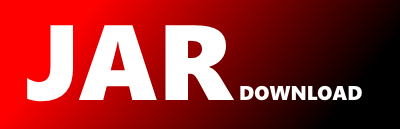
com.apollographql.apollo.api.Response Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws-android-sdk-appsync-api Show documentation
Show all versions of aws-android-sdk-appsync-api Show documentation
AWS SDK for Android GraphQL API classes
/**
* Copyright 2018-2019 Amazon.com,
* Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Amazon Software License (the "License").
* You may not use this file except in compliance with the
* License. A copy of the License is located at
*
* http://aws.amazon.com/asl/
*
* or in the "license" file accompanying this file. This file is
* distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, express or implied. See the License
* for the specific language governing permissions and
* limitations under the License.
*/
package com.apollographql.apollo.api;
import java.util.Collections;
import java.util.List;
import java.util.Set;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import static com.apollographql.apollo.api.internal.Utils.checkNotNull;
import static java.util.Collections.unmodifiableList;
import static java.util.Collections.unmodifiableSet;
/** Represents either a successful or failed response received from the GraphQL server. */
public final class Response {
private final Operation operation;
private final T data;
private final List errors;
private Set dependentKeys;
private final boolean fromCache;
public static Response.Builder builder(@Nonnull final Operation operation) {
return new Builder<>(operation);
}
Response(Builder builder) {
operation = checkNotNull(builder.operation, "operation == null");
data = builder.data;
errors = builder.errors != null ? unmodifiableList(builder.errors) : Collections.emptyList();
dependentKeys = builder.dependentKeys != null ? unmodifiableSet(builder.dependentKeys)
: Collections.emptySet();
fromCache = builder.fromCache;
}
public Operation operation() {
return operation;
}
@Nullable public T data() {
return data;
}
@Nonnull public List errors() {
return errors;
}
@Nonnull public Set dependentKeys() {
return dependentKeys;
}
public boolean hasErrors() {
return !errors.isEmpty();
}
public boolean fromCache() {
return fromCache;
}
public Builder toBuilder() {
return new Builder(operation)
.data(data)
.errors(errors)
.dependentKeys(dependentKeys)
.fromCache(fromCache);
}
public static final class Builder {
private final Operation operation;
private T data;
private List errors;
private Set dependentKeys;
private boolean fromCache;
Builder(@Nonnull final Operation operation) {
this.operation = checkNotNull(operation, "operation == null");
}
public Builder data(T data) {
this.data = data;
return this;
}
public Builder errors(@Nullable List errors) {
this.errors = errors;
return this;
}
public Builder dependentKeys(@Nullable Set dependentKeys) {
this.dependentKeys = dependentKeys;
return this;
}
public Builder fromCache(boolean fromCache) {
this.fromCache = fromCache;
return this;
}
public Response build() {
return new Response<>(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy