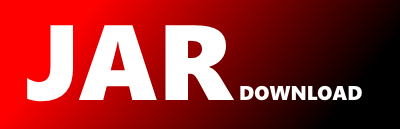
com.amazonaws.services.acmpca.model.CsrExtensions Maven / Gradle / Ivy
Show all versions of aws-java-sdk-acmpca Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.acmpca.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Describes the certificate extensions to be added to the certificate signing request (CSR).
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CsrExtensions implements Serializable, Cloneable, StructuredPojo {
/**
*
* Indicates the purpose of the certificate and of the key contained in the certificate.
*
*/
private KeyUsage keyUsage;
/**
*
* For CA certificates, provides a path to additional information pertaining to the CA, such as revocation and
* policy. For more information, see Subject
* Information Access in RFC 5280.
*
*/
private java.util.List subjectInformationAccess;
/**
*
* Indicates the purpose of the certificate and of the key contained in the certificate.
*
*
* @param keyUsage
* Indicates the purpose of the certificate and of the key contained in the certificate.
*/
public void setKeyUsage(KeyUsage keyUsage) {
this.keyUsage = keyUsage;
}
/**
*
* Indicates the purpose of the certificate and of the key contained in the certificate.
*
*
* @return Indicates the purpose of the certificate and of the key contained in the certificate.
*/
public KeyUsage getKeyUsage() {
return this.keyUsage;
}
/**
*
* Indicates the purpose of the certificate and of the key contained in the certificate.
*
*
* @param keyUsage
* Indicates the purpose of the certificate and of the key contained in the certificate.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CsrExtensions withKeyUsage(KeyUsage keyUsage) {
setKeyUsage(keyUsage);
return this;
}
/**
*
* For CA certificates, provides a path to additional information pertaining to the CA, such as revocation and
* policy. For more information, see Subject
* Information Access in RFC 5280.
*
*
* @return For CA certificates, provides a path to additional information pertaining to the CA, such as revocation
* and policy. For more information, see Subject Information Access in
* RFC 5280.
*/
public java.util.List getSubjectInformationAccess() {
return subjectInformationAccess;
}
/**
*
* For CA certificates, provides a path to additional information pertaining to the CA, such as revocation and
* policy. For more information, see Subject
* Information Access in RFC 5280.
*
*
* @param subjectInformationAccess
* For CA certificates, provides a path to additional information pertaining to the CA, such as revocation
* and policy. For more information, see Subject Information Access in RFC
* 5280.
*/
public void setSubjectInformationAccess(java.util.Collection subjectInformationAccess) {
if (subjectInformationAccess == null) {
this.subjectInformationAccess = null;
return;
}
this.subjectInformationAccess = new java.util.ArrayList(subjectInformationAccess);
}
/**
*
* For CA certificates, provides a path to additional information pertaining to the CA, such as revocation and
* policy. For more information, see Subject
* Information Access in RFC 5280.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSubjectInformationAccess(java.util.Collection)} or
* {@link #withSubjectInformationAccess(java.util.Collection)} if you want to override the existing values.
*
*
* @param subjectInformationAccess
* For CA certificates, provides a path to additional information pertaining to the CA, such as revocation
* and policy. For more information, see Subject Information Access in RFC
* 5280.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CsrExtensions withSubjectInformationAccess(AccessDescription... subjectInformationAccess) {
if (this.subjectInformationAccess == null) {
setSubjectInformationAccess(new java.util.ArrayList(subjectInformationAccess.length));
}
for (AccessDescription ele : subjectInformationAccess) {
this.subjectInformationAccess.add(ele);
}
return this;
}
/**
*
* For CA certificates, provides a path to additional information pertaining to the CA, such as revocation and
* policy. For more information, see Subject
* Information Access in RFC 5280.
*
*
* @param subjectInformationAccess
* For CA certificates, provides a path to additional information pertaining to the CA, such as revocation
* and policy. For more information, see Subject Information Access in RFC
* 5280.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CsrExtensions withSubjectInformationAccess(java.util.Collection subjectInformationAccess) {
setSubjectInformationAccess(subjectInformationAccess);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getKeyUsage() != null)
sb.append("KeyUsage: ").append(getKeyUsage()).append(",");
if (getSubjectInformationAccess() != null)
sb.append("SubjectInformationAccess: ").append(getSubjectInformationAccess());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CsrExtensions == false)
return false;
CsrExtensions other = (CsrExtensions) obj;
if (other.getKeyUsage() == null ^ this.getKeyUsage() == null)
return false;
if (other.getKeyUsage() != null && other.getKeyUsage().equals(this.getKeyUsage()) == false)
return false;
if (other.getSubjectInformationAccess() == null ^ this.getSubjectInformationAccess() == null)
return false;
if (other.getSubjectInformationAccess() != null && other.getSubjectInformationAccess().equals(this.getSubjectInformationAccess()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getKeyUsage() == null) ? 0 : getKeyUsage().hashCode());
hashCode = prime * hashCode + ((getSubjectInformationAccess() == null) ? 0 : getSubjectInformationAccess().hashCode());
return hashCode;
}
@Override
public CsrExtensions clone() {
try {
return (CsrExtensions) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.acmpca.model.transform.CsrExtensionsMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}