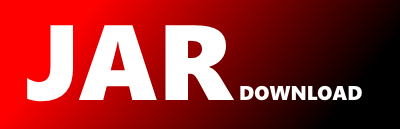
com.amazonaws.mobileconnectors.apigateway.ApiClientHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws-android-sdk-apigateway-core Show documentation
Show all versions of aws-android-sdk-apigateway-core Show documentation
The AWS Android SDK for Amazon API Gateway Runtime module holds the runtime library needed by the generated client for APIs defined in Amazon API Gateway.
/*
* Copyright 2010-2016 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.mobileconnectors.apigateway;
import com.amazonaws.ClientConfiguration;
import com.amazonaws.DefaultRequest;
import com.amazonaws.Request;
import com.amazonaws.auth.AWSCredentialsProvider;
import com.amazonaws.auth.Signer;
import com.amazonaws.http.ExecutionContext;
import com.amazonaws.http.HttpClient;
import com.amazonaws.http.HttpMethodName;
import com.amazonaws.http.HttpRequest;
import com.amazonaws.http.HttpRequestFactory;
import com.amazonaws.http.HttpResponse;
import com.amazonaws.http.UrlHttpClient;
import com.amazonaws.mobileconnectors.apigateway.annotation.Operation;
import com.amazonaws.mobileconnectors.apigateway.annotation.Parameter;
import com.amazonaws.util.IOUtils;
import com.amazonaws.util.StringUtils;
import com.google.gson.Gson;
import java.io.ByteArrayInputStream;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.Reader;
import java.lang.annotation.Annotation;
import java.lang.reflect.InvocationHandler;
import java.lang.reflect.Method;
import java.lang.reflect.Type;
import java.net.URI;
import java.util.Collection;
import java.util.Map;
/**
* Invocation handler responsible for serializing a request and deserializing a
* response.
*/
class ApiClientHandler implements InvocationHandler {
private static final Gson gson = new Gson();
private final String endpoint;
private final String apiName;
private final Signer signer;
// credentials provider. If null, request won't be signed
private final AWSCredentialsProvider provider;
// api key to invoke a method. If non null, its value will be sent in
// 'x-api-key' header.
private final String apiKey;
private final HttpClient client;
private final HttpRequestFactory requestFactory;
private final ClientConfiguration clientConfiguration;
ApiClientHandler(String endpoint, String apiName,
Signer signer, AWSCredentialsProvider provider, String apiKey) {
this.endpoint = endpoint;
this.apiName = apiName;
this.signer = signer;
this.provider = provider;
this.apiKey = apiKey;
clientConfiguration = new ClientConfiguration();
client = new UrlHttpClient(clientConfiguration);
requestFactory = new HttpRequestFactory();
}
@Override
public Object invoke(Object proxy, Method method, Object[] args)
throws Throwable {
Request> request = buildRequest(method, args);
ExecutionContext context = new ExecutionContext();
context.setContextUserAgent(apiName);
HttpRequest httpRequest = requestFactory.createHttpRequest(request, clientConfiguration,
context);
HttpResponse response = client.execute(httpRequest);
return handleResponse(response, method);
}
/**
* Build a {@link Request} object for the given method.
*
* @param method method that annotated with {@link Operation}
* @param args arguments of the method
* @return a {@link Request} object
*/
Request> buildRequest(Method method, Object[] args) {
Operation op = method.getAnnotation(Operation.class);
if (op == null) {
throw new IllegalArgumentException("Method isn't annotated with Operation");
}
Request> request = new DefaultRequest
© 2015 - 2025 Weber Informatics LLC | Privacy Policy