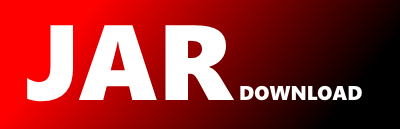
com.amazonaws.services.applicationinsights.AmazonApplicationInsights Maven / Gradle / Ivy
Show all versions of aws-java-sdk-applicationinsights Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.applicationinsights;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.applicationinsights.model.*;
/**
* Interface for accessing Application Insights.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.applicationinsights.AbstractAmazonApplicationInsights} instead.
*
*
* Amazon CloudWatch Application Insights
*
* Amazon CloudWatch Application Insights is a service that helps you detect common problems with your applications. It
* enables you to pinpoint the source of issues in your applications (built with technologies such as Microsoft IIS,
* .NET, and Microsoft SQL Server), by providing key insights into detected problems.
*
*
* After you onboard your application, CloudWatch Application Insights identifies, recommends, and sets up metrics and
* logs. It continuously analyzes and correlates your metrics and logs for unusual behavior to surface actionable
* problems with your application. For example, if your application is slow and unresponsive and leading to HTTP 500
* errors in your Application Load Balancer (ALB), Application Insights informs you that a memory pressure problem with
* your SQL Server database is occurring. It bases this analysis on impactful metrics and log errors.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonApplicationInsights {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "applicationinsights";
/**
*
* Adds a workload to a component. Each component can have at most five workloads.
*
*
* @param addWorkloadRequest
* @return Result of the AddWorkload operation returned by the service.
* @throws ResourceInUseException
* The resource is already created or in use.
* @throws ResourceNotFoundException
* The resource does not exist in the customer account.
* @throws ValidationException
* The parameter is not valid.
* @throws InternalServerException
* The server encountered an internal error and is unable to complete the request.
* @sample AmazonApplicationInsights.AddWorkload
* @see AWS API Documentation
*/
AddWorkloadResult addWorkload(AddWorkloadRequest addWorkloadRequest);
/**
*
* Adds an application that is created from a resource group.
*
*
* @param createApplicationRequest
* @return Result of the CreateApplication operation returned by the service.
* @throws ResourceInUseException
* The resource is already created or in use.
* @throws ResourceNotFoundException
* The resource does not exist in the customer account.
* @throws ValidationException
* The parameter is not valid.
* @throws InternalServerException
* The server encountered an internal error and is unable to complete the request.
* @throws TagsAlreadyExistException
* Tags are already registered for the specified application ARN.
* @throws AccessDeniedException
* User does not have permissions to perform this action.
* @sample AmazonApplicationInsights.CreateApplication
* @see AWS API Documentation
*/
CreateApplicationResult createApplication(CreateApplicationRequest createApplicationRequest);
/**
*
* Creates a custom component by grouping similar standalone instances to monitor.
*
*
* @param createComponentRequest
* @return Result of the CreateComponent operation returned by the service.
* @throws ResourceInUseException
* The resource is already created or in use.
* @throws ResourceNotFoundException
* The resource does not exist in the customer account.
* @throws ValidationException
* The parameter is not valid.
* @throws InternalServerException
* The server encountered an internal error and is unable to complete the request.
* @sample AmazonApplicationInsights.CreateComponent
* @see AWS API Documentation
*/
CreateComponentResult createComponent(CreateComponentRequest createComponentRequest);
/**
*
* Adds an log pattern to a LogPatternSet
.
*
*
* @param createLogPatternRequest
* @return Result of the CreateLogPattern operation returned by the service.
* @throws ResourceInUseException
* The resource is already created or in use.
* @throws ResourceNotFoundException
* The resource does not exist in the customer account.
* @throws ValidationException
* The parameter is not valid.
* @throws InternalServerException
* The server encountered an internal error and is unable to complete the request.
* @sample AmazonApplicationInsights.CreateLogPattern
* @see AWS API Documentation
*/
CreateLogPatternResult createLogPattern(CreateLogPatternRequest createLogPatternRequest);
/**
*
* Removes the specified application from monitoring. Does not delete the application.
*
*
* @param deleteApplicationRequest
* @return Result of the DeleteApplication operation returned by the service.
* @throws ResourceNotFoundException
* The resource does not exist in the customer account.
* @throws ValidationException
* The parameter is not valid.
* @throws BadRequestException
* The request is not understood by the server.
* @throws InternalServerException
* The server encountered an internal error and is unable to complete the request.
* @sample AmazonApplicationInsights.DeleteApplication
* @see AWS API Documentation
*/
DeleteApplicationResult deleteApplication(DeleteApplicationRequest deleteApplicationRequest);
/**
*
* Ungroups a custom component. When you ungroup custom components, all applicable monitors that are set up for the
* component are removed and the instances revert to their standalone status.
*
*
* @param deleteComponentRequest
* @return Result of the DeleteComponent operation returned by the service.
* @throws ResourceNotFoundException
* The resource does not exist in the customer account.
* @throws ValidationException
* The parameter is not valid.
* @throws InternalServerException
* The server encountered an internal error and is unable to complete the request.
* @sample AmazonApplicationInsights.DeleteComponent
* @see AWS API Documentation
*/
DeleteComponentResult deleteComponent(DeleteComponentRequest deleteComponentRequest);
/**
*
* Removes the specified log pattern from a LogPatternSet
.
*
*
* @param deleteLogPatternRequest
* @return Result of the DeleteLogPattern operation returned by the service.
* @throws ResourceNotFoundException
* The resource does not exist in the customer account.
* @throws ValidationException
* The parameter is not valid.
* @throws BadRequestException
* The request is not understood by the server.
* @throws InternalServerException
* The server encountered an internal error and is unable to complete the request.
* @sample AmazonApplicationInsights.DeleteLogPattern
* @see AWS API Documentation
*/
DeleteLogPatternResult deleteLogPattern(DeleteLogPatternRequest deleteLogPatternRequest);
/**
*
* Describes the application.
*
*
* @param describeApplicationRequest
* @return Result of the DescribeApplication operation returned by the service.
* @throws ResourceNotFoundException
* The resource does not exist in the customer account.
* @throws ValidationException
* The parameter is not valid.
* @throws InternalServerException
* The server encountered an internal error and is unable to complete the request.
* @sample AmazonApplicationInsights.DescribeApplication
* @see AWS API Documentation
*/
DescribeApplicationResult describeApplication(DescribeApplicationRequest describeApplicationRequest);
/**
*
* Describes a component and lists the resources that are grouped together in a component.
*
*
* @param describeComponentRequest
* @return Result of the DescribeComponent operation returned by the service.
* @throws ResourceNotFoundException
* The resource does not exist in the customer account.
* @throws ValidationException
* The parameter is not valid.
* @throws InternalServerException
* The server encountered an internal error and is unable to complete the request.
* @sample AmazonApplicationInsights.DescribeComponent
* @see AWS API Documentation
*/
DescribeComponentResult describeComponent(DescribeComponentRequest describeComponentRequest);
/**
*
* Describes the monitoring configuration of the component.
*
*
* @param describeComponentConfigurationRequest
* @return Result of the DescribeComponentConfiguration operation returned by the service.
* @throws ResourceNotFoundException
* The resource does not exist in the customer account.
* @throws ValidationException
* The parameter is not valid.
* @throws InternalServerException
* The server encountered an internal error and is unable to complete the request.
* @sample AmazonApplicationInsights.DescribeComponentConfiguration
* @see AWS API Documentation
*/
DescribeComponentConfigurationResult describeComponentConfiguration(DescribeComponentConfigurationRequest describeComponentConfigurationRequest);
/**
*
* Describes the recommended monitoring configuration of the component.
*
*
* @param describeComponentConfigurationRecommendationRequest
* @return Result of the DescribeComponentConfigurationRecommendation operation returned by the service.
* @throws ResourceNotFoundException
* The resource does not exist in the customer account.
* @throws ValidationException
* The parameter is not valid.
* @throws InternalServerException
* The server encountered an internal error and is unable to complete the request.
* @sample AmazonApplicationInsights.DescribeComponentConfigurationRecommendation
* @see AWS API Documentation
*/
DescribeComponentConfigurationRecommendationResult describeComponentConfigurationRecommendation(
DescribeComponentConfigurationRecommendationRequest describeComponentConfigurationRecommendationRequest);
/**
*
* Describe a specific log pattern from a LogPatternSet
.
*
*
* @param describeLogPatternRequest
* @return Result of the DescribeLogPattern operation returned by the service.
* @throws ResourceNotFoundException
* The resource does not exist in the customer account.
* @throws ValidationException
* The parameter is not valid.
* @throws InternalServerException
* The server encountered an internal error and is unable to complete the request.
* @sample AmazonApplicationInsights.DescribeLogPattern
* @see AWS API Documentation
*/
DescribeLogPatternResult describeLogPattern(DescribeLogPatternRequest describeLogPatternRequest);
/**
*
* Describes an anomaly or error with the application.
*
*
* @param describeObservationRequest
* @return Result of the DescribeObservation operation returned by the service.
* @throws InternalServerException
* The server encountered an internal error and is unable to complete the request.
* @throws ValidationException
* The parameter is not valid.
* @throws ResourceNotFoundException
* The resource does not exist in the customer account.
* @sample AmazonApplicationInsights.DescribeObservation
* @see AWS API Documentation
*/
DescribeObservationResult describeObservation(DescribeObservationRequest describeObservationRequest);
/**
*
* Describes an application problem.
*
*
* @param describeProblemRequest
* @return Result of the DescribeProblem operation returned by the service.
* @throws InternalServerException
* The server encountered an internal error and is unable to complete the request.
* @throws ValidationException
* The parameter is not valid.
* @throws ResourceNotFoundException
* The resource does not exist in the customer account.
* @sample AmazonApplicationInsights.DescribeProblem
* @see AWS API Documentation
*/
DescribeProblemResult describeProblem(DescribeProblemRequest describeProblemRequest);
/**
*
* Describes the anomalies or errors associated with the problem.
*
*
* @param describeProblemObservationsRequest
* @return Result of the DescribeProblemObservations operation returned by the service.
* @throws InternalServerException
* The server encountered an internal error and is unable to complete the request.
* @throws ValidationException
* The parameter is not valid.
* @throws ResourceNotFoundException
* The resource does not exist in the customer account.
* @sample AmazonApplicationInsights.DescribeProblemObservations
* @see AWS API Documentation
*/
DescribeProblemObservationsResult describeProblemObservations(DescribeProblemObservationsRequest describeProblemObservationsRequest);
/**
*
* Describes a workload and its configuration.
*
*
* @param describeWorkloadRequest
* @return Result of the DescribeWorkload operation returned by the service.
* @throws ResourceNotFoundException
* The resource does not exist in the customer account.
* @throws ValidationException
* The parameter is not valid.
* @throws InternalServerException
* The server encountered an internal error and is unable to complete the request.
* @sample AmazonApplicationInsights.DescribeWorkload
* @see AWS API Documentation
*/
DescribeWorkloadResult describeWorkload(DescribeWorkloadRequest describeWorkloadRequest);
/**
*
* Lists the IDs of the applications that you are monitoring.
*
*
* @param listApplicationsRequest
* @return Result of the ListApplications operation returned by the service.
* @throws ValidationException
* The parameter is not valid.
* @throws InternalServerException
* The server encountered an internal error and is unable to complete the request.
* @sample AmazonApplicationInsights.ListApplications
* @see AWS API Documentation
*/
ListApplicationsResult listApplications(ListApplicationsRequest listApplicationsRequest);
/**
*
* Lists the auto-grouped, standalone, and custom components of the application.
*
*
* @param listComponentsRequest
* @return Result of the ListComponents operation returned by the service.
* @throws ResourceNotFoundException
* The resource does not exist in the customer account.
* @throws ValidationException
* The parameter is not valid.
* @throws InternalServerException
* The server encountered an internal error and is unable to complete the request.
* @sample AmazonApplicationInsights.ListComponents
* @see AWS API Documentation
*/
ListComponentsResult listComponents(ListComponentsRequest listComponentsRequest);
/**
*
* Lists the INFO, WARN, and ERROR events for periodic configuration updates performed by Application Insights.
* Examples of events represented are:
*
*
* -
*
* INFO: creating a new alarm or updating an alarm threshold.
*
*
* -
*
* WARN: alarm not created due to insufficient data points used to predict thresholds.
*
*
* -
*
* ERROR: alarm not created due to permission errors or exceeding quotas.
*
*
*
*
* @param listConfigurationHistoryRequest
* @return Result of the ListConfigurationHistory operation returned by the service.
* @throws ValidationException
* The parameter is not valid.
* @throws ResourceNotFoundException
* The resource does not exist in the customer account.
* @throws InternalServerException
* The server encountered an internal error and is unable to complete the request.
* @sample AmazonApplicationInsights.ListConfigurationHistory
* @see AWS API Documentation
*/
ListConfigurationHistoryResult listConfigurationHistory(ListConfigurationHistoryRequest listConfigurationHistoryRequest);
/**
*
* Lists the log pattern sets in the specific application.
*
*
* @param listLogPatternSetsRequest
* @return Result of the ListLogPatternSets operation returned by the service.
* @throws ResourceNotFoundException
* The resource does not exist in the customer account.
* @throws ValidationException
* The parameter is not valid.
* @throws InternalServerException
* The server encountered an internal error and is unable to complete the request.
* @sample AmazonApplicationInsights.ListLogPatternSets
* @see AWS API Documentation
*/
ListLogPatternSetsResult listLogPatternSets(ListLogPatternSetsRequest listLogPatternSetsRequest);
/**
*
* Lists the log patterns in the specific log LogPatternSet
.
*
*
* @param listLogPatternsRequest
* @return Result of the ListLogPatterns operation returned by the service.
* @throws ResourceNotFoundException
* The resource does not exist in the customer account.
* @throws ValidationException
* The parameter is not valid.
* @throws InternalServerException
* The server encountered an internal error and is unable to complete the request.
* @sample AmazonApplicationInsights.ListLogPatterns
* @see AWS API Documentation
*/
ListLogPatternsResult listLogPatterns(ListLogPatternsRequest listLogPatternsRequest);
/**
*
* Lists the problems with your application.
*
*
* @param listProblemsRequest
* @return Result of the ListProblems operation returned by the service.
* @throws ValidationException
* The parameter is not valid.
* @throws ResourceNotFoundException
* The resource does not exist in the customer account.
* @throws InternalServerException
* The server encountered an internal error and is unable to complete the request.
* @sample AmazonApplicationInsights.ListProblems
* @see AWS API Documentation
*/
ListProblemsResult listProblems(ListProblemsRequest listProblemsRequest);
/**
*
* Retrieve a list of the tags (keys and values) that are associated with a specified application. A tag is a
* label that you optionally define and associate with an application. Each tag consists of a required tag
* key and an optional associated tag value. A tag key is a general label that acts as a category for
* more specific tag values. A tag value acts as a descriptor within a tag key.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws ResourceNotFoundException
* The resource does not exist in the customer account.
* @throws ValidationException
* The parameter is not valid.
* @sample AmazonApplicationInsights.ListTagsForResource
* @see AWS API Documentation
*/
ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Lists the workloads that are configured on a given component.
*
*
* @param listWorkloadsRequest
* @return Result of the ListWorkloads operation returned by the service.
* @throws ResourceNotFoundException
* The resource does not exist in the customer account.
* @throws ValidationException
* The parameter is not valid.
* @throws InternalServerException
* The server encountered an internal error and is unable to complete the request.
* @sample AmazonApplicationInsights.ListWorkloads
* @see AWS API Documentation
*/
ListWorkloadsResult listWorkloads(ListWorkloadsRequest listWorkloadsRequest);
/**
*
* Remove workload from a component.
*
*
* @param removeWorkloadRequest
* @return Result of the RemoveWorkload operation returned by the service.
* @throws ResourceNotFoundException
* The resource does not exist in the customer account.
* @throws ValidationException
* The parameter is not valid.
* @throws InternalServerException
* The server encountered an internal error and is unable to complete the request.
* @sample AmazonApplicationInsights.RemoveWorkload
* @see AWS API Documentation
*/
RemoveWorkloadResult removeWorkload(RemoveWorkloadRequest removeWorkloadRequest);
/**
*
* Add one or more tags (keys and values) to a specified application. A tag is a label that you optionally
* define and associate with an application. Tags can help you categorize and manage application in different ways,
* such as by purpose, owner, environment, or other criteria.
*
*
* Each tag consists of a required tag key and an associated tag value, both of which you define. A
* tag key is a general label that acts as a category for more specific tag values. A tag value acts as a descriptor
* within a tag key.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws ResourceNotFoundException
* The resource does not exist in the customer account.
* @throws TooManyTagsException
* The number of the provided tags is beyond the limit, or the number of total tags you are trying to attach
* to the specified resource exceeds the limit.
* @throws ValidationException
* The parameter is not valid.
* @sample AmazonApplicationInsights.TagResource
* @see AWS API Documentation
*/
TagResourceResult tagResource(TagResourceRequest tagResourceRequest);
/**
*
* Remove one or more tags (keys and values) from a specified application.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws ResourceNotFoundException
* The resource does not exist in the customer account.
* @throws ValidationException
* The parameter is not valid.
* @sample AmazonApplicationInsights.UntagResource
* @see AWS API Documentation
*/
UntagResourceResult untagResource(UntagResourceRequest untagResourceRequest);
/**
*
* Updates the application.
*
*
* @param updateApplicationRequest
* @return Result of the UpdateApplication operation returned by the service.
* @throws InternalServerException
* The server encountered an internal error and is unable to complete the request.
* @throws ResourceNotFoundException
* The resource does not exist in the customer account.
* @throws ValidationException
* The parameter is not valid.
* @sample AmazonApplicationInsights.UpdateApplication
* @see AWS API Documentation
*/
UpdateApplicationResult updateApplication(UpdateApplicationRequest updateApplicationRequest);
/**
*
* Updates the custom component name and/or the list of resources that make up the component.
*
*
* @param updateComponentRequest
* @return Result of the UpdateComponent operation returned by the service.
* @throws ResourceInUseException
* The resource is already created or in use.
* @throws ResourceNotFoundException
* The resource does not exist in the customer account.
* @throws ValidationException
* The parameter is not valid.
* @throws InternalServerException
* The server encountered an internal error and is unable to complete the request.
* @sample AmazonApplicationInsights.UpdateComponent
* @see AWS API Documentation
*/
UpdateComponentResult updateComponent(UpdateComponentRequest updateComponentRequest);
/**
*
* Updates the monitoring configurations for the component. The configuration input parameter is an escaped JSON of
* the configuration and should match the schema of what is returned by
* DescribeComponentConfigurationRecommendation
.
*
*
* @param updateComponentConfigurationRequest
* @return Result of the UpdateComponentConfiguration operation returned by the service.
* @throws ResourceNotFoundException
* The resource does not exist in the customer account.
* @throws ValidationException
* The parameter is not valid.
* @throws InternalServerException
* The server encountered an internal error and is unable to complete the request.
* @throws ResourceInUseException
* The resource is already created or in use.
* @sample AmazonApplicationInsights.UpdateComponentConfiguration
* @see AWS API Documentation
*/
UpdateComponentConfigurationResult updateComponentConfiguration(UpdateComponentConfigurationRequest updateComponentConfigurationRequest);
/**
*
* Adds a log pattern to a LogPatternSet
.
*
*
* @param updateLogPatternRequest
* @return Result of the UpdateLogPattern operation returned by the service.
* @throws ResourceInUseException
* The resource is already created or in use.
* @throws ResourceNotFoundException
* The resource does not exist in the customer account.
* @throws ValidationException
* The parameter is not valid.
* @throws InternalServerException
* The server encountered an internal error and is unable to complete the request.
* @sample AmazonApplicationInsights.UpdateLogPattern
* @see AWS API Documentation
*/
UpdateLogPatternResult updateLogPattern(UpdateLogPatternRequest updateLogPatternRequest);
/**
*
* Updates the visibility of the problem or specifies the problem as RESOLVED
.
*
*
* @param updateProblemRequest
* @return Result of the UpdateProblem operation returned by the service.
* @throws InternalServerException
* The server encountered an internal error and is unable to complete the request.
* @throws ValidationException
* The parameter is not valid.
* @throws ResourceNotFoundException
* The resource does not exist in the customer account.
* @sample AmazonApplicationInsights.UpdateProblem
* @see AWS API Documentation
*/
UpdateProblemResult updateProblem(UpdateProblemRequest updateProblemRequest);
/**
*
* Adds a workload to a component. Each component can have at most five workloads.
*
*
* @param updateWorkloadRequest
* @return Result of the UpdateWorkload operation returned by the service.
* @throws ResourceNotFoundException
* The resource does not exist in the customer account.
* @throws ValidationException
* The parameter is not valid.
* @throws InternalServerException
* The server encountered an internal error and is unable to complete the request.
* @sample AmazonApplicationInsights.UpdateWorkload
* @see AWS API Documentation
*/
UpdateWorkloadResult updateWorkload(UpdateWorkloadRequest updateWorkloadRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}