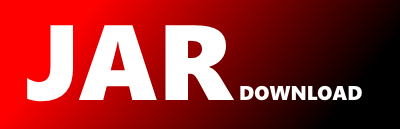
com.amazonaws.services.iot.model.OTAUpdateInfo Maven / Gradle / Ivy
/*
* Copyright 2010-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.iot.model;
import java.io.Serializable;
/**
*
* Information about an OTA update.
*
*/
public class OTAUpdateInfo implements Serializable {
/**
*
* The OTA update ID.
*
*
* Constraints:
* Length: 1 - 128
* Pattern: [a-zA-Z0-9_-]+
*/
private String otaUpdateId;
/**
*
* The OTA update ARN.
*
*/
private String otaUpdateArn;
/**
*
* The date when the OTA update was created.
*
*/
private java.util.Date creationDate;
/**
*
* The date when the OTA update was last updated.
*
*/
private java.util.Date lastModifiedDate;
/**
*
* A description of the OTA update.
*
*
* Constraints:
* Length: - 2028
* Pattern: [^\p{C}]+
*/
private String description;
/**
*
* The targets of the OTA update.
*
*/
private java.util.List targets;
/**
*
* Specifies whether the OTA update will continue to run (CONTINUOUS), or
* will be complete after all those things specified as targets have
* completed the OTA update (SNAPSHOT). If continuous, the OTA update may
* also be run on a thing when a change is detected in a target. For
* example, an OTA update will run on a thing when the thing is added to a
* target group, even after the OTA update was completed by all things
* originally in the group.
*
*
* Constraints:
* Allowed Values: CONTINUOUS, SNAPSHOT
*/
private String targetSelection;
/**
*
* A list of files associated with the OTA update.
*
*/
private java.util.List otaUpdateFiles;
/**
*
* The status of the OTA update.
*
*
* Constraints:
* Allowed Values: CREATE_PENDING, CREATE_IN_PROGRESS,
* CREATE_COMPLETE, CREATE_FAILED
*/
private String otaUpdateStatus;
/**
*
* The AWS IoT job ID associated with the OTA update.
*
*/
private String awsIotJobId;
/**
*
* The AWS IoT job ARN associated with the OTA update.
*
*/
private String awsIotJobArn;
/**
*
* Error information associated with the OTA update.
*
*/
private ErrorInfo errorInfo;
/**
*
* A collection of name/value pairs
*
*/
private java.util.Map additionalParameters;
/**
*
* The OTA update ID.
*
*
* Constraints:
* Length: 1 - 128
* Pattern: [a-zA-Z0-9_-]+
*
* @return
* The OTA update ID.
*
*/
public String getOtaUpdateId() {
return otaUpdateId;
}
/**
*
* The OTA update ID.
*
*
* Constraints:
* Length: 1 - 128
* Pattern: [a-zA-Z0-9_-]+
*
* @param otaUpdateId
* The OTA update ID.
*
*/
public void setOtaUpdateId(String otaUpdateId) {
this.otaUpdateId = otaUpdateId;
}
/**
*
* The OTA update ID.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* Constraints:
* Length: 1 - 128
* Pattern: [a-zA-Z0-9_-]+
*
* @param otaUpdateId
* The OTA update ID.
*
* @return A reference to this updated object so that method calls can be
* chained together.
*/
public OTAUpdateInfo withOtaUpdateId(String otaUpdateId) {
this.otaUpdateId = otaUpdateId;
return this;
}
/**
*
* The OTA update ARN.
*
*
* @return
* The OTA update ARN.
*
*/
public String getOtaUpdateArn() {
return otaUpdateArn;
}
/**
*
* The OTA update ARN.
*
*
* @param otaUpdateArn
* The OTA update ARN.
*
*/
public void setOtaUpdateArn(String otaUpdateArn) {
this.otaUpdateArn = otaUpdateArn;
}
/**
*
* The OTA update ARN.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* @param otaUpdateArn
* The OTA update ARN.
*
* @return A reference to this updated object so that method calls can be
* chained together.
*/
public OTAUpdateInfo withOtaUpdateArn(String otaUpdateArn) {
this.otaUpdateArn = otaUpdateArn;
return this;
}
/**
*
* The date when the OTA update was created.
*
*
* @return
* The date when the OTA update was created.
*
*/
public java.util.Date getCreationDate() {
return creationDate;
}
/**
*
* The date when the OTA update was created.
*
*
* @param creationDate
* The date when the OTA update was created.
*
*/
public void setCreationDate(java.util.Date creationDate) {
this.creationDate = creationDate;
}
/**
*
* The date when the OTA update was created.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* @param creationDate
* The date when the OTA update was created.
*
* @return A reference to this updated object so that method calls can be
* chained together.
*/
public OTAUpdateInfo withCreationDate(java.util.Date creationDate) {
this.creationDate = creationDate;
return this;
}
/**
*
* The date when the OTA update was last updated.
*
*
* @return
* The date when the OTA update was last updated.
*
*/
public java.util.Date getLastModifiedDate() {
return lastModifiedDate;
}
/**
*
* The date when the OTA update was last updated.
*
*
* @param lastModifiedDate
* The date when the OTA update was last updated.
*
*/
public void setLastModifiedDate(java.util.Date lastModifiedDate) {
this.lastModifiedDate = lastModifiedDate;
}
/**
*
* The date when the OTA update was last updated.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* @param lastModifiedDate
* The date when the OTA update was last updated.
*
* @return A reference to this updated object so that method calls can be
* chained together.
*/
public OTAUpdateInfo withLastModifiedDate(java.util.Date lastModifiedDate) {
this.lastModifiedDate = lastModifiedDate;
return this;
}
/**
*
* A description of the OTA update.
*
*
* Constraints:
* Length: - 2028
* Pattern: [^\p{C}]+
*
* @return
* A description of the OTA update.
*
*/
public String getDescription() {
return description;
}
/**
*
* A description of the OTA update.
*
*
* Constraints:
* Length: - 2028
* Pattern: [^\p{C}]+
*
* @param description
* A description of the OTA update.
*
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* A description of the OTA update.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* Constraints:
* Length: - 2028
* Pattern: [^\p{C}]+
*
* @param description
* A description of the OTA update.
*
* @return A reference to this updated object so that method calls can be
* chained together.
*/
public OTAUpdateInfo withDescription(String description) {
this.description = description;
return this;
}
/**
*
* The targets of the OTA update.
*
*
* @return
* The targets of the OTA update.
*
*/
public java.util.List getTargets() {
return targets;
}
/**
*
* The targets of the OTA update.
*
*
* @param targets
* The targets of the OTA update.
*
*/
public void setTargets(java.util.Collection targets) {
if (targets == null) {
this.targets = null;
return;
}
this.targets = new java.util.ArrayList(targets);
}
/**
*
* The targets of the OTA update.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* @param targets
* The targets of the OTA update.
*
* @return A reference to this updated object so that method calls can be
* chained together.
*/
public OTAUpdateInfo withTargets(String... targets) {
if (getTargets() == null) {
this.targets = new java.util.ArrayList(targets.length);
}
for (String value : targets) {
this.targets.add(value);
}
return this;
}
/**
*
* The targets of the OTA update.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* @param targets
* The targets of the OTA update.
*
* @return A reference to this updated object so that method calls can be
* chained together.
*/
public OTAUpdateInfo withTargets(java.util.Collection targets) {
setTargets(targets);
return this;
}
/**
*
* Specifies whether the OTA update will continue to run (CONTINUOUS), or
* will be complete after all those things specified as targets have
* completed the OTA update (SNAPSHOT). If continuous, the OTA update may
* also be run on a thing when a change is detected in a target. For
* example, an OTA update will run on a thing when the thing is added to a
* target group, even after the OTA update was completed by all things
* originally in the group.
*
*
* Constraints:
* Allowed Values: CONTINUOUS, SNAPSHOT
*
* @return
* Specifies whether the OTA update will continue to run
* (CONTINUOUS), or will be complete after all those things
* specified as targets have completed the OTA update (SNAPSHOT). If
* continuous, the OTA update may also be run on a thing when a
* change is detected in a target. For example, an OTA update will
* run on a thing when the thing is added to a target group, even
* after the OTA update was completed by all things originally in
* the group.
*
* @see TargetSelection
*/
public String getTargetSelection() {
return targetSelection;
}
/**
*
* Specifies whether the OTA update will continue to run (CONTINUOUS), or
* will be complete after all those things specified as targets have
* completed the OTA update (SNAPSHOT). If continuous, the OTA update may
* also be run on a thing when a change is detected in a target. For
* example, an OTA update will run on a thing when the thing is added to a
* target group, even after the OTA update was completed by all things
* originally in the group.
*
*
* Constraints:
* Allowed Values: CONTINUOUS, SNAPSHOT
*
* @param targetSelection
* Specifies whether the OTA update will continue to run
* (CONTINUOUS), or will be complete after all those things
* specified as targets have completed the OTA update (SNAPSHOT).
* If continuous, the OTA update may also be run on a thing when
* a change is detected in a target. For example, an OTA update
* will run on a thing when the thing is added to a target group,
* even after the OTA update was completed by all things
* originally in the group.
*
* @see TargetSelection
*/
public void setTargetSelection(String targetSelection) {
this.targetSelection = targetSelection;
}
/**
*
* Specifies whether the OTA update will continue to run (CONTINUOUS), or
* will be complete after all those things specified as targets have
* completed the OTA update (SNAPSHOT). If continuous, the OTA update may
* also be run on a thing when a change is detected in a target. For
* example, an OTA update will run on a thing when the thing is added to a
* target group, even after the OTA update was completed by all things
* originally in the group.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* Constraints:
* Allowed Values: CONTINUOUS, SNAPSHOT
*
* @param targetSelection
* Specifies whether the OTA update will continue to run
* (CONTINUOUS), or will be complete after all those things
* specified as targets have completed the OTA update (SNAPSHOT).
* If continuous, the OTA update may also be run on a thing when
* a change is detected in a target. For example, an OTA update
* will run on a thing when the thing is added to a target group,
* even after the OTA update was completed by all things
* originally in the group.
*
* @return A reference to this updated object so that method calls can be
* chained together.
* @see TargetSelection
*/
public OTAUpdateInfo withTargetSelection(String targetSelection) {
this.targetSelection = targetSelection;
return this;
}
/**
*
* Specifies whether the OTA update will continue to run (CONTINUOUS), or
* will be complete after all those things specified as targets have
* completed the OTA update (SNAPSHOT). If continuous, the OTA update may
* also be run on a thing when a change is detected in a target. For
* example, an OTA update will run on a thing when the thing is added to a
* target group, even after the OTA update was completed by all things
* originally in the group.
*
*
* Constraints:
* Allowed Values: CONTINUOUS, SNAPSHOT
*
* @param targetSelection
* Specifies whether the OTA update will continue to run
* (CONTINUOUS), or will be complete after all those things
* specified as targets have completed the OTA update (SNAPSHOT).
* If continuous, the OTA update may also be run on a thing when
* a change is detected in a target. For example, an OTA update
* will run on a thing when the thing is added to a target group,
* even after the OTA update was completed by all things
* originally in the group.
*
* @see TargetSelection
*/
public void setTargetSelection(TargetSelection targetSelection) {
this.targetSelection = targetSelection.toString();
}
/**
*
* Specifies whether the OTA update will continue to run (CONTINUOUS), or
* will be complete after all those things specified as targets have
* completed the OTA update (SNAPSHOT). If continuous, the OTA update may
* also be run on a thing when a change is detected in a target. For
* example, an OTA update will run on a thing when the thing is added to a
* target group, even after the OTA update was completed by all things
* originally in the group.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* Constraints:
* Allowed Values: CONTINUOUS, SNAPSHOT
*
* @param targetSelection
* Specifies whether the OTA update will continue to run
* (CONTINUOUS), or will be complete after all those things
* specified as targets have completed the OTA update (SNAPSHOT).
* If continuous, the OTA update may also be run on a thing when
* a change is detected in a target. For example, an OTA update
* will run on a thing when the thing is added to a target group,
* even after the OTA update was completed by all things
* originally in the group.
*
* @return A reference to this updated object so that method calls can be
* chained together.
* @see TargetSelection
*/
public OTAUpdateInfo withTargetSelection(TargetSelection targetSelection) {
this.targetSelection = targetSelection.toString();
return this;
}
/**
*
* A list of files associated with the OTA update.
*
*
* @return
* A list of files associated with the OTA update.
*
*/
public java.util.List getOtaUpdateFiles() {
return otaUpdateFiles;
}
/**
*
* A list of files associated with the OTA update.
*
*
* @param otaUpdateFiles
* A list of files associated with the OTA update.
*
*/
public void setOtaUpdateFiles(java.util.Collection otaUpdateFiles) {
if (otaUpdateFiles == null) {
this.otaUpdateFiles = null;
return;
}
this.otaUpdateFiles = new java.util.ArrayList(otaUpdateFiles);
}
/**
*
* A list of files associated with the OTA update.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* @param otaUpdateFiles
* A list of files associated with the OTA update.
*
* @return A reference to this updated object so that method calls can be
* chained together.
*/
public OTAUpdateInfo withOtaUpdateFiles(OTAUpdateFile... otaUpdateFiles) {
if (getOtaUpdateFiles() == null) {
this.otaUpdateFiles = new java.util.ArrayList(otaUpdateFiles.length);
}
for (OTAUpdateFile value : otaUpdateFiles) {
this.otaUpdateFiles.add(value);
}
return this;
}
/**
*
* A list of files associated with the OTA update.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* @param otaUpdateFiles
* A list of files associated with the OTA update.
*
* @return A reference to this updated object so that method calls can be
* chained together.
*/
public OTAUpdateInfo withOtaUpdateFiles(java.util.Collection otaUpdateFiles) {
setOtaUpdateFiles(otaUpdateFiles);
return this;
}
/**
*
* The status of the OTA update.
*
*
* Constraints:
* Allowed Values: CREATE_PENDING, CREATE_IN_PROGRESS,
* CREATE_COMPLETE, CREATE_FAILED
*
* @return
* The status of the OTA update.
*
* @see OTAUpdateStatus
*/
public String getOtaUpdateStatus() {
return otaUpdateStatus;
}
/**
*
* The status of the OTA update.
*
*
* Constraints:
* Allowed Values: CREATE_PENDING, CREATE_IN_PROGRESS,
* CREATE_COMPLETE, CREATE_FAILED
*
* @param otaUpdateStatus
* The status of the OTA update.
*
* @see OTAUpdateStatus
*/
public void setOtaUpdateStatus(String otaUpdateStatus) {
this.otaUpdateStatus = otaUpdateStatus;
}
/**
*
* The status of the OTA update.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* Constraints:
* Allowed Values: CREATE_PENDING, CREATE_IN_PROGRESS,
* CREATE_COMPLETE, CREATE_FAILED
*
* @param otaUpdateStatus
* The status of the OTA update.
*
* @return A reference to this updated object so that method calls can be
* chained together.
* @see OTAUpdateStatus
*/
public OTAUpdateInfo withOtaUpdateStatus(String otaUpdateStatus) {
this.otaUpdateStatus = otaUpdateStatus;
return this;
}
/**
*
* The status of the OTA update.
*
*
* Constraints:
* Allowed Values: CREATE_PENDING, CREATE_IN_PROGRESS,
* CREATE_COMPLETE, CREATE_FAILED
*
* @param otaUpdateStatus
* The status of the OTA update.
*
* @see OTAUpdateStatus
*/
public void setOtaUpdateStatus(OTAUpdateStatus otaUpdateStatus) {
this.otaUpdateStatus = otaUpdateStatus.toString();
}
/**
*
* The status of the OTA update.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* Constraints:
* Allowed Values: CREATE_PENDING, CREATE_IN_PROGRESS,
* CREATE_COMPLETE, CREATE_FAILED
*
* @param otaUpdateStatus
* The status of the OTA update.
*
* @return A reference to this updated object so that method calls can be
* chained together.
* @see OTAUpdateStatus
*/
public OTAUpdateInfo withOtaUpdateStatus(OTAUpdateStatus otaUpdateStatus) {
this.otaUpdateStatus = otaUpdateStatus.toString();
return this;
}
/**
*
* The AWS IoT job ID associated with the OTA update.
*
*
* @return
* The AWS IoT job ID associated with the OTA update.
*
*/
public String getAwsIotJobId() {
return awsIotJobId;
}
/**
*
* The AWS IoT job ID associated with the OTA update.
*
*
* @param awsIotJobId
* The AWS IoT job ID associated with the OTA update.
*
*/
public void setAwsIotJobId(String awsIotJobId) {
this.awsIotJobId = awsIotJobId;
}
/**
*
* The AWS IoT job ID associated with the OTA update.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* @param awsIotJobId
* The AWS IoT job ID associated with the OTA update.
*
* @return A reference to this updated object so that method calls can be
* chained together.
*/
public OTAUpdateInfo withAwsIotJobId(String awsIotJobId) {
this.awsIotJobId = awsIotJobId;
return this;
}
/**
*
* The AWS IoT job ARN associated with the OTA update.
*
*
* @return
* The AWS IoT job ARN associated with the OTA update.
*
*/
public String getAwsIotJobArn() {
return awsIotJobArn;
}
/**
*
* The AWS IoT job ARN associated with the OTA update.
*
*
* @param awsIotJobArn
* The AWS IoT job ARN associated with the OTA update.
*
*/
public void setAwsIotJobArn(String awsIotJobArn) {
this.awsIotJobArn = awsIotJobArn;
}
/**
*
* The AWS IoT job ARN associated with the OTA update.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* @param awsIotJobArn
* The AWS IoT job ARN associated with the OTA update.
*
* @return A reference to this updated object so that method calls can be
* chained together.
*/
public OTAUpdateInfo withAwsIotJobArn(String awsIotJobArn) {
this.awsIotJobArn = awsIotJobArn;
return this;
}
/**
*
* Error information associated with the OTA update.
*
*
* @return
* Error information associated with the OTA update.
*
*/
public ErrorInfo getErrorInfo() {
return errorInfo;
}
/**
*
* Error information associated with the OTA update.
*
*
* @param errorInfo
* Error information associated with the OTA update.
*
*/
public void setErrorInfo(ErrorInfo errorInfo) {
this.errorInfo = errorInfo;
}
/**
*
* Error information associated with the OTA update.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* @param errorInfo
* Error information associated with the OTA update.
*
* @return A reference to this updated object so that method calls can be
* chained together.
*/
public OTAUpdateInfo withErrorInfo(ErrorInfo errorInfo) {
this.errorInfo = errorInfo;
return this;
}
/**
*
* A collection of name/value pairs
*
*
* @return
* A collection of name/value pairs
*
*/
public java.util.Map getAdditionalParameters() {
return additionalParameters;
}
/**
*
* A collection of name/value pairs
*
*
* @param additionalParameters
* A collection of name/value pairs
*
*/
public void setAdditionalParameters(java.util.Map additionalParameters) {
this.additionalParameters = additionalParameters;
}
/**
*
* A collection of name/value pairs
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* @param additionalParameters
* A collection of name/value pairs
*
* @return A reference to this updated object so that method calls can be
* chained together.
*/
public OTAUpdateInfo withAdditionalParameters(java.util.Map additionalParameters) {
this.additionalParameters = additionalParameters;
return this;
}
/**
*
* A collection of name/value pairs
*
*
* The method adds a new key-value pair into additionalParameters parameter,
* and returns a reference to this object so that method calls can be
* chained together.
*
* @param key The key of the entry to be added into additionalParameters.
* @param value The corresponding value of the entry to be added into
* additionalParameters.
* @return A reference to this updated object so that method calls can be
* chained together.
*/
public OTAUpdateInfo addadditionalParametersEntry(String key, String value) {
if (null == this.additionalParameters) {
this.additionalParameters = new java.util.HashMap();
}
if (this.additionalParameters.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString()
+ ") are provided.");
this.additionalParameters.put(key, value);
return this;
}
/**
* Removes all the entries added into additionalParameters.
*
* Returns a reference to this object so that method calls can be chained
* together.
*/
public OTAUpdateInfo clearadditionalParametersEntries() {
this.additionalParameters = null;
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getOtaUpdateId() != null)
sb.append("otaUpdateId: " + getOtaUpdateId() + ",");
if (getOtaUpdateArn() != null)
sb.append("otaUpdateArn: " + getOtaUpdateArn() + ",");
if (getCreationDate() != null)
sb.append("creationDate: " + getCreationDate() + ",");
if (getLastModifiedDate() != null)
sb.append("lastModifiedDate: " + getLastModifiedDate() + ",");
if (getDescription() != null)
sb.append("description: " + getDescription() + ",");
if (getTargets() != null)
sb.append("targets: " + getTargets() + ",");
if (getTargetSelection() != null)
sb.append("targetSelection: " + getTargetSelection() + ",");
if (getOtaUpdateFiles() != null)
sb.append("otaUpdateFiles: " + getOtaUpdateFiles() + ",");
if (getOtaUpdateStatus() != null)
sb.append("otaUpdateStatus: " + getOtaUpdateStatus() + ",");
if (getAwsIotJobId() != null)
sb.append("awsIotJobId: " + getAwsIotJobId() + ",");
if (getAwsIotJobArn() != null)
sb.append("awsIotJobArn: " + getAwsIotJobArn() + ",");
if (getErrorInfo() != null)
sb.append("errorInfo: " + getErrorInfo() + ",");
if (getAdditionalParameters() != null)
sb.append("additionalParameters: " + getAdditionalParameters());
sb.append("}");
return sb.toString();
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode
+ ((getOtaUpdateId() == null) ? 0 : getOtaUpdateId().hashCode());
hashCode = prime * hashCode
+ ((getOtaUpdateArn() == null) ? 0 : getOtaUpdateArn().hashCode());
hashCode = prime * hashCode
+ ((getCreationDate() == null) ? 0 : getCreationDate().hashCode());
hashCode = prime * hashCode
+ ((getLastModifiedDate() == null) ? 0 : getLastModifiedDate().hashCode());
hashCode = prime * hashCode
+ ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getTargets() == null) ? 0 : getTargets().hashCode());
hashCode = prime * hashCode
+ ((getTargetSelection() == null) ? 0 : getTargetSelection().hashCode());
hashCode = prime * hashCode
+ ((getOtaUpdateFiles() == null) ? 0 : getOtaUpdateFiles().hashCode());
hashCode = prime * hashCode
+ ((getOtaUpdateStatus() == null) ? 0 : getOtaUpdateStatus().hashCode());
hashCode = prime * hashCode
+ ((getAwsIotJobId() == null) ? 0 : getAwsIotJobId().hashCode());
hashCode = prime * hashCode
+ ((getAwsIotJobArn() == null) ? 0 : getAwsIotJobArn().hashCode());
hashCode = prime * hashCode + ((getErrorInfo() == null) ? 0 : getErrorInfo().hashCode());
hashCode = prime * hashCode
+ ((getAdditionalParameters() == null) ? 0 : getAdditionalParameters().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof OTAUpdateInfo == false)
return false;
OTAUpdateInfo other = (OTAUpdateInfo) obj;
if (other.getOtaUpdateId() == null ^ this.getOtaUpdateId() == null)
return false;
if (other.getOtaUpdateId() != null
&& other.getOtaUpdateId().equals(this.getOtaUpdateId()) == false)
return false;
if (other.getOtaUpdateArn() == null ^ this.getOtaUpdateArn() == null)
return false;
if (other.getOtaUpdateArn() != null
&& other.getOtaUpdateArn().equals(this.getOtaUpdateArn()) == false)
return false;
if (other.getCreationDate() == null ^ this.getCreationDate() == null)
return false;
if (other.getCreationDate() != null
&& other.getCreationDate().equals(this.getCreationDate()) == false)
return false;
if (other.getLastModifiedDate() == null ^ this.getLastModifiedDate() == null)
return false;
if (other.getLastModifiedDate() != null
&& other.getLastModifiedDate().equals(this.getLastModifiedDate()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null
&& other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getTargets() == null ^ this.getTargets() == null)
return false;
if (other.getTargets() != null && other.getTargets().equals(this.getTargets()) == false)
return false;
if (other.getTargetSelection() == null ^ this.getTargetSelection() == null)
return false;
if (other.getTargetSelection() != null
&& other.getTargetSelection().equals(this.getTargetSelection()) == false)
return false;
if (other.getOtaUpdateFiles() == null ^ this.getOtaUpdateFiles() == null)
return false;
if (other.getOtaUpdateFiles() != null
&& other.getOtaUpdateFiles().equals(this.getOtaUpdateFiles()) == false)
return false;
if (other.getOtaUpdateStatus() == null ^ this.getOtaUpdateStatus() == null)
return false;
if (other.getOtaUpdateStatus() != null
&& other.getOtaUpdateStatus().equals(this.getOtaUpdateStatus()) == false)
return false;
if (other.getAwsIotJobId() == null ^ this.getAwsIotJobId() == null)
return false;
if (other.getAwsIotJobId() != null
&& other.getAwsIotJobId().equals(this.getAwsIotJobId()) == false)
return false;
if (other.getAwsIotJobArn() == null ^ this.getAwsIotJobArn() == null)
return false;
if (other.getAwsIotJobArn() != null
&& other.getAwsIotJobArn().equals(this.getAwsIotJobArn()) == false)
return false;
if (other.getErrorInfo() == null ^ this.getErrorInfo() == null)
return false;
if (other.getErrorInfo() != null
&& other.getErrorInfo().equals(this.getErrorInfo()) == false)
return false;
if (other.getAdditionalParameters() == null ^ this.getAdditionalParameters() == null)
return false;
if (other.getAdditionalParameters() != null
&& other.getAdditionalParameters().equals(this.getAdditionalParameters()) == false)
return false;
return true;
}
}