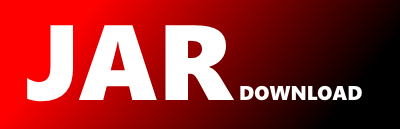
com.amazonaws.services.account.AWSAccountAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-account Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.account;
import javax.annotation.Generated;
import com.amazonaws.services.account.model.*;
/**
* Interface for accessing AWS Account asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.account.AbstractAWSAccountAsync} instead.
*
*
*
* Operations for Amazon Web Services Account Management
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSAccountAsync extends AWSAccount {
/**
*
* Accepts the request that originated from StartPrimaryEmailUpdate to update the primary email address (also
* known as the root user email address) for the specified account.
*
*
* @param acceptPrimaryEmailUpdateRequest
* @return A Java Future containing the result of the AcceptPrimaryEmailUpdate operation returned by the service.
* @sample AWSAccountAsync.AcceptPrimaryEmailUpdate
* @see AWS API Documentation
*/
java.util.concurrent.Future acceptPrimaryEmailUpdateAsync(AcceptPrimaryEmailUpdateRequest acceptPrimaryEmailUpdateRequest);
/**
*
* Accepts the request that originated from StartPrimaryEmailUpdate to update the primary email address (also
* known as the root user email address) for the specified account.
*
*
* @param acceptPrimaryEmailUpdateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AcceptPrimaryEmailUpdate operation returned by the service.
* @sample AWSAccountAsyncHandler.AcceptPrimaryEmailUpdate
* @see AWS API Documentation
*/
java.util.concurrent.Future acceptPrimaryEmailUpdateAsync(AcceptPrimaryEmailUpdateRequest acceptPrimaryEmailUpdateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified alternate contact from an Amazon Web Services account.
*
*
* For complete details about how to use the alternate contact operations, see Access or updating
* the alternate contacts.
*
*
*
* Before you can update the alternate contact information for an Amazon Web Services account that is managed by
* Organizations, you must first enable integration between Amazon Web Services Account Management and
* Organizations. For more information, see Enabling trusted
* access for Amazon Web Services Account Management.
*
*
*
* @param deleteAlternateContactRequest
* @return A Java Future containing the result of the DeleteAlternateContact operation returned by the service.
* @sample AWSAccountAsync.DeleteAlternateContact
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteAlternateContactAsync(DeleteAlternateContactRequest deleteAlternateContactRequest);
/**
*
* Deletes the specified alternate contact from an Amazon Web Services account.
*
*
* For complete details about how to use the alternate contact operations, see Access or updating
* the alternate contacts.
*
*
*
* Before you can update the alternate contact information for an Amazon Web Services account that is managed by
* Organizations, you must first enable integration between Amazon Web Services Account Management and
* Organizations. For more information, see Enabling trusted
* access for Amazon Web Services Account Management.
*
*
*
* @param deleteAlternateContactRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteAlternateContact operation returned by the service.
* @sample AWSAccountAsyncHandler.DeleteAlternateContact
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteAlternateContactAsync(DeleteAlternateContactRequest deleteAlternateContactRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disables (opts-out) a particular Region for an account.
*
*
*
* The act of disabling a Region will remove all IAM access to any resources that reside in that Region.
*
*
*
* @param disableRegionRequest
* @return A Java Future containing the result of the DisableRegion operation returned by the service.
* @sample AWSAccountAsync.DisableRegion
* @see AWS API
* Documentation
*/
java.util.concurrent.Future disableRegionAsync(DisableRegionRequest disableRegionRequest);
/**
*
* Disables (opts-out) a particular Region for an account.
*
*
*
* The act of disabling a Region will remove all IAM access to any resources that reside in that Region.
*
*
*
* @param disableRegionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisableRegion operation returned by the service.
* @sample AWSAccountAsyncHandler.DisableRegion
* @see AWS API
* Documentation
*/
java.util.concurrent.Future disableRegionAsync(DisableRegionRequest disableRegionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Enables (opts-in) a particular Region for an account.
*
*
* @param enableRegionRequest
* @return A Java Future containing the result of the EnableRegion operation returned by the service.
* @sample AWSAccountAsync.EnableRegion
* @see AWS API
* Documentation
*/
java.util.concurrent.Future enableRegionAsync(EnableRegionRequest enableRegionRequest);
/**
*
* Enables (opts-in) a particular Region for an account.
*
*
* @param enableRegionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the EnableRegion operation returned by the service.
* @sample AWSAccountAsyncHandler.EnableRegion
* @see AWS API
* Documentation
*/
java.util.concurrent.Future enableRegionAsync(EnableRegionRequest enableRegionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the specified alternate contact attached to an Amazon Web Services account.
*
*
* For complete details about how to use the alternate contact operations, see Access or updating
* the alternate contacts.
*
*
*
* Before you can update the alternate contact information for an Amazon Web Services account that is managed by
* Organizations, you must first enable integration between Amazon Web Services Account Management and
* Organizations. For more information, see Enabling trusted
* access for Amazon Web Services Account Management.
*
*
*
* @param getAlternateContactRequest
* @return A Java Future containing the result of the GetAlternateContact operation returned by the service.
* @sample AWSAccountAsync.GetAlternateContact
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getAlternateContactAsync(GetAlternateContactRequest getAlternateContactRequest);
/**
*
* Retrieves the specified alternate contact attached to an Amazon Web Services account.
*
*
* For complete details about how to use the alternate contact operations, see Access or updating
* the alternate contacts.
*
*
*
* Before you can update the alternate contact information for an Amazon Web Services account that is managed by
* Organizations, you must first enable integration between Amazon Web Services Account Management and
* Organizations. For more information, see Enabling trusted
* access for Amazon Web Services Account Management.
*
*
*
* @param getAlternateContactRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetAlternateContact operation returned by the service.
* @sample AWSAccountAsyncHandler.GetAlternateContact
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getAlternateContactAsync(GetAlternateContactRequest getAlternateContactRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the primary contact information of an Amazon Web Services account.
*
*
* For complete details about how to use the primary contact operations, see Update the primary
* and alternate contact information.
*
*
* @param getContactInformationRequest
* @return A Java Future containing the result of the GetContactInformation operation returned by the service.
* @sample AWSAccountAsync.GetContactInformation
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getContactInformationAsync(GetContactInformationRequest getContactInformationRequest);
/**
*
* Retrieves the primary contact information of an Amazon Web Services account.
*
*
* For complete details about how to use the primary contact operations, see Update the primary
* and alternate contact information.
*
*
* @param getContactInformationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetContactInformation operation returned by the service.
* @sample AWSAccountAsyncHandler.GetContactInformation
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getContactInformationAsync(GetContactInformationRequest getContactInformationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the primary email address for the specified account.
*
*
* @param getPrimaryEmailRequest
* @return A Java Future containing the result of the GetPrimaryEmail operation returned by the service.
* @sample AWSAccountAsync.GetPrimaryEmail
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getPrimaryEmailAsync(GetPrimaryEmailRequest getPrimaryEmailRequest);
/**
*
* Retrieves the primary email address for the specified account.
*
*
* @param getPrimaryEmailRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetPrimaryEmail operation returned by the service.
* @sample AWSAccountAsyncHandler.GetPrimaryEmail
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getPrimaryEmailAsync(GetPrimaryEmailRequest getPrimaryEmailRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the opt-in status of a particular Region.
*
*
* @param getRegionOptStatusRequest
* @return A Java Future containing the result of the GetRegionOptStatus operation returned by the service.
* @sample AWSAccountAsync.GetRegionOptStatus
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getRegionOptStatusAsync(GetRegionOptStatusRequest getRegionOptStatusRequest);
/**
*
* Retrieves the opt-in status of a particular Region.
*
*
* @param getRegionOptStatusRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetRegionOptStatus operation returned by the service.
* @sample AWSAccountAsyncHandler.GetRegionOptStatus
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getRegionOptStatusAsync(GetRegionOptStatusRequest getRegionOptStatusRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all the Regions for a given account and their respective opt-in statuses. Optionally, this list can be
* filtered by the region-opt-status-contains
parameter.
*
*
* @param listRegionsRequest
* @return A Java Future containing the result of the ListRegions operation returned by the service.
* @sample AWSAccountAsync.ListRegions
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listRegionsAsync(ListRegionsRequest listRegionsRequest);
/**
*
* Lists all the Regions for a given account and their respective opt-in statuses. Optionally, this list can be
* filtered by the region-opt-status-contains
parameter.
*
*
* @param listRegionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListRegions operation returned by the service.
* @sample AWSAccountAsyncHandler.ListRegions
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listRegionsAsync(ListRegionsRequest listRegionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Modifies the specified alternate contact attached to an Amazon Web Services account.
*
*
* For complete details about how to use the alternate contact operations, see Access or updating
* the alternate contacts.
*
*
*
* Before you can update the alternate contact information for an Amazon Web Services account that is managed by
* Organizations, you must first enable integration between Amazon Web Services Account Management and
* Organizations. For more information, see Enabling trusted
* access for Amazon Web Services Account Management.
*
*
*
* @param putAlternateContactRequest
* @return A Java Future containing the result of the PutAlternateContact operation returned by the service.
* @sample AWSAccountAsync.PutAlternateContact
* @see AWS
* API Documentation
*/
java.util.concurrent.Future putAlternateContactAsync(PutAlternateContactRequest putAlternateContactRequest);
/**
*
* Modifies the specified alternate contact attached to an Amazon Web Services account.
*
*
* For complete details about how to use the alternate contact operations, see Access or updating
* the alternate contacts.
*
*
*
* Before you can update the alternate contact information for an Amazon Web Services account that is managed by
* Organizations, you must first enable integration between Amazon Web Services Account Management and
* Organizations. For more information, see Enabling trusted
* access for Amazon Web Services Account Management.
*
*
*
* @param putAlternateContactRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutAlternateContact operation returned by the service.
* @sample AWSAccountAsyncHandler.PutAlternateContact
* @see AWS
* API Documentation
*/
java.util.concurrent.Future putAlternateContactAsync(PutAlternateContactRequest putAlternateContactRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the primary contact information of an Amazon Web Services account.
*
*
* For complete details about how to use the primary contact operations, see Update the primary
* and alternate contact information.
*
*
* @param putContactInformationRequest
* @return A Java Future containing the result of the PutContactInformation operation returned by the service.
* @sample AWSAccountAsync.PutContactInformation
* @see AWS
* API Documentation
*/
java.util.concurrent.Future putContactInformationAsync(PutContactInformationRequest putContactInformationRequest);
/**
*
* Updates the primary contact information of an Amazon Web Services account.
*
*
* For complete details about how to use the primary contact operations, see Update the primary
* and alternate contact information.
*
*
* @param putContactInformationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutContactInformation operation returned by the service.
* @sample AWSAccountAsyncHandler.PutContactInformation
* @see AWS
* API Documentation
*/
java.util.concurrent.Future putContactInformationAsync(PutContactInformationRequest putContactInformationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Starts the process to update the primary email address for the specified account.
*
*
* @param startPrimaryEmailUpdateRequest
* @return A Java Future containing the result of the StartPrimaryEmailUpdate operation returned by the service.
* @sample AWSAccountAsync.StartPrimaryEmailUpdate
* @see AWS API Documentation
*/
java.util.concurrent.Future startPrimaryEmailUpdateAsync(StartPrimaryEmailUpdateRequest startPrimaryEmailUpdateRequest);
/**
*
* Starts the process to update the primary email address for the specified account.
*
*
* @param startPrimaryEmailUpdateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartPrimaryEmailUpdate operation returned by the service.
* @sample AWSAccountAsyncHandler.StartPrimaryEmailUpdate
* @see AWS API Documentation
*/
java.util.concurrent.Future startPrimaryEmailUpdateAsync(StartPrimaryEmailUpdateRequest startPrimaryEmailUpdateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}