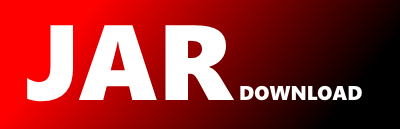
com.amazonaws.services.appfabric.AWSAppFabric Maven / Gradle / Ivy
Show all versions of aws-java-sdk-appfabric Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.appfabric;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.appfabric.model.*;
/**
* Interface for accessing AppFabric.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.appfabric.AbstractAWSAppFabric} instead.
*
*
*
* Amazon Web Services AppFabric quickly connects software as a service (SaaS) applications across your organization.
* This allows IT and security teams to easily manage and secure applications using a standard schema, and employees can
* complete everyday tasks faster using generative artificial intelligence (AI). You can use these APIs to complete
* AppFabric tasks, such as setting up audit log ingestions or viewing user access. For more information about
* AppFabric, including the required permissions to use the service, see the Amazon Web Services AppFabric Administration
* Guide. For more information about using the Command Line Interface (CLI) to manage your AppFabric resources, see
* the AppFabric section of the CLI
* Reference.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSAppFabric {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "appfabric";
/**
*
* Gets user access details in a batch request.
*
*
* This action polls data from the tasks that are kicked off by the StartUserAccessTasks
action.
*
*
* @param batchGetUserAccessTasksRequest
* @return Result of the BatchGetUserAccessTasks operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure with an internal
* server.
* @throws ThrottlingException
* The request rate exceeds the limit.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws AccessDeniedException
* You are not authorized to perform this operation.
* @sample AWSAppFabric.BatchGetUserAccessTasks
* @see AWS API Documentation
*/
BatchGetUserAccessTasksResult batchGetUserAccessTasks(BatchGetUserAccessTasksRequest batchGetUserAccessTasksRequest);
/**
*
* Establishes a connection between Amazon Web Services AppFabric and an application, which allows AppFabric to call
* the APIs of the application.
*
*
* @param connectAppAuthorizationRequest
* @return Result of the ConnectAppAuthorization operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure with an internal
* server.
* @throws ThrottlingException
* The request rate exceeds the limit.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws AccessDeniedException
* You are not authorized to perform this operation.
* @sample AWSAppFabric.ConnectAppAuthorization
* @see AWS API Documentation
*/
ConnectAppAuthorizationResult connectAppAuthorization(ConnectAppAuthorizationRequest connectAppAuthorizationRequest);
/**
*
* Creates an app authorization within an app bundle, which allows AppFabric to connect to an application.
*
*
* @param createAppAuthorizationRequest
* @return Result of the CreateAppAuthorization operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure with an internal
* server.
* @throws ServiceQuotaExceededException
* The request exceeds a service quota.
* @throws ThrottlingException
* The request rate exceeds the limit.
* @throws ConflictException
* The request has created a conflict. Check the request parameters and try again.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws AccessDeniedException
* You are not authorized to perform this operation.
* @sample AWSAppFabric.CreateAppAuthorization
* @see AWS API Documentation
*/
CreateAppAuthorizationResult createAppAuthorization(CreateAppAuthorizationRequest createAppAuthorizationRequest);
/**
*
* Creates an app bundle to collect data from an application using AppFabric.
*
*
* @param createAppBundleRequest
* @return Result of the CreateAppBundle operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure with an internal
* server.
* @throws ServiceQuotaExceededException
* The request exceeds a service quota.
* @throws ThrottlingException
* The request rate exceeds the limit.
* @throws ConflictException
* The request has created a conflict. Check the request parameters and try again.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws AccessDeniedException
* You are not authorized to perform this operation.
* @sample AWSAppFabric.CreateAppBundle
* @see AWS API
* Documentation
*/
CreateAppBundleResult createAppBundle(CreateAppBundleRequest createAppBundleRequest);
/**
*
* Creates a data ingestion for an application.
*
*
* @param createIngestionRequest
* @return Result of the CreateIngestion operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure with an internal
* server.
* @throws ServiceQuotaExceededException
* The request exceeds a service quota.
* @throws ThrottlingException
* The request rate exceeds the limit.
* @throws ConflictException
* The request has created a conflict. Check the request parameters and try again.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws AccessDeniedException
* You are not authorized to perform this operation.
* @sample AWSAppFabric.CreateIngestion
* @see AWS API
* Documentation
*/
CreateIngestionResult createIngestion(CreateIngestionRequest createIngestionRequest);
/**
*
* Creates an ingestion destination, which specifies how an application's ingested data is processed by Amazon Web
* Services AppFabric and where it's delivered.
*
*
* @param createIngestionDestinationRequest
* @return Result of the CreateIngestionDestination operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure with an internal
* server.
* @throws ServiceQuotaExceededException
* The request exceeds a service quota.
* @throws ThrottlingException
* The request rate exceeds the limit.
* @throws ConflictException
* The request has created a conflict. Check the request parameters and try again.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws AccessDeniedException
* You are not authorized to perform this operation.
* @sample AWSAppFabric.CreateIngestionDestination
* @see AWS API Documentation
*/
CreateIngestionDestinationResult createIngestionDestination(CreateIngestionDestinationRequest createIngestionDestinationRequest);
/**
*
* Deletes an app authorization. You must delete the associated ingestion before you can delete an app
* authorization.
*
*
* @param deleteAppAuthorizationRequest
* @return Result of the DeleteAppAuthorization operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure with an internal
* server.
* @throws ThrottlingException
* The request rate exceeds the limit.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws AccessDeniedException
* You are not authorized to perform this operation.
* @sample AWSAppFabric.DeleteAppAuthorization
* @see AWS API Documentation
*/
DeleteAppAuthorizationResult deleteAppAuthorization(DeleteAppAuthorizationRequest deleteAppAuthorizationRequest);
/**
*
* Deletes an app bundle. You must delete all associated app authorizations before you can delete an app bundle.
*
*
* @param deleteAppBundleRequest
* @return Result of the DeleteAppBundle operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure with an internal
* server.
* @throws ThrottlingException
* The request rate exceeds the limit.
* @throws ConflictException
* The request has created a conflict. Check the request parameters and try again.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws AccessDeniedException
* You are not authorized to perform this operation.
* @sample AWSAppFabric.DeleteAppBundle
* @see AWS API
* Documentation
*/
DeleteAppBundleResult deleteAppBundle(DeleteAppBundleRequest deleteAppBundleRequest);
/**
*
* Deletes an ingestion. You must stop (disable) the ingestion and you must delete all associated ingestion
* destinations before you can delete an app ingestion.
*
*
* @param deleteIngestionRequest
* @return Result of the DeleteIngestion operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure with an internal
* server.
* @throws ThrottlingException
* The request rate exceeds the limit.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws AccessDeniedException
* You are not authorized to perform this operation.
* @sample AWSAppFabric.DeleteIngestion
* @see AWS API
* Documentation
*/
DeleteIngestionResult deleteIngestion(DeleteIngestionRequest deleteIngestionRequest);
/**
*
* Deletes an ingestion destination.
*
*
* This deletes the association between an ingestion and it's destination. It doesn't delete previously ingested
* data or the storage destination, such as the Amazon S3 bucket where the data is delivered. If the ingestion
* destination is deleted while the associated ingestion is enabled, the ingestion will fail and is eventually
* disabled.
*
*
* @param deleteIngestionDestinationRequest
* @return Result of the DeleteIngestionDestination operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure with an internal
* server.
* @throws ThrottlingException
* The request rate exceeds the limit.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws AccessDeniedException
* You are not authorized to perform this operation.
* @sample AWSAppFabric.DeleteIngestionDestination
* @see AWS API Documentation
*/
DeleteIngestionDestinationResult deleteIngestionDestination(DeleteIngestionDestinationRequest deleteIngestionDestinationRequest);
/**
*
* Returns information about an app authorization.
*
*
* @param getAppAuthorizationRequest
* @return Result of the GetAppAuthorization operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure with an internal
* server.
* @throws ThrottlingException
* The request rate exceeds the limit.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws AccessDeniedException
* You are not authorized to perform this operation.
* @sample AWSAppFabric.GetAppAuthorization
* @see AWS
* API Documentation
*/
GetAppAuthorizationResult getAppAuthorization(GetAppAuthorizationRequest getAppAuthorizationRequest);
/**
*
* Returns information about an app bundle.
*
*
* @param getAppBundleRequest
* @return Result of the GetAppBundle operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure with an internal
* server.
* @throws ThrottlingException
* The request rate exceeds the limit.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws AccessDeniedException
* You are not authorized to perform this operation.
* @sample AWSAppFabric.GetAppBundle
* @see AWS API
* Documentation
*/
GetAppBundleResult getAppBundle(GetAppBundleRequest getAppBundleRequest);
/**
*
* Returns information about an ingestion.
*
*
* @param getIngestionRequest
* @return Result of the GetIngestion operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure with an internal
* server.
* @throws ThrottlingException
* The request rate exceeds the limit.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws AccessDeniedException
* You are not authorized to perform this operation.
* @sample AWSAppFabric.GetIngestion
* @see AWS API
* Documentation
*/
GetIngestionResult getIngestion(GetIngestionRequest getIngestionRequest);
/**
*
* Returns information about an ingestion destination.
*
*
* @param getIngestionDestinationRequest
* @return Result of the GetIngestionDestination operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure with an internal
* server.
* @throws ThrottlingException
* The request rate exceeds the limit.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws AccessDeniedException
* You are not authorized to perform this operation.
* @sample AWSAppFabric.GetIngestionDestination
* @see AWS API Documentation
*/
GetIngestionDestinationResult getIngestionDestination(GetIngestionDestinationRequest getIngestionDestinationRequest);
/**
*
* Returns a list of all app authorizations configured for an app bundle.
*
*
* @param listAppAuthorizationsRequest
* @return Result of the ListAppAuthorizations operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure with an internal
* server.
* @throws ThrottlingException
* The request rate exceeds the limit.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws AccessDeniedException
* You are not authorized to perform this operation.
* @sample AWSAppFabric.ListAppAuthorizations
* @see AWS API Documentation
*/
ListAppAuthorizationsResult listAppAuthorizations(ListAppAuthorizationsRequest listAppAuthorizationsRequest);
/**
*
* Returns a list of app bundles.
*
*
* @param listAppBundlesRequest
* @return Result of the ListAppBundles operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure with an internal
* server.
* @throws ThrottlingException
* The request rate exceeds the limit.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws AccessDeniedException
* You are not authorized to perform this operation.
* @sample AWSAppFabric.ListAppBundles
* @see AWS API
* Documentation
*/
ListAppBundlesResult listAppBundles(ListAppBundlesRequest listAppBundlesRequest);
/**
*
* Returns a list of all ingestion destinations configured for an ingestion.
*
*
* @param listIngestionDestinationsRequest
* @return Result of the ListIngestionDestinations operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure with an internal
* server.
* @throws ThrottlingException
* The request rate exceeds the limit.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws AccessDeniedException
* You are not authorized to perform this operation.
* @sample AWSAppFabric.ListIngestionDestinations
* @see AWS API Documentation
*/
ListIngestionDestinationsResult listIngestionDestinations(ListIngestionDestinationsRequest listIngestionDestinationsRequest);
/**
*
* Returns a list of all ingestions configured for an app bundle.
*
*
* @param listIngestionsRequest
* @return Result of the ListIngestions operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure with an internal
* server.
* @throws ThrottlingException
* The request rate exceeds the limit.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws AccessDeniedException
* You are not authorized to perform this operation.
* @sample AWSAppFabric.ListIngestions
* @see AWS API
* Documentation
*/
ListIngestionsResult listIngestions(ListIngestionsRequest listIngestionsRequest);
/**
*
* Returns a list of tags for a resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure with an internal
* server.
* @throws ThrottlingException
* The request rate exceeds the limit.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws AccessDeniedException
* You are not authorized to perform this operation.
* @sample AWSAppFabric.ListTagsForResource
* @see AWS
* API Documentation
*/
ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Starts (enables) an ingestion, which collects data from an application.
*
*
* @param startIngestionRequest
* @return Result of the StartIngestion operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure with an internal
* server.
* @throws ThrottlingException
* The request rate exceeds the limit.
* @throws ConflictException
* The request has created a conflict. Check the request parameters and try again.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws AccessDeniedException
* You are not authorized to perform this operation.
* @sample AWSAppFabric.StartIngestion
* @see AWS API
* Documentation
*/
StartIngestionResult startIngestion(StartIngestionRequest startIngestionRequest);
/**
*
* Starts the tasks to search user access status for a specific email address.
*
*
* The tasks are stopped when the user access status data is found. The tasks are terminated when the API calls to
* the application time out.
*
*
* @param startUserAccessTasksRequest
* @return Result of the StartUserAccessTasks operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure with an internal
* server.
* @throws ThrottlingException
* The request rate exceeds the limit.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws AccessDeniedException
* You are not authorized to perform this operation.
* @sample AWSAppFabric.StartUserAccessTasks
* @see AWS
* API Documentation
*/
StartUserAccessTasksResult startUserAccessTasks(StartUserAccessTasksRequest startUserAccessTasksRequest);
/**
*
* Stops (disables) an ingestion.
*
*
* @param stopIngestionRequest
* @return Result of the StopIngestion operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure with an internal
* server.
* @throws ThrottlingException
* The request rate exceeds the limit.
* @throws ConflictException
* The request has created a conflict. Check the request parameters and try again.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws AccessDeniedException
* You are not authorized to perform this operation.
* @sample AWSAppFabric.StopIngestion
* @see AWS API
* Documentation
*/
StopIngestionResult stopIngestion(StopIngestionRequest stopIngestionRequest);
/**
*
* Assigns one or more tags (key-value pairs) to the specified resource.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure with an internal
* server.
* @throws ThrottlingException
* The request rate exceeds the limit.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws AccessDeniedException
* You are not authorized to perform this operation.
* @sample AWSAppFabric.TagResource
* @see AWS API
* Documentation
*/
TagResourceResult tagResource(TagResourceRequest tagResourceRequest);
/**
*
* Removes a tag or tags from a resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure with an internal
* server.
* @throws ThrottlingException
* The request rate exceeds the limit.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws AccessDeniedException
* You are not authorized to perform this operation.
* @sample AWSAppFabric.UntagResource
* @see AWS API
* Documentation
*/
UntagResourceResult untagResource(UntagResourceRequest untagResourceRequest);
/**
*
* Updates an app authorization within an app bundle, which allows AppFabric to connect to an application.
*
*
* If the app authorization was in a connected
state, updating the app authorization will set it back
* to a PendingConnect
state.
*
*
* @param updateAppAuthorizationRequest
* @return Result of the UpdateAppAuthorization operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure with an internal
* server.
* @throws ThrottlingException
* The request rate exceeds the limit.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws AccessDeniedException
* You are not authorized to perform this operation.
* @sample AWSAppFabric.UpdateAppAuthorization
* @see AWS API Documentation
*/
UpdateAppAuthorizationResult updateAppAuthorization(UpdateAppAuthorizationRequest updateAppAuthorizationRequest);
/**
*
* Updates an ingestion destination, which specifies how an application's ingested data is processed by Amazon Web
* Services AppFabric and where it's delivered.
*
*
* @param updateIngestionDestinationRequest
* @return Result of the UpdateIngestionDestination operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception, or failure with an internal
* server.
* @throws ServiceQuotaExceededException
* The request exceeds a service quota.
* @throws ThrottlingException
* The request rate exceeds the limit.
* @throws ConflictException
* The request has created a conflict. Check the request parameters and try again.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws AccessDeniedException
* You are not authorized to perform this operation.
* @sample AWSAppFabric.UpdateIngestionDestination
* @see AWS API Documentation
*/
UpdateIngestionDestinationResult updateIngestionDestination(UpdateIngestionDestinationRequest updateIngestionDestinationRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}