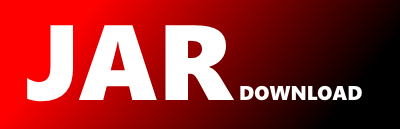
com.amazonaws.services.applicationautoscaling.AWSApplicationAutoScaling Maven / Gradle / Ivy
Show all versions of aws-java-sdk-applicationautoscaling Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.applicationautoscaling;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.applicationautoscaling.model.*;
/**
* Interface for accessing Application Auto Scaling.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.applicationautoscaling.AbstractAWSApplicationAutoScaling} instead.
*
*
*
* With Application Auto Scaling, you can configure automatic scaling for your scalable resources. You can use
* Application Auto Scaling to accomplish the following tasks:
*
*
* -
*
* Define scaling policies to automatically scale your AWS or custom resources
*
*
* -
*
* Scale your resources in response to CloudWatch alarms
*
*
* -
*
* Schedule one-time or recurring scaling actions
*
*
* -
*
* View the history of your scaling events
*
*
*
*
* Application Auto Scaling can scale the following resources:
*
*
* -
*
* Amazon ECS services. For more information, see Service Auto Scaling
* in the Amazon Elastic Container Service Developer Guide.
*
*
* -
*
* Amazon EC2 Spot fleets. For more information, see Automatic Scaling for Spot
* Fleet in the Amazon EC2 User Guide.
*
*
* -
*
* Amazon EMR clusters. For more information, see Using Automatic
* Scaling in Amazon EMR in the Amazon EMR Management Guide.
*
*
* -
*
* AppStream 2.0 fleets. For more information, see Fleet Auto Scaling for Amazon
* AppStream 2.0 in the Amazon AppStream 2.0 Developer Guide.
*
*
* -
*
* Provisioned read and write capacity for Amazon DynamoDB tables and global secondary indexes. For more information,
* see Managing Throughput
* Capacity Automatically with DynamoDB Auto Scaling in the Amazon DynamoDB Developer Guide.
*
*
* -
*
* Amazon Aurora Replicas. For more information, see Using Amazon
* Aurora Auto Scaling with Aurora Replicas.
*
*
* -
*
* Amazon SageMaker endpoint variants. For more information, see Automatically Scaling Amazon
* SageMaker Models.
*
*
* -
*
* Custom resources provided by your own applications or services. More information is available in our GitHub repository.
*
*
*
*
* To learn more about Application Auto Scaling, including information about granting IAM users required permissions for
* Application Auto Scaling actions, see the Application Auto Scaling User Guide.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSApplicationAutoScaling {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "autoscaling";
/**
* Overrides the default endpoint for this client ("https://autoscaling.us-east-1.amazonaws.com"). Callers can use
* this method to control which AWS region they want to work with.
*
* Callers can pass in just the endpoint (ex: "autoscaling.us-east-1.amazonaws.com") or a full URL, including the
* protocol (ex: "https://autoscaling.us-east-1.amazonaws.com"). If the protocol is not specified here, the default
* protocol from this client's {@link ClientConfiguration} will be used, which by default is HTTPS.
*
* For more information on using AWS regions with the AWS SDK for Java, and a complete list of all available
* endpoints for all AWS services, see: https://docs.aws.amazon.com/sdk-for-java/v1/developer-guide/java-dg-region-selection.html#region-selection-
* choose-endpoint
*
* This method is not threadsafe. An endpoint should be configured when the client is created and before any
* service requests are made. Changing it afterwards creates inevitable race conditions for any service requests in
* transit or retrying.
*
* @param endpoint
* The endpoint (ex: "autoscaling.us-east-1.amazonaws.com") or a full URL, including the protocol (ex:
* "https://autoscaling.us-east-1.amazonaws.com") of the region specific AWS endpoint this client will
* communicate with.
* @deprecated use {@link AwsClientBuilder#setEndpointConfiguration(AwsClientBuilder.EndpointConfiguration)} for
* example:
* {@code builder.setEndpointConfiguration(new EndpointConfiguration(endpoint, signingRegion));}
*/
@Deprecated
void setEndpoint(String endpoint);
/**
* An alternative to {@link AWSApplicationAutoScaling#setEndpoint(String)}, sets the regional endpoint for this
* client's service calls. Callers can use this method to control which AWS region they want to work with.
*
* By default, all service endpoints in all regions use the https protocol. To use http instead, specify it in the
* {@link ClientConfiguration} supplied at construction.
*
* This method is not threadsafe. A region should be configured when the client is created and before any service
* requests are made. Changing it afterwards creates inevitable race conditions for any service requests in transit
* or retrying.
*
* @param region
* The region this client will communicate with. See {@link Region#getRegion(com.amazonaws.regions.Regions)}
* for accessing a given region. Must not be null and must be a region where the service is available.
*
* @see Region#getRegion(com.amazonaws.regions.Regions)
* @see Region#createClient(Class, com.amazonaws.auth.AWSCredentialsProvider, ClientConfiguration)
* @see Region#isServiceSupported(String)
* @deprecated use {@link AwsClientBuilder#setRegion(String)}
*/
@Deprecated
void setRegion(Region region);
/**
*
* Deletes the specified Application Auto Scaling scaling policy.
*
*
* Deleting a policy deletes the underlying alarm action, but does not delete the CloudWatch alarm associated with
* the scaling policy, even if it no longer has an associated action.
*
*
* To create a scaling policy or update an existing one, see PutScalingPolicy.
*
*
* @param deleteScalingPolicyRequest
* @return Result of the DeleteScalingPolicy operation returned by the service.
* @throws ValidationException
* An exception was thrown for a validation issue. Review the available parameters for the API request.
* @throws ObjectNotFoundException
* The specified object could not be found. For any operation that depends on the existence of a scalable
* target, this exception is thrown if the scalable target with the specified service namespace, resource
* ID, and scalable dimension does not exist. For any operation that deletes or deregisters a resource, this
* exception is thrown if the resource cannot be found.
* @throws ConcurrentUpdateException
* Concurrent updates caused an exception, for example, if you request an update to an Application Auto
* Scaling resource that already has a pending update.
* @throws InternalServiceException
* The service encountered an internal error.
* @sample AWSApplicationAutoScaling.DeleteScalingPolicy
* @see AWS API Documentation
*/
DeleteScalingPolicyResult deleteScalingPolicy(DeleteScalingPolicyRequest deleteScalingPolicyRequest);
/**
*
* Deletes the specified Application Auto Scaling scheduled action.
*
*
* @param deleteScheduledActionRequest
* @return Result of the DeleteScheduledAction operation returned by the service.
* @throws ValidationException
* An exception was thrown for a validation issue. Review the available parameters for the API request.
* @throws ObjectNotFoundException
* The specified object could not be found. For any operation that depends on the existence of a scalable
* target, this exception is thrown if the scalable target with the specified service namespace, resource
* ID, and scalable dimension does not exist. For any operation that deletes or deregisters a resource, this
* exception is thrown if the resource cannot be found.
* @throws ConcurrentUpdateException
* Concurrent updates caused an exception, for example, if you request an update to an Application Auto
* Scaling resource that already has a pending update.
* @throws InternalServiceException
* The service encountered an internal error.
* @sample AWSApplicationAutoScaling.DeleteScheduledAction
* @see AWS API Documentation
*/
DeleteScheduledActionResult deleteScheduledAction(DeleteScheduledActionRequest deleteScheduledActionRequest);
/**
*
* Deregisters a scalable target.
*
*
* Deregistering a scalable target deletes the scaling policies that are associated with it.
*
*
* To create a scalable target or update an existing one, see RegisterScalableTarget.
*
*
* @param deregisterScalableTargetRequest
* @return Result of the DeregisterScalableTarget operation returned by the service.
* @throws ValidationException
* An exception was thrown for a validation issue. Review the available parameters for the API request.
* @throws ObjectNotFoundException
* The specified object could not be found. For any operation that depends on the existence of a scalable
* target, this exception is thrown if the scalable target with the specified service namespace, resource
* ID, and scalable dimension does not exist. For any operation that deletes or deregisters a resource, this
* exception is thrown if the resource cannot be found.
* @throws ConcurrentUpdateException
* Concurrent updates caused an exception, for example, if you request an update to an Application Auto
* Scaling resource that already has a pending update.
* @throws InternalServiceException
* The service encountered an internal error.
* @sample AWSApplicationAutoScaling.DeregisterScalableTarget
* @see AWS API Documentation
*/
DeregisterScalableTargetResult deregisterScalableTarget(DeregisterScalableTargetRequest deregisterScalableTargetRequest);
/**
*
* Gets information about the scalable targets in the specified namespace.
*
*
* You can filter the results using the ResourceIds
and ScalableDimension
parameters.
*
*
* To create a scalable target or update an existing one, see RegisterScalableTarget. If you are no longer
* using a scalable target, you can deregister it using DeregisterScalableTarget.
*
*
* @param describeScalableTargetsRequest
* @return Result of the DescribeScalableTargets operation returned by the service.
* @throws ValidationException
* An exception was thrown for a validation issue. Review the available parameters for the API request.
* @throws InvalidNextTokenException
* The next token supplied was invalid.
* @throws ConcurrentUpdateException
* Concurrent updates caused an exception, for example, if you request an update to an Application Auto
* Scaling resource that already has a pending update.
* @throws InternalServiceException
* The service encountered an internal error.
* @sample AWSApplicationAutoScaling.DescribeScalableTargets
* @see AWS API Documentation
*/
DescribeScalableTargetsResult describeScalableTargets(DescribeScalableTargetsRequest describeScalableTargetsRequest);
/**
*
* Provides descriptive information about the scaling activities in the specified namespace from the previous six
* weeks.
*
*
* You can filter the results using the ResourceId
and ScalableDimension
parameters.
*
*
* Scaling activities are triggered by CloudWatch alarms that are associated with scaling policies. To view the
* scaling policies for a service namespace, see DescribeScalingPolicies. To create a scaling policy or
* update an existing one, see PutScalingPolicy.
*
*
* @param describeScalingActivitiesRequest
* @return Result of the DescribeScalingActivities operation returned by the service.
* @throws ValidationException
* An exception was thrown for a validation issue. Review the available parameters for the API request.
* @throws InvalidNextTokenException
* The next token supplied was invalid.
* @throws ConcurrentUpdateException
* Concurrent updates caused an exception, for example, if you request an update to an Application Auto
* Scaling resource that already has a pending update.
* @throws InternalServiceException
* The service encountered an internal error.
* @sample AWSApplicationAutoScaling.DescribeScalingActivities
* @see AWS API Documentation
*/
DescribeScalingActivitiesResult describeScalingActivities(DescribeScalingActivitiesRequest describeScalingActivitiesRequest);
/**
*
* Describes the scaling policies for the specified service namespace.
*
*
* You can filter the results using the ResourceId
, ScalableDimension
, and
* PolicyNames
parameters.
*
*
* To create a scaling policy or update an existing one, see PutScalingPolicy. If you are no longer using a
* scaling policy, you can delete it using DeleteScalingPolicy.
*
*
* @param describeScalingPoliciesRequest
* @return Result of the DescribeScalingPolicies operation returned by the service.
* @throws ValidationException
* An exception was thrown for a validation issue. Review the available parameters for the API request.
* @throws FailedResourceAccessException
* Failed access to resources caused an exception. This exception is thrown when Application Auto Scaling is
* unable to retrieve the alarms associated with a scaling policy due to a client error, for example, if the
* role ARN specified for a scalable target does not have permission to call the CloudWatch DescribeAlarms on your behalf.
* @throws InvalidNextTokenException
* The next token supplied was invalid.
* @throws ConcurrentUpdateException
* Concurrent updates caused an exception, for example, if you request an update to an Application Auto
* Scaling resource that already has a pending update.
* @throws InternalServiceException
* The service encountered an internal error.
* @sample AWSApplicationAutoScaling.DescribeScalingPolicies
* @see AWS API Documentation
*/
DescribeScalingPoliciesResult describeScalingPolicies(DescribeScalingPoliciesRequest describeScalingPoliciesRequest);
/**
*
* Describes the scheduled actions for the specified service namespace.
*
*
* You can filter the results using the ResourceId
, ScalableDimension
, and
* ScheduledActionNames
parameters.
*
*
* To create a scheduled action or update an existing one, see PutScheduledAction. If you are no longer using
* a scheduled action, you can delete it using DeleteScheduledAction.
*
*
* @param describeScheduledActionsRequest
* @return Result of the DescribeScheduledActions operation returned by the service.
* @throws ValidationException
* An exception was thrown for a validation issue. Review the available parameters for the API request.
* @throws InvalidNextTokenException
* The next token supplied was invalid.
* @throws ConcurrentUpdateException
* Concurrent updates caused an exception, for example, if you request an update to an Application Auto
* Scaling resource that already has a pending update.
* @throws InternalServiceException
* The service encountered an internal error.
* @sample AWSApplicationAutoScaling.DescribeScheduledActions
* @see AWS API Documentation
*/
DescribeScheduledActionsResult describeScheduledActions(DescribeScheduledActionsRequest describeScheduledActionsRequest);
/**
*
* Creates or updates a policy for an Application Auto Scaling scalable target.
*
*
* Each scalable target is identified by a service namespace, resource ID, and scalable dimension. A scaling policy
* applies to the scalable target identified by those three attributes. You cannot create a scaling policy until you
* have registered the resource as a scalable target using RegisterScalableTarget.
*
*
* To update a policy, specify its policy name and the parameters that you want to change. Any parameters that you
* don't specify are not changed by this update request.
*
*
* You can view the scaling policies for a service namespace using DescribeScalingPolicies. If you are no
* longer using a scaling policy, you can delete it using DeleteScalingPolicy.
*
*
* Multiple scaling policies can be in force at the same time for the same scalable target. You can have one or more
* target tracking scaling policies, one or more step scaling policies, or both. However, there is a chance that
* multiple policies could conflict, instructing the scalable target to scale out or in at the same time.
* Application Auto Scaling gives precedence to the policy that provides the largest capacity for both scale in and
* scale out. For example, if one policy increases capacity by 3, another policy increases capacity by 200 percent,
* and the current capacity is 10, Application Auto Scaling uses the policy with the highest calculated capacity
* (200% of 10 = 20) and scales out to 30.
*
*
* Learn more about how to work with scaling policies in the Application Auto Scaling User Guide.
*
*
* @param putScalingPolicyRequest
* @return Result of the PutScalingPolicy operation returned by the service.
* @throws ValidationException
* An exception was thrown for a validation issue. Review the available parameters for the API request.
* @throws LimitExceededException
* A per-account resource limit is exceeded. For more information, see Application Auto Scaling Limits.
* @throws ObjectNotFoundException
* The specified object could not be found. For any operation that depends on the existence of a scalable
* target, this exception is thrown if the scalable target with the specified service namespace, resource
* ID, and scalable dimension does not exist. For any operation that deletes or deregisters a resource, this
* exception is thrown if the resource cannot be found.
* @throws ConcurrentUpdateException
* Concurrent updates caused an exception, for example, if you request an update to an Application Auto
* Scaling resource that already has a pending update.
* @throws FailedResourceAccessException
* Failed access to resources caused an exception. This exception is thrown when Application Auto Scaling is
* unable to retrieve the alarms associated with a scaling policy due to a client error, for example, if the
* role ARN specified for a scalable target does not have permission to call the CloudWatch DescribeAlarms on your behalf.
* @throws InternalServiceException
* The service encountered an internal error.
* @sample AWSApplicationAutoScaling.PutScalingPolicy
* @see AWS API Documentation
*/
PutScalingPolicyResult putScalingPolicy(PutScalingPolicyRequest putScalingPolicyRequest);
/**
*
* Creates or updates a scheduled action for an Application Auto Scaling scalable target.
*
*
* Each scalable target is identified by a service namespace, resource ID, and scalable dimension. A scheduled
* action applies to the scalable target identified by those three attributes. You cannot create a scheduled action
* until you have registered the resource as a scalable target using RegisterScalableTarget.
*
*
* To update an action, specify its name and the parameters that you want to change. If you don't specify start and
* end times, the old values are deleted. Any other parameters that you don't specify are not changed by this update
* request.
*
*
* You can view the scheduled actions using DescribeScheduledActions. If you are no longer using a scheduled
* action, you can delete it using DeleteScheduledAction.
*
*
* Learn more about how to work with scheduled actions in the Application Auto Scaling User Guide.
*
*
* @param putScheduledActionRequest
* @return Result of the PutScheduledAction operation returned by the service.
* @throws ValidationException
* An exception was thrown for a validation issue. Review the available parameters for the API request.
* @throws LimitExceededException
* A per-account resource limit is exceeded. For more information, see Application Auto Scaling Limits.
* @throws ObjectNotFoundException
* The specified object could not be found. For any operation that depends on the existence of a scalable
* target, this exception is thrown if the scalable target with the specified service namespace, resource
* ID, and scalable dimension does not exist. For any operation that deletes or deregisters a resource, this
* exception is thrown if the resource cannot be found.
* @throws ConcurrentUpdateException
* Concurrent updates caused an exception, for example, if you request an update to an Application Auto
* Scaling resource that already has a pending update.
* @throws InternalServiceException
* The service encountered an internal error.
* @sample AWSApplicationAutoScaling.PutScheduledAction
* @see AWS API Documentation
*/
PutScheduledActionResult putScheduledAction(PutScheduledActionRequest putScheduledActionRequest);
/**
*
* Registers or updates a scalable target. A scalable target is a resource that Application Auto Scaling can scale
* out and scale in. Each scalable target has a resource ID, scalable dimension, and namespace, as well as values
* for minimum and maximum capacity.
*
*
* After you register a scalable target, you do not need to register it again to use other Application Auto Scaling
* operations. To see which resources have been registered, use DescribeScalableTargets. You can also view
* the scaling policies for a service namespace using DescribeScalableTargets.
*
*
* If you no longer need a scalable target, you can deregister it using DeregisterScalableTarget.
*
*
* @param registerScalableTargetRequest
* @return Result of the RegisterScalableTarget operation returned by the service.
* @throws ValidationException
* An exception was thrown for a validation issue. Review the available parameters for the API request.
* @throws LimitExceededException
* A per-account resource limit is exceeded. For more information, see Application Auto Scaling Limits.
* @throws ConcurrentUpdateException
* Concurrent updates caused an exception, for example, if you request an update to an Application Auto
* Scaling resource that already has a pending update.
* @throws InternalServiceException
* The service encountered an internal error.
* @sample AWSApplicationAutoScaling.RegisterScalableTarget
* @see AWS API Documentation
*/
RegisterScalableTargetResult registerScalableTarget(RegisterScalableTargetRequest registerScalableTargetRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}