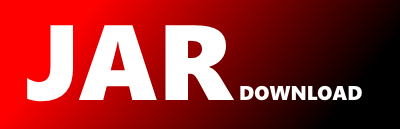
com.amazonaws.services.dynamodbv2.model.UpdateItemRequest Maven / Gradle / Ivy
/*
* Copyright 2010-2016 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.dynamodbv2.model;
import java.io.Serializable;
import com.amazonaws.AmazonWebServiceRequest;
/**
* Container for the parameters to the {@link com.amazonaws.services.dynamodbv2.AmazonDynamoDB#updateItem(UpdateItemRequest) UpdateItem operation}.
*
* Edits an existing item's attributes, or adds a new item to the table
* if it does not already exist. You can put, delete, or add attribute
* values. You can also perform a conditional update on an existing item
* (insert a new attribute name-value pair if it doesn't exist, or
* replace an existing name-value pair if it has certain expected
* attribute values).
*
*
* You can also return the item's attribute values in the same
* UpdateItem operation using the ReturnValues parameter.
*
*
* @see com.amazonaws.services.dynamodbv2.AmazonDynamoDB#updateItem(UpdateItemRequest)
*/
public class UpdateItemRequest extends AmazonWebServiceRequest implements Serializable {
/**
* The name of the table containing the item to update.
*
* Constraints:
* Length: 3 - 255
* Pattern: [a-zA-Z0-9_.-]+
*/
private String tableName;
/**
* The primary key of the item to be updated. Each element consists of an
* attribute name and a value for that attribute.
For the primary key,
* you must provide all of the attributes. For example, with a simple
* primary key, you only need to provide a value for the partition key.
* For a composite primary key, you must provide values for both the
* partition key and the sort key.
*/
private java.util.Map key;
/**
* This is a legacy parameter, for backward compatibility.
* New applications should use UpdateExpression instead. Do not
* combine legacy parameters and expression parameters in a single API
* call; otherwise, DynamoDB will return a ValidationException
* exception.
This parameter can be used for modifying top-level
* attributes; however, it does not support individual list or map
* elements.
The names of attributes to be modified, the
* action to perform on each, and the new value for each. If you are
* updating an attribute that is an index key attribute for any indexes
* on that table, the attribute type must match the index key type
* defined in the AttributesDefinition of the table description.
* You can use UpdateItem to update any non-key attributes.
*
Attribute values cannot be null. String and Binary type attributes
* must have lengths greater than zero. Set type attributes must not be
* empty. Requests with empty values will be rejected with a
* ValidationException exception.
Each AttributeUpdates
* element consists of an attribute name to modify, along with the
* following:
-
Value - The new value, if applicable,
* for this attribute.
-
Action - A value that
* specifies how to perform the update. This action is only valid for an
* existing attribute whose data type is Number or is a set; do not use
* ADD
for other data types.
If an item with the
* specified primary key is found in the table, the following values
* perform the following actions:
-
PUT
- Adds
* the specified attribute to the item. If the attribute already exists,
* it is replaced by the new value.
-
DELETE
-
* Removes the attribute and its value, if no value is specified for
* DELETE
. The data type of the specified value must match
* the existing value's data type.
If a set of values is specified,
* then those values are subtracted from the old set. For example, if the
* attribute value was the set [a,b,c]
and the
* DELETE
action specifies [a,c]
, then the
* final attribute value is [b]
. Specifying an empty set is
* an error.
-
ADD
- Adds the specified value to
* the item, if the attribute does not already exist. If the attribute
* does exist, then the behavior of ADD
depends on the data
* type of the attribute:
-
If the existing attribute is a
* number, and if Value is also a number, then Value is
* mathematically added to the existing attribute. If Value is a
* negative number, then it is subtracted from the existing attribute.
* If you use ADD
to increment or decrement a
* number value for an item that doesn't exist before the update,
* DynamoDB uses 0 as the initial value.
Similarly, if you use
* ADD
for an existing item to increment or decrement an
* attribute value that doesn't exist before the update, DynamoDB uses
* 0
as the initial value. For example, suppose that the
* item you want to update doesn't have an attribute named
* itemcount, but you decide to ADD
the number
* 3
to this attribute anyway. DynamoDB will create the
* itemcount attribute, set its initial value to 0
,
* and finally add 3
to it. The result will be a new
* itemcount attribute, with a value of 3
.
*
-
If the existing data type is a set, and if Value
* is also a set, then Value is appended to the existing set. For
* example, if the attribute value is the set [1,2]
, and the
* ADD
action specified [3]
, then the final
* attribute value is [1,2,3]
. An error occurs if an
* ADD
action is specified for a set attribute and the
* attribute type specified does not match the existing set type.
Both
* sets must have the same primitive data type. For example, if the
* existing data type is a set of strings, Value must also be a
* set of strings.
If no item with the
* specified key is found in the table, the following values perform the
* following actions:
-
PUT
- Causes DynamoDB to
* create a new item with the specified primary key, and then adds the
* attribute.
-
DELETE
- Nothing happens,
* because attributes cannot be deleted from a nonexistent item. The
* operation succeeds, but DynamoDB does not create a new item.
* -
ADD
- Causes DynamoDB to create an item with the
* supplied primary key and number (or set of numbers) for the attribute
* value. The only data types allowed are Number and Number Set.
*
If you provide any attributes that are part of an
* index key, then the data types for those attributes must match those
* of the schema in the table's attribute definition.
*/
private java.util.Map attributeUpdates;
/**
* This is a legacy parameter, for backward compatibility.
* New applications should use ConditionExpression instead. Do
* not combine legacy parameters and expression parameters in a single
* API call; otherwise, DynamoDB will return a ValidationException
* exception.
A map of attribute/condition pairs.
* Expected provides a conditional block for the UpdateItem
* operation.
Each element of Expected consists of an attribute
* name, a comparison operator, and one or more values. DynamoDB compares
* the attribute with the value(s) you supplied, using the comparison
* operator. For each Expected element, the result of the
* evaluation is either true or false.
If you specify more than one
* element in the Expected map, then by default all of the
* conditions must evaluate to true. In other words, the conditions are
* ANDed together. (You can use the ConditionalOperator parameter
* to OR the conditions instead. If you do this, then at least one of the
* conditions must evaluate to true, rather than all of them.)
If the
* Expected map evaluates to true, then the conditional operation
* succeeds; otherwise, it fails.
Expected contains the
* following:
-
AttributeValueList - One or more values
* to evaluate against the supplied attribute. The number of values in
* the list depends on the ComparisonOperator being used.
For
* type Number, value comparisons are numeric.
String value
* comparisons for greater than, equals, or less than are based on ASCII
* character code values. For example, a
is greater than
* A
, and a
is greater than B
. For
* a list of code values, see http://en.wikipedia.org/wiki/ASCII#ASCII_printable_characters.
*
For type Binary, DynamoDB treats each byte of the binary data as
* unsigned when it compares binary values.
-
*
ComparisonOperator - A comparator for evaluating attributes
* in the AttributeValueList. When performing the comparison,
* DynamoDB uses strongly consistent reads.
The following comparison
* operators are available:
EQ | NE | LE | LT | GE | GT |
* NOT_NULL | NULL | CONTAINS | NOT_CONTAINS | BEGINS_WITH | IN |
* BETWEEN
The following are descriptions of each comparison
* operator.
-
EQ
: Equal. EQ
is
* supported for all datatypes, including lists and maps.
*
AttributeValueList can contain only one
* AttributeValue element of type String, Number, Binary, String
* Set, Number Set, or Binary Set. If an item contains an
* AttributeValue element of a different type than the one
* provided in the request, the value does not match. For example,
* {"S":"6"}
does not equal {"N":"6"}
. Also,
* {"N":"6"}
does not equal {"NS":["6", "2",
* "1"]}
.
-
NE
: Not equal.
* NE
is supported for all datatypes, including lists and
* maps.
AttributeValueList can contain only one
* AttributeValue of type String, Number, Binary, String Set,
* Number Set, or Binary Set. If an item contains an
* AttributeValue of a different type than the one provided in the
* request, the value does not match. For example, {"S":"6"}
* does not equal {"N":"6"}
. Also, {"N":"6"}
* does not equal {"NS":["6", "2", "1"]}
.
-
*
LE
: Less than or equal.
AttributeValueList
* can contain only one AttributeValue element of type String,
* Number, or Binary (not a set type). If an item contains an
* AttributeValue element of a different type than the one
* provided in the request, the value does not match. For example,
* {"S":"6"}
does not equal {"N":"6"}
. Also,
* {"N":"6"}
does not compare to {"NS":["6", "2",
* "1"]}
.
-
LT
: Less than.
*
AttributeValueList can contain only one
* AttributeValue of type String, Number, or Binary (not a set
* type). If an item contains an AttributeValue element of a
* different type than the one provided in the request, the value does
* not match. For example, {"S":"6"}
does not equal
* {"N":"6"}
. Also, {"N":"6"}
does not compare
* to {"NS":["6", "2", "1"]}
.
-
*
GE
: Greater than or equal.
*
AttributeValueList can contain only one
* AttributeValue element of type String, Number, or Binary (not a
* set type). If an item contains an AttributeValue element of a
* different type than the one provided in the request, the value does
* not match. For example, {"S":"6"}
does not equal
* {"N":"6"}
. Also, {"N":"6"}
does not compare
* to {"NS":["6", "2", "1"]}
.
-
*
GT
: Greater than.
AttributeValueList can
* contain only one AttributeValue element of type String, Number,
* or Binary (not a set type). If an item contains an
* AttributeValue element of a different type than the one
* provided in the request, the value does not match. For example,
* {"S":"6"}
does not equal {"N":"6"}
. Also,
* {"N":"6"}
does not compare to {"NS":["6", "2",
* "1"]}
.
-
NOT_NULL
: The attribute
* exists. NOT_NULL
is supported for all datatypes,
* including lists and maps. This operator tests for the
* existence of an attribute, not its data type. If the data type of
* attribute "a
" is null, and you evaluate it using
* NOT_NULL
, the result is a Boolean true. This
* result is because the attribute "a
" exists; its data type
* is not relevant to the NOT_NULL
comparison operator.
*
-
NULL
: The attribute does not
* exist. NULL
is supported for all datatypes, including
* lists and maps. This operator tests for the nonexistence of
* an attribute, not its data type. If the data type of attribute
* "a
" is null, and you evaluate it using NULL
,
* the result is a Boolean false. This is because the attribute
* "a
" exists; its data type is not relevant to the
* NULL
comparison operator.
-
*
CONTAINS
: Checks for a subsequence, or value in a
* set.
AttributeValueList can contain only one
* AttributeValue element of type String, Number, or Binary (not a
* set type). If the target attribute of the comparison is of type
* String, then the operator checks for a substring match. If the target
* attribute of the comparison is of type Binary, then the operator looks
* for a subsequence of the target that matches the input. If the target
* attribute of the comparison is a set ("SS
",
* "NS
", or "BS
"), then the operator evaluates
* to true if it finds an exact match with any member of the set.
*
CONTAINS is supported for lists: When evaluating "a CONTAINS
* b
", "a
" can be a list; however, "b
"
* cannot be a set, a map, or a list.
-
*
NOT_CONTAINS
: Checks for absence of a subsequence, or
* absence of a value in a set.
AttributeValueList can contain
* only one AttributeValue element of type String, Number, or
* Binary (not a set type). If the target attribute of the comparison is
* a String, then the operator checks for the absence of a substring
* match. If the target attribute of the comparison is Binary, then the
* operator checks for the absence of a subsequence of the target that
* matches the input. If the target attribute of the comparison is a set
* ("SS
", "NS
", or "BS
"), then the
* operator evaluates to true if it does not find an exact match
* with any member of the set.
NOT_CONTAINS is supported for lists:
* When evaluating "a NOT CONTAINS b
", "a
" can
* be a list; however, "b
" cannot be a set, a map, or a
* list.
-
BEGINS_WITH
: Checks for a prefix.
*
AttributeValueList can contain only one
* AttributeValue of type String or Binary (not a Number or a set
* type). The target attribute of the comparison must be of type String
* or Binary (not a Number or a set type).
-
*
IN
: Checks for matching elements within two sets.
*
AttributeValueList can contain one or more
* AttributeValue elements of type String, Number, or Binary (not
* a set type). These attributes are compared against an existing set
* type attribute of an item. If any elements of the input set are
* present in the item attribute, the expression evaluates to true.
* -
BETWEEN
: Greater than or equal to the first
* value, and less than or equal to the second value.
*
AttributeValueList must contain two AttributeValue
* elements of the same type, either String, Number, or Binary (not a set
* type). A target attribute matches if the target value is greater than,
* or equal to, the first element and less than, or equal to, the second
* element. If an item contains an AttributeValue element of a
* different type than the one provided in the request, the value does
* not match. For example, {"S":"6"}
does not compare to
* {"N":"6"}
. Also, {"N":"6"}
does not compare
* to {"NS":["6", "2", "1"]}
For
* usage examples of AttributeValueList and
* ComparisonOperator, see Legacy
* Conditional Parameters in the Amazon DynamoDB Developer
* Guide.
For backward compatibility with previous DynamoDB
* releases, the following parameters can be used instead of
* AttributeValueList and ComparisonOperator:
-
*
Value - A value for DynamoDB to compare with an attribute.
*
-
Exists - A Boolean value that causes DynamoDB to
* evaluate the value before attempting the conditional operation:
* -
If Exists is true
, DynamoDB will check to
* see if that attribute value already exists in the table. If it is
* found, then the condition evaluates to true; otherwise the condition
* evaluate to false.
If Exists is
* false
, DynamoDB assumes that the attribute value does
* not exist in the table. If in fact the value does not exist,
* then the assumption is valid and the condition evaluates to true. If
* the value is found, despite the assumption that it does not exist, the
* condition evaluates to false.
Note that the default
* value for Exists is true
.
The
* Value and Exists parameters are incompatible with
* AttributeValueList and ComparisonOperator. Note that if
* you use both sets of parameters at once, DynamoDB will return a
* ValidationException exception. This parameter does not
* support attributes of type List or Map.
*/
private java.util.Map expected;
/**
* This is a legacy parameter, for backward compatibility.
* New applications should use ConditionExpression instead. Do not
* combine legacy parameters and expression parameters in a single API
* call; otherwise, DynamoDB will return a ValidationException
* exception.
A logical operator to apply to the
* conditions in the Expected map:
AND
-
* If all of the conditions evaluate to true, then the entire map
* evaluates to true.
OR
- If at least one of
* the conditions evaluate to true, then the entire map evaluates to
* true.
If you omit ConditionalOperator, then
* AND
is the default.
The operation will succeed only if
* the entire map evaluates to true. This parameter does not
* support attributes of type List or Map.
*
* Constraints:
* Allowed Values: AND, OR
*/
private String conditionalOperator;
/**
* Use ReturnValues if you want to get the item attributes as they
* appeared either before or after they were updated. For
* UpdateItem, the valid values are:
-
*
NONE
- If ReturnValues is not specified, or if
* its value is NONE
, then nothing is returned. (This
* setting is the default for ReturnValues.)
-
*
ALL_OLD
- If UpdateItem overwrote an attribute
* name-value pair, then the content of the old item is returned.
* -
UPDATED_OLD
- The old versions of only the
* updated attributes are returned.
-
ALL_NEW
-
* All of the attributes of the new version of the item are returned.
*
-
UPDATED_NEW
- The new versions of only the
* updated attributes are returned.
There is no additional
* cost associated with requesting a return value aside from the small
* network and processing overhead of receiving a larger response. No
* Read Capacity Units are consumed.
Values returned are strongly
* consistent
*
* Constraints:
* Allowed Values: NONE, ALL_OLD, UPDATED_OLD, ALL_NEW, UPDATED_NEW
*/
private String returnValues;
/**
* Determines the level of detail about provisioned throughput
* consumption that is returned in the response:
* INDEXES - The response includes the aggregate
* ConsumedCapacity for the operation, together with
* ConsumedCapacity for each table and secondary index that was
* accessed.
Note that some operations, such as GetItem and
* BatchGetItem, do not access any indexes at all. In these cases,
* specifying INDEXES will only return ConsumedCapacity
* information for table(s).
TOTAL - The response
* includes only the aggregate ConsumedCapacity for the
* operation.
NONE - No ConsumedCapacity
* details are included in the response.
*
* Constraints:
* Allowed Values: INDEXES, TOTAL, NONE
*/
private String returnConsumedCapacity;
/**
* Determines whether item collection metrics are returned. If set to
* SIZE
, the response includes statistics about item
* collections, if any, that were modified during the operation are
* returned in the response. If set to NONE
(the default),
* no statistics are returned.
*
* Constraints:
* Allowed Values: SIZE, NONE
*/
private String returnItemCollectionMetrics;
/**
* An expression that defines one or more attributes to be updated, the
* action to be performed on them, and new value(s) for them.
The
* following action values are available for UpdateExpression.
*
-
SET
- Adds one or more attributes and values
* to an item. If any of these attribute already exist, they are replaced
* by the new values. You can also use SET
to add or
* subtract from an attribute that is of type Number. For example:
* SET myNum = myNum + :val
SET
supports the
* following functions:
if_not_exists (path,
* operand)
- if the item does not contain an attribute at the
* specified path, then if_not_exists
evaluates to operand;
* otherwise, it evaluates to path. You can use this function to avoid
* overwriting an attribute that may already be present in the item.
* list_append (operand, operand)
- evaluates to a
* list with a new element added to it. You can append the new element to
* the start or the end of the list by reversing the order of the
* operands.
These function names are case-sensitive.
* -
REMOVE
- Removes one or more attributes from an
* item.
-
ADD
- Adds the specified value to the
* item, if the attribute does not already exist. If the attribute does
* exist, then the behavior of ADD
depends on the data type
* of the attribute:
-
If the existing attribute is a number,
* and if Value is also a number, then Value is
* mathematically added to the existing attribute. If Value is a
* negative number, then it is subtracted from the existing attribute.
* If you use ADD
to increment or decrement a
* number value for an item that doesn't exist before the update,
* DynamoDB uses 0
as the initial value.
Similarly, if
* you use ADD
for an existing item to increment or
* decrement an attribute value that doesn't exist before the update,
* DynamoDB uses 0
as the initial value. For example,
* suppose that the item you want to update doesn't have an attribute
* named itemcount, but you decide to ADD
the number
* 3
to this attribute anyway. DynamoDB will create the
* itemcount attribute, set its initial value to 0
,
* and finally add 3
to it. The result will be a new
* itemcount attribute in the item, with a value of
* 3
.
-
If the existing data type is a
* set and if Value is also a set, then Value is added to
* the existing set. For example, if the attribute value is the set
* [1,2]
, and the ADD
action specified
* [3]
, then the final attribute value is
* [1,2,3]
. An error occurs if an ADD
action is
* specified for a set attribute and the attribute type specified does
* not match the existing set type.
Both sets must have the same
* primitive data type. For example, if the existing data type is a set
* of strings, the Value must also be a set of strings.
*
The ADD
action only supports Number
* and set data types. In addition, ADD
can only be used on
* top-level attributes, not nested attributes.
-
*
DELETE
- Deletes an element from a set.
If a set of
* values is specified, then those values are subtracted from the old
* set. For example, if the attribute value was the set
* [a,b,c]
and the DELETE
action specifies
* [a,c]
, then the final attribute value is
* [b]
. Specifying an empty set is an error.
* The DELETE
action only supports set data
* types. In addition, DELETE
can only be used on top-level
* attributes, not nested attributes.
You can
* have many actions in a single expression, such as the following:
* SET a=:value1, b=:value2 DELETE :value3, :value4, :value5
*
For more information on update expressions, see Modifying
* Items and Attributes in the Amazon DynamoDB Developer
* Guide. UpdateExpression replaces the legacy
* AttributeUpdates parameter.
*/
private String updateExpression;
/**
* A condition that must be satisfied in order for a conditional update
* to succeed.
An expression can contain any of the following:
* -
Functions: attribute_exists | attribute_not_exists |
* attribute_type | contains | begins_with | size
These
* function names are case-sensitive.
-
Comparison operators:
* = | <> | < | > | <= | >= | BETWEEN | IN
-
* Logical operators: AND | OR | NOT
For more
* information on condition expressions, see Specifying
* Conditions in the Amazon DynamoDB Developer Guide.
* ConditionExpression replaces the legacy
* ConditionalOperator and Expected parameters.
*/
private String conditionExpression;
/**
* One or more substitution tokens for attribute names in an expression.
* The following are some use cases for using
* ExpressionAttributeNames:
-
To access an attribute
* whose name conflicts with a DynamoDB reserved word.
-
To
* create a placeholder for repeating occurrences of an attribute name in
* an expression.
-
To prevent special characters in an
* attribute name from being misinterpreted in an expression.
* Use the # character in an expression to dereference an
* attribute name. For example, consider the following attribute name:
*
Percentile
The name of this
* attribute conflicts with a reserved word, so it cannot be used
* directly in an expression. (For the complete list of reserved words,
* see Reserved
* Words in the Amazon DynamoDB Developer Guide). To work
* around this, you could specify the following for
* ExpressionAttributeNames:
*
{"#P":"Percentile"}
You could
* then use this substitution in an expression, as in this example:
*
#P = :val
Tokens that begin
* with the : character are expression attribute values,
* which are placeholders for the actual value at runtime.
For
* more information on expression attribute names, see Accessing
* Item Attributes in the Amazon DynamoDB Developer Guide.
*/
private java.util.Map expressionAttributeNames;
/**
* One or more values that can be substituted in an expression. Use
* the : (colon) character in an expression to dereference an
* attribute value. For example, suppose that you wanted to check whether
* the value of the ProductStatus attribute was one of the
* following:
Available | Backordered | Discontinued
*
You would first need to specify ExpressionAttributeValues as
* follows:
{ ":avail":{"S":"Available"},
* ":back":{"S":"Backordered"}, ":disc":{"S":"Discontinued"} }
*
You could then use these values in an expression, such as this:
*
ProductStatus IN (:avail, :back, :disc)
For more
* information on expression attribute values, see Specifying
* Conditions in the Amazon DynamoDB Developer Guide.
*/
private java.util.Map expressionAttributeValues;
/**
* Default constructor for a new UpdateItemRequest object. Callers should use the
* setter or fluent setter (with...) methods to initialize this object after creating it.
*/
public UpdateItemRequest() {}
/**
* Constructs a new UpdateItemRequest object.
* Callers should use the setter or fluent setter (with...) methods to
* initialize any additional object members.
*
* @param tableName The name of the table containing the item to update.
* @param key The primary key of the item to be updated. Each element
* consists of an attribute name and a value for that attribute. For
* the primary key, you must provide all of the attributes. For example,
* with a simple primary key, you only need to provide a value for the
* partition key. For a composite primary key, you must provide values
* for both the partition key and the sort key.
* @param attributeUpdates This is a legacy parameter, for
* backward compatibility. New applications should use
* UpdateExpression instead. Do not combine legacy parameters and
* expression parameters in a single API call; otherwise, DynamoDB will
* return a ValidationException exception.
This parameter can
* be used for modifying top-level attributes; however, it does not
* support individual list or map elements.
The names of
* attributes to be modified, the action to perform on each, and the new
* value for each. If you are updating an attribute that is an index key
* attribute for any indexes on that table, the attribute type must match
* the index key type defined in the AttributesDefinition of the
* table description. You can use UpdateItem to update any non-key
* attributes.
Attribute values cannot be null. String and Binary type
* attributes must have lengths greater than zero. Set type attributes
* must not be empty. Requests with empty values will be rejected with a
* ValidationException exception.
Each AttributeUpdates
* element consists of an attribute name to modify, along with the
* following:
-
Value - The new value, if applicable,
* for this attribute.
-
Action - A value that
* specifies how to perform the update. This action is only valid for an
* existing attribute whose data type is Number or is a set; do not use
* ADD
for other data types.
If an item with the
* specified primary key is found in the table, the following values
* perform the following actions:
-
PUT
- Adds
* the specified attribute to the item. If the attribute already exists,
* it is replaced by the new value.
-
DELETE
-
* Removes the attribute and its value, if no value is specified for
* DELETE
. The data type of the specified value must match
* the existing value's data type.
If a set of values is specified,
* then those values are subtracted from the old set. For example, if the
* attribute value was the set [a,b,c]
and the
* DELETE
action specifies [a,c]
, then the
* final attribute value is [b]
. Specifying an empty set is
* an error.
-
ADD
- Adds the specified value to
* the item, if the attribute does not already exist. If the attribute
* does exist, then the behavior of ADD
depends on the data
* type of the attribute:
-
If the existing attribute is a
* number, and if Value is also a number, then Value is
* mathematically added to the existing attribute. If Value is a
* negative number, then it is subtracted from the existing attribute.
* If you use ADD
to increment or decrement a
* number value for an item that doesn't exist before the update,
* DynamoDB uses 0 as the initial value.
Similarly, if you use
* ADD
for an existing item to increment or decrement an
* attribute value that doesn't exist before the update, DynamoDB uses
* 0
as the initial value. For example, suppose that the
* item you want to update doesn't have an attribute named
* itemcount, but you decide to ADD
the number
* 3
to this attribute anyway. DynamoDB will create the
* itemcount attribute, set its initial value to 0
,
* and finally add 3
to it. The result will be a new
* itemcount attribute, with a value of 3
.
*
-
If the existing data type is a set, and if Value
* is also a set, then Value is appended to the existing set. For
* example, if the attribute value is the set [1,2]
, and the
* ADD
action specified [3]
, then the final
* attribute value is [1,2,3]
. An error occurs if an
* ADD
action is specified for a set attribute and the
* attribute type specified does not match the existing set type.
Both
* sets must have the same primitive data type. For example, if the
* existing data type is a set of strings, Value must also be a
* set of strings.
If no item with the
* specified key is found in the table, the following values perform the
* following actions:
-
PUT
- Causes DynamoDB to
* create a new item with the specified primary key, and then adds the
* attribute.
-
DELETE
- Nothing happens,
* because attributes cannot be deleted from a nonexistent item. The
* operation succeeds, but DynamoDB does not create a new item.
* -
ADD
- Causes DynamoDB to create an item with the
* supplied primary key and number (or set of numbers) for the attribute
* value. The only data types allowed are Number and Number Set.
*
If you provide any attributes that are part of an
* index key, then the data types for those attributes must match those
* of the schema in the table's attribute definition.
*/
public UpdateItemRequest(String tableName, java.util.Map key, java.util.Map attributeUpdates) {
setTableName(tableName);
setKey(key);
setAttributeUpdates(attributeUpdates);
}
/**
* Constructs a new UpdateItemRequest object.
* Callers should use the setter or fluent setter (with...) methods to
* initialize any additional object members.
*
* @param tableName The name of the table containing the item to update.
* @param key The primary key of the item to be updated. Each element
* consists of an attribute name and a value for that attribute. For
* the primary key, you must provide all of the attributes. For example,
* with a simple primary key, you only need to provide a value for the
* partition key. For a composite primary key, you must provide values
* for both the partition key and the sort key.
* @param attributeUpdates This is a legacy parameter, for
* backward compatibility. New applications should use
* UpdateExpression instead. Do not combine legacy parameters and
* expression parameters in a single API call; otherwise, DynamoDB will
* return a ValidationException exception.
This parameter can
* be used for modifying top-level attributes; however, it does not
* support individual list or map elements.
The names of
* attributes to be modified, the action to perform on each, and the new
* value for each. If you are updating an attribute that is an index key
* attribute for any indexes on that table, the attribute type must match
* the index key type defined in the AttributesDefinition of the
* table description. You can use UpdateItem to update any non-key
* attributes.
Attribute values cannot be null. String and Binary type
* attributes must have lengths greater than zero. Set type attributes
* must not be empty. Requests with empty values will be rejected with a
* ValidationException exception.
Each AttributeUpdates
* element consists of an attribute name to modify, along with the
* following:
-
Value - The new value, if applicable,
* for this attribute.
-
Action - A value that
* specifies how to perform the update. This action is only valid for an
* existing attribute whose data type is Number or is a set; do not use
* ADD
for other data types.
If an item with the
* specified primary key is found in the table, the following values
* perform the following actions:
-
PUT
- Adds
* the specified attribute to the item. If the attribute already exists,
* it is replaced by the new value.
-
DELETE
-
* Removes the attribute and its value, if no value is specified for
* DELETE
. The data type of the specified value must match
* the existing value's data type.
If a set of values is specified,
* then those values are subtracted from the old set. For example, if the
* attribute value was the set [a,b,c]
and the
* DELETE
action specifies [a,c]
, then the
* final attribute value is [b]
. Specifying an empty set is
* an error.
-
ADD
- Adds the specified value to
* the item, if the attribute does not already exist. If the attribute
* does exist, then the behavior of ADD
depends on the data
* type of the attribute:
-
If the existing attribute is a
* number, and if Value is also a number, then Value is
* mathematically added to the existing attribute. If Value is a
* negative number, then it is subtracted from the existing attribute.
* If you use ADD
to increment or decrement a
* number value for an item that doesn't exist before the update,
* DynamoDB uses 0 as the initial value.
Similarly, if you use
* ADD
for an existing item to increment or decrement an
* attribute value that doesn't exist before the update, DynamoDB uses
* 0
as the initial value. For example, suppose that the
* item you want to update doesn't have an attribute named
* itemcount, but you decide to ADD
the number
* 3
to this attribute anyway. DynamoDB will create the
* itemcount attribute, set its initial value to 0
,
* and finally add 3
to it. The result will be a new
* itemcount attribute, with a value of 3
.
*
-
If the existing data type is a set, and if Value
* is also a set, then Value is appended to the existing set. For
* example, if the attribute value is the set [1,2]
, and the
* ADD
action specified [3]
, then the final
* attribute value is [1,2,3]
. An error occurs if an
* ADD
action is specified for a set attribute and the
* attribute type specified does not match the existing set type.
Both
* sets must have the same primitive data type. For example, if the
* existing data type is a set of strings, Value must also be a
* set of strings.
If no item with the
* specified key is found in the table, the following values perform the
* following actions:
-
PUT
- Causes DynamoDB to
* create a new item with the specified primary key, and then adds the
* attribute.
-
DELETE
- Nothing happens,
* because attributes cannot be deleted from a nonexistent item. The
* operation succeeds, but DynamoDB does not create a new item.
* -
ADD
- Causes DynamoDB to create an item with the
* supplied primary key and number (or set of numbers) for the attribute
* value. The only data types allowed are Number and Number Set.
*
If you provide any attributes that are part of an
* index key, then the data types for those attributes must match those
* of the schema in the table's attribute definition.
* @param returnValues Use ReturnValues if you want to get the
* item attributes as they appeared either before or after they were
* updated. For UpdateItem, the valid values are:
-
*
NONE
- If ReturnValues is not specified, or if
* its value is NONE
, then nothing is returned. (This
* setting is the default for ReturnValues.)
-
*
ALL_OLD
- If UpdateItem overwrote an attribute
* name-value pair, then the content of the old item is returned.
* -
UPDATED_OLD
- The old versions of only the
* updated attributes are returned.
-
ALL_NEW
-
* All of the attributes of the new version of the item are returned.
*
-
UPDATED_NEW
- The new versions of only the
* updated attributes are returned.
There is no additional
* cost associated with requesting a return value aside from the small
* network and processing overhead of receiving a larger response. No
* Read Capacity Units are consumed.
Values returned are strongly
* consistent
*/
public UpdateItemRequest(String tableName, java.util.Map key, java.util.Map attributeUpdates, String returnValues) {
setTableName(tableName);
setKey(key);
setAttributeUpdates(attributeUpdates);
setReturnValues(returnValues);
}
/**
* Constructs a new UpdateItemRequest object.
* Callers should use the setter or fluent setter (with...) methods to
* initialize any additional object members.
*
* @param tableName The name of the table containing the item to update.
* @param key The primary key of the item to be updated. Each element
* consists of an attribute name and a value for that attribute. For
* the primary key, you must provide all of the attributes. For example,
* with a simple primary key, you only need to provide a value for the
* partition key. For a composite primary key, you must provide values
* for both the partition key and the sort key.
* @param attributeUpdates This is a legacy parameter, for
* backward compatibility. New applications should use
* UpdateExpression instead. Do not combine legacy parameters and
* expression parameters in a single API call; otherwise, DynamoDB will
* return a ValidationException exception.
This parameter can
* be used for modifying top-level attributes; however, it does not
* support individual list or map elements.
The names of
* attributes to be modified, the action to perform on each, and the new
* value for each. If you are updating an attribute that is an index key
* attribute for any indexes on that table, the attribute type must match
* the index key type defined in the AttributesDefinition of the
* table description. You can use UpdateItem to update any non-key
* attributes.
Attribute values cannot be null. String and Binary type
* attributes must have lengths greater than zero. Set type attributes
* must not be empty. Requests with empty values will be rejected with a
* ValidationException exception.
Each AttributeUpdates
* element consists of an attribute name to modify, along with the
* following:
-
Value - The new value, if applicable,
* for this attribute.
-
Action - A value that
* specifies how to perform the update. This action is only valid for an
* existing attribute whose data type is Number or is a set; do not use
* ADD
for other data types.
If an item with the
* specified primary key is found in the table, the following values
* perform the following actions:
-
PUT
- Adds
* the specified attribute to the item. If the attribute already exists,
* it is replaced by the new value.
-
DELETE
-
* Removes the attribute and its value, if no value is specified for
* DELETE
. The data type of the specified value must match
* the existing value's data type.
If a set of values is specified,
* then those values are subtracted from the old set. For example, if the
* attribute value was the set [a,b,c]
and the
* DELETE
action specifies [a,c]
, then the
* final attribute value is [b]
. Specifying an empty set is
* an error.
-
ADD
- Adds the specified value to
* the item, if the attribute does not already exist. If the attribute
* does exist, then the behavior of ADD
depends on the data
* type of the attribute:
-
If the existing attribute is a
* number, and if Value is also a number, then Value is
* mathematically added to the existing attribute. If Value is a
* negative number, then it is subtracted from the existing attribute.
* If you use ADD
to increment or decrement a
* number value for an item that doesn't exist before the update,
* DynamoDB uses 0 as the initial value.
Similarly, if you use
* ADD
for an existing item to increment or decrement an
* attribute value that doesn't exist before the update, DynamoDB uses
* 0
as the initial value. For example, suppose that the
* item you want to update doesn't have an attribute named
* itemcount, but you decide to ADD
the number
* 3
to this attribute anyway. DynamoDB will create the
* itemcount attribute, set its initial value to 0
,
* and finally add 3
to it. The result will be a new
* itemcount attribute, with a value of 3
.
*
-
If the existing data type is a set, and if Value
* is also a set, then Value is appended to the existing set. For
* example, if the attribute value is the set [1,2]
, and the
* ADD
action specified [3]
, then the final
* attribute value is [1,2,3]
. An error occurs if an
* ADD
action is specified for a set attribute and the
* attribute type specified does not match the existing set type.
Both
* sets must have the same primitive data type. For example, if the
* existing data type is a set of strings, Value must also be a
* set of strings.
If no item with the
* specified key is found in the table, the following values perform the
* following actions:
-
PUT
- Causes DynamoDB to
* create a new item with the specified primary key, and then adds the
* attribute.
-
DELETE
- Nothing happens,
* because attributes cannot be deleted from a nonexistent item. The
* operation succeeds, but DynamoDB does not create a new item.
* -
ADD
- Causes DynamoDB to create an item with the
* supplied primary key and number (or set of numbers) for the attribute
* value. The only data types allowed are Number and Number Set.
*
If you provide any attributes that are part of an
* index key, then the data types for those attributes must match those
* of the schema in the table's attribute definition.
* @param returnValues Use ReturnValues if you want to get the
* item attributes as they appeared either before or after they were
* updated. For UpdateItem, the valid values are:
-
*
NONE
- If ReturnValues is not specified, or if
* its value is NONE
, then nothing is returned. (This
* setting is the default for ReturnValues.)
-
*
ALL_OLD
- If UpdateItem overwrote an attribute
* name-value pair, then the content of the old item is returned.
* -
UPDATED_OLD
- The old versions of only the
* updated attributes are returned.
-
ALL_NEW
-
* All of the attributes of the new version of the item are returned.
*
-
UPDATED_NEW
- The new versions of only the
* updated attributes are returned.
There is no additional
* cost associated with requesting a return value aside from the small
* network and processing overhead of receiving a larger response. No
* Read Capacity Units are consumed.
Values returned are strongly
* consistent
*/
public UpdateItemRequest(String tableName, java.util.Map key, java.util.Map attributeUpdates, ReturnValue returnValues) {
this.tableName = tableName;
this.key = key;
this.attributeUpdates = attributeUpdates;
this.returnValues = returnValues.toString();
}
/**
* The name of the table containing the item to update.
*
* Constraints:
* Length: 3 - 255
* Pattern: [a-zA-Z0-9_.-]+
*
* @return The name of the table containing the item to update.
*/
public String getTableName() {
return tableName;
}
/**
* The name of the table containing the item to update.
*
* Constraints:
* Length: 3 - 255
* Pattern: [a-zA-Z0-9_.-]+
*
* @param tableName The name of the table containing the item to update.
*/
public void setTableName(String tableName) {
this.tableName = tableName;
}
/**
* The name of the table containing the item to update.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 3 - 255
* Pattern: [a-zA-Z0-9_.-]+
*
* @param tableName The name of the table containing the item to update.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public UpdateItemRequest withTableName(String tableName) {
this.tableName = tableName;
return this;
}
/**
* The primary key of the item to be updated. Each element consists of an
* attribute name and a value for that attribute.
For the primary key,
* you must provide all of the attributes. For example, with a simple
* primary key, you only need to provide a value for the partition key.
* For a composite primary key, you must provide values for both the
* partition key and the sort key.
*
* @return The primary key of the item to be updated. Each element consists of an
* attribute name and a value for that attribute.
For the primary key,
* you must provide all of the attributes. For example, with a simple
* primary key, you only need to provide a value for the partition key.
* For a composite primary key, you must provide values for both the
* partition key and the sort key.
*/
public java.util.Map getKey() {
return key;
}
/**
* The primary key of the item to be updated. Each element consists of an
* attribute name and a value for that attribute. For the primary key,
* you must provide all of the attributes. For example, with a simple
* primary key, you only need to provide a value for the partition key.
* For a composite primary key, you must provide values for both the
* partition key and the sort key.
*
* @param key The primary key of the item to be updated. Each element consists of an
* attribute name and a value for that attribute.
For the primary key,
* you must provide all of the attributes. For example, with a simple
* primary key, you only need to provide a value for the partition key.
* For a composite primary key, you must provide values for both the
* partition key and the sort key.
*/
public void setKey(java.util.Map key) {
this.key = key;
}
/**
* The primary key of the item to be updated. Each element consists of an
* attribute name and a value for that attribute. For the primary key,
* you must provide all of the attributes. For example, with a simple
* primary key, you only need to provide a value for the partition key.
* For a composite primary key, you must provide values for both the
* partition key and the sort key.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param key The primary key of the item to be updated. Each element consists of an
* attribute name and a value for that attribute.
For the primary key,
* you must provide all of the attributes. For example, with a simple
* primary key, you only need to provide a value for the partition key.
* For a composite primary key, you must provide values for both the
* partition key and the sort key.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public UpdateItemRequest withKey(java.util.Map key) {
setKey(key);
return this;
}
/**
* The primary key of the item to be updated. Each element consists of an
* attribute name and a value for that attribute. For the primary key,
* you must provide all of the attributes. For example, with a simple
* primary key, you only need to provide a value for the partition key.
* For a composite primary key, you must provide values for both the
* partition key and the sort key.
*
* This method accepts the hashKey, rangeKey of Key as
* java.util.Map.Entry objects.
*
* @param hashKey Primary hash key.
* @param rangeKey Primary range key. (null if it a hash-only table)
*/
public void setKey(java.util.Map.Entry hashKey, java.util.Map.Entry rangeKey) throws IllegalArgumentException {
java.util.HashMap key = new java.util.HashMap();
if (hashKey != null) {
key.put(hashKey.getKey(), hashKey.getValue());
} else
throw new IllegalArgumentException("hashKey must be non-null object.");
if (rangeKey != null) {
key.put(rangeKey.getKey(), rangeKey.getValue());
}
setKey(key);
}
/**
* The primary key of the item to be updated. Each element consists of an
* attribute name and a value for that attribute. For the primary key,
* you must provide all of the attributes. For example, with a simple
* primary key, you only need to provide a value for the partition key.
* For a composite primary key, you must provide values for both the
* partition key and the sort key.
*
* This method accepts the hashKey, rangeKey of Key as
* java.util.Map.Entry objects.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param hashKey Primary hash key.
* @param rangeKey Primary range key. (null if it a hash-only table)
*/
public UpdateItemRequest withKey(java.util.Map.Entry hashKey, java.util.Map.Entry rangeKey) throws IllegalArgumentException {
setKey(hashKey, rangeKey);
return this;
}
/**
* The primary key of the item to be updated. Each element consists of an
* attribute name and a value for that attribute. For the primary key,
* you must provide all of the attributes. For example, with a simple
* primary key, you only need to provide a value for the partition key.
* For a composite primary key, you must provide values for both the
* partition key and the sort key.
*
* The method adds a new key-value pair into Key parameter, and returns a
* reference to this object so that method calls can be chained together.
*
* @param key The key of the entry to be added into Key.
* @param value The corresponding value of the entry to be added into Key.
*/
public UpdateItemRequest addKeyEntry(String key, AttributeValue value) {
if (null == this.key) {
this.key = new java.util.HashMap();
}
if (this.key.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.key.put(key, value);
return this;
}
/**
* Removes all the entries added into Key.
*
* Returns a reference to this object so that method calls can be chained together.
*/
public UpdateItemRequest clearKeyEntries() {
this.key = null;
return this;
}
/**
* This is a legacy parameter, for backward compatibility.
* New applications should use UpdateExpression instead. Do not
* combine legacy parameters and expression parameters in a single API
* call; otherwise, DynamoDB will return a ValidationException
* exception.
This parameter can be used for modifying top-level
* attributes; however, it does not support individual list or map
* elements.
The names of attributes to be modified, the
* action to perform on each, and the new value for each. If you are
* updating an attribute that is an index key attribute for any indexes
* on that table, the attribute type must match the index key type
* defined in the AttributesDefinition of the table description.
* You can use UpdateItem to update any non-key attributes.
*
Attribute values cannot be null. String and Binary type attributes
* must have lengths greater than zero. Set type attributes must not be
* empty. Requests with empty values will be rejected with a
* ValidationException exception.
Each AttributeUpdates
* element consists of an attribute name to modify, along with the
* following:
-
Value - The new value, if applicable,
* for this attribute.
-
Action - A value that
* specifies how to perform the update. This action is only valid for an
* existing attribute whose data type is Number or is a set; do not use
* ADD
for other data types.
If an item with the
* specified primary key is found in the table, the following values
* perform the following actions:
-
PUT
- Adds
* the specified attribute to the item. If the attribute already exists,
* it is replaced by the new value.
-
DELETE
-
* Removes the attribute and its value, if no value is specified for
* DELETE
. The data type of the specified value must match
* the existing value's data type.
If a set of values is specified,
* then those values are subtracted from the old set. For example, if the
* attribute value was the set [a,b,c]
and the
* DELETE
action specifies [a,c]
, then the
* final attribute value is [b]
. Specifying an empty set is
* an error.
-
ADD
- Adds the specified value to
* the item, if the attribute does not already exist. If the attribute
* does exist, then the behavior of ADD
depends on the data
* type of the attribute:
-
If the existing attribute is a
* number, and if Value is also a number, then Value is
* mathematically added to the existing attribute. If Value is a
* negative number, then it is subtracted from the existing attribute.
* If you use ADD
to increment or decrement a
* number value for an item that doesn't exist before the update,
* DynamoDB uses 0 as the initial value.
Similarly, if you use
* ADD
for an existing item to increment or decrement an
* attribute value that doesn't exist before the update, DynamoDB uses
* 0
as the initial value. For example, suppose that the
* item you want to update doesn't have an attribute named
* itemcount, but you decide to ADD
the number
* 3
to this attribute anyway. DynamoDB will create the
* itemcount attribute, set its initial value to 0
,
* and finally add 3
to it. The result will be a new
* itemcount attribute, with a value of 3
.
*
-
If the existing data type is a set, and if Value
* is also a set, then Value is appended to the existing set. For
* example, if the attribute value is the set [1,2]
, and the
* ADD
action specified [3]
, then the final
* attribute value is [1,2,3]
. An error occurs if an
* ADD
action is specified for a set attribute and the
* attribute type specified does not match the existing set type.
Both
* sets must have the same primitive data type. For example, if the
* existing data type is a set of strings, Value must also be a
* set of strings.
If no item with the
* specified key is found in the table, the following values perform the
* following actions:
-
PUT
- Causes DynamoDB to
* create a new item with the specified primary key, and then adds the
* attribute.
-
DELETE
- Nothing happens,
* because attributes cannot be deleted from a nonexistent item. The
* operation succeeds, but DynamoDB does not create a new item.
* -
ADD
- Causes DynamoDB to create an item with the
* supplied primary key and number (or set of numbers) for the attribute
* value. The only data types allowed are Number and Number Set.
*
If you provide any attributes that are part of an
* index key, then the data types for those attributes must match those
* of the schema in the table's attribute definition.
*
* @return This is a legacy parameter, for backward compatibility.
* New applications should use UpdateExpression instead. Do not
* combine legacy parameters and expression parameters in a single API
* call; otherwise, DynamoDB will return a ValidationException
* exception.
This parameter can be used for modifying top-level
* attributes; however, it does not support individual list or map
* elements.
The names of attributes to be modified, the
* action to perform on each, and the new value for each. If you are
* updating an attribute that is an index key attribute for any indexes
* on that table, the attribute type must match the index key type
* defined in the AttributesDefinition of the table description.
* You can use UpdateItem to update any non-key attributes.
*
Attribute values cannot be null. String and Binary type attributes
* must have lengths greater than zero. Set type attributes must not be
* empty. Requests with empty values will be rejected with a
* ValidationException exception.
Each AttributeUpdates
* element consists of an attribute name to modify, along with the
* following:
-
Value - The new value, if applicable,
* for this attribute.
-
Action - A value that
* specifies how to perform the update. This action is only valid for an
* existing attribute whose data type is Number or is a set; do not use
* ADD
for other data types.
If an item with the
* specified primary key is found in the table, the following values
* perform the following actions:
-
PUT
- Adds
* the specified attribute to the item. If the attribute already exists,
* it is replaced by the new value.
-
DELETE
-
* Removes the attribute and its value, if no value is specified for
* DELETE
. The data type of the specified value must match
* the existing value's data type.
If a set of values is specified,
* then those values are subtracted from the old set. For example, if the
* attribute value was the set [a,b,c]
and the
* DELETE
action specifies [a,c]
, then the
* final attribute value is [b]
. Specifying an empty set is
* an error.
-
ADD
- Adds the specified value to
* the item, if the attribute does not already exist. If the attribute
* does exist, then the behavior of ADD
depends on the data
* type of the attribute:
-
If the existing attribute is a
* number, and if Value is also a number, then Value is
* mathematically added to the existing attribute. If Value is a
* negative number, then it is subtracted from the existing attribute.
* If you use ADD
to increment or decrement a
* number value for an item that doesn't exist before the update,
* DynamoDB uses 0 as the initial value.
Similarly, if you use
* ADD
for an existing item to increment or decrement an
* attribute value that doesn't exist before the update, DynamoDB uses
* 0
as the initial value. For example, suppose that the
* item you want to update doesn't have an attribute named
* itemcount, but you decide to ADD
the number
* 3
to this attribute anyway. DynamoDB will create the
* itemcount attribute, set its initial value to 0
,
* and finally add 3
to it. The result will be a new
* itemcount attribute, with a value of 3
.
*
-
If the existing data type is a set, and if Value
* is also a set, then Value is appended to the existing set. For
* example, if the attribute value is the set [1,2]
, and the
* ADD
action specified [3]
, then the final
* attribute value is [1,2,3]
. An error occurs if an
* ADD
action is specified for a set attribute and the
* attribute type specified does not match the existing set type.
Both
* sets must have the same primitive data type. For example, if the
* existing data type is a set of strings, Value must also be a
* set of strings.
If no item with the
* specified key is found in the table, the following values perform the
* following actions:
-
PUT
- Causes DynamoDB to
* create a new item with the specified primary key, and then adds the
* attribute.
-
DELETE
- Nothing happens,
* because attributes cannot be deleted from a nonexistent item. The
* operation succeeds, but DynamoDB does not create a new item.
* -
ADD
- Causes DynamoDB to create an item with the
* supplied primary key and number (or set of numbers) for the attribute
* value. The only data types allowed are Number and Number Set.
*
If you provide any attributes that are part of an
* index key, then the data types for those attributes must match those
* of the schema in the table's attribute definition.
*/
public java.util.Map getAttributeUpdates() {
return attributeUpdates;
}
/**
* This is a legacy parameter, for backward compatibility.
* New applications should use UpdateExpression instead. Do not
* combine legacy parameters and expression parameters in a single API
* call; otherwise, DynamoDB will return a ValidationException
* exception.
This parameter can be used for modifying top-level
* attributes; however, it does not support individual list or map
* elements.
The names of attributes to be modified, the
* action to perform on each, and the new value for each. If you are
* updating an attribute that is an index key attribute for any indexes
* on that table, the attribute type must match the index key type
* defined in the AttributesDefinition of the table description.
* You can use UpdateItem to update any non-key attributes.
*
Attribute values cannot be null. String and Binary type attributes
* must have lengths greater than zero. Set type attributes must not be
* empty. Requests with empty values will be rejected with a
* ValidationException exception.
Each AttributeUpdates
* element consists of an attribute name to modify, along with the
* following:
-
Value - The new value, if applicable,
* for this attribute.
-
Action - A value that
* specifies how to perform the update. This action is only valid for an
* existing attribute whose data type is Number or is a set; do not use
* ADD
for other data types.
If an item with the
* specified primary key is found in the table, the following values
* perform the following actions:
-
PUT
- Adds
* the specified attribute to the item. If the attribute already exists,
* it is replaced by the new value.
-
DELETE
-
* Removes the attribute and its value, if no value is specified for
* DELETE
. The data type of the specified value must match
* the existing value's data type.
If a set of values is specified,
* then those values are subtracted from the old set. For example, if the
* attribute value was the set [a,b,c]
and the
* DELETE
action specifies [a,c]
, then the
* final attribute value is [b]
. Specifying an empty set is
* an error.
-
ADD
- Adds the specified value to
* the item, if the attribute does not already exist. If the attribute
* does exist, then the behavior of ADD
depends on the data
* type of the attribute:
-
If the existing attribute is a
* number, and if Value is also a number, then Value is
* mathematically added to the existing attribute. If Value is a
* negative number, then it is subtracted from the existing attribute.
* If you use ADD
to increment or decrement a
* number value for an item that doesn't exist before the update,
* DynamoDB uses 0 as the initial value.
Similarly, if you use
* ADD
for an existing item to increment or decrement an
* attribute value that doesn't exist before the update, DynamoDB uses
* 0
as the initial value. For example, suppose that the
* item you want to update doesn't have an attribute named
* itemcount, but you decide to ADD
the number
* 3
to this attribute anyway. DynamoDB will create the
* itemcount attribute, set its initial value to 0
,
* and finally add 3
to it. The result will be a new
* itemcount attribute, with a value of 3
.
*
-
If the existing data type is a set, and if Value
* is also a set, then Value is appended to the existing set. For
* example, if the attribute value is the set [1,2]
, and the
* ADD
action specified [3]
, then the final
* attribute value is [1,2,3]
. An error occurs if an
* ADD
action is specified for a set attribute and the
* attribute type specified does not match the existing set type.
Both
* sets must have the same primitive data type. For example, if the
* existing data type is a set of strings, Value must also be a
* set of strings.
If no item with the
* specified key is found in the table, the following values perform the
* following actions:
-
PUT
- Causes DynamoDB to
* create a new item with the specified primary key, and then adds the
* attribute.
-
DELETE
- Nothing happens,
* because attributes cannot be deleted from a nonexistent item. The
* operation succeeds, but DynamoDB does not create a new item.
* -
ADD
- Causes DynamoDB to create an item with the
* supplied primary key and number (or set of numbers) for the attribute
* value. The only data types allowed are Number and Number Set.
*
If you provide any attributes that are part of an
* index key, then the data types for those attributes must match those
* of the schema in the table's attribute definition.
*
* @param attributeUpdates This is a legacy parameter, for backward compatibility.
* New applications should use UpdateExpression instead. Do not
* combine legacy parameters and expression parameters in a single API
* call; otherwise, DynamoDB will return a ValidationException
* exception.
This parameter can be used for modifying top-level
* attributes; however, it does not support individual list or map
* elements.
The names of attributes to be modified, the
* action to perform on each, and the new value for each. If you are
* updating an attribute that is an index key attribute for any indexes
* on that table, the attribute type must match the index key type
* defined in the AttributesDefinition of the table description.
* You can use UpdateItem to update any non-key attributes.
*
Attribute values cannot be null. String and Binary type attributes
* must have lengths greater than zero. Set type attributes must not be
* empty. Requests with empty values will be rejected with a
* ValidationException exception.
Each AttributeUpdates
* element consists of an attribute name to modify, along with the
* following:
-
Value - The new value, if applicable,
* for this attribute.
-
Action - A value that
* specifies how to perform the update. This action is only valid for an
* existing attribute whose data type is Number or is a set; do not use
* ADD
for other data types.
If an item with the
* specified primary key is found in the table, the following values
* perform the following actions:
-
PUT
- Adds
* the specified attribute to the item. If the attribute already exists,
* it is replaced by the new value.
-
DELETE
-
* Removes the attribute and its value, if no value is specified for
* DELETE
. The data type of the specified value must match
* the existing value's data type.
If a set of values is specified,
* then those values are subtracted from the old set. For example, if the
* attribute value was the set [a,b,c]
and the
* DELETE
action specifies [a,c]
, then the
* final attribute value is [b]
. Specifying an empty set is
* an error.
-
ADD
- Adds the specified value to
* the item, if the attribute does not already exist. If the attribute
* does exist, then the behavior of ADD
depends on the data
* type of the attribute:
-
If the existing attribute is a
* number, and if Value is also a number, then Value is
* mathematically added to the existing attribute. If Value is a
* negative number, then it is subtracted from the existing attribute.
* If you use ADD
to increment or decrement a
* number value for an item that doesn't exist before the update,
* DynamoDB uses 0 as the initial value.
Similarly, if you use
* ADD
for an existing item to increment or decrement an
* attribute value that doesn't exist before the update, DynamoDB uses
* 0
as the initial value. For example, suppose that the
* item you want to update doesn't have an attribute named
* itemcount, but you decide to ADD
the number
* 3
to this attribute anyway. DynamoDB will create the
* itemcount attribute, set its initial value to 0
,
* and finally add 3
to it. The result will be a new
* itemcount attribute, with a value of 3
.
*
-
If the existing data type is a set, and if Value
* is also a set, then Value is appended to the existing set. For
* example, if the attribute value is the set [1,2]
, and the
* ADD
action specified [3]
, then the final
* attribute value is [1,2,3]
. An error occurs if an
* ADD
action is specified for a set attribute and the
* attribute type specified does not match the existing set type.
Both
* sets must have the same primitive data type. For example, if the
* existing data type is a set of strings, Value must also be a
* set of strings.
If no item with the
* specified key is found in the table, the following values perform the
* following actions:
-
PUT
- Causes DynamoDB to
* create a new item with the specified primary key, and then adds the
* attribute.
-
DELETE
- Nothing happens,
* because attributes cannot be deleted from a nonexistent item. The
* operation succeeds, but DynamoDB does not create a new item.
* -
ADD
- Causes DynamoDB to create an item with the
* supplied primary key and number (or set of numbers) for the attribute
* value. The only data types allowed are Number and Number Set.
*
If you provide any attributes that are part of an
* index key, then the data types for those attributes must match those
* of the schema in the table's attribute definition.
*/
public void setAttributeUpdates(java.util.Map attributeUpdates) {
this.attributeUpdates = attributeUpdates;
}
/**
* This is a legacy parameter, for backward compatibility.
* New applications should use UpdateExpression instead. Do not
* combine legacy parameters and expression parameters in a single API
* call; otherwise, DynamoDB will return a ValidationException
* exception.
This parameter can be used for modifying top-level
* attributes; however, it does not support individual list or map
* elements.
The names of attributes to be modified, the
* action to perform on each, and the new value for each. If you are
* updating an attribute that is an index key attribute for any indexes
* on that table, the attribute type must match the index key type
* defined in the AttributesDefinition of the table description.
* You can use UpdateItem to update any non-key attributes.
*
Attribute values cannot be null. String and Binary type attributes
* must have lengths greater than zero. Set type attributes must not be
* empty. Requests with empty values will be rejected with a
* ValidationException exception.
Each AttributeUpdates
* element consists of an attribute name to modify, along with the
* following:
-
Value - The new value, if applicable,
* for this attribute.
-
Action - A value that
* specifies how to perform the update. This action is only valid for an
* existing attribute whose data type is Number or is a set; do not use
* ADD
for other data types.
If an item with the
* specified primary key is found in the table, the following values
* perform the following actions:
-
PUT
- Adds
* the specified attribute to the item. If the attribute already exists,
* it is replaced by the new value.
-
DELETE
-
* Removes the attribute and its value, if no value is specified for
* DELETE
. The data type of the specified value must match
* the existing value's data type.
If a set of values is specified,
* then those values are subtracted from the old set. For example, if the
* attribute value was the set [a,b,c]
and the
* DELETE
action specifies [a,c]
, then the
* final attribute value is [b]
. Specifying an empty set is
* an error.
-
ADD
- Adds the specified value to
* the item, if the attribute does not already exist. If the attribute
* does exist, then the behavior of ADD
depends on the data
* type of the attribute:
-
If the existing attribute is a
* number, and if Value is also a number, then Value is
* mathematically added to the existing attribute. If Value is a
* negative number, then it is subtracted from the existing attribute.
* If you use ADD
to increment or decrement a
* number value for an item that doesn't exist before the update,
* DynamoDB uses 0 as the initial value.
Similarly, if you use
* ADD
for an existing item to increment or decrement an
* attribute value that doesn't exist before the update, DynamoDB uses
* 0
as the initial value. For example, suppose that the
* item you want to update doesn't have an attribute named
* itemcount, but you decide to ADD
the number
* 3
to this attribute anyway. DynamoDB will create the
* itemcount attribute, set its initial value to 0
,
* and finally add 3
to it. The result will be a new
* itemcount attribute, with a value of 3
.
*
-
If the existing data type is a set, and if Value
* is also a set, then Value is appended to the existing set. For
* example, if the attribute value is the set [1,2]
, and the
* ADD
action specified [3]
, then the final
* attribute value is [1,2,3]
. An error occurs if an
* ADD
action is specified for a set attribute and the
* attribute type specified does not match the existing set type.
Both
* sets must have the same primitive data type. For example, if the
* existing data type is a set of strings, Value must also be a
* set of strings.
If no item with the
* specified key is found in the table, the following values perform the
* following actions:
-
PUT
- Causes DynamoDB to
* create a new item with the specified primary key, and then adds the
* attribute.
-
DELETE
- Nothing happens,
* because attributes cannot be deleted from a nonexistent item. The
* operation succeeds, but DynamoDB does not create a new item.
* -
ADD
- Causes DynamoDB to create an item with the
* supplied primary key and number (or set of numbers) for the attribute
* value. The only data types allowed are Number and Number Set.
*
If you provide any attributes that are part of an
* index key, then the data types for those attributes must match those
* of the schema in the table's attribute definition.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param attributeUpdates This is a legacy parameter, for backward compatibility.
* New applications should use UpdateExpression instead. Do not
* combine legacy parameters and expression parameters in a single API
* call; otherwise, DynamoDB will return a ValidationException
* exception.
This parameter can be used for modifying top-level
* attributes; however, it does not support individual list or map
* elements.
The names of attributes to be modified, the
* action to perform on each, and the new value for each. If you are
* updating an attribute that is an index key attribute for any indexes
* on that table, the attribute type must match the index key type
* defined in the AttributesDefinition of the table description.
* You can use UpdateItem to update any non-key attributes.
*
Attribute values cannot be null. String and Binary type attributes
* must have lengths greater than zero. Set type attributes must not be
* empty. Requests with empty values will be rejected with a
* ValidationException exception.
Each AttributeUpdates
* element consists of an attribute name to modify, along with the
* following:
-
Value - The new value, if applicable,
* for this attribute.
-
Action - A value that
* specifies how to perform the update. This action is only valid for an
* existing attribute whose data type is Number or is a set; do not use
* ADD
for other data types.
If an item with the
* specified primary key is found in the table, the following values
* perform the following actions:
-
PUT
- Adds
* the specified attribute to the item. If the attribute already exists,
* it is replaced by the new value.
-
DELETE
-
* Removes the attribute and its value, if no value is specified for
* DELETE
. The data type of the specified value must match
* the existing value's data type.
If a set of values is specified,
* then those values are subtracted from the old set. For example, if the
* attribute value was the set [a,b,c]
and the
* DELETE
action specifies [a,c]
, then the
* final attribute value is [b]
. Specifying an empty set is
* an error.
-
ADD
- Adds the specified value to
* the item, if the attribute does not already exist. If the attribute
* does exist, then the behavior of ADD
depends on the data
* type of the attribute:
-
If the existing attribute is a
* number, and if Value is also a number, then Value is
* mathematically added to the existing attribute. If Value is a
* negative number, then it is subtracted from the existing attribute.
* If you use ADD
to increment or decrement a
* number value for an item that doesn't exist before the update,
* DynamoDB uses 0 as the initial value.
Similarly, if you use
* ADD
for an existing item to increment or decrement an
* attribute value that doesn't exist before the update, DynamoDB uses
* 0
as the initial value. For example, suppose that the
* item you want to update doesn't have an attribute named
* itemcount, but you decide to ADD
the number
* 3
to this attribute anyway. DynamoDB will create the
* itemcount attribute, set its initial value to 0
,
* and finally add 3
to it. The result will be a new
* itemcount attribute, with a value of 3
.
*
-
If the existing data type is a set, and if Value
* is also a set, then Value is appended to the existing set. For
* example, if the attribute value is the set [1,2]
, and the
* ADD
action specified [3]
, then the final
* attribute value is [1,2,3]
. An error occurs if an
* ADD
action is specified for a set attribute and the
* attribute type specified does not match the existing set type.
Both
* sets must have the same primitive data type. For example, if the
* existing data type is a set of strings, Value must also be a
* set of strings.
If no item with the
* specified key is found in the table, the following values perform the
* following actions:
-
PUT
- Causes DynamoDB to
* create a new item with the specified primary key, and then adds the
* attribute.
-
DELETE
- Nothing happens,
* because attributes cannot be deleted from a nonexistent item. The
* operation succeeds, but DynamoDB does not create a new item.
* -
ADD
- Causes DynamoDB to create an item with the
* supplied primary key and number (or set of numbers) for the attribute
* value. The only data types allowed are Number and Number Set.
*
If you provide any attributes that are part of an
* index key, then the data types for those attributes must match those
* of the schema in the table's attribute definition.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public UpdateItemRequest withAttributeUpdates(java.util.Map attributeUpdates) {
setAttributeUpdates(attributeUpdates);
return this;
}
/**
* This is a legacy parameter, for backward compatibility.
* New applications should use UpdateExpression instead. Do not
* combine legacy parameters and expression parameters in a single API
* call; otherwise, DynamoDB will return a ValidationException
* exception.
This parameter can be used for modifying top-level
* attributes; however, it does not support individual list or map
* elements.
The names of attributes to be modified, the
* action to perform on each, and the new value for each. If you are
* updating an attribute that is an index key attribute for any indexes
* on that table, the attribute type must match the index key type
* defined in the AttributesDefinition of the table description.
* You can use UpdateItem to update any non-key attributes.
*
Attribute values cannot be null. String and Binary type attributes
* must have lengths greater than zero. Set type attributes must not be
* empty. Requests with empty values will be rejected with a
* ValidationException exception.
Each AttributeUpdates
* element consists of an attribute name to modify, along with the
* following:
-
Value - The new value, if applicable,
* for this attribute.
-
Action - A value that
* specifies how to perform the update. This action is only valid for an
* existing attribute whose data type is Number or is a set; do not use
* ADD
for other data types.
If an item with the
* specified primary key is found in the table, the following values
* perform the following actions:
-
PUT
- Adds
* the specified attribute to the item. If the attribute already exists,
* it is replaced by the new value.
-
DELETE
-
* Removes the attribute and its value, if no value is specified for
* DELETE
. The data type of the specified value must match
* the existing value's data type.
If a set of values is specified,
* then those values are subtracted from the old set. For example, if the
* attribute value was the set [a,b,c]
and the
* DELETE
action specifies [a,c]
, then the
* final attribute value is [b]
. Specifying an empty set is
* an error.
-
ADD
- Adds the specified value to
* the item, if the attribute does not already exist. If the attribute
* does exist, then the behavior of ADD
depends on the data
* type of the attribute:
-
If the existing attribute is a
* number, and if Value is also a number, then Value is
* mathematically added to the existing attribute. If Value is a
* negative number, then it is subtracted from the existing attribute.
* If you use ADD
to increment or decrement a
* number value for an item that doesn't exist before the update,
* DynamoDB uses 0 as the initial value.
Similarly, if you use
* ADD
for an existing item to increment or decrement an
* attribute value that doesn't exist before the update, DynamoDB uses
* 0
as the initial value. For example, suppose that the
* item you want to update doesn't have an attribute named
* itemcount, but you decide to ADD
the number
* 3
to this attribute anyway. DynamoDB will create the
* itemcount attribute, set its initial value to 0
,
* and finally add 3
to it. The result will be a new
* itemcount attribute, with a value of 3
.
*
-
If the existing data type is a set, and if Value
* is also a set, then Value is appended to the existing set. For
* example, if the attribute value is the set [1,2]
, and the
* ADD
action specified [3]
, then the final
* attribute value is [1,2,3]
. An error occurs if an
* ADD
action is specified for a set attribute and the
* attribute type specified does not match the existing set type.
Both
* sets must have the same primitive data type. For example, if the
* existing data type is a set of strings, Value must also be a
* set of strings.
If no item with the
* specified key is found in the table, the following values perform the
* following actions:
-
PUT
- Causes DynamoDB to
* create a new item with the specified primary key, and then adds the
* attribute.
-
DELETE
- Nothing happens,
* because attributes cannot be deleted from a nonexistent item. The
* operation succeeds, but DynamoDB does not create a new item.
* -
ADD
- Causes DynamoDB to create an item with the
* supplied primary key and number (or set of numbers) for the attribute
* value. The only data types allowed are Number and Number Set.
*
If you provide any attributes that are part of an
* index key, then the data types for those attributes must match those
* of the schema in the table's attribute definition.
*
* The method adds a new key-value pair into AttributeUpdates parameter,
* and returns a reference to this object so that method calls can be
* chained together.
*
* @param key The key of the entry to be added into AttributeUpdates.
* @param value The corresponding value of the entry to be added into AttributeUpdates.
*/
public UpdateItemRequest addAttributeUpdatesEntry(String key, AttributeValueUpdate value) {
if (null == this.attributeUpdates) {
this.attributeUpdates = new java.util.HashMap();
}
if (this.attributeUpdates.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.attributeUpdates.put(key, value);
return this;
}
/**
* Removes all the entries added into AttributeUpdates.
*
* Returns a reference to this object so that method calls can be chained together.
*/
public UpdateItemRequest clearAttributeUpdatesEntries() {
this.attributeUpdates = null;
return this;
}
/**
* This is a legacy parameter, for backward compatibility.
* New applications should use ConditionExpression instead. Do
* not combine legacy parameters and expression parameters in a single
* API call; otherwise, DynamoDB will return a ValidationException
* exception.
A map of attribute/condition pairs.
* Expected provides a conditional block for the UpdateItem
* operation.
Each element of Expected consists of an attribute
* name, a comparison operator, and one or more values. DynamoDB compares
* the attribute with the value(s) you supplied, using the comparison
* operator. For each Expected element, the result of the
* evaluation is either true or false.
If you specify more than one
* element in the Expected map, then by default all of the
* conditions must evaluate to true. In other words, the conditions are
* ANDed together. (You can use the ConditionalOperator parameter
* to OR the conditions instead. If you do this, then at least one of the
* conditions must evaluate to true, rather than all of them.)
If the
* Expected map evaluates to true, then the conditional operation
* succeeds; otherwise, it fails.
Expected contains the
* following:
-
AttributeValueList - One or more values
* to evaluate against the supplied attribute. The number of values in
* the list depends on the ComparisonOperator being used.
For
* type Number, value comparisons are numeric.
String value
* comparisons for greater than, equals, or less than are based on ASCII
* character code values. For example, a
is greater than
* A
, and a
is greater than B
. For
* a list of code values, see http://en.wikipedia.org/wiki/ASCII#ASCII_printable_characters.
*
For type Binary, DynamoDB treats each byte of the binary data as
* unsigned when it compares binary values.
-
*
ComparisonOperator - A comparator for evaluating attributes
* in the AttributeValueList. When performing the comparison,
* DynamoDB uses strongly consistent reads.
The following comparison
* operators are available:
EQ | NE | LE | LT | GE | GT |
* NOT_NULL | NULL | CONTAINS | NOT_CONTAINS | BEGINS_WITH | IN |
* BETWEEN
The following are descriptions of each comparison
* operator.
-
EQ
: Equal. EQ
is
* supported for all datatypes, including lists and maps.
*
AttributeValueList can contain only one
* AttributeValue element of type String, Number, Binary, String
* Set, Number Set, or Binary Set. If an item contains an
* AttributeValue element of a different type than the one
* provided in the request, the value does not match. For example,
* {"S":"6"}
does not equal {"N":"6"}
. Also,
* {"N":"6"}
does not equal {"NS":["6", "2",
* "1"]}
.
-
NE
: Not equal.
* NE
is supported for all datatypes, including lists and
* maps.
AttributeValueList can contain only one
* AttributeValue of type String, Number, Binary, String Set,
* Number Set, or Binary Set. If an item contains an
* AttributeValue of a different type than the one provided in the
* request, the value does not match. For example, {"S":"6"}
* does not equal {"N":"6"}
. Also, {"N":"6"}
* does not equal {"NS":["6", "2", "1"]}
.
-
*
LE
: Less than or equal.
AttributeValueList
* can contain only one AttributeValue element of type String,
* Number, or Binary (not a set type). If an item contains an
* AttributeValue element of a different type than the one
* provided in the request, the value does not match. For example,
* {"S":"6"}
does not equal {"N":"6"}
. Also,
* {"N":"6"}
does not compare to {"NS":["6", "2",
* "1"]}
.
-
LT
: Less than.
*
AttributeValueList can contain only one
* AttributeValue of type String, Number, or Binary (not a set
* type). If an item contains an AttributeValue element of a
* different type than the one provided in the request, the value does
* not match. For example, {"S":"6"}
does not equal
* {"N":"6"}
. Also, {"N":"6"}
does not compare
* to {"NS":["6", "2", "1"]}
.
-
*
GE
: Greater than or equal.
*
AttributeValueList can contain only one
* AttributeValue element of type String, Number, or Binary (not a
* set type). If an item contains an AttributeValue element of a
* different type than the one provided in the request, the value does
* not match. For example, {"S":"6"}
does not equal
* {"N":"6"}
. Also, {"N":"6"}
does not compare
* to {"NS":["6", "2", "1"]}
.
-
*
GT
: Greater than.
AttributeValueList can
* contain only one AttributeValue element of type String, Number,
* or Binary (not a set type). If an item contains an
* AttributeValue element of a different type than the one
* provided in the request, the value does not match. For example,
* {"S":"6"}
does not equal {"N":"6"}
. Also,
* {"N":"6"}
does not compare to {"NS":["6", "2",
* "1"]}
.
-
NOT_NULL
: The attribute
* exists. NOT_NULL
is supported for all datatypes,
* including lists and maps. This operator tests for the
* existence of an attribute, not its data type. If the data type of
* attribute "a
" is null, and you evaluate it using
* NOT_NULL
, the result is a Boolean true. This
* result is because the attribute "a
" exists; its data type
* is not relevant to the NOT_NULL
comparison operator.
*
-
NULL
: The attribute does not
* exist. NULL
is supported for all datatypes, including
* lists and maps. This operator tests for the nonexistence of
* an attribute, not its data type. If the data type of attribute
* "a
" is null, and you evaluate it using NULL
,
* the result is a Boolean false. This is because the attribute
* "a
" exists; its data type is not relevant to the
* NULL
comparison operator.
-
*
CONTAINS
: Checks for a subsequence, or value in a
* set.
AttributeValueList can contain only one
* AttributeValue element of type String, Number, or Binary (not a
* set type). If the target attribute of the comparison is of type
* String, then the operator checks for a substring match. If the target
* attribute of the comparison is of type Binary, then the operator looks
* for a subsequence of the target that matches the input. If the target
* attribute of the comparison is a set ("SS
",
* "NS
", or "BS
"), then the operator evaluates
* to true if it finds an exact match with any member of the set.
*
CONTAINS is supported for lists: When evaluating "a CONTAINS
* b
", "a
" can be a list; however, "b
"
* cannot be a set, a map, or a list.
-
*
NOT_CONTAINS
: Checks for absence of a subsequence, or
* absence of a value in a set.
AttributeValueList can contain
* only one AttributeValue element of type String, Number, or
* Binary (not a set type). If the target attribute of the comparison is
* a String, then the operator checks for the absence of a substring
* match. If the target attribute of the comparison is Binary, then the
* operator checks for the absence of a subsequence of the target that
* matches the input. If the target attribute of the comparison is a set
* ("SS
", "NS
", or "BS
"), then the
* operator evaluates to true if it does not find an exact match
* with any member of the set.
NOT_CONTAINS is supported for lists:
* When evaluating "a NOT CONTAINS b
", "a
" can
* be a list; however, "b
" cannot be a set, a map, or a
* list.
-
BEGINS_WITH
: Checks for a prefix.
*
AttributeValueList can contain only one
* AttributeValue of type String or Binary (not a Number or a set
* type). The target attribute of the comparison must be of type String
* or Binary (not a Number or a set type).
-
*
IN
: Checks for matching elements within two sets.
*
AttributeValueList can contain one or more
* AttributeValue elements of type String, Number, or Binary (not
* a set type). These attributes are compared against an existing set
* type attribute of an item. If any elements of the input set are
* present in the item attribute, the expression evaluates to true.
* -
BETWEEN
: Greater than or equal to the first
* value, and less than or equal to the second value.
*
AttributeValueList must contain two AttributeValue
* elements of the same type, either String, Number, or Binary (not a set
* type). A target attribute matches if the target value is greater than,
* or equal to, the first element and less than, or equal to, the second
* element. If an item contains an AttributeValue element of a
* different type than the one provided in the request, the value does
* not match. For example, {"S":"6"}
does not compare to
* {"N":"6"}
. Also, {"N":"6"}
does not compare
* to {"NS":["6", "2", "1"]}
For
* usage examples of AttributeValueList and
* ComparisonOperator, see Legacy
* Conditional Parameters in the Amazon DynamoDB Developer
* Guide.
For backward compatibility with previous DynamoDB
* releases, the following parameters can be used instead of
* AttributeValueList and ComparisonOperator:
-
*
Value - A value for DynamoDB to compare with an attribute.
*
-
Exists - A Boolean value that causes DynamoDB to
* evaluate the value before attempting the conditional operation:
* -
If Exists is true
, DynamoDB will check to
* see if that attribute value already exists in the table. If it is
* found, then the condition evaluates to true; otherwise the condition
* evaluate to false.
If Exists is
* false
, DynamoDB assumes that the attribute value does
* not exist in the table. If in fact the value does not exist,
* then the assumption is valid and the condition evaluates to true. If
* the value is found, despite the assumption that it does not exist, the
* condition evaluates to false.
Note that the default
* value for Exists is true
.
The
* Value and Exists parameters are incompatible with
* AttributeValueList and ComparisonOperator. Note that if
* you use both sets of parameters at once, DynamoDB will return a
* ValidationException exception. This parameter does not
* support attributes of type List or Map.
*
* @return This is a legacy parameter, for backward compatibility.
* New applications should use ConditionExpression instead. Do
* not combine legacy parameters and expression parameters in a single
* API call; otherwise, DynamoDB will return a ValidationException
* exception.
A map of attribute/condition pairs.
* Expected provides a conditional block for the UpdateItem
* operation.
Each element of Expected consists of an attribute
* name, a comparison operator, and one or more values. DynamoDB compares
* the attribute with the value(s) you supplied, using the comparison
* operator. For each Expected element, the result of the
* evaluation is either true or false.
If you specify more than one
* element in the Expected map, then by default all of the
* conditions must evaluate to true. In other words, the conditions are
* ANDed together. (You can use the ConditionalOperator parameter
* to OR the conditions instead. If you do this, then at least one of the
* conditions must evaluate to true, rather than all of them.)
If the
* Expected map evaluates to true, then the conditional operation
* succeeds; otherwise, it fails.
Expected contains the
* following:
-
AttributeValueList - One or more values
* to evaluate against the supplied attribute. The number of values in
* the list depends on the ComparisonOperator being used.
For
* type Number, value comparisons are numeric.
String value
* comparisons for greater than, equals, or less than are based on ASCII
* character code values. For example, a
is greater than
* A
, and a
is greater than B
. For
* a list of code values, see http://en.wikipedia.org/wiki/ASCII#ASCII_printable_characters.
*
For type Binary, DynamoDB treats each byte of the binary data as
* unsigned when it compares binary values.
-
*
ComparisonOperator - A comparator for evaluating attributes
* in the AttributeValueList. When performing the comparison,
* DynamoDB uses strongly consistent reads.
The following comparison
* operators are available:
EQ | NE | LE | LT | GE | GT |
* NOT_NULL | NULL | CONTAINS | NOT_CONTAINS | BEGINS_WITH | IN |
* BETWEEN
The following are descriptions of each comparison
* operator.
-
EQ
: Equal. EQ
is
* supported for all datatypes, including lists and maps.
*
AttributeValueList can contain only one
* AttributeValue element of type String, Number, Binary, String
* Set, Number Set, or Binary Set. If an item contains an
* AttributeValue element of a different type than the one
* provided in the request, the value does not match. For example,
* {"S":"6"}
does not equal {"N":"6"}
. Also,
* {"N":"6"}
does not equal {"NS":["6", "2",
* "1"]}
.
-
NE
: Not equal.
* NE
is supported for all datatypes, including lists and
* maps.
AttributeValueList can contain only one
* AttributeValue of type String, Number, Binary, String Set,
* Number Set, or Binary Set. If an item contains an
* AttributeValue of a different type than the one provided in the
* request, the value does not match. For example, {"S":"6"}
* does not equal {"N":"6"}
. Also, {"N":"6"}
* does not equal {"NS":["6", "2", "1"]}
.
-
*
LE
: Less than or equal.
AttributeValueList
* can contain only one AttributeValue element of type String,
* Number, or Binary (not a set type). If an item contains an
* AttributeValue element of a different type than the one
* provided in the request, the value does not match. For example,
* {"S":"6"}
does not equal {"N":"6"}
. Also,
* {"N":"6"}
does not compare to {"NS":["6", "2",
* "1"]}
.
-
LT
: Less than.
*
AttributeValueList can contain only one
* AttributeValue of type String, Number, or Binary (not a set
* type). If an item contains an AttributeValue element of a
* different type than the one provided in the request, the value does
* not match. For example, {"S":"6"}
does not equal
* {"N":"6"}
. Also, {"N":"6"}
does not compare
* to {"NS":["6", "2", "1"]}
.
-
*
GE
: Greater than or equal.
*
AttributeValueList can contain only one
* AttributeValue element of type String, Number, or Binary (not a
* set type). If an item contains an AttributeValue element of a
* different type than the one provided in the request, the value does
* not match. For example, {"S":"6"}
does not equal
* {"N":"6"}
. Also, {"N":"6"}
does not compare
* to {"NS":["6", "2", "1"]}
.
-
*
GT
: Greater than.
AttributeValueList can
* contain only one AttributeValue element of type String, Number,
* or Binary (not a set type). If an item contains an
* AttributeValue element of a different type than the one
* provided in the request, the value does not match. For example,
* {"S":"6"}
does not equal {"N":"6"}
. Also,
* {"N":"6"}
does not compare to {"NS":["6", "2",
* "1"]}
.
-
NOT_NULL
: The attribute
* exists. NOT_NULL
is supported for all datatypes,
* including lists and maps. This operator tests for the
* existence of an attribute, not its data type. If the data type of
* attribute "a
" is null, and you evaluate it using
* NOT_NULL
, the result is a Boolean true. This
* result is because the attribute "a
" exists; its data type
* is not relevant to the NOT_NULL
comparison operator.
*
-
NULL
: The attribute does not
* exist. NULL
is supported for all datatypes, including
* lists and maps. This operator tests for the nonexistence of
* an attribute, not its data type. If the data type of attribute
* "a
" is null, and you evaluate it using NULL
,
* the result is a Boolean false. This is because the attribute
* "a
" exists; its data type is not relevant to the
* NULL
comparison operator.
-
*
CONTAINS
: Checks for a subsequence, or value in a
* set.
AttributeValueList can contain only one
* AttributeValue element of type String, Number, or Binary (not a
* set type). If the target attribute of the comparison is of type
* String, then the operator checks for a substring match. If the target
* attribute of the comparison is of type Binary, then the operator looks
* for a subsequence of the target that matches the input. If the target
* attribute of the comparison is a set ("SS
",
* "NS
", or "BS
"), then the operator evaluates
* to true if it finds an exact match with any member of the set.
*
CONTAINS is supported for lists: When evaluating "a CONTAINS
* b
", "a
" can be a list; however, "b
"
* cannot be a set, a map, or a list.
-
*
NOT_CONTAINS
: Checks for absence of a subsequence, or
* absence of a value in a set.
AttributeValueList can contain
* only one AttributeValue element of type String, Number, or
* Binary (not a set type). If the target attribute of the comparison is
* a String, then the operator checks for the absence of a substring
* match. If the target attribute of the comparison is Binary, then the
* operator checks for the absence of a subsequence of the target that
* matches the input. If the target attribute of the comparison is a set
* ("SS
", "NS
", or "BS
"), then the
* operator evaluates to true if it does not find an exact match
* with any member of the set.
NOT_CONTAINS is supported for lists:
* When evaluating "a NOT CONTAINS b
", "a
" can
* be a list; however, "b
" cannot be a set, a map, or a
* list.
-
BEGINS_WITH
: Checks for a prefix.
*
AttributeValueList can contain only one
* AttributeValue of type String or Binary (not a Number or a set
* type). The target attribute of the comparison must be of type String
* or Binary (not a Number or a set type).
-
*
IN
: Checks for matching elements within two sets.
*
AttributeValueList can contain one or more
* AttributeValue elements of type String, Number, or Binary (not
* a set type). These attributes are compared against an existing set
* type attribute of an item. If any elements of the input set are
* present in the item attribute, the expression evaluates to true.
* -
BETWEEN
: Greater than or equal to the first
* value, and less than or equal to the second value.
*
AttributeValueList must contain two AttributeValue
* elements of the same type, either String, Number, or Binary (not a set
* type). A target attribute matches if the target value is greater than,
* or equal to, the first element and less than, or equal to, the second
* element. If an item contains an AttributeValue element of a
* different type than the one provided in the request, the value does
* not match. For example, {"S":"6"}
does not compare to
* {"N":"6"}
. Also, {"N":"6"}
does not compare
* to {"NS":["6", "2", "1"]}
For
* usage examples of AttributeValueList and
* ComparisonOperator, see Legacy
* Conditional Parameters in the Amazon DynamoDB Developer
* Guide.
For backward compatibility with previous DynamoDB
* releases, the following parameters can be used instead of
* AttributeValueList and ComparisonOperator:
-
*
Value - A value for DynamoDB to compare with an attribute.
*
-
Exists - A Boolean value that causes DynamoDB to
* evaluate the value before attempting the conditional operation:
* -
If Exists is true
, DynamoDB will check to
* see if that attribute value already exists in the table. If it is
* found, then the condition evaluates to true; otherwise the condition
* evaluate to false.
If Exists is
* false
, DynamoDB assumes that the attribute value does
* not exist in the table. If in fact the value does not exist,
* then the assumption is valid and the condition evaluates to true. If
* the value is found, despite the assumption that it does not exist, the
* condition evaluates to false.
Note that the default
* value for Exists is true
.
The
* Value and Exists parameters are incompatible with
* AttributeValueList and ComparisonOperator. Note that if
* you use both sets of parameters at once, DynamoDB will return a
* ValidationException exception. This parameter does not
* support attributes of type List or Map.
*/
public java.util.Map getExpected() {
return expected;
}
/**
* This is a legacy parameter, for backward compatibility.
* New applications should use ConditionExpression instead. Do
* not combine legacy parameters and expression parameters in a single
* API call; otherwise, DynamoDB will return a ValidationException
* exception.
A map of attribute/condition pairs.
* Expected provides a conditional block for the UpdateItem
* operation.
Each element of Expected consists of an attribute
* name, a comparison operator, and one or more values. DynamoDB compares
* the attribute with the value(s) you supplied, using the comparison
* operator. For each Expected element, the result of the
* evaluation is either true or false.
If you specify more than one
* element in the Expected map, then by default all of the
* conditions must evaluate to true. In other words, the conditions are
* ANDed together. (You can use the ConditionalOperator parameter
* to OR the conditions instead. If you do this, then at least one of the
* conditions must evaluate to true, rather than all of them.)
If the
* Expected map evaluates to true, then the conditional operation
* succeeds; otherwise, it fails.
Expected contains the
* following:
-
AttributeValueList - One or more values
* to evaluate against the supplied attribute. The number of values in
* the list depends on the ComparisonOperator being used.
For
* type Number, value comparisons are numeric.
String value
* comparisons for greater than, equals, or less than are based on ASCII
* character code values. For example, a
is greater than
* A
, and a
is greater than B
. For
* a list of code values, see http://en.wikipedia.org/wiki/ASCII#ASCII_printable_characters.
*
For type Binary, DynamoDB treats each byte of the binary data as
* unsigned when it compares binary values.
-
*
ComparisonOperator - A comparator for evaluating attributes
* in the AttributeValueList. When performing the comparison,
* DynamoDB uses strongly consistent reads.
The following comparison
* operators are available:
EQ | NE | LE | LT | GE | GT |
* NOT_NULL | NULL | CONTAINS | NOT_CONTAINS | BEGINS_WITH | IN |
* BETWEEN
The following are descriptions of each comparison
* operator.
-
EQ
: Equal. EQ
is
* supported for all datatypes, including lists and maps.
*
AttributeValueList can contain only one
* AttributeValue element of type String, Number, Binary, String
* Set, Number Set, or Binary Set. If an item contains an
* AttributeValue element of a different type than the one
* provided in the request, the value does not match. For example,
* {"S":"6"}
does not equal {"N":"6"}
. Also,
* {"N":"6"}
does not equal {"NS":["6", "2",
* "1"]}
.
-
NE
: Not equal.
* NE
is supported for all datatypes, including lists and
* maps.
AttributeValueList can contain only one
* AttributeValue of type String, Number, Binary, String Set,
* Number Set, or Binary Set. If an item contains an
* AttributeValue of a different type than the one provided in the
* request, the value does not match. For example, {"S":"6"}
* does not equal {"N":"6"}
. Also, {"N":"6"}
* does not equal {"NS":["6", "2", "1"]}
.
-
*
LE
: Less than or equal.
AttributeValueList
* can contain only one AttributeValue element of type String,
* Number, or Binary (not a set type). If an item contains an
* AttributeValue element of a different type than the one
* provided in the request, the value does not match. For example,
* {"S":"6"}
does not equal {"N":"6"}
. Also,
* {"N":"6"}
does not compare to {"NS":["6", "2",
* "1"]}
.
-
LT
: Less than.
*
AttributeValueList can contain only one
* AttributeValue of type String, Number, or Binary (not a set
* type). If an item contains an AttributeValue element of a
* different type than the one provided in the request, the value does
* not match. For example, {"S":"6"}
does not equal
* {"N":"6"}
. Also, {"N":"6"}
does not compare
* to {"NS":["6", "2", "1"]}
.
-
*
GE
: Greater than or equal.
*
AttributeValueList can contain only one
* AttributeValue element of type String, Number, or Binary (not a
* set type). If an item contains an AttributeValue element of a
* different type than the one provided in the request, the value does
* not match. For example, {"S":"6"}
does not equal
* {"N":"6"}
. Also, {"N":"6"}
does not compare
* to {"NS":["6", "2", "1"]}
.
-
*
GT
: Greater than.
AttributeValueList can
* contain only one AttributeValue element of type String, Number,
* or Binary (not a set type). If an item contains an
* AttributeValue element of a different type than the one
* provided in the request, the value does not match. For example,
* {"S":"6"}
does not equal {"N":"6"}
. Also,
* {"N":"6"}
does not compare to {"NS":["6", "2",
* "1"]}
.
-
NOT_NULL
: The attribute
* exists. NOT_NULL
is supported for all datatypes,
* including lists and maps. This operator tests for the
* existence of an attribute, not its data type. If the data type of
* attribute "a
" is null, and you evaluate it using
* NOT_NULL
, the result is a Boolean true. This
* result is because the attribute "a
" exists; its data type
* is not relevant to the NOT_NULL
comparison operator.
*
-
NULL
: The attribute does not
* exist. NULL
is supported for all datatypes, including
* lists and maps. This operator tests for the nonexistence of
* an attribute, not its data type. If the data type of attribute
* "a
" is null, and you evaluate it using NULL
,
* the result is a Boolean false. This is because the attribute
* "a
" exists; its data type is not relevant to the
* NULL
comparison operator.
-
*
CONTAINS
: Checks for a subsequence, or value in a
* set.
AttributeValueList can contain only one
* AttributeValue element of type String, Number, or Binary (not a
* set type). If the target attribute of the comparison is of type
* String, then the operator checks for a substring match. If the target
* attribute of the comparison is of type Binary, then the operator looks
* for a subsequence of the target that matches the input. If the target
* attribute of the comparison is a set ("SS
",
* "NS
", or "BS
"), then the operator evaluates
* to true if it finds an exact match with any member of the set.
*
CONTAINS is supported for lists: When evaluating "a CONTAINS
* b
", "a
" can be a list; however, "b
"
* cannot be a set, a map, or a list.
-
*
NOT_CONTAINS
: Checks for absence of a subsequence, or
* absence of a value in a set.
AttributeValueList can contain
* only one AttributeValue element of type String, Number, or
* Binary (not a set type). If the target attribute of the comparison is
* a String, then the operator checks for the absence of a substring
* match. If the target attribute of the comparison is Binary, then the
* operator checks for the absence of a subsequence of the target that
* matches the input. If the target attribute of the comparison is a set
* ("SS
", "NS
", or "BS
"), then the
* operator evaluates to true if it does not find an exact match
* with any member of the set.
NOT_CONTAINS is supported for lists:
* When evaluating "a NOT CONTAINS b
", "a
" can
* be a list; however, "b
" cannot be a set, a map, or a
* list.
-
BEGINS_WITH
: Checks for a prefix.
*
AttributeValueList can contain only one
* AttributeValue of type String or Binary (not a Number or a set
* type). The target attribute of the comparison must be of type String
* or Binary (not a Number or a set type).
-
*
IN
: Checks for matching elements within two sets.
*
AttributeValueList can contain one or more
* AttributeValue elements of type String, Number, or Binary (not
* a set type). These attributes are compared against an existing set
* type attribute of an item. If any elements of the input set are
* present in the item attribute, the expression evaluates to true.
* -
BETWEEN
: Greater than or equal to the first
* value, and less than or equal to the second value.
*
AttributeValueList must contain two AttributeValue
* elements of the same type, either String, Number, or Binary (not a set
* type). A target attribute matches if the target value is greater than,
* or equal to, the first element and less than, or equal to, the second
* element. If an item contains an AttributeValue element of a
* different type than the one provided in the request, the value does
* not match. For example, {"S":"6"}
does not compare to
* {"N":"6"}
. Also, {"N":"6"}
does not compare
* to {"NS":["6", "2", "1"]}
For
* usage examples of AttributeValueList and
* ComparisonOperator, see Legacy
* Conditional Parameters in the Amazon DynamoDB Developer
* Guide.
For backward compatibility with previous DynamoDB
* releases, the following parameters can be used instead of
* AttributeValueList and ComparisonOperator:
-
*
Value - A value for DynamoDB to compare with an attribute.
*
-
Exists - A Boolean value that causes DynamoDB to
* evaluate the value before attempting the conditional operation:
* -
If Exists is true
, DynamoDB will check to
* see if that attribute value already exists in the table. If it is
* found, then the condition evaluates to true; otherwise the condition
* evaluate to false.
If Exists is
* false
, DynamoDB assumes that the attribute value does
* not exist in the table. If in fact the value does not exist,
* then the assumption is valid and the condition evaluates to true. If
* the value is found, despite the assumption that it does not exist, the
* condition evaluates to false.
Note that the default
* value for Exists is true
.
The
* Value and Exists parameters are incompatible with
* AttributeValueList and ComparisonOperator. Note that if
* you use both sets of parameters at once, DynamoDB will return a
* ValidationException exception. This parameter does not
* support attributes of type List or Map.
*
* @param expected This is a legacy parameter, for backward compatibility.
* New applications should use ConditionExpression instead. Do
* not combine legacy parameters and expression parameters in a single
* API call; otherwise, DynamoDB will return a ValidationException
* exception.
A map of attribute/condition pairs.
* Expected provides a conditional block for the UpdateItem
* operation.
Each element of Expected consists of an attribute
* name, a comparison operator, and one or more values. DynamoDB compares
* the attribute with the value(s) you supplied, using the comparison
* operator. For each Expected element, the result of the
* evaluation is either true or false.
If you specify more than one
* element in the Expected map, then by default all of the
* conditions must evaluate to true. In other words, the conditions are
* ANDed together. (You can use the ConditionalOperator parameter
* to OR the conditions instead. If you do this, then at least one of the
* conditions must evaluate to true, rather than all of them.)
If the
* Expected map evaluates to true, then the conditional operation
* succeeds; otherwise, it fails.
Expected contains the
* following:
-
AttributeValueList - One or more values
* to evaluate against the supplied attribute. The number of values in
* the list depends on the ComparisonOperator being used.
For
* type Number, value comparisons are numeric.
String value
* comparisons for greater than, equals, or less than are based on ASCII
* character code values. For example, a
is greater than
* A
, and a
is greater than B
. For
* a list of code values, see http://en.wikipedia.org/wiki/ASCII#ASCII_printable_characters.
*
For type Binary, DynamoDB treats each byte of the binary data as
* unsigned when it compares binary values.
-
*
ComparisonOperator - A comparator for evaluating attributes
* in the AttributeValueList. When performing the comparison,
* DynamoDB uses strongly consistent reads.
The following comparison
* operators are available:
EQ | NE | LE | LT | GE | GT |
* NOT_NULL | NULL | CONTAINS | NOT_CONTAINS | BEGINS_WITH | IN |
* BETWEEN
The following are descriptions of each comparison
* operator.
-
EQ
: Equal. EQ
is
* supported for all datatypes, including lists and maps.
*
AttributeValueList can contain only one
* AttributeValue element of type String, Number, Binary, String
* Set, Number Set, or Binary Set. If an item contains an
* AttributeValue element of a different type than the one
* provided in the request, the value does not match. For example,
* {"S":"6"}
does not equal {"N":"6"}
. Also,
* {"N":"6"}
does not equal {"NS":["6", "2",
* "1"]}
.
-
NE
: Not equal.
* NE
is supported for all datatypes, including lists and
* maps.
AttributeValueList can contain only one
* AttributeValue of type String, Number, Binary, String Set,
* Number Set, or Binary Set. If an item contains an
* AttributeValue of a different type than the one provided in the
* request, the value does not match. For example, {"S":"6"}
* does not equal {"N":"6"}
. Also, {"N":"6"}
* does not equal {"NS":["6", "2", "1"]}
.
-
*
LE
: Less than or equal.
AttributeValueList
* can contain only one AttributeValue element of type String,
* Number, or Binary (not a set type). If an item contains an
* AttributeValue element of a different type than the one
* provided in the request, the value does not match. For example,
* {"S":"6"}
does not equal {"N":"6"}
. Also,
* {"N":"6"}
does not compare to {"NS":["6", "2",
* "1"]}
.
-
LT
: Less than.
*
AttributeValueList can contain only one
* AttributeValue of type String, Number, or Binary (not a set
* type). If an item contains an AttributeValue element of a
* different type than the one provided in the request, the value does
* not match. For example, {"S":"6"}
does not equal
* {"N":"6"}
. Also, {"N":"6"}
does not compare
* to {"NS":["6", "2", "1"]}
.
-
*
GE
: Greater than or equal.
*
AttributeValueList can contain only one
* AttributeValue element of type String, Number, or Binary (not a
* set type). If an item contains an AttributeValue element of a
* different type than the one provided in the request, the value does
* not match. For example, {"S":"6"}
does not equal
* {"N":"6"}
. Also, {"N":"6"}
does not compare
* to {"NS":["6", "2", "1"]}
.
-
*
GT
: Greater than.
AttributeValueList can
* contain only one AttributeValue element of type String, Number,
* or Binary (not a set type). If an item contains an
* AttributeValue element of a different type than the one
* provided in the request, the value does not match. For example,
* {"S":"6"}
does not equal {"N":"6"}
. Also,
* {"N":"6"}
does not compare to {"NS":["6", "2",
* "1"]}
.
-
NOT_NULL
: The attribute
* exists. NOT_NULL
is supported for all datatypes,
* including lists and maps. This operator tests for the
* existence of an attribute, not its data type. If the data type of
* attribute "a
" is null, and you evaluate it using
* NOT_NULL
, the result is a Boolean true. This
* result is because the attribute "a
" exists; its data type
* is not relevant to the NOT_NULL
comparison operator.
*
-
NULL
: The attribute does not
* exist. NULL
is supported for all datatypes, including
* lists and maps. This operator tests for the nonexistence of
* an attribute, not its data type. If the data type of attribute
* "a
" is null, and you evaluate it using NULL
,
* the result is a Boolean false. This is because the attribute
* "a
" exists; its data type is not relevant to the
* NULL
comparison operator.
-
*
CONTAINS
: Checks for a subsequence, or value in a
* set.
AttributeValueList can contain only one
* AttributeValue element of type String, Number, or Binary (not a
* set type). If the target attribute of the comparison is of type
* String, then the operator checks for a substring match. If the target
* attribute of the comparison is of type Binary, then the operator looks
* for a subsequence of the target that matches the input. If the target
* attribute of the comparison is a set ("SS
",
* "NS
", or "BS
"), then the operator evaluates
* to true if it finds an exact match with any member of the set.
*
CONTAINS is supported for lists: When evaluating "a CONTAINS
* b
", "a
" can be a list; however, "b
"
* cannot be a set, a map, or a list.
-
*
NOT_CONTAINS
: Checks for absence of a subsequence, or
* absence of a value in a set.
AttributeValueList can contain
* only one AttributeValue element of type String, Number, or
* Binary (not a set type). If the target attribute of the comparison is
* a String, then the operator checks for the absence of a substring
* match. If the target attribute of the comparison is Binary, then the
* operator checks for the absence of a subsequence of the target that
* matches the input. If the target attribute of the comparison is a set
* ("SS
", "NS
", or "BS
"), then the
* operator evaluates to true if it does not find an exact match
* with any member of the set.
NOT_CONTAINS is supported for lists:
* When evaluating "a NOT CONTAINS b
", "a
" can
* be a list; however, "b
" cannot be a set, a map, or a
* list.
-
BEGINS_WITH
: Checks for a prefix.
*
AttributeValueList can contain only one
* AttributeValue of type String or Binary (not a Number or a set
* type). The target attribute of the comparison must be of type String
* or Binary (not a Number or a set type).
-
*
IN
: Checks for matching elements within two sets.
*
AttributeValueList can contain one or more
* AttributeValue elements of type String, Number, or Binary (not
* a set type). These attributes are compared against an existing set
* type attribute of an item. If any elements of the input set are
* present in the item attribute, the expression evaluates to true.
* -
BETWEEN
: Greater than or equal to the first
* value, and less than or equal to the second value.
*
AttributeValueList must contain two AttributeValue
* elements of the same type, either String, Number, or Binary (not a set
* type). A target attribute matches if the target value is greater than,
* or equal to, the first element and less than, or equal to, the second
* element. If an item contains an AttributeValue element of a
* different type than the one provided in the request, the value does
* not match. For example, {"S":"6"}
does not compare to
* {"N":"6"}
. Also, {"N":"6"}
does not compare
* to {"NS":["6", "2", "1"]}
For
* usage examples of AttributeValueList and
* ComparisonOperator, see Legacy
* Conditional Parameters in the Amazon DynamoDB Developer
* Guide.
For backward compatibility with previous DynamoDB
* releases, the following parameters can be used instead of
* AttributeValueList and ComparisonOperator:
-
*
Value - A value for DynamoDB to compare with an attribute.
*
-
Exists - A Boolean value that causes DynamoDB to
* evaluate the value before attempting the conditional operation:
* -
If Exists is true
, DynamoDB will check to
* see if that attribute value already exists in the table. If it is
* found, then the condition evaluates to true; otherwise the condition
* evaluate to false.
If Exists is
* false
, DynamoDB assumes that the attribute value does
* not exist in the table. If in fact the value does not exist,
* then the assumption is valid and the condition evaluates to true. If
* the value is found, despite the assumption that it does not exist, the
* condition evaluates to false.
Note that the default
* value for Exists is true
.
The
* Value and Exists parameters are incompatible with
* AttributeValueList and ComparisonOperator. Note that if
* you use both sets of parameters at once, DynamoDB will return a
* ValidationException exception. This parameter does not
* support attributes of type List or Map.
*/
public void setExpected(java.util.Map expected) {
this.expected = expected;
}
/**
* This is a legacy parameter, for backward compatibility.
* New applications should use ConditionExpression instead. Do
* not combine legacy parameters and expression parameters in a single
* API call; otherwise, DynamoDB will return a ValidationException
* exception.
A map of attribute/condition pairs.
* Expected provides a conditional block for the UpdateItem
* operation.
Each element of Expected consists of an attribute
* name, a comparison operator, and one or more values. DynamoDB compares
* the attribute with the value(s) you supplied, using the comparison
* operator. For each Expected element, the result of the
* evaluation is either true or false.
If you specify more than one
* element in the Expected map, then by default all of the
* conditions must evaluate to true. In other words, the conditions are
* ANDed together. (You can use the ConditionalOperator parameter
* to OR the conditions instead. If you do this, then at least one of the
* conditions must evaluate to true, rather than all of them.)
If the
* Expected map evaluates to true, then the conditional operation
* succeeds; otherwise, it fails.
Expected contains the
* following:
-
AttributeValueList - One or more values
* to evaluate against the supplied attribute. The number of values in
* the list depends on the ComparisonOperator being used.
For
* type Number, value comparisons are numeric.
String value
* comparisons for greater than, equals, or less than are based on ASCII
* character code values. For example, a
is greater than
* A
, and a
is greater than B
. For
* a list of code values, see http://en.wikipedia.org/wiki/ASCII#ASCII_printable_characters.
*
For type Binary, DynamoDB treats each byte of the binary data as
* unsigned when it compares binary values.
-
*
ComparisonOperator - A comparator for evaluating attributes
* in the AttributeValueList. When performing the comparison,
* DynamoDB uses strongly consistent reads.
The following comparison
* operators are available:
EQ | NE | LE | LT | GE | GT |
* NOT_NULL | NULL | CONTAINS | NOT_CONTAINS | BEGINS_WITH | IN |
* BETWEEN
The following are descriptions of each comparison
* operator.
-
EQ
: Equal. EQ
is
* supported for all datatypes, including lists and maps.
*
AttributeValueList can contain only one
* AttributeValue element of type String, Number, Binary, String
* Set, Number Set, or Binary Set. If an item contains an
* AttributeValue element of a different type than the one
* provided in the request, the value does not match. For example,
* {"S":"6"}
does not equal {"N":"6"}
. Also,
* {"N":"6"}
does not equal {"NS":["6", "2",
* "1"]}
.
-
NE
: Not equal.
* NE
is supported for all datatypes, including lists and
* maps.
AttributeValueList can contain only one
* AttributeValue of type String, Number, Binary, String Set,
* Number Set, or Binary Set. If an item contains an
* AttributeValue of a different type than the one provided in the
* request, the value does not match. For example, {"S":"6"}
* does not equal {"N":"6"}
. Also, {"N":"6"}
* does not equal {"NS":["6", "2", "1"]}
.
-
*
LE
: Less than or equal.
AttributeValueList
* can contain only one AttributeValue element of type String,
* Number, or Binary (not a set type). If an item contains an
* AttributeValue element of a different type than the one
* provided in the request, the value does not match. For example,
* {"S":"6"}
does not equal {"N":"6"}
. Also,
* {"N":"6"}
does not compare to {"NS":["6", "2",
* "1"]}
.
-
LT
: Less than.
*
AttributeValueList can contain only one
* AttributeValue of type String, Number, or Binary (not a set
* type). If an item contains an AttributeValue element of a
* different type than the one provided in the request, the value does
* not match. For example, {"S":"6"}
does not equal
* {"N":"6"}
. Also, {"N":"6"}
does not compare
* to {"NS":["6", "2", "1"]}
.
-
*
GE
: Greater than or equal.
*
AttributeValueList can contain only one
* AttributeValue element of type String, Number, or Binary (not a
* set type). If an item contains an AttributeValue element of a
* different type than the one provided in the request, the value does
* not match. For example, {"S":"6"}
does not equal
* {"N":"6"}
. Also, {"N":"6"}
does not compare
* to {"NS":["6", "2", "1"]}
.
-
*
GT
: Greater than.
AttributeValueList can
* contain only one AttributeValue element of type String, Number,
* or Binary (not a set type). If an item contains an
* AttributeValue element of a different type than the one
* provided in the request, the value does not match. For example,
* {"S":"6"}
does not equal {"N":"6"}
. Also,
* {"N":"6"}
does not compare to {"NS":["6", "2",
* "1"]}
.
-
NOT_NULL
: The attribute
* exists. NOT_NULL
is supported for all datatypes,
* including lists and maps. This operator tests for the
* existence of an attribute, not its data type. If the data type of
* attribute "a
" is null, and you evaluate it using
* NOT_NULL
, the result is a Boolean true. This
* result is because the attribute "a
" exists; its data type
* is not relevant to the NOT_NULL
comparison operator.
*
-
NULL
: The attribute does not
* exist. NULL
is supported for all datatypes, including
* lists and maps. This operator tests for the nonexistence of
* an attribute, not its data type. If the data type of attribute
* "a
" is null, and you evaluate it using NULL
,
* the result is a Boolean false. This is because the attribute
* "a
" exists; its data type is not relevant to the
* NULL
comparison operator.
-
*
CONTAINS
: Checks for a subsequence, or value in a
* set.
AttributeValueList can contain only one
* AttributeValue element of type String, Number, or Binary (not a
* set type). If the target attribute of the comparison is of type
* String, then the operator checks for a substring match. If the target
* attribute of the comparison is of type Binary, then the operator looks
* for a subsequence of the target that matches the input. If the target
* attribute of the comparison is a set ("SS
",
* "NS
", or "BS
"), then the operator evaluates
* to true if it finds an exact match with any member of the set.
*
CONTAINS is supported for lists: When evaluating "a CONTAINS
* b
", "a
" can be a list; however, "b
"
* cannot be a set, a map, or a list.
-
*
NOT_CONTAINS
: Checks for absence of a subsequence, or
* absence of a value in a set.
AttributeValueList can contain
* only one AttributeValue element of type String, Number, or
* Binary (not a set type). If the target attribute of the comparison is
* a String, then the operator checks for the absence of a substring
* match. If the target attribute of the comparison is Binary, then the
* operator checks for the absence of a subsequence of the target that
* matches the input. If the target attribute of the comparison is a set
* ("SS
", "NS
", or "BS
"), then the
* operator evaluates to true if it does not find an exact match
* with any member of the set.
NOT_CONTAINS is supported for lists:
* When evaluating "a NOT CONTAINS b
", "a
" can
* be a list; however, "b
" cannot be a set, a map, or a
* list.
-
BEGINS_WITH
: Checks for a prefix.
*
AttributeValueList can contain only one
* AttributeValue of type String or Binary (not a Number or a set
* type). The target attribute of the comparison must be of type String
* or Binary (not a Number or a set type).
-
*
IN
: Checks for matching elements within two sets.
*
AttributeValueList can contain one or more
* AttributeValue elements of type String, Number, or Binary (not
* a set type). These attributes are compared against an existing set
* type attribute of an item. If any elements of the input set are
* present in the item attribute, the expression evaluates to true.
* -
BETWEEN
: Greater than or equal to the first
* value, and less than or equal to the second value.
*
AttributeValueList must contain two AttributeValue
* elements of the same type, either String, Number, or Binary (not a set
* type). A target attribute matches if the target value is greater than,
* or equal to, the first element and less than, or equal to, the second
* element. If an item contains an AttributeValue element of a
* different type than the one provided in the request, the value does
* not match. For example, {"S":"6"}
does not compare to
* {"N":"6"}
. Also, {"N":"6"}
does not compare
* to {"NS":["6", "2", "1"]}
For
* usage examples of AttributeValueList and
* ComparisonOperator, see Legacy
* Conditional Parameters in the Amazon DynamoDB Developer
* Guide.
For backward compatibility with previous DynamoDB
* releases, the following parameters can be used instead of
* AttributeValueList and ComparisonOperator:
-
*
Value - A value for DynamoDB to compare with an attribute.
*
-
Exists - A Boolean value that causes DynamoDB to
* evaluate the value before attempting the conditional operation:
* -
If Exists is true
, DynamoDB will check to
* see if that attribute value already exists in the table. If it is
* found, then the condition evaluates to true; otherwise the condition
* evaluate to false.
If Exists is
* false
, DynamoDB assumes that the attribute value does
* not exist in the table. If in fact the value does not exist,
* then the assumption is valid and the condition evaluates to true. If
* the value is found, despite the assumption that it does not exist, the
* condition evaluates to false.
Note that the default
* value for Exists is true
.
The
* Value and Exists parameters are incompatible with
* AttributeValueList and ComparisonOperator. Note that if
* you use both sets of parameters at once, DynamoDB will return a
* ValidationException exception. This parameter does not
* support attributes of type List or Map.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param expected This is a legacy parameter, for backward compatibility.
* New applications should use ConditionExpression instead. Do
* not combine legacy parameters and expression parameters in a single
* API call; otherwise, DynamoDB will return a ValidationException
* exception.
A map of attribute/condition pairs.
* Expected provides a conditional block for the UpdateItem
* operation.
Each element of Expected consists of an attribute
* name, a comparison operator, and one or more values. DynamoDB compares
* the attribute with the value(s) you supplied, using the comparison
* operator. For each Expected element, the result of the
* evaluation is either true or false.
If you specify more than one
* element in the Expected map, then by default all of the
* conditions must evaluate to true. In other words, the conditions are
* ANDed together. (You can use the ConditionalOperator parameter
* to OR the conditions instead. If you do this, then at least one of the
* conditions must evaluate to true, rather than all of them.)
If the
* Expected map evaluates to true, then the conditional operation
* succeeds; otherwise, it fails.
Expected contains the
* following:
-
AttributeValueList - One or more values
* to evaluate against the supplied attribute. The number of values in
* the list depends on the ComparisonOperator being used.
For
* type Number, value comparisons are numeric.
String value
* comparisons for greater than, equals, or less than are based on ASCII
* character code values. For example, a
is greater than
* A
, and a
is greater than B
. For
* a list of code values, see http://en.wikipedia.org/wiki/ASCII#ASCII_printable_characters.
*
For type Binary, DynamoDB treats each byte of the binary data as
* unsigned when it compares binary values.
-
*
ComparisonOperator - A comparator for evaluating attributes
* in the AttributeValueList. When performing the comparison,
* DynamoDB uses strongly consistent reads.
The following comparison
* operators are available:
EQ | NE | LE | LT | GE | GT |
* NOT_NULL | NULL | CONTAINS | NOT_CONTAINS | BEGINS_WITH | IN |
* BETWEEN
The following are descriptions of each comparison
* operator.
-
EQ
: Equal. EQ
is
* supported for all datatypes, including lists and maps.
*
AttributeValueList can contain only one
* AttributeValue element of type String, Number, Binary, String
* Set, Number Set, or Binary Set. If an item contains an
* AttributeValue element of a different type than the one
* provided in the request, the value does not match. For example,
* {"S":"6"}
does not equal {"N":"6"}
. Also,
* {"N":"6"}
does not equal {"NS":["6", "2",
* "1"]}
.
-
NE
: Not equal.
* NE
is supported for all datatypes, including lists and
* maps.
AttributeValueList can contain only one
* AttributeValue of type String, Number, Binary, String Set,
* Number Set, or Binary Set. If an item contains an
* AttributeValue of a different type than the one provided in the
* request, the value does not match. For example, {"S":"6"}
* does not equal {"N":"6"}
. Also, {"N":"6"}
* does not equal {"NS":["6", "2", "1"]}
.
-
*
LE
: Less than or equal.
AttributeValueList
* can contain only one AttributeValue element of type String,
* Number, or Binary (not a set type). If an item contains an
* AttributeValue element of a different type than the one
* provided in the request, the value does not match. For example,
* {"S":"6"}
does not equal {"N":"6"}
. Also,
* {"N":"6"}
does not compare to {"NS":["6", "2",
* "1"]}
.
-
LT
: Less than.
*
AttributeValueList can contain only one
* AttributeValue of type String, Number, or Binary (not a set
* type). If an item contains an AttributeValue element of a
* different type than the one provided in the request, the value does
* not match. For example, {"S":"6"}
does not equal
* {"N":"6"}
. Also, {"N":"6"}
does not compare
* to {"NS":["6", "2", "1"]}
.
-
*
GE
: Greater than or equal.
*
AttributeValueList can contain only one
* AttributeValue element of type String, Number, or Binary (not a
* set type). If an item contains an AttributeValue element of a
* different type than the one provided in the request, the value does
* not match. For example, {"S":"6"}
does not equal
* {"N":"6"}
. Also, {"N":"6"}
does not compare
* to {"NS":["6", "2", "1"]}
.
-
*
GT
: Greater than.
AttributeValueList can
* contain only one AttributeValue element of type String, Number,
* or Binary (not a set type). If an item contains an
* AttributeValue element of a different type than the one
* provided in the request, the value does not match. For example,
* {"S":"6"}
does not equal {"N":"6"}
. Also,
* {"N":"6"}
does not compare to {"NS":["6", "2",
* "1"]}
.
-
NOT_NULL
: The attribute
* exists. NOT_NULL
is supported for all datatypes,
* including lists and maps. This operator tests for the
* existence of an attribute, not its data type. If the data type of
* attribute "a
" is null, and you evaluate it using
* NOT_NULL
, the result is a Boolean true. This
* result is because the attribute "a
" exists; its data type
* is not relevant to the NOT_NULL
comparison operator.
*
-
NULL
: The attribute does not
* exist. NULL
is supported for all datatypes, including
* lists and maps. This operator tests for the nonexistence of
* an attribute, not its data type. If the data type of attribute
* "a
" is null, and you evaluate it using NULL
,
* the result is a Boolean false. This is because the attribute
* "a
" exists; its data type is not relevant to the
* NULL
comparison operator.
-
*
CONTAINS
: Checks for a subsequence, or value in a
* set.
AttributeValueList can contain only one
* AttributeValue element of type String, Number, or Binary (not a
* set type). If the target attribute of the comparison is of type
* String, then the operator checks for a substring match. If the target
* attribute of the comparison is of type Binary, then the operator looks
* for a subsequence of the target that matches the input. If the target
* attribute of the comparison is a set ("SS
",
* "NS
", or "BS
"), then the operator evaluates
* to true if it finds an exact match with any member of the set.
*
CONTAINS is supported for lists: When evaluating "a CONTAINS
* b
", "a
" can be a list; however, "b
"
* cannot be a set, a map, or a list.
-
*
NOT_CONTAINS
: Checks for absence of a subsequence, or
* absence of a value in a set.
AttributeValueList can contain
* only one AttributeValue element of type String, Number, or
* Binary (not a set type). If the target attribute of the comparison is
* a String, then the operator checks for the absence of a substring
* match. If the target attribute of the comparison is Binary, then the
* operator checks for the absence of a subsequence of the target that
* matches the input. If the target attribute of the comparison is a set
* ("SS
", "NS
", or "BS
"), then the
* operator evaluates to true if it does not find an exact match
* with any member of the set.
NOT_CONTAINS is supported for lists:
* When evaluating "a NOT CONTAINS b
", "a
" can
* be a list; however, "b
" cannot be a set, a map, or a
* list.
-
BEGINS_WITH
: Checks for a prefix.
*
AttributeValueList can contain only one
* AttributeValue of type String or Binary (not a Number or a set
* type). The target attribute of the comparison must be of type String
* or Binary (not a Number or a set type).
-
*
IN
: Checks for matching elements within two sets.
*
AttributeValueList can contain one or more
* AttributeValue elements of type String, Number, or Binary (not
* a set type). These attributes are compared against an existing set
* type attribute of an item. If any elements of the input set are
* present in the item attribute, the expression evaluates to true.
* -
BETWEEN
: Greater than or equal to the first
* value, and less than or equal to the second value.
*
AttributeValueList must contain two AttributeValue
* elements of the same type, either String, Number, or Binary (not a set
* type). A target attribute matches if the target value is greater than,
* or equal to, the first element and less than, or equal to, the second
* element. If an item contains an AttributeValue element of a
* different type than the one provided in the request, the value does
* not match. For example, {"S":"6"}
does not compare to
* {"N":"6"}
. Also, {"N":"6"}
does not compare
* to {"NS":["6", "2", "1"]}
For
* usage examples of AttributeValueList and
* ComparisonOperator, see Legacy
* Conditional Parameters in the Amazon DynamoDB Developer
* Guide.
For backward compatibility with previous DynamoDB
* releases, the following parameters can be used instead of
* AttributeValueList and ComparisonOperator:
-
*
Value - A value for DynamoDB to compare with an attribute.
*
-
Exists - A Boolean value that causes DynamoDB to
* evaluate the value before attempting the conditional operation:
* -
If Exists is true
, DynamoDB will check to
* see if that attribute value already exists in the table. If it is
* found, then the condition evaluates to true; otherwise the condition
* evaluate to false.
If Exists is
* false
, DynamoDB assumes that the attribute value does
* not exist in the table. If in fact the value does not exist,
* then the assumption is valid and the condition evaluates to true. If
* the value is found, despite the assumption that it does not exist, the
* condition evaluates to false.
Note that the default
* value for Exists is true
.
The
* Value and Exists parameters are incompatible with
* AttributeValueList and ComparisonOperator. Note that if
* you use both sets of parameters at once, DynamoDB will return a
* ValidationException exception. This parameter does not
* support attributes of type List or Map.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public UpdateItemRequest withExpected(java.util.Map expected) {
setExpected(expected);
return this;
}
/**
* This is a legacy parameter, for backward compatibility.
* New applications should use ConditionExpression instead. Do
* not combine legacy parameters and expression parameters in a single
* API call; otherwise, DynamoDB will return a ValidationException
* exception.
A map of attribute/condition pairs.
* Expected provides a conditional block for the UpdateItem
* operation.
Each element of Expected consists of an attribute
* name, a comparison operator, and one or more values. DynamoDB compares
* the attribute with the value(s) you supplied, using the comparison
* operator. For each Expected element, the result of the
* evaluation is either true or false.
If you specify more than one
* element in the Expected map, then by default all of the
* conditions must evaluate to true. In other words, the conditions are
* ANDed together. (You can use the ConditionalOperator parameter
* to OR the conditions instead. If you do this, then at least one of the
* conditions must evaluate to true, rather than all of them.)
If the
* Expected map evaluates to true, then the conditional operation
* succeeds; otherwise, it fails.
Expected contains the
* following:
-
AttributeValueList - One or more values
* to evaluate against the supplied attribute. The number of values in
* the list depends on the ComparisonOperator being used.
For
* type Number, value comparisons are numeric.
String value
* comparisons for greater than, equals, or less than are based on ASCII
* character code values. For example, a
is greater than
* A
, and a
is greater than B
. For
* a list of code values, see http://en.wikipedia.org/wiki/ASCII#ASCII_printable_characters.
*
For type Binary, DynamoDB treats each byte of the binary data as
* unsigned when it compares binary values.
-
*
ComparisonOperator - A comparator for evaluating attributes
* in the AttributeValueList. When performing the comparison,
* DynamoDB uses strongly consistent reads.
The following comparison
* operators are available:
EQ | NE | LE | LT | GE | GT |
* NOT_NULL | NULL | CONTAINS | NOT_CONTAINS | BEGINS_WITH | IN |
* BETWEEN
The following are descriptions of each comparison
* operator.
-
EQ
: Equal. EQ
is
* supported for all datatypes, including lists and maps.
*
AttributeValueList can contain only one
* AttributeValue element of type String, Number, Binary, String
* Set, Number Set, or Binary Set. If an item contains an
* AttributeValue element of a different type than the one
* provided in the request, the value does not match. For example,
* {"S":"6"}
does not equal {"N":"6"}
. Also,
* {"N":"6"}
does not equal {"NS":["6", "2",
* "1"]}
.
-
NE
: Not equal.
* NE
is supported for all datatypes, including lists and
* maps.
AttributeValueList can contain only one
* AttributeValue of type String, Number, Binary, String Set,
* Number Set, or Binary Set. If an item contains an
* AttributeValue of a different type than the one provided in the
* request, the value does not match. For example, {"S":"6"}
* does not equal {"N":"6"}
. Also, {"N":"6"}
* does not equal {"NS":["6", "2", "1"]}
.
-
*
LE
: Less than or equal.
AttributeValueList
* can contain only one AttributeValue element of type String,
* Number, or Binary (not a set type). If an item contains an
* AttributeValue element of a different type than the one
* provided in the request, the value does not match. For example,
* {"S":"6"}
does not equal {"N":"6"}
. Also,
* {"N":"6"}
does not compare to {"NS":["6", "2",
* "1"]}
.
-
LT
: Less than.
*
AttributeValueList can contain only one
* AttributeValue of type String, Number, or Binary (not a set
* type). If an item contains an AttributeValue element of a
* different type than the one provided in the request, the value does
* not match. For example, {"S":"6"}
does not equal
* {"N":"6"}
. Also, {"N":"6"}
does not compare
* to {"NS":["6", "2", "1"]}
.
-
*
GE
: Greater than or equal.
*
AttributeValueList can contain only one
* AttributeValue element of type String, Number, or Binary (not a
* set type). If an item contains an AttributeValue element of a
* different type than the one provided in the request, the value does
* not match. For example, {"S":"6"}
does not equal
* {"N":"6"}
. Also, {"N":"6"}
does not compare
* to {"NS":["6", "2", "1"]}
.
-
*
GT
: Greater than.
AttributeValueList can
* contain only one AttributeValue element of type String, Number,
* or Binary (not a set type). If an item contains an
* AttributeValue element of a different type than the one
* provided in the request, the value does not match. For example,
* {"S":"6"}
does not equal {"N":"6"}
. Also,
* {"N":"6"}
does not compare to {"NS":["6", "2",
* "1"]}
.
-
NOT_NULL
: The attribute
* exists. NOT_NULL
is supported for all datatypes,
* including lists and maps. This operator tests for the
* existence of an attribute, not its data type. If the data type of
* attribute "a
" is null, and you evaluate it using
* NOT_NULL
, the result is a Boolean true. This
* result is because the attribute "a
" exists; its data type
* is not relevant to the NOT_NULL
comparison operator.
*
-
NULL
: The attribute does not
* exist. NULL
is supported for all datatypes, including
* lists and maps. This operator tests for the nonexistence of
* an attribute, not its data type. If the data type of attribute
* "a
" is null, and you evaluate it using NULL
,
* the result is a Boolean false. This is because the attribute
* "a
" exists; its data type is not relevant to the
* NULL
comparison operator.
-
*
CONTAINS
: Checks for a subsequence, or value in a
* set.
AttributeValueList can contain only one
* AttributeValue element of type String, Number, or Binary (not a
* set type). If the target attribute of the comparison is of type
* String, then the operator checks for a substring match. If the target
* attribute of the comparison is of type Binary, then the operator looks
* for a subsequence of the target that matches the input. If the target
* attribute of the comparison is a set ("SS
",
* "NS
", or "BS
"), then the operator evaluates
* to true if it finds an exact match with any member of the set.
*
CONTAINS is supported for lists: When evaluating "a CONTAINS
* b
", "a
" can be a list; however, "b
"
* cannot be a set, a map, or a list.
-
*
NOT_CONTAINS
: Checks for absence of a subsequence, or
* absence of a value in a set.
AttributeValueList can contain
* only one AttributeValue element of type String, Number, or
* Binary (not a set type). If the target attribute of the comparison is
* a String, then the operator checks for the absence of a substring
* match. If the target attribute of the comparison is Binary, then the
* operator checks for the absence of a subsequence of the target that
* matches the input. If the target attribute of the comparison is a set
* ("SS
", "NS
", or "BS
"), then the
* operator evaluates to true if it does not find an exact match
* with any member of the set.
NOT_CONTAINS is supported for lists:
* When evaluating "a NOT CONTAINS b
", "a
" can
* be a list; however, "b
" cannot be a set, a map, or a
* list.
-
BEGINS_WITH
: Checks for a prefix.
*
AttributeValueList can contain only one
* AttributeValue of type String or Binary (not a Number or a set
* type). The target attribute of the comparison must be of type String
* or Binary (not a Number or a set type).
-
*
IN
: Checks for matching elements within two sets.
*
AttributeValueList can contain one or more
* AttributeValue elements of type String, Number, or Binary (not
* a set type). These attributes are compared against an existing set
* type attribute of an item. If any elements of the input set are
* present in the item attribute, the expression evaluates to true.
* -
BETWEEN
: Greater than or equal to the first
* value, and less than or equal to the second value.
*
AttributeValueList must contain two AttributeValue
* elements of the same type, either String, Number, or Binary (not a set
* type). A target attribute matches if the target value is greater than,
* or equal to, the first element and less than, or equal to, the second
* element. If an item contains an AttributeValue element of a
* different type than the one provided in the request, the value does
* not match. For example, {"S":"6"}
does not compare to
* {"N":"6"}
. Also, {"N":"6"}
does not compare
* to {"NS":["6", "2", "1"]}
For
* usage examples of AttributeValueList and
* ComparisonOperator, see Legacy
* Conditional Parameters in the Amazon DynamoDB Developer
* Guide.
For backward compatibility with previous DynamoDB
* releases, the following parameters can be used instead of
* AttributeValueList and ComparisonOperator:
-
*
Value - A value for DynamoDB to compare with an attribute.
*
-
Exists - A Boolean value that causes DynamoDB to
* evaluate the value before attempting the conditional operation:
* -
If Exists is true
, DynamoDB will check to
* see if that attribute value already exists in the table. If it is
* found, then the condition evaluates to true; otherwise the condition
* evaluate to false.
If Exists is
* false
, DynamoDB assumes that the attribute value does
* not exist in the table. If in fact the value does not exist,
* then the assumption is valid and the condition evaluates to true. If
* the value is found, despite the assumption that it does not exist, the
* condition evaluates to false.
Note that the default
* value for Exists is true
.
The
* Value and Exists parameters are incompatible with
* AttributeValueList and ComparisonOperator. Note that if
* you use both sets of parameters at once, DynamoDB will return a
* ValidationException exception. This parameter does not
* support attributes of type List or Map.
*
* The method adds a new key-value pair into Expected parameter, and
* returns a reference to this object so that method calls can be chained
* together.
*
* @param key The key of the entry to be added into Expected.
* @param value The corresponding value of the entry to be added into Expected.
*/
public UpdateItemRequest addExpectedEntry(String key, ExpectedAttributeValue value) {
if (null == this.expected) {
this.expected = new java.util.HashMap();
}
if (this.expected.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.expected.put(key, value);
return this;
}
/**
* Removes all the entries added into Expected.
*
* Returns a reference to this object so that method calls can be chained together.
*/
public UpdateItemRequest clearExpectedEntries() {
this.expected = null;
return this;
}
/**
* This is a legacy parameter, for backward compatibility.
* New applications should use ConditionExpression instead. Do not
* combine legacy parameters and expression parameters in a single API
* call; otherwise, DynamoDB will return a ValidationException
* exception.
A logical operator to apply to the
* conditions in the Expected map:
AND
-
* If all of the conditions evaluate to true, then the entire map
* evaluates to true.
OR
- If at least one of
* the conditions evaluate to true, then the entire map evaluates to
* true.
If you omit ConditionalOperator, then
* AND
is the default.
The operation will succeed only if
* the entire map evaluates to true. This parameter does not
* support attributes of type List or Map.
*
* Constraints:
* Allowed Values: AND, OR
*
* @return This is a legacy parameter, for backward compatibility.
* New applications should use ConditionExpression instead. Do not
* combine legacy parameters and expression parameters in a single API
* call; otherwise, DynamoDB will return a ValidationException
* exception.
A logical operator to apply to the
* conditions in the Expected map:
AND
-
* If all of the conditions evaluate to true, then the entire map
* evaluates to true.
OR
- If at least one of
* the conditions evaluate to true, then the entire map evaluates to
* true.
If you omit ConditionalOperator, then
* AND
is the default.
The operation will succeed only if
* the entire map evaluates to true. This parameter does not
* support attributes of type List or Map.
*
* @see ConditionalOperator
*/
public String getConditionalOperator() {
return conditionalOperator;
}
/**
* This is a legacy parameter, for backward compatibility.
* New applications should use ConditionExpression instead. Do not
* combine legacy parameters and expression parameters in a single API
* call; otherwise, DynamoDB will return a ValidationException
* exception.
A logical operator to apply to the
* conditions in the Expected map:
AND
-
* If all of the conditions evaluate to true, then the entire map
* evaluates to true.
OR
- If at least one of
* the conditions evaluate to true, then the entire map evaluates to
* true.
If you omit ConditionalOperator, then
* AND
is the default.
The operation will succeed only if
* the entire map evaluates to true. This parameter does not
* support attributes of type List or Map.
*
* Constraints:
* Allowed Values: AND, OR
*
* @param conditionalOperator This is a legacy parameter, for backward compatibility.
* New applications should use ConditionExpression instead. Do not
* combine legacy parameters and expression parameters in a single API
* call; otherwise, DynamoDB will return a ValidationException
* exception.
A logical operator to apply to the
* conditions in the Expected map:
AND
-
* If all of the conditions evaluate to true, then the entire map
* evaluates to true.
OR
- If at least one of
* the conditions evaluate to true, then the entire map evaluates to
* true.
If you omit ConditionalOperator, then
* AND
is the default.
The operation will succeed only if
* the entire map evaluates to true. This parameter does not
* support attributes of type List or Map.
*
* @see ConditionalOperator
*/
public void setConditionalOperator(String conditionalOperator) {
this.conditionalOperator = conditionalOperator;
}
/**
* This is a legacy parameter, for backward compatibility.
* New applications should use ConditionExpression instead. Do not
* combine legacy parameters and expression parameters in a single API
* call; otherwise, DynamoDB will return a ValidationException
* exception.
A logical operator to apply to the
* conditions in the Expected map:
AND
-
* If all of the conditions evaluate to true, then the entire map
* evaluates to true.
OR
- If at least one of
* the conditions evaluate to true, then the entire map evaluates to
* true.
If you omit ConditionalOperator, then
* AND
is the default.
The operation will succeed only if
* the entire map evaluates to true. This parameter does not
* support attributes of type List or Map.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Allowed Values: AND, OR
*
* @param conditionalOperator This is a legacy parameter, for backward compatibility.
* New applications should use ConditionExpression instead. Do not
* combine legacy parameters and expression parameters in a single API
* call; otherwise, DynamoDB will return a ValidationException
* exception.
A logical operator to apply to the
* conditions in the Expected map:
AND
-
* If all of the conditions evaluate to true, then the entire map
* evaluates to true.
OR
- If at least one of
* the conditions evaluate to true, then the entire map evaluates to
* true.
If you omit ConditionalOperator, then
* AND
is the default.
The operation will succeed only if
* the entire map evaluates to true. This parameter does not
* support attributes of type List or Map.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*
* @see ConditionalOperator
*/
public UpdateItemRequest withConditionalOperator(String conditionalOperator) {
this.conditionalOperator = conditionalOperator;
return this;
}
/**
* This is a legacy parameter, for backward compatibility.
* New applications should use ConditionExpression instead. Do not
* combine legacy parameters and expression parameters in a single API
* call; otherwise, DynamoDB will return a ValidationException
* exception.
A logical operator to apply to the
* conditions in the Expected map:
AND
-
* If all of the conditions evaluate to true, then the entire map
* evaluates to true.
OR
- If at least one of
* the conditions evaluate to true, then the entire map evaluates to
* true.
If you omit ConditionalOperator, then
* AND
is the default.
The operation will succeed only if
* the entire map evaluates to true. This parameter does not
* support attributes of type List or Map.
*
* Constraints:
* Allowed Values: AND, OR
*
* @param conditionalOperator This is a legacy parameter, for backward compatibility.
* New applications should use ConditionExpression instead. Do not
* combine legacy parameters and expression parameters in a single API
* call; otherwise, DynamoDB will return a ValidationException
* exception.
A logical operator to apply to the
* conditions in the Expected map:
AND
-
* If all of the conditions evaluate to true, then the entire map
* evaluates to true.
OR
- If at least one of
* the conditions evaluate to true, then the entire map evaluates to
* true.
If you omit ConditionalOperator, then
* AND
is the default.
The operation will succeed only if
* the entire map evaluates to true. This parameter does not
* support attributes of type List or Map.
*
* @see ConditionalOperator
*/
public void setConditionalOperator(ConditionalOperator conditionalOperator) {
this.conditionalOperator = conditionalOperator.toString();
}
/**
* This is a legacy parameter, for backward compatibility.
* New applications should use ConditionExpression instead. Do not
* combine legacy parameters and expression parameters in a single API
* call; otherwise, DynamoDB will return a ValidationException
* exception.
A logical operator to apply to the
* conditions in the Expected map:
AND
-
* If all of the conditions evaluate to true, then the entire map
* evaluates to true.
OR
- If at least one of
* the conditions evaluate to true, then the entire map evaluates to
* true.
If you omit ConditionalOperator, then
* AND
is the default.
The operation will succeed only if
* the entire map evaluates to true. This parameter does not
* support attributes of type List or Map.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Allowed Values: AND, OR
*
* @param conditionalOperator This is a legacy parameter, for backward compatibility.
* New applications should use ConditionExpression instead. Do not
* combine legacy parameters and expression parameters in a single API
* call; otherwise, DynamoDB will return a ValidationException
* exception.
A logical operator to apply to the
* conditions in the Expected map:
AND
-
* If all of the conditions evaluate to true, then the entire map
* evaluates to true.
OR
- If at least one of
* the conditions evaluate to true, then the entire map evaluates to
* true.
If you omit ConditionalOperator, then
* AND
is the default.
The operation will succeed only if
* the entire map evaluates to true. This parameter does not
* support attributes of type List or Map.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*
* @see ConditionalOperator
*/
public UpdateItemRequest withConditionalOperator(ConditionalOperator conditionalOperator) {
this.conditionalOperator = conditionalOperator.toString();
return this;
}
/**
* Use ReturnValues if you want to get the item attributes as they
* appeared either before or after they were updated. For
* UpdateItem, the valid values are:
-
*
NONE
- If ReturnValues is not specified, or if
* its value is NONE
, then nothing is returned. (This
* setting is the default for ReturnValues.)
-
*
ALL_OLD
- If UpdateItem overwrote an attribute
* name-value pair, then the content of the old item is returned.
* -
UPDATED_OLD
- The old versions of only the
* updated attributes are returned.
-
ALL_NEW
-
* All of the attributes of the new version of the item are returned.
*
-
UPDATED_NEW
- The new versions of only the
* updated attributes are returned.
There is no additional
* cost associated with requesting a return value aside from the small
* network and processing overhead of receiving a larger response. No
* Read Capacity Units are consumed.
Values returned are strongly
* consistent
*
* Constraints:
* Allowed Values: NONE, ALL_OLD, UPDATED_OLD, ALL_NEW, UPDATED_NEW
*
* @return Use ReturnValues if you want to get the item attributes as they
* appeared either before or after they were updated. For
* UpdateItem, the valid values are:
-
*
NONE
- If ReturnValues is not specified, or if
* its value is NONE
, then nothing is returned. (This
* setting is the default for ReturnValues.)
-
*
ALL_OLD
- If UpdateItem overwrote an attribute
* name-value pair, then the content of the old item is returned.
* -
UPDATED_OLD
- The old versions of only the
* updated attributes are returned.
-
ALL_NEW
-
* All of the attributes of the new version of the item are returned.
*
-
UPDATED_NEW
- The new versions of only the
* updated attributes are returned.
There is no additional
* cost associated with requesting a return value aside from the small
* network and processing overhead of receiving a larger response. No
* Read Capacity Units are consumed.
Values returned are strongly
* consistent
*
* @see ReturnValue
*/
public String getReturnValues() {
return returnValues;
}
/**
* Use ReturnValues if you want to get the item attributes as they
* appeared either before or after they were updated. For
* UpdateItem, the valid values are:
-
*
NONE
- If ReturnValues is not specified, or if
* its value is NONE
, then nothing is returned. (This
* setting is the default for ReturnValues.)
-
*
ALL_OLD
- If UpdateItem overwrote an attribute
* name-value pair, then the content of the old item is returned.
* -
UPDATED_OLD
- The old versions of only the
* updated attributes are returned.
-
ALL_NEW
-
* All of the attributes of the new version of the item are returned.
*
-
UPDATED_NEW
- The new versions of only the
* updated attributes are returned.
There is no additional
* cost associated with requesting a return value aside from the small
* network and processing overhead of receiving a larger response. No
* Read Capacity Units are consumed.
Values returned are strongly
* consistent
*
* Constraints:
* Allowed Values: NONE, ALL_OLD, UPDATED_OLD, ALL_NEW, UPDATED_NEW
*
* @param returnValues Use ReturnValues if you want to get the item attributes as they
* appeared either before or after they were updated. For
* UpdateItem, the valid values are:
-
*
NONE
- If ReturnValues is not specified, or if
* its value is NONE
, then nothing is returned. (This
* setting is the default for ReturnValues.)
-
*
ALL_OLD
- If UpdateItem overwrote an attribute
* name-value pair, then the content of the old item is returned.
* -
UPDATED_OLD
- The old versions of only the
* updated attributes are returned.
-
ALL_NEW
-
* All of the attributes of the new version of the item are returned.
*
-
UPDATED_NEW
- The new versions of only the
* updated attributes are returned.
There is no additional
* cost associated with requesting a return value aside from the small
* network and processing overhead of receiving a larger response. No
* Read Capacity Units are consumed.
Values returned are strongly
* consistent
*
* @see ReturnValue
*/
public void setReturnValues(String returnValues) {
this.returnValues = returnValues;
}
/**
* Use ReturnValues if you want to get the item attributes as they
* appeared either before or after they were updated. For
* UpdateItem, the valid values are:
-
*
NONE
- If ReturnValues is not specified, or if
* its value is NONE
, then nothing is returned. (This
* setting is the default for ReturnValues.)
-
*
ALL_OLD
- If UpdateItem overwrote an attribute
* name-value pair, then the content of the old item is returned.
* -
UPDATED_OLD
- The old versions of only the
* updated attributes are returned.
-
ALL_NEW
-
* All of the attributes of the new version of the item are returned.
*
-
UPDATED_NEW
- The new versions of only the
* updated attributes are returned.
There is no additional
* cost associated with requesting a return value aside from the small
* network and processing overhead of receiving a larger response. No
* Read Capacity Units are consumed.
Values returned are strongly
* consistent
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Allowed Values: NONE, ALL_OLD, UPDATED_OLD, ALL_NEW, UPDATED_NEW
*
* @param returnValues Use ReturnValues if you want to get the item attributes as they
* appeared either before or after they were updated. For
* UpdateItem, the valid values are:
-
*
NONE
- If ReturnValues is not specified, or if
* its value is NONE
, then nothing is returned. (This
* setting is the default for ReturnValues.)
-
*
ALL_OLD
- If UpdateItem overwrote an attribute
* name-value pair, then the content of the old item is returned.
* -
UPDATED_OLD
- The old versions of only the
* updated attributes are returned.
-
ALL_NEW
-
* All of the attributes of the new version of the item are returned.
*
-
UPDATED_NEW
- The new versions of only the
* updated attributes are returned.
There is no additional
* cost associated with requesting a return value aside from the small
* network and processing overhead of receiving a larger response. No
* Read Capacity Units are consumed.
Values returned are strongly
* consistent
*
* @return A reference to this updated object so that method calls can be chained
* together.
*
* @see ReturnValue
*/
public UpdateItemRequest withReturnValues(String returnValues) {
this.returnValues = returnValues;
return this;
}
/**
* Use ReturnValues if you want to get the item attributes as they
* appeared either before or after they were updated. For
* UpdateItem, the valid values are:
-
*
NONE
- If ReturnValues is not specified, or if
* its value is NONE
, then nothing is returned. (This
* setting is the default for ReturnValues.)
-
*
ALL_OLD
- If UpdateItem overwrote an attribute
* name-value pair, then the content of the old item is returned.
* -
UPDATED_OLD
- The old versions of only the
* updated attributes are returned.
-
ALL_NEW
-
* All of the attributes of the new version of the item are returned.
*
-
UPDATED_NEW
- The new versions of only the
* updated attributes are returned.
There is no additional
* cost associated with requesting a return value aside from the small
* network and processing overhead of receiving a larger response. No
* Read Capacity Units are consumed.
Values returned are strongly
* consistent
*
* Constraints:
* Allowed Values: NONE, ALL_OLD, UPDATED_OLD, ALL_NEW, UPDATED_NEW
*
* @param returnValues Use ReturnValues if you want to get the item attributes as they
* appeared either before or after they were updated. For
* UpdateItem, the valid values are:
-
*
NONE
- If ReturnValues is not specified, or if
* its value is NONE
, then nothing is returned. (This
* setting is the default for ReturnValues.)
-
*
ALL_OLD
- If UpdateItem overwrote an attribute
* name-value pair, then the content of the old item is returned.
* -
UPDATED_OLD
- The old versions of only the
* updated attributes are returned.
-
ALL_NEW
-
* All of the attributes of the new version of the item are returned.
*
-
UPDATED_NEW
- The new versions of only the
* updated attributes are returned.
There is no additional
* cost associated with requesting a return value aside from the small
* network and processing overhead of receiving a larger response. No
* Read Capacity Units are consumed.
Values returned are strongly
* consistent
*
* @see ReturnValue
*/
public void setReturnValues(ReturnValue returnValues) {
this.returnValues = returnValues.toString();
}
/**
* Use ReturnValues if you want to get the item attributes as they
* appeared either before or after they were updated. For
* UpdateItem, the valid values are:
-
*
NONE
- If ReturnValues is not specified, or if
* its value is NONE
, then nothing is returned. (This
* setting is the default for ReturnValues.)
-
*
ALL_OLD
- If UpdateItem overwrote an attribute
* name-value pair, then the content of the old item is returned.
* -
UPDATED_OLD
- The old versions of only the
* updated attributes are returned.
-
ALL_NEW
-
* All of the attributes of the new version of the item are returned.
*
-
UPDATED_NEW
- The new versions of only the
* updated attributes are returned.
There is no additional
* cost associated with requesting a return value aside from the small
* network and processing overhead of receiving a larger response. No
* Read Capacity Units are consumed.
Values returned are strongly
* consistent
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Allowed Values: NONE, ALL_OLD, UPDATED_OLD, ALL_NEW, UPDATED_NEW
*
* @param returnValues Use ReturnValues if you want to get the item attributes as they
* appeared either before or after they were updated. For
* UpdateItem, the valid values are:
-
*
NONE
- If ReturnValues is not specified, or if
* its value is NONE
, then nothing is returned. (This
* setting is the default for ReturnValues.)
-
*
ALL_OLD
- If UpdateItem overwrote an attribute
* name-value pair, then the content of the old item is returned.
* -
UPDATED_OLD
- The old versions of only the
* updated attributes are returned.
-
ALL_NEW
-
* All of the attributes of the new version of the item are returned.
*
-
UPDATED_NEW
- The new versions of only the
* updated attributes are returned.
There is no additional
* cost associated with requesting a return value aside from the small
* network and processing overhead of receiving a larger response. No
* Read Capacity Units are consumed.
Values returned are strongly
* consistent
*
* @return A reference to this updated object so that method calls can be chained
* together.
*
* @see ReturnValue
*/
public UpdateItemRequest withReturnValues(ReturnValue returnValues) {
this.returnValues = returnValues.toString();
return this;
}
/**
* Determines the level of detail about provisioned throughput
* consumption that is returned in the response:
* INDEXES - The response includes the aggregate
* ConsumedCapacity for the operation, together with
* ConsumedCapacity for each table and secondary index that was
* accessed.
Note that some operations, such as GetItem and
* BatchGetItem, do not access any indexes at all. In these cases,
* specifying INDEXES will only return ConsumedCapacity
* information for table(s).
TOTAL - The response
* includes only the aggregate ConsumedCapacity for the
* operation.
NONE - No ConsumedCapacity
* details are included in the response.
*
* Constraints:
* Allowed Values: INDEXES, TOTAL, NONE
*
* @return Determines the level of detail about provisioned throughput
* consumption that is returned in the response:
* INDEXES - The response includes the aggregate
* ConsumedCapacity for the operation, together with
* ConsumedCapacity for each table and secondary index that was
* accessed.
Note that some operations, such as GetItem and
* BatchGetItem, do not access any indexes at all. In these cases,
* specifying INDEXES will only return ConsumedCapacity
* information for table(s).
TOTAL - The response
* includes only the aggregate ConsumedCapacity for the
* operation.
NONE - No ConsumedCapacity
* details are included in the response.
*
* @see ReturnConsumedCapacity
*/
public String getReturnConsumedCapacity() {
return returnConsumedCapacity;
}
/**
* Determines the level of detail about provisioned throughput
* consumption that is returned in the response:
* INDEXES - The response includes the aggregate
* ConsumedCapacity for the operation, together with
* ConsumedCapacity for each table and secondary index that was
* accessed.
Note that some operations, such as GetItem and
* BatchGetItem, do not access any indexes at all. In these cases,
* specifying INDEXES will only return ConsumedCapacity
* information for table(s).
TOTAL - The response
* includes only the aggregate ConsumedCapacity for the
* operation.
NONE - No ConsumedCapacity
* details are included in the response.
*
* Constraints:
* Allowed Values: INDEXES, TOTAL, NONE
*
* @param returnConsumedCapacity Determines the level of detail about provisioned throughput
* consumption that is returned in the response:
* INDEXES - The response includes the aggregate
* ConsumedCapacity for the operation, together with
* ConsumedCapacity for each table and secondary index that was
* accessed.
Note that some operations, such as GetItem and
* BatchGetItem, do not access any indexes at all. In these cases,
* specifying INDEXES will only return ConsumedCapacity
* information for table(s).
TOTAL - The response
* includes only the aggregate ConsumedCapacity for the
* operation.
NONE - No ConsumedCapacity
* details are included in the response.
*
* @see ReturnConsumedCapacity
*/
public void setReturnConsumedCapacity(String returnConsumedCapacity) {
this.returnConsumedCapacity = returnConsumedCapacity;
}
/**
* Determines the level of detail about provisioned throughput
* consumption that is returned in the response:
* INDEXES - The response includes the aggregate
* ConsumedCapacity for the operation, together with
* ConsumedCapacity for each table and secondary index that was
* accessed.
Note that some operations, such as GetItem and
* BatchGetItem, do not access any indexes at all. In these cases,
* specifying INDEXES will only return ConsumedCapacity
* information for table(s).
TOTAL - The response
* includes only the aggregate ConsumedCapacity for the
* operation.
NONE - No ConsumedCapacity
* details are included in the response.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Allowed Values: INDEXES, TOTAL, NONE
*
* @param returnConsumedCapacity Determines the level of detail about provisioned throughput
* consumption that is returned in the response:
* INDEXES - The response includes the aggregate
* ConsumedCapacity for the operation, together with
* ConsumedCapacity for each table and secondary index that was
* accessed.
Note that some operations, such as GetItem and
* BatchGetItem, do not access any indexes at all. In these cases,
* specifying INDEXES will only return ConsumedCapacity
* information for table(s).
TOTAL - The response
* includes only the aggregate ConsumedCapacity for the
* operation.
NONE - No ConsumedCapacity
* details are included in the response.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*
* @see ReturnConsumedCapacity
*/
public UpdateItemRequest withReturnConsumedCapacity(String returnConsumedCapacity) {
this.returnConsumedCapacity = returnConsumedCapacity;
return this;
}
/**
* Determines the level of detail about provisioned throughput
* consumption that is returned in the response:
* INDEXES - The response includes the aggregate
* ConsumedCapacity for the operation, together with
* ConsumedCapacity for each table and secondary index that was
* accessed.
Note that some operations, such as GetItem and
* BatchGetItem, do not access any indexes at all. In these cases,
* specifying INDEXES will only return ConsumedCapacity
* information for table(s).
TOTAL - The response
* includes only the aggregate ConsumedCapacity for the
* operation.
NONE - No ConsumedCapacity
* details are included in the response.
*
* Constraints:
* Allowed Values: INDEXES, TOTAL, NONE
*
* @param returnConsumedCapacity Determines the level of detail about provisioned throughput
* consumption that is returned in the response:
* INDEXES - The response includes the aggregate
* ConsumedCapacity for the operation, together with
* ConsumedCapacity for each table and secondary index that was
* accessed.
Note that some operations, such as GetItem and
* BatchGetItem, do not access any indexes at all. In these cases,
* specifying INDEXES will only return ConsumedCapacity
* information for table(s).
TOTAL - The response
* includes only the aggregate ConsumedCapacity for the
* operation.
NONE - No ConsumedCapacity
* details are included in the response.
*
* @see ReturnConsumedCapacity
*/
public void setReturnConsumedCapacity(ReturnConsumedCapacity returnConsumedCapacity) {
this.returnConsumedCapacity = returnConsumedCapacity.toString();
}
/**
* Determines the level of detail about provisioned throughput
* consumption that is returned in the response:
* INDEXES - The response includes the aggregate
* ConsumedCapacity for the operation, together with
* ConsumedCapacity for each table and secondary index that was
* accessed.
Note that some operations, such as GetItem and
* BatchGetItem, do not access any indexes at all. In these cases,
* specifying INDEXES will only return ConsumedCapacity
* information for table(s).
TOTAL - The response
* includes only the aggregate ConsumedCapacity for the
* operation.
NONE - No ConsumedCapacity
* details are included in the response.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Allowed Values: INDEXES, TOTAL, NONE
*
* @param returnConsumedCapacity Determines the level of detail about provisioned throughput
* consumption that is returned in the response:
* INDEXES - The response includes the aggregate
* ConsumedCapacity for the operation, together with
* ConsumedCapacity for each table and secondary index that was
* accessed.
Note that some operations, such as GetItem and
* BatchGetItem, do not access any indexes at all. In these cases,
* specifying INDEXES will only return ConsumedCapacity
* information for table(s).
TOTAL - The response
* includes only the aggregate ConsumedCapacity for the
* operation.
NONE - No ConsumedCapacity
* details are included in the response.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*
* @see ReturnConsumedCapacity
*/
public UpdateItemRequest withReturnConsumedCapacity(ReturnConsumedCapacity returnConsumedCapacity) {
this.returnConsumedCapacity = returnConsumedCapacity.toString();
return this;
}
/**
* Determines whether item collection metrics are returned. If set to
* SIZE
, the response includes statistics about item
* collections, if any, that were modified during the operation are
* returned in the response. If set to NONE
(the default),
* no statistics are returned.
*
* Constraints:
* Allowed Values: SIZE, NONE
*
* @return Determines whether item collection metrics are returned. If set to
* SIZE
, the response includes statistics about item
* collections, if any, that were modified during the operation are
* returned in the response. If set to NONE
(the default),
* no statistics are returned.
*
* @see ReturnItemCollectionMetrics
*/
public String getReturnItemCollectionMetrics() {
return returnItemCollectionMetrics;
}
/**
* Determines whether item collection metrics are returned. If set to
* SIZE
, the response includes statistics about item
* collections, if any, that were modified during the operation are
* returned in the response. If set to NONE
(the default),
* no statistics are returned.
*
* Constraints:
* Allowed Values: SIZE, NONE
*
* @param returnItemCollectionMetrics Determines whether item collection metrics are returned. If set to
* SIZE
, the response includes statistics about item
* collections, if any, that were modified during the operation are
* returned in the response. If set to NONE
(the default),
* no statistics are returned.
*
* @see ReturnItemCollectionMetrics
*/
public void setReturnItemCollectionMetrics(String returnItemCollectionMetrics) {
this.returnItemCollectionMetrics = returnItemCollectionMetrics;
}
/**
* Determines whether item collection metrics are returned. If set to
* SIZE
, the response includes statistics about item
* collections, if any, that were modified during the operation are
* returned in the response. If set to NONE
(the default),
* no statistics are returned.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Allowed Values: SIZE, NONE
*
* @param returnItemCollectionMetrics Determines whether item collection metrics are returned. If set to
* SIZE
, the response includes statistics about item
* collections, if any, that were modified during the operation are
* returned in the response. If set to NONE
(the default),
* no statistics are returned.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*
* @see ReturnItemCollectionMetrics
*/
public UpdateItemRequest withReturnItemCollectionMetrics(String returnItemCollectionMetrics) {
this.returnItemCollectionMetrics = returnItemCollectionMetrics;
return this;
}
/**
* Determines whether item collection metrics are returned. If set to
* SIZE
, the response includes statistics about item
* collections, if any, that were modified during the operation are
* returned in the response. If set to NONE
(the default),
* no statistics are returned.
*
* Constraints:
* Allowed Values: SIZE, NONE
*
* @param returnItemCollectionMetrics Determines whether item collection metrics are returned. If set to
* SIZE
, the response includes statistics about item
* collections, if any, that were modified during the operation are
* returned in the response. If set to NONE
(the default),
* no statistics are returned.
*
* @see ReturnItemCollectionMetrics
*/
public void setReturnItemCollectionMetrics(ReturnItemCollectionMetrics returnItemCollectionMetrics) {
this.returnItemCollectionMetrics = returnItemCollectionMetrics.toString();
}
/**
* Determines whether item collection metrics are returned. If set to
* SIZE
, the response includes statistics about item
* collections, if any, that were modified during the operation are
* returned in the response. If set to NONE
(the default),
* no statistics are returned.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Allowed Values: SIZE, NONE
*
* @param returnItemCollectionMetrics Determines whether item collection metrics are returned. If set to
* SIZE
, the response includes statistics about item
* collections, if any, that were modified during the operation are
* returned in the response. If set to NONE
(the default),
* no statistics are returned.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*
* @see ReturnItemCollectionMetrics
*/
public UpdateItemRequest withReturnItemCollectionMetrics(ReturnItemCollectionMetrics returnItemCollectionMetrics) {
this.returnItemCollectionMetrics = returnItemCollectionMetrics.toString();
return this;
}
/**
* An expression that defines one or more attributes to be updated, the
* action to be performed on them, and new value(s) for them.
The
* following action values are available for UpdateExpression.
*
-
SET
- Adds one or more attributes and values
* to an item. If any of these attribute already exist, they are replaced
* by the new values. You can also use SET
to add or
* subtract from an attribute that is of type Number. For example:
* SET myNum = myNum + :val
SET
supports the
* following functions:
if_not_exists (path,
* operand)
- if the item does not contain an attribute at the
* specified path, then if_not_exists
evaluates to operand;
* otherwise, it evaluates to path. You can use this function to avoid
* overwriting an attribute that may already be present in the item.
* list_append (operand, operand)
- evaluates to a
* list with a new element added to it. You can append the new element to
* the start or the end of the list by reversing the order of the
* operands.
These function names are case-sensitive.
* -
REMOVE
- Removes one or more attributes from an
* item.
-
ADD
- Adds the specified value to the
* item, if the attribute does not already exist. If the attribute does
* exist, then the behavior of ADD
depends on the data type
* of the attribute:
-
If the existing attribute is a number,
* and if Value is also a number, then Value is
* mathematically added to the existing attribute. If Value is a
* negative number, then it is subtracted from the existing attribute.
* If you use ADD
to increment or decrement a
* number value for an item that doesn't exist before the update,
* DynamoDB uses 0
as the initial value.
Similarly, if
* you use ADD
for an existing item to increment or
* decrement an attribute value that doesn't exist before the update,
* DynamoDB uses 0
as the initial value. For example,
* suppose that the item you want to update doesn't have an attribute
* named itemcount, but you decide to ADD
the number
* 3
to this attribute anyway. DynamoDB will create the
* itemcount attribute, set its initial value to 0
,
* and finally add 3
to it. The result will be a new
* itemcount attribute in the item, with a value of
* 3
.
-
If the existing data type is a
* set and if Value is also a set, then Value is added to
* the existing set. For example, if the attribute value is the set
* [1,2]
, and the ADD
action specified
* [3]
, then the final attribute value is
* [1,2,3]
. An error occurs if an ADD
action is
* specified for a set attribute and the attribute type specified does
* not match the existing set type.
Both sets must have the same
* primitive data type. For example, if the existing data type is a set
* of strings, the Value must also be a set of strings.
*
The ADD
action only supports Number
* and set data types. In addition, ADD
can only be used on
* top-level attributes, not nested attributes.
-
*
DELETE
- Deletes an element from a set.
If a set of
* values is specified, then those values are subtracted from the old
* set. For example, if the attribute value was the set
* [a,b,c]
and the DELETE
action specifies
* [a,c]
, then the final attribute value is
* [b]
. Specifying an empty set is an error.
* The DELETE
action only supports set data
* types. In addition, DELETE
can only be used on top-level
* attributes, not nested attributes.
You can
* have many actions in a single expression, such as the following:
* SET a=:value1, b=:value2 DELETE :value3, :value4, :value5
*
For more information on update expressions, see Modifying
* Items and Attributes in the Amazon DynamoDB Developer
* Guide. UpdateExpression replaces the legacy
* AttributeUpdates parameter.
*
* @return An expression that defines one or more attributes to be updated, the
* action to be performed on them, and new value(s) for them.
The
* following action values are available for UpdateExpression.
*
-
SET
- Adds one or more attributes and values
* to an item. If any of these attribute already exist, they are replaced
* by the new values. You can also use SET
to add or
* subtract from an attribute that is of type Number. For example:
* SET myNum = myNum + :val
SET
supports the
* following functions:
if_not_exists (path,
* operand)
- if the item does not contain an attribute at the
* specified path, then if_not_exists
evaluates to operand;
* otherwise, it evaluates to path. You can use this function to avoid
* overwriting an attribute that may already be present in the item.
* list_append (operand, operand)
- evaluates to a
* list with a new element added to it. You can append the new element to
* the start or the end of the list by reversing the order of the
* operands.
These function names are case-sensitive.
* -
REMOVE
- Removes one or more attributes from an
* item.
-
ADD
- Adds the specified value to the
* item, if the attribute does not already exist. If the attribute does
* exist, then the behavior of ADD
depends on the data type
* of the attribute:
-
If the existing attribute is a number,
* and if Value is also a number, then Value is
* mathematically added to the existing attribute. If Value is a
* negative number, then it is subtracted from the existing attribute.
* If you use ADD
to increment or decrement a
* number value for an item that doesn't exist before the update,
* DynamoDB uses 0
as the initial value.
Similarly, if
* you use ADD
for an existing item to increment or
* decrement an attribute value that doesn't exist before the update,
* DynamoDB uses 0
as the initial value. For example,
* suppose that the item you want to update doesn't have an attribute
* named itemcount, but you decide to ADD
the number
* 3
to this attribute anyway. DynamoDB will create the
* itemcount attribute, set its initial value to 0
,
* and finally add 3
to it. The result will be a new
* itemcount attribute in the item, with a value of
* 3
.
-
If the existing data type is a
* set and if Value is also a set, then Value is added to
* the existing set. For example, if the attribute value is the set
* [1,2]
, and the ADD
action specified
* [3]
, then the final attribute value is
* [1,2,3]
. An error occurs if an ADD
action is
* specified for a set attribute and the attribute type specified does
* not match the existing set type.
Both sets must have the same
* primitive data type. For example, if the existing data type is a set
* of strings, the Value must also be a set of strings.
*
The ADD
action only supports Number
* and set data types. In addition, ADD
can only be used on
* top-level attributes, not nested attributes.
-
*
DELETE
- Deletes an element from a set.
If a set of
* values is specified, then those values are subtracted from the old
* set. For example, if the attribute value was the set
* [a,b,c]
and the DELETE
action specifies
* [a,c]
, then the final attribute value is
* [b]
. Specifying an empty set is an error.
* The DELETE
action only supports set data
* types. In addition, DELETE
can only be used on top-level
* attributes, not nested attributes.
You can
* have many actions in a single expression, such as the following:
* SET a=:value1, b=:value2 DELETE :value3, :value4, :value5
*
For more information on update expressions, see Modifying
* Items and Attributes in the Amazon DynamoDB Developer
* Guide. UpdateExpression replaces the legacy
* AttributeUpdates parameter.
*/
public String getUpdateExpression() {
return updateExpression;
}
/**
* An expression that defines one or more attributes to be updated, the
* action to be performed on them, and new value(s) for them.
The
* following action values are available for UpdateExpression.
*
-
SET
- Adds one or more attributes and values
* to an item. If any of these attribute already exist, they are replaced
* by the new values. You can also use SET
to add or
* subtract from an attribute that is of type Number. For example:
* SET myNum = myNum + :val
SET
supports the
* following functions:
if_not_exists (path,
* operand)
- if the item does not contain an attribute at the
* specified path, then if_not_exists
evaluates to operand;
* otherwise, it evaluates to path. You can use this function to avoid
* overwriting an attribute that may already be present in the item.
* list_append (operand, operand)
- evaluates to a
* list with a new element added to it. You can append the new element to
* the start or the end of the list by reversing the order of the
* operands.
These function names are case-sensitive.
* -
REMOVE
- Removes one or more attributes from an
* item.
-
ADD
- Adds the specified value to the
* item, if the attribute does not already exist. If the attribute does
* exist, then the behavior of ADD
depends on the data type
* of the attribute:
-
If the existing attribute is a number,
* and if Value is also a number, then Value is
* mathematically added to the existing attribute. If Value is a
* negative number, then it is subtracted from the existing attribute.
* If you use ADD
to increment or decrement a
* number value for an item that doesn't exist before the update,
* DynamoDB uses 0
as the initial value.
Similarly, if
* you use ADD
for an existing item to increment or
* decrement an attribute value that doesn't exist before the update,
* DynamoDB uses 0
as the initial value. For example,
* suppose that the item you want to update doesn't have an attribute
* named itemcount, but you decide to ADD
the number
* 3
to this attribute anyway. DynamoDB will create the
* itemcount attribute, set its initial value to 0
,
* and finally add 3
to it. The result will be a new
* itemcount attribute in the item, with a value of
* 3
.
-
If the existing data type is a
* set and if Value is also a set, then Value is added to
* the existing set. For example, if the attribute value is the set
* [1,2]
, and the ADD
action specified
* [3]
, then the final attribute value is
* [1,2,3]
. An error occurs if an ADD
action is
* specified for a set attribute and the attribute type specified does
* not match the existing set type.
Both sets must have the same
* primitive data type. For example, if the existing data type is a set
* of strings, the Value must also be a set of strings.
*
The ADD
action only supports Number
* and set data types. In addition, ADD
can only be used on
* top-level attributes, not nested attributes.
-
*
DELETE
- Deletes an element from a set.
If a set of
* values is specified, then those values are subtracted from the old
* set. For example, if the attribute value was the set
* [a,b,c]
and the DELETE
action specifies
* [a,c]
, then the final attribute value is
* [b]
. Specifying an empty set is an error.
* The DELETE
action only supports set data
* types. In addition, DELETE
can only be used on top-level
* attributes, not nested attributes.
You can
* have many actions in a single expression, such as the following:
* SET a=:value1, b=:value2 DELETE :value3, :value4, :value5
*
For more information on update expressions, see Modifying
* Items and Attributes in the Amazon DynamoDB Developer
* Guide. UpdateExpression replaces the legacy
* AttributeUpdates parameter.
*
* @param updateExpression An expression that defines one or more attributes to be updated, the
* action to be performed on them, and new value(s) for them.
The
* following action values are available for UpdateExpression.
*
-
SET
- Adds one or more attributes and values
* to an item. If any of these attribute already exist, they are replaced
* by the new values. You can also use SET
to add or
* subtract from an attribute that is of type Number. For example:
* SET myNum = myNum + :val
SET
supports the
* following functions:
if_not_exists (path,
* operand)
- if the item does not contain an attribute at the
* specified path, then if_not_exists
evaluates to operand;
* otherwise, it evaluates to path. You can use this function to avoid
* overwriting an attribute that may already be present in the item.
* list_append (operand, operand)
- evaluates to a
* list with a new element added to it. You can append the new element to
* the start or the end of the list by reversing the order of the
* operands.
These function names are case-sensitive.
* -
REMOVE
- Removes one or more attributes from an
* item.
-
ADD
- Adds the specified value to the
* item, if the attribute does not already exist. If the attribute does
* exist, then the behavior of ADD
depends on the data type
* of the attribute:
-
If the existing attribute is a number,
* and if Value is also a number, then Value is
* mathematically added to the existing attribute. If Value is a
* negative number, then it is subtracted from the existing attribute.
* If you use ADD
to increment or decrement a
* number value for an item that doesn't exist before the update,
* DynamoDB uses 0
as the initial value.
Similarly, if
* you use ADD
for an existing item to increment or
* decrement an attribute value that doesn't exist before the update,
* DynamoDB uses 0
as the initial value. For example,
* suppose that the item you want to update doesn't have an attribute
* named itemcount, but you decide to ADD
the number
* 3
to this attribute anyway. DynamoDB will create the
* itemcount attribute, set its initial value to 0
,
* and finally add 3
to it. The result will be a new
* itemcount attribute in the item, with a value of
* 3
.
-
If the existing data type is a
* set and if Value is also a set, then Value is added to
* the existing set. For example, if the attribute value is the set
* [1,2]
, and the ADD
action specified
* [3]
, then the final attribute value is
* [1,2,3]
. An error occurs if an ADD
action is
* specified for a set attribute and the attribute type specified does
* not match the existing set type.
Both sets must have the same
* primitive data type. For example, if the existing data type is a set
* of strings, the Value must also be a set of strings.
*
The ADD
action only supports Number
* and set data types. In addition, ADD
can only be used on
* top-level attributes, not nested attributes.
-
*
DELETE
- Deletes an element from a set.
If a set of
* values is specified, then those values are subtracted from the old
* set. For example, if the attribute value was the set
* [a,b,c]
and the DELETE
action specifies
* [a,c]
, then the final attribute value is
* [b]
. Specifying an empty set is an error.
* The DELETE
action only supports set data
* types. In addition, DELETE
can only be used on top-level
* attributes, not nested attributes.
You can
* have many actions in a single expression, such as the following:
* SET a=:value1, b=:value2 DELETE :value3, :value4, :value5
*
For more information on update expressions, see Modifying
* Items and Attributes in the Amazon DynamoDB Developer
* Guide. UpdateExpression replaces the legacy
* AttributeUpdates parameter.
*/
public void setUpdateExpression(String updateExpression) {
this.updateExpression = updateExpression;
}
/**
* An expression that defines one or more attributes to be updated, the
* action to be performed on them, and new value(s) for them.
The
* following action values are available for UpdateExpression.
*
-
SET
- Adds one or more attributes and values
* to an item. If any of these attribute already exist, they are replaced
* by the new values. You can also use SET
to add or
* subtract from an attribute that is of type Number. For example:
* SET myNum = myNum + :val
SET
supports the
* following functions:
if_not_exists (path,
* operand)
- if the item does not contain an attribute at the
* specified path, then if_not_exists
evaluates to operand;
* otherwise, it evaluates to path. You can use this function to avoid
* overwriting an attribute that may already be present in the item.
* list_append (operand, operand)
- evaluates to a
* list with a new element added to it. You can append the new element to
* the start or the end of the list by reversing the order of the
* operands.
These function names are case-sensitive.
* -
REMOVE
- Removes one or more attributes from an
* item.
-
ADD
- Adds the specified value to the
* item, if the attribute does not already exist. If the attribute does
* exist, then the behavior of ADD
depends on the data type
* of the attribute:
-
If the existing attribute is a number,
* and if Value is also a number, then Value is
* mathematically added to the existing attribute. If Value is a
* negative number, then it is subtracted from the existing attribute.
* If you use ADD
to increment or decrement a
* number value for an item that doesn't exist before the update,
* DynamoDB uses 0
as the initial value.
Similarly, if
* you use ADD
for an existing item to increment or
* decrement an attribute value that doesn't exist before the update,
* DynamoDB uses 0
as the initial value. For example,
* suppose that the item you want to update doesn't have an attribute
* named itemcount, but you decide to ADD
the number
* 3
to this attribute anyway. DynamoDB will create the
* itemcount attribute, set its initial value to 0
,
* and finally add 3
to it. The result will be a new
* itemcount attribute in the item, with a value of
* 3
.
-
If the existing data type is a
* set and if Value is also a set, then Value is added to
* the existing set. For example, if the attribute value is the set
* [1,2]
, and the ADD
action specified
* [3]
, then the final attribute value is
* [1,2,3]
. An error occurs if an ADD
action is
* specified for a set attribute and the attribute type specified does
* not match the existing set type.
Both sets must have the same
* primitive data type. For example, if the existing data type is a set
* of strings, the Value must also be a set of strings.
*
The ADD
action only supports Number
* and set data types. In addition, ADD
can only be used on
* top-level attributes, not nested attributes.
-
*
DELETE
- Deletes an element from a set.
If a set of
* values is specified, then those values are subtracted from the old
* set. For example, if the attribute value was the set
* [a,b,c]
and the DELETE
action specifies
* [a,c]
, then the final attribute value is
* [b]
. Specifying an empty set is an error.
* The DELETE
action only supports set data
* types. In addition, DELETE
can only be used on top-level
* attributes, not nested attributes.
You can
* have many actions in a single expression, such as the following:
* SET a=:value1, b=:value2 DELETE :value3, :value4, :value5
*
For more information on update expressions, see Modifying
* Items and Attributes in the Amazon DynamoDB Developer
* Guide. UpdateExpression replaces the legacy
* AttributeUpdates parameter.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param updateExpression An expression that defines one or more attributes to be updated, the
* action to be performed on them, and new value(s) for them.
The
* following action values are available for UpdateExpression.
*
-
SET
- Adds one or more attributes and values
* to an item. If any of these attribute already exist, they are replaced
* by the new values. You can also use SET
to add or
* subtract from an attribute that is of type Number. For example:
* SET myNum = myNum + :val
SET
supports the
* following functions:
if_not_exists (path,
* operand)
- if the item does not contain an attribute at the
* specified path, then if_not_exists
evaluates to operand;
* otherwise, it evaluates to path. You can use this function to avoid
* overwriting an attribute that may already be present in the item.
* list_append (operand, operand)
- evaluates to a
* list with a new element added to it. You can append the new element to
* the start or the end of the list by reversing the order of the
* operands.
These function names are case-sensitive.
* -
REMOVE
- Removes one or more attributes from an
* item.
-
ADD
- Adds the specified value to the
* item, if the attribute does not already exist. If the attribute does
* exist, then the behavior of ADD
depends on the data type
* of the attribute:
-
If the existing attribute is a number,
* and if Value is also a number, then Value is
* mathematically added to the existing attribute. If Value is a
* negative number, then it is subtracted from the existing attribute.
* If you use ADD
to increment or decrement a
* number value for an item that doesn't exist before the update,
* DynamoDB uses 0
as the initial value.
Similarly, if
* you use ADD
for an existing item to increment or
* decrement an attribute value that doesn't exist before the update,
* DynamoDB uses 0
as the initial value. For example,
* suppose that the item you want to update doesn't have an attribute
* named itemcount, but you decide to ADD
the number
* 3
to this attribute anyway. DynamoDB will create the
* itemcount attribute, set its initial value to 0
,
* and finally add 3
to it. The result will be a new
* itemcount attribute in the item, with a value of
* 3
.
-
If the existing data type is a
* set and if Value is also a set, then Value is added to
* the existing set. For example, if the attribute value is the set
* [1,2]
, and the ADD
action specified
* [3]
, then the final attribute value is
* [1,2,3]
. An error occurs if an ADD
action is
* specified for a set attribute and the attribute type specified does
* not match the existing set type.
Both sets must have the same
* primitive data type. For example, if the existing data type is a set
* of strings, the Value must also be a set of strings.
*
The ADD
action only supports Number
* and set data types. In addition, ADD
can only be used on
* top-level attributes, not nested attributes.
-
*
DELETE
- Deletes an element from a set.
If a set of
* values is specified, then those values are subtracted from the old
* set. For example, if the attribute value was the set
* [a,b,c]
and the DELETE
action specifies
* [a,c]
, then the final attribute value is
* [b]
. Specifying an empty set is an error.
* The DELETE
action only supports set data
* types. In addition, DELETE
can only be used on top-level
* attributes, not nested attributes.
You can
* have many actions in a single expression, such as the following:
* SET a=:value1, b=:value2 DELETE :value3, :value4, :value5
*
For more information on update expressions, see Modifying
* Items and Attributes in the Amazon DynamoDB Developer
* Guide. UpdateExpression replaces the legacy
* AttributeUpdates parameter.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public UpdateItemRequest withUpdateExpression(String updateExpression) {
this.updateExpression = updateExpression;
return this;
}
/**
* A condition that must be satisfied in order for a conditional update
* to succeed.
An expression can contain any of the following:
* -
Functions: attribute_exists | attribute_not_exists |
* attribute_type | contains | begins_with | size
These
* function names are case-sensitive.
-
Comparison operators:
* = | <> | < | > | <= | >= | BETWEEN | IN
-
* Logical operators: AND | OR | NOT
For more
* information on condition expressions, see Specifying
* Conditions in the Amazon DynamoDB Developer Guide.
* ConditionExpression replaces the legacy
* ConditionalOperator and Expected parameters.
*
* @return A condition that must be satisfied in order for a conditional update
* to succeed.
An expression can contain any of the following:
* -
Functions: attribute_exists | attribute_not_exists |
* attribute_type | contains | begins_with | size
These
* function names are case-sensitive.
-
Comparison operators:
* = | <> | < | > | <= | >= | BETWEEN | IN
-
* Logical operators: AND | OR | NOT
For more
* information on condition expressions, see Specifying
* Conditions in the Amazon DynamoDB Developer Guide.
* ConditionExpression replaces the legacy
* ConditionalOperator and Expected parameters.
*/
public String getConditionExpression() {
return conditionExpression;
}
/**
* A condition that must be satisfied in order for a conditional update
* to succeed.
An expression can contain any of the following:
* -
Functions: attribute_exists | attribute_not_exists |
* attribute_type | contains | begins_with | size
These
* function names are case-sensitive.
-
Comparison operators:
* = | <> | < | > | <= | >= | BETWEEN | IN
-
* Logical operators: AND | OR | NOT
For more
* information on condition expressions, see Specifying
* Conditions in the Amazon DynamoDB Developer Guide.
* ConditionExpression replaces the legacy
* ConditionalOperator and Expected parameters.
*
* @param conditionExpression A condition that must be satisfied in order for a conditional update
* to succeed.
An expression can contain any of the following:
* -
Functions: attribute_exists | attribute_not_exists |
* attribute_type | contains | begins_with | size
These
* function names are case-sensitive.
-
Comparison operators:
* = | <> | < | > | <= | >= | BETWEEN | IN
-
* Logical operators: AND | OR | NOT
For more
* information on condition expressions, see Specifying
* Conditions in the Amazon DynamoDB Developer Guide.
* ConditionExpression replaces the legacy
* ConditionalOperator and Expected parameters.
*/
public void setConditionExpression(String conditionExpression) {
this.conditionExpression = conditionExpression;
}
/**
* A condition that must be satisfied in order for a conditional update
* to succeed.
An expression can contain any of the following:
* -
Functions: attribute_exists | attribute_not_exists |
* attribute_type | contains | begins_with | size
These
* function names are case-sensitive.
-
Comparison operators:
* = | <> | < | > | <= | >= | BETWEEN | IN
-
* Logical operators: AND | OR | NOT
For more
* information on condition expressions, see Specifying
* Conditions in the Amazon DynamoDB Developer Guide.
* ConditionExpression replaces the legacy
* ConditionalOperator and Expected parameters.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param conditionExpression A condition that must be satisfied in order for a conditional update
* to succeed.
An expression can contain any of the following:
* -
Functions: attribute_exists | attribute_not_exists |
* attribute_type | contains | begins_with | size
These
* function names are case-sensitive.
-
Comparison operators:
* = | <> | < | > | <= | >= | BETWEEN | IN
-
* Logical operators: AND | OR | NOT
For more
* information on condition expressions, see Specifying
* Conditions in the Amazon DynamoDB Developer Guide.
* ConditionExpression replaces the legacy
* ConditionalOperator and Expected parameters.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public UpdateItemRequest withConditionExpression(String conditionExpression) {
this.conditionExpression = conditionExpression;
return this;
}
/**
* One or more substitution tokens for attribute names in an expression.
* The following are some use cases for using
* ExpressionAttributeNames:
-
To access an attribute
* whose name conflicts with a DynamoDB reserved word.
-
To
* create a placeholder for repeating occurrences of an attribute name in
* an expression.
-
To prevent special characters in an
* attribute name from being misinterpreted in an expression.
* Use the # character in an expression to dereference an
* attribute name. For example, consider the following attribute name:
*
Percentile
The name of this
* attribute conflicts with a reserved word, so it cannot be used
* directly in an expression. (For the complete list of reserved words,
* see Reserved
* Words in the Amazon DynamoDB Developer Guide). To work
* around this, you could specify the following for
* ExpressionAttributeNames:
*
{"#P":"Percentile"}
You could
* then use this substitution in an expression, as in this example:
*
#P = :val
Tokens that begin
* with the : character are expression attribute values,
* which are placeholders for the actual value at runtime.
For
* more information on expression attribute names, see Accessing
* Item Attributes in the Amazon DynamoDB Developer Guide.
*
* @return One or more substitution tokens for attribute names in an expression.
* The following are some use cases for using
* ExpressionAttributeNames:
-
To access an attribute
* whose name conflicts with a DynamoDB reserved word.
-
To
* create a placeholder for repeating occurrences of an attribute name in
* an expression.
-
To prevent special characters in an
* attribute name from being misinterpreted in an expression.
* Use the # character in an expression to dereference an
* attribute name. For example, consider the following attribute name:
*
Percentile
The name of this
* attribute conflicts with a reserved word, so it cannot be used
* directly in an expression. (For the complete list of reserved words,
* see Reserved
* Words in the Amazon DynamoDB Developer Guide). To work
* around this, you could specify the following for
* ExpressionAttributeNames:
*
{"#P":"Percentile"}
You could
* then use this substitution in an expression, as in this example:
*
#P = :val
Tokens that begin
* with the : character are expression attribute values,
* which are placeholders for the actual value at runtime.
For
* more information on expression attribute names, see Accessing
* Item Attributes in the Amazon DynamoDB Developer Guide.
*/
public java.util.Map getExpressionAttributeNames() {
return expressionAttributeNames;
}
/**
* One or more substitution tokens for attribute names in an expression.
* The following are some use cases for using
* ExpressionAttributeNames: -
To access an attribute
* whose name conflicts with a DynamoDB reserved word.
-
To
* create a placeholder for repeating occurrences of an attribute name in
* an expression.
-
To prevent special characters in an
* attribute name from being misinterpreted in an expression.
* Use the # character in an expression to dereference an
* attribute name. For example, consider the following attribute name:
*
Percentile
The name of this
* attribute conflicts with a reserved word, so it cannot be used
* directly in an expression. (For the complete list of reserved words,
* see Reserved
* Words in the Amazon DynamoDB Developer Guide). To work
* around this, you could specify the following for
* ExpressionAttributeNames:
*
{"#P":"Percentile"}
You could
* then use this substitution in an expression, as in this example:
*
#P = :val
Tokens that begin
* with the : character are expression attribute values,
* which are placeholders for the actual value at runtime.
For
* more information on expression attribute names, see Accessing
* Item Attributes in the Amazon DynamoDB Developer Guide.
*
* @param expressionAttributeNames One or more substitution tokens for attribute names in an expression.
* The following are some use cases for using
* ExpressionAttributeNames:
-
To access an attribute
* whose name conflicts with a DynamoDB reserved word.
-
To
* create a placeholder for repeating occurrences of an attribute name in
* an expression.
-
To prevent special characters in an
* attribute name from being misinterpreted in an expression.
* Use the # character in an expression to dereference an
* attribute name. For example, consider the following attribute name:
*
Percentile
The name of this
* attribute conflicts with a reserved word, so it cannot be used
* directly in an expression. (For the complete list of reserved words,
* see Reserved
* Words in the Amazon DynamoDB Developer Guide). To work
* around this, you could specify the following for
* ExpressionAttributeNames:
*
{"#P":"Percentile"}
You could
* then use this substitution in an expression, as in this example:
*
#P = :val
Tokens that begin
* with the : character are expression attribute values,
* which are placeholders for the actual value at runtime.
For
* more information on expression attribute names, see Accessing
* Item Attributes in the Amazon DynamoDB Developer Guide.
*/
public void setExpressionAttributeNames(java.util.Map expressionAttributeNames) {
this.expressionAttributeNames = expressionAttributeNames;
}
/**
* One or more substitution tokens for attribute names in an expression.
* The following are some use cases for using
* ExpressionAttributeNames: -
To access an attribute
* whose name conflicts with a DynamoDB reserved word.
-
To
* create a placeholder for repeating occurrences of an attribute name in
* an expression.
-
To prevent special characters in an
* attribute name from being misinterpreted in an expression.
* Use the # character in an expression to dereference an
* attribute name. For example, consider the following attribute name:
*
Percentile
The name of this
* attribute conflicts with a reserved word, so it cannot be used
* directly in an expression. (For the complete list of reserved words,
* see Reserved
* Words in the Amazon DynamoDB Developer Guide). To work
* around this, you could specify the following for
* ExpressionAttributeNames:
*
{"#P":"Percentile"}
You could
* then use this substitution in an expression, as in this example:
*
#P = :val
Tokens that begin
* with the : character are expression attribute values,
* which are placeholders for the actual value at runtime.
For
* more information on expression attribute names, see Accessing
* Item Attributes in the Amazon DynamoDB Developer Guide.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param expressionAttributeNames One or more substitution tokens for attribute names in an expression.
* The following are some use cases for using
* ExpressionAttributeNames:
-
To access an attribute
* whose name conflicts with a DynamoDB reserved word.
-
To
* create a placeholder for repeating occurrences of an attribute name in
* an expression.
-
To prevent special characters in an
* attribute name from being misinterpreted in an expression.
* Use the # character in an expression to dereference an
* attribute name. For example, consider the following attribute name:
*
Percentile
The name of this
* attribute conflicts with a reserved word, so it cannot be used
* directly in an expression. (For the complete list of reserved words,
* see Reserved
* Words in the Amazon DynamoDB Developer Guide). To work
* around this, you could specify the following for
* ExpressionAttributeNames:
*
{"#P":"Percentile"}
You could
* then use this substitution in an expression, as in this example:
*
#P = :val
Tokens that begin
* with the : character are expression attribute values,
* which are placeholders for the actual value at runtime.
For
* more information on expression attribute names, see Accessing
* Item Attributes in the Amazon DynamoDB Developer Guide.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public UpdateItemRequest withExpressionAttributeNames(java.util.Map expressionAttributeNames) {
setExpressionAttributeNames(expressionAttributeNames);
return this;
}
/**
* One or more substitution tokens for attribute names in an expression.
* The following are some use cases for using
* ExpressionAttributeNames: -
To access an attribute
* whose name conflicts with a DynamoDB reserved word.
-
To
* create a placeholder for repeating occurrences of an attribute name in
* an expression.
-
To prevent special characters in an
* attribute name from being misinterpreted in an expression.
* Use the # character in an expression to dereference an
* attribute name. For example, consider the following attribute name:
*
Percentile
The name of this
* attribute conflicts with a reserved word, so it cannot be used
* directly in an expression. (For the complete list of reserved words,
* see Reserved
* Words in the Amazon DynamoDB Developer Guide). To work
* around this, you could specify the following for
* ExpressionAttributeNames:
*
{"#P":"Percentile"}
You could
* then use this substitution in an expression, as in this example:
*
#P = :val
Tokens that begin
* with the : character are expression attribute values,
* which are placeholders for the actual value at runtime.
For
* more information on expression attribute names, see Accessing
* Item Attributes in the Amazon DynamoDB Developer Guide.
*
* The method adds a new key-value pair into ExpressionAttributeNames
* parameter, and returns a reference to this object so that method calls
* can be chained together.
*
* @param key The key of the entry to be added into ExpressionAttributeNames.
* @param value The corresponding value of the entry to be added into ExpressionAttributeNames.
*/
public UpdateItemRequest addExpressionAttributeNamesEntry(String key, String value) {
if (null == this.expressionAttributeNames) {
this.expressionAttributeNames = new java.util.HashMap();
}
if (this.expressionAttributeNames.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.expressionAttributeNames.put(key, value);
return this;
}
/**
* Removes all the entries added into ExpressionAttributeNames.
*
* Returns a reference to this object so that method calls can be chained together.
*/
public UpdateItemRequest clearExpressionAttributeNamesEntries() {
this.expressionAttributeNames = null;
return this;
}
/**
* One or more values that can be substituted in an expression.
Use
* the : (colon) character in an expression to dereference an
* attribute value. For example, suppose that you wanted to check whether
* the value of the ProductStatus attribute was one of the
* following:
Available | Backordered | Discontinued
*
You would first need to specify ExpressionAttributeValues as
* follows:
{ ":avail":{"S":"Available"},
* ":back":{"S":"Backordered"}, ":disc":{"S":"Discontinued"} }
*
You could then use these values in an expression, such as this:
*
ProductStatus IN (:avail, :back, :disc)
For more
* information on expression attribute values, see Specifying
* Conditions in the Amazon DynamoDB Developer Guide.
*
* @return One or more values that can be substituted in an expression.
Use
* the : (colon) character in an expression to dereference an
* attribute value. For example, suppose that you wanted to check whether
* the value of the ProductStatus attribute was one of the
* following:
Available | Backordered | Discontinued
*
You would first need to specify ExpressionAttributeValues as
* follows:
{ ":avail":{"S":"Available"},
* ":back":{"S":"Backordered"}, ":disc":{"S":"Discontinued"} }
*
You could then use these values in an expression, such as this:
*
ProductStatus IN (:avail, :back, :disc)
For more
* information on expression attribute values, see Specifying
* Conditions in the Amazon DynamoDB Developer Guide.
*/
public java.util.Map getExpressionAttributeValues() {
return expressionAttributeValues;
}
/**
* One or more values that can be substituted in an expression. Use
* the : (colon) character in an expression to dereference an
* attribute value. For example, suppose that you wanted to check whether
* the value of the ProductStatus attribute was one of the
* following:
Available | Backordered | Discontinued
*
You would first need to specify ExpressionAttributeValues as
* follows:
{ ":avail":{"S":"Available"},
* ":back":{"S":"Backordered"}, ":disc":{"S":"Discontinued"} }
*
You could then use these values in an expression, such as this:
*
ProductStatus IN (:avail, :back, :disc)
For more
* information on expression attribute values, see Specifying
* Conditions in the Amazon DynamoDB Developer Guide.
*
* @param expressionAttributeValues One or more values that can be substituted in an expression.
Use
* the : (colon) character in an expression to dereference an
* attribute value. For example, suppose that you wanted to check whether
* the value of the ProductStatus attribute was one of the
* following:
Available | Backordered | Discontinued
*
You would first need to specify ExpressionAttributeValues as
* follows:
{ ":avail":{"S":"Available"},
* ":back":{"S":"Backordered"}, ":disc":{"S":"Discontinued"} }
*
You could then use these values in an expression, such as this:
*
ProductStatus IN (:avail, :back, :disc)
For more
* information on expression attribute values, see Specifying
* Conditions in the Amazon DynamoDB Developer Guide.
*/
public void setExpressionAttributeValues(java.util.Map expressionAttributeValues) {
this.expressionAttributeValues = expressionAttributeValues;
}
/**
* One or more values that can be substituted in an expression. Use
* the : (colon) character in an expression to dereference an
* attribute value. For example, suppose that you wanted to check whether
* the value of the ProductStatus attribute was one of the
* following:
Available | Backordered | Discontinued
*
You would first need to specify ExpressionAttributeValues as
* follows:
{ ":avail":{"S":"Available"},
* ":back":{"S":"Backordered"}, ":disc":{"S":"Discontinued"} }
*
You could then use these values in an expression, such as this:
*
ProductStatus IN (:avail, :back, :disc)
For more
* information on expression attribute values, see Specifying
* Conditions in the Amazon DynamoDB Developer Guide.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param expressionAttributeValues One or more values that can be substituted in an expression.
Use
* the : (colon) character in an expression to dereference an
* attribute value. For example, suppose that you wanted to check whether
* the value of the ProductStatus attribute was one of the
* following:
Available | Backordered | Discontinued
*
You would first need to specify ExpressionAttributeValues as
* follows:
{ ":avail":{"S":"Available"},
* ":back":{"S":"Backordered"}, ":disc":{"S":"Discontinued"} }
*
You could then use these values in an expression, such as this:
*
ProductStatus IN (:avail, :back, :disc)
For more
* information on expression attribute values, see Specifying
* Conditions in the Amazon DynamoDB Developer Guide.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public UpdateItemRequest withExpressionAttributeValues(java.util.Map expressionAttributeValues) {
setExpressionAttributeValues(expressionAttributeValues);
return this;
}
/**
* One or more values that can be substituted in an expression. Use
* the : (colon) character in an expression to dereference an
* attribute value. For example, suppose that you wanted to check whether
* the value of the ProductStatus attribute was one of the
* following:
Available | Backordered | Discontinued
*
You would first need to specify ExpressionAttributeValues as
* follows:
{ ":avail":{"S":"Available"},
* ":back":{"S":"Backordered"}, ":disc":{"S":"Discontinued"} }
*
You could then use these values in an expression, such as this:
*
ProductStatus IN (:avail, :back, :disc)
For more
* information on expression attribute values, see Specifying
* Conditions in the Amazon DynamoDB Developer Guide.
*
* The method adds a new key-value pair into ExpressionAttributeValues
* parameter, and returns a reference to this object so that method calls
* can be chained together.
*
* @param key The key of the entry to be added into ExpressionAttributeValues.
* @param value The corresponding value of the entry to be added into ExpressionAttributeValues.
*/
public UpdateItemRequest addExpressionAttributeValuesEntry(String key, AttributeValue value) {
if (null == this.expressionAttributeValues) {
this.expressionAttributeValues = new java.util.HashMap();
}
if (this.expressionAttributeValues.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.expressionAttributeValues.put(key, value);
return this;
}
/**
* Removes all the entries added into ExpressionAttributeValues.
*
* Returns a reference to this object so that method calls can be chained together.
*/
public UpdateItemRequest clearExpressionAttributeValuesEntries() {
this.expressionAttributeValues = null;
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getTableName() != null) sb.append("TableName: " + getTableName() + ",");
if (getKey() != null) sb.append("Key: " + getKey() + ",");
if (getAttributeUpdates() != null) sb.append("AttributeUpdates: " + getAttributeUpdates() + ",");
if (getExpected() != null) sb.append("Expected: " + getExpected() + ",");
if (getConditionalOperator() != null) sb.append("ConditionalOperator: " + getConditionalOperator() + ",");
if (getReturnValues() != null) sb.append("ReturnValues: " + getReturnValues() + ",");
if (getReturnConsumedCapacity() != null) sb.append("ReturnConsumedCapacity: " + getReturnConsumedCapacity() + ",");
if (getReturnItemCollectionMetrics() != null) sb.append("ReturnItemCollectionMetrics: " + getReturnItemCollectionMetrics() + ",");
if (getUpdateExpression() != null) sb.append("UpdateExpression: " + getUpdateExpression() + ",");
if (getConditionExpression() != null) sb.append("ConditionExpression: " + getConditionExpression() + ",");
if (getExpressionAttributeNames() != null) sb.append("ExpressionAttributeNames: " + getExpressionAttributeNames() + ",");
if (getExpressionAttributeValues() != null) sb.append("ExpressionAttributeValues: " + getExpressionAttributeValues() );
sb.append("}");
return sb.toString();
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getTableName() == null) ? 0 : getTableName().hashCode());
hashCode = prime * hashCode + ((getKey() == null) ? 0 : getKey().hashCode());
hashCode = prime * hashCode + ((getAttributeUpdates() == null) ? 0 : getAttributeUpdates().hashCode());
hashCode = prime * hashCode + ((getExpected() == null) ? 0 : getExpected().hashCode());
hashCode = prime * hashCode + ((getConditionalOperator() == null) ? 0 : getConditionalOperator().hashCode());
hashCode = prime * hashCode + ((getReturnValues() == null) ? 0 : getReturnValues().hashCode());
hashCode = prime * hashCode + ((getReturnConsumedCapacity() == null) ? 0 : getReturnConsumedCapacity().hashCode());
hashCode = prime * hashCode + ((getReturnItemCollectionMetrics() == null) ? 0 : getReturnItemCollectionMetrics().hashCode());
hashCode = prime * hashCode + ((getUpdateExpression() == null) ? 0 : getUpdateExpression().hashCode());
hashCode = prime * hashCode + ((getConditionExpression() == null) ? 0 : getConditionExpression().hashCode());
hashCode = prime * hashCode + ((getExpressionAttributeNames() == null) ? 0 : getExpressionAttributeNames().hashCode());
hashCode = prime * hashCode + ((getExpressionAttributeValues() == null) ? 0 : getExpressionAttributeValues().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null) return false;
if (obj instanceof UpdateItemRequest == false) return false;
UpdateItemRequest other = (UpdateItemRequest)obj;
if (other.getTableName() == null ^ this.getTableName() == null) return false;
if (other.getTableName() != null && other.getTableName().equals(this.getTableName()) == false) return false;
if (other.getKey() == null ^ this.getKey() == null) return false;
if (other.getKey() != null && other.getKey().equals(this.getKey()) == false) return false;
if (other.getAttributeUpdates() == null ^ this.getAttributeUpdates() == null) return false;
if (other.getAttributeUpdates() != null && other.getAttributeUpdates().equals(this.getAttributeUpdates()) == false) return false;
if (other.getExpected() == null ^ this.getExpected() == null) return false;
if (other.getExpected() != null && other.getExpected().equals(this.getExpected()) == false) return false;
if (other.getConditionalOperator() == null ^ this.getConditionalOperator() == null) return false;
if (other.getConditionalOperator() != null && other.getConditionalOperator().equals(this.getConditionalOperator()) == false) return false;
if (other.getReturnValues() == null ^ this.getReturnValues() == null) return false;
if (other.getReturnValues() != null && other.getReturnValues().equals(this.getReturnValues()) == false) return false;
if (other.getReturnConsumedCapacity() == null ^ this.getReturnConsumedCapacity() == null) return false;
if (other.getReturnConsumedCapacity() != null && other.getReturnConsumedCapacity().equals(this.getReturnConsumedCapacity()) == false) return false;
if (other.getReturnItemCollectionMetrics() == null ^ this.getReturnItemCollectionMetrics() == null) return false;
if (other.getReturnItemCollectionMetrics() != null && other.getReturnItemCollectionMetrics().equals(this.getReturnItemCollectionMetrics()) == false) return false;
if (other.getUpdateExpression() == null ^ this.getUpdateExpression() == null) return false;
if (other.getUpdateExpression() != null && other.getUpdateExpression().equals(this.getUpdateExpression()) == false) return false;
if (other.getConditionExpression() == null ^ this.getConditionExpression() == null) return false;
if (other.getConditionExpression() != null && other.getConditionExpression().equals(this.getConditionExpression()) == false) return false;
if (other.getExpressionAttributeNames() == null ^ this.getExpressionAttributeNames() == null) return false;
if (other.getExpressionAttributeNames() != null && other.getExpressionAttributeNames().equals(this.getExpressionAttributeNames()) == false) return false;
if (other.getExpressionAttributeValues() == null ^ this.getExpressionAttributeValues() == null) return false;
if (other.getExpressionAttributeValues() != null && other.getExpressionAttributeValues().equals(this.getExpressionAttributeValues()) == false) return false;
return true;
}
}