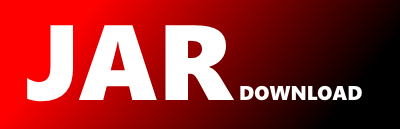
com.amazonaws.services.alexaforbusiness.AmazonAlexaForBusiness Maven / Gradle / Ivy
Show all versions of aws-java-sdk-alexaforbusiness Show documentation
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.alexaforbusiness;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.alexaforbusiness.model.*;
/**
* Interface for accessing Alexa For Business.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.alexaforbusiness.AbstractAmazonAlexaForBusiness} instead.
*
*
*
* Alexa for Business helps you use Alexa in your organization. Alexa for Business provides you with the tools to manage
* Alexa devices, enroll your users, and assign skills, at scale. You can build your own context-aware voice skills
* using the Alexa Skills Kit and the Alexa for Business API operations. You can also make these available as private
* skills for your organization. Alexa for Business makes it efficient to voice-enable your products and services, thus
* providing context-aware voice experiences for your customers. Device makers building with the Alexa Voice Service
* (AVS) can create fully integrated solutions, register their products with Alexa for Business, and manage them as
* shared devices in their organization.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonAlexaForBusiness {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "a4b";
/**
*
* Associates a skill with the organization under the customer's AWS account. If a skill is private, the user
* implicitly accepts access to this skill during enablement.
*
*
* @param approveSkillRequest
* @return Result of the ApproveSkill operation returned by the service.
* @throws LimitExceededException
* You are performing an action that would put you beyond your account's limits.
* @throws NotFoundException
* The resource is not found.
* @throws ConcurrentModificationException
* Concurrent modification of resources. HTTP Status Code: 400.
* @sample AmazonAlexaForBusiness.ApproveSkill
* @see AWS
* API Documentation
*/
ApproveSkillResult approveSkill(ApproveSkillRequest approveSkillRequest);
/**
*
* Associates a contact with a given address book.
*
*
* @param associateContactWithAddressBookRequest
* @return Result of the AssociateContactWithAddressBook operation returned by the service.
* @throws LimitExceededException
* You are performing an action that would put you beyond your account's limits.
* @sample AmazonAlexaForBusiness.AssociateContactWithAddressBook
* @see AWS API Documentation
*/
AssociateContactWithAddressBookResult associateContactWithAddressBook(AssociateContactWithAddressBookRequest associateContactWithAddressBookRequest);
/**
*
* Associates a device with a given room. This applies all the settings from the room profile to the device, and all
* the skills in any skill groups added to that room. This operation requires the device to be online, or else a
* manual sync is required.
*
*
* @param associateDeviceWithRoomRequest
* @return Result of the AssociateDeviceWithRoom operation returned by the service.
* @throws LimitExceededException
* You are performing an action that would put you beyond your account's limits.
* @throws ConcurrentModificationException
* Concurrent modification of resources. HTTP Status Code: 400.
* @throws DeviceNotRegisteredException
* The request failed because this device is no longer registered and therefore no longer managed by this
* account.
* @sample AmazonAlexaForBusiness.AssociateDeviceWithRoom
* @see AWS API Documentation
*/
AssociateDeviceWithRoomResult associateDeviceWithRoom(AssociateDeviceWithRoomRequest associateDeviceWithRoomRequest);
/**
*
* Associates a skill group with a given room. This enables all skills in the associated skill group on all devices
* in the room.
*
*
* @param associateSkillGroupWithRoomRequest
* @return Result of the AssociateSkillGroupWithRoom operation returned by the service.
* @throws ConcurrentModificationException
* Concurrent modification of resources. HTTP Status Code: 400.
* @sample AmazonAlexaForBusiness.AssociateSkillGroupWithRoom
* @see AWS API Documentation
*/
AssociateSkillGroupWithRoomResult associateSkillGroupWithRoom(AssociateSkillGroupWithRoomRequest associateSkillGroupWithRoomRequest);
/**
*
* Associates a skill with a skill group.
*
*
* @param associateSkillWithSkillGroupRequest
* @return Result of the AssociateSkillWithSkillGroup operation returned by the service.
* @throws ConcurrentModificationException
* Concurrent modification of resources. HTTP Status Code: 400.
* @throws NotFoundException
* The resource is not found.
* @throws SkillNotLinkedException
* The skill must be linked to a third-party account.
* @sample AmazonAlexaForBusiness.AssociateSkillWithSkillGroup
* @see AWS API Documentation
*/
AssociateSkillWithSkillGroupResult associateSkillWithSkillGroup(AssociateSkillWithSkillGroupRequest associateSkillWithSkillGroupRequest);
/**
*
* Makes a private skill available for enrolled users to enable on their devices.
*
*
* @param associateSkillWithUsersRequest
* @return Result of the AssociateSkillWithUsers operation returned by the service.
* @throws ConcurrentModificationException
* Concurrent modification of resources. HTTP Status Code: 400.
* @sample AmazonAlexaForBusiness.AssociateSkillWithUsers
* @see AWS API Documentation
*/
AssociateSkillWithUsersResult associateSkillWithUsers(AssociateSkillWithUsersRequest associateSkillWithUsersRequest);
/**
*
* Creates an address book with the specified details.
*
*
* @param createAddressBookRequest
* @return Result of the CreateAddressBook operation returned by the service.
* @throws AlreadyExistsException
* The resource being created already exists.
* @throws LimitExceededException
* You are performing an action that would put you beyond your account's limits.
* @sample AmazonAlexaForBusiness.CreateAddressBook
* @see AWS API Documentation
*/
CreateAddressBookResult createAddressBook(CreateAddressBookRequest createAddressBookRequest);
/**
*
* Creates a recurring schedule for usage reports to deliver to the specified S3 location with a specified daily or
* weekly interval.
*
*
* @param createBusinessReportScheduleRequest
* @return Result of the CreateBusinessReportSchedule operation returned by the service.
* @throws AlreadyExistsException
* The resource being created already exists.
* @sample AmazonAlexaForBusiness.CreateBusinessReportSchedule
* @see AWS API Documentation
*/
CreateBusinessReportScheduleResult createBusinessReportSchedule(CreateBusinessReportScheduleRequest createBusinessReportScheduleRequest);
/**
*
* Adds a new conference provider under the user's AWS account.
*
*
* @param createConferenceProviderRequest
* @return Result of the CreateConferenceProvider operation returned by the service.
* @throws AlreadyExistsException
* The resource being created already exists.
* @sample AmazonAlexaForBusiness.CreateConferenceProvider
* @see AWS API Documentation
*/
CreateConferenceProviderResult createConferenceProvider(CreateConferenceProviderRequest createConferenceProviderRequest);
/**
*
* Creates a contact with the specified details.
*
*
* @param createContactRequest
* @return Result of the CreateContact operation returned by the service.
* @throws AlreadyExistsException
* The resource being created already exists.
* @throws LimitExceededException
* You are performing an action that would put you beyond your account's limits.
* @sample AmazonAlexaForBusiness.CreateContact
* @see AWS
* API Documentation
*/
CreateContactResult createContact(CreateContactRequest createContactRequest);
/**
*
* Creates a new room profile with the specified details.
*
*
* @param createProfileRequest
* @return Result of the CreateProfile operation returned by the service.
* @throws LimitExceededException
* You are performing an action that would put you beyond your account's limits.
* @throws AlreadyExistsException
* The resource being created already exists.
* @throws ConcurrentModificationException
* Concurrent modification of resources. HTTP Status Code: 400.
* @sample AmazonAlexaForBusiness.CreateProfile
* @see AWS
* API Documentation
*/
CreateProfileResult createProfile(CreateProfileRequest createProfileRequest);
/**
*
* Creates a room with the specified details.
*
*
* @param createRoomRequest
* @return Result of the CreateRoom operation returned by the service.
* @throws AlreadyExistsException
* The resource being created already exists.
* @throws LimitExceededException
* You are performing an action that would put you beyond your account's limits.
* @sample AmazonAlexaForBusiness.CreateRoom
* @see AWS
* API Documentation
*/
CreateRoomResult createRoom(CreateRoomRequest createRoomRequest);
/**
*
* Creates a skill group with a specified name and description.
*
*
* @param createSkillGroupRequest
* @return Result of the CreateSkillGroup operation returned by the service.
* @throws AlreadyExistsException
* The resource being created already exists.
* @throws LimitExceededException
* You are performing an action that would put you beyond your account's limits.
* @throws ConcurrentModificationException
* Concurrent modification of resources. HTTP Status Code: 400.
* @sample AmazonAlexaForBusiness.CreateSkillGroup
* @see AWS API Documentation
*/
CreateSkillGroupResult createSkillGroup(CreateSkillGroupRequest createSkillGroupRequest);
/**
*
* Creates a user.
*
*
* @param createUserRequest
* @return Result of the CreateUser operation returned by the service.
* @throws ResourceInUseException
* The resource in the request is already in use.
* @throws LimitExceededException
* You are performing an action that would put you beyond your account's limits.
* @throws ConcurrentModificationException
* Concurrent modification of resources. HTTP Status Code: 400.
* @sample AmazonAlexaForBusiness.CreateUser
* @see AWS
* API Documentation
*/
CreateUserResult createUser(CreateUserRequest createUserRequest);
/**
*
* Deletes an address book by the address book ARN.
*
*
* @param deleteAddressBookRequest
* @return Result of the DeleteAddressBook operation returned by the service.
* @throws NotFoundException
* The resource is not found.
* @throws ConcurrentModificationException
* Concurrent modification of resources. HTTP Status Code: 400.
* @sample AmazonAlexaForBusiness.DeleteAddressBook
* @see AWS API Documentation
*/
DeleteAddressBookResult deleteAddressBook(DeleteAddressBookRequest deleteAddressBookRequest);
/**
*
* Deletes the recurring report delivery schedule with the specified schedule ARN.
*
*
* @param deleteBusinessReportScheduleRequest
* @return Result of the DeleteBusinessReportSchedule operation returned by the service.
* @throws NotFoundException
* The resource is not found.
* @throws ConcurrentModificationException
* Concurrent modification of resources. HTTP Status Code: 400.
* @sample AmazonAlexaForBusiness.DeleteBusinessReportSchedule
* @see AWS API Documentation
*/
DeleteBusinessReportScheduleResult deleteBusinessReportSchedule(DeleteBusinessReportScheduleRequest deleteBusinessReportScheduleRequest);
/**
*
* Deletes a conference provider.
*
*
* @param deleteConferenceProviderRequest
* @return Result of the DeleteConferenceProvider operation returned by the service.
* @throws NotFoundException
* The resource is not found.
* @sample AmazonAlexaForBusiness.DeleteConferenceProvider
* @see AWS API Documentation
*/
DeleteConferenceProviderResult deleteConferenceProvider(DeleteConferenceProviderRequest deleteConferenceProviderRequest);
/**
*
* Deletes a contact by the contact ARN.
*
*
* @param deleteContactRequest
* @return Result of the DeleteContact operation returned by the service.
* @throws NotFoundException
* The resource is not found.
* @throws ConcurrentModificationException
* Concurrent modification of resources. HTTP Status Code: 400.
* @sample AmazonAlexaForBusiness.DeleteContact
* @see AWS
* API Documentation
*/
DeleteContactResult deleteContact(DeleteContactRequest deleteContactRequest);
/**
*
* Removes a device from Alexa For Business.
*
*
* @param deleteDeviceRequest
* @return Result of the DeleteDevice operation returned by the service.
* @throws NotFoundException
* The resource is not found.
* @throws ConcurrentModificationException
* Concurrent modification of resources. HTTP Status Code: 400.
* @throws InvalidCertificateAuthorityException
* The Certificate Authority can't issue or revoke a certificate.
* @sample AmazonAlexaForBusiness.DeleteDevice
* @see AWS
* API Documentation
*/
DeleteDeviceResult deleteDevice(DeleteDeviceRequest deleteDeviceRequest);
/**
*
* Deletes a room profile by the profile ARN.
*
*
* @param deleteProfileRequest
* @return Result of the DeleteProfile operation returned by the service.
* @throws NotFoundException
* The resource is not found.
* @throws ConcurrentModificationException
* Concurrent modification of resources. HTTP Status Code: 400.
* @sample AmazonAlexaForBusiness.DeleteProfile
* @see AWS
* API Documentation
*/
DeleteProfileResult deleteProfile(DeleteProfileRequest deleteProfileRequest);
/**
*
* Deletes a room by the room ARN.
*
*
* @param deleteRoomRequest
* @return Result of the DeleteRoom operation returned by the service.
* @throws NotFoundException
* The resource is not found.
* @throws ConcurrentModificationException
* Concurrent modification of resources. HTTP Status Code: 400.
* @sample AmazonAlexaForBusiness.DeleteRoom
* @see AWS
* API Documentation
*/
DeleteRoomResult deleteRoom(DeleteRoomRequest deleteRoomRequest);
/**
*
* Deletes room skill parameter details by room, skill, and parameter key ID.
*
*
* @param deleteRoomSkillParameterRequest
* @return Result of the DeleteRoomSkillParameter operation returned by the service.
* @throws ConcurrentModificationException
* Concurrent modification of resources. HTTP Status Code: 400.
* @sample AmazonAlexaForBusiness.DeleteRoomSkillParameter
* @see AWS API Documentation
*/
DeleteRoomSkillParameterResult deleteRoomSkillParameter(DeleteRoomSkillParameterRequest deleteRoomSkillParameterRequest);
/**
*
* Unlinks a third-party account from a skill.
*
*
* @param deleteSkillAuthorizationRequest
* @return Result of the DeleteSkillAuthorization operation returned by the service.
* @throws NotFoundException
* The resource is not found.
* @throws ConcurrentModificationException
* Concurrent modification of resources. HTTP Status Code: 400.
* @sample AmazonAlexaForBusiness.DeleteSkillAuthorization
* @see AWS API Documentation
*/
DeleteSkillAuthorizationResult deleteSkillAuthorization(DeleteSkillAuthorizationRequest deleteSkillAuthorizationRequest);
/**
*
* Deletes a skill group by skill group ARN.
*
*
* @param deleteSkillGroupRequest
* @return Result of the DeleteSkillGroup operation returned by the service.
* @throws NotFoundException
* The resource is not found.
* @throws ConcurrentModificationException
* Concurrent modification of resources. HTTP Status Code: 400.
* @sample AmazonAlexaForBusiness.DeleteSkillGroup
* @see AWS API Documentation
*/
DeleteSkillGroupResult deleteSkillGroup(DeleteSkillGroupRequest deleteSkillGroupRequest);
/**
*
* Deletes a specified user by user ARN and enrollment ARN.
*
*
* @param deleteUserRequest
* @return Result of the DeleteUser operation returned by the service.
* @throws NotFoundException
* The resource is not found.
* @throws ConcurrentModificationException
* Concurrent modification of resources. HTTP Status Code: 400.
* @sample AmazonAlexaForBusiness.DeleteUser
* @see AWS
* API Documentation
*/
DeleteUserResult deleteUser(DeleteUserRequest deleteUserRequest);
/**
*
* Disassociates a contact from a given address book.
*
*
* @param disassociateContactFromAddressBookRequest
* @return Result of the DisassociateContactFromAddressBook operation returned by the service.
* @sample AmazonAlexaForBusiness.DisassociateContactFromAddressBook
* @see AWS API Documentation
*/
DisassociateContactFromAddressBookResult disassociateContactFromAddressBook(
DisassociateContactFromAddressBookRequest disassociateContactFromAddressBookRequest);
/**
*
* Disassociates a device from its current room. The device continues to be connected to the Wi-Fi network and is
* still registered to the account. The device settings and skills are removed from the room.
*
*
* @param disassociateDeviceFromRoomRequest
* @return Result of the DisassociateDeviceFromRoom operation returned by the service.
* @throws ConcurrentModificationException
* Concurrent modification of resources. HTTP Status Code: 400.
* @throws DeviceNotRegisteredException
* The request failed because this device is no longer registered and therefore no longer managed by this
* account.
* @sample AmazonAlexaForBusiness.DisassociateDeviceFromRoom
* @see AWS API Documentation
*/
DisassociateDeviceFromRoomResult disassociateDeviceFromRoom(DisassociateDeviceFromRoomRequest disassociateDeviceFromRoomRequest);
/**
*
* Disassociates a skill from a skill group.
*
*
* @param disassociateSkillFromSkillGroupRequest
* @return Result of the DisassociateSkillFromSkillGroup operation returned by the service.
* @throws ConcurrentModificationException
* Concurrent modification of resources. HTTP Status Code: 400.
* @throws NotFoundException
* The resource is not found.
* @sample AmazonAlexaForBusiness.DisassociateSkillFromSkillGroup
* @see AWS API Documentation
*/
DisassociateSkillFromSkillGroupResult disassociateSkillFromSkillGroup(DisassociateSkillFromSkillGroupRequest disassociateSkillFromSkillGroupRequest);
/**
*
* Makes a private skill unavailable for enrolled users and prevents them from enabling it on their devices.
*
*
* @param disassociateSkillFromUsersRequest
* @return Result of the DisassociateSkillFromUsers operation returned by the service.
* @throws ConcurrentModificationException
* Concurrent modification of resources. HTTP Status Code: 400.
* @sample AmazonAlexaForBusiness.DisassociateSkillFromUsers
* @see AWS API Documentation
*/
DisassociateSkillFromUsersResult disassociateSkillFromUsers(DisassociateSkillFromUsersRequest disassociateSkillFromUsersRequest);
/**
*
* Disassociates a skill group from a specified room. This disables all skills in the skill group on all devices in
* the room.
*
*
* @param disassociateSkillGroupFromRoomRequest
* @return Result of the DisassociateSkillGroupFromRoom operation returned by the service.
* @throws ConcurrentModificationException
* Concurrent modification of resources. HTTP Status Code: 400.
* @sample AmazonAlexaForBusiness.DisassociateSkillGroupFromRoom
* @see AWS API Documentation
*/
DisassociateSkillGroupFromRoomResult disassociateSkillGroupFromRoom(DisassociateSkillGroupFromRoomRequest disassociateSkillGroupFromRoomRequest);
/**
*
* Forgets smart home appliances associated to a room.
*
*
* @param forgetSmartHomeAppliancesRequest
* @return Result of the ForgetSmartHomeAppliances operation returned by the service.
* @throws NotFoundException
* The resource is not found.
* @sample AmazonAlexaForBusiness.ForgetSmartHomeAppliances
* @see AWS API Documentation
*/
ForgetSmartHomeAppliancesResult forgetSmartHomeAppliances(ForgetSmartHomeAppliancesRequest forgetSmartHomeAppliancesRequest);
/**
*
* Gets address the book details by the address book ARN.
*
*
* @param getAddressBookRequest
* @return Result of the GetAddressBook operation returned by the service.
* @throws NotFoundException
* The resource is not found.
* @sample AmazonAlexaForBusiness.GetAddressBook
* @see AWS API Documentation
*/
GetAddressBookResult getAddressBook(GetAddressBookRequest getAddressBookRequest);
/**
*
* Retrieves the existing conference preferences.
*
*
* @param getConferencePreferenceRequest
* @return Result of the GetConferencePreference operation returned by the service.
* @throws NotFoundException
* The resource is not found.
* @sample AmazonAlexaForBusiness.GetConferencePreference
* @see AWS API Documentation
*/
GetConferencePreferenceResult getConferencePreference(GetConferencePreferenceRequest getConferencePreferenceRequest);
/**
*
* Gets details about a specific conference provider.
*
*
* @param getConferenceProviderRequest
* @return Result of the GetConferenceProvider operation returned by the service.
* @throws NotFoundException
* The resource is not found.
* @sample AmazonAlexaForBusiness.GetConferenceProvider
* @see AWS API Documentation
*/
GetConferenceProviderResult getConferenceProvider(GetConferenceProviderRequest getConferenceProviderRequest);
/**
*
* Gets the contact details by the contact ARN.
*
*
* @param getContactRequest
* @return Result of the GetContact operation returned by the service.
* @throws NotFoundException
* The resource is not found.
* @sample AmazonAlexaForBusiness.GetContact
* @see AWS
* API Documentation
*/
GetContactResult getContact(GetContactRequest getContactRequest);
/**
*
* Gets the details of a device by device ARN.
*
*
* @param getDeviceRequest
* @return Result of the GetDevice operation returned by the service.
* @throws NotFoundException
* The resource is not found.
* @sample AmazonAlexaForBusiness.GetDevice
* @see AWS API
* Documentation
*/
GetDeviceResult getDevice(GetDeviceRequest getDeviceRequest);
/**
*
* Gets the details of a room profile by profile ARN.
*
*
* @param getProfileRequest
* @return Result of the GetProfile operation returned by the service.
* @throws NotFoundException
* The resource is not found.
* @sample AmazonAlexaForBusiness.GetProfile
* @see AWS
* API Documentation
*/
GetProfileResult getProfile(GetProfileRequest getProfileRequest);
/**
*
* Gets room details by room ARN.
*
*
* @param getRoomRequest
* @return Result of the GetRoom operation returned by the service.
* @throws NotFoundException
* The resource is not found.
* @sample AmazonAlexaForBusiness.GetRoom
* @see AWS API
* Documentation
*/
GetRoomResult getRoom(GetRoomRequest getRoomRequest);
/**
*
* Gets room skill parameter details by room, skill, and parameter key ARN.
*
*
* @param getRoomSkillParameterRequest
* @return Result of the GetRoomSkillParameter operation returned by the service.
* @throws NotFoundException
* The resource is not found.
* @sample AmazonAlexaForBusiness.GetRoomSkillParameter
* @see AWS API Documentation
*/
GetRoomSkillParameterResult getRoomSkillParameter(GetRoomSkillParameterRequest getRoomSkillParameterRequest);
/**
*
* Gets skill group details by skill group ARN.
*
*
* @param getSkillGroupRequest
* @return Result of the GetSkillGroup operation returned by the service.
* @throws NotFoundException
* The resource is not found.
* @sample AmazonAlexaForBusiness.GetSkillGroup
* @see AWS
* API Documentation
*/
GetSkillGroupResult getSkillGroup(GetSkillGroupRequest getSkillGroupRequest);
/**
*
* Lists the details of the schedules that a user configured.
*
*
* @param listBusinessReportSchedulesRequest
* @return Result of the ListBusinessReportSchedules operation returned by the service.
* @sample AmazonAlexaForBusiness.ListBusinessReportSchedules
* @see AWS API Documentation
*/
ListBusinessReportSchedulesResult listBusinessReportSchedules(ListBusinessReportSchedulesRequest listBusinessReportSchedulesRequest);
/**
*
* Lists conference providers under a specific AWS account.
*
*
* @param listConferenceProvidersRequest
* @return Result of the ListConferenceProviders operation returned by the service.
* @sample AmazonAlexaForBusiness.ListConferenceProviders
* @see AWS API Documentation
*/
ListConferenceProvidersResult listConferenceProviders(ListConferenceProvidersRequest listConferenceProvidersRequest);
/**
*
* Lists the device event history, including device connection status, for up to 30 days.
*
*
* @param listDeviceEventsRequest
* @return Result of the ListDeviceEvents operation returned by the service.
* @throws NotFoundException
* The resource is not found.
* @sample AmazonAlexaForBusiness.ListDeviceEvents
* @see AWS API Documentation
*/
ListDeviceEventsResult listDeviceEvents(ListDeviceEventsRequest listDeviceEventsRequest);
/**
*
* Lists all enabled skills in a specific skill group.
*
*
* @param listSkillsRequest
* @return Result of the ListSkills operation returned by the service.
* @sample AmazonAlexaForBusiness.ListSkills
* @see AWS
* API Documentation
*/
ListSkillsResult listSkills(ListSkillsRequest listSkillsRequest);
/**
*
* Lists all categories in the Alexa skill store.
*
*
* @param listSkillsStoreCategoriesRequest
* @return Result of the ListSkillsStoreCategories operation returned by the service.
* @sample AmazonAlexaForBusiness.ListSkillsStoreCategories
* @see AWS API Documentation
*/
ListSkillsStoreCategoriesResult listSkillsStoreCategories(ListSkillsStoreCategoriesRequest listSkillsStoreCategoriesRequest);
/**
*
* Lists all skills in the Alexa skill store by category.
*
*
* @param listSkillsStoreSkillsByCategoryRequest
* @return Result of the ListSkillsStoreSkillsByCategory operation returned by the service.
* @sample AmazonAlexaForBusiness.ListSkillsStoreSkillsByCategory
* @see AWS API Documentation
*/
ListSkillsStoreSkillsByCategoryResult listSkillsStoreSkillsByCategory(ListSkillsStoreSkillsByCategoryRequest listSkillsStoreSkillsByCategoryRequest);
/**
*
* Lists all of the smart home appliances associated with a room.
*
*
* @param listSmartHomeAppliancesRequest
* @return Result of the ListSmartHomeAppliances operation returned by the service.
* @throws NotFoundException
* The resource is not found.
* @sample AmazonAlexaForBusiness.ListSmartHomeAppliances
* @see AWS API Documentation
*/
ListSmartHomeAppliancesResult listSmartHomeAppliances(ListSmartHomeAppliancesRequest listSmartHomeAppliancesRequest);
/**
*
* Lists all tags for the specified resource.
*
*
* @param listTagsRequest
* @return Result of the ListTags operation returned by the service.
* @throws NotFoundException
* The resource is not found.
* @sample AmazonAlexaForBusiness.ListTags
* @see AWS API
* Documentation
*/
ListTagsResult listTags(ListTagsRequest listTagsRequest);
/**
*
* Sets the conference preferences on a specific conference provider at the account level.
*
*
* @param putConferencePreferenceRequest
* @return Result of the PutConferencePreference operation returned by the service.
* @throws NotFoundException
* The resource is not found.
* @sample AmazonAlexaForBusiness.PutConferencePreference
* @see AWS API Documentation
*/
PutConferencePreferenceResult putConferencePreference(PutConferencePreferenceRequest putConferencePreferenceRequest);
/**
*
* Updates room skill parameter details by room, skill, and parameter key ID. Not all skills have a room skill
* parameter.
*
*
* @param putRoomSkillParameterRequest
* @return Result of the PutRoomSkillParameter operation returned by the service.
* @throws ConcurrentModificationException
* Concurrent modification of resources. HTTP Status Code: 400.
* @sample AmazonAlexaForBusiness.PutRoomSkillParameter
* @see AWS API Documentation
*/
PutRoomSkillParameterResult putRoomSkillParameter(PutRoomSkillParameterRequest putRoomSkillParameterRequest);
/**
*
* Links a user's account to a third-party skill provider. If this API operation is called by an assumed IAM role,
* the skill being linked must be a private skill. Also, the skill must be owned by the AWS account that assumed the
* IAM role.
*
*
* @param putSkillAuthorizationRequest
* @return Result of the PutSkillAuthorization operation returned by the service.
* @throws UnauthorizedException
* The caller has no permissions to operate on the resource involved in the API call.
* @throws ConcurrentModificationException
* Concurrent modification of resources. HTTP Status Code: 400.
* @sample AmazonAlexaForBusiness.PutSkillAuthorization
* @see AWS API Documentation
*/
PutSkillAuthorizationResult putSkillAuthorization(PutSkillAuthorizationRequest putSkillAuthorizationRequest);
/**
*
* Registers an Alexa-enabled device built by an Original Equipment Manufacturer (OEM) using Alexa Voice Service
* (AVS).
*
*
* @param registerAVSDeviceRequest
* @return Result of the RegisterAVSDevice operation returned by the service.
* @throws LimitExceededException
* You are performing an action that would put you beyond your account's limits.
* @throws ConcurrentModificationException
* Concurrent modification of resources. HTTP Status Code: 400.
* @throws InvalidDeviceException
* The device is in an invalid state.
* @sample AmazonAlexaForBusiness.RegisterAVSDevice
* @see AWS API Documentation
*/
RegisterAVSDeviceResult registerAVSDevice(RegisterAVSDeviceRequest registerAVSDeviceRequest);
/**
*
* Disassociates a skill from the organization under a user's AWS account. If the skill is a private skill, it moves
* to an AcceptStatus of PENDING. Any private or public skill that is rejected can be added later by calling the
* ApproveSkill API.
*
*
* @param rejectSkillRequest
* @return Result of the RejectSkill operation returned by the service.
* @throws ConcurrentModificationException
* Concurrent modification of resources. HTTP Status Code: 400.
* @throws NotFoundException
* The resource is not found.
* @sample AmazonAlexaForBusiness.RejectSkill
* @see AWS
* API Documentation
*/
RejectSkillResult rejectSkill(RejectSkillRequest rejectSkillRequest);
/**
*
* Determines the details for the room from which a skill request was invoked. This operation is used by skill
* developers.
*
*
* @param resolveRoomRequest
* @return Result of the ResolveRoom operation returned by the service.
* @throws NotFoundException
* The resource is not found.
* @sample AmazonAlexaForBusiness.ResolveRoom
* @see AWS
* API Documentation
*/
ResolveRoomResult resolveRoom(ResolveRoomRequest resolveRoomRequest);
/**
*
* Revokes an invitation and invalidates the enrollment URL.
*
*
* @param revokeInvitationRequest
* @return Result of the RevokeInvitation operation returned by the service.
* @throws NotFoundException
* The resource is not found.
* @throws ConcurrentModificationException
* Concurrent modification of resources. HTTP Status Code: 400.
* @sample AmazonAlexaForBusiness.RevokeInvitation
* @see AWS API Documentation
*/
RevokeInvitationResult revokeInvitation(RevokeInvitationRequest revokeInvitationRequest);
/**
*
* Searches address books and lists the ones that meet a set of filter and sort criteria.
*
*
* @param searchAddressBooksRequest
* @return Result of the SearchAddressBooks operation returned by the service.
* @sample AmazonAlexaForBusiness.SearchAddressBooks
* @see AWS API Documentation
*/
SearchAddressBooksResult searchAddressBooks(SearchAddressBooksRequest searchAddressBooksRequest);
/**
*
* Searches contacts and lists the ones that meet a set of filter and sort criteria.
*
*
* @param searchContactsRequest
* @return Result of the SearchContacts operation returned by the service.
* @sample AmazonAlexaForBusiness.SearchContacts
* @see AWS API Documentation
*/
SearchContactsResult searchContacts(SearchContactsRequest searchContactsRequest);
/**
*
* Searches devices and lists the ones that meet a set of filter criteria.
*
*
* @param searchDevicesRequest
* @return Result of the SearchDevices operation returned by the service.
* @sample AmazonAlexaForBusiness.SearchDevices
* @see AWS
* API Documentation
*/
SearchDevicesResult searchDevices(SearchDevicesRequest searchDevicesRequest);
/**
*
* Searches room profiles and lists the ones that meet a set of filter criteria.
*
*
* @param searchProfilesRequest
* @return Result of the SearchProfiles operation returned by the service.
* @sample AmazonAlexaForBusiness.SearchProfiles
* @see AWS API Documentation
*/
SearchProfilesResult searchProfiles(SearchProfilesRequest searchProfilesRequest);
/**
*
* Searches rooms and lists the ones that meet a set of filter and sort criteria.
*
*
* @param searchRoomsRequest
* @return Result of the SearchRooms operation returned by the service.
* @sample AmazonAlexaForBusiness.SearchRooms
* @see AWS
* API Documentation
*/
SearchRoomsResult searchRooms(SearchRoomsRequest searchRoomsRequest);
/**
*
* Searches skill groups and lists the ones that meet a set of filter and sort criteria.
*
*
* @param searchSkillGroupsRequest
* @return Result of the SearchSkillGroups operation returned by the service.
* @sample AmazonAlexaForBusiness.SearchSkillGroups
* @see AWS API Documentation
*/
SearchSkillGroupsResult searchSkillGroups(SearchSkillGroupsRequest searchSkillGroupsRequest);
/**
*
* Searches users and lists the ones that meet a set of filter and sort criteria.
*
*
* @param searchUsersRequest
* @return Result of the SearchUsers operation returned by the service.
* @sample AmazonAlexaForBusiness.SearchUsers
* @see AWS
* API Documentation
*/
SearchUsersResult searchUsers(SearchUsersRequest searchUsersRequest);
/**
*
* Sends an enrollment invitation email with a URL to a user. The URL is valid for 72 hours or until you call this
* operation again, whichever comes first.
*
*
* @param sendInvitationRequest
* @return Result of the SendInvitation operation returned by the service.
* @throws NotFoundException
* The resource is not found.
* @throws InvalidUserStatusException
* The attempt to update a user is invalid due to the user's current status.
* @throws ConcurrentModificationException
* Concurrent modification of resources. HTTP Status Code: 400.
* @sample AmazonAlexaForBusiness.SendInvitation
* @see AWS API Documentation
*/
SendInvitationResult sendInvitation(SendInvitationRequest sendInvitationRequest);
/**
*
* Resets a device and its account to the known default settings, by clearing all information and settings set by
* previous users.
*
*
* @param startDeviceSyncRequest
* @return Result of the StartDeviceSync operation returned by the service.
* @throws DeviceNotRegisteredException
* The request failed because this device is no longer registered and therefore no longer managed by this
* account.
* @sample AmazonAlexaForBusiness.StartDeviceSync
* @see AWS API Documentation
*/
StartDeviceSyncResult startDeviceSync(StartDeviceSyncRequest startDeviceSyncRequest);
/**
*
* Initiates the discovery of any smart home appliances associated with the room.
*
*
* @param startSmartHomeApplianceDiscoveryRequest
* @return Result of the StartSmartHomeApplianceDiscovery operation returned by the service.
* @throws NotFoundException
* The resource is not found.
* @sample AmazonAlexaForBusiness.StartSmartHomeApplianceDiscovery
* @see AWS API Documentation
*/
StartSmartHomeApplianceDiscoveryResult startSmartHomeApplianceDiscovery(StartSmartHomeApplianceDiscoveryRequest startSmartHomeApplianceDiscoveryRequest);
/**
*
* Adds metadata tags to a specified resource.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws NotFoundException
* The resource is not found.
* @sample AmazonAlexaForBusiness.TagResource
* @see AWS
* API Documentation
*/
TagResourceResult tagResource(TagResourceRequest tagResourceRequest);
/**
*
* Removes metadata tags from a specified resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws NotFoundException
* The resource is not found.
* @sample AmazonAlexaForBusiness.UntagResource
* @see AWS
* API Documentation
*/
UntagResourceResult untagResource(UntagResourceRequest untagResourceRequest);
/**
*
* Updates address book details by the address book ARN.
*
*
* @param updateAddressBookRequest
* @return Result of the UpdateAddressBook operation returned by the service.
* @throws NotFoundException
* The resource is not found.
* @throws NameInUseException
* The name sent in the request is already in use.
* @throws ConcurrentModificationException
* Concurrent modification of resources. HTTP Status Code: 400.
* @sample AmazonAlexaForBusiness.UpdateAddressBook
* @see AWS API Documentation
*/
UpdateAddressBookResult updateAddressBook(UpdateAddressBookRequest updateAddressBookRequest);
/**
*
* Updates the configuration of the report delivery schedule with the specified schedule ARN.
*
*
* @param updateBusinessReportScheduleRequest
* @return Result of the UpdateBusinessReportSchedule operation returned by the service.
* @throws NotFoundException
* The resource is not found.
* @throws ConcurrentModificationException
* Concurrent modification of resources. HTTP Status Code: 400.
* @sample AmazonAlexaForBusiness.UpdateBusinessReportSchedule
* @see AWS API Documentation
*/
UpdateBusinessReportScheduleResult updateBusinessReportSchedule(UpdateBusinessReportScheduleRequest updateBusinessReportScheduleRequest);
/**
*
* Updates an existing conference provider's settings.
*
*
* @param updateConferenceProviderRequest
* @return Result of the UpdateConferenceProvider operation returned by the service.
* @throws NotFoundException
* The resource is not found.
* @sample AmazonAlexaForBusiness.UpdateConferenceProvider
* @see AWS API Documentation
*/
UpdateConferenceProviderResult updateConferenceProvider(UpdateConferenceProviderRequest updateConferenceProviderRequest);
/**
*
* Updates the contact details by the contact ARN.
*
*
* @param updateContactRequest
* @return Result of the UpdateContact operation returned by the service.
* @throws NotFoundException
* The resource is not found.
* @throws ConcurrentModificationException
* Concurrent modification of resources. HTTP Status Code: 400.
* @sample AmazonAlexaForBusiness.UpdateContact
* @see AWS
* API Documentation
*/
UpdateContactResult updateContact(UpdateContactRequest updateContactRequest);
/**
*
* Updates the device name by device ARN.
*
*
* @param updateDeviceRequest
* @return Result of the UpdateDevice operation returned by the service.
* @throws NotFoundException
* The resource is not found.
* @throws ConcurrentModificationException
* Concurrent modification of resources. HTTP Status Code: 400.
* @throws DeviceNotRegisteredException
* The request failed because this device is no longer registered and therefore no longer managed by this
* account.
* @sample AmazonAlexaForBusiness.UpdateDevice
* @see AWS
* API Documentation
*/
UpdateDeviceResult updateDevice(UpdateDeviceRequest updateDeviceRequest);
/**
*
* Updates an existing room profile by room profile ARN.
*
*
* @param updateProfileRequest
* @return Result of the UpdateProfile operation returned by the service.
* @throws NotFoundException
* The resource is not found.
* @throws NameInUseException
* The name sent in the request is already in use.
* @throws ConcurrentModificationException
* Concurrent modification of resources. HTTP Status Code: 400.
* @sample AmazonAlexaForBusiness.UpdateProfile
* @see AWS
* API Documentation
*/
UpdateProfileResult updateProfile(UpdateProfileRequest updateProfileRequest);
/**
*
* Updates room details by room ARN.
*
*
* @param updateRoomRequest
* @return Result of the UpdateRoom operation returned by the service.
* @throws NotFoundException
* The resource is not found.
* @throws NameInUseException
* The name sent in the request is already in use.
* @sample AmazonAlexaForBusiness.UpdateRoom
* @see AWS
* API Documentation
*/
UpdateRoomResult updateRoom(UpdateRoomRequest updateRoomRequest);
/**
*
* Updates skill group details by skill group ARN.
*
*
* @param updateSkillGroupRequest
* @return Result of the UpdateSkillGroup operation returned by the service.
* @throws NotFoundException
* The resource is not found.
* @throws NameInUseException
* The name sent in the request is already in use.
* @throws ConcurrentModificationException
* Concurrent modification of resources. HTTP Status Code: 400.
* @sample AmazonAlexaForBusiness.UpdateSkillGroup
* @see AWS API Documentation
*/
UpdateSkillGroupResult updateSkillGroup(UpdateSkillGroupRequest updateSkillGroupRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}