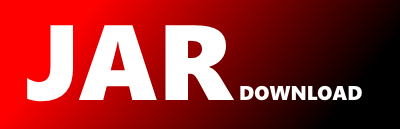
com.amazonaws.services.applicationautoscaling.model.ScalableTarget Maven / Gradle / Ivy
Show all versions of aws-java-sdk-applicationautoscaling Show documentation
/*
* Copyright 2011-2016 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.applicationautoscaling.model;
import java.io.Serializable;
/**
*
* Represents a scalable target.
*
*/
public class ScalableTarget implements Serializable, Cloneable {
/**
*
* The namespace of the AWS service. For more information, see AWS Service Namespaces in the Amazon Web Services General Reference.
*
*/
private String serviceNamespace;
/**
*
* The identifier of the resource associated with the scalable target. This string consists of the resource type and
* unique identifier.
*
*
* -
*
* ECS service - The resource type is service
and the unique identifier is the cluster name and service
* name. Example: service/default/sample-webapp
.
*
*
* -
*
* Spot fleet request - The resource type is spot-fleet-request
and the unique identifier is the Spot
* fleet request ID. Example: spot-fleet-request/sfr-73fbd2ce-aa30-494c-8788-1cee4EXAMPLE
.
*
*
* -
*
* EMR cluster - The resource type is instancegroup
and the unique identifier is the cluster ID and
* instance group ID. Example: instancegroup/j-2EEZNYKUA1NTV/ig-1791Y4E1L8YI0
.
*
*
*
*/
private String resourceId;
/**
*
* The scalable dimension associated with the scalable target. This string consists of the service namespace,
* resource type, and scaling property.
*
*
* -
*
* ecs:service:DesiredCount
- The desired task count of an ECS service.
*
*
* -
*
* ec2:spot-fleet-request:TargetCapacity
- The target capacity of a Spot fleet request.
*
*
* -
*
* elasticmapreduce:instancegroup:InstanceCount
- The instance count of an EMR Instance Group.
*
*
*
*/
private String scalableDimension;
/**
*
* The minimum value to scale to in response to a scale in event.
*
*/
private Integer minCapacity;
/**
*
* The maximum value to scale to in response to a scale out event.
*
*/
private Integer maxCapacity;
/**
*
* The ARN of an IAM role that allows Application Auto Scaling to modify the scalable target on your behalf.
*
*/
private String roleARN;
/**
*
* The Unix timestamp for when the scalable target was created.
*
*/
private java.util.Date creationTime;
/**
*
* The namespace of the AWS service. For more information, see AWS Service Namespaces in the Amazon Web Services General Reference.
*
*
* @param serviceNamespace
* The namespace of the AWS service. For more information, see AWS Service Namespaces in the Amazon Web Services General Reference.
* @see ServiceNamespace
*/
public void setServiceNamespace(String serviceNamespace) {
this.serviceNamespace = serviceNamespace;
}
/**
*
* The namespace of the AWS service. For more information, see AWS Service Namespaces in the Amazon Web Services General Reference.
*
*
* @return The namespace of the AWS service. For more information, see AWS Service Namespaces in the Amazon Web Services General Reference.
* @see ServiceNamespace
*/
public String getServiceNamespace() {
return this.serviceNamespace;
}
/**
*
* The namespace of the AWS service. For more information, see AWS Service Namespaces in the Amazon Web Services General Reference.
*
*
* @param serviceNamespace
* The namespace of the AWS service. For more information, see AWS Service Namespaces in the Amazon Web Services General Reference.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ServiceNamespace
*/
public ScalableTarget withServiceNamespace(String serviceNamespace) {
setServiceNamespace(serviceNamespace);
return this;
}
/**
*
* The namespace of the AWS service. For more information, see AWS Service Namespaces in the Amazon Web Services General Reference.
*
*
* @param serviceNamespace
* The namespace of the AWS service. For more information, see AWS Service Namespaces in the Amazon Web Services General Reference.
* @see ServiceNamespace
*/
public void setServiceNamespace(ServiceNamespace serviceNamespace) {
this.serviceNamespace = serviceNamespace.toString();
}
/**
*
* The namespace of the AWS service. For more information, see AWS Service Namespaces in the Amazon Web Services General Reference.
*
*
* @param serviceNamespace
* The namespace of the AWS service. For more information, see AWS Service Namespaces in the Amazon Web Services General Reference.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ServiceNamespace
*/
public ScalableTarget withServiceNamespace(ServiceNamespace serviceNamespace) {
setServiceNamespace(serviceNamespace);
return this;
}
/**
*
* The identifier of the resource associated with the scalable target. This string consists of the resource type and
* unique identifier.
*
*
* -
*
* ECS service - The resource type is service
and the unique identifier is the cluster name and service
* name. Example: service/default/sample-webapp
.
*
*
* -
*
* Spot fleet request - The resource type is spot-fleet-request
and the unique identifier is the Spot
* fleet request ID. Example: spot-fleet-request/sfr-73fbd2ce-aa30-494c-8788-1cee4EXAMPLE
.
*
*
* -
*
* EMR cluster - The resource type is instancegroup
and the unique identifier is the cluster ID and
* instance group ID. Example: instancegroup/j-2EEZNYKUA1NTV/ig-1791Y4E1L8YI0
.
*
*
*
*
* @param resourceId
* The identifier of the resource associated with the scalable target. This string consists of the resource
* type and unique identifier.
*
* -
*
* ECS service - The resource type is service
and the unique identifier is the cluster name and
* service name. Example: service/default/sample-webapp
.
*
*
* -
*
* Spot fleet request - The resource type is spot-fleet-request
and the unique identifier is the
* Spot fleet request ID. Example: spot-fleet-request/sfr-73fbd2ce-aa30-494c-8788-1cee4EXAMPLE
.
*
*
* -
*
* EMR cluster - The resource type is instancegroup
and the unique identifier is the cluster ID
* and instance group ID. Example: instancegroup/j-2EEZNYKUA1NTV/ig-1791Y4E1L8YI0
.
*
*
*/
public void setResourceId(String resourceId) {
this.resourceId = resourceId;
}
/**
*
* The identifier of the resource associated with the scalable target. This string consists of the resource type and
* unique identifier.
*
*
* -
*
* ECS service - The resource type is service
and the unique identifier is the cluster name and service
* name. Example: service/default/sample-webapp
.
*
*
* -
*
* Spot fleet request - The resource type is spot-fleet-request
and the unique identifier is the Spot
* fleet request ID. Example: spot-fleet-request/sfr-73fbd2ce-aa30-494c-8788-1cee4EXAMPLE
.
*
*
* -
*
* EMR cluster - The resource type is instancegroup
and the unique identifier is the cluster ID and
* instance group ID. Example: instancegroup/j-2EEZNYKUA1NTV/ig-1791Y4E1L8YI0
.
*
*
*
*
* @return The identifier of the resource associated with the scalable target. This string consists of the resource
* type and unique identifier.
*
* -
*
* ECS service - The resource type is service
and the unique identifier is the cluster name and
* service name. Example: service/default/sample-webapp
.
*
*
* -
*
* Spot fleet request - The resource type is spot-fleet-request
and the unique identifier is
* the Spot fleet request ID. Example:
* spot-fleet-request/sfr-73fbd2ce-aa30-494c-8788-1cee4EXAMPLE
.
*
*
* -
*
* EMR cluster - The resource type is instancegroup
and the unique identifier is the cluster ID
* and instance group ID. Example: instancegroup/j-2EEZNYKUA1NTV/ig-1791Y4E1L8YI0
.
*
*
*/
public String getResourceId() {
return this.resourceId;
}
/**
*
* The identifier of the resource associated with the scalable target. This string consists of the resource type and
* unique identifier.
*
*
* -
*
* ECS service - The resource type is service
and the unique identifier is the cluster name and service
* name. Example: service/default/sample-webapp
.
*
*
* -
*
* Spot fleet request - The resource type is spot-fleet-request
and the unique identifier is the Spot
* fleet request ID. Example: spot-fleet-request/sfr-73fbd2ce-aa30-494c-8788-1cee4EXAMPLE
.
*
*
* -
*
* EMR cluster - The resource type is instancegroup
and the unique identifier is the cluster ID and
* instance group ID. Example: instancegroup/j-2EEZNYKUA1NTV/ig-1791Y4E1L8YI0
.
*
*
*
*
* @param resourceId
* The identifier of the resource associated with the scalable target. This string consists of the resource
* type and unique identifier.
*
* -
*
* ECS service - The resource type is service
and the unique identifier is the cluster name and
* service name. Example: service/default/sample-webapp
.
*
*
* -
*
* Spot fleet request - The resource type is spot-fleet-request
and the unique identifier is the
* Spot fleet request ID. Example: spot-fleet-request/sfr-73fbd2ce-aa30-494c-8788-1cee4EXAMPLE
.
*
*
* -
*
* EMR cluster - The resource type is instancegroup
and the unique identifier is the cluster ID
* and instance group ID. Example: instancegroup/j-2EEZNYKUA1NTV/ig-1791Y4E1L8YI0
.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ScalableTarget withResourceId(String resourceId) {
setResourceId(resourceId);
return this;
}
/**
*
* The scalable dimension associated with the scalable target. This string consists of the service namespace,
* resource type, and scaling property.
*
*
* -
*
* ecs:service:DesiredCount
- The desired task count of an ECS service.
*
*
* -
*
* ec2:spot-fleet-request:TargetCapacity
- The target capacity of a Spot fleet request.
*
*
* -
*
* elasticmapreduce:instancegroup:InstanceCount
- The instance count of an EMR Instance Group.
*
*
*
*
* @param scalableDimension
* The scalable dimension associated with the scalable target. This string consists of the service namespace,
* resource type, and scaling property.
*
* -
*
* ecs:service:DesiredCount
- The desired task count of an ECS service.
*
*
* -
*
* ec2:spot-fleet-request:TargetCapacity
- The target capacity of a Spot fleet request.
*
*
* -
*
* elasticmapreduce:instancegroup:InstanceCount
- The instance count of an EMR Instance Group.
*
*
* @see ScalableDimension
*/
public void setScalableDimension(String scalableDimension) {
this.scalableDimension = scalableDimension;
}
/**
*
* The scalable dimension associated with the scalable target. This string consists of the service namespace,
* resource type, and scaling property.
*
*
* -
*
* ecs:service:DesiredCount
- The desired task count of an ECS service.
*
*
* -
*
* ec2:spot-fleet-request:TargetCapacity
- The target capacity of a Spot fleet request.
*
*
* -
*
* elasticmapreduce:instancegroup:InstanceCount
- The instance count of an EMR Instance Group.
*
*
*
*
* @return The scalable dimension associated with the scalable target. This string consists of the service
* namespace, resource type, and scaling property.
*
* -
*
* ecs:service:DesiredCount
- The desired task count of an ECS service.
*
*
* -
*
* ec2:spot-fleet-request:TargetCapacity
- The target capacity of a Spot fleet request.
*
*
* -
*
* elasticmapreduce:instancegroup:InstanceCount
- The instance count of an EMR Instance Group.
*
*
* @see ScalableDimension
*/
public String getScalableDimension() {
return this.scalableDimension;
}
/**
*
* The scalable dimension associated with the scalable target. This string consists of the service namespace,
* resource type, and scaling property.
*
*
* -
*
* ecs:service:DesiredCount
- The desired task count of an ECS service.
*
*
* -
*
* ec2:spot-fleet-request:TargetCapacity
- The target capacity of a Spot fleet request.
*
*
* -
*
* elasticmapreduce:instancegroup:InstanceCount
- The instance count of an EMR Instance Group.
*
*
*
*
* @param scalableDimension
* The scalable dimension associated with the scalable target. This string consists of the service namespace,
* resource type, and scaling property.
*
* -
*
* ecs:service:DesiredCount
- The desired task count of an ECS service.
*
*
* -
*
* ec2:spot-fleet-request:TargetCapacity
- The target capacity of a Spot fleet request.
*
*
* -
*
* elasticmapreduce:instancegroup:InstanceCount
- The instance count of an EMR Instance Group.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ScalableDimension
*/
public ScalableTarget withScalableDimension(String scalableDimension) {
setScalableDimension(scalableDimension);
return this;
}
/**
*
* The scalable dimension associated with the scalable target. This string consists of the service namespace,
* resource type, and scaling property.
*
*
* -
*
* ecs:service:DesiredCount
- The desired task count of an ECS service.
*
*
* -
*
* ec2:spot-fleet-request:TargetCapacity
- The target capacity of a Spot fleet request.
*
*
* -
*
* elasticmapreduce:instancegroup:InstanceCount
- The instance count of an EMR Instance Group.
*
*
*
*
* @param scalableDimension
* The scalable dimension associated with the scalable target. This string consists of the service namespace,
* resource type, and scaling property.
*
* -
*
* ecs:service:DesiredCount
- The desired task count of an ECS service.
*
*
* -
*
* ec2:spot-fleet-request:TargetCapacity
- The target capacity of a Spot fleet request.
*
*
* -
*
* elasticmapreduce:instancegroup:InstanceCount
- The instance count of an EMR Instance Group.
*
*
* @see ScalableDimension
*/
public void setScalableDimension(ScalableDimension scalableDimension) {
this.scalableDimension = scalableDimension.toString();
}
/**
*
* The scalable dimension associated with the scalable target. This string consists of the service namespace,
* resource type, and scaling property.
*
*
* -
*
* ecs:service:DesiredCount
- The desired task count of an ECS service.
*
*
* -
*
* ec2:spot-fleet-request:TargetCapacity
- The target capacity of a Spot fleet request.
*
*
* -
*
* elasticmapreduce:instancegroup:InstanceCount
- The instance count of an EMR Instance Group.
*
*
*
*
* @param scalableDimension
* The scalable dimension associated with the scalable target. This string consists of the service namespace,
* resource type, and scaling property.
*
* -
*
* ecs:service:DesiredCount
- The desired task count of an ECS service.
*
*
* -
*
* ec2:spot-fleet-request:TargetCapacity
- The target capacity of a Spot fleet request.
*
*
* -
*
* elasticmapreduce:instancegroup:InstanceCount
- The instance count of an EMR Instance Group.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ScalableDimension
*/
public ScalableTarget withScalableDimension(ScalableDimension scalableDimension) {
setScalableDimension(scalableDimension);
return this;
}
/**
*
* The minimum value to scale to in response to a scale in event.
*
*
* @param minCapacity
* The minimum value to scale to in response to a scale in event.
*/
public void setMinCapacity(Integer minCapacity) {
this.minCapacity = minCapacity;
}
/**
*
* The minimum value to scale to in response to a scale in event.
*
*
* @return The minimum value to scale to in response to a scale in event.
*/
public Integer getMinCapacity() {
return this.minCapacity;
}
/**
*
* The minimum value to scale to in response to a scale in event.
*
*
* @param minCapacity
* The minimum value to scale to in response to a scale in event.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ScalableTarget withMinCapacity(Integer minCapacity) {
setMinCapacity(minCapacity);
return this;
}
/**
*
* The maximum value to scale to in response to a scale out event.
*
*
* @param maxCapacity
* The maximum value to scale to in response to a scale out event.
*/
public void setMaxCapacity(Integer maxCapacity) {
this.maxCapacity = maxCapacity;
}
/**
*
* The maximum value to scale to in response to a scale out event.
*
*
* @return The maximum value to scale to in response to a scale out event.
*/
public Integer getMaxCapacity() {
return this.maxCapacity;
}
/**
*
* The maximum value to scale to in response to a scale out event.
*
*
* @param maxCapacity
* The maximum value to scale to in response to a scale out event.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ScalableTarget withMaxCapacity(Integer maxCapacity) {
setMaxCapacity(maxCapacity);
return this;
}
/**
*
* The ARN of an IAM role that allows Application Auto Scaling to modify the scalable target on your behalf.
*
*
* @param roleARN
* The ARN of an IAM role that allows Application Auto Scaling to modify the scalable target on your behalf.
*/
public void setRoleARN(String roleARN) {
this.roleARN = roleARN;
}
/**
*
* The ARN of an IAM role that allows Application Auto Scaling to modify the scalable target on your behalf.
*
*
* @return The ARN of an IAM role that allows Application Auto Scaling to modify the scalable target on your behalf.
*/
public String getRoleARN() {
return this.roleARN;
}
/**
*
* The ARN of an IAM role that allows Application Auto Scaling to modify the scalable target on your behalf.
*
*
* @param roleARN
* The ARN of an IAM role that allows Application Auto Scaling to modify the scalable target on your behalf.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ScalableTarget withRoleARN(String roleARN) {
setRoleARN(roleARN);
return this;
}
/**
*
* The Unix timestamp for when the scalable target was created.
*
*
* @param creationTime
* The Unix timestamp for when the scalable target was created.
*/
public void setCreationTime(java.util.Date creationTime) {
this.creationTime = creationTime;
}
/**
*
* The Unix timestamp for when the scalable target was created.
*
*
* @return The Unix timestamp for when the scalable target was created.
*/
public java.util.Date getCreationTime() {
return this.creationTime;
}
/**
*
* The Unix timestamp for when the scalable target was created.
*
*
* @param creationTime
* The Unix timestamp for when the scalable target was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ScalableTarget withCreationTime(java.util.Date creationTime) {
setCreationTime(creationTime);
return this;
}
/**
* Returns a string representation of this object; useful for testing and debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getServiceNamespace() != null)
sb.append("ServiceNamespace: ").append(getServiceNamespace()).append(",");
if (getResourceId() != null)
sb.append("ResourceId: ").append(getResourceId()).append(",");
if (getScalableDimension() != null)
sb.append("ScalableDimension: ").append(getScalableDimension()).append(",");
if (getMinCapacity() != null)
sb.append("MinCapacity: ").append(getMinCapacity()).append(",");
if (getMaxCapacity() != null)
sb.append("MaxCapacity: ").append(getMaxCapacity()).append(",");
if (getRoleARN() != null)
sb.append("RoleARN: ").append(getRoleARN()).append(",");
if (getCreationTime() != null)
sb.append("CreationTime: ").append(getCreationTime());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ScalableTarget == false)
return false;
ScalableTarget other = (ScalableTarget) obj;
if (other.getServiceNamespace() == null ^ this.getServiceNamespace() == null)
return false;
if (other.getServiceNamespace() != null && other.getServiceNamespace().equals(this.getServiceNamespace()) == false)
return false;
if (other.getResourceId() == null ^ this.getResourceId() == null)
return false;
if (other.getResourceId() != null && other.getResourceId().equals(this.getResourceId()) == false)
return false;
if (other.getScalableDimension() == null ^ this.getScalableDimension() == null)
return false;
if (other.getScalableDimension() != null && other.getScalableDimension().equals(this.getScalableDimension()) == false)
return false;
if (other.getMinCapacity() == null ^ this.getMinCapacity() == null)
return false;
if (other.getMinCapacity() != null && other.getMinCapacity().equals(this.getMinCapacity()) == false)
return false;
if (other.getMaxCapacity() == null ^ this.getMaxCapacity() == null)
return false;
if (other.getMaxCapacity() != null && other.getMaxCapacity().equals(this.getMaxCapacity()) == false)
return false;
if (other.getRoleARN() == null ^ this.getRoleARN() == null)
return false;
if (other.getRoleARN() != null && other.getRoleARN().equals(this.getRoleARN()) == false)
return false;
if (other.getCreationTime() == null ^ this.getCreationTime() == null)
return false;
if (other.getCreationTime() != null && other.getCreationTime().equals(this.getCreationTime()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getServiceNamespace() == null) ? 0 : getServiceNamespace().hashCode());
hashCode = prime * hashCode + ((getResourceId() == null) ? 0 : getResourceId().hashCode());
hashCode = prime * hashCode + ((getScalableDimension() == null) ? 0 : getScalableDimension().hashCode());
hashCode = prime * hashCode + ((getMinCapacity() == null) ? 0 : getMinCapacity().hashCode());
hashCode = prime * hashCode + ((getMaxCapacity() == null) ? 0 : getMaxCapacity().hashCode());
hashCode = prime * hashCode + ((getRoleARN() == null) ? 0 : getRoleARN().hashCode());
hashCode = prime * hashCode + ((getCreationTime() == null) ? 0 : getCreationTime().hashCode());
return hashCode;
}
@Override
public ScalableTarget clone() {
try {
return (ScalableTarget) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}