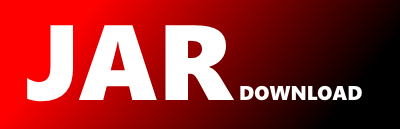
com.amazonaws.services.applicationautoscaling.AWSApplicationAutoScalingAsyncClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-applicationautoscaling Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.applicationautoscaling;
import static java.util.concurrent.Executors.newFixedThreadPool;
import javax.annotation.Generated;
import com.amazonaws.services.applicationautoscaling.model.*;
import com.amazonaws.client.AwsAsyncClientParams;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.ClientConfiguration;
import com.amazonaws.auth.AWSCredentials;
import com.amazonaws.auth.AWSCredentialsProvider;
import java.util.concurrent.ExecutorService;
import com.amazonaws.auth.DefaultAWSCredentialsProviderChain;
/**
* Client for accessing Application Auto Scaling asynchronously. Each asynchronous method will return a Java Future
* object representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
*
* With Application Auto Scaling, you can configure automatic scaling for your scalable resources. You can use
* Application Auto Scaling to accomplish the following tasks:
*
*
* -
*
* Define scaling policies to automatically scale your AWS or custom resources
*
*
* -
*
* Scale your resources in response to CloudWatch alarms
*
*
* -
*
* Schedule one-time or recurring scaling actions
*
*
* -
*
* View the history of your scaling events
*
*
*
*
* Application Auto Scaling can scale the following resources:
*
*
* -
*
* Amazon ECS services. For more information, see Service Auto Scaling
* in the Amazon Elastic Container Service Developer Guide.
*
*
* -
*
* Amazon EC2 Spot fleets. For more information, see Automatic Scaling for Spot
* Fleet in the Amazon EC2 User Guide.
*
*
* -
*
* Amazon EMR clusters. For more information, see Using Automatic
* Scaling in Amazon EMR in the Amazon EMR Management Guide.
*
*
* -
*
* AppStream 2.0 fleets. For more information, see Fleet Auto Scaling for Amazon
* AppStream 2.0 in the Amazon AppStream 2.0 Developer Guide.
*
*
* -
*
* Provisioned read and write capacity for Amazon DynamoDB tables and global secondary indexes. For more information,
* see Managing Throughput
* Capacity Automatically with DynamoDB Auto Scaling in the Amazon DynamoDB Developer Guide.
*
*
* -
*
* Amazon Aurora Replicas. For more information, see Using Amazon
* Aurora Auto Scaling with Aurora Replicas.
*
*
* -
*
* Amazon SageMaker endpoint variants. For more information, see Automatically Scaling Amazon
* SageMaker Models.
*
*
* -
*
* Custom resources provided by your own applications or services. More information is available in our GitHub repository.
*
*
*
*
* To learn more about Application Auto Scaling, including information about granting IAM users required permissions for
* Application Auto Scaling actions, see the Application Auto Scaling User Guide.
*
*/
@ThreadSafe
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AWSApplicationAutoScalingAsyncClient extends AWSApplicationAutoScalingClient implements AWSApplicationAutoScalingAsync {
private static final int DEFAULT_THREAD_POOL_SIZE = 50;
private final java.util.concurrent.ExecutorService executorService;
/**
* Constructs a new asynchronous client to invoke service methods on Application Auto Scaling. A credentials
* provider chain will be used that searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Credential profiles file at the default location (~/.aws/credentials) shared by all AWS SDKs and the AWS CLI
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
* Asynchronous methods are delegated to a fixed-size thread pool containing 50 threads (to match the default
* maximum number of concurrent connections to the service).
*
* @see com.amazonaws.auth.DefaultAWSCredentialsProviderChain
* @see java.util.concurrent.Executors#newFixedThreadPool(int)
* @deprecated use {@link AWSApplicationAutoScalingAsyncClientBuilder#defaultClient()}
*/
@Deprecated
public AWSApplicationAutoScalingAsyncClient() {
this(DefaultAWSCredentialsProviderChain.getInstance());
}
/**
* Constructs a new asynchronous client to invoke service methods on Application Auto Scaling. A credentials
* provider chain will be used that searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Credential profiles file at the default location (~/.aws/credentials) shared by all AWS SDKs and the AWS CLI
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
* Asynchronous methods are delegated to a fixed-size thread pool containing a number of threads equal to the
* maximum number of concurrent connections configured via {@code ClientConfiguration.getMaxConnections()}.
*
* @param clientConfiguration
* The client configuration options controlling how this client connects to Application Auto Scaling (ex:
* proxy settings, retry counts, etc).
*
* @see com.amazonaws.auth.DefaultAWSCredentialsProviderChain
* @see java.util.concurrent.Executors#newFixedThreadPool(int)
* @deprecated use {@link AWSApplicationAutoScalingAsyncClientBuilder#withClientConfiguration(ClientConfiguration)}
*/
@Deprecated
public AWSApplicationAutoScalingAsyncClient(ClientConfiguration clientConfiguration) {
this(DefaultAWSCredentialsProviderChain.getInstance(), clientConfiguration, newFixedThreadPool(clientConfiguration.getMaxConnections()));
}
/**
* Constructs a new asynchronous client to invoke service methods on Application Auto Scaling using the specified
* AWS account credentials.
*
* Asynchronous methods are delegated to a fixed-size thread pool containing 50 threads (to match the default
* maximum number of concurrent connections to the service).
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when authenticating with AWS services.
* @see java.util.concurrent.Executors#newFixedThreadPool(int)
* @deprecated use {@link AWSApplicationAutoScalingAsyncClientBuilder#withCredentials(AWSCredentialsProvider)}
*/
@Deprecated
public AWSApplicationAutoScalingAsyncClient(AWSCredentials awsCredentials) {
this(awsCredentials, newFixedThreadPool(DEFAULT_THREAD_POOL_SIZE));
}
/**
* Constructs a new asynchronous client to invoke service methods on Application Auto Scaling using the specified
* AWS account credentials and executor service. Default client settings will be used.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when authenticating with AWS services.
* @param executorService
* The executor service by which all asynchronous requests will be executed.
* @deprecated use {@link AWSApplicationAutoScalingAsyncClientBuilder#withCredentials(AWSCredentialsProvider)} and
* {@link AWSApplicationAutoScalingAsyncClientBuilder#withExecutorFactory(com.amazonaws.client.builder.ExecutorFactory)}
*/
@Deprecated
public AWSApplicationAutoScalingAsyncClient(AWSCredentials awsCredentials, ExecutorService executorService) {
this(awsCredentials, configFactory.getConfig(), executorService);
}
/**
* Constructs a new asynchronous client to invoke service methods on Application Auto Scaling using the specified
* AWS account credentials, executor service, and client configuration options.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when authenticating with AWS services.
* @param clientConfiguration
* Client configuration options (ex: max retry limit, proxy settings, etc).
* @param executorService
* The executor service by which all asynchronous requests will be executed.
* @deprecated use {@link AWSApplicationAutoScalingAsyncClientBuilder#withCredentials(AWSCredentialsProvider)} and
* {@link AWSApplicationAutoScalingAsyncClientBuilder#withClientConfiguration(ClientConfiguration)} and
* {@link AWSApplicationAutoScalingAsyncClientBuilder#withExecutorFactory(com.amazonaws.client.builder.ExecutorFactory)}
*/
@Deprecated
public AWSApplicationAutoScalingAsyncClient(AWSCredentials awsCredentials, ClientConfiguration clientConfiguration, ExecutorService executorService) {
super(awsCredentials, clientConfiguration);
this.executorService = executorService;
}
/**
* Constructs a new asynchronous client to invoke service methods on Application Auto Scaling using the specified
* AWS account credentials provider. Default client settings will be used.
*
* Asynchronous methods are delegated to a fixed-size thread pool containing 50 threads (to match the default
* maximum number of concurrent connections to the service).
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to authenticate requests with AWS services.
* @see java.util.concurrent.Executors#newFixedThreadPool(int)
* @deprecated use {@link AWSApplicationAutoScalingAsyncClientBuilder#withCredentials(AWSCredentialsProvider)}
*/
@Deprecated
public AWSApplicationAutoScalingAsyncClient(AWSCredentialsProvider awsCredentialsProvider) {
this(awsCredentialsProvider, newFixedThreadPool(DEFAULT_THREAD_POOL_SIZE));
}
/**
* Constructs a new asynchronous client to invoke service methods on Application Auto Scaling using the provided AWS
* account credentials provider and client configuration options.
*
* Asynchronous methods are delegated to a fixed-size thread pool containing a number of threads equal to the
* maximum number of concurrent connections configured via {@code ClientConfiguration.getMaxConnections()}.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to authenticate requests with AWS services.
* @param clientConfiguration
* Client configuration options (ex: max retry limit, proxy settings, etc).
*
* @see com.amazonaws.auth.DefaultAWSCredentialsProviderChain
* @see java.util.concurrent.Executors#newFixedThreadPool(int)
* @deprecated use {@link AWSApplicationAutoScalingAsyncClientBuilder#withCredentials(AWSCredentialsProvider)} and
* {@link AWSApplicationAutoScalingAsyncClientBuilder#withClientConfiguration(ClientConfiguration)}
*/
@Deprecated
public AWSApplicationAutoScalingAsyncClient(AWSCredentialsProvider awsCredentialsProvider, ClientConfiguration clientConfiguration) {
this(awsCredentialsProvider, clientConfiguration, newFixedThreadPool(clientConfiguration.getMaxConnections()));
}
/**
* Constructs a new asynchronous client to invoke service methods on Application Auto Scaling using the specified
* AWS account credentials provider and executor service. Default client settings will be used.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to authenticate requests with AWS services.
* @param executorService
* The executor service by which all asynchronous requests will be executed.
* @deprecated use {@link AWSApplicationAutoScalingAsyncClientBuilder#withCredentials(AWSCredentialsProvider)} and
* {@link AWSApplicationAutoScalingAsyncClientBuilder#withExecutorFactory(com.amazonaws.client.builder.ExecutorFactory)}
*/
@Deprecated
public AWSApplicationAutoScalingAsyncClient(AWSCredentialsProvider awsCredentialsProvider, ExecutorService executorService) {
this(awsCredentialsProvider, configFactory.getConfig(), executorService);
}
/**
* Constructs a new asynchronous client to invoke service methods on Application Auto Scaling using the specified
* AWS account credentials provider, executor service, and client configuration options.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to authenticate requests with AWS services.
* @param clientConfiguration
* Client configuration options (ex: max retry limit, proxy settings, etc).
* @param executorService
* The executor service by which all asynchronous requests will be executed.
* @deprecated use {@link AWSApplicationAutoScalingAsyncClientBuilder#withCredentials(AWSCredentialsProvider)} and
* {@link AWSApplicationAutoScalingAsyncClientBuilder#withClientConfiguration(ClientConfiguration)} and
* {@link AWSApplicationAutoScalingAsyncClientBuilder#withExecutorFactory(com.amazonaws.client.builder.ExecutorFactory)}
*/
@Deprecated
public AWSApplicationAutoScalingAsyncClient(AWSCredentialsProvider awsCredentialsProvider, ClientConfiguration clientConfiguration,
ExecutorService executorService) {
super(awsCredentialsProvider, clientConfiguration);
this.executorService = executorService;
}
public static AWSApplicationAutoScalingAsyncClientBuilder asyncBuilder() {
return AWSApplicationAutoScalingAsyncClientBuilder.standard();
}
/**
* Constructs a new asynchronous client to invoke service methods on Application Auto Scaling using the specified
* parameters.
*
* @param asyncClientParams
* Object providing client parameters.
*/
AWSApplicationAutoScalingAsyncClient(AwsAsyncClientParams asyncClientParams) {
super(asyncClientParams);
this.executorService = asyncClientParams.getExecutor();
}
/**
* Returns the executor service used by this client to execute async requests.
*
* @return The executor service used by this client to execute async requests.
*/
public ExecutorService getExecutorService() {
return executorService;
}
@Override
public java.util.concurrent.Future deleteScalingPolicyAsync(DeleteScalingPolicyRequest request) {
return deleteScalingPolicyAsync(request, null);
}
@Override
public java.util.concurrent.Future deleteScalingPolicyAsync(final DeleteScalingPolicyRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
final DeleteScalingPolicyRequest finalRequest = beforeClientExecution(request);
return executorService.submit(new java.util.concurrent.Callable() {
@Override
public DeleteScalingPolicyResult call() throws Exception {
DeleteScalingPolicyResult result = null;
try {
result = executeDeleteScalingPolicy(finalRequest);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(finalRequest, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future deleteScheduledActionAsync(DeleteScheduledActionRequest request) {
return deleteScheduledActionAsync(request, null);
}
@Override
public java.util.concurrent.Future deleteScheduledActionAsync(final DeleteScheduledActionRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
final DeleteScheduledActionRequest finalRequest = beforeClientExecution(request);
return executorService.submit(new java.util.concurrent.Callable() {
@Override
public DeleteScheduledActionResult call() throws Exception {
DeleteScheduledActionResult result = null;
try {
result = executeDeleteScheduledAction(finalRequest);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(finalRequest, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future deregisterScalableTargetAsync(DeregisterScalableTargetRequest request) {
return deregisterScalableTargetAsync(request, null);
}
@Override
public java.util.concurrent.Future deregisterScalableTargetAsync(final DeregisterScalableTargetRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
final DeregisterScalableTargetRequest finalRequest = beforeClientExecution(request);
return executorService.submit(new java.util.concurrent.Callable() {
@Override
public DeregisterScalableTargetResult call() throws Exception {
DeregisterScalableTargetResult result = null;
try {
result = executeDeregisterScalableTarget(finalRequest);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(finalRequest, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future describeScalableTargetsAsync(DescribeScalableTargetsRequest request) {
return describeScalableTargetsAsync(request, null);
}
@Override
public java.util.concurrent.Future describeScalableTargetsAsync(final DescribeScalableTargetsRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
final DescribeScalableTargetsRequest finalRequest = beforeClientExecution(request);
return executorService.submit(new java.util.concurrent.Callable() {
@Override
public DescribeScalableTargetsResult call() throws Exception {
DescribeScalableTargetsResult result = null;
try {
result = executeDescribeScalableTargets(finalRequest);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(finalRequest, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future describeScalingActivitiesAsync(DescribeScalingActivitiesRequest request) {
return describeScalingActivitiesAsync(request, null);
}
@Override
public java.util.concurrent.Future describeScalingActivitiesAsync(final DescribeScalingActivitiesRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
final DescribeScalingActivitiesRequest finalRequest = beforeClientExecution(request);
return executorService.submit(new java.util.concurrent.Callable() {
@Override
public DescribeScalingActivitiesResult call() throws Exception {
DescribeScalingActivitiesResult result = null;
try {
result = executeDescribeScalingActivities(finalRequest);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(finalRequest, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future describeScalingPoliciesAsync(DescribeScalingPoliciesRequest request) {
return describeScalingPoliciesAsync(request, null);
}
@Override
public java.util.concurrent.Future describeScalingPoliciesAsync(final DescribeScalingPoliciesRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
final DescribeScalingPoliciesRequest finalRequest = beforeClientExecution(request);
return executorService.submit(new java.util.concurrent.Callable() {
@Override
public DescribeScalingPoliciesResult call() throws Exception {
DescribeScalingPoliciesResult result = null;
try {
result = executeDescribeScalingPolicies(finalRequest);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(finalRequest, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future describeScheduledActionsAsync(DescribeScheduledActionsRequest request) {
return describeScheduledActionsAsync(request, null);
}
@Override
public java.util.concurrent.Future describeScheduledActionsAsync(final DescribeScheduledActionsRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
final DescribeScheduledActionsRequest finalRequest = beforeClientExecution(request);
return executorService.submit(new java.util.concurrent.Callable() {
@Override
public DescribeScheduledActionsResult call() throws Exception {
DescribeScheduledActionsResult result = null;
try {
result = executeDescribeScheduledActions(finalRequest);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(finalRequest, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future putScalingPolicyAsync(PutScalingPolicyRequest request) {
return putScalingPolicyAsync(request, null);
}
@Override
public java.util.concurrent.Future putScalingPolicyAsync(final PutScalingPolicyRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
final PutScalingPolicyRequest finalRequest = beforeClientExecution(request);
return executorService.submit(new java.util.concurrent.Callable() {
@Override
public PutScalingPolicyResult call() throws Exception {
PutScalingPolicyResult result = null;
try {
result = executePutScalingPolicy(finalRequest);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(finalRequest, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future putScheduledActionAsync(PutScheduledActionRequest request) {
return putScheduledActionAsync(request, null);
}
@Override
public java.util.concurrent.Future putScheduledActionAsync(final PutScheduledActionRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
final PutScheduledActionRequest finalRequest = beforeClientExecution(request);
return executorService.submit(new java.util.concurrent.Callable() {
@Override
public PutScheduledActionResult call() throws Exception {
PutScheduledActionResult result = null;
try {
result = executePutScheduledAction(finalRequest);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(finalRequest, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future registerScalableTargetAsync(RegisterScalableTargetRequest request) {
return registerScalableTargetAsync(request, null);
}
@Override
public java.util.concurrent.Future registerScalableTargetAsync(final RegisterScalableTargetRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
final RegisterScalableTargetRequest finalRequest = beforeClientExecution(request);
return executorService.submit(new java.util.concurrent.Callable() {
@Override
public RegisterScalableTargetResult call() throws Exception {
RegisterScalableTargetResult result = null;
try {
result = executeRegisterScalableTarget(finalRequest);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(finalRequest, result);
}
return result;
}
});
}
/**
* Shuts down the client, releasing all managed resources. This includes forcibly terminating all pending
* asynchronous service calls. Clients who wish to give pending asynchronous service calls time to complete should
* call {@code getExecutorService().shutdown()} followed by {@code getExecutorService().awaitTermination()} prior to
* calling this method.
*/
@Override
public void shutdown() {
super.shutdown();
executorService.shutdownNow();
}
}