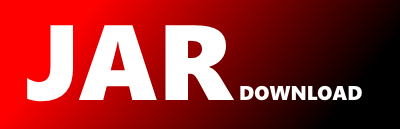
com.amazonaws.services.applicationautoscaling.model.ScalableTargetAction Maven / Gradle / Ivy
Show all versions of aws-java-sdk-applicationautoscaling Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.applicationautoscaling.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Represents the minimum and maximum capacity for a scheduled action.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ScalableTargetAction implements Serializable, Cloneable, StructuredPojo {
/**
*
* The minimum capacity.
*
*
* When the scheduled action runs, the resource will have at least this much capacity, but it might have more
* depending on other settings, such as the target utilization level of a target tracking scaling policy.
*
*/
private Integer minCapacity;
/**
*
* The maximum capacity.
*
*
* Although you can specify a large maximum capacity, note that service quotas may impose lower limits. Each service
* has its own default quotas for the maximum capacity of the resource. If you want to specify a higher limit, you
* can request an increase. For more information, consult the documentation for that service. For information about
* the default quotas for each service, see Service endpoints and
* quotas in the Amazon Web Services General Reference.
*
*/
private Integer maxCapacity;
/**
*
* The minimum capacity.
*
*
* When the scheduled action runs, the resource will have at least this much capacity, but it might have more
* depending on other settings, such as the target utilization level of a target tracking scaling policy.
*
*
* @param minCapacity
* The minimum capacity.
*
* When the scheduled action runs, the resource will have at least this much capacity, but it might have more
* depending on other settings, such as the target utilization level of a target tracking scaling policy.
*/
public void setMinCapacity(Integer minCapacity) {
this.minCapacity = minCapacity;
}
/**
*
* The minimum capacity.
*
*
* When the scheduled action runs, the resource will have at least this much capacity, but it might have more
* depending on other settings, such as the target utilization level of a target tracking scaling policy.
*
*
* @return The minimum capacity.
*
* When the scheduled action runs, the resource will have at least this much capacity, but it might have
* more depending on other settings, such as the target utilization level of a target tracking scaling
* policy.
*/
public Integer getMinCapacity() {
return this.minCapacity;
}
/**
*
* The minimum capacity.
*
*
* When the scheduled action runs, the resource will have at least this much capacity, but it might have more
* depending on other settings, such as the target utilization level of a target tracking scaling policy.
*
*
* @param minCapacity
* The minimum capacity.
*
* When the scheduled action runs, the resource will have at least this much capacity, but it might have more
* depending on other settings, such as the target utilization level of a target tracking scaling policy.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ScalableTargetAction withMinCapacity(Integer minCapacity) {
setMinCapacity(minCapacity);
return this;
}
/**
*
* The maximum capacity.
*
*
* Although you can specify a large maximum capacity, note that service quotas may impose lower limits. Each service
* has its own default quotas for the maximum capacity of the resource. If you want to specify a higher limit, you
* can request an increase. For more information, consult the documentation for that service. For information about
* the default quotas for each service, see Service endpoints and
* quotas in the Amazon Web Services General Reference.
*
*
* @param maxCapacity
* The maximum capacity.
*
* Although you can specify a large maximum capacity, note that service quotas may impose lower limits. Each
* service has its own default quotas for the maximum capacity of the resource. If you want to specify a
* higher limit, you can request an increase. For more information, consult the documentation for that
* service. For information about the default quotas for each service, see Service endpoints and
* quotas in the Amazon Web Services General Reference.
*/
public void setMaxCapacity(Integer maxCapacity) {
this.maxCapacity = maxCapacity;
}
/**
*
* The maximum capacity.
*
*
* Although you can specify a large maximum capacity, note that service quotas may impose lower limits. Each service
* has its own default quotas for the maximum capacity of the resource. If you want to specify a higher limit, you
* can request an increase. For more information, consult the documentation for that service. For information about
* the default quotas for each service, see Service endpoints and
* quotas in the Amazon Web Services General Reference.
*
*
* @return The maximum capacity.
*
* Although you can specify a large maximum capacity, note that service quotas may impose lower limits. Each
* service has its own default quotas for the maximum capacity of the resource. If you want to specify a
* higher limit, you can request an increase. For more information, consult the documentation for that
* service. For information about the default quotas for each service, see Service endpoints and
* quotas in the Amazon Web Services General Reference.
*/
public Integer getMaxCapacity() {
return this.maxCapacity;
}
/**
*
* The maximum capacity.
*
*
* Although you can specify a large maximum capacity, note that service quotas may impose lower limits. Each service
* has its own default quotas for the maximum capacity of the resource. If you want to specify a higher limit, you
* can request an increase. For more information, consult the documentation for that service. For information about
* the default quotas for each service, see Service endpoints and
* quotas in the Amazon Web Services General Reference.
*
*
* @param maxCapacity
* The maximum capacity.
*
* Although you can specify a large maximum capacity, note that service quotas may impose lower limits. Each
* service has its own default quotas for the maximum capacity of the resource. If you want to specify a
* higher limit, you can request an increase. For more information, consult the documentation for that
* service. For information about the default quotas for each service, see Service endpoints and
* quotas in the Amazon Web Services General Reference.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ScalableTargetAction withMaxCapacity(Integer maxCapacity) {
setMaxCapacity(maxCapacity);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getMinCapacity() != null)
sb.append("MinCapacity: ").append(getMinCapacity()).append(",");
if (getMaxCapacity() != null)
sb.append("MaxCapacity: ").append(getMaxCapacity());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ScalableTargetAction == false)
return false;
ScalableTargetAction other = (ScalableTargetAction) obj;
if (other.getMinCapacity() == null ^ this.getMinCapacity() == null)
return false;
if (other.getMinCapacity() != null && other.getMinCapacity().equals(this.getMinCapacity()) == false)
return false;
if (other.getMaxCapacity() == null ^ this.getMaxCapacity() == null)
return false;
if (other.getMaxCapacity() != null && other.getMaxCapacity().equals(this.getMaxCapacity()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getMinCapacity() == null) ? 0 : getMinCapacity().hashCode());
hashCode = prime * hashCode + ((getMaxCapacity() == null) ? 0 : getMaxCapacity().hashCode());
return hashCode;
}
@Override
public ScalableTargetAction clone() {
try {
return (ScalableTargetAction) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.applicationautoscaling.model.transform.ScalableTargetActionMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}