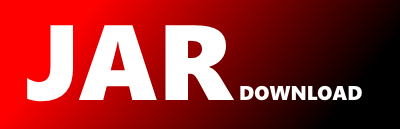
com.amazonaws.services.kinesis.producer.UserRecordResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of amazon-kinesis-producer Show documentation
Show all versions of amazon-kinesis-producer Show documentation
The Amazon Kinesis Producer Library for Java enables developers to easily and reliably put data into Amazon Kinesis.
/*
* Copyright 2015 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Amazon Software License (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/asl/
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.kinesis.producer;
import java.util.ArrayList;
import java.util.List;
import com.amazonaws.services.kinesis.producer.protobuf.Messages;
import com.google.common.collect.ImmutableList;
/**
* The result of a {@link KinesisProducer#addUserRecord} operation. If
* successful, the shard id and sequence number assigned by the backend are
* provided. A list of {@link Attempt}s is also provided with details about each
* attempt made.
*
* @author chaodeng
* @see Attempt
*/
public class UserRecordResult {
private List attempts;
private String sequenceNumber;
private String shardId;
private boolean successful;
private UserRecordResult(List attempts, String sequenceNumber, String shardId, boolean successful) {
this.attempts = attempts;
this.sequenceNumber = sequenceNumber;
this.shardId = shardId;
this.successful = successful;
}
/**
*
* @return List of {@link Attempt}s, in the order they were made.
*/
public List getAttempts() {
return attempts;
}
/**
*
* @return The sequence number assigned by the backend to this record.
* Multiple records may have the same sequence number if aggregation
* is enabled. Will be null if the put failed.
*/
public String getSequenceNumber() {
return sequenceNumber;
}
/**
*
* @return Shard ID returned by the backend. The record was written to this
* shard. Will be null if the put failed.
*/
public String getShardId() {
return shardId;
}
/**
*
* @return Whether the record put was successful. If true, then the record
* has been confirmed by the backend.
*/
public boolean isSuccessful() {
return successful;
}
protected static UserRecordResult fromProtobufMessage(Messages.PutRecordResult r) {
final List attempts = new ArrayList<>(r.getAttemptsCount());
for (Messages.Attempt a : r.getAttemptsList()) {
attempts.add(Attempt.fromProtobufMessage(a));
}
return new UserRecordResult(
new ImmutableList.Builder().addAll(attempts).build(),
r.hasSequenceNumber() ? r.getSequenceNumber() : null,
r.hasShardId() ? r.getShardId() : null,
r.getSuccess());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy