn("No LIVE_REFRESH_TIME parameter has been put in the web.xml.");
}
}
try {
setPreviewRefreshTime(servletContext.getInitParameter(PREVIEW_REFRESH_TIME));
} catch (Exception e) {
if (LOGGER.isWarnEnabled()) {
LOGGER.warn("No PREVIEW_REFRESH_TIME parameter has been put in the web.xml.");
}
}
try {
setRefreshTimeEnabled(servletContext.getInitParameter(REFRESH_TIME_ENABLED));
} catch (Exception e) {
if (LOGGER.isWarnEnabled()) {
LOGGER.warn("No REFRESH_TIME_ENABLED parameter has been put in the web.xml");
}
}
try {
setSmtpServer(servletContext.getInitParameter(SMTP_SERVER));
} catch (Exception e) {
if (LOGGER.isWarnEnabled()) {
LOGGER.warn("No REFRESH_TIME_ENABLED parameter has been put in the web.xml");
}
}
@SuppressWarnings("unchecked")
Enumeration initParamEnum = servletContext.getInitParameterNames();
while (initParamEnum.hasMoreElements()) {
String key = initParamEnum.nextElement();
if (!InternalCameleonConfig.configs.containsKey(key)) {
InternalCameleonConfig.addConfig(key, servletContext.getInitParameter(key));
}
}
if (configuration != null) {
@SuppressWarnings("unchecked")
Iterator configurationKeys = configuration.getKeys();
while (configurationKeys.hasNext()) {
String key = configurationKeys.next();
if (!InternalCameleonConfig.configs.containsKey(key)) {
InternalCameleonConfig.addConfig(key, configuration.getString(key));
}
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy