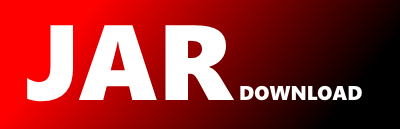
com.amazonaws.services.applicationautoscaling.model.DescribeScheduledActionsRequest Maven / Gradle / Ivy
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.applicationautoscaling.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class DescribeScheduledActionsRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The names of the scheduled actions to describe.
*
*/
private java.util.List scheduledActionNames;
/**
*
* The namespace of the AWS service that provides the resource or custom-resource
for a resource
* provided by your own application or service. For more information, see AWS Service Namespaces in the Amazon Web Services General Reference.
*
*/
private String serviceNamespace;
/**
*
* The identifier of the resource associated with the scheduled action. This string consists of the resource type
* and unique identifier. If you specify a scalable dimension, you must also specify a resource ID.
*
*
* -
*
* ECS service - The resource type is service
and the unique identifier is the cluster name and service
* name. Example: service/default/sample-webapp
.
*
*
* -
*
* Spot fleet request - The resource type is spot-fleet-request
and the unique identifier is the Spot
* fleet request ID. Example: spot-fleet-request/sfr-73fbd2ce-aa30-494c-8788-1cee4EXAMPLE
.
*
*
* -
*
* EMR cluster - The resource type is instancegroup
and the unique identifier is the cluster ID and
* instance group ID. Example: instancegroup/j-2EEZNYKUA1NTV/ig-1791Y4E1L8YI0
.
*
*
* -
*
* AppStream 2.0 fleet - The resource type is fleet
and the unique identifier is the fleet name.
* Example: fleet/sample-fleet
.
*
*
* -
*
* DynamoDB table - The resource type is table
and the unique identifier is the resource ID. Example:
* table/my-table
.
*
*
* -
*
* DynamoDB global secondary index - The resource type is index
and the unique identifier is the
* resource ID. Example: table/my-table/index/my-table-index
.
*
*
* -
*
* Aurora DB cluster - The resource type is cluster
and the unique identifier is the cluster name.
* Example: cluster:my-db-cluster
.
*
*
* -
*
* Amazon SageMaker endpoint variants - The resource type is variant
and the unique identifier is the
* resource ID. Example: endpoint/my-end-point/variant/KMeansClustering
.
*
*
* -
*
* Custom resources are not supported with a resource type. This parameter must specify the OutputValue
* from the CloudFormation template stack used to access the resources. The unique identifier is defined by the
* service provider.
*
*
*
*/
private String resourceId;
/**
*
* The scalable dimension. This string consists of the service namespace, resource type, and scaling property. If
* you specify a scalable dimension, you must also specify a resource ID.
*
*
* -
*
* ecs:service:DesiredCount
- The desired task count of an ECS service.
*
*
* -
*
* ec2:spot-fleet-request:TargetCapacity
- The target capacity of a Spot fleet request.
*
*
* -
*
* elasticmapreduce:instancegroup:InstanceCount
- The instance count of an EMR Instance Group.
*
*
* -
*
* appstream:fleet:DesiredCapacity
- The desired capacity of an AppStream 2.0 fleet.
*
*
* -
*
* dynamodb:table:ReadCapacityUnits
- The provisioned read capacity for a DynamoDB table.
*
*
* -
*
* dynamodb:table:WriteCapacityUnits
- The provisioned write capacity for a DynamoDB table.
*
*
* -
*
* dynamodb:index:ReadCapacityUnits
- The provisioned read capacity for a DynamoDB global secondary
* index.
*
*
* -
*
* dynamodb:index:WriteCapacityUnits
- The provisioned write capacity for a DynamoDB global secondary
* index.
*
*
* -
*
* rds:cluster:ReadReplicaCount
- The count of Aurora Replicas in an Aurora DB cluster. Available for
* Aurora MySQL-compatible edition.
*
*
* -
*
* sagemaker:variant:DesiredInstanceCount
- The number of EC2 instances for an Amazon SageMaker model
* endpoint variant.
*
*
* -
*
* custom-resource:ResourceType:Property
- The scalable dimension for a custom resource provided by
* your own application or service.
*
*
*
*/
private String scalableDimension;
/**
*
* The maximum number of scheduled action results. This value can be between 1 and 50. The default value is 50.
*
*
* If this parameter is used, the operation returns up to MaxResults
results at a time, along with a
* NextToken
value. To get the next set of results, include the NextToken
value in a
* subsequent call. If this parameter is not used, the operation returns up to 50 results and a
* NextToken
value, if applicable.
*
*/
private Integer maxResults;
/**
*
* The token for the next set of results.
*
*/
private String nextToken;
/**
*
* The names of the scheduled actions to describe.
*
*
* @return The names of the scheduled actions to describe.
*/
public java.util.List getScheduledActionNames() {
return scheduledActionNames;
}
/**
*
* The names of the scheduled actions to describe.
*
*
* @param scheduledActionNames
* The names of the scheduled actions to describe.
*/
public void setScheduledActionNames(java.util.Collection scheduledActionNames) {
if (scheduledActionNames == null) {
this.scheduledActionNames = null;
return;
}
this.scheduledActionNames = new java.util.ArrayList(scheduledActionNames);
}
/**
*
* The names of the scheduled actions to describe.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setScheduledActionNames(java.util.Collection)} or {@link #withScheduledActionNames(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param scheduledActionNames
* The names of the scheduled actions to describe.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeScheduledActionsRequest withScheduledActionNames(String... scheduledActionNames) {
if (this.scheduledActionNames == null) {
setScheduledActionNames(new java.util.ArrayList(scheduledActionNames.length));
}
for (String ele : scheduledActionNames) {
this.scheduledActionNames.add(ele);
}
return this;
}
/**
*
* The names of the scheduled actions to describe.
*
*
* @param scheduledActionNames
* The names of the scheduled actions to describe.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeScheduledActionsRequest withScheduledActionNames(java.util.Collection scheduledActionNames) {
setScheduledActionNames(scheduledActionNames);
return this;
}
/**
*
* The namespace of the AWS service that provides the resource or custom-resource
for a resource
* provided by your own application or service. For more information, see AWS Service Namespaces in the Amazon Web Services General Reference.
*
*
* @param serviceNamespace
* The namespace of the AWS service that provides the resource or custom-resource
for a resource
* provided by your own application or service. For more information, see AWS Service Namespaces in the Amazon Web Services General Reference.
* @see ServiceNamespace
*/
public void setServiceNamespace(String serviceNamespace) {
this.serviceNamespace = serviceNamespace;
}
/**
*
* The namespace of the AWS service that provides the resource or custom-resource
for a resource
* provided by your own application or service. For more information, see AWS Service Namespaces in the Amazon Web Services General Reference.
*
*
* @return The namespace of the AWS service that provides the resource or custom-resource
for a
* resource provided by your own application or service. For more information, see AWS Service Namespaces in the Amazon Web Services General Reference.
* @see ServiceNamespace
*/
public String getServiceNamespace() {
return this.serviceNamespace;
}
/**
*
* The namespace of the AWS service that provides the resource or custom-resource
for a resource
* provided by your own application or service. For more information, see AWS Service Namespaces in the Amazon Web Services General Reference.
*
*
* @param serviceNamespace
* The namespace of the AWS service that provides the resource or custom-resource
for a resource
* provided by your own application or service. For more information, see AWS Service Namespaces in the Amazon Web Services General Reference.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ServiceNamespace
*/
public DescribeScheduledActionsRequest withServiceNamespace(String serviceNamespace) {
setServiceNamespace(serviceNamespace);
return this;
}
/**
*
* The namespace of the AWS service that provides the resource or custom-resource
for a resource
* provided by your own application or service. For more information, see AWS Service Namespaces in the Amazon Web Services General Reference.
*
*
* @param serviceNamespace
* The namespace of the AWS service that provides the resource or custom-resource
for a resource
* provided by your own application or service. For more information, see AWS Service Namespaces in the Amazon Web Services General Reference.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ServiceNamespace
*/
public DescribeScheduledActionsRequest withServiceNamespace(ServiceNamespace serviceNamespace) {
this.serviceNamespace = serviceNamespace.toString();
return this;
}
/**
*
* The identifier of the resource associated with the scheduled action. This string consists of the resource type
* and unique identifier. If you specify a scalable dimension, you must also specify a resource ID.
*
*
* -
*
* ECS service - The resource type is service
and the unique identifier is the cluster name and service
* name. Example: service/default/sample-webapp
.
*
*
* -
*
* Spot fleet request - The resource type is spot-fleet-request
and the unique identifier is the Spot
* fleet request ID. Example: spot-fleet-request/sfr-73fbd2ce-aa30-494c-8788-1cee4EXAMPLE
.
*
*
* -
*
* EMR cluster - The resource type is instancegroup
and the unique identifier is the cluster ID and
* instance group ID. Example: instancegroup/j-2EEZNYKUA1NTV/ig-1791Y4E1L8YI0
.
*
*
* -
*
* AppStream 2.0 fleet - The resource type is fleet
and the unique identifier is the fleet name.
* Example: fleet/sample-fleet
.
*
*
* -
*
* DynamoDB table - The resource type is table
and the unique identifier is the resource ID. Example:
* table/my-table
.
*
*
* -
*
* DynamoDB global secondary index - The resource type is index
and the unique identifier is the
* resource ID. Example: table/my-table/index/my-table-index
.
*
*
* -
*
* Aurora DB cluster - The resource type is cluster
and the unique identifier is the cluster name.
* Example: cluster:my-db-cluster
.
*
*
* -
*
* Amazon SageMaker endpoint variants - The resource type is variant
and the unique identifier is the
* resource ID. Example: endpoint/my-end-point/variant/KMeansClustering
.
*
*
* -
*
* Custom resources are not supported with a resource type. This parameter must specify the OutputValue
* from the CloudFormation template stack used to access the resources. The unique identifier is defined by the
* service provider.
*
*
*
*
* @param resourceId
* The identifier of the resource associated with the scheduled action. This string consists of the resource
* type and unique identifier. If you specify a scalable dimension, you must also specify a resource ID.
*
* -
*
* ECS service - The resource type is service
and the unique identifier is the cluster name and
* service name. Example: service/default/sample-webapp
.
*
*
* -
*
* Spot fleet request - The resource type is spot-fleet-request
and the unique identifier is the
* Spot fleet request ID. Example: spot-fleet-request/sfr-73fbd2ce-aa30-494c-8788-1cee4EXAMPLE
.
*
*
* -
*
* EMR cluster - The resource type is instancegroup
and the unique identifier is the cluster ID
* and instance group ID. Example: instancegroup/j-2EEZNYKUA1NTV/ig-1791Y4E1L8YI0
.
*
*
* -
*
* AppStream 2.0 fleet - The resource type is fleet
and the unique identifier is the fleet name.
* Example: fleet/sample-fleet
.
*
*
* -
*
* DynamoDB table - The resource type is table
and the unique identifier is the resource ID.
* Example: table/my-table
.
*
*
* -
*
* DynamoDB global secondary index - The resource type is index
and the unique identifier is the
* resource ID. Example: table/my-table/index/my-table-index
.
*
*
* -
*
* Aurora DB cluster - The resource type is cluster
and the unique identifier is the cluster
* name. Example: cluster:my-db-cluster
.
*
*
* -
*
* Amazon SageMaker endpoint variants - The resource type is variant
and the unique identifier
* is the resource ID. Example: endpoint/my-end-point/variant/KMeansClustering
.
*
*
* -
*
* Custom resources are not supported with a resource type. This parameter must specify the
* OutputValue
from the CloudFormation template stack used to access the resources. The unique
* identifier is defined by the service provider.
*
*
*/
public void setResourceId(String resourceId) {
this.resourceId = resourceId;
}
/**
*
* The identifier of the resource associated with the scheduled action. This string consists of the resource type
* and unique identifier. If you specify a scalable dimension, you must also specify a resource ID.
*
*
* -
*
* ECS service - The resource type is service
and the unique identifier is the cluster name and service
* name. Example: service/default/sample-webapp
.
*
*
* -
*
* Spot fleet request - The resource type is spot-fleet-request
and the unique identifier is the Spot
* fleet request ID. Example: spot-fleet-request/sfr-73fbd2ce-aa30-494c-8788-1cee4EXAMPLE
.
*
*
* -
*
* EMR cluster - The resource type is instancegroup
and the unique identifier is the cluster ID and
* instance group ID. Example: instancegroup/j-2EEZNYKUA1NTV/ig-1791Y4E1L8YI0
.
*
*
* -
*
* AppStream 2.0 fleet - The resource type is fleet
and the unique identifier is the fleet name.
* Example: fleet/sample-fleet
.
*
*
* -
*
* DynamoDB table - The resource type is table
and the unique identifier is the resource ID. Example:
* table/my-table
.
*
*
* -
*
* DynamoDB global secondary index - The resource type is index
and the unique identifier is the
* resource ID. Example: table/my-table/index/my-table-index
.
*
*
* -
*
* Aurora DB cluster - The resource type is cluster
and the unique identifier is the cluster name.
* Example: cluster:my-db-cluster
.
*
*
* -
*
* Amazon SageMaker endpoint variants - The resource type is variant
and the unique identifier is the
* resource ID. Example: endpoint/my-end-point/variant/KMeansClustering
.
*
*
* -
*
* Custom resources are not supported with a resource type. This parameter must specify the OutputValue
* from the CloudFormation template stack used to access the resources. The unique identifier is defined by the
* service provider.
*
*
*
*
* @return The identifier of the resource associated with the scheduled action. This string consists of the resource
* type and unique identifier. If you specify a scalable dimension, you must also specify a resource ID.
*
* -
*
* ECS service - The resource type is service
and the unique identifier is the cluster name and
* service name. Example: service/default/sample-webapp
.
*
*
* -
*
* Spot fleet request - The resource type is spot-fleet-request
and the unique identifier is
* the Spot fleet request ID. Example:
* spot-fleet-request/sfr-73fbd2ce-aa30-494c-8788-1cee4EXAMPLE
.
*
*
* -
*
* EMR cluster - The resource type is instancegroup
and the unique identifier is the cluster ID
* and instance group ID. Example: instancegroup/j-2EEZNYKUA1NTV/ig-1791Y4E1L8YI0
.
*
*
* -
*
* AppStream 2.0 fleet - The resource type is fleet
and the unique identifier is the fleet
* name. Example: fleet/sample-fleet
.
*
*
* -
*
* DynamoDB table - The resource type is table
and the unique identifier is the resource ID.
* Example: table/my-table
.
*
*
* -
*
* DynamoDB global secondary index - The resource type is index
and the unique identifier is
* the resource ID. Example: table/my-table/index/my-table-index
.
*
*
* -
*
* Aurora DB cluster - The resource type is cluster
and the unique identifier is the cluster
* name. Example: cluster:my-db-cluster
.
*
*
* -
*
* Amazon SageMaker endpoint variants - The resource type is variant
and the unique identifier
* is the resource ID. Example: endpoint/my-end-point/variant/KMeansClustering
.
*
*
* -
*
* Custom resources are not supported with a resource type. This parameter must specify the
* OutputValue
from the CloudFormation template stack used to access the resources. The unique
* identifier is defined by the service provider.
*
*
*/
public String getResourceId() {
return this.resourceId;
}
/**
*
* The identifier of the resource associated with the scheduled action. This string consists of the resource type
* and unique identifier. If you specify a scalable dimension, you must also specify a resource ID.
*
*
* -
*
* ECS service - The resource type is service
and the unique identifier is the cluster name and service
* name. Example: service/default/sample-webapp
.
*
*
* -
*
* Spot fleet request - The resource type is spot-fleet-request
and the unique identifier is the Spot
* fleet request ID. Example: spot-fleet-request/sfr-73fbd2ce-aa30-494c-8788-1cee4EXAMPLE
.
*
*
* -
*
* EMR cluster - The resource type is instancegroup
and the unique identifier is the cluster ID and
* instance group ID. Example: instancegroup/j-2EEZNYKUA1NTV/ig-1791Y4E1L8YI0
.
*
*
* -
*
* AppStream 2.0 fleet - The resource type is fleet
and the unique identifier is the fleet name.
* Example: fleet/sample-fleet
.
*
*
* -
*
* DynamoDB table - The resource type is table
and the unique identifier is the resource ID. Example:
* table/my-table
.
*
*
* -
*
* DynamoDB global secondary index - The resource type is index
and the unique identifier is the
* resource ID. Example: table/my-table/index/my-table-index
.
*
*
* -
*
* Aurora DB cluster - The resource type is cluster
and the unique identifier is the cluster name.
* Example: cluster:my-db-cluster
.
*
*
* -
*
* Amazon SageMaker endpoint variants - The resource type is variant
and the unique identifier is the
* resource ID. Example: endpoint/my-end-point/variant/KMeansClustering
.
*
*
* -
*
* Custom resources are not supported with a resource type. This parameter must specify the OutputValue
* from the CloudFormation template stack used to access the resources. The unique identifier is defined by the
* service provider.
*
*
*
*
* @param resourceId
* The identifier of the resource associated with the scheduled action. This string consists of the resource
* type and unique identifier. If you specify a scalable dimension, you must also specify a resource ID.
*
* -
*
* ECS service - The resource type is service
and the unique identifier is the cluster name and
* service name. Example: service/default/sample-webapp
.
*
*
* -
*
* Spot fleet request - The resource type is spot-fleet-request
and the unique identifier is the
* Spot fleet request ID. Example: spot-fleet-request/sfr-73fbd2ce-aa30-494c-8788-1cee4EXAMPLE
.
*
*
* -
*
* EMR cluster - The resource type is instancegroup
and the unique identifier is the cluster ID
* and instance group ID. Example: instancegroup/j-2EEZNYKUA1NTV/ig-1791Y4E1L8YI0
.
*
*
* -
*
* AppStream 2.0 fleet - The resource type is fleet
and the unique identifier is the fleet name.
* Example: fleet/sample-fleet
.
*
*
* -
*
* DynamoDB table - The resource type is table
and the unique identifier is the resource ID.
* Example: table/my-table
.
*
*
* -
*
* DynamoDB global secondary index - The resource type is index
and the unique identifier is the
* resource ID. Example: table/my-table/index/my-table-index
.
*
*
* -
*
* Aurora DB cluster - The resource type is cluster
and the unique identifier is the cluster
* name. Example: cluster:my-db-cluster
.
*
*
* -
*
* Amazon SageMaker endpoint variants - The resource type is variant
and the unique identifier
* is the resource ID. Example: endpoint/my-end-point/variant/KMeansClustering
.
*
*
* -
*
* Custom resources are not supported with a resource type. This parameter must specify the
* OutputValue
from the CloudFormation template stack used to access the resources. The unique
* identifier is defined by the service provider.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeScheduledActionsRequest withResourceId(String resourceId) {
setResourceId(resourceId);
return this;
}
/**
*
* The scalable dimension. This string consists of the service namespace, resource type, and scaling property. If
* you specify a scalable dimension, you must also specify a resource ID.
*
*
* -
*
* ecs:service:DesiredCount
- The desired task count of an ECS service.
*
*
* -
*
* ec2:spot-fleet-request:TargetCapacity
- The target capacity of a Spot fleet request.
*
*
* -
*
* elasticmapreduce:instancegroup:InstanceCount
- The instance count of an EMR Instance Group.
*
*
* -
*
* appstream:fleet:DesiredCapacity
- The desired capacity of an AppStream 2.0 fleet.
*
*
* -
*
* dynamodb:table:ReadCapacityUnits
- The provisioned read capacity for a DynamoDB table.
*
*
* -
*
* dynamodb:table:WriteCapacityUnits
- The provisioned write capacity for a DynamoDB table.
*
*
* -
*
* dynamodb:index:ReadCapacityUnits
- The provisioned read capacity for a DynamoDB global secondary
* index.
*
*
* -
*
* dynamodb:index:WriteCapacityUnits
- The provisioned write capacity for a DynamoDB global secondary
* index.
*
*
* -
*
* rds:cluster:ReadReplicaCount
- The count of Aurora Replicas in an Aurora DB cluster. Available for
* Aurora MySQL-compatible edition.
*
*
* -
*
* sagemaker:variant:DesiredInstanceCount
- The number of EC2 instances for an Amazon SageMaker model
* endpoint variant.
*
*
* -
*
* custom-resource:ResourceType:Property
- The scalable dimension for a custom resource provided by
* your own application or service.
*
*
*
*
* @param scalableDimension
* The scalable dimension. This string consists of the service namespace, resource type, and scaling
* property. If you specify a scalable dimension, you must also specify a resource ID.
*
* -
*
* ecs:service:DesiredCount
- The desired task count of an ECS service.
*
*
* -
*
* ec2:spot-fleet-request:TargetCapacity
- The target capacity of a Spot fleet request.
*
*
* -
*
* elasticmapreduce:instancegroup:InstanceCount
- The instance count of an EMR Instance Group.
*
*
* -
*
* appstream:fleet:DesiredCapacity
- The desired capacity of an AppStream 2.0 fleet.
*
*
* -
*
* dynamodb:table:ReadCapacityUnits
- The provisioned read capacity for a DynamoDB table.
*
*
* -
*
* dynamodb:table:WriteCapacityUnits
- The provisioned write capacity for a DynamoDB table.
*
*
* -
*
* dynamodb:index:ReadCapacityUnits
- The provisioned read capacity for a DynamoDB global
* secondary index.
*
*
* -
*
* dynamodb:index:WriteCapacityUnits
- The provisioned write capacity for a DynamoDB global
* secondary index.
*
*
* -
*
* rds:cluster:ReadReplicaCount
- The count of Aurora Replicas in an Aurora DB cluster.
* Available for Aurora MySQL-compatible edition.
*
*
* -
*
* sagemaker:variant:DesiredInstanceCount
- The number of EC2 instances for an Amazon SageMaker
* model endpoint variant.
*
*
* -
*
* custom-resource:ResourceType:Property
- The scalable dimension for a custom resource provided
* by your own application or service.
*
*
* @see ScalableDimension
*/
public void setScalableDimension(String scalableDimension) {
this.scalableDimension = scalableDimension;
}
/**
*
* The scalable dimension. This string consists of the service namespace, resource type, and scaling property. If
* you specify a scalable dimension, you must also specify a resource ID.
*
*
* -
*
* ecs:service:DesiredCount
- The desired task count of an ECS service.
*
*
* -
*
* ec2:spot-fleet-request:TargetCapacity
- The target capacity of a Spot fleet request.
*
*
* -
*
* elasticmapreduce:instancegroup:InstanceCount
- The instance count of an EMR Instance Group.
*
*
* -
*
* appstream:fleet:DesiredCapacity
- The desired capacity of an AppStream 2.0 fleet.
*
*
* -
*
* dynamodb:table:ReadCapacityUnits
- The provisioned read capacity for a DynamoDB table.
*
*
* -
*
* dynamodb:table:WriteCapacityUnits
- The provisioned write capacity for a DynamoDB table.
*
*
* -
*
* dynamodb:index:ReadCapacityUnits
- The provisioned read capacity for a DynamoDB global secondary
* index.
*
*
* -
*
* dynamodb:index:WriteCapacityUnits
- The provisioned write capacity for a DynamoDB global secondary
* index.
*
*
* -
*
* rds:cluster:ReadReplicaCount
- The count of Aurora Replicas in an Aurora DB cluster. Available for
* Aurora MySQL-compatible edition.
*
*
* -
*
* sagemaker:variant:DesiredInstanceCount
- The number of EC2 instances for an Amazon SageMaker model
* endpoint variant.
*
*
* -
*
* custom-resource:ResourceType:Property
- The scalable dimension for a custom resource provided by
* your own application or service.
*
*
*
*
* @return The scalable dimension. This string consists of the service namespace, resource type, and scaling
* property. If you specify a scalable dimension, you must also specify a resource ID.
*
* -
*
* ecs:service:DesiredCount
- The desired task count of an ECS service.
*
*
* -
*
* ec2:spot-fleet-request:TargetCapacity
- The target capacity of a Spot fleet request.
*
*
* -
*
* elasticmapreduce:instancegroup:InstanceCount
- The instance count of an EMR Instance Group.
*
*
* -
*
* appstream:fleet:DesiredCapacity
- The desired capacity of an AppStream 2.0 fleet.
*
*
* -
*
* dynamodb:table:ReadCapacityUnits
- The provisioned read capacity for a DynamoDB table.
*
*
* -
*
* dynamodb:table:WriteCapacityUnits
- The provisioned write capacity for a DynamoDB table.
*
*
* -
*
* dynamodb:index:ReadCapacityUnits
- The provisioned read capacity for a DynamoDB global
* secondary index.
*
*
* -
*
* dynamodb:index:WriteCapacityUnits
- The provisioned write capacity for a DynamoDB global
* secondary index.
*
*
* -
*
* rds:cluster:ReadReplicaCount
- The count of Aurora Replicas in an Aurora DB cluster.
* Available for Aurora MySQL-compatible edition.
*
*
* -
*
* sagemaker:variant:DesiredInstanceCount
- The number of EC2 instances for an Amazon SageMaker
* model endpoint variant.
*
*
* -
*
* custom-resource:ResourceType:Property
- The scalable dimension for a custom resource
* provided by your own application or service.
*
*
* @see ScalableDimension
*/
public String getScalableDimension() {
return this.scalableDimension;
}
/**
*
* The scalable dimension. This string consists of the service namespace, resource type, and scaling property. If
* you specify a scalable dimension, you must also specify a resource ID.
*
*
* -
*
* ecs:service:DesiredCount
- The desired task count of an ECS service.
*
*
* -
*
* ec2:spot-fleet-request:TargetCapacity
- The target capacity of a Spot fleet request.
*
*
* -
*
* elasticmapreduce:instancegroup:InstanceCount
- The instance count of an EMR Instance Group.
*
*
* -
*
* appstream:fleet:DesiredCapacity
- The desired capacity of an AppStream 2.0 fleet.
*
*
* -
*
* dynamodb:table:ReadCapacityUnits
- The provisioned read capacity for a DynamoDB table.
*
*
* -
*
* dynamodb:table:WriteCapacityUnits
- The provisioned write capacity for a DynamoDB table.
*
*
* -
*
* dynamodb:index:ReadCapacityUnits
- The provisioned read capacity for a DynamoDB global secondary
* index.
*
*
* -
*
* dynamodb:index:WriteCapacityUnits
- The provisioned write capacity for a DynamoDB global secondary
* index.
*
*
* -
*
* rds:cluster:ReadReplicaCount
- The count of Aurora Replicas in an Aurora DB cluster. Available for
* Aurora MySQL-compatible edition.
*
*
* -
*
* sagemaker:variant:DesiredInstanceCount
- The number of EC2 instances for an Amazon SageMaker model
* endpoint variant.
*
*
* -
*
* custom-resource:ResourceType:Property
- The scalable dimension for a custom resource provided by
* your own application or service.
*
*
*
*
* @param scalableDimension
* The scalable dimension. This string consists of the service namespace, resource type, and scaling
* property. If you specify a scalable dimension, you must also specify a resource ID.
*
* -
*
* ecs:service:DesiredCount
- The desired task count of an ECS service.
*
*
* -
*
* ec2:spot-fleet-request:TargetCapacity
- The target capacity of a Spot fleet request.
*
*
* -
*
* elasticmapreduce:instancegroup:InstanceCount
- The instance count of an EMR Instance Group.
*
*
* -
*
* appstream:fleet:DesiredCapacity
- The desired capacity of an AppStream 2.0 fleet.
*
*
* -
*
* dynamodb:table:ReadCapacityUnits
- The provisioned read capacity for a DynamoDB table.
*
*
* -
*
* dynamodb:table:WriteCapacityUnits
- The provisioned write capacity for a DynamoDB table.
*
*
* -
*
* dynamodb:index:ReadCapacityUnits
- The provisioned read capacity for a DynamoDB global
* secondary index.
*
*
* -
*
* dynamodb:index:WriteCapacityUnits
- The provisioned write capacity for a DynamoDB global
* secondary index.
*
*
* -
*
* rds:cluster:ReadReplicaCount
- The count of Aurora Replicas in an Aurora DB cluster.
* Available for Aurora MySQL-compatible edition.
*
*
* -
*
* sagemaker:variant:DesiredInstanceCount
- The number of EC2 instances for an Amazon SageMaker
* model endpoint variant.
*
*
* -
*
* custom-resource:ResourceType:Property
- The scalable dimension for a custom resource provided
* by your own application or service.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ScalableDimension
*/
public DescribeScheduledActionsRequest withScalableDimension(String scalableDimension) {
setScalableDimension(scalableDimension);
return this;
}
/**
*
* The scalable dimension. This string consists of the service namespace, resource type, and scaling property. If
* you specify a scalable dimension, you must also specify a resource ID.
*
*
* -
*
* ecs:service:DesiredCount
- The desired task count of an ECS service.
*
*
* -
*
* ec2:spot-fleet-request:TargetCapacity
- The target capacity of a Spot fleet request.
*
*
* -
*
* elasticmapreduce:instancegroup:InstanceCount
- The instance count of an EMR Instance Group.
*
*
* -
*
* appstream:fleet:DesiredCapacity
- The desired capacity of an AppStream 2.0 fleet.
*
*
* -
*
* dynamodb:table:ReadCapacityUnits
- The provisioned read capacity for a DynamoDB table.
*
*
* -
*
* dynamodb:table:WriteCapacityUnits
- The provisioned write capacity for a DynamoDB table.
*
*
* -
*
* dynamodb:index:ReadCapacityUnits
- The provisioned read capacity for a DynamoDB global secondary
* index.
*
*
* -
*
* dynamodb:index:WriteCapacityUnits
- The provisioned write capacity for a DynamoDB global secondary
* index.
*
*
* -
*
* rds:cluster:ReadReplicaCount
- The count of Aurora Replicas in an Aurora DB cluster. Available for
* Aurora MySQL-compatible edition.
*
*
* -
*
* sagemaker:variant:DesiredInstanceCount
- The number of EC2 instances for an Amazon SageMaker model
* endpoint variant.
*
*
* -
*
* custom-resource:ResourceType:Property
- The scalable dimension for a custom resource provided by
* your own application or service.
*
*
*
*
* @param scalableDimension
* The scalable dimension. This string consists of the service namespace, resource type, and scaling
* property. If you specify a scalable dimension, you must also specify a resource ID.
*
* -
*
* ecs:service:DesiredCount
- The desired task count of an ECS service.
*
*
* -
*
* ec2:spot-fleet-request:TargetCapacity
- The target capacity of a Spot fleet request.
*
*
* -
*
* elasticmapreduce:instancegroup:InstanceCount
- The instance count of an EMR Instance Group.
*
*
* -
*
* appstream:fleet:DesiredCapacity
- The desired capacity of an AppStream 2.0 fleet.
*
*
* -
*
* dynamodb:table:ReadCapacityUnits
- The provisioned read capacity for a DynamoDB table.
*
*
* -
*
* dynamodb:table:WriteCapacityUnits
- The provisioned write capacity for a DynamoDB table.
*
*
* -
*
* dynamodb:index:ReadCapacityUnits
- The provisioned read capacity for a DynamoDB global
* secondary index.
*
*
* -
*
* dynamodb:index:WriteCapacityUnits
- The provisioned write capacity for a DynamoDB global
* secondary index.
*
*
* -
*
* rds:cluster:ReadReplicaCount
- The count of Aurora Replicas in an Aurora DB cluster.
* Available for Aurora MySQL-compatible edition.
*
*
* -
*
* sagemaker:variant:DesiredInstanceCount
- The number of EC2 instances for an Amazon SageMaker
* model endpoint variant.
*
*
* -
*
* custom-resource:ResourceType:Property
- The scalable dimension for a custom resource provided
* by your own application or service.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ScalableDimension
*/
public DescribeScheduledActionsRequest withScalableDimension(ScalableDimension scalableDimension) {
this.scalableDimension = scalableDimension.toString();
return this;
}
/**
*
* The maximum number of scheduled action results. This value can be between 1 and 50. The default value is 50.
*
*
* If this parameter is used, the operation returns up to MaxResults
results at a time, along with a
* NextToken
value. To get the next set of results, include the NextToken
value in a
* subsequent call. If this parameter is not used, the operation returns up to 50 results and a
* NextToken
value, if applicable.
*
*
* @param maxResults
* The maximum number of scheduled action results. This value can be between 1 and 50. The default value is
* 50.
*
* If this parameter is used, the operation returns up to MaxResults
results at a time, along
* with a NextToken
value. To get the next set of results, include the NextToken
* value in a subsequent call. If this parameter is not used, the operation returns up to 50 results and a
* NextToken
value, if applicable.
*/
public void setMaxResults(Integer maxResults) {
this.maxResults = maxResults;
}
/**
*
* The maximum number of scheduled action results. This value can be between 1 and 50. The default value is 50.
*
*
* If this parameter is used, the operation returns up to MaxResults
results at a time, along with a
* NextToken
value. To get the next set of results, include the NextToken
value in a
* subsequent call. If this parameter is not used, the operation returns up to 50 results and a
* NextToken
value, if applicable.
*
*
* @return The maximum number of scheduled action results. This value can be between 1 and 50. The default value is
* 50.
*
* If this parameter is used, the operation returns up to MaxResults
results at a time, along
* with a NextToken
value. To get the next set of results, include the NextToken
* value in a subsequent call. If this parameter is not used, the operation returns up to 50 results and a
* NextToken
value, if applicable.
*/
public Integer getMaxResults() {
return this.maxResults;
}
/**
*
* The maximum number of scheduled action results. This value can be between 1 and 50. The default value is 50.
*
*
* If this parameter is used, the operation returns up to MaxResults
results at a time, along with a
* NextToken
value. To get the next set of results, include the NextToken
value in a
* subsequent call. If this parameter is not used, the operation returns up to 50 results and a
* NextToken
value, if applicable.
*
*
* @param maxResults
* The maximum number of scheduled action results. This value can be between 1 and 50. The default value is
* 50.
*
* If this parameter is used, the operation returns up to MaxResults
results at a time, along
* with a NextToken
value. To get the next set of results, include the NextToken
* value in a subsequent call. If this parameter is not used, the operation returns up to 50 results and a
* NextToken
value, if applicable.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeScheduledActionsRequest withMaxResults(Integer maxResults) {
setMaxResults(maxResults);
return this;
}
/**
*
* The token for the next set of results.
*
*
* @param nextToken
* The token for the next set of results.
*/
public void setNextToken(String nextToken) {
this.nextToken = nextToken;
}
/**
*
* The token for the next set of results.
*
*
* @return The token for the next set of results.
*/
public String getNextToken() {
return this.nextToken;
}
/**
*
* The token for the next set of results.
*
*
* @param nextToken
* The token for the next set of results.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeScheduledActionsRequest withNextToken(String nextToken) {
setNextToken(nextToken);
return this;
}
/**
* Returns a string representation of this object; useful for testing and debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getScheduledActionNames() != null)
sb.append("ScheduledActionNames: ").append(getScheduledActionNames()).append(",");
if (getServiceNamespace() != null)
sb.append("ServiceNamespace: ").append(getServiceNamespace()).append(",");
if (getResourceId() != null)
sb.append("ResourceId: ").append(getResourceId()).append(",");
if (getScalableDimension() != null)
sb.append("ScalableDimension: ").append(getScalableDimension()).append(",");
if (getMaxResults() != null)
sb.append("MaxResults: ").append(getMaxResults()).append(",");
if (getNextToken() != null)
sb.append("NextToken: ").append(getNextToken());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof DescribeScheduledActionsRequest == false)
return false;
DescribeScheduledActionsRequest other = (DescribeScheduledActionsRequest) obj;
if (other.getScheduledActionNames() == null ^ this.getScheduledActionNames() == null)
return false;
if (other.getScheduledActionNames() != null && other.getScheduledActionNames().equals(this.getScheduledActionNames()) == false)
return false;
if (other.getServiceNamespace() == null ^ this.getServiceNamespace() == null)
return false;
if (other.getServiceNamespace() != null && other.getServiceNamespace().equals(this.getServiceNamespace()) == false)
return false;
if (other.getResourceId() == null ^ this.getResourceId() == null)
return false;
if (other.getResourceId() != null && other.getResourceId().equals(this.getResourceId()) == false)
return false;
if (other.getScalableDimension() == null ^ this.getScalableDimension() == null)
return false;
if (other.getScalableDimension() != null && other.getScalableDimension().equals(this.getScalableDimension()) == false)
return false;
if (other.getMaxResults() == null ^ this.getMaxResults() == null)
return false;
if (other.getMaxResults() != null && other.getMaxResults().equals(this.getMaxResults()) == false)
return false;
if (other.getNextToken() == null ^ this.getNextToken() == null)
return false;
if (other.getNextToken() != null && other.getNextToken().equals(this.getNextToken()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getScheduledActionNames() == null) ? 0 : getScheduledActionNames().hashCode());
hashCode = prime * hashCode + ((getServiceNamespace() == null) ? 0 : getServiceNamespace().hashCode());
hashCode = prime * hashCode + ((getResourceId() == null) ? 0 : getResourceId().hashCode());
hashCode = prime * hashCode + ((getScalableDimension() == null) ? 0 : getScalableDimension().hashCode());
hashCode = prime * hashCode + ((getMaxResults() == null) ? 0 : getMaxResults().hashCode());
hashCode = prime * hashCode + ((getNextToken() == null) ? 0 : getNextToken().hashCode());
return hashCode;
}
@Override
public DescribeScheduledActionsRequest clone() {
return (DescribeScheduledActionsRequest) super.clone();
}
}