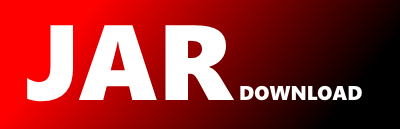
com.amazonaws.services.iot.model.JobSummary Maven / Gradle / Ivy
/*
* Copyright 2010-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.iot.model;
import java.io.Serializable;
/**
*
* The job summary.
*
*/
public class JobSummary implements Serializable {
/**
*
* The job ARN.
*
*/
private String jobArn;
/**
*
* The unique identifier you assigned to this job when it was created.
*
*
* Constraints:
* Length: 1 - 64
* Pattern: [a-zA-Z0-9_-]+
*/
private String jobId;
/**
*
* The ID of the thing group.
*
*
* Constraints:
* Length: 1 - 128
* Pattern: [a-zA-Z0-9\-]+
*/
private String thingGroupId;
/**
*
* Specifies whether the job will continue to run (CONTINUOUS), or will be
* complete after all those things specified as targets have completed the
* job (SNAPSHOT). If continuous, the job may also be run on a thing when a
* change is detected in a target. For example, a job will run on a thing
* when the thing is added to a target group, even after the job was
* completed by all things originally in the group.
*
*
* Constraints:
* Allowed Values: CONTINUOUS, SNAPSHOT
*/
private String targetSelection;
/**
*
* The job summary status.
*
*
* Constraints:
* Allowed Values: IN_PROGRESS, CANCELED, COMPLETED,
* DELETION_IN_PROGRESS
*/
private String status;
/**
*
* The time, in milliseconds since the epoch, when the job was created.
*
*/
private java.util.Date createdAt;
/**
*
* The time, in milliseconds since the epoch, when the job was last updated.
*
*/
private java.util.Date lastUpdatedAt;
/**
*
* The time, in milliseconds since the epoch, when the job completed.
*
*/
private java.util.Date completedAt;
/**
*
* The job ARN.
*
*
* @return
* The job ARN.
*
*/
public String getJobArn() {
return jobArn;
}
/**
*
* The job ARN.
*
*
* @param jobArn
* The job ARN.
*
*/
public void setJobArn(String jobArn) {
this.jobArn = jobArn;
}
/**
*
* The job ARN.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* @param jobArn
* The job ARN.
*
* @return A reference to this updated object so that method calls can be
* chained together.
*/
public JobSummary withJobArn(String jobArn) {
this.jobArn = jobArn;
return this;
}
/**
*
* The unique identifier you assigned to this job when it was created.
*
*
* Constraints:
* Length: 1 - 64
* Pattern: [a-zA-Z0-9_-]+
*
* @return
* The unique identifier you assigned to this job when it was
* created.
*
*/
public String getJobId() {
return jobId;
}
/**
*
* The unique identifier you assigned to this job when it was created.
*
*
* Constraints:
* Length: 1 - 64
* Pattern: [a-zA-Z0-9_-]+
*
* @param jobId
* The unique identifier you assigned to this job when it was
* created.
*
*/
public void setJobId(String jobId) {
this.jobId = jobId;
}
/**
*
* The unique identifier you assigned to this job when it was created.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* Constraints:
* Length: 1 - 64
* Pattern: [a-zA-Z0-9_-]+
*
* @param jobId
* The unique identifier you assigned to this job when it was
* created.
*
* @return A reference to this updated object so that method calls can be
* chained together.
*/
public JobSummary withJobId(String jobId) {
this.jobId = jobId;
return this;
}
/**
*
* The ID of the thing group.
*
*
* Constraints:
* Length: 1 - 128
* Pattern: [a-zA-Z0-9\-]+
*
* @return
* The ID of the thing group.
*
*/
public String getThingGroupId() {
return thingGroupId;
}
/**
*
* The ID of the thing group.
*
*
* Constraints:
* Length: 1 - 128
* Pattern: [a-zA-Z0-9\-]+
*
* @param thingGroupId
* The ID of the thing group.
*
*/
public void setThingGroupId(String thingGroupId) {
this.thingGroupId = thingGroupId;
}
/**
*
* The ID of the thing group.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* Constraints:
* Length: 1 - 128
* Pattern: [a-zA-Z0-9\-]+
*
* @param thingGroupId
* The ID of the thing group.
*
* @return A reference to this updated object so that method calls can be
* chained together.
*/
public JobSummary withThingGroupId(String thingGroupId) {
this.thingGroupId = thingGroupId;
return this;
}
/**
*
* Specifies whether the job will continue to run (CONTINUOUS), or will be
* complete after all those things specified as targets have completed the
* job (SNAPSHOT). If continuous, the job may also be run on a thing when a
* change is detected in a target. For example, a job will run on a thing
* when the thing is added to a target group, even after the job was
* completed by all things originally in the group.
*
*
* Constraints:
* Allowed Values: CONTINUOUS, SNAPSHOT
*
* @return
* Specifies whether the job will continue to run (CONTINUOUS), or
* will be complete after all those things specified as targets have
* completed the job (SNAPSHOT). If continuous, the job may also be
* run on a thing when a change is detected in a target. For
* example, a job will run on a thing when the thing is added to a
* target group, even after the job was completed by all things
* originally in the group.
*
* @see TargetSelection
*/
public String getTargetSelection() {
return targetSelection;
}
/**
*
* Specifies whether the job will continue to run (CONTINUOUS), or will be
* complete after all those things specified as targets have completed the
* job (SNAPSHOT). If continuous, the job may also be run on a thing when a
* change is detected in a target. For example, a job will run on a thing
* when the thing is added to a target group, even after the job was
* completed by all things originally in the group.
*
*
* Constraints:
* Allowed Values: CONTINUOUS, SNAPSHOT
*
* @param targetSelection
* Specifies whether the job will continue to run (CONTINUOUS),
* or will be complete after all those things specified as
* targets have completed the job (SNAPSHOT). If continuous, the
* job may also be run on a thing when a change is detected in a
* target. For example, a job will run on a thing when the thing
* is added to a target group, even after the job was completed
* by all things originally in the group.
*
* @see TargetSelection
*/
public void setTargetSelection(String targetSelection) {
this.targetSelection = targetSelection;
}
/**
*
* Specifies whether the job will continue to run (CONTINUOUS), or will be
* complete after all those things specified as targets have completed the
* job (SNAPSHOT). If continuous, the job may also be run on a thing when a
* change is detected in a target. For example, a job will run on a thing
* when the thing is added to a target group, even after the job was
* completed by all things originally in the group.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* Constraints:
* Allowed Values: CONTINUOUS, SNAPSHOT
*
* @param targetSelection
* Specifies whether the job will continue to run (CONTINUOUS),
* or will be complete after all those things specified as
* targets have completed the job (SNAPSHOT). If continuous, the
* job may also be run on a thing when a change is detected in a
* target. For example, a job will run on a thing when the thing
* is added to a target group, even after the job was completed
* by all things originally in the group.
*
* @return A reference to this updated object so that method calls can be
* chained together.
* @see TargetSelection
*/
public JobSummary withTargetSelection(String targetSelection) {
this.targetSelection = targetSelection;
return this;
}
/**
*
* Specifies whether the job will continue to run (CONTINUOUS), or will be
* complete after all those things specified as targets have completed the
* job (SNAPSHOT). If continuous, the job may also be run on a thing when a
* change is detected in a target. For example, a job will run on a thing
* when the thing is added to a target group, even after the job was
* completed by all things originally in the group.
*
*
* Constraints:
* Allowed Values: CONTINUOUS, SNAPSHOT
*
* @param targetSelection
* Specifies whether the job will continue to run (CONTINUOUS),
* or will be complete after all those things specified as
* targets have completed the job (SNAPSHOT). If continuous, the
* job may also be run on a thing when a change is detected in a
* target. For example, a job will run on a thing when the thing
* is added to a target group, even after the job was completed
* by all things originally in the group.
*
* @see TargetSelection
*/
public void setTargetSelection(TargetSelection targetSelection) {
this.targetSelection = targetSelection.toString();
}
/**
*
* Specifies whether the job will continue to run (CONTINUOUS), or will be
* complete after all those things specified as targets have completed the
* job (SNAPSHOT). If continuous, the job may also be run on a thing when a
* change is detected in a target. For example, a job will run on a thing
* when the thing is added to a target group, even after the job was
* completed by all things originally in the group.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* Constraints:
* Allowed Values: CONTINUOUS, SNAPSHOT
*
* @param targetSelection
* Specifies whether the job will continue to run (CONTINUOUS),
* or will be complete after all those things specified as
* targets have completed the job (SNAPSHOT). If continuous, the
* job may also be run on a thing when a change is detected in a
* target. For example, a job will run on a thing when the thing
* is added to a target group, even after the job was completed
* by all things originally in the group.
*
* @return A reference to this updated object so that method calls can be
* chained together.
* @see TargetSelection
*/
public JobSummary withTargetSelection(TargetSelection targetSelection) {
this.targetSelection = targetSelection.toString();
return this;
}
/**
*
* The job summary status.
*
*
* Constraints:
* Allowed Values: IN_PROGRESS, CANCELED, COMPLETED,
* DELETION_IN_PROGRESS
*
* @return
* The job summary status.
*
* @see JobStatus
*/
public String getStatus() {
return status;
}
/**
*
* The job summary status.
*
*
* Constraints:
* Allowed Values: IN_PROGRESS, CANCELED, COMPLETED,
* DELETION_IN_PROGRESS
*
* @param status
* The job summary status.
*
* @see JobStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The job summary status.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* Constraints:
* Allowed Values: IN_PROGRESS, CANCELED, COMPLETED,
* DELETION_IN_PROGRESS
*
* @param status
* The job summary status.
*
* @return A reference to this updated object so that method calls can be
* chained together.
* @see JobStatus
*/
public JobSummary withStatus(String status) {
this.status = status;
return this;
}
/**
*
* The job summary status.
*
*
* Constraints:
* Allowed Values: IN_PROGRESS, CANCELED, COMPLETED,
* DELETION_IN_PROGRESS
*
* @param status
* The job summary status.
*
* @see JobStatus
*/
public void setStatus(JobStatus status) {
this.status = status.toString();
}
/**
*
* The job summary status.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* Constraints:
* Allowed Values: IN_PROGRESS, CANCELED, COMPLETED,
* DELETION_IN_PROGRESS
*
* @param status
* The job summary status.
*
* @return A reference to this updated object so that method calls can be
* chained together.
* @see JobStatus
*/
public JobSummary withStatus(JobStatus status) {
this.status = status.toString();
return this;
}
/**
*
* The time, in milliseconds since the epoch, when the job was created.
*
*
* @return
* The time, in milliseconds since the epoch, when the job was
* created.
*
*/
public java.util.Date getCreatedAt() {
return createdAt;
}
/**
*
* The time, in milliseconds since the epoch, when the job was created.
*
*
* @param createdAt
* The time, in milliseconds since the epoch, when the job was
* created.
*
*/
public void setCreatedAt(java.util.Date createdAt) {
this.createdAt = createdAt;
}
/**
*
* The time, in milliseconds since the epoch, when the job was created.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* @param createdAt
* The time, in milliseconds since the epoch, when the job was
* created.
*
* @return A reference to this updated object so that method calls can be
* chained together.
*/
public JobSummary withCreatedAt(java.util.Date createdAt) {
this.createdAt = createdAt;
return this;
}
/**
*
* The time, in milliseconds since the epoch, when the job was last updated.
*
*
* @return
* The time, in milliseconds since the epoch, when the job was last
* updated.
*
*/
public java.util.Date getLastUpdatedAt() {
return lastUpdatedAt;
}
/**
*
* The time, in milliseconds since the epoch, when the job was last updated.
*
*
* @param lastUpdatedAt
* The time, in milliseconds since the epoch, when the job was
* last updated.
*
*/
public void setLastUpdatedAt(java.util.Date lastUpdatedAt) {
this.lastUpdatedAt = lastUpdatedAt;
}
/**
*
* The time, in milliseconds since the epoch, when the job was last updated.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* @param lastUpdatedAt
* The time, in milliseconds since the epoch, when the job was
* last updated.
*
* @return A reference to this updated object so that method calls can be
* chained together.
*/
public JobSummary withLastUpdatedAt(java.util.Date lastUpdatedAt) {
this.lastUpdatedAt = lastUpdatedAt;
return this;
}
/**
*
* The time, in milliseconds since the epoch, when the job completed.
*
*
* @return
* The time, in milliseconds since the epoch, when the job
* completed.
*
*/
public java.util.Date getCompletedAt() {
return completedAt;
}
/**
*
* The time, in milliseconds since the epoch, when the job completed.
*
*
* @param completedAt
* The time, in milliseconds since the epoch, when the job
* completed.
*
*/
public void setCompletedAt(java.util.Date completedAt) {
this.completedAt = completedAt;
}
/**
*
* The time, in milliseconds since the epoch, when the job completed.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* @param completedAt
* The time, in milliseconds since the epoch, when the job
* completed.
*
* @return A reference to this updated object so that method calls can be
* chained together.
*/
public JobSummary withCompletedAt(java.util.Date completedAt) {
this.completedAt = completedAt;
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getJobArn() != null)
sb.append("jobArn: " + getJobArn() + ",");
if (getJobId() != null)
sb.append("jobId: " + getJobId() + ",");
if (getThingGroupId() != null)
sb.append("thingGroupId: " + getThingGroupId() + ",");
if (getTargetSelection() != null)
sb.append("targetSelection: " + getTargetSelection() + ",");
if (getStatus() != null)
sb.append("status: " + getStatus() + ",");
if (getCreatedAt() != null)
sb.append("createdAt: " + getCreatedAt() + ",");
if (getLastUpdatedAt() != null)
sb.append("lastUpdatedAt: " + getLastUpdatedAt() + ",");
if (getCompletedAt() != null)
sb.append("completedAt: " + getCompletedAt());
sb.append("}");
return sb.toString();
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getJobArn() == null) ? 0 : getJobArn().hashCode());
hashCode = prime * hashCode + ((getJobId() == null) ? 0 : getJobId().hashCode());
hashCode = prime * hashCode
+ ((getThingGroupId() == null) ? 0 : getThingGroupId().hashCode());
hashCode = prime * hashCode
+ ((getTargetSelection() == null) ? 0 : getTargetSelection().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getCreatedAt() == null) ? 0 : getCreatedAt().hashCode());
hashCode = prime * hashCode
+ ((getLastUpdatedAt() == null) ? 0 : getLastUpdatedAt().hashCode());
hashCode = prime * hashCode
+ ((getCompletedAt() == null) ? 0 : getCompletedAt().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof JobSummary == false)
return false;
JobSummary other = (JobSummary) obj;
if (other.getJobArn() == null ^ this.getJobArn() == null)
return false;
if (other.getJobArn() != null && other.getJobArn().equals(this.getJobArn()) == false)
return false;
if (other.getJobId() == null ^ this.getJobId() == null)
return false;
if (other.getJobId() != null && other.getJobId().equals(this.getJobId()) == false)
return false;
if (other.getThingGroupId() == null ^ this.getThingGroupId() == null)
return false;
if (other.getThingGroupId() != null
&& other.getThingGroupId().equals(this.getThingGroupId()) == false)
return false;
if (other.getTargetSelection() == null ^ this.getTargetSelection() == null)
return false;
if (other.getTargetSelection() != null
&& other.getTargetSelection().equals(this.getTargetSelection()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getCreatedAt() == null ^ this.getCreatedAt() == null)
return false;
if (other.getCreatedAt() != null
&& other.getCreatedAt().equals(this.getCreatedAt()) == false)
return false;
if (other.getLastUpdatedAt() == null ^ this.getLastUpdatedAt() == null)
return false;
if (other.getLastUpdatedAt() != null
&& other.getLastUpdatedAt().equals(this.getLastUpdatedAt()) == false)
return false;
if (other.getCompletedAt() == null ^ this.getCompletedAt() == null)
return false;
if (other.getCompletedAt() != null
&& other.getCompletedAt().equals(this.getCompletedAt()) == false)
return false;
return true;
}
}