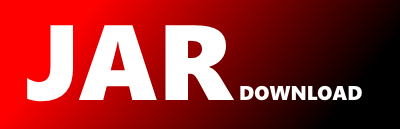
com.amazonaws.services.acmpca.model.ListCertificateAuthoritiesRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-acmpca Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.acmpca.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ListCertificateAuthoritiesRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* Use this parameter when paginating results to specify the maximum number of items to return in the response on
* each page. If additional items exist beyond the number you specify, the NextToken
element is sent in
* the response. Use this NextToken
value in a subsequent request to retrieve additional items.
*
*
* Although the maximum value is 1000, the action only returns a maximum of 100 items.
*
*/
private Integer maxResults;
/**
*
* Use this parameter when paginating results in a subsequent request after you receive a response with truncated
* results. Set it to the value of the NextToken
parameter from the response you just received.
*
*/
private String nextToken;
/**
*
* Use this parameter to filter the returned set of certificate authorities based on their owner. The default is
* SELF.
*
*/
private String resourceOwner;
/**
*
* Use this parameter when paginating results to specify the maximum number of items to return in the response on
* each page. If additional items exist beyond the number you specify, the NextToken
element is sent in
* the response. Use this NextToken
value in a subsequent request to retrieve additional items.
*
*
* Although the maximum value is 1000, the action only returns a maximum of 100 items.
*
*
* @param maxResults
* Use this parameter when paginating results to specify the maximum number of items to return in the
* response on each page. If additional items exist beyond the number you specify, the NextToken
* element is sent in the response. Use this NextToken
value in a subsequent request to retrieve
* additional items.
*
* Although the maximum value is 1000, the action only returns a maximum of 100 items.
*/
public void setMaxResults(Integer maxResults) {
this.maxResults = maxResults;
}
/**
*
* Use this parameter when paginating results to specify the maximum number of items to return in the response on
* each page. If additional items exist beyond the number you specify, the NextToken
element is sent in
* the response. Use this NextToken
value in a subsequent request to retrieve additional items.
*
*
* Although the maximum value is 1000, the action only returns a maximum of 100 items.
*
*
* @return Use this parameter when paginating results to specify the maximum number of items to return in the
* response on each page. If additional items exist beyond the number you specify, the
* NextToken
element is sent in the response. Use this NextToken
value in a
* subsequent request to retrieve additional items.
*
* Although the maximum value is 1000, the action only returns a maximum of 100 items.
*/
public Integer getMaxResults() {
return this.maxResults;
}
/**
*
* Use this parameter when paginating results to specify the maximum number of items to return in the response on
* each page. If additional items exist beyond the number you specify, the NextToken
element is sent in
* the response. Use this NextToken
value in a subsequent request to retrieve additional items.
*
*
* Although the maximum value is 1000, the action only returns a maximum of 100 items.
*
*
* @param maxResults
* Use this parameter when paginating results to specify the maximum number of items to return in the
* response on each page. If additional items exist beyond the number you specify, the NextToken
* element is sent in the response. Use this NextToken
value in a subsequent request to retrieve
* additional items.
*
* Although the maximum value is 1000, the action only returns a maximum of 100 items.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListCertificateAuthoritiesRequest withMaxResults(Integer maxResults) {
setMaxResults(maxResults);
return this;
}
/**
*
* Use this parameter when paginating results in a subsequent request after you receive a response with truncated
* results. Set it to the value of the NextToken
parameter from the response you just received.
*
*
* @param nextToken
* Use this parameter when paginating results in a subsequent request after you receive a response with
* truncated results. Set it to the value of the NextToken
parameter from the response you just
* received.
*/
public void setNextToken(String nextToken) {
this.nextToken = nextToken;
}
/**
*
* Use this parameter when paginating results in a subsequent request after you receive a response with truncated
* results. Set it to the value of the NextToken
parameter from the response you just received.
*
*
* @return Use this parameter when paginating results in a subsequent request after you receive a response with
* truncated results. Set it to the value of the NextToken
parameter from the response you just
* received.
*/
public String getNextToken() {
return this.nextToken;
}
/**
*
* Use this parameter when paginating results in a subsequent request after you receive a response with truncated
* results. Set it to the value of the NextToken
parameter from the response you just received.
*
*
* @param nextToken
* Use this parameter when paginating results in a subsequent request after you receive a response with
* truncated results. Set it to the value of the NextToken
parameter from the response you just
* received.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListCertificateAuthoritiesRequest withNextToken(String nextToken) {
setNextToken(nextToken);
return this;
}
/**
*
* Use this parameter to filter the returned set of certificate authorities based on their owner. The default is
* SELF.
*
*
* @param resourceOwner
* Use this parameter to filter the returned set of certificate authorities based on their owner. The default
* is SELF.
* @see ResourceOwner
*/
public void setResourceOwner(String resourceOwner) {
this.resourceOwner = resourceOwner;
}
/**
*
* Use this parameter to filter the returned set of certificate authorities based on their owner. The default is
* SELF.
*
*
* @return Use this parameter to filter the returned set of certificate authorities based on their owner. The
* default is SELF.
* @see ResourceOwner
*/
public String getResourceOwner() {
return this.resourceOwner;
}
/**
*
* Use this parameter to filter the returned set of certificate authorities based on their owner. The default is
* SELF.
*
*
* @param resourceOwner
* Use this parameter to filter the returned set of certificate authorities based on their owner. The default
* is SELF.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ResourceOwner
*/
public ListCertificateAuthoritiesRequest withResourceOwner(String resourceOwner) {
setResourceOwner(resourceOwner);
return this;
}
/**
*
* Use this parameter to filter the returned set of certificate authorities based on their owner. The default is
* SELF.
*
*
* @param resourceOwner
* Use this parameter to filter the returned set of certificate authorities based on their owner. The default
* is SELF.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ResourceOwner
*/
public ListCertificateAuthoritiesRequest withResourceOwner(ResourceOwner resourceOwner) {
this.resourceOwner = resourceOwner.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getMaxResults() != null)
sb.append("MaxResults: ").append(getMaxResults()).append(",");
if (getNextToken() != null)
sb.append("NextToken: ").append(getNextToken()).append(",");
if (getResourceOwner() != null)
sb.append("ResourceOwner: ").append(getResourceOwner());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ListCertificateAuthoritiesRequest == false)
return false;
ListCertificateAuthoritiesRequest other = (ListCertificateAuthoritiesRequest) obj;
if (other.getMaxResults() == null ^ this.getMaxResults() == null)
return false;
if (other.getMaxResults() != null && other.getMaxResults().equals(this.getMaxResults()) == false)
return false;
if (other.getNextToken() == null ^ this.getNextToken() == null)
return false;
if (other.getNextToken() != null && other.getNextToken().equals(this.getNextToken()) == false)
return false;
if (other.getResourceOwner() == null ^ this.getResourceOwner() == null)
return false;
if (other.getResourceOwner() != null && other.getResourceOwner().equals(this.getResourceOwner()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getMaxResults() == null) ? 0 : getMaxResults().hashCode());
hashCode = prime * hashCode + ((getNextToken() == null) ? 0 : getNextToken().hashCode());
hashCode = prime * hashCode + ((getResourceOwner() == null) ? 0 : getResourceOwner().hashCode());
return hashCode;
}
@Override
public ListCertificateAuthoritiesRequest clone() {
return (ListCertificateAuthoritiesRequest) super.clone();
}
}