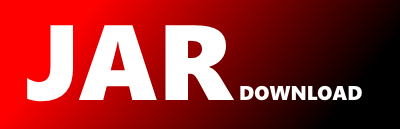
com.amazonaws.services.iot.model.CertificateDescription Maven / Gradle / Ivy
Show all versions of aws-android-sdk-iot Show documentation
/*
* Copyright 2010-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.iot.model;
import java.io.Serializable;
/**
*
* Describes a certificate.
*
*/
public class CertificateDescription implements Serializable {
/**
*
* The ARN of the certificate.
*
*/
private String certificateArn;
/**
*
* The ID of the certificate.
*
*
* Constraints:
* Length: 64 - 64
* Pattern: (0x)?[a-fA-F0-9]+
*/
private String certificateId;
/**
*
* The certificate ID of the CA certificate used to sign this certificate.
*
*
* Constraints:
* Length: 64 - 64
* Pattern: (0x)?[a-fA-F0-9]+
*/
private String caCertificateId;
/**
*
* The status of the certificate.
*
*
* Constraints:
* Allowed Values: ACTIVE, INACTIVE, REVOKED, PENDING_TRANSFER,
* REGISTER_INACTIVE, PENDING_ACTIVATION
*/
private String status;
/**
*
* The certificate data, in PEM format.
*
*
* Constraints:
* Length: 1 - 65536
*/
private String certificatePem;
/**
*
* The ID of the AWS account that owns the certificate.
*
*
* Constraints:
* Pattern: [0-9]{12}
*/
private String ownedBy;
/**
*
* The ID of the AWS account of the previous owner of the certificate.
*
*
* Constraints:
* Pattern: [0-9]{12}
*/
private String previousOwnedBy;
/**
*
* The date and time the certificate was created.
*
*/
private java.util.Date creationDate;
/**
*
* The date and time the certificate was last modified.
*
*/
private java.util.Date lastModifiedDate;
/**
*
* The customer version of the certificate.
*
*
* Constraints:
* Range: 1 -
*/
private Integer customerVersion;
/**
*
* The transfer data.
*
*/
private TransferData transferData;
/**
*
* The generation ID of the certificate.
*
*/
private String generationId;
/**
*
* The ARN of the certificate.
*
*
* @return
* The ARN of the certificate.
*
*/
public String getCertificateArn() {
return certificateArn;
}
/**
*
* The ARN of the certificate.
*
*
* @param certificateArn
* The ARN of the certificate.
*
*/
public void setCertificateArn(String certificateArn) {
this.certificateArn = certificateArn;
}
/**
*
* The ARN of the certificate.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* @param certificateArn
* The ARN of the certificate.
*
* @return A reference to this updated object so that method calls can be
* chained together.
*/
public CertificateDescription withCertificateArn(String certificateArn) {
this.certificateArn = certificateArn;
return this;
}
/**
*
* The ID of the certificate.
*
*
* Constraints:
* Length: 64 - 64
* Pattern: (0x)?[a-fA-F0-9]+
*
* @return
* The ID of the certificate.
*
*/
public String getCertificateId() {
return certificateId;
}
/**
*
* The ID of the certificate.
*
*
* Constraints:
* Length: 64 - 64
* Pattern: (0x)?[a-fA-F0-9]+
*
* @param certificateId
* The ID of the certificate.
*
*/
public void setCertificateId(String certificateId) {
this.certificateId = certificateId;
}
/**
*
* The ID of the certificate.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* Constraints:
* Length: 64 - 64
* Pattern: (0x)?[a-fA-F0-9]+
*
* @param certificateId
* The ID of the certificate.
*
* @return A reference to this updated object so that method calls can be
* chained together.
*/
public CertificateDescription withCertificateId(String certificateId) {
this.certificateId = certificateId;
return this;
}
/**
*
* The certificate ID of the CA certificate used to sign this certificate.
*
*
* Constraints:
* Length: 64 - 64
* Pattern: (0x)?[a-fA-F0-9]+
*
* @return
* The certificate ID of the CA certificate used to sign this
* certificate.
*
*/
public String getCaCertificateId() {
return caCertificateId;
}
/**
*
* The certificate ID of the CA certificate used to sign this certificate.
*
*
* Constraints:
* Length: 64 - 64
* Pattern: (0x)?[a-fA-F0-9]+
*
* @param caCertificateId
* The certificate ID of the CA certificate used to sign this
* certificate.
*
*/
public void setCaCertificateId(String caCertificateId) {
this.caCertificateId = caCertificateId;
}
/**
*
* The certificate ID of the CA certificate used to sign this certificate.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* Constraints:
* Length: 64 - 64
* Pattern: (0x)?[a-fA-F0-9]+
*
* @param caCertificateId
* The certificate ID of the CA certificate used to sign this
* certificate.
*
* @return A reference to this updated object so that method calls can be
* chained together.
*/
public CertificateDescription withCaCertificateId(String caCertificateId) {
this.caCertificateId = caCertificateId;
return this;
}
/**
*
* The status of the certificate.
*
*
* Constraints:
* Allowed Values: ACTIVE, INACTIVE, REVOKED, PENDING_TRANSFER,
* REGISTER_INACTIVE, PENDING_ACTIVATION
*
* @return
* The status of the certificate.
*
* @see CertificateStatus
*/
public String getStatus() {
return status;
}
/**
*
* The status of the certificate.
*
*
* Constraints:
* Allowed Values: ACTIVE, INACTIVE, REVOKED, PENDING_TRANSFER,
* REGISTER_INACTIVE, PENDING_ACTIVATION
*
* @param status
* The status of the certificate.
*
* @see CertificateStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The status of the certificate.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* Constraints:
* Allowed Values: ACTIVE, INACTIVE, REVOKED, PENDING_TRANSFER,
* REGISTER_INACTIVE, PENDING_ACTIVATION
*
* @param status
* The status of the certificate.
*
* @return A reference to this updated object so that method calls can be
* chained together.
* @see CertificateStatus
*/
public CertificateDescription withStatus(String status) {
this.status = status;
return this;
}
/**
*
* The status of the certificate.
*
*
* Constraints:
* Allowed Values: ACTIVE, INACTIVE, REVOKED, PENDING_TRANSFER,
* REGISTER_INACTIVE, PENDING_ACTIVATION
*
* @param status
* The status of the certificate.
*
* @see CertificateStatus
*/
public void setStatus(CertificateStatus status) {
this.status = status.toString();
}
/**
*
* The status of the certificate.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* Constraints:
* Allowed Values: ACTIVE, INACTIVE, REVOKED, PENDING_TRANSFER,
* REGISTER_INACTIVE, PENDING_ACTIVATION
*
* @param status
* The status of the certificate.
*
* @return A reference to this updated object so that method calls can be
* chained together.
* @see CertificateStatus
*/
public CertificateDescription withStatus(CertificateStatus status) {
this.status = status.toString();
return this;
}
/**
*
* The certificate data, in PEM format.
*
*
* Constraints:
* Length: 1 - 65536
*
* @return
* The certificate data, in PEM format.
*
*/
public String getCertificatePem() {
return certificatePem;
}
/**
*
* The certificate data, in PEM format.
*
*
* Constraints:
* Length: 1 - 65536
*
* @param certificatePem
* The certificate data, in PEM format.
*
*/
public void setCertificatePem(String certificatePem) {
this.certificatePem = certificatePem;
}
/**
*
* The certificate data, in PEM format.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* Constraints:
* Length: 1 - 65536
*
* @param certificatePem
* The certificate data, in PEM format.
*
* @return A reference to this updated object so that method calls can be
* chained together.
*/
public CertificateDescription withCertificatePem(String certificatePem) {
this.certificatePem = certificatePem;
return this;
}
/**
*
* The ID of the AWS account that owns the certificate.
*
*
* Constraints:
* Pattern: [0-9]{12}
*
* @return
* The ID of the AWS account that owns the certificate.
*
*/
public String getOwnedBy() {
return ownedBy;
}
/**
*
* The ID of the AWS account that owns the certificate.
*
*
* Constraints:
* Pattern: [0-9]{12}
*
* @param ownedBy
* The ID of the AWS account that owns the certificate.
*
*/
public void setOwnedBy(String ownedBy) {
this.ownedBy = ownedBy;
}
/**
*
* The ID of the AWS account that owns the certificate.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* Constraints:
* Pattern: [0-9]{12}
*
* @param ownedBy
* The ID of the AWS account that owns the certificate.
*
* @return A reference to this updated object so that method calls can be
* chained together.
*/
public CertificateDescription withOwnedBy(String ownedBy) {
this.ownedBy = ownedBy;
return this;
}
/**
*
* The ID of the AWS account of the previous owner of the certificate.
*
*
* Constraints:
* Pattern: [0-9]{12}
*
* @return
* The ID of the AWS account of the previous owner of the
* certificate.
*
*/
public String getPreviousOwnedBy() {
return previousOwnedBy;
}
/**
*
* The ID of the AWS account of the previous owner of the certificate.
*
*
* Constraints:
* Pattern: [0-9]{12}
*
* @param previousOwnedBy
* The ID of the AWS account of the previous owner of the
* certificate.
*
*/
public void setPreviousOwnedBy(String previousOwnedBy) {
this.previousOwnedBy = previousOwnedBy;
}
/**
*
* The ID of the AWS account of the previous owner of the certificate.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* Constraints:
* Pattern: [0-9]{12}
*
* @param previousOwnedBy
* The ID of the AWS account of the previous owner of the
* certificate.
*
* @return A reference to this updated object so that method calls can be
* chained together.
*/
public CertificateDescription withPreviousOwnedBy(String previousOwnedBy) {
this.previousOwnedBy = previousOwnedBy;
return this;
}
/**
*
* The date and time the certificate was created.
*
*
* @return
* The date and time the certificate was created.
*
*/
public java.util.Date getCreationDate() {
return creationDate;
}
/**
*
* The date and time the certificate was created.
*
*
* @param creationDate
* The date and time the certificate was created.
*
*/
public void setCreationDate(java.util.Date creationDate) {
this.creationDate = creationDate;
}
/**
*
* The date and time the certificate was created.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* @param creationDate
* The date and time the certificate was created.
*
* @return A reference to this updated object so that method calls can be
* chained together.
*/
public CertificateDescription withCreationDate(java.util.Date creationDate) {
this.creationDate = creationDate;
return this;
}
/**
*
* The date and time the certificate was last modified.
*
*
* @return
* The date and time the certificate was last modified.
*
*/
public java.util.Date getLastModifiedDate() {
return lastModifiedDate;
}
/**
*
* The date and time the certificate was last modified.
*
*
* @param lastModifiedDate
* The date and time the certificate was last modified.
*
*/
public void setLastModifiedDate(java.util.Date lastModifiedDate) {
this.lastModifiedDate = lastModifiedDate;
}
/**
*
* The date and time the certificate was last modified.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* @param lastModifiedDate
* The date and time the certificate was last modified.
*
* @return A reference to this updated object so that method calls can be
* chained together.
*/
public CertificateDescription withLastModifiedDate(java.util.Date lastModifiedDate) {
this.lastModifiedDate = lastModifiedDate;
return this;
}
/**
*
* The customer version of the certificate.
*
*
* Constraints:
* Range: 1 -
*
* @return
* The customer version of the certificate.
*
*/
public Integer getCustomerVersion() {
return customerVersion;
}
/**
*
* The customer version of the certificate.
*
*
* Constraints:
* Range: 1 -
*
* @param customerVersion
* The customer version of the certificate.
*
*/
public void setCustomerVersion(Integer customerVersion) {
this.customerVersion = customerVersion;
}
/**
*
* The customer version of the certificate.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* Constraints:
* Range: 1 -
*
* @param customerVersion
* The customer version of the certificate.
*
* @return A reference to this updated object so that method calls can be
* chained together.
*/
public CertificateDescription withCustomerVersion(Integer customerVersion) {
this.customerVersion = customerVersion;
return this;
}
/**
*
* The transfer data.
*
*
* @return
* The transfer data.
*
*/
public TransferData getTransferData() {
return transferData;
}
/**
*
* The transfer data.
*
*
* @param transferData
* The transfer data.
*
*/
public void setTransferData(TransferData transferData) {
this.transferData = transferData;
}
/**
*
* The transfer data.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* @param transferData
* The transfer data.
*
* @return A reference to this updated object so that method calls can be
* chained together.
*/
public CertificateDescription withTransferData(TransferData transferData) {
this.transferData = transferData;
return this;
}
/**
*
* The generation ID of the certificate.
*
*
* @return
* The generation ID of the certificate.
*
*/
public String getGenerationId() {
return generationId;
}
/**
*
* The generation ID of the certificate.
*
*
* @param generationId
* The generation ID of the certificate.
*
*/
public void setGenerationId(String generationId) {
this.generationId = generationId;
}
/**
*
* The generation ID of the certificate.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* @param generationId
* The generation ID of the certificate.
*
* @return A reference to this updated object so that method calls can be
* chained together.
*/
public CertificateDescription withGenerationId(String generationId) {
this.generationId = generationId;
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getCertificateArn() != null)
sb.append("certificateArn: " + getCertificateArn() + ",");
if (getCertificateId() != null)
sb.append("certificateId: " + getCertificateId() + ",");
if (getCaCertificateId() != null)
sb.append("caCertificateId: " + getCaCertificateId() + ",");
if (getStatus() != null)
sb.append("status: " + getStatus() + ",");
if (getCertificatePem() != null)
sb.append("certificatePem: " + getCertificatePem() + ",");
if (getOwnedBy() != null)
sb.append("ownedBy: " + getOwnedBy() + ",");
if (getPreviousOwnedBy() != null)
sb.append("previousOwnedBy: " + getPreviousOwnedBy() + ",");
if (getCreationDate() != null)
sb.append("creationDate: " + getCreationDate() + ",");
if (getLastModifiedDate() != null)
sb.append("lastModifiedDate: " + getLastModifiedDate() + ",");
if (getCustomerVersion() != null)
sb.append("customerVersion: " + getCustomerVersion() + ",");
if (getTransferData() != null)
sb.append("transferData: " + getTransferData() + ",");
if (getGenerationId() != null)
sb.append("generationId: " + getGenerationId());
sb.append("}");
return sb.toString();
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode
+ ((getCertificateArn() == null) ? 0 : getCertificateArn().hashCode());
hashCode = prime * hashCode
+ ((getCertificateId() == null) ? 0 : getCertificateId().hashCode());
hashCode = prime * hashCode
+ ((getCaCertificateId() == null) ? 0 : getCaCertificateId().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode
+ ((getCertificatePem() == null) ? 0 : getCertificatePem().hashCode());
hashCode = prime * hashCode + ((getOwnedBy() == null) ? 0 : getOwnedBy().hashCode());
hashCode = prime * hashCode
+ ((getPreviousOwnedBy() == null) ? 0 : getPreviousOwnedBy().hashCode());
hashCode = prime * hashCode
+ ((getCreationDate() == null) ? 0 : getCreationDate().hashCode());
hashCode = prime * hashCode
+ ((getLastModifiedDate() == null) ? 0 : getLastModifiedDate().hashCode());
hashCode = prime * hashCode
+ ((getCustomerVersion() == null) ? 0 : getCustomerVersion().hashCode());
hashCode = prime * hashCode
+ ((getTransferData() == null) ? 0 : getTransferData().hashCode());
hashCode = prime * hashCode
+ ((getGenerationId() == null) ? 0 : getGenerationId().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CertificateDescription == false)
return false;
CertificateDescription other = (CertificateDescription) obj;
if (other.getCertificateArn() == null ^ this.getCertificateArn() == null)
return false;
if (other.getCertificateArn() != null
&& other.getCertificateArn().equals(this.getCertificateArn()) == false)
return false;
if (other.getCertificateId() == null ^ this.getCertificateId() == null)
return false;
if (other.getCertificateId() != null
&& other.getCertificateId().equals(this.getCertificateId()) == false)
return false;
if (other.getCaCertificateId() == null ^ this.getCaCertificateId() == null)
return false;
if (other.getCaCertificateId() != null
&& other.getCaCertificateId().equals(this.getCaCertificateId()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getCertificatePem() == null ^ this.getCertificatePem() == null)
return false;
if (other.getCertificatePem() != null
&& other.getCertificatePem().equals(this.getCertificatePem()) == false)
return false;
if (other.getOwnedBy() == null ^ this.getOwnedBy() == null)
return false;
if (other.getOwnedBy() != null && other.getOwnedBy().equals(this.getOwnedBy()) == false)
return false;
if (other.getPreviousOwnedBy() == null ^ this.getPreviousOwnedBy() == null)
return false;
if (other.getPreviousOwnedBy() != null
&& other.getPreviousOwnedBy().equals(this.getPreviousOwnedBy()) == false)
return false;
if (other.getCreationDate() == null ^ this.getCreationDate() == null)
return false;
if (other.getCreationDate() != null
&& other.getCreationDate().equals(this.getCreationDate()) == false)
return false;
if (other.getLastModifiedDate() == null ^ this.getLastModifiedDate() == null)
return false;
if (other.getLastModifiedDate() != null
&& other.getLastModifiedDate().equals(this.getLastModifiedDate()) == false)
return false;
if (other.getCustomerVersion() == null ^ this.getCustomerVersion() == null)
return false;
if (other.getCustomerVersion() != null
&& other.getCustomerVersion().equals(this.getCustomerVersion()) == false)
return false;
if (other.getTransferData() == null ^ this.getTransferData() == null)
return false;
if (other.getTransferData() != null
&& other.getTransferData().equals(this.getTransferData()) == false)
return false;
if (other.getGenerationId() == null ^ this.getGenerationId() == null)
return false;
if (other.getGenerationId() != null
&& other.getGenerationId().equals(this.getGenerationId()) == false)
return false;
return true;
}
}