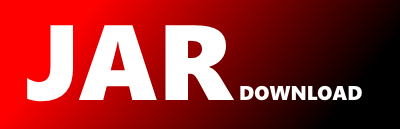
com.amazonaws.services.applicationinsights.model.CreateApplicationRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-applicationinsights Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.applicationinsights.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateApplicationRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The name of the resource group.
*
*/
private String resourceGroupName;
/**
*
* When set to true
, creates opsItems for any problems detected on an application.
*
*/
private Boolean opsCenterEnabled;
/**
*
* Indicates whether Application Insights can listen to CloudWatch events for the application resources, such as
* instance terminated
, failed deployment
, and others.
*
*/
private Boolean cWEMonitorEnabled;
/**
*
* The SNS topic provided to Application Insights that is associated to the created opsItem. Allows you to receive
* notifications for updates to the opsItem.
*
*/
private String opsItemSNSTopicArn;
/**
*
* List of tags to add to the application. tag key (Key
) and an associated tag value (
* Value
). The maximum length of a tag key is 128 characters. The maximum length of a tag value is 256
* characters.
*
*/
private java.util.List tags;
/**
*
* Indicates whether Application Insights automatically configures unmonitored resources in the resource group.
*
*/
private Boolean autoConfigEnabled;
/**
*
* Configures all of the resources in the resource group by applying the recommended configurations.
*
*/
private Boolean autoCreate;
/**
*
* Application Insights can create applications based on a resource group or on an account. To create an
* account-based application using all of the resources in the account, set this parameter to
* ACCOUNT_BASED
.
*
*/
private String groupingType;
/**
*
* If set to true, the managed policies for SSM and CW will be attached to the instance roles if they are missing.
*
*/
private Boolean attachMissingPermission;
/**
*
* The name of the resource group.
*
*
* @param resourceGroupName
* The name of the resource group.
*/
public void setResourceGroupName(String resourceGroupName) {
this.resourceGroupName = resourceGroupName;
}
/**
*
* The name of the resource group.
*
*
* @return The name of the resource group.
*/
public String getResourceGroupName() {
return this.resourceGroupName;
}
/**
*
* The name of the resource group.
*
*
* @param resourceGroupName
* The name of the resource group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateApplicationRequest withResourceGroupName(String resourceGroupName) {
setResourceGroupName(resourceGroupName);
return this;
}
/**
*
* When set to true
, creates opsItems for any problems detected on an application.
*
*
* @param opsCenterEnabled
* When set to true
, creates opsItems for any problems detected on an application.
*/
public void setOpsCenterEnabled(Boolean opsCenterEnabled) {
this.opsCenterEnabled = opsCenterEnabled;
}
/**
*
* When set to true
, creates opsItems for any problems detected on an application.
*
*
* @return When set to true
, creates opsItems for any problems detected on an application.
*/
public Boolean getOpsCenterEnabled() {
return this.opsCenterEnabled;
}
/**
*
* When set to true
, creates opsItems for any problems detected on an application.
*
*
* @param opsCenterEnabled
* When set to true
, creates opsItems for any problems detected on an application.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateApplicationRequest withOpsCenterEnabled(Boolean opsCenterEnabled) {
setOpsCenterEnabled(opsCenterEnabled);
return this;
}
/**
*
* When set to true
, creates opsItems for any problems detected on an application.
*
*
* @return When set to true
, creates opsItems for any problems detected on an application.
*/
public Boolean isOpsCenterEnabled() {
return this.opsCenterEnabled;
}
/**
*
* Indicates whether Application Insights can listen to CloudWatch events for the application resources, such as
* instance terminated
, failed deployment
, and others.
*
*
* @param cWEMonitorEnabled
* Indicates whether Application Insights can listen to CloudWatch events for the application resources, such
* as instance terminated
, failed deployment
, and others.
*/
public void setCWEMonitorEnabled(Boolean cWEMonitorEnabled) {
this.cWEMonitorEnabled = cWEMonitorEnabled;
}
/**
*
* Indicates whether Application Insights can listen to CloudWatch events for the application resources, such as
* instance terminated
, failed deployment
, and others.
*
*
* @return Indicates whether Application Insights can listen to CloudWatch events for the application resources,
* such as instance terminated
, failed deployment
, and others.
*/
public Boolean getCWEMonitorEnabled() {
return this.cWEMonitorEnabled;
}
/**
*
* Indicates whether Application Insights can listen to CloudWatch events for the application resources, such as
* instance terminated
, failed deployment
, and others.
*
*
* @param cWEMonitorEnabled
* Indicates whether Application Insights can listen to CloudWatch events for the application resources, such
* as instance terminated
, failed deployment
, and others.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateApplicationRequest withCWEMonitorEnabled(Boolean cWEMonitorEnabled) {
setCWEMonitorEnabled(cWEMonitorEnabled);
return this;
}
/**
*
* Indicates whether Application Insights can listen to CloudWatch events for the application resources, such as
* instance terminated
, failed deployment
, and others.
*
*
* @return Indicates whether Application Insights can listen to CloudWatch events for the application resources,
* such as instance terminated
, failed deployment
, and others.
*/
public Boolean isCWEMonitorEnabled() {
return this.cWEMonitorEnabled;
}
/**
*
* The SNS topic provided to Application Insights that is associated to the created opsItem. Allows you to receive
* notifications for updates to the opsItem.
*
*
* @param opsItemSNSTopicArn
* The SNS topic provided to Application Insights that is associated to the created opsItem. Allows you to
* receive notifications for updates to the opsItem.
*/
public void setOpsItemSNSTopicArn(String opsItemSNSTopicArn) {
this.opsItemSNSTopicArn = opsItemSNSTopicArn;
}
/**
*
* The SNS topic provided to Application Insights that is associated to the created opsItem. Allows you to receive
* notifications for updates to the opsItem.
*
*
* @return The SNS topic provided to Application Insights that is associated to the created opsItem. Allows you to
* receive notifications for updates to the opsItem.
*/
public String getOpsItemSNSTopicArn() {
return this.opsItemSNSTopicArn;
}
/**
*
* The SNS topic provided to Application Insights that is associated to the created opsItem. Allows you to receive
* notifications for updates to the opsItem.
*
*
* @param opsItemSNSTopicArn
* The SNS topic provided to Application Insights that is associated to the created opsItem. Allows you to
* receive notifications for updates to the opsItem.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateApplicationRequest withOpsItemSNSTopicArn(String opsItemSNSTopicArn) {
setOpsItemSNSTopicArn(opsItemSNSTopicArn);
return this;
}
/**
*
* List of tags to add to the application. tag key (Key
) and an associated tag value (
* Value
). The maximum length of a tag key is 128 characters. The maximum length of a tag value is 256
* characters.
*
*
* @return List of tags to add to the application. tag key (Key
) and an associated tag value (
* Value
). The maximum length of a tag key is 128 characters. The maximum length of a tag value
* is 256 characters.
*/
public java.util.List getTags() {
return tags;
}
/**
*
* List of tags to add to the application. tag key (Key
) and an associated tag value (
* Value
). The maximum length of a tag key is 128 characters. The maximum length of a tag value is 256
* characters.
*
*
* @param tags
* List of tags to add to the application. tag key (Key
) and an associated tag value (
* Value
). The maximum length of a tag key is 128 characters. The maximum length of a tag value
* is 256 characters.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new java.util.ArrayList(tags);
}
/**
*
* List of tags to add to the application. tag key (Key
) and an associated tag value (
* Value
). The maximum length of a tag key is 128 characters. The maximum length of a tag value is 256
* characters.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* List of tags to add to the application. tag key (Key
) and an associated tag value (
* Value
). The maximum length of a tag key is 128 characters. The maximum length of a tag value
* is 256 characters.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateApplicationRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new java.util.ArrayList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* List of tags to add to the application. tag key (Key
) and an associated tag value (
* Value
). The maximum length of a tag key is 128 characters. The maximum length of a tag value is 256
* characters.
*
*
* @param tags
* List of tags to add to the application. tag key (Key
) and an associated tag value (
* Value
). The maximum length of a tag key is 128 characters. The maximum length of a tag value
* is 256 characters.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateApplicationRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
*
* Indicates whether Application Insights automatically configures unmonitored resources in the resource group.
*
*
* @param autoConfigEnabled
* Indicates whether Application Insights automatically configures unmonitored resources in the resource
* group.
*/
public void setAutoConfigEnabled(Boolean autoConfigEnabled) {
this.autoConfigEnabled = autoConfigEnabled;
}
/**
*
* Indicates whether Application Insights automatically configures unmonitored resources in the resource group.
*
*
* @return Indicates whether Application Insights automatically configures unmonitored resources in the resource
* group.
*/
public Boolean getAutoConfigEnabled() {
return this.autoConfigEnabled;
}
/**
*
* Indicates whether Application Insights automatically configures unmonitored resources in the resource group.
*
*
* @param autoConfigEnabled
* Indicates whether Application Insights automatically configures unmonitored resources in the resource
* group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateApplicationRequest withAutoConfigEnabled(Boolean autoConfigEnabled) {
setAutoConfigEnabled(autoConfigEnabled);
return this;
}
/**
*
* Indicates whether Application Insights automatically configures unmonitored resources in the resource group.
*
*
* @return Indicates whether Application Insights automatically configures unmonitored resources in the resource
* group.
*/
public Boolean isAutoConfigEnabled() {
return this.autoConfigEnabled;
}
/**
*
* Configures all of the resources in the resource group by applying the recommended configurations.
*
*
* @param autoCreate
* Configures all of the resources in the resource group by applying the recommended configurations.
*/
public void setAutoCreate(Boolean autoCreate) {
this.autoCreate = autoCreate;
}
/**
*
* Configures all of the resources in the resource group by applying the recommended configurations.
*
*
* @return Configures all of the resources in the resource group by applying the recommended configurations.
*/
public Boolean getAutoCreate() {
return this.autoCreate;
}
/**
*
* Configures all of the resources in the resource group by applying the recommended configurations.
*
*
* @param autoCreate
* Configures all of the resources in the resource group by applying the recommended configurations.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateApplicationRequest withAutoCreate(Boolean autoCreate) {
setAutoCreate(autoCreate);
return this;
}
/**
*
* Configures all of the resources in the resource group by applying the recommended configurations.
*
*
* @return Configures all of the resources in the resource group by applying the recommended configurations.
*/
public Boolean isAutoCreate() {
return this.autoCreate;
}
/**
*
* Application Insights can create applications based on a resource group or on an account. To create an
* account-based application using all of the resources in the account, set this parameter to
* ACCOUNT_BASED
.
*
*
* @param groupingType
* Application Insights can create applications based on a resource group or on an account. To create an
* account-based application using all of the resources in the account, set this parameter to
* ACCOUNT_BASED
.
* @see GroupingType
*/
public void setGroupingType(String groupingType) {
this.groupingType = groupingType;
}
/**
*
* Application Insights can create applications based on a resource group or on an account. To create an
* account-based application using all of the resources in the account, set this parameter to
* ACCOUNT_BASED
.
*
*
* @return Application Insights can create applications based on a resource group or on an account. To create an
* account-based application using all of the resources in the account, set this parameter to
* ACCOUNT_BASED
.
* @see GroupingType
*/
public String getGroupingType() {
return this.groupingType;
}
/**
*
* Application Insights can create applications based on a resource group or on an account. To create an
* account-based application using all of the resources in the account, set this parameter to
* ACCOUNT_BASED
.
*
*
* @param groupingType
* Application Insights can create applications based on a resource group or on an account. To create an
* account-based application using all of the resources in the account, set this parameter to
* ACCOUNT_BASED
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see GroupingType
*/
public CreateApplicationRequest withGroupingType(String groupingType) {
setGroupingType(groupingType);
return this;
}
/**
*
* Application Insights can create applications based on a resource group or on an account. To create an
* account-based application using all of the resources in the account, set this parameter to
* ACCOUNT_BASED
.
*
*
* @param groupingType
* Application Insights can create applications based on a resource group or on an account. To create an
* account-based application using all of the resources in the account, set this parameter to
* ACCOUNT_BASED
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see GroupingType
*/
public CreateApplicationRequest withGroupingType(GroupingType groupingType) {
this.groupingType = groupingType.toString();
return this;
}
/**
*
* If set to true, the managed policies for SSM and CW will be attached to the instance roles if they are missing.
*
*
* @param attachMissingPermission
* If set to true, the managed policies for SSM and CW will be attached to the instance roles if they are
* missing.
*/
public void setAttachMissingPermission(Boolean attachMissingPermission) {
this.attachMissingPermission = attachMissingPermission;
}
/**
*
* If set to true, the managed policies for SSM and CW will be attached to the instance roles if they are missing.
*
*
* @return If set to true, the managed policies for SSM and CW will be attached to the instance roles if they are
* missing.
*/
public Boolean getAttachMissingPermission() {
return this.attachMissingPermission;
}
/**
*
* If set to true, the managed policies for SSM and CW will be attached to the instance roles if they are missing.
*
*
* @param attachMissingPermission
* If set to true, the managed policies for SSM and CW will be attached to the instance roles if they are
* missing.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateApplicationRequest withAttachMissingPermission(Boolean attachMissingPermission) {
setAttachMissingPermission(attachMissingPermission);
return this;
}
/**
*
* If set to true, the managed policies for SSM and CW will be attached to the instance roles if they are missing.
*
*
* @return If set to true, the managed policies for SSM and CW will be attached to the instance roles if they are
* missing.
*/
public Boolean isAttachMissingPermission() {
return this.attachMissingPermission;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getResourceGroupName() != null)
sb.append("ResourceGroupName: ").append(getResourceGroupName()).append(",");
if (getOpsCenterEnabled() != null)
sb.append("OpsCenterEnabled: ").append(getOpsCenterEnabled()).append(",");
if (getCWEMonitorEnabled() != null)
sb.append("CWEMonitorEnabled: ").append(getCWEMonitorEnabled()).append(",");
if (getOpsItemSNSTopicArn() != null)
sb.append("OpsItemSNSTopicArn: ").append(getOpsItemSNSTopicArn()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getAutoConfigEnabled() != null)
sb.append("AutoConfigEnabled: ").append(getAutoConfigEnabled()).append(",");
if (getAutoCreate() != null)
sb.append("AutoCreate: ").append(getAutoCreate()).append(",");
if (getGroupingType() != null)
sb.append("GroupingType: ").append(getGroupingType()).append(",");
if (getAttachMissingPermission() != null)
sb.append("AttachMissingPermission: ").append(getAttachMissingPermission());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateApplicationRequest == false)
return false;
CreateApplicationRequest other = (CreateApplicationRequest) obj;
if (other.getResourceGroupName() == null ^ this.getResourceGroupName() == null)
return false;
if (other.getResourceGroupName() != null && other.getResourceGroupName().equals(this.getResourceGroupName()) == false)
return false;
if (other.getOpsCenterEnabled() == null ^ this.getOpsCenterEnabled() == null)
return false;
if (other.getOpsCenterEnabled() != null && other.getOpsCenterEnabled().equals(this.getOpsCenterEnabled()) == false)
return false;
if (other.getCWEMonitorEnabled() == null ^ this.getCWEMonitorEnabled() == null)
return false;
if (other.getCWEMonitorEnabled() != null && other.getCWEMonitorEnabled().equals(this.getCWEMonitorEnabled()) == false)
return false;
if (other.getOpsItemSNSTopicArn() == null ^ this.getOpsItemSNSTopicArn() == null)
return false;
if (other.getOpsItemSNSTopicArn() != null && other.getOpsItemSNSTopicArn().equals(this.getOpsItemSNSTopicArn()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getAutoConfigEnabled() == null ^ this.getAutoConfigEnabled() == null)
return false;
if (other.getAutoConfigEnabled() != null && other.getAutoConfigEnabled().equals(this.getAutoConfigEnabled()) == false)
return false;
if (other.getAutoCreate() == null ^ this.getAutoCreate() == null)
return false;
if (other.getAutoCreate() != null && other.getAutoCreate().equals(this.getAutoCreate()) == false)
return false;
if (other.getGroupingType() == null ^ this.getGroupingType() == null)
return false;
if (other.getGroupingType() != null && other.getGroupingType().equals(this.getGroupingType()) == false)
return false;
if (other.getAttachMissingPermission() == null ^ this.getAttachMissingPermission() == null)
return false;
if (other.getAttachMissingPermission() != null && other.getAttachMissingPermission().equals(this.getAttachMissingPermission()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getResourceGroupName() == null) ? 0 : getResourceGroupName().hashCode());
hashCode = prime * hashCode + ((getOpsCenterEnabled() == null) ? 0 : getOpsCenterEnabled().hashCode());
hashCode = prime * hashCode + ((getCWEMonitorEnabled() == null) ? 0 : getCWEMonitorEnabled().hashCode());
hashCode = prime * hashCode + ((getOpsItemSNSTopicArn() == null) ? 0 : getOpsItemSNSTopicArn().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getAutoConfigEnabled() == null) ? 0 : getAutoConfigEnabled().hashCode());
hashCode = prime * hashCode + ((getAutoCreate() == null) ? 0 : getAutoCreate().hashCode());
hashCode = prime * hashCode + ((getGroupingType() == null) ? 0 : getGroupingType().hashCode());
hashCode = prime * hashCode + ((getAttachMissingPermission() == null) ? 0 : getAttachMissingPermission().hashCode());
return hashCode;
}
@Override
public CreateApplicationRequest clone() {
return (CreateApplicationRequest) super.clone();
}
}