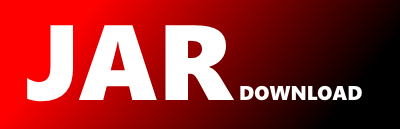
com.amazonaws.services.amplifyuibuilder.model.FieldInputConfig Maven / Gradle / Ivy
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.amplifyuibuilder.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Describes the configuration for the default input values to display for a field.
*
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class FieldInputConfig implements Serializable, Cloneable, StructuredPojo {
/**
*
* The input type for the field.
*
*/
private String type;
/**
*
* Specifies a field that requires input.
*
*/
private Boolean required;
/**
*
* Specifies a read only field.
*
*/
private Boolean readOnly;
/**
*
* The text to display as a placeholder for the field.
*
*/
private String placeholder;
/**
*
* The default value for the field.
*
*/
private String defaultValue;
/**
*
* The text to display to describe the field.
*
*/
private String descriptiveText;
/**
*
* Specifies whether a field has a default value.
*
*/
private Boolean defaultChecked;
/**
*
* The default country code for a phone number.
*
*/
private String defaultCountryCode;
/**
*
* The information to use to customize the input fields with data at runtime.
*
*/
private ValueMappings valueMappings;
/**
*
* The name of the field.
*
*/
private String name;
/**
*
* The minimum value to display for the field.
*
*/
private Float minValue;
/**
*
* The maximum value to display for the field.
*
*/
private Float maxValue;
/**
*
* The stepping increment for a numeric value in a field.
*
*/
private Float step;
/**
*
* The value for the field.
*
*/
private String value;
/**
*
* Specifies whether to render the field as an array. This property is ignored if the dataSourceType
* for the form is a Data Store.
*
*/
private Boolean isArray;
/**
*
* The configuration for the file uploader field.
*
*/
private FileUploaderFieldConfig fileUploaderConfig;
/**
*
* The input type for the field.
*
*
* @param type
* The input type for the field.
*/
public void setType(String type) {
this.type = type;
}
/**
*
* The input type for the field.
*
*
* @return The input type for the field.
*/
public String getType() {
return this.type;
}
/**
*
* The input type for the field.
*
*
* @param type
* The input type for the field.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FieldInputConfig withType(String type) {
setType(type);
return this;
}
/**
*
* Specifies a field that requires input.
*
*
* @param required
* Specifies a field that requires input.
*/
public void setRequired(Boolean required) {
this.required = required;
}
/**
*
* Specifies a field that requires input.
*
*
* @return Specifies a field that requires input.
*/
public Boolean getRequired() {
return this.required;
}
/**
*
* Specifies a field that requires input.
*
*
* @param required
* Specifies a field that requires input.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FieldInputConfig withRequired(Boolean required) {
setRequired(required);
return this;
}
/**
*
* Specifies a field that requires input.
*
*
* @return Specifies a field that requires input.
*/
public Boolean isRequired() {
return this.required;
}
/**
*
* Specifies a read only field.
*
*
* @param readOnly
* Specifies a read only field.
*/
public void setReadOnly(Boolean readOnly) {
this.readOnly = readOnly;
}
/**
*
* Specifies a read only field.
*
*
* @return Specifies a read only field.
*/
public Boolean getReadOnly() {
return this.readOnly;
}
/**
*
* Specifies a read only field.
*
*
* @param readOnly
* Specifies a read only field.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FieldInputConfig withReadOnly(Boolean readOnly) {
setReadOnly(readOnly);
return this;
}
/**
*
* Specifies a read only field.
*
*
* @return Specifies a read only field.
*/
public Boolean isReadOnly() {
return this.readOnly;
}
/**
*
* The text to display as a placeholder for the field.
*
*
* @param placeholder
* The text to display as a placeholder for the field.
*/
public void setPlaceholder(String placeholder) {
this.placeholder = placeholder;
}
/**
*
* The text to display as a placeholder for the field.
*
*
* @return The text to display as a placeholder for the field.
*/
public String getPlaceholder() {
return this.placeholder;
}
/**
*
* The text to display as a placeholder for the field.
*
*
* @param placeholder
* The text to display as a placeholder for the field.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FieldInputConfig withPlaceholder(String placeholder) {
setPlaceholder(placeholder);
return this;
}
/**
*
* The default value for the field.
*
*
* @param defaultValue
* The default value for the field.
*/
public void setDefaultValue(String defaultValue) {
this.defaultValue = defaultValue;
}
/**
*
* The default value for the field.
*
*
* @return The default value for the field.
*/
public String getDefaultValue() {
return this.defaultValue;
}
/**
*
* The default value for the field.
*
*
* @param defaultValue
* The default value for the field.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FieldInputConfig withDefaultValue(String defaultValue) {
setDefaultValue(defaultValue);
return this;
}
/**
*
* The text to display to describe the field.
*
*
* @param descriptiveText
* The text to display to describe the field.
*/
public void setDescriptiveText(String descriptiveText) {
this.descriptiveText = descriptiveText;
}
/**
*
* The text to display to describe the field.
*
*
* @return The text to display to describe the field.
*/
public String getDescriptiveText() {
return this.descriptiveText;
}
/**
*
* The text to display to describe the field.
*
*
* @param descriptiveText
* The text to display to describe the field.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FieldInputConfig withDescriptiveText(String descriptiveText) {
setDescriptiveText(descriptiveText);
return this;
}
/**
*
* Specifies whether a field has a default value.
*
*
* @param defaultChecked
* Specifies whether a field has a default value.
*/
public void setDefaultChecked(Boolean defaultChecked) {
this.defaultChecked = defaultChecked;
}
/**
*
* Specifies whether a field has a default value.
*
*
* @return Specifies whether a field has a default value.
*/
public Boolean getDefaultChecked() {
return this.defaultChecked;
}
/**
*
* Specifies whether a field has a default value.
*
*
* @param defaultChecked
* Specifies whether a field has a default value.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FieldInputConfig withDefaultChecked(Boolean defaultChecked) {
setDefaultChecked(defaultChecked);
return this;
}
/**
*
* Specifies whether a field has a default value.
*
*
* @return Specifies whether a field has a default value.
*/
public Boolean isDefaultChecked() {
return this.defaultChecked;
}
/**
*
* The default country code for a phone number.
*
*
* @param defaultCountryCode
* The default country code for a phone number.
*/
public void setDefaultCountryCode(String defaultCountryCode) {
this.defaultCountryCode = defaultCountryCode;
}
/**
*
* The default country code for a phone number.
*
*
* @return The default country code for a phone number.
*/
public String getDefaultCountryCode() {
return this.defaultCountryCode;
}
/**
*
* The default country code for a phone number.
*
*
* @param defaultCountryCode
* The default country code for a phone number.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FieldInputConfig withDefaultCountryCode(String defaultCountryCode) {
setDefaultCountryCode(defaultCountryCode);
return this;
}
/**
*
* The information to use to customize the input fields with data at runtime.
*
*
* @param valueMappings
* The information to use to customize the input fields with data at runtime.
*/
public void setValueMappings(ValueMappings valueMappings) {
this.valueMappings = valueMappings;
}
/**
*
* The information to use to customize the input fields with data at runtime.
*
*
* @return The information to use to customize the input fields with data at runtime.
*/
public ValueMappings getValueMappings() {
return this.valueMappings;
}
/**
*
* The information to use to customize the input fields with data at runtime.
*
*
* @param valueMappings
* The information to use to customize the input fields with data at runtime.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FieldInputConfig withValueMappings(ValueMappings valueMappings) {
setValueMappings(valueMappings);
return this;
}
/**
*
* The name of the field.
*
*
* @param name
* The name of the field.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The name of the field.
*
*
* @return The name of the field.
*/
public String getName() {
return this.name;
}
/**
*
* The name of the field.
*
*
* @param name
* The name of the field.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FieldInputConfig withName(String name) {
setName(name);
return this;
}
/**
*
* The minimum value to display for the field.
*
*
* @param minValue
* The minimum value to display for the field.
*/
public void setMinValue(Float minValue) {
this.minValue = minValue;
}
/**
*
* The minimum value to display for the field.
*
*
* @return The minimum value to display for the field.
*/
public Float getMinValue() {
return this.minValue;
}
/**
*
* The minimum value to display for the field.
*
*
* @param minValue
* The minimum value to display for the field.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FieldInputConfig withMinValue(Float minValue) {
setMinValue(minValue);
return this;
}
/**
*
* The maximum value to display for the field.
*
*
* @param maxValue
* The maximum value to display for the field.
*/
public void setMaxValue(Float maxValue) {
this.maxValue = maxValue;
}
/**
*
* The maximum value to display for the field.
*
*
* @return The maximum value to display for the field.
*/
public Float getMaxValue() {
return this.maxValue;
}
/**
*
* The maximum value to display for the field.
*
*
* @param maxValue
* The maximum value to display for the field.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FieldInputConfig withMaxValue(Float maxValue) {
setMaxValue(maxValue);
return this;
}
/**
*
* The stepping increment for a numeric value in a field.
*
*
* @param step
* The stepping increment for a numeric value in a field.
*/
public void setStep(Float step) {
this.step = step;
}
/**
*
* The stepping increment for a numeric value in a field.
*
*
* @return The stepping increment for a numeric value in a field.
*/
public Float getStep() {
return this.step;
}
/**
*
* The stepping increment for a numeric value in a field.
*
*
* @param step
* The stepping increment for a numeric value in a field.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FieldInputConfig withStep(Float step) {
setStep(step);
return this;
}
/**
*
* The value for the field.
*
*
* @param value
* The value for the field.
*/
public void setValue(String value) {
this.value = value;
}
/**
*
* The value for the field.
*
*
* @return The value for the field.
*/
public String getValue() {
return this.value;
}
/**
*
* The value for the field.
*
*
* @param value
* The value for the field.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FieldInputConfig withValue(String value) {
setValue(value);
return this;
}
/**
*
* Specifies whether to render the field as an array. This property is ignored if the dataSourceType
* for the form is a Data Store.
*
*
* @param isArray
* Specifies whether to render the field as an array. This property is ignored if the
* dataSourceType
for the form is a Data Store.
*/
public void setIsArray(Boolean isArray) {
this.isArray = isArray;
}
/**
*
* Specifies whether to render the field as an array. This property is ignored if the dataSourceType
* for the form is a Data Store.
*
*
* @return Specifies whether to render the field as an array. This property is ignored if the
* dataSourceType
for the form is a Data Store.
*/
public Boolean getIsArray() {
return this.isArray;
}
/**
*
* Specifies whether to render the field as an array. This property is ignored if the dataSourceType
* for the form is a Data Store.
*
*
* @param isArray
* Specifies whether to render the field as an array. This property is ignored if the
* dataSourceType
for the form is a Data Store.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FieldInputConfig withIsArray(Boolean isArray) {
setIsArray(isArray);
return this;
}
/**
*
* Specifies whether to render the field as an array. This property is ignored if the dataSourceType
* for the form is a Data Store.
*
*
* @return Specifies whether to render the field as an array. This property is ignored if the
* dataSourceType
for the form is a Data Store.
*/
public Boolean isArray() {
return this.isArray;
}
/**
*
* The configuration for the file uploader field.
*
*
* @param fileUploaderConfig
* The configuration for the file uploader field.
*/
public void setFileUploaderConfig(FileUploaderFieldConfig fileUploaderConfig) {
this.fileUploaderConfig = fileUploaderConfig;
}
/**
*
* The configuration for the file uploader field.
*
*
* @return The configuration for the file uploader field.
*/
public FileUploaderFieldConfig getFileUploaderConfig() {
return this.fileUploaderConfig;
}
/**
*
* The configuration for the file uploader field.
*
*
* @param fileUploaderConfig
* The configuration for the file uploader field.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FieldInputConfig withFileUploaderConfig(FileUploaderFieldConfig fileUploaderConfig) {
setFileUploaderConfig(fileUploaderConfig);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getType() != null)
sb.append("Type: ").append(getType()).append(",");
if (getRequired() != null)
sb.append("Required: ").append(getRequired()).append(",");
if (getReadOnly() != null)
sb.append("ReadOnly: ").append(getReadOnly()).append(",");
if (getPlaceholder() != null)
sb.append("Placeholder: ").append(getPlaceholder()).append(",");
if (getDefaultValue() != null)
sb.append("DefaultValue: ").append(getDefaultValue()).append(",");
if (getDescriptiveText() != null)
sb.append("DescriptiveText: ").append(getDescriptiveText()).append(",");
if (getDefaultChecked() != null)
sb.append("DefaultChecked: ").append(getDefaultChecked()).append(",");
if (getDefaultCountryCode() != null)
sb.append("DefaultCountryCode: ").append(getDefaultCountryCode()).append(",");
if (getValueMappings() != null)
sb.append("ValueMappings: ").append(getValueMappings()).append(",");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getMinValue() != null)
sb.append("MinValue: ").append(getMinValue()).append(",");
if (getMaxValue() != null)
sb.append("MaxValue: ").append(getMaxValue()).append(",");
if (getStep() != null)
sb.append("Step: ").append(getStep()).append(",");
if (getValue() != null)
sb.append("Value: ").append(getValue()).append(",");
if (getIsArray() != null)
sb.append("IsArray: ").append(getIsArray()).append(",");
if (getFileUploaderConfig() != null)
sb.append("FileUploaderConfig: ").append(getFileUploaderConfig());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof FieldInputConfig == false)
return false;
FieldInputConfig other = (FieldInputConfig) obj;
if (other.getType() == null ^ this.getType() == null)
return false;
if (other.getType() != null && other.getType().equals(this.getType()) == false)
return false;
if (other.getRequired() == null ^ this.getRequired() == null)
return false;
if (other.getRequired() != null && other.getRequired().equals(this.getRequired()) == false)
return false;
if (other.getReadOnly() == null ^ this.getReadOnly() == null)
return false;
if (other.getReadOnly() != null && other.getReadOnly().equals(this.getReadOnly()) == false)
return false;
if (other.getPlaceholder() == null ^ this.getPlaceholder() == null)
return false;
if (other.getPlaceholder() != null && other.getPlaceholder().equals(this.getPlaceholder()) == false)
return false;
if (other.getDefaultValue() == null ^ this.getDefaultValue() == null)
return false;
if (other.getDefaultValue() != null && other.getDefaultValue().equals(this.getDefaultValue()) == false)
return false;
if (other.getDescriptiveText() == null ^ this.getDescriptiveText() == null)
return false;
if (other.getDescriptiveText() != null && other.getDescriptiveText().equals(this.getDescriptiveText()) == false)
return false;
if (other.getDefaultChecked() == null ^ this.getDefaultChecked() == null)
return false;
if (other.getDefaultChecked() != null && other.getDefaultChecked().equals(this.getDefaultChecked()) == false)
return false;
if (other.getDefaultCountryCode() == null ^ this.getDefaultCountryCode() == null)
return false;
if (other.getDefaultCountryCode() != null && other.getDefaultCountryCode().equals(this.getDefaultCountryCode()) == false)
return false;
if (other.getValueMappings() == null ^ this.getValueMappings() == null)
return false;
if (other.getValueMappings() != null && other.getValueMappings().equals(this.getValueMappings()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getMinValue() == null ^ this.getMinValue() == null)
return false;
if (other.getMinValue() != null && other.getMinValue().equals(this.getMinValue()) == false)
return false;
if (other.getMaxValue() == null ^ this.getMaxValue() == null)
return false;
if (other.getMaxValue() != null && other.getMaxValue().equals(this.getMaxValue()) == false)
return false;
if (other.getStep() == null ^ this.getStep() == null)
return false;
if (other.getStep() != null && other.getStep().equals(this.getStep()) == false)
return false;
if (other.getValue() == null ^ this.getValue() == null)
return false;
if (other.getValue() != null && other.getValue().equals(this.getValue()) == false)
return false;
if (other.getIsArray() == null ^ this.getIsArray() == null)
return false;
if (other.getIsArray() != null && other.getIsArray().equals(this.getIsArray()) == false)
return false;
if (other.getFileUploaderConfig() == null ^ this.getFileUploaderConfig() == null)
return false;
if (other.getFileUploaderConfig() != null && other.getFileUploaderConfig().equals(this.getFileUploaderConfig()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getType() == null) ? 0 : getType().hashCode());
hashCode = prime * hashCode + ((getRequired() == null) ? 0 : getRequired().hashCode());
hashCode = prime * hashCode + ((getReadOnly() == null) ? 0 : getReadOnly().hashCode());
hashCode = prime * hashCode + ((getPlaceholder() == null) ? 0 : getPlaceholder().hashCode());
hashCode = prime * hashCode + ((getDefaultValue() == null) ? 0 : getDefaultValue().hashCode());
hashCode = prime * hashCode + ((getDescriptiveText() == null) ? 0 : getDescriptiveText().hashCode());
hashCode = prime * hashCode + ((getDefaultChecked() == null) ? 0 : getDefaultChecked().hashCode());
hashCode = prime * hashCode + ((getDefaultCountryCode() == null) ? 0 : getDefaultCountryCode().hashCode());
hashCode = prime * hashCode + ((getValueMappings() == null) ? 0 : getValueMappings().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getMinValue() == null) ? 0 : getMinValue().hashCode());
hashCode = prime * hashCode + ((getMaxValue() == null) ? 0 : getMaxValue().hashCode());
hashCode = prime * hashCode + ((getStep() == null) ? 0 : getStep().hashCode());
hashCode = prime * hashCode + ((getValue() == null) ? 0 : getValue().hashCode());
hashCode = prime * hashCode + ((getIsArray() == null) ? 0 : getIsArray().hashCode());
hashCode = prime * hashCode + ((getFileUploaderConfig() == null) ? 0 : getFileUploaderConfig().hashCode());
return hashCode;
}
@Override
public FieldInputConfig clone() {
try {
return (FieldInputConfig) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.amplifyuibuilder.model.transform.FieldInputConfigMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}