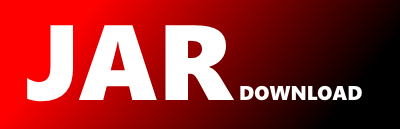
com.amazonaws.services.iot.model.AuthorizerDescription Maven / Gradle / Ivy
Show all versions of aws-android-sdk-iot Show documentation
/*
* Copyright 2010-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.iot.model;
import java.io.Serializable;
/**
*
* The authorizer description.
*
*/
public class AuthorizerDescription implements Serializable {
/**
*
* The authorizer name.
*
*
* Constraints:
* Length: 1 - 128
* Pattern: [\w=,@-]+
*/
private String authorizerName;
/**
*
* The authorizer ARN.
*
*/
private String authorizerArn;
/**
*
* The authorizer's Lambda function ARN.
*
*/
private String authorizerFunctionArn;
/**
*
* The key used to extract the token from the HTTP headers.
*
*
* Constraints:
* Length: 1 - 128
* Pattern: [a-zA-Z0-9_-]+
*/
private String tokenKeyName;
/**
*
* The public keys used to validate the token signature returned by your
* custom authentication service.
*
*/
private java.util.Map tokenSigningPublicKeys;
/**
*
* The status of the authorizer.
*
*
* Constraints:
* Allowed Values: ACTIVE, INACTIVE
*/
private String status;
/**
*
* The UNIX timestamp of when the authorizer was created.
*
*/
private java.util.Date creationDate;
/**
*
* The UNIX timestamp of when the authorizer was last updated.
*
*/
private java.util.Date lastModifiedDate;
/**
*
* The authorizer name.
*
*
* Constraints:
* Length: 1 - 128
* Pattern: [\w=,@-]+
*
* @return
* The authorizer name.
*
*/
public String getAuthorizerName() {
return authorizerName;
}
/**
*
* The authorizer name.
*
*
* Constraints:
* Length: 1 - 128
* Pattern: [\w=,@-]+
*
* @param authorizerName
* The authorizer name.
*
*/
public void setAuthorizerName(String authorizerName) {
this.authorizerName = authorizerName;
}
/**
*
* The authorizer name.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* Constraints:
* Length: 1 - 128
* Pattern: [\w=,@-]+
*
* @param authorizerName
* The authorizer name.
*
* @return A reference to this updated object so that method calls can be
* chained together.
*/
public AuthorizerDescription withAuthorizerName(String authorizerName) {
this.authorizerName = authorizerName;
return this;
}
/**
*
* The authorizer ARN.
*
*
* @return
* The authorizer ARN.
*
*/
public String getAuthorizerArn() {
return authorizerArn;
}
/**
*
* The authorizer ARN.
*
*
* @param authorizerArn
* The authorizer ARN.
*
*/
public void setAuthorizerArn(String authorizerArn) {
this.authorizerArn = authorizerArn;
}
/**
*
* The authorizer ARN.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* @param authorizerArn
* The authorizer ARN.
*
* @return A reference to this updated object so that method calls can be
* chained together.
*/
public AuthorizerDescription withAuthorizerArn(String authorizerArn) {
this.authorizerArn = authorizerArn;
return this;
}
/**
*
* The authorizer's Lambda function ARN.
*
*
* @return
* The authorizer's Lambda function ARN.
*
*/
public String getAuthorizerFunctionArn() {
return authorizerFunctionArn;
}
/**
*
* The authorizer's Lambda function ARN.
*
*
* @param authorizerFunctionArn
* The authorizer's Lambda function ARN.
*
*/
public void setAuthorizerFunctionArn(String authorizerFunctionArn) {
this.authorizerFunctionArn = authorizerFunctionArn;
}
/**
*
* The authorizer's Lambda function ARN.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* @param authorizerFunctionArn
* The authorizer's Lambda function ARN.
*
* @return A reference to this updated object so that method calls can be
* chained together.
*/
public AuthorizerDescription withAuthorizerFunctionArn(String authorizerFunctionArn) {
this.authorizerFunctionArn = authorizerFunctionArn;
return this;
}
/**
*
* The key used to extract the token from the HTTP headers.
*
*
* Constraints:
* Length: 1 - 128
* Pattern: [a-zA-Z0-9_-]+
*
* @return
* The key used to extract the token from the HTTP headers.
*
*/
public String getTokenKeyName() {
return tokenKeyName;
}
/**
*
* The key used to extract the token from the HTTP headers.
*
*
* Constraints:
* Length: 1 - 128
* Pattern: [a-zA-Z0-9_-]+
*
* @param tokenKeyName
* The key used to extract the token from the HTTP headers.
*
*/
public void setTokenKeyName(String tokenKeyName) {
this.tokenKeyName = tokenKeyName;
}
/**
*
* The key used to extract the token from the HTTP headers.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* Constraints:
* Length: 1 - 128
* Pattern: [a-zA-Z0-9_-]+
*
* @param tokenKeyName
* The key used to extract the token from the HTTP headers.
*
* @return A reference to this updated object so that method calls can be
* chained together.
*/
public AuthorizerDescription withTokenKeyName(String tokenKeyName) {
this.tokenKeyName = tokenKeyName;
return this;
}
/**
*
* The public keys used to validate the token signature returned by your
* custom authentication service.
*
*
* @return
* The public keys used to validate the token signature returned by
* your custom authentication service.
*
*/
public java.util.Map getTokenSigningPublicKeys() {
return tokenSigningPublicKeys;
}
/**
*
* The public keys used to validate the token signature returned by your
* custom authentication service.
*
*
* @param tokenSigningPublicKeys
* The public keys used to validate the token signature returned
* by your custom authentication service.
*
*/
public void setTokenSigningPublicKeys(java.util.Map tokenSigningPublicKeys) {
this.tokenSigningPublicKeys = tokenSigningPublicKeys;
}
/**
*
* The public keys used to validate the token signature returned by your
* custom authentication service.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* @param tokenSigningPublicKeys
* The public keys used to validate the token signature returned
* by your custom authentication service.
*
* @return A reference to this updated object so that method calls can be
* chained together.
*/
public AuthorizerDescription withTokenSigningPublicKeys(
java.util.Map tokenSigningPublicKeys) {
this.tokenSigningPublicKeys = tokenSigningPublicKeys;
return this;
}
/**
*
* The public keys used to validate the token signature returned by your
* custom authentication service.
*
*
* The method adds a new key-value pair into tokenSigningPublicKeys
* parameter, and returns a reference to this object so that method calls
* can be chained together.
*
* @param key The key of the entry to be added into tokenSigningPublicKeys.
* @param value The corresponding value of the entry to be added into
* tokenSigningPublicKeys.
* @return A reference to this updated object so that method calls can be
* chained together.
*/
public AuthorizerDescription addtokenSigningPublicKeysEntry(String key, String value) {
if (null == this.tokenSigningPublicKeys) {
this.tokenSigningPublicKeys = new java.util.HashMap();
}
if (this.tokenSigningPublicKeys.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString()
+ ") are provided.");
this.tokenSigningPublicKeys.put(key, value);
return this;
}
/**
* Removes all the entries added into tokenSigningPublicKeys.
*
* Returns a reference to this object so that method calls can be chained
* together.
*/
public AuthorizerDescription cleartokenSigningPublicKeysEntries() {
this.tokenSigningPublicKeys = null;
return this;
}
/**
*
* The status of the authorizer.
*
*
* Constraints:
* Allowed Values: ACTIVE, INACTIVE
*
* @return
* The status of the authorizer.
*
* @see AuthorizerStatus
*/
public String getStatus() {
return status;
}
/**
*
* The status of the authorizer.
*
*
* Constraints:
* Allowed Values: ACTIVE, INACTIVE
*
* @param status
* The status of the authorizer.
*
* @see AuthorizerStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The status of the authorizer.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* Constraints:
* Allowed Values: ACTIVE, INACTIVE
*
* @param status
* The status of the authorizer.
*
* @return A reference to this updated object so that method calls can be
* chained together.
* @see AuthorizerStatus
*/
public AuthorizerDescription withStatus(String status) {
this.status = status;
return this;
}
/**
*
* The status of the authorizer.
*
*
* Constraints:
* Allowed Values: ACTIVE, INACTIVE
*
* @param status
* The status of the authorizer.
*
* @see AuthorizerStatus
*/
public void setStatus(AuthorizerStatus status) {
this.status = status.toString();
}
/**
*
* The status of the authorizer.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* Constraints:
* Allowed Values: ACTIVE, INACTIVE
*
* @param status
* The status of the authorizer.
*
* @return A reference to this updated object so that method calls can be
* chained together.
* @see AuthorizerStatus
*/
public AuthorizerDescription withStatus(AuthorizerStatus status) {
this.status = status.toString();
return this;
}
/**
*
* The UNIX timestamp of when the authorizer was created.
*
*
* @return
* The UNIX timestamp of when the authorizer was created.
*
*/
public java.util.Date getCreationDate() {
return creationDate;
}
/**
*
* The UNIX timestamp of when the authorizer was created.
*
*
* @param creationDate
* The UNIX timestamp of when the authorizer was created.
*
*/
public void setCreationDate(java.util.Date creationDate) {
this.creationDate = creationDate;
}
/**
*
* The UNIX timestamp of when the authorizer was created.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* @param creationDate
* The UNIX timestamp of when the authorizer was created.
*
* @return A reference to this updated object so that method calls can be
* chained together.
*/
public AuthorizerDescription withCreationDate(java.util.Date creationDate) {
this.creationDate = creationDate;
return this;
}
/**
*
* The UNIX timestamp of when the authorizer was last updated.
*
*
* @return
* The UNIX timestamp of when the authorizer was last updated.
*
*/
public java.util.Date getLastModifiedDate() {
return lastModifiedDate;
}
/**
*
* The UNIX timestamp of when the authorizer was last updated.
*
*
* @param lastModifiedDate
* The UNIX timestamp of when the authorizer was last updated.
*
*/
public void setLastModifiedDate(java.util.Date lastModifiedDate) {
this.lastModifiedDate = lastModifiedDate;
}
/**
*
* The UNIX timestamp of when the authorizer was last updated.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* @param lastModifiedDate
* The UNIX timestamp of when the authorizer was last updated.
*
* @return A reference to this updated object so that method calls can be
* chained together.
*/
public AuthorizerDescription withLastModifiedDate(java.util.Date lastModifiedDate) {
this.lastModifiedDate = lastModifiedDate;
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAuthorizerName() != null)
sb.append("authorizerName: " + getAuthorizerName() + ",");
if (getAuthorizerArn() != null)
sb.append("authorizerArn: " + getAuthorizerArn() + ",");
if (getAuthorizerFunctionArn() != null)
sb.append("authorizerFunctionArn: " + getAuthorizerFunctionArn() + ",");
if (getTokenKeyName() != null)
sb.append("tokenKeyName: " + getTokenKeyName() + ",");
if (getTokenSigningPublicKeys() != null)
sb.append("tokenSigningPublicKeys: " + getTokenSigningPublicKeys() + ",");
if (getStatus() != null)
sb.append("status: " + getStatus() + ",");
if (getCreationDate() != null)
sb.append("creationDate: " + getCreationDate() + ",");
if (getLastModifiedDate() != null)
sb.append("lastModifiedDate: " + getLastModifiedDate());
sb.append("}");
return sb.toString();
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode
+ ((getAuthorizerName() == null) ? 0 : getAuthorizerName().hashCode());
hashCode = prime * hashCode
+ ((getAuthorizerArn() == null) ? 0 : getAuthorizerArn().hashCode());
hashCode = prime
* hashCode
+ ((getAuthorizerFunctionArn() == null) ? 0 : getAuthorizerFunctionArn().hashCode());
hashCode = prime * hashCode
+ ((getTokenKeyName() == null) ? 0 : getTokenKeyName().hashCode());
hashCode = prime
* hashCode
+ ((getTokenSigningPublicKeys() == null) ? 0 : getTokenSigningPublicKeys()
.hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode
+ ((getCreationDate() == null) ? 0 : getCreationDate().hashCode());
hashCode = prime * hashCode
+ ((getLastModifiedDate() == null) ? 0 : getLastModifiedDate().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof AuthorizerDescription == false)
return false;
AuthorizerDescription other = (AuthorizerDescription) obj;
if (other.getAuthorizerName() == null ^ this.getAuthorizerName() == null)
return false;
if (other.getAuthorizerName() != null
&& other.getAuthorizerName().equals(this.getAuthorizerName()) == false)
return false;
if (other.getAuthorizerArn() == null ^ this.getAuthorizerArn() == null)
return false;
if (other.getAuthorizerArn() != null
&& other.getAuthorizerArn().equals(this.getAuthorizerArn()) == false)
return false;
if (other.getAuthorizerFunctionArn() == null ^ this.getAuthorizerFunctionArn() == null)
return false;
if (other.getAuthorizerFunctionArn() != null
&& other.getAuthorizerFunctionArn().equals(this.getAuthorizerFunctionArn()) == false)
return false;
if (other.getTokenKeyName() == null ^ this.getTokenKeyName() == null)
return false;
if (other.getTokenKeyName() != null
&& other.getTokenKeyName().equals(this.getTokenKeyName()) == false)
return false;
if (other.getTokenSigningPublicKeys() == null ^ this.getTokenSigningPublicKeys() == null)
return false;
if (other.getTokenSigningPublicKeys() != null
&& other.getTokenSigningPublicKeys().equals(this.getTokenSigningPublicKeys()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getCreationDate() == null ^ this.getCreationDate() == null)
return false;
if (other.getCreationDate() != null
&& other.getCreationDate().equals(this.getCreationDate()) == false)
return false;
if (other.getLastModifiedDate() == null ^ this.getLastModifiedDate() == null)
return false;
if (other.getLastModifiedDate() != null
&& other.getLastModifiedDate().equals(this.getLastModifiedDate()) == false)
return false;
return true;
}
}