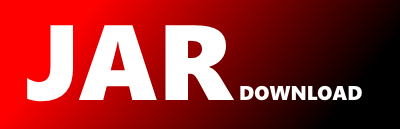
com.amazonaws.services.iot.model.StartThingRegistrationTaskRequest Maven / Gradle / Ivy
Show all versions of aws-android-sdk-iot Show documentation
/*
* Copyright 2010-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.iot.model;
import java.io.Serializable;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* Creates a bulk thing provisioning task.
*
*/
public class StartThingRegistrationTaskRequest extends AmazonWebServiceRequest implements
Serializable {
/**
*
* The provisioning template.
*
*/
private String templateBody;
/**
*
* The S3 bucket that contains the input file.
*
*
* Constraints:
* Length: 3 - 256
* Pattern: [a-zA-Z0-9._-]+
*/
private String inputFileBucket;
/**
*
* The name of input file within the S3 bucket. This file contains a newline
* delimited JSON file. Each line contains the parameter values to provision
* one device (thing).
*
*
* Constraints:
* Length: 1 - 1024
* Pattern: [a-zA-Z0-9!_.*'()-\/]+
*/
private String inputFileKey;
/**
*
* The IAM role ARN that grants permission the input file.
*
*
* Constraints:
* Length: 20 - 2048
*/
private String roleArn;
/**
*
* The provisioning template.
*
*
* @return
* The provisioning template.
*
*/
public String getTemplateBody() {
return templateBody;
}
/**
*
* The provisioning template.
*
*
* @param templateBody
* The provisioning template.
*
*/
public void setTemplateBody(String templateBody) {
this.templateBody = templateBody;
}
/**
*
* The provisioning template.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* @param templateBody
* The provisioning template.
*
* @return A reference to this updated object so that method calls can be
* chained together.
*/
public StartThingRegistrationTaskRequest withTemplateBody(String templateBody) {
this.templateBody = templateBody;
return this;
}
/**
*
* The S3 bucket that contains the input file.
*
*
* Constraints:
* Length: 3 - 256
* Pattern: [a-zA-Z0-9._-]+
*
* @return
* The S3 bucket that contains the input file.
*
*/
public String getInputFileBucket() {
return inputFileBucket;
}
/**
*
* The S3 bucket that contains the input file.
*
*
* Constraints:
* Length: 3 - 256
* Pattern: [a-zA-Z0-9._-]+
*
* @param inputFileBucket
* The S3 bucket that contains the input file.
*
*/
public void setInputFileBucket(String inputFileBucket) {
this.inputFileBucket = inputFileBucket;
}
/**
*
* The S3 bucket that contains the input file.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* Constraints:
* Length: 3 - 256
* Pattern: [a-zA-Z0-9._-]+
*
* @param inputFileBucket
* The S3 bucket that contains the input file.
*
* @return A reference to this updated object so that method calls can be
* chained together.
*/
public StartThingRegistrationTaskRequest withInputFileBucket(String inputFileBucket) {
this.inputFileBucket = inputFileBucket;
return this;
}
/**
*
* The name of input file within the S3 bucket. This file contains a newline
* delimited JSON file. Each line contains the parameter values to provision
* one device (thing).
*
*
* Constraints:
* Length: 1 - 1024
* Pattern: [a-zA-Z0-9!_.*'()-\/]+
*
* @return
* The name of input file within the S3 bucket. This file contains a
* newline delimited JSON file. Each line contains the parameter
* values to provision one device (thing).
*
*/
public String getInputFileKey() {
return inputFileKey;
}
/**
*
* The name of input file within the S3 bucket. This file contains a newline
* delimited JSON file. Each line contains the parameter values to provision
* one device (thing).
*
*
* Constraints:
* Length: 1 - 1024
* Pattern: [a-zA-Z0-9!_.*'()-\/]+
*
* @param inputFileKey
* The name of input file within the S3 bucket. This file
* contains a newline delimited JSON file. Each line contains the
* parameter values to provision one device (thing).
*
*/
public void setInputFileKey(String inputFileKey) {
this.inputFileKey = inputFileKey;
}
/**
*
* The name of input file within the S3 bucket. This file contains a newline
* delimited JSON file. Each line contains the parameter values to provision
* one device (thing).
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* Constraints:
* Length: 1 - 1024
* Pattern: [a-zA-Z0-9!_.*'()-\/]+
*
* @param inputFileKey
* The name of input file within the S3 bucket. This file
* contains a newline delimited JSON file. Each line contains the
* parameter values to provision one device (thing).
*
* @return A reference to this updated object so that method calls can be
* chained together.
*/
public StartThingRegistrationTaskRequest withInputFileKey(String inputFileKey) {
this.inputFileKey = inputFileKey;
return this;
}
/**
*
* The IAM role ARN that grants permission the input file.
*
*
* Constraints:
* Length: 20 - 2048
*
* @return
* The IAM role ARN that grants permission the input file.
*
*/
public String getRoleArn() {
return roleArn;
}
/**
*
* The IAM role ARN that grants permission the input file.
*
*
* Constraints:
* Length: 20 - 2048
*
* @param roleArn
* The IAM role ARN that grants permission the input file.
*
*/
public void setRoleArn(String roleArn) {
this.roleArn = roleArn;
}
/**
*
* The IAM role ARN that grants permission the input file.
*
*
* Returns a reference to this object so that method calls can be chained
* together.
*
* Constraints:
* Length: 20 - 2048
*
* @param roleArn
* The IAM role ARN that grants permission the input file.
*
* @return A reference to this updated object so that method calls can be
* chained together.
*/
public StartThingRegistrationTaskRequest withRoleArn(String roleArn) {
this.roleArn = roleArn;
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getTemplateBody() != null)
sb.append("templateBody: " + getTemplateBody() + ",");
if (getInputFileBucket() != null)
sb.append("inputFileBucket: " + getInputFileBucket() + ",");
if (getInputFileKey() != null)
sb.append("inputFileKey: " + getInputFileKey() + ",");
if (getRoleArn() != null)
sb.append("roleArn: " + getRoleArn());
sb.append("}");
return sb.toString();
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode
+ ((getTemplateBody() == null) ? 0 : getTemplateBody().hashCode());
hashCode = prime * hashCode
+ ((getInputFileBucket() == null) ? 0 : getInputFileBucket().hashCode());
hashCode = prime * hashCode
+ ((getInputFileKey() == null) ? 0 : getInputFileKey().hashCode());
hashCode = prime * hashCode + ((getRoleArn() == null) ? 0 : getRoleArn().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof StartThingRegistrationTaskRequest == false)
return false;
StartThingRegistrationTaskRequest other = (StartThingRegistrationTaskRequest) obj;
if (other.getTemplateBody() == null ^ this.getTemplateBody() == null)
return false;
if (other.getTemplateBody() != null
&& other.getTemplateBody().equals(this.getTemplateBody()) == false)
return false;
if (other.getInputFileBucket() == null ^ this.getInputFileBucket() == null)
return false;
if (other.getInputFileBucket() != null
&& other.getInputFileBucket().equals(this.getInputFileBucket()) == false)
return false;
if (other.getInputFileKey() == null ^ this.getInputFileKey() == null)
return false;
if (other.getInputFileKey() != null
&& other.getInputFileKey().equals(this.getInputFileKey()) == false)
return false;
if (other.getRoleArn() == null ^ this.getRoleArn() == null)
return false;
if (other.getRoleArn() != null && other.getRoleArn().equals(this.getRoleArn()) == false)
return false;
return true;
}
}