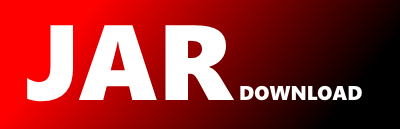
com.amazonaws.services.applicationautoscaling.AWSApplicationAutoScalingAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-applicationautoscaling Show documentation
/*
* Copyright 2015-2020 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.applicationautoscaling;
import javax.annotation.Generated;
import com.amazonaws.services.applicationautoscaling.model.*;
/**
* Interface for accessing Application Auto Scaling asynchronously. Each asynchronous method will return a Java Future
* object representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.applicationautoscaling.AbstractAWSApplicationAutoScalingAsync} instead.
*
*
*
* With Application Auto Scaling, you can configure automatic scaling for the following resources:
*
*
* -
*
* Amazon ECS services
*
*
* -
*
* Amazon EC2 Spot Fleet requests
*
*
* -
*
* Amazon EMR clusters
*
*
* -
*
* Amazon AppStream 2.0 fleets
*
*
* -
*
* Amazon DynamoDB tables and global secondary indexes throughput capacity
*
*
* -
*
* Amazon Aurora Replicas
*
*
* -
*
* Amazon SageMaker endpoint variants
*
*
* -
*
* Custom resources provided by your own applications or services
*
*
* -
*
* Amazon Comprehend document classification endpoints
*
*
* -
*
* AWS Lambda function provisioned concurrency
*
*
* -
*
* Amazon Keyspaces (for Apache Cassandra) tables
*
*
*
*
* API Summary
*
*
* The Application Auto Scaling service API includes three key sets of actions:
*
*
* -
*
* Register and manage scalable targets - Register AWS or custom resources as scalable targets (a resource that
* Application Auto Scaling can scale), set minimum and maximum capacity limits, and retrieve information on existing
* scalable targets.
*
*
* -
*
* Configure and manage automatic scaling - Define scaling policies to dynamically scale your resources in response to
* CloudWatch alarms, schedule one-time or recurring scaling actions, and retrieve your recent scaling activity history.
*
*
* -
*
* Suspend and resume scaling - Temporarily suspend and later resume automatic scaling by calling the RegisterScalableTarget API action for any Application Auto Scaling scalable target. You can suspend and resume
* (individually or in combination) scale-out activities that are triggered by a scaling policy, scale-in activities
* that are triggered by a scaling policy, and scheduled scaling.
*
*
*
*
* To learn more about Application Auto Scaling, including information about granting IAM users required permissions for
* Application Auto Scaling actions, see the Application Auto Scaling User Guide.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSApplicationAutoScalingAsync extends AWSApplicationAutoScaling {
/**
*
* Deletes the specified scaling policy for an Application Auto Scaling scalable target.
*
*
* Deleting a step scaling policy deletes the underlying alarm action, but does not delete the CloudWatch alarm
* associated with the scaling policy, even if it no longer has an associated action.
*
*
* For more information, see Delete a Step Scaling Policy and Delete a Target Tracking Scaling Policy in the Application Auto Scaling User Guide.
*
*
* @param deleteScalingPolicyRequest
* @return A Java Future containing the result of the DeleteScalingPolicy operation returned by the service.
* @sample AWSApplicationAutoScalingAsync.DeleteScalingPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteScalingPolicyAsync(DeleteScalingPolicyRequest deleteScalingPolicyRequest);
/**
*
* Deletes the specified scaling policy for an Application Auto Scaling scalable target.
*
*
* Deleting a step scaling policy deletes the underlying alarm action, but does not delete the CloudWatch alarm
* associated with the scaling policy, even if it no longer has an associated action.
*
*
* For more information, see Delete a Step Scaling Policy and Delete a Target Tracking Scaling Policy in the Application Auto Scaling User Guide.
*
*
* @param deleteScalingPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteScalingPolicy operation returned by the service.
* @sample AWSApplicationAutoScalingAsyncHandler.DeleteScalingPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteScalingPolicyAsync(DeleteScalingPolicyRequest deleteScalingPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified scheduled action for an Application Auto Scaling scalable target.
*
*
* For more information, see Delete a Scheduled Action in the Application Auto Scaling User Guide.
*
*
* @param deleteScheduledActionRequest
* @return A Java Future containing the result of the DeleteScheduledAction operation returned by the service.
* @sample AWSApplicationAutoScalingAsync.DeleteScheduledAction
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteScheduledActionAsync(DeleteScheduledActionRequest deleteScheduledActionRequest);
/**
*
* Deletes the specified scheduled action for an Application Auto Scaling scalable target.
*
*
* For more information, see Delete a Scheduled Action in the Application Auto Scaling User Guide.
*
*
* @param deleteScheduledActionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteScheduledAction operation returned by the service.
* @sample AWSApplicationAutoScalingAsyncHandler.DeleteScheduledAction
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteScheduledActionAsync(DeleteScheduledActionRequest deleteScheduledActionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deregisters an Application Auto Scaling scalable target when you have finished using it. To see which resources
* have been registered, use DescribeScalableTargets.
*
*
*
* Deregistering a scalable target deletes the scaling policies and the scheduled actions that are associated with
* it.
*
*
*
* @param deregisterScalableTargetRequest
* @return A Java Future containing the result of the DeregisterScalableTarget operation returned by the service.
* @sample AWSApplicationAutoScalingAsync.DeregisterScalableTarget
* @see AWS API Documentation
*/
java.util.concurrent.Future deregisterScalableTargetAsync(DeregisterScalableTargetRequest deregisterScalableTargetRequest);
/**
*
* Deregisters an Application Auto Scaling scalable target when you have finished using it. To see which resources
* have been registered, use DescribeScalableTargets.
*
*
*
* Deregistering a scalable target deletes the scaling policies and the scheduled actions that are associated with
* it.
*
*
*
* @param deregisterScalableTargetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeregisterScalableTarget operation returned by the service.
* @sample AWSApplicationAutoScalingAsyncHandler.DeregisterScalableTarget
* @see AWS API Documentation
*/
java.util.concurrent.Future deregisterScalableTargetAsync(DeregisterScalableTargetRequest deregisterScalableTargetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about the scalable targets in the specified namespace.
*
*
* You can filter the results using ResourceIds
and ScalableDimension
.
*
*
* @param describeScalableTargetsRequest
* @return A Java Future containing the result of the DescribeScalableTargets operation returned by the service.
* @sample AWSApplicationAutoScalingAsync.DescribeScalableTargets
* @see AWS API Documentation
*/
java.util.concurrent.Future describeScalableTargetsAsync(DescribeScalableTargetsRequest describeScalableTargetsRequest);
/**
*
* Gets information about the scalable targets in the specified namespace.
*
*
* You can filter the results using ResourceIds
and ScalableDimension
.
*
*
* @param describeScalableTargetsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeScalableTargets operation returned by the service.
* @sample AWSApplicationAutoScalingAsyncHandler.DescribeScalableTargets
* @see AWS API Documentation
*/
java.util.concurrent.Future describeScalableTargetsAsync(DescribeScalableTargetsRequest describeScalableTargetsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides descriptive information about the scaling activities in the specified namespace from the previous six
* weeks.
*
*
* You can filter the results using ResourceId
and ScalableDimension
.
*
*
* @param describeScalingActivitiesRequest
* @return A Java Future containing the result of the DescribeScalingActivities operation returned by the service.
* @sample AWSApplicationAutoScalingAsync.DescribeScalingActivities
* @see AWS API Documentation
*/
java.util.concurrent.Future describeScalingActivitiesAsync(
DescribeScalingActivitiesRequest describeScalingActivitiesRequest);
/**
*
* Provides descriptive information about the scaling activities in the specified namespace from the previous six
* weeks.
*
*
* You can filter the results using ResourceId
and ScalableDimension
.
*
*
* @param describeScalingActivitiesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeScalingActivities operation returned by the service.
* @sample AWSApplicationAutoScalingAsyncHandler.DescribeScalingActivities
* @see AWS API Documentation
*/
java.util.concurrent.Future describeScalingActivitiesAsync(
DescribeScalingActivitiesRequest describeScalingActivitiesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the Application Auto Scaling scaling policies for the specified service namespace.
*
*
* You can filter the results using ResourceId
, ScalableDimension
, and
* PolicyNames
.
*
*
* For more information, see Target Tracking Scaling Policies and Step Scaling Policies in the Application Auto Scaling User Guide.
*
*
* @param describeScalingPoliciesRequest
* @return A Java Future containing the result of the DescribeScalingPolicies operation returned by the service.
* @sample AWSApplicationAutoScalingAsync.DescribeScalingPolicies
* @see AWS API Documentation
*/
java.util.concurrent.Future describeScalingPoliciesAsync(DescribeScalingPoliciesRequest describeScalingPoliciesRequest);
/**
*
* Describes the Application Auto Scaling scaling policies for the specified service namespace.
*
*
* You can filter the results using ResourceId
, ScalableDimension
, and
* PolicyNames
.
*
*
* For more information, see Target Tracking Scaling Policies and Step Scaling Policies in the Application Auto Scaling User Guide.
*
*
* @param describeScalingPoliciesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeScalingPolicies operation returned by the service.
* @sample AWSApplicationAutoScalingAsyncHandler.DescribeScalingPolicies
* @see AWS API Documentation
*/
java.util.concurrent.Future describeScalingPoliciesAsync(DescribeScalingPoliciesRequest describeScalingPoliciesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the Application Auto Scaling scheduled actions for the specified service namespace.
*
*
* You can filter the results using the ResourceId
, ScalableDimension
, and
* ScheduledActionNames
parameters.
*
*
* For more information, see Scheduled Scaling in the Application Auto Scaling User Guide.
*
*
* @param describeScheduledActionsRequest
* @return A Java Future containing the result of the DescribeScheduledActions operation returned by the service.
* @sample AWSApplicationAutoScalingAsync.DescribeScheduledActions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeScheduledActionsAsync(DescribeScheduledActionsRequest describeScheduledActionsRequest);
/**
*
* Describes the Application Auto Scaling scheduled actions for the specified service namespace.
*
*
* You can filter the results using the ResourceId
, ScalableDimension
, and
* ScheduledActionNames
parameters.
*
*
* For more information, see Scheduled Scaling in the Application Auto Scaling User Guide.
*
*
* @param describeScheduledActionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeScheduledActions operation returned by the service.
* @sample AWSApplicationAutoScalingAsyncHandler.DescribeScheduledActions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeScheduledActionsAsync(DescribeScheduledActionsRequest describeScheduledActionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates or updates a scaling policy for an Application Auto Scaling scalable target.
*
*
* Each scalable target is identified by a service namespace, resource ID, and scalable dimension. A scaling policy
* applies to the scalable target identified by those three attributes. You cannot create a scaling policy until you
* have registered the resource as a scalable target.
*
*
* Multiple scaling policies can be in force at the same time for the same scalable target. You can have one or more
* target tracking scaling policies, one or more step scaling policies, or both. However, there is a chance that
* multiple policies could conflict, instructing the scalable target to scale out or in at the same time.
* Application Auto Scaling gives precedence to the policy that provides the largest capacity for both scale out and
* scale in. For example, if one policy increases capacity by 3, another policy increases capacity by 200 percent,
* and the current capacity is 10, Application Auto Scaling uses the policy with the highest calculated capacity
* (200% of 10 = 20) and scales out to 30.
*
*
* We recommend caution, however, when using target tracking scaling policies with step scaling policies because
* conflicts between these policies can cause undesirable behavior. For example, if the step scaling policy
* initiates a scale-in activity before the target tracking policy is ready to scale in, the scale-in activity will
* not be blocked. After the scale-in activity completes, the target tracking policy could instruct the scalable
* target to scale out again.
*
*
* For more information, see Target Tracking Scaling Policies and Step Scaling Policies in the Application Auto Scaling User Guide.
*
*
*
* If a scalable target is deregistered, the scalable target is no longer available to execute scaling policies. Any
* scaling policies that were specified for the scalable target are deleted.
*
*
*
* @param putScalingPolicyRequest
* @return A Java Future containing the result of the PutScalingPolicy operation returned by the service.
* @sample AWSApplicationAutoScalingAsync.PutScalingPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future putScalingPolicyAsync(PutScalingPolicyRequest putScalingPolicyRequest);
/**
*
* Creates or updates a scaling policy for an Application Auto Scaling scalable target.
*
*
* Each scalable target is identified by a service namespace, resource ID, and scalable dimension. A scaling policy
* applies to the scalable target identified by those three attributes. You cannot create a scaling policy until you
* have registered the resource as a scalable target.
*
*
* Multiple scaling policies can be in force at the same time for the same scalable target. You can have one or more
* target tracking scaling policies, one or more step scaling policies, or both. However, there is a chance that
* multiple policies could conflict, instructing the scalable target to scale out or in at the same time.
* Application Auto Scaling gives precedence to the policy that provides the largest capacity for both scale out and
* scale in. For example, if one policy increases capacity by 3, another policy increases capacity by 200 percent,
* and the current capacity is 10, Application Auto Scaling uses the policy with the highest calculated capacity
* (200% of 10 = 20) and scales out to 30.
*
*
* We recommend caution, however, when using target tracking scaling policies with step scaling policies because
* conflicts between these policies can cause undesirable behavior. For example, if the step scaling policy
* initiates a scale-in activity before the target tracking policy is ready to scale in, the scale-in activity will
* not be blocked. After the scale-in activity completes, the target tracking policy could instruct the scalable
* target to scale out again.
*
*
* For more information, see Target Tracking Scaling Policies and Step Scaling Policies in the Application Auto Scaling User Guide.
*
*
*
* If a scalable target is deregistered, the scalable target is no longer available to execute scaling policies. Any
* scaling policies that were specified for the scalable target are deleted.
*
*
*
* @param putScalingPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutScalingPolicy operation returned by the service.
* @sample AWSApplicationAutoScalingAsyncHandler.PutScalingPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future putScalingPolicyAsync(PutScalingPolicyRequest putScalingPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates or updates a scheduled action for an Application Auto Scaling scalable target.
*
*
* Each scalable target is identified by a service namespace, resource ID, and scalable dimension. A scheduled
* action applies to the scalable target identified by those three attributes. You cannot create a scheduled action
* until you have registered the resource as a scalable target.
*
*
* When start and end times are specified with a recurring schedule using a cron expression or rates, they form the
* boundaries of when the recurring action starts and stops.
*
*
* To update a scheduled action, specify the parameters that you want to change. If you don't specify start and end
* times, the old values are deleted.
*
*
* For more information, see Scheduled Scaling in the Application Auto Scaling User Guide.
*
*
*
* If a scalable target is deregistered, the scalable target is no longer available to run scheduled actions. Any
* scheduled actions that were specified for the scalable target are deleted.
*
*
*
* @param putScheduledActionRequest
* @return A Java Future containing the result of the PutScheduledAction operation returned by the service.
* @sample AWSApplicationAutoScalingAsync.PutScheduledAction
* @see AWS API Documentation
*/
java.util.concurrent.Future putScheduledActionAsync(PutScheduledActionRequest putScheduledActionRequest);
/**
*
* Creates or updates a scheduled action for an Application Auto Scaling scalable target.
*
*
* Each scalable target is identified by a service namespace, resource ID, and scalable dimension. A scheduled
* action applies to the scalable target identified by those three attributes. You cannot create a scheduled action
* until you have registered the resource as a scalable target.
*
*
* When start and end times are specified with a recurring schedule using a cron expression or rates, they form the
* boundaries of when the recurring action starts and stops.
*
*
* To update a scheduled action, specify the parameters that you want to change. If you don't specify start and end
* times, the old values are deleted.
*
*
* For more information, see Scheduled Scaling in the Application Auto Scaling User Guide.
*
*
*
* If a scalable target is deregistered, the scalable target is no longer available to run scheduled actions. Any
* scheduled actions that were specified for the scalable target are deleted.
*
*
*
* @param putScheduledActionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutScheduledAction operation returned by the service.
* @sample AWSApplicationAutoScalingAsyncHandler.PutScheduledAction
* @see AWS API Documentation
*/
java.util.concurrent.Future putScheduledActionAsync(PutScheduledActionRequest putScheduledActionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Registers or updates a scalable target.
*
*
* A scalable target is a resource that Application Auto Scaling can scale out and scale in. Scalable targets are
* uniquely identified by the combination of resource ID, scalable dimension, and namespace.
*
*
* When you register a new scalable target, you must specify values for minimum and maximum capacity. Application
* Auto Scaling scaling policies will not scale capacity to values that are outside of this range.
*
*
* After you register a scalable target, you do not need to register it again to use other Application Auto Scaling
* operations. To see which resources have been registered, use DescribeScalableTargets. You can also view the scaling policies for a service namespace by using DescribeScalableTargets. If you no longer need a scalable target, you can deregister it by using DeregisterScalableTarget.
*
*
* To update a scalable target, specify the parameters that you want to change. Include the parameters that identify
* the scalable target: resource ID, scalable dimension, and namespace. Any parameters that you don't specify are
* not changed by this update request.
*
*
* @param registerScalableTargetRequest
* @return A Java Future containing the result of the RegisterScalableTarget operation returned by the service.
* @sample AWSApplicationAutoScalingAsync.RegisterScalableTarget
* @see AWS API Documentation
*/
java.util.concurrent.Future registerScalableTargetAsync(RegisterScalableTargetRequest registerScalableTargetRequest);
/**
*
* Registers or updates a scalable target.
*
*
* A scalable target is a resource that Application Auto Scaling can scale out and scale in. Scalable targets are
* uniquely identified by the combination of resource ID, scalable dimension, and namespace.
*
*
* When you register a new scalable target, you must specify values for minimum and maximum capacity. Application
* Auto Scaling scaling policies will not scale capacity to values that are outside of this range.
*
*
* After you register a scalable target, you do not need to register it again to use other Application Auto Scaling
* operations. To see which resources have been registered, use DescribeScalableTargets. You can also view the scaling policies for a service namespace by using DescribeScalableTargets. If you no longer need a scalable target, you can deregister it by using DeregisterScalableTarget.
*
*
* To update a scalable target, specify the parameters that you want to change. Include the parameters that identify
* the scalable target: resource ID, scalable dimension, and namespace. Any parameters that you don't specify are
* not changed by this update request.
*
*
* @param registerScalableTargetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RegisterScalableTarget operation returned by the service.
* @sample AWSApplicationAutoScalingAsyncHandler.RegisterScalableTarget
* @see AWS API Documentation
*/
java.util.concurrent.Future registerScalableTargetAsync(RegisterScalableTargetRequest registerScalableTargetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}