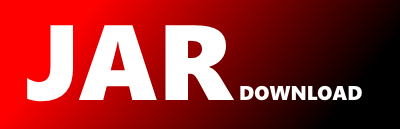
com.amazonaws.services.appflow.AmazonAppflow Maven / Gradle / Ivy
Show all versions of aws-java-sdk-appflow Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.appflow;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.appflow.model.*;
/**
* Interface for accessing Amazon Appflow.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.appflow.AbstractAmazonAppflow} instead.
*
*
*
* Welcome to the Amazon AppFlow API reference. This guide is for developers who need detailed information about the
* Amazon AppFlow API operations, data types, and errors.
*
*
* Amazon AppFlow is a fully managed integration service that enables you to securely transfer data between software as
* a service (SaaS) applications like Salesforce, Marketo, Slack, and ServiceNow, and Amazon Web Services like Amazon S3
* and Amazon Redshift.
*
*
* Use the following links to get started on the Amazon AppFlow API:
*
*
* -
*
* Actions: An alphabetical list
* of all Amazon AppFlow API operations.
*
*
* -
*
* Data types: An alphabetical list of
* all Amazon AppFlow data types.
*
*
* -
*
* Common parameters:
* Parameters that all Query operations can use.
*
*
* -
*
* Common errors: Client and server
* errors that all operations can return.
*
*
*
*
* If you're new to Amazon AppFlow, we recommend that you review the Amazon AppFlow User Guide.
*
*
* Amazon AppFlow API users can use vendor-specific mechanisms for OAuth, and include applicable OAuth attributes (such
* as auth-code
and redirecturi
) with the connector-specific
* ConnectorProfileProperties
when creating a new connector profile using Amazon AppFlow API operations.
* For example, Salesforce users can refer to the Authorize Apps with OAuth
* documentation.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonAppflow {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "appflow";
/**
*
* Cancels active runs for a flow.
*
*
* You can cancel all of the active runs for a flow, or you can cancel specific runs by providing their IDs.
*
*
* You can cancel a flow run only when the run is in progress. You can't cancel a run that has already completed or
* failed. You also can't cancel a run that's scheduled to occur but hasn't started yet. To prevent a scheduled run,
* you can deactivate the flow with the StopFlow
action.
*
*
* You cannot resume a run after you cancel it.
*
*
* When you send your request, the status for each run becomes CancelStarted
. When the cancellation
* completes, the status becomes Canceled
.
*
*
*
* When you cancel a run, you still incur charges for any data that the run already processed before the
* cancellation. If the run had already written some data to the flow destination, then that data remains in the
* destination. If you configured the flow to use a batch API (such as the Salesforce Bulk API 2.0), then the run
* will finish reading or writing its entire batch of data after the cancellation. For these operations, the data
* processing charges for Amazon AppFlow apply. For the pricing information, see Amazon AppFlow pricing.
*
*
*
* @param cancelFlowExecutionsRequest
* @return Result of the CancelFlowExecutions operation returned by the service.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws AccessDeniedException
* AppFlow/Requester has invalid or missing permissions.
* @throws ResourceNotFoundException
* The resource specified in the request (such as the source or destination connector profile) is not found.
* @throws ThrottlingException
* API calls have exceeded the maximum allowed API request rate per account and per Region.
* @throws InternalServerException
* An internal service error occurred during the processing of your request. Try again later.
* @sample AmazonAppflow.CancelFlowExecutions
* @see AWS
* API Documentation
*/
CancelFlowExecutionsResult cancelFlowExecutions(CancelFlowExecutionsRequest cancelFlowExecutionsRequest);
/**
*
* Creates a new connector profile associated with your Amazon Web Services account. There is a soft quota of 100
* connector profiles per Amazon Web Services account. If you need more connector profiles than this quota allows,
* you can submit a request to the Amazon AppFlow team through the Amazon AppFlow support channel. In each connector
* profile that you create, you can provide the credentials and properties for only one connector.
*
*
* @param createConnectorProfileRequest
* @return Result of the CreateConnectorProfile operation returned by the service.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws ConflictException
* There was a conflict when processing the request (for example, a flow with the given name already exists
* within the account. Check for conflicting resource names and try again.
* @throws ServiceQuotaExceededException
* The request would cause a service quota (such as the number of flows) to be exceeded.
* @throws ConnectorAuthenticationException
* An error occurred when authenticating with the connector endpoint.
* @throws InternalServerException
* An internal service error occurred during the processing of your request. Try again later.
* @sample AmazonAppflow.CreateConnectorProfile
* @see AWS
* API Documentation
*/
CreateConnectorProfileResult createConnectorProfile(CreateConnectorProfileRequest createConnectorProfileRequest);
/**
*
* Enables your application to create a new flow using Amazon AppFlow. You must create a connector profile before
* calling this API. Please note that the Request Syntax below shows syntax for multiple destinations, however, you
* can only transfer data to one item in this list at a time. Amazon AppFlow does not currently support flows to
* multiple destinations at once.
*
*
* @param createFlowRequest
* @return Result of the CreateFlow operation returned by the service.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws InternalServerException
* An internal service error occurred during the processing of your request. Try again later.
* @throws ResourceNotFoundException
* The resource specified in the request (such as the source or destination connector profile) is not found.
* @throws ServiceQuotaExceededException
* The request would cause a service quota (such as the number of flows) to be exceeded.
* @throws ConflictException
* There was a conflict when processing the request (for example, a flow with the given name already exists
* within the account. Check for conflicting resource names and try again.
* @throws ConnectorAuthenticationException
* An error occurred when authenticating with the connector endpoint.
* @throws ConnectorServerException
* An error occurred when retrieving data from the connector endpoint.
* @throws AccessDeniedException
* AppFlow/Requester has invalid or missing permissions.
* @sample AmazonAppflow.CreateFlow
* @see AWS API
* Documentation
*/
CreateFlowResult createFlow(CreateFlowRequest createFlowRequest);
/**
*
* Enables you to delete an existing connector profile.
*
*
* @param deleteConnectorProfileRequest
* @return Result of the DeleteConnectorProfile operation returned by the service.
* @throws ResourceNotFoundException
* The resource specified in the request (such as the source or destination connector profile) is not found.
* @throws ConflictException
* There was a conflict when processing the request (for example, a flow with the given name already exists
* within the account. Check for conflicting resource names and try again.
* @throws InternalServerException
* An internal service error occurred during the processing of your request. Try again later.
* @sample AmazonAppflow.DeleteConnectorProfile
* @see AWS
* API Documentation
*/
DeleteConnectorProfileResult deleteConnectorProfile(DeleteConnectorProfileRequest deleteConnectorProfileRequest);
/**
*
* Enables your application to delete an existing flow. Before deleting the flow, Amazon AppFlow validates the
* request by checking the flow configuration and status. You can delete flows one at a time.
*
*
* @param deleteFlowRequest
* @return Result of the DeleteFlow operation returned by the service.
* @throws ResourceNotFoundException
* The resource specified in the request (such as the source or destination connector profile) is not found.
* @throws ConflictException
* There was a conflict when processing the request (for example, a flow with the given name already exists
* within the account. Check for conflicting resource names and try again.
* @throws InternalServerException
* An internal service error occurred during the processing of your request. Try again later.
* @sample AmazonAppflow.DeleteFlow
* @see AWS API
* Documentation
*/
DeleteFlowResult deleteFlow(DeleteFlowRequest deleteFlowRequest);
/**
*
* Describes the given custom connector registered in your Amazon Web Services account. This API can be used for
* custom connectors that are registered in your account and also for Amazon authored connectors.
*
*
* @param describeConnectorRequest
* @return Result of the DescribeConnector operation returned by the service.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws InternalServerException
* An internal service error occurred during the processing of your request. Try again later.
* @throws ResourceNotFoundException
* The resource specified in the request (such as the source or destination connector profile) is not found.
* @sample AmazonAppflow.DescribeConnector
* @see AWS API
* Documentation
*/
DescribeConnectorResult describeConnector(DescribeConnectorRequest describeConnectorRequest);
/**
*
* Provides details regarding the entity used with the connector, with a description of the data model for each
* field in that entity.
*
*
* @param describeConnectorEntityRequest
* @return Result of the DescribeConnectorEntity operation returned by the service.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws ResourceNotFoundException
* The resource specified in the request (such as the source or destination connector profile) is not found.
* @throws ConnectorAuthenticationException
* An error occurred when authenticating with the connector endpoint.
* @throws ConnectorServerException
* An error occurred when retrieving data from the connector endpoint.
* @throws InternalServerException
* An internal service error occurred during the processing of your request. Try again later.
* @sample AmazonAppflow.DescribeConnectorEntity
* @see AWS API Documentation
*/
DescribeConnectorEntityResult describeConnectorEntity(DescribeConnectorEntityRequest describeConnectorEntityRequest);
/**
*
* Returns a list of connector-profile
details matching the provided connector-profile
* names and connector-types
. Both input lists are optional, and you can use them to filter the result.
*
*
* If no names or connector-types
are provided, returns all connector profiles in a paginated form. If
* there is no match, this operation returns an empty list.
*
*
* @param describeConnectorProfilesRequest
* @return Result of the DescribeConnectorProfiles operation returned by the service.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws InternalServerException
* An internal service error occurred during the processing of your request. Try again later.
* @sample AmazonAppflow.DescribeConnectorProfiles
* @see AWS API Documentation
*/
DescribeConnectorProfilesResult describeConnectorProfiles(DescribeConnectorProfilesRequest describeConnectorProfilesRequest);
/**
*
* Describes the connectors vended by Amazon AppFlow for specified connector types. If you don't specify a connector
* type, this operation describes all connectors vended by Amazon AppFlow. If there are more connectors than can be
* returned in one page, the response contains a nextToken
object, which can be be passed in to the
* next call to the DescribeConnectors
API operation to retrieve the next page.
*
*
* @param describeConnectorsRequest
* @return Result of the DescribeConnectors operation returned by the service.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws InternalServerException
* An internal service error occurred during the processing of your request. Try again later.
* @sample AmazonAppflow.DescribeConnectors
* @see AWS API
* Documentation
*/
DescribeConnectorsResult describeConnectors(DescribeConnectorsRequest describeConnectorsRequest);
/**
*
* Provides a description of the specified flow.
*
*
* @param describeFlowRequest
* @return Result of the DescribeFlow operation returned by the service.
* @throws ResourceNotFoundException
* The resource specified in the request (such as the source or destination connector profile) is not found.
* @throws InternalServerException
* An internal service error occurred during the processing of your request. Try again later.
* @sample AmazonAppflow.DescribeFlow
* @see AWS API
* Documentation
*/
DescribeFlowResult describeFlow(DescribeFlowRequest describeFlowRequest);
/**
*
* Fetches the execution history of the flow.
*
*
* @param describeFlowExecutionRecordsRequest
* @return Result of the DescribeFlowExecutionRecords operation returned by the service.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws ResourceNotFoundException
* The resource specified in the request (such as the source or destination connector profile) is not found.
* @throws InternalServerException
* An internal service error occurred during the processing of your request. Try again later.
* @sample AmazonAppflow.DescribeFlowExecutionRecords
* @see AWS API Documentation
*/
DescribeFlowExecutionRecordsResult describeFlowExecutionRecords(DescribeFlowExecutionRecordsRequest describeFlowExecutionRecordsRequest);
/**
*
* Returns the list of available connector entities supported by Amazon AppFlow. For example, you can query
* Salesforce for Account and Opportunity entities, or query ServiceNow for the Incident
* entity.
*
*
* @param listConnectorEntitiesRequest
* @return Result of the ListConnectorEntities operation returned by the service.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws ResourceNotFoundException
* The resource specified in the request (such as the source or destination connector profile) is not found.
* @throws ConnectorAuthenticationException
* An error occurred when authenticating with the connector endpoint.
* @throws ConnectorServerException
* An error occurred when retrieving data from the connector endpoint.
* @throws InternalServerException
* An internal service error occurred during the processing of your request. Try again later.
* @sample AmazonAppflow.ListConnectorEntities
* @see AWS
* API Documentation
*/
ListConnectorEntitiesResult listConnectorEntities(ListConnectorEntitiesRequest listConnectorEntitiesRequest);
/**
*
* Returns the list of all registered custom connectors in your Amazon Web Services account. This API lists only
* custom connectors registered in this account, not the Amazon Web Services authored connectors.
*
*
* @param listConnectorsRequest
* @return Result of the ListConnectors operation returned by the service.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws InternalServerException
* An internal service error occurred during the processing of your request. Try again later.
* @sample AmazonAppflow.ListConnectors
* @see AWS API
* Documentation
*/
ListConnectorsResult listConnectors(ListConnectorsRequest listConnectorsRequest);
/**
*
* Lists all of the flows associated with your account.
*
*
* @param listFlowsRequest
* @return Result of the ListFlows operation returned by the service.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws InternalServerException
* An internal service error occurred during the processing of your request. Try again later.
* @sample AmazonAppflow.ListFlows
* @see AWS API
* Documentation
*/
ListFlowsResult listFlows(ListFlowsRequest listFlowsRequest);
/**
*
* Retrieves the tags that are associated with a specified flow.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws InternalServerException
* An internal service error occurred during the processing of your request. Try again later.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws ResourceNotFoundException
* The resource specified in the request (such as the source or destination connector profile) is not found.
* @sample AmazonAppflow.ListTagsForResource
* @see AWS
* API Documentation
*/
ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Registers a new custom connector with your Amazon Web Services account. Before you can register the connector,
* you must deploy the associated AWS lambda function in your account.
*
*
* @param registerConnectorRequest
* @return Result of the RegisterConnector operation returned by the service.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws ConflictException
* There was a conflict when processing the request (for example, a flow with the given name already exists
* within the account. Check for conflicting resource names and try again.
* @throws AccessDeniedException
* AppFlow/Requester has invalid or missing permissions.
* @throws ResourceNotFoundException
* The resource specified in the request (such as the source or destination connector profile) is not found.
* @throws ServiceQuotaExceededException
* The request would cause a service quota (such as the number of flows) to be exceeded.
* @throws ThrottlingException
* API calls have exceeded the maximum allowed API request rate per account and per Region.
* @throws InternalServerException
* An internal service error occurred during the processing of your request. Try again later.
* @throws ConnectorServerException
* An error occurred when retrieving data from the connector endpoint.
* @throws ConnectorAuthenticationException
* An error occurred when authenticating with the connector endpoint.
* @sample AmazonAppflow.RegisterConnector
* @see AWS API
* Documentation
*/
RegisterConnectorResult registerConnector(RegisterConnectorRequest registerConnectorRequest);
/**
*
* Resets metadata about your connector entities that Amazon AppFlow stored in its cache. Use this action when you
* want Amazon AppFlow to return the latest information about the data that you have in a source application.
*
*
* Amazon AppFlow returns metadata about your entities when you use the ListConnectorEntities or
* DescribeConnectorEntities actions. Following these actions, Amazon AppFlow caches the metadata to reduce the
* number of API requests that it must send to the source application. Amazon AppFlow automatically resets the cache
* once every hour, but you can use this action when you want to get the latest metadata right away.
*
*
* @param resetConnectorMetadataCacheRequest
* @return Result of the ResetConnectorMetadataCache operation returned by the service.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws ResourceNotFoundException
* The resource specified in the request (such as the source or destination connector profile) is not found.
* @throws ConflictException
* There was a conflict when processing the request (for example, a flow with the given name already exists
* within the account. Check for conflicting resource names and try again.
* @throws InternalServerException
* An internal service error occurred during the processing of your request. Try again later.
* @sample AmazonAppflow.ResetConnectorMetadataCache
* @see AWS API Documentation
*/
ResetConnectorMetadataCacheResult resetConnectorMetadataCache(ResetConnectorMetadataCacheRequest resetConnectorMetadataCacheRequest);
/**
*
* Activates an existing flow. For on-demand flows, this operation runs the flow immediately. For schedule and
* event-triggered flows, this operation activates the flow.
*
*
* @param startFlowRequest
* @return Result of the StartFlow operation returned by the service.
* @throws ResourceNotFoundException
* The resource specified in the request (such as the source or destination connector profile) is not found.
* @throws InternalServerException
* An internal service error occurred during the processing of your request. Try again later.
* @throws ServiceQuotaExceededException
* The request would cause a service quota (such as the number of flows) to be exceeded.
* @throws ConflictException
* There was a conflict when processing the request (for example, a flow with the given name already exists
* within the account. Check for conflicting resource names and try again.
* @sample AmazonAppflow.StartFlow
* @see AWS API
* Documentation
*/
StartFlowResult startFlow(StartFlowRequest startFlowRequest);
/**
*
* Deactivates the existing flow. For on-demand flows, this operation returns an
* unsupportedOperationException
error message. For schedule and event-triggered flows, this operation
* deactivates the flow.
*
*
* @param stopFlowRequest
* @return Result of the StopFlow operation returned by the service.
* @throws ConflictException
* There was a conflict when processing the request (for example, a flow with the given name already exists
* within the account. Check for conflicting resource names and try again.
* @throws ResourceNotFoundException
* The resource specified in the request (such as the source or destination connector profile) is not found.
* @throws UnsupportedOperationException
* The requested operation is not supported for the current flow.
* @throws InternalServerException
* An internal service error occurred during the processing of your request. Try again later.
* @sample AmazonAppflow.StopFlow
* @see AWS API
* Documentation
*/
StopFlowResult stopFlow(StopFlowRequest stopFlowRequest);
/**
*
* Applies a tag to the specified flow.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws InternalServerException
* An internal service error occurred during the processing of your request. Try again later.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws ResourceNotFoundException
* The resource specified in the request (such as the source or destination connector profile) is not found.
* @sample AmazonAppflow.TagResource
* @see AWS API
* Documentation
*/
TagResourceResult tagResource(TagResourceRequest tagResourceRequest);
/**
*
* Unregisters the custom connector registered in your account that matches the connector label provided in the
* request.
*
*
* @param unregisterConnectorRequest
* @return Result of the UnregisterConnector operation returned by the service.
* @throws ResourceNotFoundException
* The resource specified in the request (such as the source or destination connector profile) is not found.
* @throws ConflictException
* There was a conflict when processing the request (for example, a flow with the given name already exists
* within the account. Check for conflicting resource names and try again.
* @throws InternalServerException
* An internal service error occurred during the processing of your request. Try again later.
* @sample AmazonAppflow.UnregisterConnector
* @see AWS
* API Documentation
*/
UnregisterConnectorResult unregisterConnector(UnregisterConnectorRequest unregisterConnectorRequest);
/**
*
* Removes a tag from the specified flow.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws InternalServerException
* An internal service error occurred during the processing of your request. Try again later.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws ResourceNotFoundException
* The resource specified in the request (such as the source or destination connector profile) is not found.
* @sample AmazonAppflow.UntagResource
* @see AWS API
* Documentation
*/
UntagResourceResult untagResource(UntagResourceRequest untagResourceRequest);
/**
*
* Updates a given connector profile associated with your account.
*
*
* @param updateConnectorProfileRequest
* @return Result of the UpdateConnectorProfile operation returned by the service.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws ResourceNotFoundException
* The resource specified in the request (such as the source or destination connector profile) is not found.
* @throws ConflictException
* There was a conflict when processing the request (for example, a flow with the given name already exists
* within the account. Check for conflicting resource names and try again.
* @throws ConnectorAuthenticationException
* An error occurred when authenticating with the connector endpoint.
* @throws InternalServerException
* An internal service error occurred during the processing of your request. Try again later.
* @sample AmazonAppflow.UpdateConnectorProfile
* @see AWS
* API Documentation
*/
UpdateConnectorProfileResult updateConnectorProfile(UpdateConnectorProfileRequest updateConnectorProfileRequest);
/**
*
* Updates a custom connector that you've previously registered. This operation updates the connector with one of
* the following:
*
*
* -
*
* The latest version of the AWS Lambda function that's assigned to the connector
*
*
* -
*
* A new AWS Lambda function that you specify
*
*
*
*
* @param updateConnectorRegistrationRequest
* @return Result of the UpdateConnectorRegistration operation returned by the service.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws ConflictException
* There was a conflict when processing the request (for example, a flow with the given name already exists
* within the account. Check for conflicting resource names and try again.
* @throws AccessDeniedException
* AppFlow/Requester has invalid or missing permissions.
* @throws ResourceNotFoundException
* The resource specified in the request (such as the source or destination connector profile) is not found.
* @throws ServiceQuotaExceededException
* The request would cause a service quota (such as the number of flows) to be exceeded.
* @throws ThrottlingException
* API calls have exceeded the maximum allowed API request rate per account and per Region.
* @throws InternalServerException
* An internal service error occurred during the processing of your request. Try again later.
* @throws ConnectorServerException
* An error occurred when retrieving data from the connector endpoint.
* @throws ConnectorAuthenticationException
* An error occurred when authenticating with the connector endpoint.
* @sample AmazonAppflow.UpdateConnectorRegistration
* @see AWS API Documentation
*/
UpdateConnectorRegistrationResult updateConnectorRegistration(UpdateConnectorRegistrationRequest updateConnectorRegistrationRequest);
/**
*
* Updates an existing flow.
*
*
* @param updateFlowRequest
* @return Result of the UpdateFlow operation returned by the service.
* @throws ValidationException
* The request has invalid or missing parameters.
* @throws ResourceNotFoundException
* The resource specified in the request (such as the source or destination connector profile) is not found.
* @throws ServiceQuotaExceededException
* The request would cause a service quota (such as the number of flows) to be exceeded.
* @throws ConflictException
* There was a conflict when processing the request (for example, a flow with the given name already exists
* within the account. Check for conflicting resource names and try again.
* @throws ConnectorAuthenticationException
* An error occurred when authenticating with the connector endpoint.
* @throws ConnectorServerException
* An error occurred when retrieving data from the connector endpoint.
* @throws InternalServerException
* An internal service error occurred during the processing of your request. Try again later.
* @throws AccessDeniedException
* AppFlow/Requester has invalid or missing permissions.
* @sample AmazonAppflow.UpdateFlow
* @see AWS API
* Documentation
*/
UpdateFlowResult updateFlow(UpdateFlowRequest updateFlowRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}