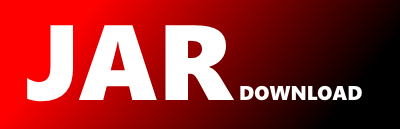
com.amazonaws.services.amplifyuibuilder.model.UpdateComponentData Maven / Gradle / Ivy
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.amplifyuibuilder.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Updates and saves all of the information about a component, based on component ID.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class UpdateComponentData implements Serializable, Cloneable, StructuredPojo {
/**
*
* The unique ID of the component to update.
*
*/
private String id;
/**
*
* The name of the component to update.
*
*/
private String name;
/**
*
* The unique ID of the component in its original source system, such as Figma.
*
*/
private String sourceId;
/**
*
* The type of the component. This can be an Amplify custom UI component or another custom component.
*
*/
private String componentType;
/**
*
* Describes the component's properties.
*
*/
private java.util.Map properties;
/**
*
* The components that are instances of the main component.
*
*/
private java.util.List children;
/**
*
* A list of the unique variants of the main component being updated.
*
*/
private java.util.List variants;
/**
*
* Describes the properties that can be overriden to customize the component.
*
*/
private java.util.Map> overrides;
/**
*
* The data binding information for the component's properties.
*
*/
private java.util.Map bindingProperties;
/**
*
* The configuration for binding a component's properties to a data model. Use this for a collection component.
*
*/
private java.util.Map collectionProperties;
/**
*
* The event configuration for the component. Use for the workflow feature in Amplify Studio that allows you to bind
* events and actions to components.
*
*/
private java.util.Map events;
/**
*
* The schema version of the component when it was imported.
*
*/
private String schemaVersion;
/**
*
* The unique ID of the component to update.
*
*
* @param id
* The unique ID of the component to update.
*/
public void setId(String id) {
this.id = id;
}
/**
*
* The unique ID of the component to update.
*
*
* @return The unique ID of the component to update.
*/
public String getId() {
return this.id;
}
/**
*
* The unique ID of the component to update.
*
*
* @param id
* The unique ID of the component to update.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateComponentData withId(String id) {
setId(id);
return this;
}
/**
*
* The name of the component to update.
*
*
* @param name
* The name of the component to update.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The name of the component to update.
*
*
* @return The name of the component to update.
*/
public String getName() {
return this.name;
}
/**
*
* The name of the component to update.
*
*
* @param name
* The name of the component to update.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateComponentData withName(String name) {
setName(name);
return this;
}
/**
*
* The unique ID of the component in its original source system, such as Figma.
*
*
* @param sourceId
* The unique ID of the component in its original source system, such as Figma.
*/
public void setSourceId(String sourceId) {
this.sourceId = sourceId;
}
/**
*
* The unique ID of the component in its original source system, such as Figma.
*
*
* @return The unique ID of the component in its original source system, such as Figma.
*/
public String getSourceId() {
return this.sourceId;
}
/**
*
* The unique ID of the component in its original source system, such as Figma.
*
*
* @param sourceId
* The unique ID of the component in its original source system, such as Figma.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateComponentData withSourceId(String sourceId) {
setSourceId(sourceId);
return this;
}
/**
*
* The type of the component. This can be an Amplify custom UI component or another custom component.
*
*
* @param componentType
* The type of the component. This can be an Amplify custom UI component or another custom component.
*/
public void setComponentType(String componentType) {
this.componentType = componentType;
}
/**
*
* The type of the component. This can be an Amplify custom UI component or another custom component.
*
*
* @return The type of the component. This can be an Amplify custom UI component or another custom component.
*/
public String getComponentType() {
return this.componentType;
}
/**
*
* The type of the component. This can be an Amplify custom UI component or another custom component.
*
*
* @param componentType
* The type of the component. This can be an Amplify custom UI component or another custom component.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateComponentData withComponentType(String componentType) {
setComponentType(componentType);
return this;
}
/**
*
* Describes the component's properties.
*
*
* @return Describes the component's properties.
*/
public java.util.Map getProperties() {
return properties;
}
/**
*
* Describes the component's properties.
*
*
* @param properties
* Describes the component's properties.
*/
public void setProperties(java.util.Map properties) {
this.properties = properties;
}
/**
*
* Describes the component's properties.
*
*
* @param properties
* Describes the component's properties.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateComponentData withProperties(java.util.Map properties) {
setProperties(properties);
return this;
}
/**
* Add a single Properties entry
*
* @see UpdateComponentData#withProperties
* @returns a reference to this object so that method calls can be chained together.
*/
public UpdateComponentData addPropertiesEntry(String key, ComponentProperty value) {
if (null == this.properties) {
this.properties = new java.util.HashMap();
}
if (this.properties.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.properties.put(key, value);
return this;
}
/**
* Removes all the entries added into Properties.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateComponentData clearPropertiesEntries() {
this.properties = null;
return this;
}
/**
*
* The components that are instances of the main component.
*
*
* @return The components that are instances of the main component.
*/
public java.util.List getChildren() {
return children;
}
/**
*
* The components that are instances of the main component.
*
*
* @param children
* The components that are instances of the main component.
*/
public void setChildren(java.util.Collection children) {
if (children == null) {
this.children = null;
return;
}
this.children = new java.util.ArrayList(children);
}
/**
*
* The components that are instances of the main component.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setChildren(java.util.Collection)} or {@link #withChildren(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param children
* The components that are instances of the main component.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateComponentData withChildren(ComponentChild... children) {
if (this.children == null) {
setChildren(new java.util.ArrayList(children.length));
}
for (ComponentChild ele : children) {
this.children.add(ele);
}
return this;
}
/**
*
* The components that are instances of the main component.
*
*
* @param children
* The components that are instances of the main component.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateComponentData withChildren(java.util.Collection children) {
setChildren(children);
return this;
}
/**
*
* A list of the unique variants of the main component being updated.
*
*
* @return A list of the unique variants of the main component being updated.
*/
public java.util.List getVariants() {
return variants;
}
/**
*
* A list of the unique variants of the main component being updated.
*
*
* @param variants
* A list of the unique variants of the main component being updated.
*/
public void setVariants(java.util.Collection variants) {
if (variants == null) {
this.variants = null;
return;
}
this.variants = new java.util.ArrayList(variants);
}
/**
*
* A list of the unique variants of the main component being updated.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setVariants(java.util.Collection)} or {@link #withVariants(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param variants
* A list of the unique variants of the main component being updated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateComponentData withVariants(ComponentVariant... variants) {
if (this.variants == null) {
setVariants(new java.util.ArrayList(variants.length));
}
for (ComponentVariant ele : variants) {
this.variants.add(ele);
}
return this;
}
/**
*
* A list of the unique variants of the main component being updated.
*
*
* @param variants
* A list of the unique variants of the main component being updated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateComponentData withVariants(java.util.Collection variants) {
setVariants(variants);
return this;
}
/**
*
* Describes the properties that can be overriden to customize the component.
*
*
* @return Describes the properties that can be overriden to customize the component.
*/
public java.util.Map> getOverrides() {
return overrides;
}
/**
*
* Describes the properties that can be overriden to customize the component.
*
*
* @param overrides
* Describes the properties that can be overriden to customize the component.
*/
public void setOverrides(java.util.Map> overrides) {
this.overrides = overrides;
}
/**
*
* Describes the properties that can be overriden to customize the component.
*
*
* @param overrides
* Describes the properties that can be overriden to customize the component.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateComponentData withOverrides(java.util.Map> overrides) {
setOverrides(overrides);
return this;
}
/**
* Add a single Overrides entry
*
* @see UpdateComponentData#withOverrides
* @returns a reference to this object so that method calls can be chained together.
*/
public UpdateComponentData addOverridesEntry(String key, java.util.Map value) {
if (null == this.overrides) {
this.overrides = new java.util.HashMap>();
}
if (this.overrides.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.overrides.put(key, value);
return this;
}
/**
* Removes all the entries added into Overrides.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateComponentData clearOverridesEntries() {
this.overrides = null;
return this;
}
/**
*
* The data binding information for the component's properties.
*
*
* @return The data binding information for the component's properties.
*/
public java.util.Map getBindingProperties() {
return bindingProperties;
}
/**
*
* The data binding information for the component's properties.
*
*
* @param bindingProperties
* The data binding information for the component's properties.
*/
public void setBindingProperties(java.util.Map bindingProperties) {
this.bindingProperties = bindingProperties;
}
/**
*
* The data binding information for the component's properties.
*
*
* @param bindingProperties
* The data binding information for the component's properties.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateComponentData withBindingProperties(java.util.Map bindingProperties) {
setBindingProperties(bindingProperties);
return this;
}
/**
* Add a single BindingProperties entry
*
* @see UpdateComponentData#withBindingProperties
* @returns a reference to this object so that method calls can be chained together.
*/
public UpdateComponentData addBindingPropertiesEntry(String key, ComponentBindingPropertiesValue value) {
if (null == this.bindingProperties) {
this.bindingProperties = new java.util.HashMap();
}
if (this.bindingProperties.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.bindingProperties.put(key, value);
return this;
}
/**
* Removes all the entries added into BindingProperties.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateComponentData clearBindingPropertiesEntries() {
this.bindingProperties = null;
return this;
}
/**
*
* The configuration for binding a component's properties to a data model. Use this for a collection component.
*
*
* @return The configuration for binding a component's properties to a data model. Use this for a collection
* component.
*/
public java.util.Map getCollectionProperties() {
return collectionProperties;
}
/**
*
* The configuration for binding a component's properties to a data model. Use this for a collection component.
*
*
* @param collectionProperties
* The configuration for binding a component's properties to a data model. Use this for a collection
* component.
*/
public void setCollectionProperties(java.util.Map collectionProperties) {
this.collectionProperties = collectionProperties;
}
/**
*
* The configuration for binding a component's properties to a data model. Use this for a collection component.
*
*
* @param collectionProperties
* The configuration for binding a component's properties to a data model. Use this for a collection
* component.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateComponentData withCollectionProperties(java.util.Map collectionProperties) {
setCollectionProperties(collectionProperties);
return this;
}
/**
* Add a single CollectionProperties entry
*
* @see UpdateComponentData#withCollectionProperties
* @returns a reference to this object so that method calls can be chained together.
*/
public UpdateComponentData addCollectionPropertiesEntry(String key, ComponentDataConfiguration value) {
if (null == this.collectionProperties) {
this.collectionProperties = new java.util.HashMap();
}
if (this.collectionProperties.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.collectionProperties.put(key, value);
return this;
}
/**
* Removes all the entries added into CollectionProperties.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateComponentData clearCollectionPropertiesEntries() {
this.collectionProperties = null;
return this;
}
/**
*
* The event configuration for the component. Use for the workflow feature in Amplify Studio that allows you to bind
* events and actions to components.
*
*
* @return The event configuration for the component. Use for the workflow feature in Amplify Studio that allows you
* to bind events and actions to components.
*/
public java.util.Map getEvents() {
return events;
}
/**
*
* The event configuration for the component. Use for the workflow feature in Amplify Studio that allows you to bind
* events and actions to components.
*
*
* @param events
* The event configuration for the component. Use for the workflow feature in Amplify Studio that allows you
* to bind events and actions to components.
*/
public void setEvents(java.util.Map events) {
this.events = events;
}
/**
*
* The event configuration for the component. Use for the workflow feature in Amplify Studio that allows you to bind
* events and actions to components.
*
*
* @param events
* The event configuration for the component. Use for the workflow feature in Amplify Studio that allows you
* to bind events and actions to components.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateComponentData withEvents(java.util.Map events) {
setEvents(events);
return this;
}
/**
* Add a single Events entry
*
* @see UpdateComponentData#withEvents
* @returns a reference to this object so that method calls can be chained together.
*/
public UpdateComponentData addEventsEntry(String key, ComponentEvent value) {
if (null == this.events) {
this.events = new java.util.HashMap();
}
if (this.events.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.events.put(key, value);
return this;
}
/**
* Removes all the entries added into Events.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateComponentData clearEventsEntries() {
this.events = null;
return this;
}
/**
*
* The schema version of the component when it was imported.
*
*
* @param schemaVersion
* The schema version of the component when it was imported.
*/
public void setSchemaVersion(String schemaVersion) {
this.schemaVersion = schemaVersion;
}
/**
*
* The schema version of the component when it was imported.
*
*
* @return The schema version of the component when it was imported.
*/
public String getSchemaVersion() {
return this.schemaVersion;
}
/**
*
* The schema version of the component when it was imported.
*
*
* @param schemaVersion
* The schema version of the component when it was imported.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateComponentData withSchemaVersion(String schemaVersion) {
setSchemaVersion(schemaVersion);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getId() != null)
sb.append("Id: ").append(getId()).append(",");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getSourceId() != null)
sb.append("SourceId: ").append(getSourceId()).append(",");
if (getComponentType() != null)
sb.append("ComponentType: ").append(getComponentType()).append(",");
if (getProperties() != null)
sb.append("Properties: ").append(getProperties()).append(",");
if (getChildren() != null)
sb.append("Children: ").append(getChildren()).append(",");
if (getVariants() != null)
sb.append("Variants: ").append(getVariants()).append(",");
if (getOverrides() != null)
sb.append("Overrides: ").append(getOverrides()).append(",");
if (getBindingProperties() != null)
sb.append("BindingProperties: ").append(getBindingProperties()).append(",");
if (getCollectionProperties() != null)
sb.append("CollectionProperties: ").append(getCollectionProperties()).append(",");
if (getEvents() != null)
sb.append("Events: ").append(getEvents()).append(",");
if (getSchemaVersion() != null)
sb.append("SchemaVersion: ").append(getSchemaVersion());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof UpdateComponentData == false)
return false;
UpdateComponentData other = (UpdateComponentData) obj;
if (other.getId() == null ^ this.getId() == null)
return false;
if (other.getId() != null && other.getId().equals(this.getId()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getSourceId() == null ^ this.getSourceId() == null)
return false;
if (other.getSourceId() != null && other.getSourceId().equals(this.getSourceId()) == false)
return false;
if (other.getComponentType() == null ^ this.getComponentType() == null)
return false;
if (other.getComponentType() != null && other.getComponentType().equals(this.getComponentType()) == false)
return false;
if (other.getProperties() == null ^ this.getProperties() == null)
return false;
if (other.getProperties() != null && other.getProperties().equals(this.getProperties()) == false)
return false;
if (other.getChildren() == null ^ this.getChildren() == null)
return false;
if (other.getChildren() != null && other.getChildren().equals(this.getChildren()) == false)
return false;
if (other.getVariants() == null ^ this.getVariants() == null)
return false;
if (other.getVariants() != null && other.getVariants().equals(this.getVariants()) == false)
return false;
if (other.getOverrides() == null ^ this.getOverrides() == null)
return false;
if (other.getOverrides() != null && other.getOverrides().equals(this.getOverrides()) == false)
return false;
if (other.getBindingProperties() == null ^ this.getBindingProperties() == null)
return false;
if (other.getBindingProperties() != null && other.getBindingProperties().equals(this.getBindingProperties()) == false)
return false;
if (other.getCollectionProperties() == null ^ this.getCollectionProperties() == null)
return false;
if (other.getCollectionProperties() != null && other.getCollectionProperties().equals(this.getCollectionProperties()) == false)
return false;
if (other.getEvents() == null ^ this.getEvents() == null)
return false;
if (other.getEvents() != null && other.getEvents().equals(this.getEvents()) == false)
return false;
if (other.getSchemaVersion() == null ^ this.getSchemaVersion() == null)
return false;
if (other.getSchemaVersion() != null && other.getSchemaVersion().equals(this.getSchemaVersion()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getId() == null) ? 0 : getId().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getSourceId() == null) ? 0 : getSourceId().hashCode());
hashCode = prime * hashCode + ((getComponentType() == null) ? 0 : getComponentType().hashCode());
hashCode = prime * hashCode + ((getProperties() == null) ? 0 : getProperties().hashCode());
hashCode = prime * hashCode + ((getChildren() == null) ? 0 : getChildren().hashCode());
hashCode = prime * hashCode + ((getVariants() == null) ? 0 : getVariants().hashCode());
hashCode = prime * hashCode + ((getOverrides() == null) ? 0 : getOverrides().hashCode());
hashCode = prime * hashCode + ((getBindingProperties() == null) ? 0 : getBindingProperties().hashCode());
hashCode = prime * hashCode + ((getCollectionProperties() == null) ? 0 : getCollectionProperties().hashCode());
hashCode = prime * hashCode + ((getEvents() == null) ? 0 : getEvents().hashCode());
hashCode = prime * hashCode + ((getSchemaVersion() == null) ? 0 : getSchemaVersion().hashCode());
return hashCode;
}
@Override
public UpdateComponentData clone() {
try {
return (UpdateComponentData) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.amplifyuibuilder.model.transform.UpdateComponentDataMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}