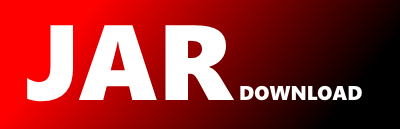
com.amazonaws.services.sqs.scala.AmazonSQSClient.scala Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2015 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.sqs.scala
import com.amazonaws.services.sqs.AmazonSQSAsync
import com.amazonaws.services.sqs.AmazonSQSAsyncClient
import com.amazonaws.services.sqs.model._
/** A Scala-friendly wrapper around an {@code AmazonSQSAsync}. */
class AmazonSQSClient(private val client: AmazonSQSAsync) {
/** Creates a client for the given region.
*
* @param region the region
*/
def this(region: com.amazonaws.regions.Region) =
this({
val client = new AmazonSQSAsyncClient()
client.setRegion(region)
client
})
/** Creates a client for the given region.
*
* @param region the region
*/
def this(region: com.amazonaws.regions.Regions) =
this(com.amazonaws.regions.Region.getRegion(region))
/** Creates a client for the given region.
*
* @param region the region
*/
def this(region: String) =
this(com.amazonaws.regions.RegionUtils.getRegion(region))
/** Invokes the {@code removePermissionAsync} method of the underlying
* client and adapts the result to a Scala {@code Future}.
*
* @param request the request to send
* @return the future result
*/
def removePermission(request: RemovePermissionRequest):
scala.concurrent.Future[scala.runtime.BoxedUnit] = {
val opts = request.getRequestClientOptions()
if (opts.getClientMarker(com.amazonaws.RequestClientOptions.Marker.USER_AGENT) == null) {
opts.appendUserAgent("aws-scala-sdk")
}
val promise = scala.concurrent.Promise[scala.runtime.BoxedUnit]
client.removePermissionAsync(request, new com.amazonaws.handlers.AsyncHandler[RemovePermissionRequest, Void]() {
override def onSuccess(request: RemovePermissionRequest, result: Void) = promise.success(scala.runtime.BoxedUnit.UNIT)
override def onError(exception: Exception) = promise.failure(exception)
})
promise.future
}
/** Invokes the {@code setQueueAttributesAsync} method of the underlying
* client and adapts the result to a Scala {@code Future}.
*
* @param request the request to send
* @return the future result
*/
def setQueueAttributes(request: SetQueueAttributesRequest):
scala.concurrent.Future[scala.runtime.BoxedUnit] = {
val opts = request.getRequestClientOptions()
if (opts.getClientMarker(com.amazonaws.RequestClientOptions.Marker.USER_AGENT) == null) {
opts.appendUserAgent("aws-scala-sdk")
}
val promise = scala.concurrent.Promise[scala.runtime.BoxedUnit]
client.setQueueAttributesAsync(request, new com.amazonaws.handlers.AsyncHandler[SetQueueAttributesRequest, Void]() {
override def onSuccess(request: SetQueueAttributesRequest, result: Void) = promise.success(scala.runtime.BoxedUnit.UNIT)
override def onError(exception: Exception) = promise.failure(exception)
})
promise.future
}
/** Invokes the {@code getQueueUrlAsync} method of the underlying
* client and adapts the result to a Scala {@code Future}.
*
* @param request the request to send
* @return the future result
*/
def getQueueUrl(request: GetQueueUrlRequest):
scala.concurrent.Future[GetQueueUrlResult] = {
val opts = request.getRequestClientOptions()
if (opts.getClientMarker(com.amazonaws.RequestClientOptions.Marker.USER_AGENT) == null) {
opts.appendUserAgent("aws-scala-sdk")
}
val promise = scala.concurrent.Promise[GetQueueUrlResult]
client.getQueueUrlAsync(request, new com.amazonaws.handlers.AsyncHandler[GetQueueUrlRequest, GetQueueUrlResult]() {
override def onSuccess(request: GetQueueUrlRequest, result: GetQueueUrlResult) = promise.success(result)
override def onError(exception: Exception) = promise.failure(exception)
})
promise.future
}
/** Invokes the {@code listDeadLetterSourceQueuesAsync} method of the underlying
* client and adapts the result to a Scala {@code Future}.
*
* @param request the request to send
* @return the future result
*/
def listDeadLetterSourceQueues(request: ListDeadLetterSourceQueuesRequest):
scala.concurrent.Future[ListDeadLetterSourceQueuesResult] = {
val opts = request.getRequestClientOptions()
if (opts.getClientMarker(com.amazonaws.RequestClientOptions.Marker.USER_AGENT) == null) {
opts.appendUserAgent("aws-scala-sdk")
}
val promise = scala.concurrent.Promise[ListDeadLetterSourceQueuesResult]
client.listDeadLetterSourceQueuesAsync(request, new com.amazonaws.handlers.AsyncHandler[ListDeadLetterSourceQueuesRequest, ListDeadLetterSourceQueuesResult]() {
override def onSuccess(request: ListDeadLetterSourceQueuesRequest, result: ListDeadLetterSourceQueuesResult) = promise.success(result)
override def onError(exception: Exception) = promise.failure(exception)
})
promise.future
}
/** Invokes the {@code purgeQueueAsync} method of the underlying
* client and adapts the result to a Scala {@code Future}.
*
* @param request the request to send
* @return the future result
*/
def purgeQueue(request: PurgeQueueRequest):
scala.concurrent.Future[scala.runtime.BoxedUnit] = {
val opts = request.getRequestClientOptions()
if (opts.getClientMarker(com.amazonaws.RequestClientOptions.Marker.USER_AGENT) == null) {
opts.appendUserAgent("aws-scala-sdk")
}
val promise = scala.concurrent.Promise[scala.runtime.BoxedUnit]
client.purgeQueueAsync(request, new com.amazonaws.handlers.AsyncHandler[PurgeQueueRequest, Void]() {
override def onSuccess(request: PurgeQueueRequest, result: Void) = promise.success(scala.runtime.BoxedUnit.UNIT)
override def onError(exception: Exception) = promise.failure(exception)
})
promise.future
}
/** Invokes the {@code receiveMessageAsync} method of the underlying
* client and adapts the result to a Scala {@code Future}.
*
* @param request the request to send
* @return the future result
*/
def receiveMessage(request: ReceiveMessageRequest):
scala.concurrent.Future[ReceiveMessageResult] = {
val opts = request.getRequestClientOptions()
if (opts.getClientMarker(com.amazonaws.RequestClientOptions.Marker.USER_AGENT) == null) {
opts.appendUserAgent("aws-scala-sdk")
}
val promise = scala.concurrent.Promise[ReceiveMessageResult]
client.receiveMessageAsync(request, new com.amazonaws.handlers.AsyncHandler[ReceiveMessageRequest, ReceiveMessageResult]() {
override def onSuccess(request: ReceiveMessageRequest, result: ReceiveMessageResult) = promise.success(result)
override def onError(exception: Exception) = promise.failure(exception)
})
promise.future
}
/** Invokes the {@code deleteQueueAsync} method of the underlying
* client and adapts the result to a Scala {@code Future}.
*
* @param request the request to send
* @return the future result
*/
def deleteQueue(request: DeleteQueueRequest):
scala.concurrent.Future[scala.runtime.BoxedUnit] = {
val opts = request.getRequestClientOptions()
if (opts.getClientMarker(com.amazonaws.RequestClientOptions.Marker.USER_AGENT) == null) {
opts.appendUserAgent("aws-scala-sdk")
}
val promise = scala.concurrent.Promise[scala.runtime.BoxedUnit]
client.deleteQueueAsync(request, new com.amazonaws.handlers.AsyncHandler[DeleteQueueRequest, Void]() {
override def onSuccess(request: DeleteQueueRequest, result: Void) = promise.success(scala.runtime.BoxedUnit.UNIT)
override def onError(exception: Exception) = promise.failure(exception)
})
promise.future
}
/** Invokes the {@code createQueueAsync} method of the underlying
* client and adapts the result to a Scala {@code Future}.
*
* @param request the request to send
* @return the future result
*/
def createQueue(request: CreateQueueRequest):
scala.concurrent.Future[CreateQueueResult] = {
val opts = request.getRequestClientOptions()
if (opts.getClientMarker(com.amazonaws.RequestClientOptions.Marker.USER_AGENT) == null) {
opts.appendUserAgent("aws-scala-sdk")
}
val promise = scala.concurrent.Promise[CreateQueueResult]
client.createQueueAsync(request, new com.amazonaws.handlers.AsyncHandler[CreateQueueRequest, CreateQueueResult]() {
override def onSuccess(request: CreateQueueRequest, result: CreateQueueResult) = promise.success(result)
override def onError(exception: Exception) = promise.failure(exception)
})
promise.future
}
/** Invokes the {@code deleteMessageAsync} method of the underlying
* client and adapts the result to a Scala {@code Future}.
*
* @param request the request to send
* @return the future result
*/
def deleteMessage(request: DeleteMessageRequest):
scala.concurrent.Future[scala.runtime.BoxedUnit] = {
val opts = request.getRequestClientOptions()
if (opts.getClientMarker(com.amazonaws.RequestClientOptions.Marker.USER_AGENT) == null) {
opts.appendUserAgent("aws-scala-sdk")
}
val promise = scala.concurrent.Promise[scala.runtime.BoxedUnit]
client.deleteMessageAsync(request, new com.amazonaws.handlers.AsyncHandler[DeleteMessageRequest, Void]() {
override def onSuccess(request: DeleteMessageRequest, result: Void) = promise.success(scala.runtime.BoxedUnit.UNIT)
override def onError(exception: Exception) = promise.failure(exception)
})
promise.future
}
/** Invokes the {@code sendMessageBatchAsync} method of the underlying
* client and adapts the result to a Scala {@code Future}.
*
* @param request the request to send
* @return the future result
*/
def sendMessageBatch(request: SendMessageBatchRequest):
scala.concurrent.Future[SendMessageBatchResult] = {
val opts = request.getRequestClientOptions()
if (opts.getClientMarker(com.amazonaws.RequestClientOptions.Marker.USER_AGENT) == null) {
opts.appendUserAgent("aws-scala-sdk")
}
val promise = scala.concurrent.Promise[SendMessageBatchResult]
client.sendMessageBatchAsync(request, new com.amazonaws.handlers.AsyncHandler[SendMessageBatchRequest, SendMessageBatchResult]() {
override def onSuccess(request: SendMessageBatchRequest, result: SendMessageBatchResult) = promise.success(result)
override def onError(exception: Exception) = promise.failure(exception)
})
promise.future
}
/** Invokes the {@code changeMessageVisibilityBatchAsync} method of the underlying
* client and adapts the result to a Scala {@code Future}.
*
* @param request the request to send
* @return the future result
*/
def changeMessageVisibilityBatch(request: ChangeMessageVisibilityBatchRequest):
scala.concurrent.Future[ChangeMessageVisibilityBatchResult] = {
val opts = request.getRequestClientOptions()
if (opts.getClientMarker(com.amazonaws.RequestClientOptions.Marker.USER_AGENT) == null) {
opts.appendUserAgent("aws-scala-sdk")
}
val promise = scala.concurrent.Promise[ChangeMessageVisibilityBatchResult]
client.changeMessageVisibilityBatchAsync(request, new com.amazonaws.handlers.AsyncHandler[ChangeMessageVisibilityBatchRequest, ChangeMessageVisibilityBatchResult]() {
override def onSuccess(request: ChangeMessageVisibilityBatchRequest, result: ChangeMessageVisibilityBatchResult) = promise.success(result)
override def onError(exception: Exception) = promise.failure(exception)
})
promise.future
}
/** Invokes the {@code listQueuesAsync} method of the underlying
* client and adapts the result to a Scala {@code Future}.
*
* @param request the request to send
* @return the future result
*/
def listQueues(request: ListQueuesRequest):
scala.concurrent.Future[ListQueuesResult] = {
val opts = request.getRequestClientOptions()
if (opts.getClientMarker(com.amazonaws.RequestClientOptions.Marker.USER_AGENT) == null) {
opts.appendUserAgent("aws-scala-sdk")
}
val promise = scala.concurrent.Promise[ListQueuesResult]
client.listQueuesAsync(request, new com.amazonaws.handlers.AsyncHandler[ListQueuesRequest, ListQueuesResult]() {
override def onSuccess(request: ListQueuesRequest, result: ListQueuesResult) = promise.success(result)
override def onError(exception: Exception) = promise.failure(exception)
})
promise.future
}
/** Invokes the {@code deleteMessageBatchAsync} method of the underlying
* client and adapts the result to a Scala {@code Future}.
*
* @param request the request to send
* @return the future result
*/
def deleteMessageBatch(request: DeleteMessageBatchRequest):
scala.concurrent.Future[DeleteMessageBatchResult] = {
val opts = request.getRequestClientOptions()
if (opts.getClientMarker(com.amazonaws.RequestClientOptions.Marker.USER_AGENT) == null) {
opts.appendUserAgent("aws-scala-sdk")
}
val promise = scala.concurrent.Promise[DeleteMessageBatchResult]
client.deleteMessageBatchAsync(request, new com.amazonaws.handlers.AsyncHandler[DeleteMessageBatchRequest, DeleteMessageBatchResult]() {
override def onSuccess(request: DeleteMessageBatchRequest, result: DeleteMessageBatchResult) = promise.success(result)
override def onError(exception: Exception) = promise.failure(exception)
})
promise.future
}
/** Invokes the {@code changeMessageVisibilityAsync} method of the underlying
* client and adapts the result to a Scala {@code Future}.
*
* @param request the request to send
* @return the future result
*/
def changeMessageVisibility(request: ChangeMessageVisibilityRequest):
scala.concurrent.Future[scala.runtime.BoxedUnit] = {
val opts = request.getRequestClientOptions()
if (opts.getClientMarker(com.amazonaws.RequestClientOptions.Marker.USER_AGENT) == null) {
opts.appendUserAgent("aws-scala-sdk")
}
val promise = scala.concurrent.Promise[scala.runtime.BoxedUnit]
client.changeMessageVisibilityAsync(request, new com.amazonaws.handlers.AsyncHandler[ChangeMessageVisibilityRequest, Void]() {
override def onSuccess(request: ChangeMessageVisibilityRequest, result: Void) = promise.success(scala.runtime.BoxedUnit.UNIT)
override def onError(exception: Exception) = promise.failure(exception)
})
promise.future
}
/** Invokes the {@code sendMessageAsync} method of the underlying
* client and adapts the result to a Scala {@code Future}.
*
* @param request the request to send
* @return the future result
*/
def sendMessage(request: SendMessageRequest):
scala.concurrent.Future[SendMessageResult] = {
val opts = request.getRequestClientOptions()
if (opts.getClientMarker(com.amazonaws.RequestClientOptions.Marker.USER_AGENT) == null) {
opts.appendUserAgent("aws-scala-sdk")
}
val promise = scala.concurrent.Promise[SendMessageResult]
client.sendMessageAsync(request, new com.amazonaws.handlers.AsyncHandler[SendMessageRequest, SendMessageResult]() {
override def onSuccess(request: SendMessageRequest, result: SendMessageResult) = promise.success(result)
override def onError(exception: Exception) = promise.failure(exception)
})
promise.future
}
/** Invokes the {@code getQueueAttributesAsync} method of the underlying
* client and adapts the result to a Scala {@code Future}.
*
* @param request the request to send
* @return the future result
*/
def getQueueAttributes(request: GetQueueAttributesRequest):
scala.concurrent.Future[GetQueueAttributesResult] = {
val opts = request.getRequestClientOptions()
if (opts.getClientMarker(com.amazonaws.RequestClientOptions.Marker.USER_AGENT) == null) {
opts.appendUserAgent("aws-scala-sdk")
}
val promise = scala.concurrent.Promise[GetQueueAttributesResult]
client.getQueueAttributesAsync(request, new com.amazonaws.handlers.AsyncHandler[GetQueueAttributesRequest, GetQueueAttributesResult]() {
override def onSuccess(request: GetQueueAttributesRequest, result: GetQueueAttributesResult) = promise.success(result)
override def onError(exception: Exception) = promise.failure(exception)
})
promise.future
}
/** Invokes the {@code addPermissionAsync} method of the underlying
* client and adapts the result to a Scala {@code Future}.
*
* @param request the request to send
* @return the future result
*/
def addPermission(request: AddPermissionRequest):
scala.concurrent.Future[scala.runtime.BoxedUnit] = {
val opts = request.getRequestClientOptions()
if (opts.getClientMarker(com.amazonaws.RequestClientOptions.Marker.USER_AGENT) == null) {
opts.appendUserAgent("aws-scala-sdk")
}
val promise = scala.concurrent.Promise[scala.runtime.BoxedUnit]
client.addPermissionAsync(request, new com.amazonaws.handlers.AsyncHandler[AddPermissionRequest, Void]() {
override def onSuccess(request: AddPermissionRequest, result: Void) = promise.success(scala.runtime.BoxedUnit.UNIT)
override def onError(exception: Exception) = promise.failure(exception)
})
promise.future
}
/** Shuts down this client. */
def shutdown(): Unit = client.shutdown()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy