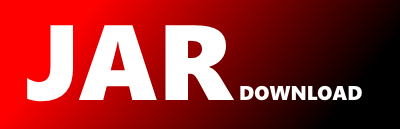
com.amazonaws.services.applicationautoscaling.AWSApplicationAutoScaling Maven / Gradle / Ivy
Show all versions of aws-java-sdk-applicationautoscaling Show documentation
/*
* Copyright 2011-2016 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not
* use this file except in compliance with the License. A copy of the License is
* located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.applicationautoscaling;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.applicationautoscaling.model.*;
/**
* Interface for accessing Application Auto Scaling.
*
*
* Application Auto Scaling is a general purpose Auto Scaling service for
* supported elastic AWS resources. With Application Auto Scaling, you can
* automatically scale your AWS resources, with an experience similar to that of
* Auto Scaling.
*
*
* Application Auto Scaling supports scaling the following AWS resources:
*
*
* -
*
* Amazon ECS services
*
*
* -
*
* Amazon EC2 Spot fleet instances
*
*
*
*
* You can use Application Auto Scaling to accomplish the following tasks:
*
*
* -
*
* Define scaling policies for automatically adjusting your AWS resources
*
*
* -
*
* Scale your resources in response to CloudWatch alarms
*
*
* -
*
* View history of your scaling events
*
*
*
*
* Application Auto Scaling is available in the following regions:
*
*
* -
*
* us-east-1
*
*
* -
*
* us-west-1
*
*
* -
*
* us-west-2
*
*
* -
*
* ap-southeast-1
*
*
* -
*
* ap-southeast-2
*
*
* -
*
* ap-northeast-1
*
*
* -
*
* eu-central-1
*
*
* -
*
* eu-west-1
*
*
*
*/
public interface AWSApplicationAutoScaling {
/**
* The region metadata service name for computing region endpoints. You can
* use this value to retrieve metadata (such as supported regions) of the
* service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "autoscaling";
/**
* Overrides the default endpoint for this client
* ("https://autoscaling.us-east-1.amazonaws.com"). Callers can use this
* method to control which AWS region they want to work with.
*
* Callers can pass in just the endpoint (ex:
* "autoscaling.us-east-1.amazonaws.com") or a full URL, including the
* protocol (ex: "https://autoscaling.us-east-1.amazonaws.com"). If the
* protocol is not specified here, the default protocol from this client's
* {@link ClientConfiguration} will be used, which by default is HTTPS.
*
* For more information on using AWS regions with the AWS SDK for Java, and
* a complete list of all available endpoints for all AWS services, see: http://developer.amazonwebservices.com/connect/entry.jspa?externalID=
* 3912
*
* This method is not threadsafe. An endpoint should be configured when
* the client is created and before any service requests are made. Changing
* it afterwards creates inevitable race conditions for any service requests
* in transit or retrying.
*
* @param endpoint
* The endpoint (ex: "autoscaling.us-east-1.amazonaws.com") or a full
* URL, including the protocol (ex:
* "https://autoscaling.us-east-1.amazonaws.com") of the region
* specific AWS endpoint this client will communicate with.
*/
void setEndpoint(String endpoint);
/**
* An alternative to {@link AWSApplicationAutoScaling#setEndpoint(String)},
* sets the regional endpoint for this client's service calls. Callers can
* use this method to control which AWS region they want to work with.
*
* By default, all service endpoints in all regions use the https protocol.
* To use http instead, specify it in the {@link ClientConfiguration}
* supplied at construction.
*
* This method is not threadsafe. A region should be configured when the
* client is created and before any service requests are made. Changing it
* afterwards creates inevitable race conditions for any service requests in
* transit or retrying.
*
* @param region
* The region this client will communicate with. See
* {@link Region#getRegion(com.amazonaws.regions.Regions)} for
* accessing a given region. Must not be null and must be a region
* where the service is available.
*
* @see Region#getRegion(com.amazonaws.regions.Regions)
* @see Region#createClient(Class,
* com.amazonaws.auth.AWSCredentialsProvider, ClientConfiguration)
* @see Region#isServiceSupported(String)
*/
void setRegion(Region region);
/**
*
* Deletes an Application Auto Scaling scaling policy that was previously
* created. If you are no longer using a scaling policy, you can delete it
* with this operation.
*
*
* Deleting a policy deletes the underlying alarm action, but does not
* delete the CloudWatch alarm associated with the scaling policy, even if
* it no longer has an associated action.
*
*
* To create a new scaling policy or update an existing one, see
* PutScalingPolicy.
*
*
* @param deleteScalingPolicyRequest
* @return Result of the DeleteScalingPolicy operation returned by the
* service.
* @throws ValidationException
* An exception was thrown for a validation issue. Review the
* available parameters for the API request.
* @throws ObjectNotFoundException
* The specified object could not be found. For any Put
* or Register
API operation, which depends on the
* existence of a scalable target, this exception is thrown if the
* scalable target with the specified service namespace, resource
* ID, and scalable dimension does not exist. For any
* Delete
or Deregister
API operation,
* this exception is thrown if the resource that is to be deleted or
* deregistered cannot be found.
* @throws ConcurrentUpdateException
* Concurrent updates caused an exception, for example, if you
* request an update to an Application Auto Scaling resource that
* already has a pending update.
* @throws InternalServiceException
* The service encountered an internal error.
* @sample AWSApplicationAutoScaling.DeleteScalingPolicy
*/
DeleteScalingPolicyResult deleteScalingPolicy(
DeleteScalingPolicyRequest deleteScalingPolicyRequest);
/**
*
* Deregisters a scalable target that was previously registered. If you are
* no longer using a scalable target, you can delete it with this operation.
* When you deregister a scalable target, all of the scaling policies that
* are associated with that scalable target are deleted.
*
*
* To create a new scalable target or update an existing one, see
* RegisterScalableTarget.
*
*
* @param deregisterScalableTargetRequest
* @return Result of the DeregisterScalableTarget operation returned by the
* service.
* @throws ValidationException
* An exception was thrown for a validation issue. Review the
* available parameters for the API request.
* @throws ObjectNotFoundException
* The specified object could not be found. For any Put
* or Register
API operation, which depends on the
* existence of a scalable target, this exception is thrown if the
* scalable target with the specified service namespace, resource
* ID, and scalable dimension does not exist. For any
* Delete
or Deregister
API operation,
* this exception is thrown if the resource that is to be deleted or
* deregistered cannot be found.
* @throws ConcurrentUpdateException
* Concurrent updates caused an exception, for example, if you
* request an update to an Application Auto Scaling resource that
* already has a pending update.
* @throws InternalServiceException
* The service encountered an internal error.
* @sample AWSApplicationAutoScaling.DeregisterScalableTarget
*/
DeregisterScalableTargetResult deregisterScalableTarget(
DeregisterScalableTargetRequest deregisterScalableTargetRequest);
/**
*
* Provides descriptive information for scalable targets with a specified
* service namespace.
*
*
* You can filter the results in a service namespace with the
* ResourceIds
and ScalableDimension
parameters.
*
*
* To create a new scalable target or update an existing one, see
* RegisterScalableTarget. If you are no longer using a scalable
* target, you can deregister it with DeregisterScalableTarget.
*
*
* @param describeScalableTargetsRequest
* @return Result of the DescribeScalableTargets operation returned by the
* service.
* @throws ValidationException
* An exception was thrown for a validation issue. Review the
* available parameters for the API request.
* @throws InvalidNextTokenException
* The next token supplied was invalid.
* @throws ConcurrentUpdateException
* Concurrent updates caused an exception, for example, if you
* request an update to an Application Auto Scaling resource that
* already has a pending update.
* @throws InternalServiceException
* The service encountered an internal error.
* @sample AWSApplicationAutoScaling.DescribeScalableTargets
*/
DescribeScalableTargetsResult describeScalableTargets(
DescribeScalableTargetsRequest describeScalableTargetsRequest);
/**
*
* Provides descriptive information for scaling activities with a specified
* service namespace for the previous six weeks.
*
*
* You can filter the results in a service namespace with the
* ResourceId
and ScalableDimension
parameters.
*
*
* Scaling activities are triggered by CloudWatch alarms that are associated
* with scaling policies. To view the existing scaling policies for a
* service namespace, see DescribeScalingPolicies. To create a new
* scaling policy or update an existing one, see PutScalingPolicy.
*
*
* @param describeScalingActivitiesRequest
* @return Result of the DescribeScalingActivities operation returned by the
* service.
* @throws ValidationException
* An exception was thrown for a validation issue. Review the
* available parameters for the API request.
* @throws InvalidNextTokenException
* The next token supplied was invalid.
* @throws ConcurrentUpdateException
* Concurrent updates caused an exception, for example, if you
* request an update to an Application Auto Scaling resource that
* already has a pending update.
* @throws InternalServiceException
* The service encountered an internal error.
* @sample AWSApplicationAutoScaling.DescribeScalingActivities
*/
DescribeScalingActivitiesResult describeScalingActivities(
DescribeScalingActivitiesRequest describeScalingActivitiesRequest);
/**
*
* Provides descriptive information for scaling policies with a specified
* service namespace.
*
*
* You can filter the results in a service namespace with the
* ResourceId
, ScalableDimension
, and
* PolicyNames
parameters.
*
*
* To create a new scaling policy or update an existing one, see
* PutScalingPolicy. If you are no longer using a scaling policy, you
* can delete it with DeleteScalingPolicy.
*
*
* @param describeScalingPoliciesRequest
* @return Result of the DescribeScalingPolicies operation returned by the
* service.
* @throws ValidationException
* An exception was thrown for a validation issue. Review the
* available parameters for the API request.
* @throws FailedResourceAccessException
* Failed access to resources caused an exception. This exception
* currently only applies to DescribeScalingPolicies. It is
* thrown when Application Auto Scaling is unable to retrieve the
* alarms associated with a scaling policy due to a client error,
* for example, if the role ARN specified for a scalable target does
* not have the proper permissions to call the CloudWatch DescribeAlarms API operation on behalf of your account.
* @throws InvalidNextTokenException
* The next token supplied was invalid.
* @throws ConcurrentUpdateException
* Concurrent updates caused an exception, for example, if you
* request an update to an Application Auto Scaling resource that
* already has a pending update.
* @throws InternalServiceException
* The service encountered an internal error.
* @sample AWSApplicationAutoScaling.DescribeScalingPolicies
*/
DescribeScalingPoliciesResult describeScalingPolicies(
DescribeScalingPoliciesRequest describeScalingPoliciesRequest);
/**
*
* Creates or updates a policy for an existing Application Auto Scaling
* scalable target. Each scalable target is identified by service namespace,
* a resource ID, and a scalable dimension, and a scaling policy applies to
* a scalable target that is identified by those three attributes. You
* cannot create a scaling policy without first registering a scalable
* target with RegisterScalableTarget.
*
*
* To update an existing policy, use the existing policy name and set the
* parameters you want to change. Any existing parameter not changed in an
* update to an existing policy is not changed in this update request.
*
*
* You can view the existing scaling policies for a service namespace with
* DescribeScalingPolicies. If you are no longer using a scaling
* policy, you can delete it with DeleteScalingPolicy.
*
*
* @param putScalingPolicyRequest
* @return Result of the PutScalingPolicy operation returned by the service.
* @throws ValidationException
* An exception was thrown for a validation issue. Review the
* available parameters for the API request.
* @throws LimitExceededException
* Your account exceeded a limit. This exception is thrown when a
* per-account resource limit is exceeded. For more information, see
* Application Auto Scaling Limits.
* @throws ObjectNotFoundException
* The specified object could not be found. For any Put
* or Register
API operation, which depends on the
* existence of a scalable target, this exception is thrown if the
* scalable target with the specified service namespace, resource
* ID, and scalable dimension does not exist. For any
* Delete
or Deregister
API operation,
* this exception is thrown if the resource that is to be deleted or
* deregistered cannot be found.
* @throws ConcurrentUpdateException
* Concurrent updates caused an exception, for example, if you
* request an update to an Application Auto Scaling resource that
* already has a pending update.
* @throws InternalServiceException
* The service encountered an internal error.
* @sample AWSApplicationAutoScaling.PutScalingPolicy
*/
PutScalingPolicyResult putScalingPolicy(
PutScalingPolicyRequest putScalingPolicyRequest);
/**
*
* Registers or updates a scalable target. A scalable target is a resource
* that can be scaled out or in with Application Auto Scaling. After you
* have registered a scalable target, you can use this operation to update
* the minimum and maximum values for your scalable dimension.
*
*
* After you register a scalable target with Application Auto Scaling, you
* can create and apply scaling policies to it with PutScalingPolicy.
* You can view the existing scaling policies for a service namespace with
* DescribeScalableTargets. If you are no longer using a scalable
* target, you can deregister it with DeregisterScalableTarget.
*
*
* @param registerScalableTargetRequest
* @return Result of the RegisterScalableTarget operation returned by the
* service.
* @throws ValidationException
* An exception was thrown for a validation issue. Review the
* available parameters for the API request.
* @throws LimitExceededException
* Your account exceeded a limit. This exception is thrown when a
* per-account resource limit is exceeded. For more information, see
* Application Auto Scaling Limits.
* @throws ConcurrentUpdateException
* Concurrent updates caused an exception, for example, if you
* request an update to an Application Auto Scaling resource that
* already has a pending update.
* @throws InternalServiceException
* The service encountered an internal error.
* @sample AWSApplicationAutoScaling.RegisterScalableTarget
*/
RegisterScalableTargetResult registerScalableTarget(
RegisterScalableTargetRequest registerScalableTargetRequest);
/**
* Shuts down this client object, releasing any resources that might be held
* open. This is an optional method, and callers are not expected to call
* it, but can if they want to explicitly release any open resources. Once a
* client has been shutdown, it should not be used to make any more
* requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request,
* typically used for debugging issues where a service isn't acting as
* expected. This data isn't considered part of the result data returned by
* an operation, so it's available through this separate, diagnostic
* interface.
*
* Response metadata is only cached for a limited period of time, so if you
* need to access this extra diagnostic information for an executed request,
* you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none
* is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}