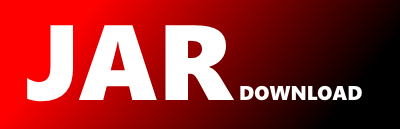
com.amazonaws.services.acmpca.model.CrlConfiguration Maven / Gradle / Ivy
Show all versions of aws-java-sdk-acmpca Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.acmpca.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Contains configuration information for a certificate revocation list (CRL). Your private certificate authority (CA)
* creates base CRLs. Delta CRLs are not supported. You can enable CRLs for your new or an existing private CA by
* setting the Enabled parameter to true
. Your private CA writes CRLs to an S3 bucket that you
* specify in the S3BucketName parameter. You can hide the name of your bucket by specifying a value for the
* CustomCname parameter. Your private CA by default copies the CNAME or the S3 bucket name to the CRL
* Distribution Points extension of each certificate it issues. If you want to configure this default behavior to be
* something different, you can set the CrlDistributionPointExtensionConfiguration parameter. Your S3 bucket
* policy must give write permission to Amazon Web Services Private CA.
*
*
* Amazon Web Services Private CA assets that are stored in Amazon S3 can be protected with encryption. For more
* information, see Encrypting Your
* CRLs.
*
*
* Your private CA uses the value in the ExpirationInDays parameter to calculate the nextUpdate field in
* the CRL. The CRL is refreshed prior to a certificate's expiration date or when a certificate is revoked. When a
* certificate is revoked, it appears in the CRL until the certificate expires, and then in one additional CRL after
* expiration, and it always appears in the audit report.
*
*
* A CRL is typically updated approximately 30 minutes after a certificate is revoked. If for any reason a CRL update
* fails, Amazon Web Services Private CA makes further attempts every 15 minutes.
*
*
* CRLs contain the following fields:
*
*
* -
*
* Version: The current version number defined in RFC 5280 is V2. The integer value is 0x1.
*
*
* -
*
* Signature Algorithm: The name of the algorithm used to sign the CRL.
*
*
* -
*
* Issuer: The X.500 distinguished name of your private CA that issued the CRL.
*
*
* -
*
* Last Update: The issue date and time of this CRL.
*
*
* -
*
* Next Update: The day and time by which the next CRL will be issued.
*
*
* -
*
* Revoked Certificates: List of revoked certificates. Each list item contains the following information.
*
*
* -
*
* Serial Number: The serial number, in hexadecimal format, of the revoked certificate.
*
*
* -
*
* Revocation Date: Date and time the certificate was revoked.
*
*
* -
*
* CRL Entry Extensions: Optional extensions for the CRL entry.
*
*
* -
*
* X509v3 CRL Reason Code: Reason the certificate was revoked.
*
*
*
*
*
*
* -
*
* CRL Extensions: Optional extensions for the CRL.
*
*
* -
*
* X509v3 Authority Key Identifier: Identifies the public key associated with the private key used to sign the
* certificate.
*
*
* -
*
* X509v3 CRL Number:: Decimal sequence number for the CRL.
*
*
*
*
* -
*
* Signature Algorithm: Algorithm used by your private CA to sign the CRL.
*
*
* -
*
* Signature Value: Signature computed over the CRL.
*
*
*
*
* Certificate revocation lists created by Amazon Web Services Private CA are DER-encoded. You can use the following
* OpenSSL command to list a CRL.
*
*
* openssl crl -inform DER -text -in crl_path -noout
*
*
* For more information, see Planning
* a certificate revocation list (CRL) in the Amazon Web Services Private Certificate Authority User Guide
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CrlConfiguration implements Serializable, Cloneable, StructuredPojo {
/**
*
* Boolean value that specifies whether certificate revocation lists (CRLs) are enabled. You can use this value to
* enable certificate revocation for a new CA when you call the CreateCertificateAuthority action or for an existing CA when you call the UpdateCertificateAuthority action.
*
*/
private Boolean enabled;
/**
*
* Validity period of the CRL in days.
*
*/
private Integer expirationInDays;
/**
*
* Name inserted into the certificate CRL Distribution Points extension that enables the use of an alias for
* the CRL distribution point. Use this value if you don't want the name of your S3 bucket to be public.
*
*
*
* The content of a Canonical Name (CNAME) record must conform to RFC2396 restrictions on the use of special characters in URIs.
* Additionally, the value of the CNAME must not include a protocol prefix such as "http://" or "https://".
*
*
*/
private String customCname;
/**
*
* Name of the S3 bucket that contains the CRL. If you do not provide a value for the CustomCname argument,
* the name of your S3 bucket is placed into the CRL Distribution Points extension of the issued certificate.
* You can change the name of your bucket by calling the UpdateCertificateAuthority operation. You must specify a bucket policy that
* allows Amazon Web Services Private CA to write the CRL to your bucket.
*
*
*
* The S3BucketName
parameter must conform to the S3 bucket naming rules.
*
*
*/
private String s3BucketName;
/**
*
* Determines whether the CRL will be publicly readable or privately held in the CRL Amazon S3 bucket. If you choose
* PUBLIC_READ, the CRL will be accessible over the public internet. If you choose BUCKET_OWNER_FULL_CONTROL, only
* the owner of the CRL S3 bucket can access the CRL, and your PKI clients may need an alternative method of access.
*
*
* If no value is specified, the default is PUBLIC_READ
.
*
*
* Note: This default can cause CA creation to fail in some circumstances. If you have have enabled the Block
* Public Access (BPA) feature in your S3 account, then you must specify the value of this parameter as
* BUCKET_OWNER_FULL_CONTROL
, and not doing so results in an error. If you have disabled BPA in S3,
* then you can specify either BUCKET_OWNER_FULL_CONTROL
or PUBLIC_READ
as the value.
*
*
* For more information, see Blocking public access to
* the S3 bucket.
*
*/
private String s3ObjectAcl;
/**
*
* Configures the behavior of the CRL Distribution Point extension for certificates issued by your certificate
* authority. If this field is not provided, then the CRl Distribution Point Extension will be present and contain
* the default CRL URL.
*
*/
private CrlDistributionPointExtensionConfiguration crlDistributionPointExtensionConfiguration;
/**
*
* Boolean value that specifies whether certificate revocation lists (CRLs) are enabled. You can use this value to
* enable certificate revocation for a new CA when you call the CreateCertificateAuthority action or for an existing CA when you call the UpdateCertificateAuthority action.
*
*
* @param enabled
* Boolean value that specifies whether certificate revocation lists (CRLs) are enabled. You can use this
* value to enable certificate revocation for a new CA when you call the CreateCertificateAuthority action or for an existing CA when you call the UpdateCertificateAuthority action.
*/
public void setEnabled(Boolean enabled) {
this.enabled = enabled;
}
/**
*
* Boolean value that specifies whether certificate revocation lists (CRLs) are enabled. You can use this value to
* enable certificate revocation for a new CA when you call the CreateCertificateAuthority action or for an existing CA when you call the UpdateCertificateAuthority action.
*
*
* @return Boolean value that specifies whether certificate revocation lists (CRLs) are enabled. You can use this
* value to enable certificate revocation for a new CA when you call the CreateCertificateAuthority action or for an existing CA when you call the UpdateCertificateAuthority action.
*/
public Boolean getEnabled() {
return this.enabled;
}
/**
*
* Boolean value that specifies whether certificate revocation lists (CRLs) are enabled. You can use this value to
* enable certificate revocation for a new CA when you call the CreateCertificateAuthority action or for an existing CA when you call the UpdateCertificateAuthority action.
*
*
* @param enabled
* Boolean value that specifies whether certificate revocation lists (CRLs) are enabled. You can use this
* value to enable certificate revocation for a new CA when you call the CreateCertificateAuthority action or for an existing CA when you call the UpdateCertificateAuthority action.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CrlConfiguration withEnabled(Boolean enabled) {
setEnabled(enabled);
return this;
}
/**
*
* Boolean value that specifies whether certificate revocation lists (CRLs) are enabled. You can use this value to
* enable certificate revocation for a new CA when you call the CreateCertificateAuthority action or for an existing CA when you call the UpdateCertificateAuthority action.
*
*
* @return Boolean value that specifies whether certificate revocation lists (CRLs) are enabled. You can use this
* value to enable certificate revocation for a new CA when you call the CreateCertificateAuthority action or for an existing CA when you call the UpdateCertificateAuthority action.
*/
public Boolean isEnabled() {
return this.enabled;
}
/**
*
* Validity period of the CRL in days.
*
*
* @param expirationInDays
* Validity period of the CRL in days.
*/
public void setExpirationInDays(Integer expirationInDays) {
this.expirationInDays = expirationInDays;
}
/**
*
* Validity period of the CRL in days.
*
*
* @return Validity period of the CRL in days.
*/
public Integer getExpirationInDays() {
return this.expirationInDays;
}
/**
*
* Validity period of the CRL in days.
*
*
* @param expirationInDays
* Validity period of the CRL in days.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CrlConfiguration withExpirationInDays(Integer expirationInDays) {
setExpirationInDays(expirationInDays);
return this;
}
/**
*
* Name inserted into the certificate CRL Distribution Points extension that enables the use of an alias for
* the CRL distribution point. Use this value if you don't want the name of your S3 bucket to be public.
*
*
*
* The content of a Canonical Name (CNAME) record must conform to RFC2396 restrictions on the use of special characters in URIs.
* Additionally, the value of the CNAME must not include a protocol prefix such as "http://" or "https://".
*
*
*
* @param customCname
* Name inserted into the certificate CRL Distribution Points extension that enables the use of an
* alias for the CRL distribution point. Use this value if you don't want the name of your S3 bucket to be
* public.
*
* The content of a Canonical Name (CNAME) record must conform to RFC2396 restrictions on the use of special characters in
* URIs. Additionally, the value of the CNAME must not include a protocol prefix such as "http://" or
* "https://".
*
*/
public void setCustomCname(String customCname) {
this.customCname = customCname;
}
/**
*
* Name inserted into the certificate CRL Distribution Points extension that enables the use of an alias for
* the CRL distribution point. Use this value if you don't want the name of your S3 bucket to be public.
*
*
*
* The content of a Canonical Name (CNAME) record must conform to RFC2396 restrictions on the use of special characters in URIs.
* Additionally, the value of the CNAME must not include a protocol prefix such as "http://" or "https://".
*
*
*
* @return Name inserted into the certificate CRL Distribution Points extension that enables the use of an
* alias for the CRL distribution point. Use this value if you don't want the name of your S3 bucket to be
* public.
*
* The content of a Canonical Name (CNAME) record must conform to RFC2396 restrictions on the use of special characters in
* URIs. Additionally, the value of the CNAME must not include a protocol prefix such as "http://" or
* "https://".
*
*/
public String getCustomCname() {
return this.customCname;
}
/**
*
* Name inserted into the certificate CRL Distribution Points extension that enables the use of an alias for
* the CRL distribution point. Use this value if you don't want the name of your S3 bucket to be public.
*
*
*
* The content of a Canonical Name (CNAME) record must conform to RFC2396 restrictions on the use of special characters in URIs.
* Additionally, the value of the CNAME must not include a protocol prefix such as "http://" or "https://".
*
*
*
* @param customCname
* Name inserted into the certificate CRL Distribution Points extension that enables the use of an
* alias for the CRL distribution point. Use this value if you don't want the name of your S3 bucket to be
* public.
*
* The content of a Canonical Name (CNAME) record must conform to RFC2396 restrictions on the use of special characters in
* URIs. Additionally, the value of the CNAME must not include a protocol prefix such as "http://" or
* "https://".
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CrlConfiguration withCustomCname(String customCname) {
setCustomCname(customCname);
return this;
}
/**
*
* Name of the S3 bucket that contains the CRL. If you do not provide a value for the CustomCname argument,
* the name of your S3 bucket is placed into the CRL Distribution Points extension of the issued certificate.
* You can change the name of your bucket by calling the UpdateCertificateAuthority operation. You must specify a bucket policy that
* allows Amazon Web Services Private CA to write the CRL to your bucket.
*
*
*
* The S3BucketName
parameter must conform to the S3 bucket naming rules.
*
*
*
* @param s3BucketName
* Name of the S3 bucket that contains the CRL. If you do not provide a value for the CustomCname
* argument, the name of your S3 bucket is placed into the CRL Distribution Points extension of the
* issued certificate. You can change the name of your bucket by calling the UpdateCertificateAuthority operation. You must specify a bucket
* policy that allows Amazon Web Services Private CA to write the CRL to your bucket.
*
* The S3BucketName
parameter must conform to the S3 bucket naming
* rules.
*
*/
public void setS3BucketName(String s3BucketName) {
this.s3BucketName = s3BucketName;
}
/**
*
* Name of the S3 bucket that contains the CRL. If you do not provide a value for the CustomCname argument,
* the name of your S3 bucket is placed into the CRL Distribution Points extension of the issued certificate.
* You can change the name of your bucket by calling the UpdateCertificateAuthority operation. You must specify a bucket policy that
* allows Amazon Web Services Private CA to write the CRL to your bucket.
*
*
*
* The S3BucketName
parameter must conform to the S3 bucket naming rules.
*
*
*
* @return Name of the S3 bucket that contains the CRL. If you do not provide a value for the CustomCname
* argument, the name of your S3 bucket is placed into the CRL Distribution Points extension of the
* issued certificate. You can change the name of your bucket by calling the UpdateCertificateAuthority operation. You must specify a bucket
* policy that allows Amazon Web Services Private CA to write the CRL to your bucket.
*
* The S3BucketName
parameter must conform to the S3 bucket naming
* rules.
*
*/
public String getS3BucketName() {
return this.s3BucketName;
}
/**
*
* Name of the S3 bucket that contains the CRL. If you do not provide a value for the CustomCname argument,
* the name of your S3 bucket is placed into the CRL Distribution Points extension of the issued certificate.
* You can change the name of your bucket by calling the UpdateCertificateAuthority operation. You must specify a bucket policy that
* allows Amazon Web Services Private CA to write the CRL to your bucket.
*
*
*
* The S3BucketName
parameter must conform to the S3 bucket naming rules.
*
*
*
* @param s3BucketName
* Name of the S3 bucket that contains the CRL. If you do not provide a value for the CustomCname
* argument, the name of your S3 bucket is placed into the CRL Distribution Points extension of the
* issued certificate. You can change the name of your bucket by calling the UpdateCertificateAuthority operation. You must specify a bucket
* policy that allows Amazon Web Services Private CA to write the CRL to your bucket.
*
* The S3BucketName
parameter must conform to the S3 bucket naming
* rules.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CrlConfiguration withS3BucketName(String s3BucketName) {
setS3BucketName(s3BucketName);
return this;
}
/**
*
* Determines whether the CRL will be publicly readable or privately held in the CRL Amazon S3 bucket. If you choose
* PUBLIC_READ, the CRL will be accessible over the public internet. If you choose BUCKET_OWNER_FULL_CONTROL, only
* the owner of the CRL S3 bucket can access the CRL, and your PKI clients may need an alternative method of access.
*
*
* If no value is specified, the default is PUBLIC_READ
.
*
*
* Note: This default can cause CA creation to fail in some circumstances. If you have have enabled the Block
* Public Access (BPA) feature in your S3 account, then you must specify the value of this parameter as
* BUCKET_OWNER_FULL_CONTROL
, and not doing so results in an error. If you have disabled BPA in S3,
* then you can specify either BUCKET_OWNER_FULL_CONTROL
or PUBLIC_READ
as the value.
*
*
* For more information, see Blocking public access to
* the S3 bucket.
*
*
* @param s3ObjectAcl
* Determines whether the CRL will be publicly readable or privately held in the CRL Amazon S3 bucket. If you
* choose PUBLIC_READ, the CRL will be accessible over the public internet. If you choose
* BUCKET_OWNER_FULL_CONTROL, only the owner of the CRL S3 bucket can access the CRL, and your PKI clients
* may need an alternative method of access.
*
* If no value is specified, the default is PUBLIC_READ
.
*
*
* Note: This default can cause CA creation to fail in some circumstances. If you have have enabled
* the Block Public Access (BPA) feature in your S3 account, then you must specify the value of this
* parameter as BUCKET_OWNER_FULL_CONTROL
, and not doing so results in an error. If you have
* disabled BPA in S3, then you can specify either BUCKET_OWNER_FULL_CONTROL
or
* PUBLIC_READ
as the value.
*
*
* For more information, see Blocking public
* access to the S3 bucket.
* @see S3ObjectAcl
*/
public void setS3ObjectAcl(String s3ObjectAcl) {
this.s3ObjectAcl = s3ObjectAcl;
}
/**
*
* Determines whether the CRL will be publicly readable or privately held in the CRL Amazon S3 bucket. If you choose
* PUBLIC_READ, the CRL will be accessible over the public internet. If you choose BUCKET_OWNER_FULL_CONTROL, only
* the owner of the CRL S3 bucket can access the CRL, and your PKI clients may need an alternative method of access.
*
*
* If no value is specified, the default is PUBLIC_READ
.
*
*
* Note: This default can cause CA creation to fail in some circumstances. If you have have enabled the Block
* Public Access (BPA) feature in your S3 account, then you must specify the value of this parameter as
* BUCKET_OWNER_FULL_CONTROL
, and not doing so results in an error. If you have disabled BPA in S3,
* then you can specify either BUCKET_OWNER_FULL_CONTROL
or PUBLIC_READ
as the value.
*
*
* For more information, see Blocking public access to
* the S3 bucket.
*
*
* @return Determines whether the CRL will be publicly readable or privately held in the CRL Amazon S3 bucket. If
* you choose PUBLIC_READ, the CRL will be accessible over the public internet. If you choose
* BUCKET_OWNER_FULL_CONTROL, only the owner of the CRL S3 bucket can access the CRL, and your PKI clients
* may need an alternative method of access.
*
* If no value is specified, the default is PUBLIC_READ
.
*
*
* Note: This default can cause CA creation to fail in some circumstances. If you have have enabled
* the Block Public Access (BPA) feature in your S3 account, then you must specify the value of this
* parameter as BUCKET_OWNER_FULL_CONTROL
, and not doing so results in an error. If you have
* disabled BPA in S3, then you can specify either BUCKET_OWNER_FULL_CONTROL
or
* PUBLIC_READ
as the value.
*
*
* For more information, see Blocking public
* access to the S3 bucket.
* @see S3ObjectAcl
*/
public String getS3ObjectAcl() {
return this.s3ObjectAcl;
}
/**
*
* Determines whether the CRL will be publicly readable or privately held in the CRL Amazon S3 bucket. If you choose
* PUBLIC_READ, the CRL will be accessible over the public internet. If you choose BUCKET_OWNER_FULL_CONTROL, only
* the owner of the CRL S3 bucket can access the CRL, and your PKI clients may need an alternative method of access.
*
*
* If no value is specified, the default is PUBLIC_READ
.
*
*
* Note: This default can cause CA creation to fail in some circumstances. If you have have enabled the Block
* Public Access (BPA) feature in your S3 account, then you must specify the value of this parameter as
* BUCKET_OWNER_FULL_CONTROL
, and not doing so results in an error. If you have disabled BPA in S3,
* then you can specify either BUCKET_OWNER_FULL_CONTROL
or PUBLIC_READ
as the value.
*
*
* For more information, see Blocking public access to
* the S3 bucket.
*
*
* @param s3ObjectAcl
* Determines whether the CRL will be publicly readable or privately held in the CRL Amazon S3 bucket. If you
* choose PUBLIC_READ, the CRL will be accessible over the public internet. If you choose
* BUCKET_OWNER_FULL_CONTROL, only the owner of the CRL S3 bucket can access the CRL, and your PKI clients
* may need an alternative method of access.
*
* If no value is specified, the default is PUBLIC_READ
.
*
*
* Note: This default can cause CA creation to fail in some circumstances. If you have have enabled
* the Block Public Access (BPA) feature in your S3 account, then you must specify the value of this
* parameter as BUCKET_OWNER_FULL_CONTROL
, and not doing so results in an error. If you have
* disabled BPA in S3, then you can specify either BUCKET_OWNER_FULL_CONTROL
or
* PUBLIC_READ
as the value.
*
*
* For more information, see Blocking public
* access to the S3 bucket.
* @return Returns a reference to this object so that method calls can be chained together.
* @see S3ObjectAcl
*/
public CrlConfiguration withS3ObjectAcl(String s3ObjectAcl) {
setS3ObjectAcl(s3ObjectAcl);
return this;
}
/**
*
* Determines whether the CRL will be publicly readable or privately held in the CRL Amazon S3 bucket. If you choose
* PUBLIC_READ, the CRL will be accessible over the public internet. If you choose BUCKET_OWNER_FULL_CONTROL, only
* the owner of the CRL S3 bucket can access the CRL, and your PKI clients may need an alternative method of access.
*
*
* If no value is specified, the default is PUBLIC_READ
.
*
*
* Note: This default can cause CA creation to fail in some circumstances. If you have have enabled the Block
* Public Access (BPA) feature in your S3 account, then you must specify the value of this parameter as
* BUCKET_OWNER_FULL_CONTROL
, and not doing so results in an error. If you have disabled BPA in S3,
* then you can specify either BUCKET_OWNER_FULL_CONTROL
or PUBLIC_READ
as the value.
*
*
* For more information, see Blocking public access to
* the S3 bucket.
*
*
* @param s3ObjectAcl
* Determines whether the CRL will be publicly readable or privately held in the CRL Amazon S3 bucket. If you
* choose PUBLIC_READ, the CRL will be accessible over the public internet. If you choose
* BUCKET_OWNER_FULL_CONTROL, only the owner of the CRL S3 bucket can access the CRL, and your PKI clients
* may need an alternative method of access.
*
* If no value is specified, the default is PUBLIC_READ
.
*
*
* Note: This default can cause CA creation to fail in some circumstances. If you have have enabled
* the Block Public Access (BPA) feature in your S3 account, then you must specify the value of this
* parameter as BUCKET_OWNER_FULL_CONTROL
, and not doing so results in an error. If you have
* disabled BPA in S3, then you can specify either BUCKET_OWNER_FULL_CONTROL
or
* PUBLIC_READ
as the value.
*
*
* For more information, see Blocking public
* access to the S3 bucket.
* @return Returns a reference to this object so that method calls can be chained together.
* @see S3ObjectAcl
*/
public CrlConfiguration withS3ObjectAcl(S3ObjectAcl s3ObjectAcl) {
this.s3ObjectAcl = s3ObjectAcl.toString();
return this;
}
/**
*
* Configures the behavior of the CRL Distribution Point extension for certificates issued by your certificate
* authority. If this field is not provided, then the CRl Distribution Point Extension will be present and contain
* the default CRL URL.
*
*
* @param crlDistributionPointExtensionConfiguration
* Configures the behavior of the CRL Distribution Point extension for certificates issued by your
* certificate authority. If this field is not provided, then the CRl Distribution Point Extension will be
* present and contain the default CRL URL.
*/
public void setCrlDistributionPointExtensionConfiguration(CrlDistributionPointExtensionConfiguration crlDistributionPointExtensionConfiguration) {
this.crlDistributionPointExtensionConfiguration = crlDistributionPointExtensionConfiguration;
}
/**
*
* Configures the behavior of the CRL Distribution Point extension for certificates issued by your certificate
* authority. If this field is not provided, then the CRl Distribution Point Extension will be present and contain
* the default CRL URL.
*
*
* @return Configures the behavior of the CRL Distribution Point extension for certificates issued by your
* certificate authority. If this field is not provided, then the CRl Distribution Point Extension will be
* present and contain the default CRL URL.
*/
public CrlDistributionPointExtensionConfiguration getCrlDistributionPointExtensionConfiguration() {
return this.crlDistributionPointExtensionConfiguration;
}
/**
*
* Configures the behavior of the CRL Distribution Point extension for certificates issued by your certificate
* authority. If this field is not provided, then the CRl Distribution Point Extension will be present and contain
* the default CRL URL.
*
*
* @param crlDistributionPointExtensionConfiguration
* Configures the behavior of the CRL Distribution Point extension for certificates issued by your
* certificate authority. If this field is not provided, then the CRl Distribution Point Extension will be
* present and contain the default CRL URL.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CrlConfiguration withCrlDistributionPointExtensionConfiguration(CrlDistributionPointExtensionConfiguration crlDistributionPointExtensionConfiguration) {
setCrlDistributionPointExtensionConfiguration(crlDistributionPointExtensionConfiguration);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getEnabled() != null)
sb.append("Enabled: ").append(getEnabled()).append(",");
if (getExpirationInDays() != null)
sb.append("ExpirationInDays: ").append(getExpirationInDays()).append(",");
if (getCustomCname() != null)
sb.append("CustomCname: ").append(getCustomCname()).append(",");
if (getS3BucketName() != null)
sb.append("S3BucketName: ").append(getS3BucketName()).append(",");
if (getS3ObjectAcl() != null)
sb.append("S3ObjectAcl: ").append(getS3ObjectAcl()).append(",");
if (getCrlDistributionPointExtensionConfiguration() != null)
sb.append("CrlDistributionPointExtensionConfiguration: ").append(getCrlDistributionPointExtensionConfiguration());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CrlConfiguration == false)
return false;
CrlConfiguration other = (CrlConfiguration) obj;
if (other.getEnabled() == null ^ this.getEnabled() == null)
return false;
if (other.getEnabled() != null && other.getEnabled().equals(this.getEnabled()) == false)
return false;
if (other.getExpirationInDays() == null ^ this.getExpirationInDays() == null)
return false;
if (other.getExpirationInDays() != null && other.getExpirationInDays().equals(this.getExpirationInDays()) == false)
return false;
if (other.getCustomCname() == null ^ this.getCustomCname() == null)
return false;
if (other.getCustomCname() != null && other.getCustomCname().equals(this.getCustomCname()) == false)
return false;
if (other.getS3BucketName() == null ^ this.getS3BucketName() == null)
return false;
if (other.getS3BucketName() != null && other.getS3BucketName().equals(this.getS3BucketName()) == false)
return false;
if (other.getS3ObjectAcl() == null ^ this.getS3ObjectAcl() == null)
return false;
if (other.getS3ObjectAcl() != null && other.getS3ObjectAcl().equals(this.getS3ObjectAcl()) == false)
return false;
if (other.getCrlDistributionPointExtensionConfiguration() == null ^ this.getCrlDistributionPointExtensionConfiguration() == null)
return false;
if (other.getCrlDistributionPointExtensionConfiguration() != null
&& other.getCrlDistributionPointExtensionConfiguration().equals(this.getCrlDistributionPointExtensionConfiguration()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getEnabled() == null) ? 0 : getEnabled().hashCode());
hashCode = prime * hashCode + ((getExpirationInDays() == null) ? 0 : getExpirationInDays().hashCode());
hashCode = prime * hashCode + ((getCustomCname() == null) ? 0 : getCustomCname().hashCode());
hashCode = prime * hashCode + ((getS3BucketName() == null) ? 0 : getS3BucketName().hashCode());
hashCode = prime * hashCode + ((getS3ObjectAcl() == null) ? 0 : getS3ObjectAcl().hashCode());
hashCode = prime * hashCode
+ ((getCrlDistributionPointExtensionConfiguration() == null) ? 0 : getCrlDistributionPointExtensionConfiguration().hashCode());
return hashCode;
}
@Override
public CrlConfiguration clone() {
try {
return (CrlConfiguration) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.acmpca.model.transform.CrlConfigurationMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}