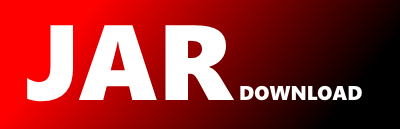
com.alogient.cameleon.sdk.content.validator.CmsConstraintViolationToHtmlFunction Maven / Gradle / Ivy
package com.alogient.cameleon.sdk.content.validator;
import static com.alogient.cameleon.sdk.content.validator.CmsConstraintViolationUtil.getCmsTemplateName;
import static com.alogient.cameleon.sdk.content.validator.CmsConstraintViolationUtil.getLeafModelBean;
import static com.alogient.cameleon.sdk.content.validator.CmsConstraintViolationUtil.getModelAnnotationNameEnd;
import static com.alogient.cameleon.sdk.content.validator.CmsConstraintViolationUtil.getRootModelBean;
import static com.alogient.cameleon.sdk.content.validator.ConstraintViolationUtil.getConstraintAnnotationName;
import static com.alogient.cameleon.sdk.content.validator.ConstraintViolationUtil.getLeafProperty;
import static com.alogient.cameleon.sdk.content.validator.ConstraintViolationUtil.getPropertyPath;
import static com.alogient.cameleon.sdk.content.validator.ConstraintViolationUtil.getRootBeanName;
import static com.alogient.cameleon.sdk.content.validator.ConstraintViolationUtil.isRootAndLeafBeanTheSame;
import java.lang.annotation.Annotation;
import java.lang.reflect.Field;
import java.util.Arrays;
import javax.validation.ConstraintViolation;
import com.alogient.cameleon.sdk.content.model.AbstractCameleonModel;
import com.alogient.cameleon.sdk.content.ui.injection.CameleonModelAllElements;
import com.alogient.cameleon.sdk.content.ui.injection.CameleonModelClosestUpElement;
import com.alogient.cameleon.sdk.content.ui.injection.CameleonModelElement;
import com.alogient.cameleon.sdk.content.ui.injection.CameleonModelFirstElement;
import com.alogient.cameleon.sdk.content.ui.injection.CameleonModelImage;
import com.alogient.cameleon.sdk.content.ui.injection.CameleonModelLinkedElement;
import com.alogient.cameleon.sdk.content.ui.injection.CameleonModelLinkedElements;
import com.alogient.cameleon.sdk.content.ui.injection.CameleonModelParentElement;
import com.alogient.cameleon.sdk.content.ui.injection.CameleonModelReverseLinkedElements;
import com.alogient.cameleon.sdk.content.ui.injection.CameleonModelSubElements;
import com.google.common.base.Objects;
import com.google.common.base.Preconditions;
import com.google.common.base.Predicate;
import com.google.common.base.Strings;
import com.google.common.collect.Iterables;
//TODO l10n properly
//TODO consider printing all annotations of the field
//TODO consider printing violated annotation's arguments too (didn't like it)
//TODO properly walk through Model hierarchies
//TODO logging for prod
public class CmsConstraintViolationToHtmlFunction extends CmsConstraintViolationToInfoFunction {
private static final long serialVersionUID = -3251571288465786386L;
public CmsConstraintViolationToHtmlFunction(final boolean reportViolationsForProgrammers) {
super(reportViolationsForProgrammers);
}
@Override
public String apply(/* @javax.annotation.Nullable */final ConstraintViolation> input) {
return new StringBuilder().append("Constraint violation:").append(violation(input)).append(cmsElement(input)).append(cmsTemplate(input)).append(beanProperty(input))
.append(constraintAnnotation(input)).append(actualValue(input)).append("
").toString();
}
private static String violation(final ConstraintViolation> input) {
return html("Violation: ", ConstraintViolationUtil.getViolationMessage(input));
}
private static String cmsElement(final ConstraintViolation> input) {
return html("CMS Element: ", cmsNode(getRootModelBean(input)) + (isRootAndLeafBeanTheSame(input) ? "" : "..." + cmsNode(getLeafModelBean(input)))); // todo:
// return
// the
// complete
// path
// from
// root
// to
// leaf,
// using
// a
// tree
// traversal.
}
private static String cmsNode(final AbstractCameleonModel node) {
return "[" + node.getNavId() + ':' + node.getPageName() + ']';
}
private static String cmsTemplate(final ConstraintViolation> input) {
final String template = getCmsTemplateName(input);
try {
final String element = cmsAnnotation(getLeafProperty(input));
return html("CMS Template: ", template + '.' + element);
} catch (NoSuchFieldException e) {
return html("CMS Template: ", template + ".[EXCEPTION: " + e.getClass().getSimpleName() + ": " + e.getMessage() + ']');
}
}
private static String cmsAnnotation(final Field field) {
final Annotation camAnno = Iterables.find(Arrays.asList(field.getAnnotations()), new Predicate() {
@Override
public boolean apply(/* @javax.annotation.Nullable */final Annotation input) {
return input.annotationType().getSimpleName().startsWith(CmsConstraintViolationUtil.START_OF_ANNOTATION_NAME);
}
});
if (camAnno instanceof CameleonModelElement) {
return ((CameleonModelElement) camAnno).elementName();
} else if (camAnno instanceof CameleonModelAllElements) {
return asCollection(camAnno, getCmsTemplateName(((CameleonModelAllElements) camAnno).templateClass()));
} else if (camAnno instanceof CameleonModelClosestUpElement) {
return asCollection(camAnno, getCmsTemplateName(((CameleonModelClosestUpElement) camAnno).templateClass()));
} else if (camAnno instanceof CameleonModelFirstElement) {
return asCollection(camAnno, getCmsTemplateName(((CameleonModelFirstElement) camAnno).templateClass()));
} else if (camAnno instanceof CameleonModelImage) {
return ((CameleonModelImage) camAnno).elementName();
} else if (camAnno instanceof CameleonModelLinkedElement) {
final CameleonModelLinkedElement anno = (CameleonModelLinkedElement) camAnno;
return anno.elementName() + asCollection(anno, getCmsTemplateName(anno.templateClass()));
} else if (camAnno instanceof CameleonModelLinkedElements) {
final CameleonModelLinkedElements anno = (CameleonModelLinkedElements) camAnno;
return anno.elementName() + asCollection(anno, getCmsTemplateName(anno.templateClass()));
} else if (camAnno instanceof CameleonModelParentElement) {
return asCollection(camAnno, getCmsTemplateName(((CameleonModelParentElement) camAnno).templateClass()));
} else if (camAnno instanceof CameleonModelReverseLinkedElements) {
final CameleonModelReverseLinkedElements anno = (CameleonModelReverseLinkedElements) camAnno;
return anno.elementName() + asCollection(anno, getCmsTemplateName(anno.templateClass()));
} else if (camAnno instanceof CameleonModelSubElements) {
final CameleonModelSubElements anno = (CameleonModelSubElements) camAnno;
try {
return anno.elementName() + asCollection(anno, getCmsTemplateName(anno.templateClass()[0]));// TODO
// investigate
// why it's
// an array
// and what
// to do
// with the
// other
// elements
} catch (ArrayIndexOutOfBoundsException e) {
return anno.elementName();
}
}
return "";
}
private static String asCollection(final Annotation typeOfAssociation, final String collectionTemplateName) {
return '[' + getModelAnnotationNameEnd(typeOfAssociation) + ": " + collectionTemplateName + ']';
}
private static String actualValue(final ConstraintViolation> input) {
return html("Actual value: ", input.getInvalidValue() == null ? "" : input.getInvalidValue().toString());
}
private String constraintAnnotation(final ConstraintViolation> input) {
return html(reportForProgrammers, "Annotation: ", '@' + getConstraintAnnotationName(input));
}
private String beanProperty(final ConstraintViolation> input) {
return html(reportForProgrammers, "Bean Property: ", getRootBeanName(input) + '.' + getPropertyPath(input));
}
private static String html(final boolean falseIfYouWantAnEmptyString, final String title, final String s) {
return falseIfYouWantAnEmptyString ? html(title, s) : "";
}
private static String html(final String title, final String s) {
final String strongTitle = tagIfNB("strong", title);
return tagIfNB("li", strongTitle + s);
}
private static String tagIfNB(final String tagName, final String s) {
Preconditions.checkNotNull(tagName);
return Strings.isNullOrEmpty(s) ? "" : new StringBuilder().append('<').append(tagName).append('>').append(s).append("").append(tagName).append('>').toString();
}
private static String surroundIfNB(final char start, final String s, final char end) {
Preconditions.checkNotNull(start);
Preconditions.checkNotNull(end);
return start + s + end;
}
@Override
public String toString() {
return Objects.toStringHelper(this).add("reportForProgrammers", reportForProgrammers).toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy