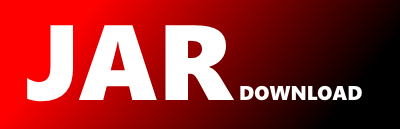
com.amazonaws.services.applicationautoscaling.model.ScalableTarget Maven / Gradle / Ivy
Show all versions of aws-java-sdk-applicationautoscaling Show documentation
/*
* Copyright 2011-2016 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not
* use this file except in compliance with the License. A copy of the License is
* located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.applicationautoscaling.model;
import java.io.Serializable;
/**
*
* An object representing a scalable target.
*
*/
public class ScalableTarget implements Serializable, Cloneable {
/**
*
* The namespace for the AWS service that the scalable target is associated
* with. For more information, see AWS Service Namespaces in the Amazon Web Services General Reference.
*
*/
private String serviceNamespace;
/**
*
* The resource type and unique identifier string for the resource
* associated with the scalable target. For Amazon ECS services, the
* resource type is services
, and the identifier is the cluster
* name and service name; for example,
* service/default/sample-webapp
. For Amazon EC2 Spot fleet
* requests, the resource type is spot-fleet-request
, and the
* identifier is the Spot fleet request ID; for example,
* spot-fleet-request/sfr-73fbd2ce-aa30-494c-8788-1cee4EXAMPLE
.
*
*/
private String resourceId;
/**
*
* The scalable dimension associated with the scalable target. The scalable
* dimension contains the service namespace, resource type, and scaling
* property, such as ecs:service:DesiredCount
for the desired
* task count of an Amazon ECS service, or
* ec2:spot-fleet-request:TargetCapacity
for the target
* capacity of an Amazon EC2 Spot fleet request.
*
*/
private String scalableDimension;
/**
*
* The minimum value for this scalable target to scale in to in response to
* scaling activities.
*
*/
private Integer minCapacity;
/**
*
* The maximum value for this scalable target to scale out to in response to
* scaling activities.
*
*/
private Integer maxCapacity;
/**
*
* The ARN of the IAM role that allows Application Auto Scaling to modify
* your scalable target on your behalf.
*
*/
private String roleARN;
/**
*
* The Unix timestamp for when the scalable target was created.
*
*/
private java.util.Date creationTime;
/**
*
* The namespace for the AWS service that the scalable target is associated
* with. For more information, see AWS Service Namespaces in the Amazon Web Services General Reference.
*
*
* @param serviceNamespace
* The namespace for the AWS service that the scalable target is
* associated with. For more information, see AWS Service Namespaces in the Amazon Web Services General
* Reference.
* @see ServiceNamespace
*/
public void setServiceNamespace(String serviceNamespace) {
this.serviceNamespace = serviceNamespace;
}
/**
*
* The namespace for the AWS service that the scalable target is associated
* with. For more information, see AWS Service Namespaces in the Amazon Web Services General Reference.
*
*
* @return The namespace for the AWS service that the scalable target is
* associated with. For more information, see AWS Service Namespaces in the Amazon Web Services General
* Reference.
* @see ServiceNamespace
*/
public String getServiceNamespace() {
return this.serviceNamespace;
}
/**
*
* The namespace for the AWS service that the scalable target is associated
* with. For more information, see AWS Service Namespaces in the Amazon Web Services General Reference.
*
*
* @param serviceNamespace
* The namespace for the AWS service that the scalable target is
* associated with. For more information, see AWS Service Namespaces in the Amazon Web Services General
* Reference.
* @return Returns a reference to this object so that method calls can be
* chained together.
* @see ServiceNamespace
*/
public ScalableTarget withServiceNamespace(String serviceNamespace) {
setServiceNamespace(serviceNamespace);
return this;
}
/**
*
* The namespace for the AWS service that the scalable target is associated
* with. For more information, see AWS Service Namespaces in the Amazon Web Services General Reference.
*
*
* @param serviceNamespace
* The namespace for the AWS service that the scalable target is
* associated with. For more information, see AWS Service Namespaces in the Amazon Web Services General
* Reference.
* @see ServiceNamespace
*/
public void setServiceNamespace(ServiceNamespace serviceNamespace) {
this.serviceNamespace = serviceNamespace.toString();
}
/**
*
* The namespace for the AWS service that the scalable target is associated
* with. For more information, see AWS Service Namespaces in the Amazon Web Services General Reference.
*
*
* @param serviceNamespace
* The namespace for the AWS service that the scalable target is
* associated with. For more information, see AWS Service Namespaces in the Amazon Web Services General
* Reference.
* @return Returns a reference to this object so that method calls can be
* chained together.
* @see ServiceNamespace
*/
public ScalableTarget withServiceNamespace(ServiceNamespace serviceNamespace) {
setServiceNamespace(serviceNamespace);
return this;
}
/**
*
* The resource type and unique identifier string for the resource
* associated with the scalable target. For Amazon ECS services, the
* resource type is services
, and the identifier is the cluster
* name and service name; for example,
* service/default/sample-webapp
. For Amazon EC2 Spot fleet
* requests, the resource type is spot-fleet-request
, and the
* identifier is the Spot fleet request ID; for example,
* spot-fleet-request/sfr-73fbd2ce-aa30-494c-8788-1cee4EXAMPLE
.
*
*
* @param resourceId
* The resource type and unique identifier string for the resource
* associated with the scalable target. For Amazon ECS services, the
* resource type is services
, and the identifier is the
* cluster name and service name; for example,
* service/default/sample-webapp
. For Amazon EC2 Spot
* fleet requests, the resource type is
* spot-fleet-request
, and the identifier is the Spot
* fleet request ID; for example,
* spot-fleet-request/sfr-73fbd2ce-aa30-494c-8788-1cee4EXAMPLE
* .
*/
public void setResourceId(String resourceId) {
this.resourceId = resourceId;
}
/**
*
* The resource type and unique identifier string for the resource
* associated with the scalable target. For Amazon ECS services, the
* resource type is services
, and the identifier is the cluster
* name and service name; for example,
* service/default/sample-webapp
. For Amazon EC2 Spot fleet
* requests, the resource type is spot-fleet-request
, and the
* identifier is the Spot fleet request ID; for example,
* spot-fleet-request/sfr-73fbd2ce-aa30-494c-8788-1cee4EXAMPLE
.
*
*
* @return The resource type and unique identifier string for the resource
* associated with the scalable target. For Amazon ECS services, the
* resource type is services
, and the identifier is the
* cluster name and service name; for example,
* service/default/sample-webapp
. For Amazon EC2 Spot
* fleet requests, the resource type is
* spot-fleet-request
, and the identifier is the Spot
* fleet request ID; for example,
* spot-fleet-request/sfr-73fbd2ce-aa30-494c-8788-1cee4EXAMPLE
* .
*/
public String getResourceId() {
return this.resourceId;
}
/**
*
* The resource type and unique identifier string for the resource
* associated with the scalable target. For Amazon ECS services, the
* resource type is services
, and the identifier is the cluster
* name and service name; for example,
* service/default/sample-webapp
. For Amazon EC2 Spot fleet
* requests, the resource type is spot-fleet-request
, and the
* identifier is the Spot fleet request ID; for example,
* spot-fleet-request/sfr-73fbd2ce-aa30-494c-8788-1cee4EXAMPLE
.
*
*
* @param resourceId
* The resource type and unique identifier string for the resource
* associated with the scalable target. For Amazon ECS services, the
* resource type is services
, and the identifier is the
* cluster name and service name; for example,
* service/default/sample-webapp
. For Amazon EC2 Spot
* fleet requests, the resource type is
* spot-fleet-request
, and the identifier is the Spot
* fleet request ID; for example,
* spot-fleet-request/sfr-73fbd2ce-aa30-494c-8788-1cee4EXAMPLE
* .
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ScalableTarget withResourceId(String resourceId) {
setResourceId(resourceId);
return this;
}
/**
*
* The scalable dimension associated with the scalable target. The scalable
* dimension contains the service namespace, resource type, and scaling
* property, such as ecs:service:DesiredCount
for the desired
* task count of an Amazon ECS service, or
* ec2:spot-fleet-request:TargetCapacity
for the target
* capacity of an Amazon EC2 Spot fleet request.
*
*
* @param scalableDimension
* The scalable dimension associated with the scalable target. The
* scalable dimension contains the service namespace, resource type,
* and scaling property, such as
* ecs:service:DesiredCount
for the desired task count
* of an Amazon ECS service, or
* ec2:spot-fleet-request:TargetCapacity
for the target
* capacity of an Amazon EC2 Spot fleet request.
* @see ScalableDimension
*/
public void setScalableDimension(String scalableDimension) {
this.scalableDimension = scalableDimension;
}
/**
*
* The scalable dimension associated with the scalable target. The scalable
* dimension contains the service namespace, resource type, and scaling
* property, such as ecs:service:DesiredCount
for the desired
* task count of an Amazon ECS service, or
* ec2:spot-fleet-request:TargetCapacity
for the target
* capacity of an Amazon EC2 Spot fleet request.
*
*
* @return The scalable dimension associated with the scalable target. The
* scalable dimension contains the service namespace, resource type,
* and scaling property, such as
* ecs:service:DesiredCount
for the desired task count
* of an Amazon ECS service, or
* ec2:spot-fleet-request:TargetCapacity
for the target
* capacity of an Amazon EC2 Spot fleet request.
* @see ScalableDimension
*/
public String getScalableDimension() {
return this.scalableDimension;
}
/**
*
* The scalable dimension associated with the scalable target. The scalable
* dimension contains the service namespace, resource type, and scaling
* property, such as ecs:service:DesiredCount
for the desired
* task count of an Amazon ECS service, or
* ec2:spot-fleet-request:TargetCapacity
for the target
* capacity of an Amazon EC2 Spot fleet request.
*
*
* @param scalableDimension
* The scalable dimension associated with the scalable target. The
* scalable dimension contains the service namespace, resource type,
* and scaling property, such as
* ecs:service:DesiredCount
for the desired task count
* of an Amazon ECS service, or
* ec2:spot-fleet-request:TargetCapacity
for the target
* capacity of an Amazon EC2 Spot fleet request.
* @return Returns a reference to this object so that method calls can be
* chained together.
* @see ScalableDimension
*/
public ScalableTarget withScalableDimension(String scalableDimension) {
setScalableDimension(scalableDimension);
return this;
}
/**
*
* The scalable dimension associated with the scalable target. The scalable
* dimension contains the service namespace, resource type, and scaling
* property, such as ecs:service:DesiredCount
for the desired
* task count of an Amazon ECS service, or
* ec2:spot-fleet-request:TargetCapacity
for the target
* capacity of an Amazon EC2 Spot fleet request.
*
*
* @param scalableDimension
* The scalable dimension associated with the scalable target. The
* scalable dimension contains the service namespace, resource type,
* and scaling property, such as
* ecs:service:DesiredCount
for the desired task count
* of an Amazon ECS service, or
* ec2:spot-fleet-request:TargetCapacity
for the target
* capacity of an Amazon EC2 Spot fleet request.
* @see ScalableDimension
*/
public void setScalableDimension(ScalableDimension scalableDimension) {
this.scalableDimension = scalableDimension.toString();
}
/**
*
* The scalable dimension associated with the scalable target. The scalable
* dimension contains the service namespace, resource type, and scaling
* property, such as ecs:service:DesiredCount
for the desired
* task count of an Amazon ECS service, or
* ec2:spot-fleet-request:TargetCapacity
for the target
* capacity of an Amazon EC2 Spot fleet request.
*
*
* @param scalableDimension
* The scalable dimension associated with the scalable target. The
* scalable dimension contains the service namespace, resource type,
* and scaling property, such as
* ecs:service:DesiredCount
for the desired task count
* of an Amazon ECS service, or
* ec2:spot-fleet-request:TargetCapacity
for the target
* capacity of an Amazon EC2 Spot fleet request.
* @return Returns a reference to this object so that method calls can be
* chained together.
* @see ScalableDimension
*/
public ScalableTarget withScalableDimension(
ScalableDimension scalableDimension) {
setScalableDimension(scalableDimension);
return this;
}
/**
*
* The minimum value for this scalable target to scale in to in response to
* scaling activities.
*
*
* @param minCapacity
* The minimum value for this scalable target to scale in to in
* response to scaling activities.
*/
public void setMinCapacity(Integer minCapacity) {
this.minCapacity = minCapacity;
}
/**
*
* The minimum value for this scalable target to scale in to in response to
* scaling activities.
*
*
* @return The minimum value for this scalable target to scale in to in
* response to scaling activities.
*/
public Integer getMinCapacity() {
return this.minCapacity;
}
/**
*
* The minimum value for this scalable target to scale in to in response to
* scaling activities.
*
*
* @param minCapacity
* The minimum value for this scalable target to scale in to in
* response to scaling activities.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ScalableTarget withMinCapacity(Integer minCapacity) {
setMinCapacity(minCapacity);
return this;
}
/**
*
* The maximum value for this scalable target to scale out to in response to
* scaling activities.
*
*
* @param maxCapacity
* The maximum value for this scalable target to scale out to in
* response to scaling activities.
*/
public void setMaxCapacity(Integer maxCapacity) {
this.maxCapacity = maxCapacity;
}
/**
*
* The maximum value for this scalable target to scale out to in response to
* scaling activities.
*
*
* @return The maximum value for this scalable target to scale out to in
* response to scaling activities.
*/
public Integer getMaxCapacity() {
return this.maxCapacity;
}
/**
*
* The maximum value for this scalable target to scale out to in response to
* scaling activities.
*
*
* @param maxCapacity
* The maximum value for this scalable target to scale out to in
* response to scaling activities.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ScalableTarget withMaxCapacity(Integer maxCapacity) {
setMaxCapacity(maxCapacity);
return this;
}
/**
*
* The ARN of the IAM role that allows Application Auto Scaling to modify
* your scalable target on your behalf.
*
*
* @param roleARN
* The ARN of the IAM role that allows Application Auto Scaling to
* modify your scalable target on your behalf.
*/
public void setRoleARN(String roleARN) {
this.roleARN = roleARN;
}
/**
*
* The ARN of the IAM role that allows Application Auto Scaling to modify
* your scalable target on your behalf.
*
*
* @return The ARN of the IAM role that allows Application Auto Scaling to
* modify your scalable target on your behalf.
*/
public String getRoleARN() {
return this.roleARN;
}
/**
*
* The ARN of the IAM role that allows Application Auto Scaling to modify
* your scalable target on your behalf.
*
*
* @param roleARN
* The ARN of the IAM role that allows Application Auto Scaling to
* modify your scalable target on your behalf.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ScalableTarget withRoleARN(String roleARN) {
setRoleARN(roleARN);
return this;
}
/**
*
* The Unix timestamp for when the scalable target was created.
*
*
* @param creationTime
* The Unix timestamp for when the scalable target was created.
*/
public void setCreationTime(java.util.Date creationTime) {
this.creationTime = creationTime;
}
/**
*
* The Unix timestamp for when the scalable target was created.
*
*
* @return The Unix timestamp for when the scalable target was created.
*/
public java.util.Date getCreationTime() {
return this.creationTime;
}
/**
*
* The Unix timestamp for when the scalable target was created.
*
*
* @param creationTime
* The Unix timestamp for when the scalable target was created.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ScalableTarget withCreationTime(java.util.Date creationTime) {
setCreationTime(creationTime);
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getServiceNamespace() != null)
sb.append("ServiceNamespace: " + getServiceNamespace() + ",");
if (getResourceId() != null)
sb.append("ResourceId: " + getResourceId() + ",");
if (getScalableDimension() != null)
sb.append("ScalableDimension: " + getScalableDimension() + ",");
if (getMinCapacity() != null)
sb.append("MinCapacity: " + getMinCapacity() + ",");
if (getMaxCapacity() != null)
sb.append("MaxCapacity: " + getMaxCapacity() + ",");
if (getRoleARN() != null)
sb.append("RoleARN: " + getRoleARN() + ",");
if (getCreationTime() != null)
sb.append("CreationTime: " + getCreationTime());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ScalableTarget == false)
return false;
ScalableTarget other = (ScalableTarget) obj;
if (other.getServiceNamespace() == null
^ this.getServiceNamespace() == null)
return false;
if (other.getServiceNamespace() != null
&& other.getServiceNamespace().equals(
this.getServiceNamespace()) == false)
return false;
if (other.getResourceId() == null ^ this.getResourceId() == null)
return false;
if (other.getResourceId() != null
&& other.getResourceId().equals(this.getResourceId()) == false)
return false;
if (other.getScalableDimension() == null
^ this.getScalableDimension() == null)
return false;
if (other.getScalableDimension() != null
&& other.getScalableDimension().equals(
this.getScalableDimension()) == false)
return false;
if (other.getMinCapacity() == null ^ this.getMinCapacity() == null)
return false;
if (other.getMinCapacity() != null
&& other.getMinCapacity().equals(this.getMinCapacity()) == false)
return false;
if (other.getMaxCapacity() == null ^ this.getMaxCapacity() == null)
return false;
if (other.getMaxCapacity() != null
&& other.getMaxCapacity().equals(this.getMaxCapacity()) == false)
return false;
if (other.getRoleARN() == null ^ this.getRoleARN() == null)
return false;
if (other.getRoleARN() != null
&& other.getRoleARN().equals(this.getRoleARN()) == false)
return false;
if (other.getCreationTime() == null ^ this.getCreationTime() == null)
return false;
if (other.getCreationTime() != null
&& other.getCreationTime().equals(this.getCreationTime()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime
* hashCode
+ ((getServiceNamespace() == null) ? 0 : getServiceNamespace()
.hashCode());
hashCode = prime * hashCode
+ ((getResourceId() == null) ? 0 : getResourceId().hashCode());
hashCode = prime
* hashCode
+ ((getScalableDimension() == null) ? 0
: getScalableDimension().hashCode());
hashCode = prime
* hashCode
+ ((getMinCapacity() == null) ? 0 : getMinCapacity().hashCode());
hashCode = prime
* hashCode
+ ((getMaxCapacity() == null) ? 0 : getMaxCapacity().hashCode());
hashCode = prime * hashCode
+ ((getRoleARN() == null) ? 0 : getRoleARN().hashCode());
hashCode = prime
* hashCode
+ ((getCreationTime() == null) ? 0 : getCreationTime()
.hashCode());
return hashCode;
}
@Override
public ScalableTarget clone() {
try {
return (ScalableTarget) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException(
"Got a CloneNotSupportedException from Object.clone() "
+ "even though we're Cloneable!", e);
}
}
}