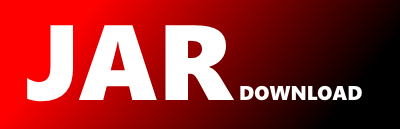
com.alogient.cameleon.sdk.form.util.SubmissionBeanToModelConverter Maven / Gradle / Ivy
package com.alogient.cameleon.sdk.form.util;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.Serializable;
import java.lang.reflect.InvocationTargetException;
import java.util.Collection;
import java.util.Date;
import java.util.HashSet;
import org.apache.commons.beanutils.PropertyUtils;
import org.apache.commons.io.IOUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.alogient.cameleon.sdk.common.dao.model.Culture;
import com.alogient.cameleon.sdk.content.util.InternalCameleonConfig;
import com.alogient.cameleon.sdk.form.dao.model.Form;
import com.alogient.cameleon.sdk.form.dao.model.FormElement;
import com.alogient.cameleon.sdk.form.dao.model.formelement.CheckboxFEModel;
import com.alogient.cameleon.sdk.form.dao.model.formelement.DateFEModel;
import com.alogient.cameleon.sdk.form.dao.model.formelement.DateRangeFEModel;
import com.alogient.cameleon.sdk.form.dao.model.formelement.NumberFEModel;
import com.alogient.cameleon.sdk.form.dao.model.formelement.RadioFEModel;
import com.alogient.cameleon.sdk.form.dao.model.formelement.RatingFEModel;
import com.alogient.cameleon.sdk.form.dao.model.formelement.SelectFEModel;
import com.alogient.cameleon.sdk.form.dao.model.formelement.TextAreaFEModel;
import com.alogient.cameleon.sdk.form.dao.model.formelement.TextBlockFEModel;
import com.alogient.cameleon.sdk.form.dao.model.formelement.TextFEModel;
import com.alogient.cameleon.sdk.form.dao.model.formelement.UploadFEModel;
import com.alogient.cameleon.sdk.form.dao.model.submission.Submission;
import com.alogient.cameleon.sdk.form.dao.model.submission.SubmissionValue;
import com.alogient.cameleon.sdk.form.dao.model.submission.SubmissionValueItem;
import com.alogient.cameleon.sdk.form.vo.formelement.AbstractElementVo;
import com.alogient.cameleon.sdk.form.vo.formelement.NameValueElementVo;
import com.alogient.cameleon.sdk.form.vo.formelement.SelectGroupElementsVo;
import com.alogient.cameleon.sdk.form.vo.submission.BasicCameleonFormSubmission;
public final class SubmissionBeanToModelConverter {
/**
* The logger for this class
*/
private final static Logger LOG = LoggerFactory.getLogger(SubmissionBeanToModelConverter.class);
/**
* Utility classes should not have ctor. This private ctor is used to mask the default public ctor.
*/
private SubmissionBeanToModelConverter() {
}
/**
* Converts an abitrary bean into a submission model
*
* @param bean The bean to convert
* @param form The form to map the convertion to
* @param culture The culture to mark the submission has
* @return The converted submission
*/
public static Submission convertBeanToModel(BasicCameleonFormSubmission bean, Form form, Culture culture) {
Submission submission = new Submission();
submission.setForm(form);
submission.setCulture(culture);
submission.setSubmissionDate(bean.getSubmissionDate());
submission.setSourceIp(bean.getSourceIP());
submission.setUserAgent(bean.getUserAgent());
submission.setReferenceUrl(bean.getReferenceUrl());
submission.setPageUrl(bean.getPageUrl());
submission.setEmail(bean.getEmail());
submission.setUserName(bean.getUserName());
// Convert the submission values
if (bean.getValues() != null && !bean.getValues().isEmpty()) {
submission.setValues(new HashSet());
for (AbstractElementVo extends Serializable> aev : bean.getValues()) {
FormElement formElement = getFormElement(aev, form);
if (formElement != null) {
SubmissionValue value;
if (aev instanceof SelectGroupElementsVo) {
if (((SelectGroupElementsVo) aev).isMultipleSelect() != null && ((SelectGroupElementsVo) aev).isMultipleSelect()) {
value = convertElement(formElement, aev.getValue());
} else {
value = convertElement(formElement, ((SelectGroupElementsVo) aev).getSingleValue());
}
} else {
value = convertElement(formElement, aev.getValue());
}
value.setSubmission(submission);
submission.getValues().add(value);
}
}
}
return submission;
}
private static SubmissionValue convertElement(FormElement formElement, Object value) {
SubmissionValue submissionValue = new SubmissionValue();
submissionValue.setFormElement(formElement);
if (value == null) {
return submissionValue;
}
// Test for coherence
if (CheckboxFEModel.class == formElement.getClass()) {
convertMultipleValueElement(submissionValue, value);
} else if (DateFEModel.class == formElement.getClass()) {
submissionValue.setValue(convertValue(value));
} else if (DateRangeFEModel.class == formElement.getClass()) {
// TODO: to be implemented - DateRange
} else if (NumberFEModel.class == formElement.getClass()) {
submissionValue.setValue(convertValue(value));
} else if (RadioFEModel.class == formElement.getClass()) {
convertMultipleValueElement(submissionValue, value);
} else if (RatingFEModel.class == formElement.getClass()) {
submissionValue.setValue(convertValue(value));
} else if (SelectFEModel.class == formElement.getClass()) {
convertMultipleValueElement(submissionValue, value);
} else if (TextFEModel.class == formElement.getClass()) {
submissionValue.setValue(convertValue(value));
} else if (TextAreaFEModel.class == formElement.getClass()) {
submissionValue.setValue(convertValue(value));
} else if (TextBlockFEModel.class == formElement.getClass()) {
submissionValue.setValue(convertValue(value));
} else if (UploadFEModel.class == formElement.getClass()) {
try {
String targetFileName = System.currentTimeMillis() + "_" + (String) PropertyUtils.getProperty(value, "clientFileName");
submissionValue.setValue(targetFileName);
InputStream data = (InputStream) PropertyUtils.getProperty(value, "inputStream");
// Copy file to correct directory
String path = InternalCameleonConfig.getString(InternalCameleonConfig.CAMELEON_CMS_FORM_MEDIA_PATH, "/tmp");
File targetFile = new File(path + targetFileName);
targetFile.createNewFile();
FileOutputStream fos = new FileOutputStream(targetFile);
IOUtils.copy(data, fos);
fos.close();
} catch (IllegalAccessException e) {
LOG.error(e.getMessage(), e);
} catch (InvocationTargetException e) {
LOG.error(e.getMessage(), e);
} catch (NoSuchMethodException e) {
LOG.error(e.getMessage(), e);
} catch (IOException e) {
LOG.error(e.getMessage(), e);
}
}
return submissionValue;
}
@SuppressWarnings("unchecked")
private static void convertMultipleValueElement(SubmissionValue submissionValue, Object value) {
submissionValue.setItems(new HashSet());
StringBuilder refValue = new StringBuilder();
if (value.getClass().isArray()) {
for (Object subValue : (Object[]) value) {
String actValue = convertValue(subValue);
refValue.append(actValue);
refValue.append(" | ");
SubmissionValueItem item = new SubmissionValueItem();
item.setSubmissionValue(submissionValue);
if (subValue instanceof NameValueElementVo) {
item.setValue(((NameValueElementVo) subValue).getValue());
} else {
item.setValue(actValue);
}
submissionValue.getItems().add(item);
}
} else if (value instanceof Collection) {
for (Object subValue : (Collection) value) {
String actValue = convertValue(subValue);
refValue.append(actValue);
refValue.append(" | ");
SubmissionValueItem item = new SubmissionValueItem();
item.setSubmissionValue(submissionValue);
if (subValue instanceof NameValueElementVo) {
item.setValue(((NameValueElementVo) subValue).getValue());
} else {
item.setValue(actValue);
}
submissionValue.getItems().add(item);
}
} else {
String actValue = convertValue(value);
refValue.append(actValue);
refValue.append(" | ");
SubmissionValueItem item = new SubmissionValueItem();
item.setSubmissionValue(submissionValue);
if (value instanceof NameValueElementVo) {
item.setValue(((NameValueElementVo) value).getValue());
} else {
item.setValue(actValue);
}
submissionValue.getItems().add(item);
}
// FIXME Set submissionValue value
submissionValue.setValue(refValue.toString());
}
private static String convertValue(Object value) {
if (value == null) {
return null;
} else if (value instanceof Date) {
// Convert the java date to C# ticks
return Long.toString((((Date) value).getTime() + 621355968000000000L) * 10000L);
} else if (value instanceof NameValueElementVo) {
NameValueElementVo nveVo = (NameValueElementVo) value;
// FIXME Handle other case differently ?
if (nveVo.getValue() == null) {
return nveVo.getName();
}
return nveVo.getName();
} else {
// As a last resort, trust the JVM to do ... something with the
// value
return value.toString();
}
}
private static FormElement getFormElement(AbstractElementVo extends Serializable> key, Form form) {
// Do we have the required data to find the element
Integer formElementId = key.getId();
if (formElementId == null || form == null || form.getFormElements() == null || form.getFormElements().isEmpty()) {
return null;
}
// Try to find the element
for (FormElement formElement : form.getFormElements()) {
if (formElementId.equals(formElement.getFormElementId())) {
return formElement;
}
}
/**
* FIXME Here we might consider throwing an exception since there is a clear indication that the bean doesn't
* math the database.
*/
// The element was not fou
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy