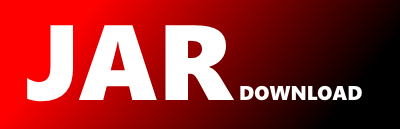
com.amazonaws.services.certificatemanager.model.RequestCertificateRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-acm Show documentation
/*
* Copyright 2010-2016 Amazon.com, Inc. or its affiliates. All Rights
* Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.certificatemanager.model;
import java.io.Serializable;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
*/
public class RequestCertificateRequest extends AmazonWebServiceRequest
implements Serializable, Cloneable {
/**
*
* Fully qualified domain name (FQDN), such as www.example.com, of the site
* you want to secure with an ACM Certificate. Use an asterisk (*) to create
* a wildcard certificate that protects several sites in the same domain.
* For example, *.example.com protects www.example.com, site.example.com,
* and images.example.com.
*
*/
private String domainName;
/**
*
* Additional FQDNs to be included in the Subject Alternative Name extension
* of the ACM Certificate. For example, add the name www.example.net to a
* certificate for which the DomainName
field is
* www.example.com if users can reach your site by using either name.
*
*/
private java.util.List subjectAlternativeNames;
/**
*
* Customer chosen string that can be used to distinguish between calls to
* RequestCertificate
. Idempotency tokens time out after one
* hour. Therefore, if you call RequestCertificate
multiple
* times with the same idempotency token within one hour, ACM recognizes
* that you are requesting only one certificate and will issue only one. If
* you change the idempotency token for each call, ACM recognizes that you
* are requesting multiple certificates.
*
*/
private String idempotencyToken;
/**
*
* The base validation domain that will act as the suffix of the email
* addresses that are used to send the emails. This must be the same as the
* Domain
value or a superdomain of the Domain
* value. For example, if you requested a certificate for
* test.example.com
and specify DomainValidationOptions
* of example.com
, ACM sends email to the domain registrant,
* technical contact, and administrative contact in WHOIS and the following
* five addresses:
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
*
*/
private java.util.List domainValidationOptions;
/**
*
* Fully qualified domain name (FQDN), such as www.example.com, of the site
* you want to secure with an ACM Certificate. Use an asterisk (*) to create
* a wildcard certificate that protects several sites in the same domain.
* For example, *.example.com protects www.example.com, site.example.com,
* and images.example.com.
*
*
* @param domainName
* Fully qualified domain name (FQDN), such as www.example.com, of
* the site you want to secure with an ACM Certificate. Use an
* asterisk (*) to create a wildcard certificate that protects
* several sites in the same domain. For example, *.example.com
* protects www.example.com, site.example.com, and
* images.example.com.
*/
public void setDomainName(String domainName) {
this.domainName = domainName;
}
/**
*
* Fully qualified domain name (FQDN), such as www.example.com, of the site
* you want to secure with an ACM Certificate. Use an asterisk (*) to create
* a wildcard certificate that protects several sites in the same domain.
* For example, *.example.com protects www.example.com, site.example.com,
* and images.example.com.
*
*
* @return Fully qualified domain name (FQDN), such as www.example.com, of
* the site you want to secure with an ACM Certificate. Use an
* asterisk (*) to create a wildcard certificate that protects
* several sites in the same domain. For example, *.example.com
* protects www.example.com, site.example.com, and
* images.example.com.
*/
public String getDomainName() {
return this.domainName;
}
/**
*
* Fully qualified domain name (FQDN), such as www.example.com, of the site
* you want to secure with an ACM Certificate. Use an asterisk (*) to create
* a wildcard certificate that protects several sites in the same domain.
* For example, *.example.com protects www.example.com, site.example.com,
* and images.example.com.
*
*
* @param domainName
* Fully qualified domain name (FQDN), such as www.example.com, of
* the site you want to secure with an ACM Certificate. Use an
* asterisk (*) to create a wildcard certificate that protects
* several sites in the same domain. For example, *.example.com
* protects www.example.com, site.example.com, and
* images.example.com.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public RequestCertificateRequest withDomainName(String domainName) {
setDomainName(domainName);
return this;
}
/**
*
* Additional FQDNs to be included in the Subject Alternative Name extension
* of the ACM Certificate. For example, add the name www.example.net to a
* certificate for which the DomainName
field is
* www.example.com if users can reach your site by using either name.
*
*
* @return Additional FQDNs to be included in the Subject Alternative Name
* extension of the ACM Certificate. For example, add the name
* www.example.net to a certificate for which the
* DomainName
field is www.example.com if users can
* reach your site by using either name.
*/
public java.util.List getSubjectAlternativeNames() {
return subjectAlternativeNames;
}
/**
*
* Additional FQDNs to be included in the Subject Alternative Name extension
* of the ACM Certificate. For example, add the name www.example.net to a
* certificate for which the DomainName
field is
* www.example.com if users can reach your site by using either name.
*
*
* @param subjectAlternativeNames
* Additional FQDNs to be included in the Subject Alternative Name
* extension of the ACM Certificate. For example, add the name
* www.example.net to a certificate for which the
* DomainName
field is www.example.com if users can
* reach your site by using either name.
*/
public void setSubjectAlternativeNames(
java.util.Collection subjectAlternativeNames) {
if (subjectAlternativeNames == null) {
this.subjectAlternativeNames = null;
return;
}
this.subjectAlternativeNames = new java.util.ArrayList(
subjectAlternativeNames);
}
/**
*
* Additional FQDNs to be included in the Subject Alternative Name extension
* of the ACM Certificate. For example, add the name www.example.net to a
* certificate for which the DomainName
field is
* www.example.com if users can reach your site by using either name.
*
*
* NOTE: This method appends the values to the existing list (if
* any). Use {@link #setSubjectAlternativeNames(java.util.Collection)} or
* {@link #withSubjectAlternativeNames(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param subjectAlternativeNames
* Additional FQDNs to be included in the Subject Alternative Name
* extension of the ACM Certificate. For example, add the name
* www.example.net to a certificate for which the
* DomainName
field is www.example.com if users can
* reach your site by using either name.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public RequestCertificateRequest withSubjectAlternativeNames(
String... subjectAlternativeNames) {
if (this.subjectAlternativeNames == null) {
setSubjectAlternativeNames(new java.util.ArrayList(
subjectAlternativeNames.length));
}
for (String ele : subjectAlternativeNames) {
this.subjectAlternativeNames.add(ele);
}
return this;
}
/**
*
* Additional FQDNs to be included in the Subject Alternative Name extension
* of the ACM Certificate. For example, add the name www.example.net to a
* certificate for which the DomainName
field is
* www.example.com if users can reach your site by using either name.
*
*
* @param subjectAlternativeNames
* Additional FQDNs to be included in the Subject Alternative Name
* extension of the ACM Certificate. For example, add the name
* www.example.net to a certificate for which the
* DomainName
field is www.example.com if users can
* reach your site by using either name.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public RequestCertificateRequest withSubjectAlternativeNames(
java.util.Collection subjectAlternativeNames) {
setSubjectAlternativeNames(subjectAlternativeNames);
return this;
}
/**
*
* Customer chosen string that can be used to distinguish between calls to
* RequestCertificate
. Idempotency tokens time out after one
* hour. Therefore, if you call RequestCertificate
multiple
* times with the same idempotency token within one hour, ACM recognizes
* that you are requesting only one certificate and will issue only one. If
* you change the idempotency token for each call, ACM recognizes that you
* are requesting multiple certificates.
*
*
* @param idempotencyToken
* Customer chosen string that can be used to distinguish between
* calls to RequestCertificate
. Idempotency tokens time
* out after one hour. Therefore, if you call
* RequestCertificate
multiple times with the same
* idempotency token within one hour, ACM recognizes that you are
* requesting only one certificate and will issue only one. If you
* change the idempotency token for each call, ACM recognizes that
* you are requesting multiple certificates.
*/
public void setIdempotencyToken(String idempotencyToken) {
this.idempotencyToken = idempotencyToken;
}
/**
*
* Customer chosen string that can be used to distinguish between calls to
* RequestCertificate
. Idempotency tokens time out after one
* hour. Therefore, if you call RequestCertificate
multiple
* times with the same idempotency token within one hour, ACM recognizes
* that you are requesting only one certificate and will issue only one. If
* you change the idempotency token for each call, ACM recognizes that you
* are requesting multiple certificates.
*
*
* @return Customer chosen string that can be used to distinguish between
* calls to RequestCertificate
. Idempotency tokens time
* out after one hour. Therefore, if you call
* RequestCertificate
multiple times with the same
* idempotency token within one hour, ACM recognizes that you are
* requesting only one certificate and will issue only one. If you
* change the idempotency token for each call, ACM recognizes that
* you are requesting multiple certificates.
*/
public String getIdempotencyToken() {
return this.idempotencyToken;
}
/**
*
* Customer chosen string that can be used to distinguish between calls to
* RequestCertificate
. Idempotency tokens time out after one
* hour. Therefore, if you call RequestCertificate
multiple
* times with the same idempotency token within one hour, ACM recognizes
* that you are requesting only one certificate and will issue only one. If
* you change the idempotency token for each call, ACM recognizes that you
* are requesting multiple certificates.
*
*
* @param idempotencyToken
* Customer chosen string that can be used to distinguish between
* calls to RequestCertificate
. Idempotency tokens time
* out after one hour. Therefore, if you call
* RequestCertificate
multiple times with the same
* idempotency token within one hour, ACM recognizes that you are
* requesting only one certificate and will issue only one. If you
* change the idempotency token for each call, ACM recognizes that
* you are requesting multiple certificates.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public RequestCertificateRequest withIdempotencyToken(
String idempotencyToken) {
setIdempotencyToken(idempotencyToken);
return this;
}
/**
*
* The base validation domain that will act as the suffix of the email
* addresses that are used to send the emails. This must be the same as the
* Domain
value or a superdomain of the Domain
* value. For example, if you requested a certificate for
* test.example.com
and specify DomainValidationOptions
* of example.com
, ACM sends email to the domain registrant,
* technical contact, and administrative contact in WHOIS and the following
* five addresses:
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @return The base validation domain that will act as the suffix of the
* email addresses that are used to send the emails. This must be
* the same as the Domain
value or a superdomain of the
* Domain
value. For example, if you requested a
* certificate for test.example.com
and specify
* DomainValidationOptions of example.com
, ACM
* sends email to the domain registrant, technical contact, and
* administrative contact in WHOIS and the following five
* addresses:
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
*/
public java.util.List getDomainValidationOptions() {
return domainValidationOptions;
}
/**
*
* The base validation domain that will act as the suffix of the email
* addresses that are used to send the emails. This must be the same as the
* Domain
value or a superdomain of the Domain
* value. For example, if you requested a certificate for
* test.example.com
and specify DomainValidationOptions
* of example.com
, ACM sends email to the domain registrant,
* technical contact, and administrative contact in WHOIS and the following
* five addresses:
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @param domainValidationOptions
* The base validation domain that will act as the suffix of the
* email addresses that are used to send the emails. This must be the
* same as the Domain
value or a superdomain of the
* Domain
value. For example, if you requested a
* certificate for test.example.com
and specify
* DomainValidationOptions of example.com
, ACM
* sends email to the domain registrant, technical contact, and
* administrative contact in WHOIS and the following five
* addresses:
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
*/
public void setDomainValidationOptions(
java.util.Collection domainValidationOptions) {
if (domainValidationOptions == null) {
this.domainValidationOptions = null;
return;
}
this.domainValidationOptions = new java.util.ArrayList(
domainValidationOptions);
}
/**
*
* The base validation domain that will act as the suffix of the email
* addresses that are used to send the emails. This must be the same as the
* Domain
value or a superdomain of the Domain
* value. For example, if you requested a certificate for
* test.example.com
and specify DomainValidationOptions
* of example.com
, ACM sends email to the domain registrant,
* technical contact, and administrative contact in WHOIS and the following
* five addresses:
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* NOTE: This method appends the values to the existing list (if
* any). Use {@link #setDomainValidationOptions(java.util.Collection)} or
* {@link #withDomainValidationOptions(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param domainValidationOptions
* The base validation domain that will act as the suffix of the
* email addresses that are used to send the emails. This must be the
* same as the Domain
value or a superdomain of the
* Domain
value. For example, if you requested a
* certificate for test.example.com
and specify
* DomainValidationOptions of example.com
, ACM
* sends email to the domain registrant, technical contact, and
* administrative contact in WHOIS and the following five
* addresses:
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public RequestCertificateRequest withDomainValidationOptions(
DomainValidationOption... domainValidationOptions) {
if (this.domainValidationOptions == null) {
setDomainValidationOptions(new java.util.ArrayList(
domainValidationOptions.length));
}
for (DomainValidationOption ele : domainValidationOptions) {
this.domainValidationOptions.add(ele);
}
return this;
}
/**
*
* The base validation domain that will act as the suffix of the email
* addresses that are used to send the emails. This must be the same as the
* Domain
value or a superdomain of the Domain
* value. For example, if you requested a certificate for
* test.example.com
and specify DomainValidationOptions
* of example.com
, ACM sends email to the domain registrant,
* technical contact, and administrative contact in WHOIS and the following
* five addresses:
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @param domainValidationOptions
* The base validation domain that will act as the suffix of the
* email addresses that are used to send the emails. This must be the
* same as the Domain
value or a superdomain of the
* Domain
value. For example, if you requested a
* certificate for test.example.com
and specify
* DomainValidationOptions of example.com
, ACM
* sends email to the domain registrant, technical contact, and
* administrative contact in WHOIS and the following five
* addresses:
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public RequestCertificateRequest withDomainValidationOptions(
java.util.Collection domainValidationOptions) {
setDomainValidationOptions(domainValidationOptions);
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getDomainName() != null)
sb.append("DomainName: " + getDomainName() + ",");
if (getSubjectAlternativeNames() != null)
sb.append("SubjectAlternativeNames: "
+ getSubjectAlternativeNames() + ",");
if (getIdempotencyToken() != null)
sb.append("IdempotencyToken: " + getIdempotencyToken() + ",");
if (getDomainValidationOptions() != null)
sb.append("DomainValidationOptions: "
+ getDomainValidationOptions());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof RequestCertificateRequest == false)
return false;
RequestCertificateRequest other = (RequestCertificateRequest) obj;
if (other.getDomainName() == null ^ this.getDomainName() == null)
return false;
if (other.getDomainName() != null
&& other.getDomainName().equals(this.getDomainName()) == false)
return false;
if (other.getSubjectAlternativeNames() == null
^ this.getSubjectAlternativeNames() == null)
return false;
if (other.getSubjectAlternativeNames() != null
&& other.getSubjectAlternativeNames().equals(
this.getSubjectAlternativeNames()) == false)
return false;
if (other.getIdempotencyToken() == null
^ this.getIdempotencyToken() == null)
return false;
if (other.getIdempotencyToken() != null
&& other.getIdempotencyToken().equals(
this.getIdempotencyToken()) == false)
return false;
if (other.getDomainValidationOptions() == null
^ this.getDomainValidationOptions() == null)
return false;
if (other.getDomainValidationOptions() != null
&& other.getDomainValidationOptions().equals(
this.getDomainValidationOptions()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode
+ ((getDomainName() == null) ? 0 : getDomainName().hashCode());
hashCode = prime
* hashCode
+ ((getSubjectAlternativeNames() == null) ? 0
: getSubjectAlternativeNames().hashCode());
hashCode = prime
* hashCode
+ ((getIdempotencyToken() == null) ? 0 : getIdempotencyToken()
.hashCode());
hashCode = prime
* hashCode
+ ((getDomainValidationOptions() == null) ? 0
: getDomainValidationOptions().hashCode());
return hashCode;
}
@Override
public RequestCertificateRequest clone() {
return (RequestCertificateRequest) super.clone();
}
}