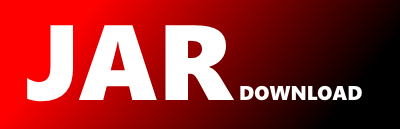
com.apollographql.apollo.internal.subscription.SubscriptionManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws-android-sdk-appsync-runtime Show documentation
Show all versions of aws-android-sdk-appsync-runtime Show documentation
AWS AppSync GraphQL runtime library to support generated code
/**
* Copyright 2018-2019 Amazon.com,
* Inc. or its affiliates. All Rights Reserved.
*
* SPDX-License-Identifier: Apache-2.0
*/
package com.apollographql.apollo.internal.subscription;
import com.amazonaws.mobileconnectors.appsync.AppSyncSubscriptionCall;
import com.amazonaws.mobileconnectors.appsync.subscription.SubscriptionResponse;
import com.apollographql.apollo.api.Response;
import com.apollographql.apollo.api.Subscription;
import com.apollographql.apollo.cache.normalized.ApolloStore;
import com.apollographql.apollo.internal.cache.normalized.ResponseNormalizer;
import com.apollographql.apollo.internal.response.ScalarTypeAdapters;
import java.util.List;
import java.util.Map;
import javax.annotation.Nonnull;
public interface SubscriptionManager {
/**
* Make a connection and subscribe to all topics.
* @param The type of the repsonse data
* @param subscription The operation that has parsing logic, operation id, etc.
* @param subbedTopics The topics relevant to the subscription.
* @param response list of mqtt connections and topics
* @param mapResponseNormalizer
*/
void subscribe(
@Nonnull Subscription, T, ?> subscription,
@Nonnull final List subbedTopics,
@Nonnull SubscriptionResponse response,
ResponseNormalizer
© 2015 - 2025 Weber Informatics LLC | Privacy Policy