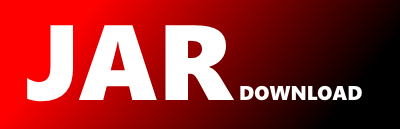
com.amazonaws.services.applicationautoscaling.model.ScalingPolicy Maven / Gradle / Ivy
Show all versions of aws-java-sdk-applicationautoscaling Show documentation
/*
* Copyright 2011-2016 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.applicationautoscaling.model;
import java.io.Serializable;
/**
*
* An object representing a scaling policy.
*
*/
public class ScalingPolicy implements Serializable, Cloneable {
/**
*
* The Amazon Resource Name (ARN) of the scaling policy.
*
*/
private String policyARN;
/**
*
* The name of the scaling policy.
*
*/
private String policyName;
/**
*
* The namespace for the AWS service that the scaling policy is associated with. For more information, see AWS Service Namespaces in the Amazon Web Services General Reference.
*
*/
private String serviceNamespace;
/**
*
* The resource type and unique identifier string for the resource associated with the scaling policy. For Amazon
* ECS services, the resource type is services
, and the identifier is the cluster name and service
* name; for example, service/default/sample-webapp
. For Amazon EC2 Spot fleet requests, the resource
* type is spot-fleet-request
, and the identifier is the Spot fleet request ID; for example,
* spot-fleet-request/sfr-73fbd2ce-aa30-494c-8788-1cee4EXAMPLE
.
*
*/
private String resourceId;
/**
*
* The scalable dimension associated with the scaling policy. The scalable dimension contains the service namespace,
* resource type, and scaling property, such as ecs:service:DesiredCount
for the desired task count of
* an Amazon ECS service, or ec2:spot-fleet-request:TargetCapacity
for the target capacity of an Amazon
* EC2 Spot fleet request.
*
*/
private String scalableDimension;
/**
*
* The scaling policy type.
*
*/
private String policyType;
/**
*
* The configuration for the step scaling policy.
*
*/
private StepScalingPolicyConfiguration stepScalingPolicyConfiguration;
/**
*
* The CloudWatch alarms that are associated with the scaling policy.
*
*/
private java.util.List alarms;
/**
*
* The Unix timestamp for when the scaling policy was created.
*
*/
private java.util.Date creationTime;
/**
*
* The Amazon Resource Name (ARN) of the scaling policy.
*
*
* @param policyARN
* The Amazon Resource Name (ARN) of the scaling policy.
*/
public void setPolicyARN(String policyARN) {
this.policyARN = policyARN;
}
/**
*
* The Amazon Resource Name (ARN) of the scaling policy.
*
*
* @return The Amazon Resource Name (ARN) of the scaling policy.
*/
public String getPolicyARN() {
return this.policyARN;
}
/**
*
* The Amazon Resource Name (ARN) of the scaling policy.
*
*
* @param policyARN
* The Amazon Resource Name (ARN) of the scaling policy.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ScalingPolicy withPolicyARN(String policyARN) {
setPolicyARN(policyARN);
return this;
}
/**
*
* The name of the scaling policy.
*
*
* @param policyName
* The name of the scaling policy.
*/
public void setPolicyName(String policyName) {
this.policyName = policyName;
}
/**
*
* The name of the scaling policy.
*
*
* @return The name of the scaling policy.
*/
public String getPolicyName() {
return this.policyName;
}
/**
*
* The name of the scaling policy.
*
*
* @param policyName
* The name of the scaling policy.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ScalingPolicy withPolicyName(String policyName) {
setPolicyName(policyName);
return this;
}
/**
*
* The namespace for the AWS service that the scaling policy is associated with. For more information, see AWS Service Namespaces in the Amazon Web Services General Reference.
*
*
* @param serviceNamespace
* The namespace for the AWS service that the scaling policy is associated with. For more information, see AWS Service Namespaces in the Amazon Web Services General Reference.
* @see ServiceNamespace
*/
public void setServiceNamespace(String serviceNamespace) {
this.serviceNamespace = serviceNamespace;
}
/**
*
* The namespace for the AWS service that the scaling policy is associated with. For more information, see AWS Service Namespaces in the Amazon Web Services General Reference.
*
*
* @return The namespace for the AWS service that the scaling policy is associated with. For more information, see
* AWS Service Namespaces in the Amazon Web Services General Reference.
* @see ServiceNamespace
*/
public String getServiceNamespace() {
return this.serviceNamespace;
}
/**
*
* The namespace for the AWS service that the scaling policy is associated with. For more information, see AWS Service Namespaces in the Amazon Web Services General Reference.
*
*
* @param serviceNamespace
* The namespace for the AWS service that the scaling policy is associated with. For more information, see AWS Service Namespaces in the Amazon Web Services General Reference.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ServiceNamespace
*/
public ScalingPolicy withServiceNamespace(String serviceNamespace) {
setServiceNamespace(serviceNamespace);
return this;
}
/**
*
* The namespace for the AWS service that the scaling policy is associated with. For more information, see AWS Service Namespaces in the Amazon Web Services General Reference.
*
*
* @param serviceNamespace
* The namespace for the AWS service that the scaling policy is associated with. For more information, see AWS Service Namespaces in the Amazon Web Services General Reference.
* @see ServiceNamespace
*/
public void setServiceNamespace(ServiceNamespace serviceNamespace) {
this.serviceNamespace = serviceNamespace.toString();
}
/**
*
* The namespace for the AWS service that the scaling policy is associated with. For more information, see AWS Service Namespaces in the Amazon Web Services General Reference.
*
*
* @param serviceNamespace
* The namespace for the AWS service that the scaling policy is associated with. For more information, see AWS Service Namespaces in the Amazon Web Services General Reference.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ServiceNamespace
*/
public ScalingPolicy withServiceNamespace(ServiceNamespace serviceNamespace) {
setServiceNamespace(serviceNamespace);
return this;
}
/**
*
* The resource type and unique identifier string for the resource associated with the scaling policy. For Amazon
* ECS services, the resource type is services
, and the identifier is the cluster name and service
* name; for example, service/default/sample-webapp
. For Amazon EC2 Spot fleet requests, the resource
* type is spot-fleet-request
, and the identifier is the Spot fleet request ID; for example,
* spot-fleet-request/sfr-73fbd2ce-aa30-494c-8788-1cee4EXAMPLE
.
*
*
* @param resourceId
* The resource type and unique identifier string for the resource associated with the scaling policy. For
* Amazon ECS services, the resource type is services
, and the identifier is the cluster name
* and service name; for example, service/default/sample-webapp
. For Amazon EC2 Spot fleet
* requests, the resource type is spot-fleet-request
, and the identifier is the Spot fleet
* request ID; for example, spot-fleet-request/sfr-73fbd2ce-aa30-494c-8788-1cee4EXAMPLE
.
*/
public void setResourceId(String resourceId) {
this.resourceId = resourceId;
}
/**
*
* The resource type and unique identifier string for the resource associated with the scaling policy. For Amazon
* ECS services, the resource type is services
, and the identifier is the cluster name and service
* name; for example, service/default/sample-webapp
. For Amazon EC2 Spot fleet requests, the resource
* type is spot-fleet-request
, and the identifier is the Spot fleet request ID; for example,
* spot-fleet-request/sfr-73fbd2ce-aa30-494c-8788-1cee4EXAMPLE
.
*
*
* @return The resource type and unique identifier string for the resource associated with the scaling policy. For
* Amazon ECS services, the resource type is services
, and the identifier is the cluster name
* and service name; for example, service/default/sample-webapp
. For Amazon EC2 Spot fleet
* requests, the resource type is spot-fleet-request
, and the identifier is the Spot fleet
* request ID; for example, spot-fleet-request/sfr-73fbd2ce-aa30-494c-8788-1cee4EXAMPLE
.
*/
public String getResourceId() {
return this.resourceId;
}
/**
*
* The resource type and unique identifier string for the resource associated with the scaling policy. For Amazon
* ECS services, the resource type is services
, and the identifier is the cluster name and service
* name; for example, service/default/sample-webapp
. For Amazon EC2 Spot fleet requests, the resource
* type is spot-fleet-request
, and the identifier is the Spot fleet request ID; for example,
* spot-fleet-request/sfr-73fbd2ce-aa30-494c-8788-1cee4EXAMPLE
.
*
*
* @param resourceId
* The resource type and unique identifier string for the resource associated with the scaling policy. For
* Amazon ECS services, the resource type is services
, and the identifier is the cluster name
* and service name; for example, service/default/sample-webapp
. For Amazon EC2 Spot fleet
* requests, the resource type is spot-fleet-request
, and the identifier is the Spot fleet
* request ID; for example, spot-fleet-request/sfr-73fbd2ce-aa30-494c-8788-1cee4EXAMPLE
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ScalingPolicy withResourceId(String resourceId) {
setResourceId(resourceId);
return this;
}
/**
*
* The scalable dimension associated with the scaling policy. The scalable dimension contains the service namespace,
* resource type, and scaling property, such as ecs:service:DesiredCount
for the desired task count of
* an Amazon ECS service, or ec2:spot-fleet-request:TargetCapacity
for the target capacity of an Amazon
* EC2 Spot fleet request.
*
*
* @param scalableDimension
* The scalable dimension associated with the scaling policy. The scalable dimension contains the service
* namespace, resource type, and scaling property, such as ecs:service:DesiredCount
for the
* desired task count of an Amazon ECS service, or ec2:spot-fleet-request:TargetCapacity
for the
* target capacity of an Amazon EC2 Spot fleet request.
* @see ScalableDimension
*/
public void setScalableDimension(String scalableDimension) {
this.scalableDimension = scalableDimension;
}
/**
*
* The scalable dimension associated with the scaling policy. The scalable dimension contains the service namespace,
* resource type, and scaling property, such as ecs:service:DesiredCount
for the desired task count of
* an Amazon ECS service, or ec2:spot-fleet-request:TargetCapacity
for the target capacity of an Amazon
* EC2 Spot fleet request.
*
*
* @return The scalable dimension associated with the scaling policy. The scalable dimension contains the service
* namespace, resource type, and scaling property, such as ecs:service:DesiredCount
for the
* desired task count of an Amazon ECS service, or ec2:spot-fleet-request:TargetCapacity
for
* the target capacity of an Amazon EC2 Spot fleet request.
* @see ScalableDimension
*/
public String getScalableDimension() {
return this.scalableDimension;
}
/**
*
* The scalable dimension associated with the scaling policy. The scalable dimension contains the service namespace,
* resource type, and scaling property, such as ecs:service:DesiredCount
for the desired task count of
* an Amazon ECS service, or ec2:spot-fleet-request:TargetCapacity
for the target capacity of an Amazon
* EC2 Spot fleet request.
*
*
* @param scalableDimension
* The scalable dimension associated with the scaling policy. The scalable dimension contains the service
* namespace, resource type, and scaling property, such as ecs:service:DesiredCount
for the
* desired task count of an Amazon ECS service, or ec2:spot-fleet-request:TargetCapacity
for the
* target capacity of an Amazon EC2 Spot fleet request.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ScalableDimension
*/
public ScalingPolicy withScalableDimension(String scalableDimension) {
setScalableDimension(scalableDimension);
return this;
}
/**
*
* The scalable dimension associated with the scaling policy. The scalable dimension contains the service namespace,
* resource type, and scaling property, such as ecs:service:DesiredCount
for the desired task count of
* an Amazon ECS service, or ec2:spot-fleet-request:TargetCapacity
for the target capacity of an Amazon
* EC2 Spot fleet request.
*
*
* @param scalableDimension
* The scalable dimension associated with the scaling policy. The scalable dimension contains the service
* namespace, resource type, and scaling property, such as ecs:service:DesiredCount
for the
* desired task count of an Amazon ECS service, or ec2:spot-fleet-request:TargetCapacity
for the
* target capacity of an Amazon EC2 Spot fleet request.
* @see ScalableDimension
*/
public void setScalableDimension(ScalableDimension scalableDimension) {
this.scalableDimension = scalableDimension.toString();
}
/**
*
* The scalable dimension associated with the scaling policy. The scalable dimension contains the service namespace,
* resource type, and scaling property, such as ecs:service:DesiredCount
for the desired task count of
* an Amazon ECS service, or ec2:spot-fleet-request:TargetCapacity
for the target capacity of an Amazon
* EC2 Spot fleet request.
*
*
* @param scalableDimension
* The scalable dimension associated with the scaling policy. The scalable dimension contains the service
* namespace, resource type, and scaling property, such as ecs:service:DesiredCount
for the
* desired task count of an Amazon ECS service, or ec2:spot-fleet-request:TargetCapacity
for the
* target capacity of an Amazon EC2 Spot fleet request.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ScalableDimension
*/
public ScalingPolicy withScalableDimension(ScalableDimension scalableDimension) {
setScalableDimension(scalableDimension);
return this;
}
/**
*
* The scaling policy type.
*
*
* @param policyType
* The scaling policy type.
* @see PolicyType
*/
public void setPolicyType(String policyType) {
this.policyType = policyType;
}
/**
*
* The scaling policy type.
*
*
* @return The scaling policy type.
* @see PolicyType
*/
public String getPolicyType() {
return this.policyType;
}
/**
*
* The scaling policy type.
*
*
* @param policyType
* The scaling policy type.
* @return Returns a reference to this object so that method calls can be chained together.
* @see PolicyType
*/
public ScalingPolicy withPolicyType(String policyType) {
setPolicyType(policyType);
return this;
}
/**
*
* The scaling policy type.
*
*
* @param policyType
* The scaling policy type.
* @see PolicyType
*/
public void setPolicyType(PolicyType policyType) {
this.policyType = policyType.toString();
}
/**
*
* The scaling policy type.
*
*
* @param policyType
* The scaling policy type.
* @return Returns a reference to this object so that method calls can be chained together.
* @see PolicyType
*/
public ScalingPolicy withPolicyType(PolicyType policyType) {
setPolicyType(policyType);
return this;
}
/**
*
* The configuration for the step scaling policy.
*
*
* @param stepScalingPolicyConfiguration
* The configuration for the step scaling policy.
*/
public void setStepScalingPolicyConfiguration(StepScalingPolicyConfiguration stepScalingPolicyConfiguration) {
this.stepScalingPolicyConfiguration = stepScalingPolicyConfiguration;
}
/**
*
* The configuration for the step scaling policy.
*
*
* @return The configuration for the step scaling policy.
*/
public StepScalingPolicyConfiguration getStepScalingPolicyConfiguration() {
return this.stepScalingPolicyConfiguration;
}
/**
*
* The configuration for the step scaling policy.
*
*
* @param stepScalingPolicyConfiguration
* The configuration for the step scaling policy.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ScalingPolicy withStepScalingPolicyConfiguration(StepScalingPolicyConfiguration stepScalingPolicyConfiguration) {
setStepScalingPolicyConfiguration(stepScalingPolicyConfiguration);
return this;
}
/**
*
* The CloudWatch alarms that are associated with the scaling policy.
*
*
* @return The CloudWatch alarms that are associated with the scaling policy.
*/
public java.util.List getAlarms() {
return alarms;
}
/**
*
* The CloudWatch alarms that are associated with the scaling policy.
*
*
* @param alarms
* The CloudWatch alarms that are associated with the scaling policy.
*/
public void setAlarms(java.util.Collection alarms) {
if (alarms == null) {
this.alarms = null;
return;
}
this.alarms = new java.util.ArrayList(alarms);
}
/**
*
* The CloudWatch alarms that are associated with the scaling policy.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAlarms(java.util.Collection)} or {@link #withAlarms(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param alarms
* The CloudWatch alarms that are associated with the scaling policy.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ScalingPolicy withAlarms(Alarm... alarms) {
if (this.alarms == null) {
setAlarms(new java.util.ArrayList(alarms.length));
}
for (Alarm ele : alarms) {
this.alarms.add(ele);
}
return this;
}
/**
*
* The CloudWatch alarms that are associated with the scaling policy.
*
*
* @param alarms
* The CloudWatch alarms that are associated with the scaling policy.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ScalingPolicy withAlarms(java.util.Collection alarms) {
setAlarms(alarms);
return this;
}
/**
*
* The Unix timestamp for when the scaling policy was created.
*
*
* @param creationTime
* The Unix timestamp for when the scaling policy was created.
*/
public void setCreationTime(java.util.Date creationTime) {
this.creationTime = creationTime;
}
/**
*
* The Unix timestamp for when the scaling policy was created.
*
*
* @return The Unix timestamp for when the scaling policy was created.
*/
public java.util.Date getCreationTime() {
return this.creationTime;
}
/**
*
* The Unix timestamp for when the scaling policy was created.
*
*
* @param creationTime
* The Unix timestamp for when the scaling policy was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ScalingPolicy withCreationTime(java.util.Date creationTime) {
setCreationTime(creationTime);
return this;
}
/**
* Returns a string representation of this object; useful for testing and debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getPolicyARN() != null)
sb.append("PolicyARN: " + getPolicyARN() + ",");
if (getPolicyName() != null)
sb.append("PolicyName: " + getPolicyName() + ",");
if (getServiceNamespace() != null)
sb.append("ServiceNamespace: " + getServiceNamespace() + ",");
if (getResourceId() != null)
sb.append("ResourceId: " + getResourceId() + ",");
if (getScalableDimension() != null)
sb.append("ScalableDimension: " + getScalableDimension() + ",");
if (getPolicyType() != null)
sb.append("PolicyType: " + getPolicyType() + ",");
if (getStepScalingPolicyConfiguration() != null)
sb.append("StepScalingPolicyConfiguration: " + getStepScalingPolicyConfiguration() + ",");
if (getAlarms() != null)
sb.append("Alarms: " + getAlarms() + ",");
if (getCreationTime() != null)
sb.append("CreationTime: " + getCreationTime());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ScalingPolicy == false)
return false;
ScalingPolicy other = (ScalingPolicy) obj;
if (other.getPolicyARN() == null ^ this.getPolicyARN() == null)
return false;
if (other.getPolicyARN() != null && other.getPolicyARN().equals(this.getPolicyARN()) == false)
return false;
if (other.getPolicyName() == null ^ this.getPolicyName() == null)
return false;
if (other.getPolicyName() != null && other.getPolicyName().equals(this.getPolicyName()) == false)
return false;
if (other.getServiceNamespace() == null ^ this.getServiceNamespace() == null)
return false;
if (other.getServiceNamespace() != null && other.getServiceNamespace().equals(this.getServiceNamespace()) == false)
return false;
if (other.getResourceId() == null ^ this.getResourceId() == null)
return false;
if (other.getResourceId() != null && other.getResourceId().equals(this.getResourceId()) == false)
return false;
if (other.getScalableDimension() == null ^ this.getScalableDimension() == null)
return false;
if (other.getScalableDimension() != null && other.getScalableDimension().equals(this.getScalableDimension()) == false)
return false;
if (other.getPolicyType() == null ^ this.getPolicyType() == null)
return false;
if (other.getPolicyType() != null && other.getPolicyType().equals(this.getPolicyType()) == false)
return false;
if (other.getStepScalingPolicyConfiguration() == null ^ this.getStepScalingPolicyConfiguration() == null)
return false;
if (other.getStepScalingPolicyConfiguration() != null
&& other.getStepScalingPolicyConfiguration().equals(this.getStepScalingPolicyConfiguration()) == false)
return false;
if (other.getAlarms() == null ^ this.getAlarms() == null)
return false;
if (other.getAlarms() != null && other.getAlarms().equals(this.getAlarms()) == false)
return false;
if (other.getCreationTime() == null ^ this.getCreationTime() == null)
return false;
if (other.getCreationTime() != null && other.getCreationTime().equals(this.getCreationTime()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getPolicyARN() == null) ? 0 : getPolicyARN().hashCode());
hashCode = prime * hashCode + ((getPolicyName() == null) ? 0 : getPolicyName().hashCode());
hashCode = prime * hashCode + ((getServiceNamespace() == null) ? 0 : getServiceNamespace().hashCode());
hashCode = prime * hashCode + ((getResourceId() == null) ? 0 : getResourceId().hashCode());
hashCode = prime * hashCode + ((getScalableDimension() == null) ? 0 : getScalableDimension().hashCode());
hashCode = prime * hashCode + ((getPolicyType() == null) ? 0 : getPolicyType().hashCode());
hashCode = prime * hashCode + ((getStepScalingPolicyConfiguration() == null) ? 0 : getStepScalingPolicyConfiguration().hashCode());
hashCode = prime * hashCode + ((getAlarms() == null) ? 0 : getAlarms().hashCode());
hashCode = prime * hashCode + ((getCreationTime() == null) ? 0 : getCreationTime().hashCode());
return hashCode;
}
@Override
public ScalingPolicy clone() {
try {
return (ScalingPolicy) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}