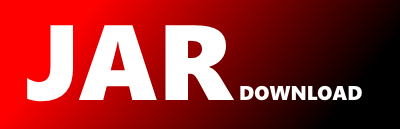
com.amazonaws.services.applicationautoscaling.AWSApplicationAutoScalingAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-applicationautoscaling Show documentation
/*
* Copyright 2011-2016 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not
* use this file except in compliance with the License. A copy of the License is
* located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.applicationautoscaling;
import com.amazonaws.services.applicationautoscaling.model.*;
/**
* Interface for accessing Application Auto Scaling asynchronously. Each
* asynchronous method will return a Java Future object representing the
* asynchronous operation; overloads which accept an {@code AsyncHandler} can be
* used to receive notification when an asynchronous operation completes.
*
*
* Application Auto Scaling is a general purpose Auto Scaling service for
* supported elastic AWS resources. With Application Auto Scaling, you can
* automatically scale your AWS resources, with an experience similar to that of
* Auto Scaling.
*
*
* Application Auto Scaling supports scaling the following AWS resources:
*
*
* -
*
* Amazon ECS services
*
*
* -
*
* Amazon EC2 Spot fleet instances
*
*
*
*
* You can use Application Auto Scaling to accomplish the following tasks:
*
*
* -
*
* Define scaling policies for automatically adjusting your AWS resources
*
*
* -
*
* Scale your resources in response to CloudWatch alarms
*
*
* -
*
* View history of your scaling events
*
*
*
*
* Application Auto Scaling is available in the following regions:
*
*
* -
*
* us-east-1
*
*
* -
*
* us-west-1
*
*
* -
*
* us-west-2
*
*
* -
*
* ap-southeast-1
*
*
* -
*
* ap-southeast-2
*
*
* -
*
* ap-northeast-1
*
*
* -
*
* eu-central-1
*
*
* -
*
* eu-west-1
*
*
*
*/
public interface AWSApplicationAutoScalingAsync extends
AWSApplicationAutoScaling {
/**
*
* Deletes an Application Auto Scaling scaling policy that was previously
* created. If you are no longer using a scaling policy, you can delete it
* with this operation.
*
*
* Deleting a policy deletes the underlying alarm action, but does not
* delete the CloudWatch alarm associated with the scaling policy, even if
* it no longer has an associated action.
*
*
* To create a new scaling policy or update an existing one, see
* PutScalingPolicy.
*
*
* @param deleteScalingPolicyRequest
* @return A Java Future containing the result of the DeleteScalingPolicy
* operation returned by the service.
* @sample AWSApplicationAutoScalingAsync.DeleteScalingPolicy
*/
java.util.concurrent.Future deleteScalingPolicyAsync(
DeleteScalingPolicyRequest deleteScalingPolicyRequest);
/**
*
* Deletes an Application Auto Scaling scaling policy that was previously
* created. If you are no longer using a scaling policy, you can delete it
* with this operation.
*
*
* Deleting a policy deletes the underlying alarm action, but does not
* delete the CloudWatch alarm associated with the scaling policy, even if
* it no longer has an associated action.
*
*
* To create a new scaling policy or update an existing one, see
* PutScalingPolicy.
*
*
* @param deleteScalingPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteScalingPolicy
* operation returned by the service.
* @sample AWSApplicationAutoScalingAsyncHandler.DeleteScalingPolicy
*/
java.util.concurrent.Future deleteScalingPolicyAsync(
DeleteScalingPolicyRequest deleteScalingPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deregisters a scalable target that was previously registered. If you are
* no longer using a scalable target, you can delete it with this operation.
* When you deregister a scalable target, all of the scaling policies that
* are associated with that scalable target are deleted.
*
*
* To create a new scalable target or update an existing one, see
* RegisterScalableTarget.
*
*
* @param deregisterScalableTargetRequest
* @return A Java Future containing the result of the
* DeregisterScalableTarget operation returned by the service.
* @sample AWSApplicationAutoScalingAsync.DeregisterScalableTarget
*/
java.util.concurrent.Future deregisterScalableTargetAsync(
DeregisterScalableTargetRequest deregisterScalableTargetRequest);
/**
*
* Deregisters a scalable target that was previously registered. If you are
* no longer using a scalable target, you can delete it with this operation.
* When you deregister a scalable target, all of the scaling policies that
* are associated with that scalable target are deleted.
*
*
* To create a new scalable target or update an existing one, see
* RegisterScalableTarget.
*
*
* @param deregisterScalableTargetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DeregisterScalableTarget operation returned by the service.
* @sample AWSApplicationAutoScalingAsyncHandler.DeregisterScalableTarget
*/
java.util.concurrent.Future deregisterScalableTargetAsync(
DeregisterScalableTargetRequest deregisterScalableTargetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides descriptive information for scalable targets with a specified
* service namespace.
*
*
* You can filter the results in a service namespace with the
* ResourceIds
and ScalableDimension
parameters.
*
*
* To create a new scalable target or update an existing one, see
* RegisterScalableTarget. If you are no longer using a scalable
* target, you can deregister it with DeregisterScalableTarget.
*
*
* @param describeScalableTargetsRequest
* @return A Java Future containing the result of the
* DescribeScalableTargets operation returned by the service.
* @sample AWSApplicationAutoScalingAsync.DescribeScalableTargets
*/
java.util.concurrent.Future describeScalableTargetsAsync(
DescribeScalableTargetsRequest describeScalableTargetsRequest);
/**
*
* Provides descriptive information for scalable targets with a specified
* service namespace.
*
*
* You can filter the results in a service namespace with the
* ResourceIds
and ScalableDimension
parameters.
*
*
* To create a new scalable target or update an existing one, see
* RegisterScalableTarget. If you are no longer using a scalable
* target, you can deregister it with DeregisterScalableTarget.
*
*
* @param describeScalableTargetsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DescribeScalableTargets operation returned by the service.
* @sample AWSApplicationAutoScalingAsyncHandler.DescribeScalableTargets
*/
java.util.concurrent.Future describeScalableTargetsAsync(
DescribeScalableTargetsRequest describeScalableTargetsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides descriptive information for scaling activities with a specified
* service namespace for the previous six weeks.
*
*
* You can filter the results in a service namespace with the
* ResourceId
and ScalableDimension
parameters.
*
*
* Scaling activities are triggered by CloudWatch alarms that are associated
* with scaling policies. To view the existing scaling policies for a
* service namespace, see DescribeScalingPolicies. To create a new
* scaling policy or update an existing one, see PutScalingPolicy.
*
*
* @param describeScalingActivitiesRequest
* @return A Java Future containing the result of the
* DescribeScalingActivities operation returned by the service.
* @sample AWSApplicationAutoScalingAsync.DescribeScalingActivities
*/
java.util.concurrent.Future describeScalingActivitiesAsync(
DescribeScalingActivitiesRequest describeScalingActivitiesRequest);
/**
*
* Provides descriptive information for scaling activities with a specified
* service namespace for the previous six weeks.
*
*
* You can filter the results in a service namespace with the
* ResourceId
and ScalableDimension
parameters.
*
*
* Scaling activities are triggered by CloudWatch alarms that are associated
* with scaling policies. To view the existing scaling policies for a
* service namespace, see DescribeScalingPolicies. To create a new
* scaling policy or update an existing one, see PutScalingPolicy.
*
*
* @param describeScalingActivitiesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DescribeScalingActivities operation returned by the service.
* @sample AWSApplicationAutoScalingAsyncHandler.DescribeScalingActivities
*/
java.util.concurrent.Future describeScalingActivitiesAsync(
DescribeScalingActivitiesRequest describeScalingActivitiesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides descriptive information for scaling policies with a specified
* service namespace.
*
*
* You can filter the results in a service namespace with the
* ResourceId
, ScalableDimension
, and
* PolicyNames
parameters.
*
*
* To create a new scaling policy or update an existing one, see
* PutScalingPolicy. If you are no longer using a scaling policy, you
* can delete it with DeleteScalingPolicy.
*
*
* @param describeScalingPoliciesRequest
* @return A Java Future containing the result of the
* DescribeScalingPolicies operation returned by the service.
* @sample AWSApplicationAutoScalingAsync.DescribeScalingPolicies
*/
java.util.concurrent.Future describeScalingPoliciesAsync(
DescribeScalingPoliciesRequest describeScalingPoliciesRequest);
/**
*
* Provides descriptive information for scaling policies with a specified
* service namespace.
*
*
* You can filter the results in a service namespace with the
* ResourceId
, ScalableDimension
, and
* PolicyNames
parameters.
*
*
* To create a new scaling policy or update an existing one, see
* PutScalingPolicy. If you are no longer using a scaling policy, you
* can delete it with DeleteScalingPolicy.
*
*
* @param describeScalingPoliciesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DescribeScalingPolicies operation returned by the service.
* @sample AWSApplicationAutoScalingAsyncHandler.DescribeScalingPolicies
*/
java.util.concurrent.Future describeScalingPoliciesAsync(
DescribeScalingPoliciesRequest describeScalingPoliciesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates or updates a policy for an existing Application Auto Scaling
* scalable target. Each scalable target is identified by service namespace,
* a resource ID, and a scalable dimension, and a scaling policy applies to
* a scalable target that is identified by those three attributes. You
* cannot create a scaling policy without first registering a scalable
* target with RegisterScalableTarget.
*
*
* To update an existing policy, use the existing policy name and set the
* parameters you want to change. Any existing parameter not changed in an
* update to an existing policy is not changed in this update request.
*
*
* You can view the existing scaling policies for a service namespace with
* DescribeScalingPolicies. If you are no longer using a scaling
* policy, you can delete it with DeleteScalingPolicy.
*
*
* @param putScalingPolicyRequest
* @return A Java Future containing the result of the PutScalingPolicy
* operation returned by the service.
* @sample AWSApplicationAutoScalingAsync.PutScalingPolicy
*/
java.util.concurrent.Future putScalingPolicyAsync(
PutScalingPolicyRequest putScalingPolicyRequest);
/**
*
* Creates or updates a policy for an existing Application Auto Scaling
* scalable target. Each scalable target is identified by service namespace,
* a resource ID, and a scalable dimension, and a scaling policy applies to
* a scalable target that is identified by those three attributes. You
* cannot create a scaling policy without first registering a scalable
* target with RegisterScalableTarget.
*
*
* To update an existing policy, use the existing policy name and set the
* parameters you want to change. Any existing parameter not changed in an
* update to an existing policy is not changed in this update request.
*
*
* You can view the existing scaling policies for a service namespace with
* DescribeScalingPolicies. If you are no longer using a scaling
* policy, you can delete it with DeleteScalingPolicy.
*
*
* @param putScalingPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutScalingPolicy
* operation returned by the service.
* @sample AWSApplicationAutoScalingAsyncHandler.PutScalingPolicy
*/
java.util.concurrent.Future putScalingPolicyAsync(
PutScalingPolicyRequest putScalingPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Registers or updates a scalable target. A scalable target is a resource
* that can be scaled out or in with Application Auto Scaling. After you
* have registered a scalable target, you can use this operation to update
* the minimum and maximum values for your scalable dimension.
*
*
* After you register a scalable target with Application Auto Scaling, you
* can create and apply scaling policies to it with PutScalingPolicy.
* You can view the existing scaling policies for a service namespace with
* DescribeScalableTargets. If you are no longer using a scalable
* target, you can deregister it with DeregisterScalableTarget.
*
*
* @param registerScalableTargetRequest
* @return A Java Future containing the result of the RegisterScalableTarget
* operation returned by the service.
* @sample AWSApplicationAutoScalingAsync.RegisterScalableTarget
*/
java.util.concurrent.Future registerScalableTargetAsync(
RegisterScalableTargetRequest registerScalableTargetRequest);
/**
*
* Registers or updates a scalable target. A scalable target is a resource
* that can be scaled out or in with Application Auto Scaling. After you
* have registered a scalable target, you can use this operation to update
* the minimum and maximum values for your scalable dimension.
*
*
* After you register a scalable target with Application Auto Scaling, you
* can create and apply scaling policies to it with PutScalingPolicy.
* You can view the existing scaling policies for a service namespace with
* DescribeScalableTargets. If you are no longer using a scalable
* target, you can deregister it with DeregisterScalableTarget.
*
*
* @param registerScalableTargetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RegisterScalableTarget
* operation returned by the service.
* @sample AWSApplicationAutoScalingAsyncHandler.RegisterScalableTarget
*/
java.util.concurrent.Future registerScalableTargetAsync(
RegisterScalableTargetRequest registerScalableTargetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}