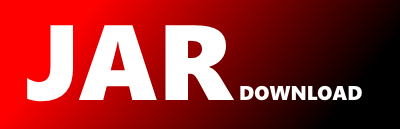
com.amazonaws.services.applicationinsights.AmazonApplicationInsightsAsync Maven / Gradle / Ivy
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.applicationinsights;
import javax.annotation.Generated;
import com.amazonaws.services.applicationinsights.model.*;
/**
* Interface for accessing Application Insights asynchronously. Each asynchronous method will return a Java Future
* object representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.applicationinsights.AbstractAmazonApplicationInsightsAsync} instead.
*
*
* Amazon CloudWatch Application Insights
*
* Amazon CloudWatch Application Insights is a service that helps you detect common problems with your applications. It
* enables you to pinpoint the source of issues in your applications (built with technologies such as Microsoft IIS,
* .NET, and Microsoft SQL Server), by providing key insights into detected problems.
*
*
* After you onboard your application, CloudWatch Application Insights identifies, recommends, and sets up metrics and
* logs. It continuously analyzes and correlates your metrics and logs for unusual behavior to surface actionable
* problems with your application. For example, if your application is slow and unresponsive and leading to HTTP 500
* errors in your Application Load Balancer (ALB), Application Insights informs you that a memory pressure problem with
* your SQL Server database is occurring. It bases this analysis on impactful metrics and log errors.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonApplicationInsightsAsync extends AmazonApplicationInsights {
/**
*
* Adds a workload to a component. Each component can have at most five workloads.
*
*
* @param addWorkloadRequest
* @return A Java Future containing the result of the AddWorkload operation returned by the service.
* @sample AmazonApplicationInsightsAsync.AddWorkload
* @see AWS API Documentation
*/
java.util.concurrent.Future addWorkloadAsync(AddWorkloadRequest addWorkloadRequest);
/**
*
* Adds a workload to a component. Each component can have at most five workloads.
*
*
* @param addWorkloadRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AddWorkload operation returned by the service.
* @sample AmazonApplicationInsightsAsyncHandler.AddWorkload
* @see AWS API Documentation
*/
java.util.concurrent.Future addWorkloadAsync(AddWorkloadRequest addWorkloadRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds an application that is created from a resource group.
*
*
* @param createApplicationRequest
* @return A Java Future containing the result of the CreateApplication operation returned by the service.
* @sample AmazonApplicationInsightsAsync.CreateApplication
* @see AWS API Documentation
*/
java.util.concurrent.Future createApplicationAsync(CreateApplicationRequest createApplicationRequest);
/**
*
* Adds an application that is created from a resource group.
*
*
* @param createApplicationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateApplication operation returned by the service.
* @sample AmazonApplicationInsightsAsyncHandler.CreateApplication
* @see AWS API Documentation
*/
java.util.concurrent.Future createApplicationAsync(CreateApplicationRequest createApplicationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a custom component by grouping similar standalone instances to monitor.
*
*
* @param createComponentRequest
* @return A Java Future containing the result of the CreateComponent operation returned by the service.
* @sample AmazonApplicationInsightsAsync.CreateComponent
* @see AWS API Documentation
*/
java.util.concurrent.Future createComponentAsync(CreateComponentRequest createComponentRequest);
/**
*
* Creates a custom component by grouping similar standalone instances to monitor.
*
*
* @param createComponentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateComponent operation returned by the service.
* @sample AmazonApplicationInsightsAsyncHandler.CreateComponent
* @see AWS API Documentation
*/
java.util.concurrent.Future createComponentAsync(CreateComponentRequest createComponentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds an log pattern to a LogPatternSet
.
*
*
* @param createLogPatternRequest
* @return A Java Future containing the result of the CreateLogPattern operation returned by the service.
* @sample AmazonApplicationInsightsAsync.CreateLogPattern
* @see AWS API Documentation
*/
java.util.concurrent.Future createLogPatternAsync(CreateLogPatternRequest createLogPatternRequest);
/**
*
* Adds an log pattern to a LogPatternSet
.
*
*
* @param createLogPatternRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateLogPattern operation returned by the service.
* @sample AmazonApplicationInsightsAsyncHandler.CreateLogPattern
* @see AWS API Documentation
*/
java.util.concurrent.Future createLogPatternAsync(CreateLogPatternRequest createLogPatternRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes the specified application from monitoring. Does not delete the application.
*
*
* @param deleteApplicationRequest
* @return A Java Future containing the result of the DeleteApplication operation returned by the service.
* @sample AmazonApplicationInsightsAsync.DeleteApplication
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteApplicationAsync(DeleteApplicationRequest deleteApplicationRequest);
/**
*
* Removes the specified application from monitoring. Does not delete the application.
*
*
* @param deleteApplicationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteApplication operation returned by the service.
* @sample AmazonApplicationInsightsAsyncHandler.DeleteApplication
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteApplicationAsync(DeleteApplicationRequest deleteApplicationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Ungroups a custom component. When you ungroup custom components, all applicable monitors that are set up for the
* component are removed and the instances revert to their standalone status.
*
*
* @param deleteComponentRequest
* @return A Java Future containing the result of the DeleteComponent operation returned by the service.
* @sample AmazonApplicationInsightsAsync.DeleteComponent
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteComponentAsync(DeleteComponentRequest deleteComponentRequest);
/**
*
* Ungroups a custom component. When you ungroup custom components, all applicable monitors that are set up for the
* component are removed and the instances revert to their standalone status.
*
*
* @param deleteComponentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteComponent operation returned by the service.
* @sample AmazonApplicationInsightsAsyncHandler.DeleteComponent
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteComponentAsync(DeleteComponentRequest deleteComponentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes the specified log pattern from a LogPatternSet
.
*
*
* @param deleteLogPatternRequest
* @return A Java Future containing the result of the DeleteLogPattern operation returned by the service.
* @sample AmazonApplicationInsightsAsync.DeleteLogPattern
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteLogPatternAsync(DeleteLogPatternRequest deleteLogPatternRequest);
/**
*
* Removes the specified log pattern from a LogPatternSet
.
*
*
* @param deleteLogPatternRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteLogPattern operation returned by the service.
* @sample AmazonApplicationInsightsAsyncHandler.DeleteLogPattern
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteLogPatternAsync(DeleteLogPatternRequest deleteLogPatternRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the application.
*
*
* @param describeApplicationRequest
* @return A Java Future containing the result of the DescribeApplication operation returned by the service.
* @sample AmazonApplicationInsightsAsync.DescribeApplication
* @see AWS API Documentation
*/
java.util.concurrent.Future describeApplicationAsync(DescribeApplicationRequest describeApplicationRequest);
/**
*
* Describes the application.
*
*
* @param describeApplicationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeApplication operation returned by the service.
* @sample AmazonApplicationInsightsAsyncHandler.DescribeApplication
* @see AWS API Documentation
*/
java.util.concurrent.Future describeApplicationAsync(DescribeApplicationRequest describeApplicationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes a component and lists the resources that are grouped together in a component.
*
*
* @param describeComponentRequest
* @return A Java Future containing the result of the DescribeComponent operation returned by the service.
* @sample AmazonApplicationInsightsAsync.DescribeComponent
* @see AWS API Documentation
*/
java.util.concurrent.Future describeComponentAsync(DescribeComponentRequest describeComponentRequest);
/**
*
* Describes a component and lists the resources that are grouped together in a component.
*
*
* @param describeComponentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeComponent operation returned by the service.
* @sample AmazonApplicationInsightsAsyncHandler.DescribeComponent
* @see AWS API Documentation
*/
java.util.concurrent.Future describeComponentAsync(DescribeComponentRequest describeComponentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the monitoring configuration of the component.
*
*
* @param describeComponentConfigurationRequest
* @return A Java Future containing the result of the DescribeComponentConfiguration operation returned by the
* service.
* @sample AmazonApplicationInsightsAsync.DescribeComponentConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future describeComponentConfigurationAsync(
DescribeComponentConfigurationRequest describeComponentConfigurationRequest);
/**
*
* Describes the monitoring configuration of the component.
*
*
* @param describeComponentConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeComponentConfiguration operation returned by the
* service.
* @sample AmazonApplicationInsightsAsyncHandler.DescribeComponentConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future describeComponentConfigurationAsync(
DescribeComponentConfigurationRequest describeComponentConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the recommended monitoring configuration of the component.
*
*
* @param describeComponentConfigurationRecommendationRequest
* @return A Java Future containing the result of the DescribeComponentConfigurationRecommendation operation
* returned by the service.
* @sample AmazonApplicationInsightsAsync.DescribeComponentConfigurationRecommendation
* @see AWS API Documentation
*/
java.util.concurrent.Future describeComponentConfigurationRecommendationAsync(
DescribeComponentConfigurationRecommendationRequest describeComponentConfigurationRecommendationRequest);
/**
*
* Describes the recommended monitoring configuration of the component.
*
*
* @param describeComponentConfigurationRecommendationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeComponentConfigurationRecommendation operation
* returned by the service.
* @sample AmazonApplicationInsightsAsyncHandler.DescribeComponentConfigurationRecommendation
* @see AWS API Documentation
*/
java.util.concurrent.Future describeComponentConfigurationRecommendationAsync(
DescribeComponentConfigurationRecommendationRequest describeComponentConfigurationRecommendationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describe a specific log pattern from a LogPatternSet
.
*
*
* @param describeLogPatternRequest
* @return A Java Future containing the result of the DescribeLogPattern operation returned by the service.
* @sample AmazonApplicationInsightsAsync.DescribeLogPattern
* @see AWS API Documentation
*/
java.util.concurrent.Future describeLogPatternAsync(DescribeLogPatternRequest describeLogPatternRequest);
/**
*
* Describe a specific log pattern from a LogPatternSet
.
*
*
* @param describeLogPatternRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeLogPattern operation returned by the service.
* @sample AmazonApplicationInsightsAsyncHandler.DescribeLogPattern
* @see AWS API Documentation
*/
java.util.concurrent.Future describeLogPatternAsync(DescribeLogPatternRequest describeLogPatternRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes an anomaly or error with the application.
*
*
* @param describeObservationRequest
* @return A Java Future containing the result of the DescribeObservation operation returned by the service.
* @sample AmazonApplicationInsightsAsync.DescribeObservation
* @see AWS API Documentation
*/
java.util.concurrent.Future describeObservationAsync(DescribeObservationRequest describeObservationRequest);
/**
*
* Describes an anomaly or error with the application.
*
*
* @param describeObservationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeObservation operation returned by the service.
* @sample AmazonApplicationInsightsAsyncHandler.DescribeObservation
* @see AWS API Documentation
*/
java.util.concurrent.Future describeObservationAsync(DescribeObservationRequest describeObservationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes an application problem.
*
*
* @param describeProblemRequest
* @return A Java Future containing the result of the DescribeProblem operation returned by the service.
* @sample AmazonApplicationInsightsAsync.DescribeProblem
* @see AWS API Documentation
*/
java.util.concurrent.Future describeProblemAsync(DescribeProblemRequest describeProblemRequest);
/**
*
* Describes an application problem.
*
*
* @param describeProblemRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeProblem operation returned by the service.
* @sample AmazonApplicationInsightsAsyncHandler.DescribeProblem
* @see AWS API Documentation
*/
java.util.concurrent.Future describeProblemAsync(DescribeProblemRequest describeProblemRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the anomalies or errors associated with the problem.
*
*
* @param describeProblemObservationsRequest
* @return A Java Future containing the result of the DescribeProblemObservations operation returned by the service.
* @sample AmazonApplicationInsightsAsync.DescribeProblemObservations
* @see AWS API Documentation
*/
java.util.concurrent.Future describeProblemObservationsAsync(
DescribeProblemObservationsRequest describeProblemObservationsRequest);
/**
*
* Describes the anomalies or errors associated with the problem.
*
*
* @param describeProblemObservationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeProblemObservations operation returned by the service.
* @sample AmazonApplicationInsightsAsyncHandler.DescribeProblemObservations
* @see AWS API Documentation
*/
java.util.concurrent.Future describeProblemObservationsAsync(
DescribeProblemObservationsRequest describeProblemObservationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes a workload and its configuration.
*
*
* @param describeWorkloadRequest
* @return A Java Future containing the result of the DescribeWorkload operation returned by the service.
* @sample AmazonApplicationInsightsAsync.DescribeWorkload
* @see AWS API Documentation
*/
java.util.concurrent.Future describeWorkloadAsync(DescribeWorkloadRequest describeWorkloadRequest);
/**
*
* Describes a workload and its configuration.
*
*
* @param describeWorkloadRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeWorkload operation returned by the service.
* @sample AmazonApplicationInsightsAsyncHandler.DescribeWorkload
* @see AWS API Documentation
*/
java.util.concurrent.Future describeWorkloadAsync(DescribeWorkloadRequest describeWorkloadRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the IDs of the applications that you are monitoring.
*
*
* @param listApplicationsRequest
* @return A Java Future containing the result of the ListApplications operation returned by the service.
* @sample AmazonApplicationInsightsAsync.ListApplications
* @see AWS API Documentation
*/
java.util.concurrent.Future listApplicationsAsync(ListApplicationsRequest listApplicationsRequest);
/**
*
* Lists the IDs of the applications that you are monitoring.
*
*
* @param listApplicationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListApplications operation returned by the service.
* @sample AmazonApplicationInsightsAsyncHandler.ListApplications
* @see AWS API Documentation
*/
java.util.concurrent.Future listApplicationsAsync(ListApplicationsRequest listApplicationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the auto-grouped, standalone, and custom components of the application.
*
*
* @param listComponentsRequest
* @return A Java Future containing the result of the ListComponents operation returned by the service.
* @sample AmazonApplicationInsightsAsync.ListComponents
* @see AWS API Documentation
*/
java.util.concurrent.Future listComponentsAsync(ListComponentsRequest listComponentsRequest);
/**
*
* Lists the auto-grouped, standalone, and custom components of the application.
*
*
* @param listComponentsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListComponents operation returned by the service.
* @sample AmazonApplicationInsightsAsyncHandler.ListComponents
* @see AWS API Documentation
*/
java.util.concurrent.Future listComponentsAsync(ListComponentsRequest listComponentsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the INFO, WARN, and ERROR events for periodic configuration updates performed by Application Insights.
* Examples of events represented are:
*
*
* -
*
* INFO: creating a new alarm or updating an alarm threshold.
*
*
* -
*
* WARN: alarm not created due to insufficient data points used to predict thresholds.
*
*
* -
*
* ERROR: alarm not created due to permission errors or exceeding quotas.
*
*
*
*
* @param listConfigurationHistoryRequest
* @return A Java Future containing the result of the ListConfigurationHistory operation returned by the service.
* @sample AmazonApplicationInsightsAsync.ListConfigurationHistory
* @see AWS API Documentation
*/
java.util.concurrent.Future listConfigurationHistoryAsync(ListConfigurationHistoryRequest listConfigurationHistoryRequest);
/**
*
* Lists the INFO, WARN, and ERROR events for periodic configuration updates performed by Application Insights.
* Examples of events represented are:
*
*
* -
*
* INFO: creating a new alarm or updating an alarm threshold.
*
*
* -
*
* WARN: alarm not created due to insufficient data points used to predict thresholds.
*
*
* -
*
* ERROR: alarm not created due to permission errors or exceeding quotas.
*
*
*
*
* @param listConfigurationHistoryRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListConfigurationHistory operation returned by the service.
* @sample AmazonApplicationInsightsAsyncHandler.ListConfigurationHistory
* @see AWS API Documentation
*/
java.util.concurrent.Future listConfigurationHistoryAsync(ListConfigurationHistoryRequest listConfigurationHistoryRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the log pattern sets in the specific application.
*
*
* @param listLogPatternSetsRequest
* @return A Java Future containing the result of the ListLogPatternSets operation returned by the service.
* @sample AmazonApplicationInsightsAsync.ListLogPatternSets
* @see AWS API Documentation
*/
java.util.concurrent.Future listLogPatternSetsAsync(ListLogPatternSetsRequest listLogPatternSetsRequest);
/**
*
* Lists the log pattern sets in the specific application.
*
*
* @param listLogPatternSetsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListLogPatternSets operation returned by the service.
* @sample AmazonApplicationInsightsAsyncHandler.ListLogPatternSets
* @see AWS API Documentation
*/
java.util.concurrent.Future listLogPatternSetsAsync(ListLogPatternSetsRequest listLogPatternSetsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the log patterns in the specific log LogPatternSet
.
*
*
* @param listLogPatternsRequest
* @return A Java Future containing the result of the ListLogPatterns operation returned by the service.
* @sample AmazonApplicationInsightsAsync.ListLogPatterns
* @see AWS API Documentation
*/
java.util.concurrent.Future listLogPatternsAsync(ListLogPatternsRequest listLogPatternsRequest);
/**
*
* Lists the log patterns in the specific log LogPatternSet
.
*
*
* @param listLogPatternsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListLogPatterns operation returned by the service.
* @sample AmazonApplicationInsightsAsyncHandler.ListLogPatterns
* @see AWS API Documentation
*/
java.util.concurrent.Future listLogPatternsAsync(ListLogPatternsRequest listLogPatternsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the problems with your application.
*
*
* @param listProblemsRequest
* @return A Java Future containing the result of the ListProblems operation returned by the service.
* @sample AmazonApplicationInsightsAsync.ListProblems
* @see AWS API Documentation
*/
java.util.concurrent.Future listProblemsAsync(ListProblemsRequest listProblemsRequest);
/**
*
* Lists the problems with your application.
*
*
* @param listProblemsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListProblems operation returned by the service.
* @sample AmazonApplicationInsightsAsyncHandler.ListProblems
* @see AWS API Documentation
*/
java.util.concurrent.Future listProblemsAsync(ListProblemsRequest listProblemsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieve a list of the tags (keys and values) that are associated with a specified application. A tag is a
* label that you optionally define and associate with an application. Each tag consists of a required tag
* key and an optional associated tag value. A tag key is a general label that acts as a category for
* more specific tag values. A tag value acts as a descriptor within a tag key.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonApplicationInsightsAsync.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Retrieve a list of the tags (keys and values) that are associated with a specified application. A tag is a
* label that you optionally define and associate with an application. Each tag consists of a required tag
* key and an optional associated tag value. A tag key is a general label that acts as a category for
* more specific tag values. A tag value acts as a descriptor within a tag key.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonApplicationInsightsAsyncHandler.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the workloads that are configured on a given component.
*
*
* @param listWorkloadsRequest
* @return A Java Future containing the result of the ListWorkloads operation returned by the service.
* @sample AmazonApplicationInsightsAsync.ListWorkloads
* @see AWS API Documentation
*/
java.util.concurrent.Future listWorkloadsAsync(ListWorkloadsRequest listWorkloadsRequest);
/**
*
* Lists the workloads that are configured on a given component.
*
*
* @param listWorkloadsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListWorkloads operation returned by the service.
* @sample AmazonApplicationInsightsAsyncHandler.ListWorkloads
* @see AWS API Documentation
*/
java.util.concurrent.Future listWorkloadsAsync(ListWorkloadsRequest listWorkloadsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Remove workload from a component.
*
*
* @param removeWorkloadRequest
* @return A Java Future containing the result of the RemoveWorkload operation returned by the service.
* @sample AmazonApplicationInsightsAsync.RemoveWorkload
* @see AWS API Documentation
*/
java.util.concurrent.Future removeWorkloadAsync(RemoveWorkloadRequest removeWorkloadRequest);
/**
*
* Remove workload from a component.
*
*
* @param removeWorkloadRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RemoveWorkload operation returned by the service.
* @sample AmazonApplicationInsightsAsyncHandler.RemoveWorkload
* @see AWS API Documentation
*/
java.util.concurrent.Future removeWorkloadAsync(RemoveWorkloadRequest removeWorkloadRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Add one or more tags (keys and values) to a specified application. A tag is a label that you optionally
* define and associate with an application. Tags can help you categorize and manage application in different ways,
* such as by purpose, owner, environment, or other criteria.
*
*
* Each tag consists of a required tag key and an associated tag value, both of which you define. A
* tag key is a general label that acts as a category for more specific tag values. A tag value acts as a descriptor
* within a tag key.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonApplicationInsightsAsync.TagResource
* @see AWS API Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Add one or more tags (keys and values) to a specified application. A tag is a label that you optionally
* define and associate with an application. Tags can help you categorize and manage application in different ways,
* such as by purpose, owner, environment, or other criteria.
*
*
* Each tag consists of a required tag key and an associated tag value, both of which you define. A
* tag key is a general label that acts as a category for more specific tag values. A tag value acts as a descriptor
* within a tag key.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonApplicationInsightsAsyncHandler.TagResource
* @see AWS API Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Remove one or more tags (keys and values) from a specified application.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonApplicationInsightsAsync.UntagResource
* @see AWS API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Remove one or more tags (keys and values) from a specified application.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonApplicationInsightsAsyncHandler.UntagResource
* @see AWS API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the application.
*
*
* @param updateApplicationRequest
* @return A Java Future containing the result of the UpdateApplication operation returned by the service.
* @sample AmazonApplicationInsightsAsync.UpdateApplication
* @see AWS API Documentation
*/
java.util.concurrent.Future updateApplicationAsync(UpdateApplicationRequest updateApplicationRequest);
/**
*
* Updates the application.
*
*
* @param updateApplicationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateApplication operation returned by the service.
* @sample AmazonApplicationInsightsAsyncHandler.UpdateApplication
* @see AWS API Documentation
*/
java.util.concurrent.Future updateApplicationAsync(UpdateApplicationRequest updateApplicationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the custom component name and/or the list of resources that make up the component.
*
*
* @param updateComponentRequest
* @return A Java Future containing the result of the UpdateComponent operation returned by the service.
* @sample AmazonApplicationInsightsAsync.UpdateComponent
* @see AWS API Documentation
*/
java.util.concurrent.Future updateComponentAsync(UpdateComponentRequest updateComponentRequest);
/**
*
* Updates the custom component name and/or the list of resources that make up the component.
*
*
* @param updateComponentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateComponent operation returned by the service.
* @sample AmazonApplicationInsightsAsyncHandler.UpdateComponent
* @see AWS API Documentation
*/
java.util.concurrent.Future updateComponentAsync(UpdateComponentRequest updateComponentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the monitoring configurations for the component. The configuration input parameter is an escaped JSON of
* the configuration and should match the schema of what is returned by
* DescribeComponentConfigurationRecommendation
.
*
*
* @param updateComponentConfigurationRequest
* @return A Java Future containing the result of the UpdateComponentConfiguration operation returned by the
* service.
* @sample AmazonApplicationInsightsAsync.UpdateComponentConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future updateComponentConfigurationAsync(
UpdateComponentConfigurationRequest updateComponentConfigurationRequest);
/**
*
* Updates the monitoring configurations for the component. The configuration input parameter is an escaped JSON of
* the configuration and should match the schema of what is returned by
* DescribeComponentConfigurationRecommendation
.
*
*
* @param updateComponentConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateComponentConfiguration operation returned by the
* service.
* @sample AmazonApplicationInsightsAsyncHandler.UpdateComponentConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future updateComponentConfigurationAsync(
UpdateComponentConfigurationRequest updateComponentConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds a log pattern to a LogPatternSet
.
*
*
* @param updateLogPatternRequest
* @return A Java Future containing the result of the UpdateLogPattern operation returned by the service.
* @sample AmazonApplicationInsightsAsync.UpdateLogPattern
* @see AWS API Documentation
*/
java.util.concurrent.Future updateLogPatternAsync(UpdateLogPatternRequest updateLogPatternRequest);
/**
*
* Adds a log pattern to a LogPatternSet
.
*
*
* @param updateLogPatternRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateLogPattern operation returned by the service.
* @sample AmazonApplicationInsightsAsyncHandler.UpdateLogPattern
* @see AWS API Documentation
*/
java.util.concurrent.Future updateLogPatternAsync(UpdateLogPatternRequest updateLogPatternRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the visibility of the problem or specifies the problem as RESOLVED
.
*
*
* @param updateProblemRequest
* @return A Java Future containing the result of the UpdateProblem operation returned by the service.
* @sample AmazonApplicationInsightsAsync.UpdateProblem
* @see AWS API Documentation
*/
java.util.concurrent.Future updateProblemAsync(UpdateProblemRequest updateProblemRequest);
/**
*
* Updates the visibility of the problem or specifies the problem as RESOLVED
.
*
*
* @param updateProblemRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateProblem operation returned by the service.
* @sample AmazonApplicationInsightsAsyncHandler.UpdateProblem
* @see AWS API Documentation
*/
java.util.concurrent.Future updateProblemAsync(UpdateProblemRequest updateProblemRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds a workload to a component. Each component can have at most five workloads.
*
*
* @param updateWorkloadRequest
* @return A Java Future containing the result of the UpdateWorkload operation returned by the service.
* @sample AmazonApplicationInsightsAsync.UpdateWorkload
* @see AWS API Documentation
*/
java.util.concurrent.Future updateWorkloadAsync(UpdateWorkloadRequest updateWorkloadRequest);
/**
*
* Adds a workload to a component. Each component can have at most five workloads.
*
*
* @param updateWorkloadRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateWorkload operation returned by the service.
* @sample AmazonApplicationInsightsAsyncHandler.UpdateWorkload
* @see AWS API Documentation
*/
java.util.concurrent.Future updateWorkloadAsync(UpdateWorkloadRequest updateWorkloadRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}