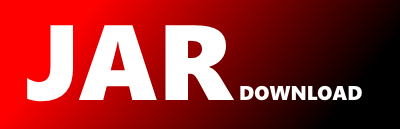
com.amazonaws.services.amplifyuibuilder.model.Predicate Maven / Gradle / Ivy
Show all versions of aws-java-sdk-amplifyuibuilder Show documentation
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.amplifyuibuilder.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Stores information for generating Amplify DataStore queries. Use a Predicate
to retrieve a subset of the
* data in a collection.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class Predicate implements Serializable, Cloneable, StructuredPojo {
/**
*
* A list of predicates to combine logically.
*
*/
private java.util.List or;
/**
*
* A list of predicates to combine logically.
*
*/
private java.util.List and;
/**
*
* The field to query.
*
*/
private String field;
/**
*
* The operator to use to perform the evaluation.
*
*/
private String operator;
/**
*
* The value to use when performing the evaluation.
*
*/
private String operand;
/**
*
* The type of value to use when performing the evaluation.
*
*/
private String operandType;
/**
*
* A list of predicates to combine logically.
*
*
* @return A list of predicates to combine logically.
*/
public java.util.List getOr() {
return or;
}
/**
*
* A list of predicates to combine logically.
*
*
* @param or
* A list of predicates to combine logically.
*/
public void setOr(java.util.Collection or) {
if (or == null) {
this.or = null;
return;
}
this.or = new java.util.ArrayList(or);
}
/**
*
* A list of predicates to combine logically.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setOr(java.util.Collection)} or {@link #withOr(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param or
* A list of predicates to combine logically.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Predicate withOr(Predicate... or) {
if (this.or == null) {
setOr(new java.util.ArrayList(or.length));
}
for (Predicate ele : or) {
this.or.add(ele);
}
return this;
}
/**
*
* A list of predicates to combine logically.
*
*
* @param or
* A list of predicates to combine logically.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Predicate withOr(java.util.Collection or) {
setOr(or);
return this;
}
/**
*
* A list of predicates to combine logically.
*
*
* @return A list of predicates to combine logically.
*/
public java.util.List getAnd() {
return and;
}
/**
*
* A list of predicates to combine logically.
*
*
* @param and
* A list of predicates to combine logically.
*/
public void setAnd(java.util.Collection and) {
if (and == null) {
this.and = null;
return;
}
this.and = new java.util.ArrayList(and);
}
/**
*
* A list of predicates to combine logically.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAnd(java.util.Collection)} or {@link #withAnd(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param and
* A list of predicates to combine logically.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Predicate withAnd(Predicate... and) {
if (this.and == null) {
setAnd(new java.util.ArrayList(and.length));
}
for (Predicate ele : and) {
this.and.add(ele);
}
return this;
}
/**
*
* A list of predicates to combine logically.
*
*
* @param and
* A list of predicates to combine logically.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Predicate withAnd(java.util.Collection and) {
setAnd(and);
return this;
}
/**
*
* The field to query.
*
*
* @param field
* The field to query.
*/
public void setField(String field) {
this.field = field;
}
/**
*
* The field to query.
*
*
* @return The field to query.
*/
public String getField() {
return this.field;
}
/**
*
* The field to query.
*
*
* @param field
* The field to query.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Predicate withField(String field) {
setField(field);
return this;
}
/**
*
* The operator to use to perform the evaluation.
*
*
* @param operator
* The operator to use to perform the evaluation.
*/
public void setOperator(String operator) {
this.operator = operator;
}
/**
*
* The operator to use to perform the evaluation.
*
*
* @return The operator to use to perform the evaluation.
*/
public String getOperator() {
return this.operator;
}
/**
*
* The operator to use to perform the evaluation.
*
*
* @param operator
* The operator to use to perform the evaluation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Predicate withOperator(String operator) {
setOperator(operator);
return this;
}
/**
*
* The value to use when performing the evaluation.
*
*
* @param operand
* The value to use when performing the evaluation.
*/
public void setOperand(String operand) {
this.operand = operand;
}
/**
*
* The value to use when performing the evaluation.
*
*
* @return The value to use when performing the evaluation.
*/
public String getOperand() {
return this.operand;
}
/**
*
* The value to use when performing the evaluation.
*
*
* @param operand
* The value to use when performing the evaluation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Predicate withOperand(String operand) {
setOperand(operand);
return this;
}
/**
*
* The type of value to use when performing the evaluation.
*
*
* @param operandType
* The type of value to use when performing the evaluation.
*/
public void setOperandType(String operandType) {
this.operandType = operandType;
}
/**
*
* The type of value to use when performing the evaluation.
*
*
* @return The type of value to use when performing the evaluation.
*/
public String getOperandType() {
return this.operandType;
}
/**
*
* The type of value to use when performing the evaluation.
*
*
* @param operandType
* The type of value to use when performing the evaluation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Predicate withOperandType(String operandType) {
setOperandType(operandType);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getOr() != null)
sb.append("Or: ").append(getOr()).append(",");
if (getAnd() != null)
sb.append("And: ").append(getAnd()).append(",");
if (getField() != null)
sb.append("Field: ").append(getField()).append(",");
if (getOperator() != null)
sb.append("Operator: ").append(getOperator()).append(",");
if (getOperand() != null)
sb.append("Operand: ").append(getOperand()).append(",");
if (getOperandType() != null)
sb.append("OperandType: ").append(getOperandType());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof Predicate == false)
return false;
Predicate other = (Predicate) obj;
if (other.getOr() == null ^ this.getOr() == null)
return false;
if (other.getOr() != null && other.getOr().equals(this.getOr()) == false)
return false;
if (other.getAnd() == null ^ this.getAnd() == null)
return false;
if (other.getAnd() != null && other.getAnd().equals(this.getAnd()) == false)
return false;
if (other.getField() == null ^ this.getField() == null)
return false;
if (other.getField() != null && other.getField().equals(this.getField()) == false)
return false;
if (other.getOperator() == null ^ this.getOperator() == null)
return false;
if (other.getOperator() != null && other.getOperator().equals(this.getOperator()) == false)
return false;
if (other.getOperand() == null ^ this.getOperand() == null)
return false;
if (other.getOperand() != null && other.getOperand().equals(this.getOperand()) == false)
return false;
if (other.getOperandType() == null ^ this.getOperandType() == null)
return false;
if (other.getOperandType() != null && other.getOperandType().equals(this.getOperandType()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getOr() == null) ? 0 : getOr().hashCode());
hashCode = prime * hashCode + ((getAnd() == null) ? 0 : getAnd().hashCode());
hashCode = prime * hashCode + ((getField() == null) ? 0 : getField().hashCode());
hashCode = prime * hashCode + ((getOperator() == null) ? 0 : getOperator().hashCode());
hashCode = prime * hashCode + ((getOperand() == null) ? 0 : getOperand().hashCode());
hashCode = prime * hashCode + ((getOperandType() == null) ? 0 : getOperandType().hashCode());
return hashCode;
}
@Override
public Predicate clone() {
try {
return (Predicate) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.amplifyuibuilder.model.transform.PredicateMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}