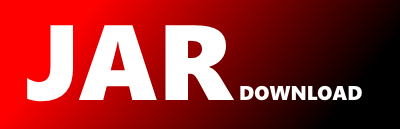
com.amazonaws.services.ec2.AmazonEC2AsyncClient Maven / Gradle / Ivy
Show all versions of aws-android-sdk-ec2 Show documentation
/*
* Copyright 2010-2016 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.ec2;
import java.util.concurrent.Callable;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.Future;
import com.amazonaws.AmazonClientException;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.handlers.AsyncHandler;
import com.amazonaws.ClientConfiguration;
import com.amazonaws.auth.AWSCredentials;
import com.amazonaws.auth.AWSCredentialsProvider;
import com.amazonaws.auth.DefaultAWSCredentialsProviderChain;
import com.amazonaws.services.ec2.model.*;
/**
* Asynchronous client for accessing AmazonEC2.
* All asynchronous calls made using this client are non-blocking. Callers could either
* process the result and handle the exceptions in the worker thread by providing a callback handler
* when making the call, or use the returned Future object to check the result of the call in the calling thread.
* Amazon Elastic Compute Cloud
* Amazon Elastic Compute Cloud (Amazon EC2) provides resizable computing
* capacity in the Amazon Web Services (AWS) cloud. Using Amazon EC2
* eliminates your need to invest in hardware up front, so you can
* develop and deploy applications faster.
*
*/
public class AmazonEC2AsyncClient extends AmazonEC2Client
implements AmazonEC2Async {
/**
* Executor service for executing asynchronous requests.
*/
private ExecutorService executorService;
private static final int DEFAULT_THREAD_POOL_SIZE = 10;
/**
* Constructs a new asynchronous client to invoke service methods on
* AmazonEC2. A credentials provider chain will be used
* that searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @see DefaultAWSCredentialsProviderChain
*/
@Deprecated
public AmazonEC2AsyncClient() {
this(new DefaultAWSCredentialsProviderChain());
}
/**
* Constructs a new asynchronous client to invoke service methods on
* AmazonEC2. A credentials provider chain will be used
* that searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @param clientConfiguration The client configuration options controlling how this
* client connects to AmazonEC2
* (ex: proxy settings, retry counts, etc.).
*
* @see DefaultAWSCredentialsProviderChain
*/
@Deprecated
public AmazonEC2AsyncClient(ClientConfiguration clientConfiguration) {
this(new DefaultAWSCredentialsProviderChain(), clientConfiguration, Executors.newFixedThreadPool(clientConfiguration.getMaxConnections()));
}
/**
* Constructs a new asynchronous client to invoke service methods on
* AmazonEC2 using the specified AWS account credentials.
* Default client settings will be used, and a fixed size thread pool will be
* created for executing the asynchronous tasks.
*
*
* All calls made using this new client object are non-blocking, and will immediately
* return a Java Future object that the caller can later check to see if the service
* call has actually completed.
*
* @param awsCredentials The AWS credentials (access key ID and secret key) to use
* when authenticating with AWS services.
*/
public AmazonEC2AsyncClient(AWSCredentials awsCredentials) {
this(awsCredentials, Executors.newFixedThreadPool(DEFAULT_THREAD_POOL_SIZE));
}
/**
* Constructs a new asynchronous client to invoke service methods on
* AmazonEC2 using the specified AWS account credentials
* and executor service. Default client settings will be used.
*
*
* All calls made using this new client object are non-blocking, and will immediately
* return a Java Future object that the caller can later check to see if the service
* call has actually completed.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use
* when authenticating with AWS services.
* @param executorService
* The executor service by which all asynchronous requests will
* be executed.
*/
public AmazonEC2AsyncClient(AWSCredentials awsCredentials, ExecutorService executorService) {
super(awsCredentials);
this.executorService = executorService;
}
/**
* Constructs a new asynchronous client to invoke service methods on
* AmazonEC2 using the specified AWS account credentials,
* executor service, and client configuration options.
*
*
* All calls made using this new client object are non-blocking, and will immediately
* return a Java Future object that the caller can later check to see if the service
* call has actually completed.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use
* when authenticating with AWS services.
* @param clientConfiguration
* Client configuration options (ex: max retry limit, proxy
* settings, etc).
* @param executorService
* The executor service by which all asynchronous requests will
* be executed.
*/
public AmazonEC2AsyncClient(AWSCredentials awsCredentials,
ClientConfiguration clientConfiguration, ExecutorService executorService) {
super(awsCredentials, clientConfiguration);
this.executorService = executorService;
}
/**
* Constructs a new asynchronous client to invoke service methods on
* AmazonEC2 using the specified AWS account credentials provider.
* Default client settings will be used, and a fixed size thread pool will be
* created for executing the asynchronous tasks.
*
*
* All calls made using this new client object are non-blocking, and will immediately
* return a Java Future object that the caller can later check to see if the service
* call has actually completed.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials
* to authenticate requests with AWS services.
*/
public AmazonEC2AsyncClient(AWSCredentialsProvider awsCredentialsProvider) {
this(awsCredentialsProvider, Executors.newFixedThreadPool(DEFAULT_THREAD_POOL_SIZE));
}
/**
* Constructs a new asynchronous client to invoke service methods on
* AmazonEC2 using the specified AWS account credentials provider
* and executor service. Default client settings will be used.
*
*
* All calls made using this new client object are non-blocking, and will immediately
* return a Java Future object that the caller can later check to see if the service
* call has actually completed.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials
* to authenticate requests with AWS services.
* @param executorService
* The executor service by which all asynchronous requests will
* be executed.
*/
public AmazonEC2AsyncClient(AWSCredentialsProvider awsCredentialsProvider, ExecutorService executorService) {
this(awsCredentialsProvider, new ClientConfiguration(), executorService);
}
/**
* Constructs a new asynchronous client to invoke service methods on
* AmazonEC2 using the specified AWS account credentials
* provider and client configuration options.
*
*
* All calls made using this new client object are non-blocking, and will immediately
* return a Java Future object that the caller can later check to see if the service
* call has actually completed.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials
* to authenticate requests with AWS services.
* @param clientConfiguration
* Client configuration options (ex: max retry limit, proxy
* settings, etc).
*/
public AmazonEC2AsyncClient(AWSCredentialsProvider awsCredentialsProvider,
ClientConfiguration clientConfiguration) {
this(awsCredentialsProvider, clientConfiguration, Executors.newFixedThreadPool(clientConfiguration.getMaxConnections()));
}
/**
* Constructs a new asynchronous client to invoke service methods on
* AmazonEC2 using the specified AWS account credentials
* provider, executor service, and client configuration options.
*
*
* All calls made using this new client object are non-blocking, and will immediately
* return a Java Future object that the caller can later check to see if the service
* call has actually completed.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials
* to authenticate requests with AWS services.
* @param clientConfiguration
* Client configuration options (ex: max retry limit, proxy
* settings, etc).
* @param executorService
* The executor service by which all asynchronous requests will
* be executed.
*/
public AmazonEC2AsyncClient(AWSCredentialsProvider awsCredentialsProvider,
ClientConfiguration clientConfiguration, ExecutorService executorService) {
super(awsCredentialsProvider, clientConfiguration);
this.executorService = executorService;
}
/**
* Returns the executor service used by this async client to execute
* requests.
*
* @return The executor service used by this async client to execute
* requests.
*/
public ExecutorService getExecutorService() {
return executorService;
}
/**
* Shuts down the client, releasing all managed resources. This includes
* forcibly terminating all pending asynchronous service calls. Clients who
* wish to give pending asynchronous service calls time to complete should
* call getExecutorService().shutdown() followed by
* getExecutorService().awaitTermination() prior to calling this method.
*/
@Override
public void shutdown() {
super.shutdown();
executorService.shutdownNow();
}
/**
*
* Deletes the specified placement group. You must terminate all
* instances in the placement group before you can delete the placement
* group. For more information about placement groups and cluster
* instances, see
* Cluster Instances
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param deletePlacementGroupRequest Container for the necessary
* parameters to execute the DeletePlacementGroup operation on AmazonEC2.
*
* @return A Java Future object containing the response from the
* DeletePlacementGroup service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deletePlacementGroupAsync(final DeletePlacementGroupRequest deletePlacementGroupRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
deletePlacementGroup(deletePlacementGroupRequest);
return null;
}
});
}
/**
*
* Deletes the specified placement group. You must terminate all
* instances in the placement group before you can delete the placement
* group. For more information about placement groups and cluster
* instances, see
* Cluster Instances
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param deletePlacementGroupRequest Container for the necessary
* parameters to execute the DeletePlacementGroup operation on AmazonEC2.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DeletePlacementGroup service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deletePlacementGroupAsync(
final DeletePlacementGroupRequest deletePlacementGroupRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
try {
deletePlacementGroup(deletePlacementGroupRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(deletePlacementGroupRequest, null);
return null;
}
});
}
/**
*
* Creates a set of DHCP options for your VPC. After creating the set,
* you must associate it with the VPC, causing all existing and new
* instances that you launch in the VPC to use this set of DHCP options.
* The following are the individual DHCP options you can specify. For
* more information about the options, see
* RFC 2132
* .
*
*
*
* -
domain-name-servers
- The IP addresses of up to
* four domain name servers, or AmazonProvidedDNS
. The
* default DHCP option set specifies AmazonProvidedDNS
. If
* specifying more than one domain name server, specify the IP addresses
* in a single parameter, separated by commas.
* -
domain-name
- If you're using AmazonProvidedDNS in
* us-east-1
, specify ec2.internal
. If you're
* using AmazonProvidedDNS in another region, specify
* region.compute.internal
(for example,
* ap-northeast-1.compute.internal
). Otherwise, specify a
* domain name (for example, MyCompany.com
).
* Important : Some Linux operating systems accept multiple domain
* names separated by spaces. However, Windows and other Linux operating
* systems treat the value as a single domain, which results in
* unexpected behavior. If your DHCP options set is associated with a VPC
* that has instances with multiple operating systems, specify only one
* domain name.
* -
ntp-servers
- The IP addresses of up to four
* Network Time Protocol (NTP) servers.
* -
netbios-name-servers
- The IP addresses of up to
* four NetBIOS name servers.
* -
netbios-node-type
- The NetBIOS node type (1, 2, 4,
* or 8). We recommend that you specify 2 (broadcast and multicast are
* not currently supported). For more information about these node types,
* see
* RFC 2132
* .
*
*
*
* Your VPC automatically starts out with a set of DHCP options that
* includes only a DNS server that we provide (AmazonProvidedDNS). If you
* create a set of options, and if your VPC has an Internet gateway, make
* sure to set the domain-name-servers
option either to
* AmazonProvidedDNS
or to a domain name server of your
* choice. For more information about DHCP options, see
* DHCP Options Sets
* in the Amazon Virtual Private Cloud User Guide .
*
*
* @param createDhcpOptionsRequest Container for the necessary parameters
* to execute the CreateDhcpOptions operation on AmazonEC2.
*
* @return A Java Future object containing the response from the
* CreateDhcpOptions service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createDhcpOptionsAsync(final CreateDhcpOptionsRequest createDhcpOptionsRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public CreateDhcpOptionsResult call() throws Exception {
return createDhcpOptions(createDhcpOptionsRequest);
}
});
}
/**
*
* Creates a set of DHCP options for your VPC. After creating the set,
* you must associate it with the VPC, causing all existing and new
* instances that you launch in the VPC to use this set of DHCP options.
* The following are the individual DHCP options you can specify. For
* more information about the options, see
* RFC 2132
* .
*
*
*
* -
domain-name-servers
- The IP addresses of up to
* four domain name servers, or AmazonProvidedDNS
. The
* default DHCP option set specifies AmazonProvidedDNS
. If
* specifying more than one domain name server, specify the IP addresses
* in a single parameter, separated by commas.
* -
domain-name
- If you're using AmazonProvidedDNS in
* us-east-1
, specify ec2.internal
. If you're
* using AmazonProvidedDNS in another region, specify
* region.compute.internal
(for example,
* ap-northeast-1.compute.internal
). Otherwise, specify a
* domain name (for example, MyCompany.com
).
* Important : Some Linux operating systems accept multiple domain
* names separated by spaces. However, Windows and other Linux operating
* systems treat the value as a single domain, which results in
* unexpected behavior. If your DHCP options set is associated with a VPC
* that has instances with multiple operating systems, specify only one
* domain name.
* -
ntp-servers
- The IP addresses of up to four
* Network Time Protocol (NTP) servers.
* -
netbios-name-servers
- The IP addresses of up to
* four NetBIOS name servers.
* -
netbios-node-type
- The NetBIOS node type (1, 2, 4,
* or 8). We recommend that you specify 2 (broadcast and multicast are
* not currently supported). For more information about these node types,
* see
* RFC 2132
* .
*
*
*
* Your VPC automatically starts out with a set of DHCP options that
* includes only a DNS server that we provide (AmazonProvidedDNS). If you
* create a set of options, and if your VPC has an Internet gateway, make
* sure to set the domain-name-servers
option either to
* AmazonProvidedDNS
or to a domain name server of your
* choice. For more information about DHCP options, see
* DHCP Options Sets
* in the Amazon Virtual Private Cloud User Guide .
*
*
* @param createDhcpOptionsRequest Container for the necessary parameters
* to execute the CreateDhcpOptions operation on AmazonEC2.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* CreateDhcpOptions service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createDhcpOptionsAsync(
final CreateDhcpOptionsRequest createDhcpOptionsRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public CreateDhcpOptionsResult call() throws Exception {
CreateDhcpOptionsResult result;
try {
result = createDhcpOptions(createDhcpOptionsRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(createDhcpOptionsRequest, result);
return result;
}
});
}
/**
*
* Creates a VPN connection between an existing virtual private gateway
* and a VPN customer gateway. The only supported connection type is
* ipsec.1
.
*
*
* The response includes information that you need to give to your
* network administrator to configure your customer gateway.
*
*
* IMPORTANT: We strongly recommend that you use HTTPS when
* calling this operation because the response contains sensitive
* cryptographic information for configuring your customer gateway.
*
*
* If you decide to shut down your VPN connection for any reason and
* later create a new VPN connection, you must reconfigure your customer
* gateway with the new information returned from this call.
*
*
* For more information about VPN connections, see
* Adding a Hardware Virtual Private Gateway to Your VPC
* in the Amazon Virtual Private Cloud User Guide .
*
*
* @param createVpnConnectionRequest Container for the necessary
* parameters to execute the CreateVpnConnection operation on AmazonEC2.
*
* @return A Java Future object containing the response from the
* CreateVpnConnection service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createVpnConnectionAsync(final CreateVpnConnectionRequest createVpnConnectionRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public CreateVpnConnectionResult call() throws Exception {
return createVpnConnection(createVpnConnectionRequest);
}
});
}
/**
*
* Creates a VPN connection between an existing virtual private gateway
* and a VPN customer gateway. The only supported connection type is
* ipsec.1
.
*
*
* The response includes information that you need to give to your
* network administrator to configure your customer gateway.
*
*
* IMPORTANT: We strongly recommend that you use HTTPS when
* calling this operation because the response contains sensitive
* cryptographic information for configuring your customer gateway.
*
*
* If you decide to shut down your VPN connection for any reason and
* later create a new VPN connection, you must reconfigure your customer
* gateway with the new information returned from this call.
*
*
* For more information about VPN connections, see
* Adding a Hardware Virtual Private Gateway to Your VPC
* in the Amazon Virtual Private Cloud User Guide .
*
*
* @param createVpnConnectionRequest Container for the necessary
* parameters to execute the CreateVpnConnection operation on AmazonEC2.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* CreateVpnConnection service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createVpnConnectionAsync(
final CreateVpnConnectionRequest createVpnConnectionRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public CreateVpnConnectionResult call() throws Exception {
CreateVpnConnectionResult result;
try {
result = createVpnConnection(createVpnConnectionRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(createVpnConnectionRequest, result);
return result;
}
});
}
/**
*
* Disassociates a subnet from a route table.
*
*
* After you perform this action, the subnet no longer uses the routes
* in the route table. Instead, it uses the routes in the VPC's main
* route table. For more information about route tables, see
* Route Tables
* in the Amazon Virtual Private Cloud User Guide .
*
*
* @param disassociateRouteTableRequest Container for the necessary
* parameters to execute the DisassociateRouteTable operation on
* AmazonEC2.
*
* @return A Java Future object containing the response from the
* DisassociateRouteTable service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future disassociateRouteTableAsync(final DisassociateRouteTableRequest disassociateRouteTableRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
disassociateRouteTable(disassociateRouteTableRequest);
return null;
}
});
}
/**
*
* Disassociates a subnet from a route table.
*
*
* After you perform this action, the subnet no longer uses the routes
* in the route table. Instead, it uses the routes in the VPC's main
* route table. For more information about route tables, see
* Route Tables
* in the Amazon Virtual Private Cloud User Guide .
*
*
* @param disassociateRouteTableRequest Container for the necessary
* parameters to execute the DisassociateRouteTable operation on
* AmazonEC2.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DisassociateRouteTable service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future disassociateRouteTableAsync(
final DisassociateRouteTableRequest disassociateRouteTableRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
try {
disassociateRouteTable(disassociateRouteTableRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(disassociateRouteTableRequest, null);
return null;
}
});
}
/**
*
* Modifies the Availability Zone, instance count, instance type, or
* network platform (EC2-Classic or EC2-VPC) of your Reserved Instances.
* The Reserved Instances to be modified must be identical, except for
* Availability Zone, network platform, and instance type.
*
*
* For more information, see
* Modifying Reserved Instances
* in the Amazon Elastic Compute Cloud User Guide.
*
*
* @param modifyReservedInstancesRequest Container for the necessary
* parameters to execute the ModifyReservedInstances operation on
* AmazonEC2.
*
* @return A Java Future object containing the response from the
* ModifyReservedInstances service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future modifyReservedInstancesAsync(final ModifyReservedInstancesRequest modifyReservedInstancesRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public ModifyReservedInstancesResult call() throws Exception {
return modifyReservedInstances(modifyReservedInstancesRequest);
}
});
}
/**
*
* Modifies the Availability Zone, instance count, instance type, or
* network platform (EC2-Classic or EC2-VPC) of your Reserved Instances.
* The Reserved Instances to be modified must be identical, except for
* Availability Zone, network platform, and instance type.
*
*
* For more information, see
* Modifying Reserved Instances
* in the Amazon Elastic Compute Cloud User Guide.
*
*
* @param modifyReservedInstancesRequest Container for the necessary
* parameters to execute the ModifyReservedInstances operation on
* AmazonEC2.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* ModifyReservedInstances service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future modifyReservedInstancesAsync(
final ModifyReservedInstancesRequest modifyReservedInstancesRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public ModifyReservedInstancesResult call() throws Exception {
ModifyReservedInstancesResult result;
try {
result = modifyReservedInstances(modifyReservedInstancesRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(modifyReservedInstancesRequest, result);
return result;
}
});
}
/**
*
* Imports a disk into an EBS snapshot.
*
*
* @param importSnapshotRequest Container for the necessary parameters to
* execute the ImportSnapshot operation on AmazonEC2.
*
* @return A Java Future object containing the response from the
* ImportSnapshot service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future importSnapshotAsync(final ImportSnapshotRequest importSnapshotRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public ImportSnapshotResult call() throws Exception {
return importSnapshot(importSnapshotRequest);
}
});
}
/**
*
* Imports a disk into an EBS snapshot.
*
*
* @param importSnapshotRequest Container for the necessary parameters to
* execute the ImportSnapshot operation on AmazonEC2.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* ImportSnapshot service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future importSnapshotAsync(
final ImportSnapshotRequest importSnapshotRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public ImportSnapshotResult call() throws Exception {
ImportSnapshotResult result;
try {
result = importSnapshot(importSnapshotRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(importSnapshotRequest, result);
return result;
}
});
}
/**
*
* Shuts down one or more instances. This operation is idempotent; if
* you terminate an instance more than once, each call succeeds.
*
*
* Terminated instances remain visible after termination (for
* approximately one hour).
*
*
* By default, Amazon EC2 deletes all EBS volumes that were attached
* when the instance launched. Volumes attached after instance launch
* continue running.
*
*
* You can stop, start, and terminate EBS-backed instances. You can only
* terminate instance store-backed instances. What happens to an instance
* differs if you stop it or terminate it. For example, when you stop an
* instance, the root device and any other devices attached to the
* instance persist. When you terminate an instance, any attached EBS
* volumes with the DeleteOnTermination
block device mapping
* parameter set to true
are automatically deleted. For more
* information about the differences between stopping and terminating
* instances, see
* Instance Lifecycle
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* For more information about troubleshooting, see
* Troubleshooting Terminating Your Instance
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param terminateInstancesRequest Container for the necessary
* parameters to execute the TerminateInstances operation on AmazonEC2.
*
* @return A Java Future object containing the response from the
* TerminateInstances service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future terminateInstancesAsync(final TerminateInstancesRequest terminateInstancesRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public TerminateInstancesResult call() throws Exception {
return terminateInstances(terminateInstancesRequest);
}
});
}
/**
*
* Shuts down one or more instances. This operation is idempotent; if
* you terminate an instance more than once, each call succeeds.
*
*
* Terminated instances remain visible after termination (for
* approximately one hour).
*
*
* By default, Amazon EC2 deletes all EBS volumes that were attached
* when the instance launched. Volumes attached after instance launch
* continue running.
*
*
* You can stop, start, and terminate EBS-backed instances. You can only
* terminate instance store-backed instances. What happens to an instance
* differs if you stop it or terminate it. For example, when you stop an
* instance, the root device and any other devices attached to the
* instance persist. When you terminate an instance, any attached EBS
* volumes with the DeleteOnTermination
block device mapping
* parameter set to true
are automatically deleted. For more
* information about the differences between stopping and terminating
* instances, see
* Instance Lifecycle
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* For more information about troubleshooting, see
* Troubleshooting Terminating Your Instance
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param terminateInstancesRequest Container for the necessary
* parameters to execute the TerminateInstances operation on AmazonEC2.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* TerminateInstances service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future terminateInstancesAsync(
final TerminateInstancesRequest terminateInstancesRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public TerminateInstancesResult call() throws Exception {
TerminateInstancesResult result;
try {
result = terminateInstances(terminateInstancesRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(terminateInstancesRequest, result);
return result;
}
});
}
/**
*
* Determines whether a product code is associated with an instance.
* This action can only be used by the owner of the product code. It is
* useful when a product code owner needs to verify whether another
* user's instance is eligible for support.
*
*
* @param confirmProductInstanceRequest Container for the necessary
* parameters to execute the ConfirmProductInstance operation on
* AmazonEC2.
*
* @return A Java Future object containing the response from the
* ConfirmProductInstance service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future confirmProductInstanceAsync(final ConfirmProductInstanceRequest confirmProductInstanceRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public ConfirmProductInstanceResult call() throws Exception {
return confirmProductInstance(confirmProductInstanceRequest);
}
});
}
/**
*
* Determines whether a product code is associated with an instance.
* This action can only be used by the owner of the product code. It is
* useful when a product code owner needs to verify whether another
* user's instance is eligible for support.
*
*
* @param confirmProductInstanceRequest Container for the necessary
* parameters to execute the ConfirmProductInstance operation on
* AmazonEC2.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* ConfirmProductInstance service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future confirmProductInstanceAsync(
final ConfirmProductInstanceRequest confirmProductInstanceRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public ConfirmProductInstanceResult call() throws Exception {
ConfirmProductInstanceResult result;
try {
result = confirmProductInstance(confirmProductInstanceRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(confirmProductInstanceRequest, result);
return result;
}
});
}
/**
*
* Deletes the specified snapshot.
*
*
* When you make periodic snapshots of a volume, the snapshots are
* incremental, and only the blocks on the device that have changed since
* your last snapshot are saved in the new snapshot. When you delete a
* snapshot, only the data not needed for any other snapshot is removed.
* So regardless of which prior snapshots have been deleted, all active
* snapshots will have access to all the information needed to restore
* the volume.
*
*
* You cannot delete a snapshot of the root device of an EBS volume used
* by a registered AMI. You must first de-register the AMI before you can
* delete the snapshot.
*
*
* For more information, see
* Deleting an Amazon EBS Snapshot
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param deleteSnapshotRequest Container for the necessary parameters to
* execute the DeleteSnapshot operation on AmazonEC2.
*
* @return A Java Future object containing the response from the
* DeleteSnapshot service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteSnapshotAsync(final DeleteSnapshotRequest deleteSnapshotRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
deleteSnapshot(deleteSnapshotRequest);
return null;
}
});
}
/**
*
* Deletes the specified snapshot.
*
*
* When you make periodic snapshots of a volume, the snapshots are
* incremental, and only the blocks on the device that have changed since
* your last snapshot are saved in the new snapshot. When you delete a
* snapshot, only the data not needed for any other snapshot is removed.
* So regardless of which prior snapshots have been deleted, all active
* snapshots will have access to all the information needed to restore
* the volume.
*
*
* You cannot delete a snapshot of the root device of an EBS volume used
* by a registered AMI. You must first de-register the AMI before you can
* delete the snapshot.
*
*
* For more information, see
* Deleting an Amazon EBS Snapshot
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param deleteSnapshotRequest Container for the necessary parameters to
* execute the DeleteSnapshot operation on AmazonEC2.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DeleteSnapshot service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteSnapshotAsync(
final DeleteSnapshotRequest deleteSnapshotRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
try {
deleteSnapshot(deleteSnapshotRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(deleteSnapshotRequest, null);
return null;
}
});
}
/**
*
* Describes the modifications made to your Reserved Instances. If no
* parameter is specified, information about all your Reserved Instances
* modification requests is returned. If a modification ID is specified,
* only information about the specific modification is returned.
*
*
* For more information, see
* Modifying Reserved Instances
* in the Amazon Elastic Compute Cloud User Guide.
*
*
* @param describeReservedInstancesModificationsRequest Container for the
* necessary parameters to execute the
* DescribeReservedInstancesModifications operation on AmazonEC2.
*
* @return A Java Future object containing the response from the
* DescribeReservedInstancesModifications service method, as returned by
* AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeReservedInstancesModificationsAsync(final DescribeReservedInstancesModificationsRequest describeReservedInstancesModificationsRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeReservedInstancesModificationsResult call() throws Exception {
return describeReservedInstancesModifications(describeReservedInstancesModificationsRequest);
}
});
}
/**
*
* Describes the modifications made to your Reserved Instances. If no
* parameter is specified, information about all your Reserved Instances
* modification requests is returned. If a modification ID is specified,
* only information about the specific modification is returned.
*
*
* For more information, see
* Modifying Reserved Instances
* in the Amazon Elastic Compute Cloud User Guide.
*
*
* @param describeReservedInstancesModificationsRequest Container for the
* necessary parameters to execute the
* DescribeReservedInstancesModifications operation on AmazonEC2.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeReservedInstancesModifications service method, as returned by
* AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeReservedInstancesModificationsAsync(
final DescribeReservedInstancesModificationsRequest describeReservedInstancesModificationsRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeReservedInstancesModificationsResult call() throws Exception {
DescribeReservedInstancesModificationsResult result;
try {
result = describeReservedInstancesModifications(describeReservedInstancesModificationsRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(describeReservedInstancesModificationsRequest, result);
return result;
}
});
}
/**
*
* Creates an import instance task using metadata from the specified
* disk image. ImportInstance
only supports single-volume
* VMs. To import multi-volume VMs, use ImportImage. After importing the
* image, you then upload it using the ec2-import-volume
* command in the EC2 command line tools. For more information, see
* Using the Command Line Tools to Import Your Virtual Machine to Amazon EC2
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* For information about the import manifest referenced by this API
* action, see
* VM Import Manifest
* .
*
*
* @param importInstanceRequest Container for the necessary parameters to
* execute the ImportInstance operation on AmazonEC2.
*
* @return A Java Future object containing the response from the
* ImportInstance service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future importInstanceAsync(final ImportInstanceRequest importInstanceRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public ImportInstanceResult call() throws Exception {
return importInstance(importInstanceRequest);
}
});
}
/**
*
* Creates an import instance task using metadata from the specified
* disk image. ImportInstance
only supports single-volume
* VMs. To import multi-volume VMs, use ImportImage. After importing the
* image, you then upload it using the ec2-import-volume
* command in the EC2 command line tools. For more information, see
* Using the Command Line Tools to Import Your Virtual Machine to Amazon EC2
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* For information about the import manifest referenced by this API
* action, see
* VM Import Manifest
* .
*
*
* @param importInstanceRequest Container for the necessary parameters to
* execute the ImportInstance operation on AmazonEC2.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* ImportInstance service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future importInstanceAsync(
final ImportInstanceRequest importInstanceRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public ImportInstanceResult call() throws Exception {
ImportInstanceResult result;
try {
result = importInstance(importInstanceRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(importInstanceRequest, result);
return result;
}
});
}
/**
*
* Describes one or more of the Availability Zones that are available to
* you. The results include zones only for the region you're currently
* using. If there is an event impacting an Availability Zone, you can
* use this request to view the state and any provided message for that
* Availability Zone.
*
*
* For more information, see
* Regions and Availability Zones
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param describeAvailabilityZonesRequest Container for the necessary
* parameters to execute the DescribeAvailabilityZones operation on
* AmazonEC2.
*
* @return A Java Future object containing the response from the
* DescribeAvailabilityZones service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeAvailabilityZonesAsync(final DescribeAvailabilityZonesRequest describeAvailabilityZonesRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeAvailabilityZonesResult call() throws Exception {
return describeAvailabilityZones(describeAvailabilityZonesRequest);
}
});
}
/**
*
* Describes one or more of the Availability Zones that are available to
* you. The results include zones only for the region you're currently
* using. If there is an event impacting an Availability Zone, you can
* use this request to view the state and any provided message for that
* Availability Zone.
*
*
* For more information, see
* Regions and Availability Zones
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param describeAvailabilityZonesRequest Container for the necessary
* parameters to execute the DescribeAvailabilityZones operation on
* AmazonEC2.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeAvailabilityZones service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeAvailabilityZonesAsync(
final DescribeAvailabilityZonesRequest describeAvailabilityZonesRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeAvailabilityZonesResult call() throws Exception {
DescribeAvailabilityZonesResult result;
try {
result = describeAvailabilityZones(describeAvailabilityZonesRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(describeAvailabilityZonesRequest, result);
return result;
}
});
}
/**
*
* Attaches an EBS volume to a running or stopped instance and exposes
* it to the instance with the specified device name.
*
*
* Encrypted EBS volumes may only be attached to instances that support
* Amazon EBS encryption. For more information, see
* Amazon EBS Encryption
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* For a list of supported device names, see
* Attaching an EBS Volume to an Instance . Any device names that aren't reserved for instance store volumes can be used for EBS volumes. For more information, see Amazon EC2 Instance Store
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* If a volume has an AWS Marketplace product code:
*
*
*
* - The volume can be attached only to a stopped instance.
* - AWS Marketplace product codes are copied from the volume to the
* instance.
* - You must be subscribed to the product.
* - The instance type and operating system of the instance must
* support the product. For example, you can't detach a volume from a
* Windows instance and attach it to a Linux instance.
*
*
*
* For an overview of the AWS Marketplace, see
* Introducing AWS Marketplace
* .
*
*
* For more information about EBS volumes, see
* Attaching Amazon EBS Volumes
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param attachVolumeRequest Container for the necessary parameters to
* execute the AttachVolume operation on AmazonEC2.
*
* @return A Java Future object containing the response from the
* AttachVolume service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future attachVolumeAsync(final AttachVolumeRequest attachVolumeRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public AttachVolumeResult call() throws Exception {
return attachVolume(attachVolumeRequest);
}
});
}
/**
*
* Attaches an EBS volume to a running or stopped instance and exposes
* it to the instance with the specified device name.
*
*
* Encrypted EBS volumes may only be attached to instances that support
* Amazon EBS encryption. For more information, see
* Amazon EBS Encryption
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* For a list of supported device names, see
* Attaching an EBS Volume to an Instance . Any device names that aren't reserved for instance store volumes can be used for EBS volumes. For more information, see Amazon EC2 Instance Store
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* If a volume has an AWS Marketplace product code:
*
*
*
* - The volume can be attached only to a stopped instance.
* - AWS Marketplace product codes are copied from the volume to the
* instance.
* - You must be subscribed to the product.
* - The instance type and operating system of the instance must
* support the product. For example, you can't detach a volume from a
* Windows instance and attach it to a Linux instance.
*
*
*
* For an overview of the AWS Marketplace, see
* Introducing AWS Marketplace
* .
*
*
* For more information about EBS volumes, see
* Attaching Amazon EBS Volumes
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param attachVolumeRequest Container for the necessary parameters to
* execute the AttachVolume operation on AmazonEC2.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* AttachVolume service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future attachVolumeAsync(
final AttachVolumeRequest attachVolumeRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public AttachVolumeResult call() throws Exception {
AttachVolumeResult result;
try {
result = attachVolume(attachVolumeRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(attachVolumeRequest, result);
return result;
}
});
}
/**
*
* Disables ClassicLink DNS support for a VPC. If disabled, DNS
* hostnames resolve to public IP addresses when addressed between a
* linked EC2-Classic instance and instances in the VPC to which it's
* linked. For more information about ClassicLink, see
* ClassicLink
* in the Amazon Elastic Compute Cloud User Guide.
*
*
* @param disableVpcClassicLinkDnsSupportRequest Container for the
* necessary parameters to execute the DisableVpcClassicLinkDnsSupport
* operation on AmazonEC2.
*
* @return A Java Future object containing the response from the
* DisableVpcClassicLinkDnsSupport service method, as returned by
* AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future disableVpcClassicLinkDnsSupportAsync(final DisableVpcClassicLinkDnsSupportRequest disableVpcClassicLinkDnsSupportRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DisableVpcClassicLinkDnsSupportResult call() throws Exception {
return disableVpcClassicLinkDnsSupport(disableVpcClassicLinkDnsSupportRequest);
}
});
}
/**
*
* Disables ClassicLink DNS support for a VPC. If disabled, DNS
* hostnames resolve to public IP addresses when addressed between a
* linked EC2-Classic instance and instances in the VPC to which it's
* linked. For more information about ClassicLink, see
* ClassicLink
* in the Amazon Elastic Compute Cloud User Guide.
*
*
* @param disableVpcClassicLinkDnsSupportRequest Container for the
* necessary parameters to execute the DisableVpcClassicLinkDnsSupport
* operation on AmazonEC2.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DisableVpcClassicLinkDnsSupport service method, as returned by
* AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future disableVpcClassicLinkDnsSupportAsync(
final DisableVpcClassicLinkDnsSupportRequest disableVpcClassicLinkDnsSupportRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DisableVpcClassicLinkDnsSupportResult call() throws Exception {
DisableVpcClassicLinkDnsSupportResult result;
try {
result = disableVpcClassicLinkDnsSupport(disableVpcClassicLinkDnsSupportRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(disableVpcClassicLinkDnsSupportRequest, result);
return result;
}
});
}
/**
*
* Attaches an Internet gateway to a VPC, enabling connectivity between
* the Internet and the VPC. For more information about your VPC and
* Internet gateway, see the
* Amazon Virtual Private Cloud User Guide
* .
*
*
* @param attachInternetGatewayRequest Container for the necessary
* parameters to execute the AttachInternetGateway operation on
* AmazonEC2.
*
* @return A Java Future object containing the response from the
* AttachInternetGateway service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future attachInternetGatewayAsync(final AttachInternetGatewayRequest attachInternetGatewayRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
attachInternetGateway(attachInternetGatewayRequest);
return null;
}
});
}
/**
*
* Attaches an Internet gateway to a VPC, enabling connectivity between
* the Internet and the VPC. For more information about your VPC and
* Internet gateway, see the
* Amazon Virtual Private Cloud User Guide
* .
*
*
* @param attachInternetGatewayRequest Container for the necessary
* parameters to execute the AttachInternetGateway operation on
* AmazonEC2.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* AttachInternetGateway service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future attachInternetGatewayAsync(
final AttachInternetGatewayRequest attachInternetGatewayRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
try {
attachInternetGateway(attachInternetGatewayRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(attachInternetGatewayRequest, null);
return null;
}
});
}
/**
*
* Enables a virtual private gateway (VGW) to propagate routes to the
* specified route table of a VPC.
*
*
* @param enableVgwRoutePropagationRequest Container for the necessary
* parameters to execute the EnableVgwRoutePropagation operation on
* AmazonEC2.
*
* @return A Java Future object containing the response from the
* EnableVgwRoutePropagation service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future enableVgwRoutePropagationAsync(final EnableVgwRoutePropagationRequest enableVgwRoutePropagationRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
enableVgwRoutePropagation(enableVgwRoutePropagationRequest);
return null;
}
});
}
/**
*
* Enables a virtual private gateway (VGW) to propagate routes to the
* specified route table of a VPC.
*
*
* @param enableVgwRoutePropagationRequest Container for the necessary
* parameters to execute the EnableVgwRoutePropagation operation on
* AmazonEC2.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* EnableVgwRoutePropagation service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future enableVgwRoutePropagationAsync(
final EnableVgwRoutePropagationRequest enableVgwRoutePropagationRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
try {
enableVgwRoutePropagation(enableVgwRoutePropagationRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(enableVgwRoutePropagationRequest, null);
return null;
}
});
}
/**
*
* Allocates a Dedicated host to your account. At minimum you need to
* specify the instance size type, Availability Zone, and quantity of
* hosts you want to allocate.
*
*
* @param allocateHostsRequest Container for the necessary parameters to
* execute the AllocateHosts operation on AmazonEC2.
*
* @return A Java Future object containing the response from the
* AllocateHosts service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future allocateHostsAsync(final AllocateHostsRequest allocateHostsRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public AllocateHostsResult call() throws Exception {
return allocateHosts(allocateHostsRequest);
}
});
}
/**
*
* Allocates a Dedicated host to your account. At minimum you need to
* specify the instance size type, Availability Zone, and quantity of
* hosts you want to allocate.
*
*
* @param allocateHostsRequest Container for the necessary parameters to
* execute the AllocateHosts operation on AmazonEC2.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* AllocateHosts service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future allocateHostsAsync(
final AllocateHostsRequest allocateHostsRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public AllocateHostsResult call() throws Exception {
AllocateHostsResult result;
try {
result = allocateHosts(allocateHostsRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(allocateHostsRequest, result);
return result;
}
});
}
/**
*
* Describes the ClassicLink status of one or more VPCs.
*
*
* @param describeVpcClassicLinkRequest Container for the necessary
* parameters to execute the DescribeVpcClassicLink operation on
* AmazonEC2.
*
* @return A Java Future object containing the response from the
* DescribeVpcClassicLink service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeVpcClassicLinkAsync(final DescribeVpcClassicLinkRequest describeVpcClassicLinkRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeVpcClassicLinkResult call() throws Exception {
return describeVpcClassicLink(describeVpcClassicLinkRequest);
}
});
}
/**
*
* Describes the ClassicLink status of one or more VPCs.
*
*
* @param describeVpcClassicLinkRequest Container for the necessary
* parameters to execute the DescribeVpcClassicLink operation on
* AmazonEC2.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeVpcClassicLink service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeVpcClassicLinkAsync(
final DescribeVpcClassicLinkRequest describeVpcClassicLinkRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeVpcClassicLinkResult call() throws Exception {
DescribeVpcClassicLinkResult result;
try {
result = describeVpcClassicLink(describeVpcClassicLinkRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(describeVpcClassicLinkRequest, result);
return result;
}
});
}
/**
*
* Attaches a virtual private gateway to a VPC. For more information,
* see
* Adding a Hardware Virtual Private Gateway to Your VPC
* in the Amazon Virtual Private Cloud User Guide .
*
*
* @param attachVpnGatewayRequest Container for the necessary parameters
* to execute the AttachVpnGateway operation on AmazonEC2.
*
* @return A Java Future object containing the response from the
* AttachVpnGateway service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future attachVpnGatewayAsync(final AttachVpnGatewayRequest attachVpnGatewayRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public AttachVpnGatewayResult call() throws Exception {
return attachVpnGateway(attachVpnGatewayRequest);
}
});
}
/**
*
* Attaches a virtual private gateway to a VPC. For more information,
* see
* Adding a Hardware Virtual Private Gateway to Your VPC
* in the Amazon Virtual Private Cloud User Guide .
*
*
* @param attachVpnGatewayRequest Container for the necessary parameters
* to execute the AttachVpnGateway operation on AmazonEC2.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* AttachVpnGateway service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future attachVpnGatewayAsync(
final AttachVpnGatewayRequest attachVpnGatewayRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public AttachVpnGatewayResult call() throws Exception {
AttachVpnGatewayResult result;
try {
result = attachVpnGateway(attachVpnGatewayRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(attachVpnGatewayRequest, result);
return result;
}
});
}
/**
*
* Cancels an active export task. The request removes all artifacts of
* the export, including any partially-created Amazon S3 objects. If the
* export task is complete or is in the process of transferring the final
* disk image, the command fails and returns an error.
*
*
* @param cancelExportTaskRequest Container for the necessary parameters
* to execute the CancelExportTask operation on AmazonEC2.
*
* @return A Java Future object containing the response from the
* CancelExportTask service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future cancelExportTaskAsync(final CancelExportTaskRequest cancelExportTaskRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
cancelExportTask(cancelExportTaskRequest);
return null;
}
});
}
/**
*
* Cancels an active export task. The request removes all artifacts of
* the export, including any partially-created Amazon S3 objects. If the
* export task is complete or is in the process of transferring the final
* disk image, the command fails and returns an error.
*
*
* @param cancelExportTaskRequest Container for the necessary parameters
* to execute the CancelExportTask operation on AmazonEC2.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* CancelExportTask service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future cancelExportTaskAsync(
final CancelExportTaskRequest cancelExportTaskRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
try {
cancelExportTask(cancelExportTaskRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(cancelExportTaskRequest, null);
return null;
}
});
}
/**
*
* Describes one or more of your instances.
*
*
* If you specify one or more instance IDs, Amazon EC2 returns
* information for those instances. If you do not specify instance IDs,
* Amazon EC2 returns information for all relevant instances. If you
* specify an instance ID that is not valid, an error is returned. If you
* specify an instance that you do not own, it is not included in the
* returned results.
*
*
* Recently terminated instances might appear in the returned results.
* This interval is usually less than one hour.
*
*
* @param describeInstancesRequest Container for the necessary parameters
* to execute the DescribeInstances operation on AmazonEC2.
*
* @return A Java Future object containing the response from the
* DescribeInstances service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeInstancesAsync(final DescribeInstancesRequest describeInstancesRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeInstancesResult call() throws Exception {
return describeInstances(describeInstancesRequest);
}
});
}
/**
*
* Describes one or more of your instances.
*
*
* If you specify one or more instance IDs, Amazon EC2 returns
* information for those instances. If you do not specify instance IDs,
* Amazon EC2 returns information for all relevant instances. If you
* specify an instance ID that is not valid, an error is returned. If you
* specify an instance that you do not own, it is not included in the
* returned results.
*
*
* Recently terminated instances might appear in the returned results.
* This interval is usually less than one hour.
*
*
* @param describeInstancesRequest Container for the necessary parameters
* to execute the DescribeInstances operation on AmazonEC2.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeInstances service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeInstancesAsync(
final DescribeInstancesRequest describeInstancesRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeInstancesResult call() throws Exception {
DescribeInstancesResult result;
try {
result = describeInstances(describeInstancesRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(describeInstancesRequest, result);
return result;
}
});
}
/**
*
* Launches the specified Scheduled Instances.
*
*
* Before you can launch a Scheduled Instance, you must purchase it and
* obtain an identifier using PurchaseScheduledInstances.
*
*
* You must launch a Scheduled Instance during its scheduled time
* period. You can't stop or reboot a Scheduled Instance, but you can
* terminate it as needed. If you terminate a Scheduled Instance before
* the current scheduled time period ends, you can launch it again after
* a few minutes.
*
*
* @param runScheduledInstancesRequest Container for the necessary
* parameters to execute the RunScheduledInstances operation on
* AmazonEC2.
*
* @return A Java Future object containing the response from the
* RunScheduledInstances service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future runScheduledInstancesAsync(final RunScheduledInstancesRequest runScheduledInstancesRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public RunScheduledInstancesResult call() throws Exception {
return runScheduledInstances(runScheduledInstancesRequest);
}
});
}
/**
*
* Launches the specified Scheduled Instances.
*
*
* Before you can launch a Scheduled Instance, you must purchase it and
* obtain an identifier using PurchaseScheduledInstances.
*
*
* You must launch a Scheduled Instance during its scheduled time
* period. You can't stop or reboot a Scheduled Instance, but you can
* terminate it as needed. If you terminate a Scheduled Instance before
* the current scheduled time period ends, you can launch it again after
* a few minutes.
*
*
* @param runScheduledInstancesRequest Container for the necessary
* parameters to execute the RunScheduledInstances operation on
* AmazonEC2.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* RunScheduledInstances service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future runScheduledInstancesAsync(
final RunScheduledInstancesRequest runScheduledInstancesRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public RunScheduledInstancesResult call() throws Exception {
RunScheduledInstancesResult result;
try {
result = runScheduledInstances(runScheduledInstancesRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(runScheduledInstancesRequest, result);
return result;
}
});
}
/**
*
* Import single or multi-volume disk images or EBS snapshots into an
* Amazon Machine Image (AMI).
*
*
* @param importImageRequest Container for the necessary parameters to
* execute the ImportImage operation on AmazonEC2.
*
* @return A Java Future object containing the response from the
* ImportImage service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future importImageAsync(final ImportImageRequest importImageRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public ImportImageResult call() throws Exception {
return importImage(importImageRequest);
}
});
}
/**
*
* Import single or multi-volume disk images or EBS snapshots into an
* Amazon Machine Image (AMI).
*
*
* @param importImageRequest Container for the necessary parameters to
* execute the ImportImage operation on AmazonEC2.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* ImportImage service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future importImageAsync(
final ImportImageRequest importImageRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public ImportImageResult call() throws Exception {
ImportImageResult result;
try {
result = importImage(importImageRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(importImageRequest, result);
return result;
}
});
}
/**
*
* Creates an Amazon EBS-backed AMI from an Amazon EBS-backed instance
* that is either running or stopped.
*
*
* If you customized your instance with instance store volumes or EBS
* volumes in addition to the root device volume, the new AMI contains
* block device mapping information for those volumes. When you launch an
* instance from this new AMI, the instance automatically launches with
* those additional volumes.
*
*
* For more information, see
* Creating Amazon EBS-Backed Linux AMIs
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param createImageRequest Container for the necessary parameters to
* execute the CreateImage operation on AmazonEC2.
*
* @return A Java Future object containing the response from the
* CreateImage service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createImageAsync(final CreateImageRequest createImageRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public CreateImageResult call() throws Exception {
return createImage(createImageRequest);
}
});
}
/**
*
* Creates an Amazon EBS-backed AMI from an Amazon EBS-backed instance
* that is either running or stopped.
*
*
* If you customized your instance with instance store volumes or EBS
* volumes in addition to the root device volume, the new AMI contains
* block device mapping information for those volumes. When you launch an
* instance from this new AMI, the instance automatically launches with
* those additional volumes.
*
*
* For more information, see
* Creating Amazon EBS-Backed Linux AMIs
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param createImageRequest Container for the necessary parameters to
* execute the CreateImage operation on AmazonEC2.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* CreateImage service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createImageAsync(
final CreateImageRequest createImageRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public CreateImageResult call() throws Exception {
CreateImageResult result;
try {
result = createImage(createImageRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(createImageRequest, result);
return result;
}
});
}
/**
*
* Deletes the specified EBS volume. The volume must be in the
* available
state (not attached to an instance).
*
*
* NOTE: The volume may remain in the deleting state for several
* minutes.
*
*
* For more information, see
* Deleting an Amazon EBS Volume
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param deleteVolumeRequest Container for the necessary parameters to
* execute the DeleteVolume operation on AmazonEC2.
*
* @return A Java Future object containing the response from the
* DeleteVolume service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteVolumeAsync(final DeleteVolumeRequest deleteVolumeRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
deleteVolume(deleteVolumeRequest);
return null;
}
});
}
/**
*
* Deletes the specified EBS volume. The volume must be in the
* available
state (not attached to an instance).
*
*
* NOTE: The volume may remain in the deleting state for several
* minutes.
*
*
* For more information, see
* Deleting an Amazon EBS Volume
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param deleteVolumeRequest Container for the necessary parameters to
* execute the DeleteVolume operation on AmazonEC2.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DeleteVolume service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteVolumeAsync(
final DeleteVolumeRequest deleteVolumeRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
try {
deleteVolume(deleteVolumeRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(deleteVolumeRequest, null);
return null;
}
});
}
/**
*
* Describes your Elastic IP addresses that are being moved to the
* EC2-VPC platform, or that are being restored to the EC2-Classic
* platform. This request does not return information about any other
* Elastic IP addresses in your account.
*
*
* @param describeMovingAddressesRequest Container for the necessary
* parameters to execute the DescribeMovingAddresses operation on
* AmazonEC2.
*
* @return A Java Future object containing the response from the
* DescribeMovingAddresses service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeMovingAddressesAsync(final DescribeMovingAddressesRequest describeMovingAddressesRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeMovingAddressesResult call() throws Exception {
return describeMovingAddresses(describeMovingAddressesRequest);
}
});
}
/**
*
* Describes your Elastic IP addresses that are being moved to the
* EC2-VPC platform, or that are being restored to the EC2-Classic
* platform. This request does not return information about any other
* Elastic IP addresses in your account.
*
*
* @param describeMovingAddressesRequest Container for the necessary
* parameters to execute the DescribeMovingAddresses operation on
* AmazonEC2.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeMovingAddresses service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeMovingAddressesAsync(
final DescribeMovingAddressesRequest describeMovingAddressesRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeMovingAddressesResult call() throws Exception {
DescribeMovingAddressesResult result;
try {
result = describeMovingAddresses(describeMovingAddressesRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(describeMovingAddressesRequest, result);
return result;
}
});
}
/**
*
* Describes one or more of your VPC endpoints.
*
*
* @param describeVpcEndpointsRequest Container for the necessary
* parameters to execute the DescribeVpcEndpoints operation on AmazonEC2.
*
* @return A Java Future object containing the response from the
* DescribeVpcEndpoints service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeVpcEndpointsAsync(final DescribeVpcEndpointsRequest describeVpcEndpointsRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeVpcEndpointsResult call() throws Exception {
return describeVpcEndpoints(describeVpcEndpointsRequest);
}
});
}
/**
*
* Describes one or more of your VPC endpoints.
*
*
* @param describeVpcEndpointsRequest Container for the necessary
* parameters to execute the DescribeVpcEndpoints operation on AmazonEC2.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeVpcEndpoints service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeVpcEndpointsAsync(
final DescribeVpcEndpointsRequest describeVpcEndpointsRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeVpcEndpointsResult call() throws Exception {
DescribeVpcEndpointsResult result;
try {
result = describeVpcEndpoints(describeVpcEndpointsRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(describeVpcEndpointsRequest, result);
return result;
}
});
}
/**
*
* Deletes the specified subnet. You must terminate all running
* instances in the subnet before you can delete the subnet.
*
*
* @param deleteSubnetRequest Container for the necessary parameters to
* execute the DeleteSubnet operation on AmazonEC2.
*
* @return A Java Future object containing the response from the
* DeleteSubnet service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteSubnetAsync(final DeleteSubnetRequest deleteSubnetRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
deleteSubnet(deleteSubnetRequest);
return null;
}
});
}
/**
*
* Deletes the specified subnet. You must terminate all running
* instances in the subnet before you can delete the subnet.
*
*
* @param deleteSubnetRequest Container for the necessary parameters to
* execute the DeleteSubnet operation on AmazonEC2.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DeleteSubnet service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteSubnetAsync(
final DeleteSubnetRequest deleteSubnetRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
try {
deleteSubnet(deleteSubnetRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(deleteSubnetRequest, null);
return null;
}
});
}
/**
*
* Describes one or more of your linked EC2-Classic instances. This
* request only returns information about EC2-Classic instances linked to
* a VPC through ClassicLink; you cannot use this request to return
* information about other instances.
*
*
* @param describeClassicLinkInstancesRequest Container for the necessary
* parameters to execute the DescribeClassicLinkInstances operation on
* AmazonEC2.
*
* @return A Java Future object containing the response from the
* DescribeClassicLinkInstances service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeClassicLinkInstancesAsync(final DescribeClassicLinkInstancesRequest describeClassicLinkInstancesRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeClassicLinkInstancesResult call() throws Exception {
return describeClassicLinkInstances(describeClassicLinkInstancesRequest);
}
});
}
/**
*
* Describes one or more of your linked EC2-Classic instances. This
* request only returns information about EC2-Classic instances linked to
* a VPC through ClassicLink; you cannot use this request to return
* information about other instances.
*
*
* @param describeClassicLinkInstancesRequest Container for the necessary
* parameters to execute the DescribeClassicLinkInstances operation on
* AmazonEC2.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeClassicLinkInstances service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeClassicLinkInstancesAsync(
final DescribeClassicLinkInstancesRequest describeClassicLinkInstancesRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeClassicLinkInstancesResult call() throws Exception {
DescribeClassicLinkInstancesResult result;
try {
result = describeClassicLinkInstances(describeClassicLinkInstancesRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(describeClassicLinkInstancesRequest, result);
return result;
}
});
}
/**
*
* Describes Reserved Instance offerings that are available for
* purchase. With Reserved Instances, you purchase the right to launch
* instances for a period of time. During that time period, you do not
* receive insufficient capacity errors, and you pay a lower usage rate
* than the rate charged for On-Demand instances for the actual time
* used.
*
*
* If you have listed your own Reserved Instances for sale in the
* Reserved Instance Marketplace, they will be excluded from these
* results. This is to ensure that you do not purchase your own Reserved
* Instances.
*
*
* For more information, see
* Reserved Instance Marketplace
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param describeReservedInstancesOfferingsRequest Container for the
* necessary parameters to execute the DescribeReservedInstancesOfferings
* operation on AmazonEC2.
*
* @return A Java Future object containing the response from the
* DescribeReservedInstancesOfferings service method, as returned by
* AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeReservedInstancesOfferingsAsync(final DescribeReservedInstancesOfferingsRequest describeReservedInstancesOfferingsRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeReservedInstancesOfferingsResult call() throws Exception {
return describeReservedInstancesOfferings(describeReservedInstancesOfferingsRequest);
}
});
}
/**
*
* Describes Reserved Instance offerings that are available for
* purchase. With Reserved Instances, you purchase the right to launch
* instances for a period of time. During that time period, you do not
* receive insufficient capacity errors, and you pay a lower usage rate
* than the rate charged for On-Demand instances for the actual time
* used.
*
*
* If you have listed your own Reserved Instances for sale in the
* Reserved Instance Marketplace, they will be excluded from these
* results. This is to ensure that you do not purchase your own Reserved
* Instances.
*
*
* For more information, see
* Reserved Instance Marketplace
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param describeReservedInstancesOfferingsRequest Container for the
* necessary parameters to execute the DescribeReservedInstancesOfferings
* operation on AmazonEC2.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeReservedInstancesOfferings service method, as returned by
* AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeReservedInstancesOfferingsAsync(
final DescribeReservedInstancesOfferingsRequest describeReservedInstancesOfferingsRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeReservedInstancesOfferingsResult call() throws Exception {
DescribeReservedInstancesOfferingsResult result;
try {
result = describeReservedInstancesOfferings(describeReservedInstancesOfferingsRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(describeReservedInstancesOfferingsRequest, result);
return result;
}
});
}
/**
*
* Deletes a VPC peering connection. Either the owner of the requester
* VPC or the owner of the peer VPC can delete the VPC peering connection
* if it's in the active
state. The owner of the requester
* VPC can delete a VPC peering connection in the
* pending-acceptance
state.
*
*
* @param deleteVpcPeeringConnectionRequest Container for the necessary
* parameters to execute the DeleteVpcPeeringConnection operation on
* AmazonEC2.
*
* @return A Java Future object containing the response from the
* DeleteVpcPeeringConnection service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteVpcPeeringConnectionAsync(final DeleteVpcPeeringConnectionRequest deleteVpcPeeringConnectionRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DeleteVpcPeeringConnectionResult call() throws Exception {
return deleteVpcPeeringConnection(deleteVpcPeeringConnectionRequest);
}
});
}
/**
*
* Deletes a VPC peering connection. Either the owner of the requester
* VPC or the owner of the peer VPC can delete the VPC peering connection
* if it's in the active
state. The owner of the requester
* VPC can delete a VPC peering connection in the
* pending-acceptance
state.
*
*
* @param deleteVpcPeeringConnectionRequest Container for the necessary
* parameters to execute the DeleteVpcPeeringConnection operation on
* AmazonEC2.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DeleteVpcPeeringConnection service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteVpcPeeringConnectionAsync(
final DeleteVpcPeeringConnectionRequest deleteVpcPeeringConnectionRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DeleteVpcPeeringConnectionResult call() throws Exception {
DeleteVpcPeeringConnectionResult result;
try {
result = deleteVpcPeeringConnection(deleteVpcPeeringConnectionRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(deleteVpcPeeringConnectionRequest, result);
return result;
}
});
}
/**
*
* Launches the specified number of instances using an AMI for which you
* have permissions.
*
*
* When you launch an instance, it enters the pending
* state. After the instance is ready for you, it enters the
* running
state. To check the state of your instance, call
* DescribeInstances.
*
*
* To ensure faster instance launches, break up large requests into
* smaller batches. For example, create five separate launch requests for
* 100 instances each instead of one launch request for 500 instances.
*
*
* If you don't specify a security group when launching an instance,
* Amazon EC2 uses the default security group. For more information, see
* Security Groups
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* [EC2-VPC only accounts] If you don't specify a subnet in the request,
* we choose a default subnet from your default VPC for you.
*
*
* [EC2-Classic accounts] If you're launching into EC2-Classic and you
* don't specify an Availability Zone, we choose one for you.
*
*
* Linux instances have access to the public key of the key pair at
* boot. You can use this key to provide secure access to the instance.
* Amazon EC2 public images use this feature to provide secure access
* without passwords. For more information, see
* Key Pairs
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* You can provide optional user data when launching an instance. For
* more information, see
* Instance Metadata
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* If any of the AMIs have a product code attached for which the user
* has not subscribed, RunInstances
fails.
*
*
* Some instance types can only be launched into a VPC. If you do not
* have a default VPC, or if you do not specify a subnet ID in the
* request, RunInstances
fails. For more information, see
* Instance Types Available Only in a VPC
* .
*
*
* For more information about troubleshooting, see
* What To Do If An Instance Immediately Terminates , and Troubleshooting Connecting to Your Instance
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param runInstancesRequest Container for the necessary parameters to
* execute the RunInstances operation on AmazonEC2.
*
* @return A Java Future object containing the response from the
* RunInstances service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future runInstancesAsync(final RunInstancesRequest runInstancesRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public RunInstancesResult call() throws Exception {
return runInstances(runInstancesRequest);
}
});
}
/**
*
* Launches the specified number of instances using an AMI for which you
* have permissions.
*
*
* When you launch an instance, it enters the pending
* state. After the instance is ready for you, it enters the
* running
state. To check the state of your instance, call
* DescribeInstances.
*
*
* To ensure faster instance launches, break up large requests into
* smaller batches. For example, create five separate launch requests for
* 100 instances each instead of one launch request for 500 instances.
*
*
* If you don't specify a security group when launching an instance,
* Amazon EC2 uses the default security group. For more information, see
* Security Groups
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* [EC2-VPC only accounts] If you don't specify a subnet in the request,
* we choose a default subnet from your default VPC for you.
*
*
* [EC2-Classic accounts] If you're launching into EC2-Classic and you
* don't specify an Availability Zone, we choose one for you.
*
*
* Linux instances have access to the public key of the key pair at
* boot. You can use this key to provide secure access to the instance.
* Amazon EC2 public images use this feature to provide secure access
* without passwords. For more information, see
* Key Pairs
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* You can provide optional user data when launching an instance. For
* more information, see
* Instance Metadata
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* If any of the AMIs have a product code attached for which the user
* has not subscribed, RunInstances
fails.
*
*
* Some instance types can only be launched into a VPC. If you do not
* have a default VPC, or if you do not specify a subnet ID in the
* request, RunInstances
fails. For more information, see
* Instance Types Available Only in a VPC
* .
*
*
* For more information about troubleshooting, see
* What To Do If An Instance Immediately Terminates , and Troubleshooting Connecting to Your Instance
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param runInstancesRequest Container for the necessary parameters to
* execute the RunInstances operation on AmazonEC2.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* RunInstances service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future runInstancesAsync(
final RunInstancesRequest runInstancesRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public RunInstancesResult call() throws Exception {
RunInstancesResult result;
try {
result = runInstances(runInstancesRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(runInstancesRequest, result);
return result;
}
});
}
/**
*
* Enables I/O operations for a volume that had I/O operations disabled
* because the data on the volume was potentially inconsistent.
*
*
* @param enableVolumeIORequest Container for the necessary parameters to
* execute the EnableVolumeIO operation on AmazonEC2.
*
* @return A Java Future object containing the response from the
* EnableVolumeIO service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future enableVolumeIOAsync(final EnableVolumeIORequest enableVolumeIORequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
enableVolumeIO(enableVolumeIORequest);
return null;
}
});
}
/**
*
* Enables I/O operations for a volume that had I/O operations disabled
* because the data on the volume was potentially inconsistent.
*
*
* @param enableVolumeIORequest Container for the necessary parameters to
* execute the EnableVolumeIO operation on AmazonEC2.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* EnableVolumeIO service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future enableVolumeIOAsync(
final EnableVolumeIORequest enableVolumeIORequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
try {
enableVolumeIO(enableVolumeIORequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(enableVolumeIORequest, null);
return null;
}
});
}
/**
*
* Describes the events for the specified Spot fleet request during the
* specified time.
*
*
* Spot fleet events are delayed by up to 30 seconds before they can be
* described. This ensures that you can query by the last evaluated time
* and not miss a recorded event.
*
*
* @param describeSpotFleetRequestHistoryRequest Container for the
* necessary parameters to execute the DescribeSpotFleetRequestHistory
* operation on AmazonEC2.
*
* @return A Java Future object containing the response from the
* DescribeSpotFleetRequestHistory service method, as returned by
* AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeSpotFleetRequestHistoryAsync(final DescribeSpotFleetRequestHistoryRequest describeSpotFleetRequestHistoryRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeSpotFleetRequestHistoryResult call() throws Exception {
return describeSpotFleetRequestHistory(describeSpotFleetRequestHistoryRequest);
}
});
}
/**
*
* Describes the events for the specified Spot fleet request during the
* specified time.
*
*
* Spot fleet events are delayed by up to 30 seconds before they can be
* described. This ensures that you can query by the last evaluated time
* and not miss a recorded event.
*
*
* @param describeSpotFleetRequestHistoryRequest Container for the
* necessary parameters to execute the DescribeSpotFleetRequestHistory
* operation on AmazonEC2.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeSpotFleetRequestHistory service method, as returned by
* AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeSpotFleetRequestHistoryAsync(
final DescribeSpotFleetRequestHistoryRequest describeSpotFleetRequestHistoryRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeSpotFleetRequestHistoryResult call() throws Exception {
DescribeSpotFleetRequestHistoryResult result;
try {
result = describeSpotFleetRequestHistory(describeSpotFleetRequestHistoryRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(describeSpotFleetRequestHistoryRequest, result);
return result;
}
});
}
/**
*
* Creates a data feed for Spot instances, enabling you to view Spot
* instance usage logs. You can create one data feed per AWS account. For
* more information, see
* Spot Instance Data Feed
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param createSpotDatafeedSubscriptionRequest Container for the
* necessary parameters to execute the CreateSpotDatafeedSubscription
* operation on AmazonEC2.
*
* @return A Java Future object containing the response from the
* CreateSpotDatafeedSubscription service method, as returned by
* AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createSpotDatafeedSubscriptionAsync(final CreateSpotDatafeedSubscriptionRequest createSpotDatafeedSubscriptionRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public CreateSpotDatafeedSubscriptionResult call() throws Exception {
return createSpotDatafeedSubscription(createSpotDatafeedSubscriptionRequest);
}
});
}
/**
*
* Creates a data feed for Spot instances, enabling you to view Spot
* instance usage logs. You can create one data feed per AWS account. For
* more information, see
* Spot Instance Data Feed
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param createSpotDatafeedSubscriptionRequest Container for the
* necessary parameters to execute the CreateSpotDatafeedSubscription
* operation on AmazonEC2.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* CreateSpotDatafeedSubscription service method, as returned by
* AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createSpotDatafeedSubscriptionAsync(
final CreateSpotDatafeedSubscriptionRequest createSpotDatafeedSubscriptionRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public CreateSpotDatafeedSubscriptionResult call() throws Exception {
CreateSpotDatafeedSubscriptionResult result;
try {
result = createSpotDatafeedSubscription(createSpotDatafeedSubscriptionRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(createSpotDatafeedSubscriptionRequest, result);
return result;
}
});
}
/**
*
* Restores an Elastic IP address that was previously moved to the
* EC2-VPC platform back to the EC2-Classic platform. You cannot move an
* Elastic IP address that was originally allocated for use in EC2-VPC.
* The Elastic IP address must not be associated with an instance or
* network interface. You cannot restore an Elastic IP address that's
* associated with a reverse DNS record. Contact AWS account and billing
* support to remove the reverse DNS record.
*
*
* @param restoreAddressToClassicRequest Container for the necessary
* parameters to execute the RestoreAddressToClassic operation on
* AmazonEC2.
*
* @return A Java Future object containing the response from the
* RestoreAddressToClassic service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future restoreAddressToClassicAsync(final RestoreAddressToClassicRequest restoreAddressToClassicRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public RestoreAddressToClassicResult call() throws Exception {
return restoreAddressToClassic(restoreAddressToClassicRequest);
}
});
}
/**
*
* Restores an Elastic IP address that was previously moved to the
* EC2-VPC platform back to the EC2-Classic platform. You cannot move an
* Elastic IP address that was originally allocated for use in EC2-VPC.
* The Elastic IP address must not be associated with an instance or
* network interface. You cannot restore an Elastic IP address that's
* associated with a reverse DNS record. Contact AWS account and billing
* support to remove the reverse DNS record.
*
*
* @param restoreAddressToClassicRequest Container for the necessary
* parameters to execute the RestoreAddressToClassic operation on
* AmazonEC2.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* RestoreAddressToClassic service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future restoreAddressToClassicAsync(
final RestoreAddressToClassicRequest restoreAddressToClassicRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public RestoreAddressToClassicResult call() throws Exception {
RestoreAddressToClassicResult result;
try {
result = restoreAddressToClassic(restoreAddressToClassicRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(restoreAddressToClassicRequest, result);
return result;
}
});
}
/**
*
* Describes one or more of the images (AMIs, AKIs, and ARIs) available
* to you. Images available to you include public images, private images
* that you own, and private images owned by other AWS accounts but for
* which you have explicit launch permissions.
*
*
* NOTE: Deregistered images are included in the returned results
* for an unspecified interval after deregistration.
*
*
* @param describeImagesRequest Container for the necessary parameters to
* execute the DescribeImages operation on AmazonEC2.
*
* @return A Java Future object containing the response from the
* DescribeImages service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeImagesAsync(final DescribeImagesRequest describeImagesRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeImagesResult call() throws Exception {
return describeImages(describeImagesRequest);
}
});
}
/**
*
* Describes one or more of the images (AMIs, AKIs, and ARIs) available
* to you. Images available to you include public images, private images
* that you own, and private images owned by other AWS accounts but for
* which you have explicit launch permissions.
*
*
* NOTE: Deregistered images are included in the returned results
* for an unspecified interval after deregistration.
*
*
* @param describeImagesRequest Container for the necessary parameters to
* execute the DescribeImages operation on AmazonEC2.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeImages service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeImagesAsync(
final DescribeImagesRequest describeImagesRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeImagesResult call() throws Exception {
DescribeImagesResult result;
try {
result = describeImages(describeImagesRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(describeImagesRequest, result);
return result;
}
});
}
/**
*
* Describes one or more of your VPCs.
*
*
* @param describeVpcsRequest Container for the necessary parameters to
* execute the DescribeVpcs operation on AmazonEC2.
*
* @return A Java Future object containing the response from the
* DescribeVpcs service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeVpcsAsync(final DescribeVpcsRequest describeVpcsRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeVpcsResult call() throws Exception {
return describeVpcs(describeVpcsRequest);
}
});
}
/**
*
* Describes one or more of your VPCs.
*
*
* @param describeVpcsRequest Container for the necessary parameters to
* execute the DescribeVpcs operation on AmazonEC2.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeVpcs service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeVpcsAsync(
final DescribeVpcsRequest describeVpcsRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeVpcsResult call() throws Exception {
DescribeVpcsResult result;
try {
result = describeVpcs(describeVpcsRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(describeVpcsRequest, result);
return result;
}
});
}
/**
*
* [EC2-VPC only] Adds one or more egress rules to a security group for
* use with a VPC. Specifically, this action permits instances to send
* traffic to one or more destination CIDR IP address ranges, or to one
* or more destination security groups for the same VPC. This action
* doesn't apply to security groups for use in EC2-Classic. For more
* information, see
* Security Groups for Your VPC
* in the Amazon Virtual Private Cloud User Guide .
*
*
* IMPORTANT: You can have up to 50 rules per security group
* (covering both ingress and egress rules).
*
*
* Each rule consists of the protocol (for example, TCP), plus either a
* CIDR range or a source group. For the TCP and UDP protocols, you must
* also specify the destination port or port range. For the ICMP
* protocol, you must also specify the ICMP type and code. You can use -1
* for the type or code to mean all types or all codes.
*
*
* Rule changes are propagated to affected instances as quickly as
* possible. However, a small delay might occur.
*
*
* @param authorizeSecurityGroupEgressRequest Container for the necessary
* parameters to execute the AuthorizeSecurityGroupEgress operation on
* AmazonEC2.
*
* @return A Java Future object containing the response from the
* AuthorizeSecurityGroupEgress service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future authorizeSecurityGroupEgressAsync(final AuthorizeSecurityGroupEgressRequest authorizeSecurityGroupEgressRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
authorizeSecurityGroupEgress(authorizeSecurityGroupEgressRequest);
return null;
}
});
}
/**
*
* [EC2-VPC only] Adds one or more egress rules to a security group for
* use with a VPC. Specifically, this action permits instances to send
* traffic to one or more destination CIDR IP address ranges, or to one
* or more destination security groups for the same VPC. This action
* doesn't apply to security groups for use in EC2-Classic. For more
* information, see
* Security Groups for Your VPC
* in the Amazon Virtual Private Cloud User Guide .
*
*
* IMPORTANT: You can have up to 50 rules per security group
* (covering both ingress and egress rules).
*
*
* Each rule consists of the protocol (for example, TCP), plus either a
* CIDR range or a source group. For the TCP and UDP protocols, you must
* also specify the destination port or port range. For the ICMP
* protocol, you must also specify the ICMP type and code. You can use -1
* for the type or code to mean all types or all codes.
*
*
* Rule changes are propagated to affected instances as quickly as
* possible. However, a small delay might occur.
*
*
* @param authorizeSecurityGroupEgressRequest Container for the necessary
* parameters to execute the AuthorizeSecurityGroupEgress operation on
* AmazonEC2.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* AuthorizeSecurityGroupEgress service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future authorizeSecurityGroupEgressAsync(
final AuthorizeSecurityGroupEgressRequest authorizeSecurityGroupEgressRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
try {
authorizeSecurityGroupEgress(authorizeSecurityGroupEgressRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(authorizeSecurityGroupEgressRequest, null);
return null;
}
});
}
/**
*
* Copies a point-in-time snapshot of an EBS volume and stores it in
* Amazon S3. You can copy the snapshot within the same region or from
* one region to another. You can use the snapshot to create EBS volumes
* or Amazon Machine Images (AMIs). The snapshot is copied to the
* regional endpoint that you send the HTTP request to.
*
*
* Copies of encrypted EBS snapshots remain encrypted. Copies of
* unencrypted snapshots remain unencrypted, unless the
* Encrypted
flag is specified during the snapshot copy
* operation. By default, encrypted snapshot copies use the default AWS
* Key Management Service (AWS KMS) customer master key (CMK); however,
* you can specify a non-default CMK with the KmsKeyId
* parameter.
*
*
* For more information, see
* Copying an Amazon EBS Snapshot
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param copySnapshotRequest Container for the necessary parameters to
* execute the CopySnapshot operation on AmazonEC2.
*
* @return A Java Future object containing the response from the
* CopySnapshot service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future copySnapshotAsync(final CopySnapshotRequest copySnapshotRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public CopySnapshotResult call() throws Exception {
return copySnapshot(copySnapshotRequest);
}
});
}
/**
*
* Copies a point-in-time snapshot of an EBS volume and stores it in
* Amazon S3. You can copy the snapshot within the same region or from
* one region to another. You can use the snapshot to create EBS volumes
* or Amazon Machine Images (AMIs). The snapshot is copied to the
* regional endpoint that you send the HTTP request to.
*
*
* Copies of encrypted EBS snapshots remain encrypted. Copies of
* unencrypted snapshots remain unencrypted, unless the
* Encrypted
flag is specified during the snapshot copy
* operation. By default, encrypted snapshot copies use the default AWS
* Key Management Service (AWS KMS) customer master key (CMK); however,
* you can specify a non-default CMK with the KmsKeyId
* parameter.
*
*
* For more information, see
* Copying an Amazon EBS Snapshot
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param copySnapshotRequest Container for the necessary parameters to
* execute the CopySnapshot operation on AmazonEC2.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* CopySnapshot service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future copySnapshotAsync(
final CopySnapshotRequest copySnapshotRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public CopySnapshotResult call() throws Exception {
CopySnapshotResult result;
try {
result = copySnapshot(copySnapshotRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(copySnapshotRequest, result);
return result;
}
});
}
/**
*
* Detaches a virtual private gateway from a VPC. You do this if you're
* planning to turn off the VPC and not use it anymore. You can confirm a
* virtual private gateway has been completely detached from a VPC by
* describing the virtual private gateway (any attachments to the virtual
* private gateway are also described).
*
*
* You must wait for the attachment's state to switch to
* detached
before you can delete the VPC or attach a
* different VPC to the virtual private gateway.
*
*
* @param detachVpnGatewayRequest Container for the necessary parameters
* to execute the DetachVpnGateway operation on AmazonEC2.
*
* @return A Java Future object containing the response from the
* DetachVpnGateway service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future detachVpnGatewayAsync(final DetachVpnGatewayRequest detachVpnGatewayRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
detachVpnGateway(detachVpnGatewayRequest);
return null;
}
});
}
/**
*
* Detaches a virtual private gateway from a VPC. You do this if you're
* planning to turn off the VPC and not use it anymore. You can confirm a
* virtual private gateway has been completely detached from a VPC by
* describing the virtual private gateway (any attachments to the virtual
* private gateway are also described).
*
*
* You must wait for the attachment's state to switch to
* detached
before you can delete the VPC or attach a
* different VPC to the virtual private gateway.
*
*
* @param detachVpnGatewayRequest Container for the necessary parameters
* to execute the DetachVpnGateway operation on AmazonEC2.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DetachVpnGateway service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future detachVpnGatewayAsync(
final DetachVpnGatewayRequest detachVpnGatewayRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
try {
detachVpnGateway(detachVpnGatewayRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(detachVpnGatewayRequest, null);
return null;
}
});
}
/**
*
* Describes the specified attribute of the specified instance. You can
* specify only one attribute at a time. Valid attribute values are:
* instanceType
| kernel
| ramdisk
* | userData
| disableApiTermination
|
* instanceInitiatedShutdownBehavior
|
* rootDeviceName
| blockDeviceMapping
|
* productCodes
| sourceDestCheck
|
* groupSet
| ebsOptimized
|
* sriovNetSupport
*
*
* @param describeInstanceAttributeRequest Container for the necessary
* parameters to execute the DescribeInstanceAttribute operation on
* AmazonEC2.
*
* @return A Java Future object containing the response from the
* DescribeInstanceAttribute service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeInstanceAttributeAsync(final DescribeInstanceAttributeRequest describeInstanceAttributeRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeInstanceAttributeResult call() throws Exception {
return describeInstanceAttribute(describeInstanceAttributeRequest);
}
});
}
/**
*
* Describes the specified attribute of the specified instance. You can
* specify only one attribute at a time. Valid attribute values are:
* instanceType
| kernel
| ramdisk
* | userData
| disableApiTermination
|
* instanceInitiatedShutdownBehavior
|
* rootDeviceName
| blockDeviceMapping
|
* productCodes
| sourceDestCheck
|
* groupSet
| ebsOptimized
|
* sriovNetSupport
*
*
* @param describeInstanceAttributeRequest Container for the necessary
* parameters to execute the DescribeInstanceAttribute operation on
* AmazonEC2.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeInstanceAttribute service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeInstanceAttributeAsync(
final DescribeInstanceAttributeRequest describeInstanceAttributeRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeInstanceAttributeResult call() throws Exception {
DescribeInstanceAttributeResult result;
try {
result = describeInstanceAttribute(describeInstanceAttributeRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(describeInstanceAttributeRequest, result);
return result;
}
});
}
/**
*
* Describes the running instances for the specified Spot fleet.
*
*
* @param describeSpotFleetInstancesRequest Container for the necessary
* parameters to execute the DescribeSpotFleetInstances operation on
* AmazonEC2.
*
* @return A Java Future object containing the response from the
* DescribeSpotFleetInstances service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeSpotFleetInstancesAsync(final DescribeSpotFleetInstancesRequest describeSpotFleetInstancesRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeSpotFleetInstancesResult call() throws Exception {
return describeSpotFleetInstances(describeSpotFleetInstancesRequest);
}
});
}
/**
*
* Describes the running instances for the specified Spot fleet.
*
*
* @param describeSpotFleetInstancesRequest Container for the necessary
* parameters to execute the DescribeSpotFleetInstances operation on
* AmazonEC2.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeSpotFleetInstances service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeSpotFleetInstancesAsync(
final DescribeSpotFleetInstancesRequest describeSpotFleetInstancesRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeSpotFleetInstancesResult call() throws Exception {
DescribeSpotFleetInstancesResult result;
try {
result = describeSpotFleetInstances(describeSpotFleetInstancesRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(describeSpotFleetInstancesRequest, result);
return result;
}
});
}
/**
*
* Creates a network ACL in a VPC. Network ACLs provide an optional
* layer of security (in addition to security groups) for the instances
* in your VPC.
*
*
* For more information about network ACLs, see
* Network ACLs
* in the Amazon Virtual Private Cloud User Guide .
*
*
* @param createNetworkAclRequest Container for the necessary parameters
* to execute the CreateNetworkAcl operation on AmazonEC2.
*
* @return A Java Future object containing the response from the
* CreateNetworkAcl service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createNetworkAclAsync(final CreateNetworkAclRequest createNetworkAclRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public CreateNetworkAclResult call() throws Exception {
return createNetworkAcl(createNetworkAclRequest);
}
});
}
/**
*
* Creates a network ACL in a VPC. Network ACLs provide an optional
* layer of security (in addition to security groups) for the instances
* in your VPC.
*
*
* For more information about network ACLs, see
* Network ACLs
* in the Amazon Virtual Private Cloud User Guide .
*
*
* @param createNetworkAclRequest Container for the necessary parameters
* to execute the CreateNetworkAcl operation on AmazonEC2.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* CreateNetworkAcl service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createNetworkAclAsync(
final CreateNetworkAclRequest createNetworkAclRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public CreateNetworkAclResult call() throws Exception {
CreateNetworkAclResult result;
try {
result = createNetworkAcl(createNetworkAclRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(createNetworkAclRequest, result);
return result;
}
});
}
/**
*
* Describes the Spot price history. The prices returned are listed in
* chronological order, from the oldest to the most recent, for up to the
* past 90 days. For more information, see
* Spot Instance Pricing History
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* When you specify a start and end time, this operation returns the
* prices of the instance types within the time range that you specified
* and the time when the price changed. The price is valid within the
* time period that you specified; the response merely indicates the last
* time that the price changed.
*
*
* @param describeSpotPriceHistoryRequest Container for the necessary
* parameters to execute the DescribeSpotPriceHistory operation on
* AmazonEC2.
*
* @return A Java Future object containing the response from the
* DescribeSpotPriceHistory service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeSpotPriceHistoryAsync(final DescribeSpotPriceHistoryRequest describeSpotPriceHistoryRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeSpotPriceHistoryResult call() throws Exception {
return describeSpotPriceHistory(describeSpotPriceHistoryRequest);
}
});
}
/**
*
* Describes the Spot price history. The prices returned are listed in
* chronological order, from the oldest to the most recent, for up to the
* past 90 days. For more information, see
* Spot Instance Pricing History
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* When you specify a start and end time, this operation returns the
* prices of the instance types within the time range that you specified
* and the time when the price changed. The price is valid within the
* time period that you specified; the response merely indicates the last
* time that the price changed.
*
*
* @param describeSpotPriceHistoryRequest Container for the necessary
* parameters to execute the DescribeSpotPriceHistory operation on
* AmazonEC2.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeSpotPriceHistory service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeSpotPriceHistoryAsync(
final DescribeSpotPriceHistoryRequest describeSpotPriceHistoryRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeSpotPriceHistoryResult call() throws Exception {
DescribeSpotPriceHistoryResult result;
try {
result = describeSpotPriceHistory(describeSpotPriceHistoryRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(describeSpotPriceHistoryRequest, result);
return result;
}
});
}
/**
*
* Displays details about an import virtual machine or import snapshot
* tasks that are already created.
*
*
* @param describeImportImageTasksRequest Container for the necessary
* parameters to execute the DescribeImportImageTasks operation on
* AmazonEC2.
*
* @return A Java Future object containing the response from the
* DescribeImportImageTasks service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeImportImageTasksAsync(final DescribeImportImageTasksRequest describeImportImageTasksRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeImportImageTasksResult call() throws Exception {
return describeImportImageTasks(describeImportImageTasksRequest);
}
});
}
/**
*
* Displays details about an import virtual machine or import snapshot
* tasks that are already created.
*
*
* @param describeImportImageTasksRequest Container for the necessary
* parameters to execute the DescribeImportImageTasks operation on
* AmazonEC2.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeImportImageTasks service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeImportImageTasksAsync(
final DescribeImportImageTasksRequest describeImportImageTasksRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeImportImageTasksResult call() throws Exception {
DescribeImportImageTasksResult result;
try {
result = describeImportImageTasks(describeImportImageTasksRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(describeImportImageTasksRequest, result);
return result;
}
});
}
/**
*
* Describes one or more of your Internet gateways.
*
*
* @param describeInternetGatewaysRequest Container for the necessary
* parameters to execute the DescribeInternetGateways operation on
* AmazonEC2.
*
* @return A Java Future object containing the response from the
* DescribeInternetGateways service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeInternetGatewaysAsync(final DescribeInternetGatewaysRequest describeInternetGatewaysRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeInternetGatewaysResult call() throws Exception {
return describeInternetGateways(describeInternetGatewaysRequest);
}
});
}
/**
*
* Describes one or more of your Internet gateways.
*
*
* @param describeInternetGatewaysRequest Container for the necessary
* parameters to execute the DescribeInternetGateways operation on
* AmazonEC2.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeInternetGateways service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeInternetGatewaysAsync(
final DescribeInternetGatewaysRequest describeInternetGatewaysRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeInternetGatewaysResult call() throws Exception {
DescribeInternetGatewaysResult result;
try {
result = describeInternetGateways(describeInternetGatewaysRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(describeInternetGatewaysRequest, result);
return result;
}
});
}
/**
*
* Describes one or more of your Dedicated hosts.
*
*
* The results describe only the Dedicated hosts in the region you're
* currently using. All listed instances consume capacity on your
* Dedicated host. Dedicated hosts that have recently been released will
* be listed with the state released
.
*
*
* @param describeHostsRequest Container for the necessary parameters to
* execute the DescribeHosts operation on AmazonEC2.
*
* @return A Java Future object containing the response from the
* DescribeHosts service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeHostsAsync(final DescribeHostsRequest describeHostsRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeHostsResult call() throws Exception {
return describeHosts(describeHostsRequest);
}
});
}
/**
*
* Describes one or more of your Dedicated hosts.
*
*
* The results describe only the Dedicated hosts in the region you're
* currently using. All listed instances consume capacity on your
* Dedicated host. Dedicated hosts that have recently been released will
* be listed with the state released
.
*
*
* @param describeHostsRequest Container for the necessary parameters to
* execute the DescribeHosts operation on AmazonEC2.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeHosts service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeHostsAsync(
final DescribeHostsRequest describeHostsRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeHostsResult call() throws Exception {
DescribeHostsResult result;
try {
result = describeHosts(describeHostsRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(describeHostsRequest, result);
return result;
}
});
}
/**
*
* Describes one or more of your subnets.
*
*
* For more information about subnets, see
* Your VPC and Subnets
* in the Amazon Virtual Private Cloud User Guide .
*
*
* @param describeSubnetsRequest Container for the necessary parameters
* to execute the DescribeSubnets operation on AmazonEC2.
*
* @return A Java Future object containing the response from the
* DescribeSubnets service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeSubnetsAsync(final DescribeSubnetsRequest describeSubnetsRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeSubnetsResult call() throws Exception {
return describeSubnets(describeSubnetsRequest);
}
});
}
/**
*
* Describes one or more of your subnets.
*
*
* For more information about subnets, see
* Your VPC and Subnets
* in the Amazon Virtual Private Cloud User Guide .
*
*
* @param describeSubnetsRequest Container for the necessary parameters
* to execute the DescribeSubnets operation on AmazonEC2.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeSubnets service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeSubnetsAsync(
final DescribeSubnetsRequest describeSubnetsRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeSubnetsResult call() throws Exception {
DescribeSubnetsResult result;
try {
result = describeSubnets(describeSubnetsRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(describeSubnetsRequest, result);
return result;
}
});
}
/**
*
* Retrieves the encrypted administrator password for an instance
* running Windows.
*
*
* The Windows password is generated at boot if the
* EC2Config
service plugin, Ec2SetPassword
,
* is enabled. This usually only happens the first time an AMI is
* launched, and then Ec2SetPassword
is automatically
* disabled. The password is not generated for rebundled AMIs unless
* Ec2SetPassword
is enabled before bundling.
*
*
* The password is encrypted using the key pair that you specified when
* you launched the instance. You must provide the corresponding key pair
* file.
*
*
* Password generation and encryption takes a few moments. We recommend
* that you wait up to 15 minutes after launching an instance before
* trying to retrieve the generated password.
*
*
* @param getPasswordDataRequest Container for the necessary parameters
* to execute the GetPasswordData operation on AmazonEC2.
*
* @return A Java Future object containing the response from the
* GetPasswordData service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future getPasswordDataAsync(final GetPasswordDataRequest getPasswordDataRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public GetPasswordDataResult call() throws Exception {
return getPasswordData(getPasswordDataRequest);
}
});
}
/**
*
* Retrieves the encrypted administrator password for an instance
* running Windows.
*
*
* The Windows password is generated at boot if the
* EC2Config
service plugin, Ec2SetPassword
,
* is enabled. This usually only happens the first time an AMI is
* launched, and then Ec2SetPassword
is automatically
* disabled. The password is not generated for rebundled AMIs unless
* Ec2SetPassword
is enabled before bundling.
*
*
* The password is encrypted using the key pair that you specified when
* you launched the instance. You must provide the corresponding key pair
* file.
*
*
* Password generation and encryption takes a few moments. We recommend
* that you wait up to 15 minutes after launching an instance before
* trying to retrieve the generated password.
*
*
* @param getPasswordDataRequest Container for the necessary parameters
* to execute the GetPasswordData operation on AmazonEC2.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* GetPasswordData service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future getPasswordDataAsync(
final GetPasswordDataRequest getPasswordDataRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public GetPasswordDataResult call() throws Exception {
GetPasswordDataResult result;
try {
result = getPasswordData(getPasswordDataRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(getPasswordDataRequest, result);
return result;
}
});
}
/**
*