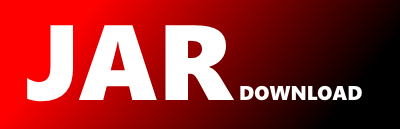
com.amazonaws.services.iot.model.AuthResult Maven / Gradle / Ivy
/*
* Copyright 2010-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.iot.model;
import java.io.Serializable;
/**
*
* The authorizer result.
*
*/
public class AuthResult implements Serializable {
/**
*
* Authorization information.
*
*/
private AuthInfo authInfo;
/**
*
* The policies and statements that allowed the specified action.
*
*/
private Allowed allowed;
/**
*
* The policies and statements that denied the specified action.
*
*/
private Denied denied;
/**
*
* The final authorization decision of this scenario. Multiple statements
* are taken into account when determining the authorization decision. An
* explicit deny statement can override multiple allow statements.
*
*
* Constraints:
* Allowed Values: ALLOWED, EXPLICIT_DENY, IMPLICIT_DENY
*/
private String authDecision;
/**
*
* Contains any missing context values found while evaluating policy.
*
*/
private java.util.List missingContextValues;
/**
*
* Authorization information.
*
*
* @return
* Authorization information.
*
*/
public AuthInfo getAuthInfo() {
return authInfo;
}
/**
*
* Authorization information.
*
*
* @param authInfo
* Authorization information.
*
*/
public void setAuthInfo(AuthInfo authInfo) {
this.authInfo = authInfo;
}
/**
*
* Authorization information.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* @param authInfo
* Authorization information.
*
* @return A reference to this updated object so that method calls can be
* chained together.
*/
public AuthResult withAuthInfo(AuthInfo authInfo) {
this.authInfo = authInfo;
return this;
}
/**
*
* The policies and statements that allowed the specified action.
*
*
* @return
* The policies and statements that allowed the specified action.
*
*/
public Allowed getAllowed() {
return allowed;
}
/**
*
* The policies and statements that allowed the specified action.
*
*
* @param allowed
* The policies and statements that allowed the specified action.
*
*/
public void setAllowed(Allowed allowed) {
this.allowed = allowed;
}
/**
*
* The policies and statements that allowed the specified action.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* @param allowed
* The policies and statements that allowed the specified action.
*
* @return A reference to this updated object so that method calls can be
* chained together.
*/
public AuthResult withAllowed(Allowed allowed) {
this.allowed = allowed;
return this;
}
/**
*
* The policies and statements that denied the specified action.
*
*
* @return
* The policies and statements that denied the specified action.
*
*/
public Denied getDenied() {
return denied;
}
/**
*
* The policies and statements that denied the specified action.
*
*
* @param denied
* The policies and statements that denied the specified action.
*
*/
public void setDenied(Denied denied) {
this.denied = denied;
}
/**
*
* The policies and statements that denied the specified action.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* @param denied
* The policies and statements that denied the specified action.
*
* @return A reference to this updated object so that method calls can be
* chained together.
*/
public AuthResult withDenied(Denied denied) {
this.denied = denied;
return this;
}
/**
*
* The final authorization decision of this scenario. Multiple statements
* are taken into account when determining the authorization decision. An
* explicit deny statement can override multiple allow statements.
*
*
* Constraints:
* Allowed Values: ALLOWED, EXPLICIT_DENY, IMPLICIT_DENY
*
* @return
* The final authorization decision of this scenario. Multiple
* statements are taken into account when determining the
* authorization decision. An explicit deny statement can override
* multiple allow statements.
*
* @see AuthDecision
*/
public String getAuthDecision() {
return authDecision;
}
/**
*
* The final authorization decision of this scenario. Multiple statements
* are taken into account when determining the authorization decision. An
* explicit deny statement can override multiple allow statements.
*
*
* Constraints:
* Allowed Values: ALLOWED, EXPLICIT_DENY, IMPLICIT_DENY
*
* @param authDecision
* The final authorization decision of this scenario. Multiple
* statements are taken into account when determining the
* authorization decision. An explicit deny statement can
* override multiple allow statements.
*
* @see AuthDecision
*/
public void setAuthDecision(String authDecision) {
this.authDecision = authDecision;
}
/**
*
* The final authorization decision of this scenario. Multiple statements
* are taken into account when determining the authorization decision. An
* explicit deny statement can override multiple allow statements.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* Constraints:
* Allowed Values: ALLOWED, EXPLICIT_DENY, IMPLICIT_DENY
*
* @param authDecision
* The final authorization decision of this scenario. Multiple
* statements are taken into account when determining the
* authorization decision. An explicit deny statement can
* override multiple allow statements.
*
* @return A reference to this updated object so that method calls can be
* chained together.
* @see AuthDecision
*/
public AuthResult withAuthDecision(String authDecision) {
this.authDecision = authDecision;
return this;
}
/**
*
* The final authorization decision of this scenario. Multiple statements
* are taken into account when determining the authorization decision. An
* explicit deny statement can override multiple allow statements.
*
*
* Constraints:
* Allowed Values: ALLOWED, EXPLICIT_DENY, IMPLICIT_DENY
*
* @param authDecision
* The final authorization decision of this scenario. Multiple
* statements are taken into account when determining the
* authorization decision. An explicit deny statement can
* override multiple allow statements.
*
* @see AuthDecision
*/
public void setAuthDecision(AuthDecision authDecision) {
this.authDecision = authDecision.toString();
}
/**
*
* The final authorization decision of this scenario. Multiple statements
* are taken into account when determining the authorization decision. An
* explicit deny statement can override multiple allow statements.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* Constraints:
* Allowed Values: ALLOWED, EXPLICIT_DENY, IMPLICIT_DENY
*
* @param authDecision
* The final authorization decision of this scenario. Multiple
* statements are taken into account when determining the
* authorization decision. An explicit deny statement can
* override multiple allow statements.
*
* @return A reference to this updated object so that method calls can be
* chained together.
* @see AuthDecision
*/
public AuthResult withAuthDecision(AuthDecision authDecision) {
this.authDecision = authDecision.toString();
return this;
}
/**
*
* Contains any missing context values found while evaluating policy.
*
*
* @return
* Contains any missing context values found while evaluating
* policy.
*
*/
public java.util.List getMissingContextValues() {
return missingContextValues;
}
/**
*
* Contains any missing context values found while evaluating policy.
*
*
* @param missingContextValues
* Contains any missing context values found while evaluating
* policy.
*
*/
public void setMissingContextValues(java.util.Collection missingContextValues) {
if (missingContextValues == null) {
this.missingContextValues = null;
return;
}
this.missingContextValues = new java.util.ArrayList(missingContextValues);
}
/**
*
* Contains any missing context values found while evaluating policy.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* @param missingContextValues
* Contains any missing context values found while evaluating
* policy.
*
* @return A reference to this updated object so that method calls can be
* chained together.
*/
public AuthResult withMissingContextValues(String... missingContextValues) {
if (getMissingContextValues() == null) {
this.missingContextValues = new java.util.ArrayList(missingContextValues.length);
}
for (String value : missingContextValues) {
this.missingContextValues.add(value);
}
return this;
}
/**
*
* Contains any missing context values found while evaluating policy.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* @param missingContextValues
* Contains any missing context values found while evaluating
* policy.
*
* @return A reference to this updated object so that method calls can be
* chained together.
*/
public AuthResult withMissingContextValues(java.util.Collection missingContextValues) {
setMissingContextValues(missingContextValues);
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAuthInfo() != null)
sb.append("authInfo: " + getAuthInfo() + ",");
if (getAllowed() != null)
sb.append("allowed: " + getAllowed() + ",");
if (getDenied() != null)
sb.append("denied: " + getDenied() + ",");
if (getAuthDecision() != null)
sb.append("authDecision: " + getAuthDecision() + ",");
if (getMissingContextValues() != null)
sb.append("missingContextValues: " + getMissingContextValues());
sb.append("}");
return sb.toString();
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAuthInfo() == null) ? 0 : getAuthInfo().hashCode());
hashCode = prime * hashCode + ((getAllowed() == null) ? 0 : getAllowed().hashCode());
hashCode = prime * hashCode + ((getDenied() == null) ? 0 : getDenied().hashCode());
hashCode = prime * hashCode
+ ((getAuthDecision() == null) ? 0 : getAuthDecision().hashCode());
hashCode = prime * hashCode
+ ((getMissingContextValues() == null) ? 0 : getMissingContextValues().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof AuthResult == false)
return false;
AuthResult other = (AuthResult) obj;
if (other.getAuthInfo() == null ^ this.getAuthInfo() == null)
return false;
if (other.getAuthInfo() != null && other.getAuthInfo().equals(this.getAuthInfo()) == false)
return false;
if (other.getAllowed() == null ^ this.getAllowed() == null)
return false;
if (other.getAllowed() != null && other.getAllowed().equals(this.getAllowed()) == false)
return false;
if (other.getDenied() == null ^ this.getDenied() == null)
return false;
if (other.getDenied() != null && other.getDenied().equals(this.getDenied()) == false)
return false;
if (other.getAuthDecision() == null ^ this.getAuthDecision() == null)
return false;
if (other.getAuthDecision() != null
&& other.getAuthDecision().equals(this.getAuthDecision()) == false)
return false;
if (other.getMissingContextValues() == null ^ this.getMissingContextValues() == null)
return false;
if (other.getMissingContextValues() != null
&& other.getMissingContextValues().equals(this.getMissingContextValues()) == false)
return false;
return true;
}
}