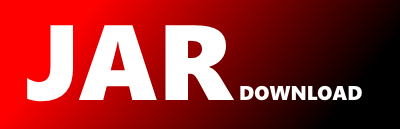
com.amazonaws.services.applicationinsights.model.ConfigurationEvent Maven / Gradle / Ivy
Show all versions of aws-java-sdk-applicationinsights Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.applicationinsights.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* The event information.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ConfigurationEvent implements Serializable, Cloneable, StructuredPojo {
/**
*
* The name of the resource group of the application to which the configuration event belongs.
*
*/
private String resourceGroupName;
/**
*
* The AWS account ID for the owner of the application to which the configuration event belongs.
*
*/
private String accountId;
/**
*
* The resource monitored by Application Insights.
*
*/
private String monitoredResourceARN;
/**
*
* The status of the configuration update event. Possible values include INFO, WARN, and ERROR.
*
*/
private String eventStatus;
/**
*
* The resource type that Application Insights attempted to configure, for example, CLOUDWATCH_ALARM.
*
*/
private String eventResourceType;
/**
*
* The timestamp of the event.
*
*/
private java.util.Date eventTime;
/**
*
* The details of the event in plain text.
*
*/
private String eventDetail;
/**
*
* The name of the resource Application Insights attempted to configure.
*
*/
private String eventResourceName;
/**
*
* The name of the resource group of the application to which the configuration event belongs.
*
*
* @param resourceGroupName
* The name of the resource group of the application to which the configuration event belongs.
*/
public void setResourceGroupName(String resourceGroupName) {
this.resourceGroupName = resourceGroupName;
}
/**
*
* The name of the resource group of the application to which the configuration event belongs.
*
*
* @return The name of the resource group of the application to which the configuration event belongs.
*/
public String getResourceGroupName() {
return this.resourceGroupName;
}
/**
*
* The name of the resource group of the application to which the configuration event belongs.
*
*
* @param resourceGroupName
* The name of the resource group of the application to which the configuration event belongs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ConfigurationEvent withResourceGroupName(String resourceGroupName) {
setResourceGroupName(resourceGroupName);
return this;
}
/**
*
* The AWS account ID for the owner of the application to which the configuration event belongs.
*
*
* @param accountId
* The AWS account ID for the owner of the application to which the configuration event belongs.
*/
public void setAccountId(String accountId) {
this.accountId = accountId;
}
/**
*
* The AWS account ID for the owner of the application to which the configuration event belongs.
*
*
* @return The AWS account ID for the owner of the application to which the configuration event belongs.
*/
public String getAccountId() {
return this.accountId;
}
/**
*
* The AWS account ID for the owner of the application to which the configuration event belongs.
*
*
* @param accountId
* The AWS account ID for the owner of the application to which the configuration event belongs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ConfigurationEvent withAccountId(String accountId) {
setAccountId(accountId);
return this;
}
/**
*
* The resource monitored by Application Insights.
*
*
* @param monitoredResourceARN
* The resource monitored by Application Insights.
*/
public void setMonitoredResourceARN(String monitoredResourceARN) {
this.monitoredResourceARN = monitoredResourceARN;
}
/**
*
* The resource monitored by Application Insights.
*
*
* @return The resource monitored by Application Insights.
*/
public String getMonitoredResourceARN() {
return this.monitoredResourceARN;
}
/**
*
* The resource monitored by Application Insights.
*
*
* @param monitoredResourceARN
* The resource monitored by Application Insights.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ConfigurationEvent withMonitoredResourceARN(String monitoredResourceARN) {
setMonitoredResourceARN(monitoredResourceARN);
return this;
}
/**
*
* The status of the configuration update event. Possible values include INFO, WARN, and ERROR.
*
*
* @param eventStatus
* The status of the configuration update event. Possible values include INFO, WARN, and ERROR.
* @see ConfigurationEventStatus
*/
public void setEventStatus(String eventStatus) {
this.eventStatus = eventStatus;
}
/**
*
* The status of the configuration update event. Possible values include INFO, WARN, and ERROR.
*
*
* @return The status of the configuration update event. Possible values include INFO, WARN, and ERROR.
* @see ConfigurationEventStatus
*/
public String getEventStatus() {
return this.eventStatus;
}
/**
*
* The status of the configuration update event. Possible values include INFO, WARN, and ERROR.
*
*
* @param eventStatus
* The status of the configuration update event. Possible values include INFO, WARN, and ERROR.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ConfigurationEventStatus
*/
public ConfigurationEvent withEventStatus(String eventStatus) {
setEventStatus(eventStatus);
return this;
}
/**
*
* The status of the configuration update event. Possible values include INFO, WARN, and ERROR.
*
*
* @param eventStatus
* The status of the configuration update event. Possible values include INFO, WARN, and ERROR.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ConfigurationEventStatus
*/
public ConfigurationEvent withEventStatus(ConfigurationEventStatus eventStatus) {
this.eventStatus = eventStatus.toString();
return this;
}
/**
*
* The resource type that Application Insights attempted to configure, for example, CLOUDWATCH_ALARM.
*
*
* @param eventResourceType
* The resource type that Application Insights attempted to configure, for example, CLOUDWATCH_ALARM.
* @see ConfigurationEventResourceType
*/
public void setEventResourceType(String eventResourceType) {
this.eventResourceType = eventResourceType;
}
/**
*
* The resource type that Application Insights attempted to configure, for example, CLOUDWATCH_ALARM.
*
*
* @return The resource type that Application Insights attempted to configure, for example, CLOUDWATCH_ALARM.
* @see ConfigurationEventResourceType
*/
public String getEventResourceType() {
return this.eventResourceType;
}
/**
*
* The resource type that Application Insights attempted to configure, for example, CLOUDWATCH_ALARM.
*
*
* @param eventResourceType
* The resource type that Application Insights attempted to configure, for example, CLOUDWATCH_ALARM.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ConfigurationEventResourceType
*/
public ConfigurationEvent withEventResourceType(String eventResourceType) {
setEventResourceType(eventResourceType);
return this;
}
/**
*
* The resource type that Application Insights attempted to configure, for example, CLOUDWATCH_ALARM.
*
*
* @param eventResourceType
* The resource type that Application Insights attempted to configure, for example, CLOUDWATCH_ALARM.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ConfigurationEventResourceType
*/
public ConfigurationEvent withEventResourceType(ConfigurationEventResourceType eventResourceType) {
this.eventResourceType = eventResourceType.toString();
return this;
}
/**
*
* The timestamp of the event.
*
*
* @param eventTime
* The timestamp of the event.
*/
public void setEventTime(java.util.Date eventTime) {
this.eventTime = eventTime;
}
/**
*
* The timestamp of the event.
*
*
* @return The timestamp of the event.
*/
public java.util.Date getEventTime() {
return this.eventTime;
}
/**
*
* The timestamp of the event.
*
*
* @param eventTime
* The timestamp of the event.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ConfigurationEvent withEventTime(java.util.Date eventTime) {
setEventTime(eventTime);
return this;
}
/**
*
* The details of the event in plain text.
*
*
* @param eventDetail
* The details of the event in plain text.
*/
public void setEventDetail(String eventDetail) {
this.eventDetail = eventDetail;
}
/**
*
* The details of the event in plain text.
*
*
* @return The details of the event in plain text.
*/
public String getEventDetail() {
return this.eventDetail;
}
/**
*
* The details of the event in plain text.
*
*
* @param eventDetail
* The details of the event in plain text.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ConfigurationEvent withEventDetail(String eventDetail) {
setEventDetail(eventDetail);
return this;
}
/**
*
* The name of the resource Application Insights attempted to configure.
*
*
* @param eventResourceName
* The name of the resource Application Insights attempted to configure.
*/
public void setEventResourceName(String eventResourceName) {
this.eventResourceName = eventResourceName;
}
/**
*
* The name of the resource Application Insights attempted to configure.
*
*
* @return The name of the resource Application Insights attempted to configure.
*/
public String getEventResourceName() {
return this.eventResourceName;
}
/**
*
* The name of the resource Application Insights attempted to configure.
*
*
* @param eventResourceName
* The name of the resource Application Insights attempted to configure.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ConfigurationEvent withEventResourceName(String eventResourceName) {
setEventResourceName(eventResourceName);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getResourceGroupName() != null)
sb.append("ResourceGroupName: ").append(getResourceGroupName()).append(",");
if (getAccountId() != null)
sb.append("AccountId: ").append(getAccountId()).append(",");
if (getMonitoredResourceARN() != null)
sb.append("MonitoredResourceARN: ").append(getMonitoredResourceARN()).append(",");
if (getEventStatus() != null)
sb.append("EventStatus: ").append(getEventStatus()).append(",");
if (getEventResourceType() != null)
sb.append("EventResourceType: ").append(getEventResourceType()).append(",");
if (getEventTime() != null)
sb.append("EventTime: ").append(getEventTime()).append(",");
if (getEventDetail() != null)
sb.append("EventDetail: ").append(getEventDetail()).append(",");
if (getEventResourceName() != null)
sb.append("EventResourceName: ").append(getEventResourceName());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ConfigurationEvent == false)
return false;
ConfigurationEvent other = (ConfigurationEvent) obj;
if (other.getResourceGroupName() == null ^ this.getResourceGroupName() == null)
return false;
if (other.getResourceGroupName() != null && other.getResourceGroupName().equals(this.getResourceGroupName()) == false)
return false;
if (other.getAccountId() == null ^ this.getAccountId() == null)
return false;
if (other.getAccountId() != null && other.getAccountId().equals(this.getAccountId()) == false)
return false;
if (other.getMonitoredResourceARN() == null ^ this.getMonitoredResourceARN() == null)
return false;
if (other.getMonitoredResourceARN() != null && other.getMonitoredResourceARN().equals(this.getMonitoredResourceARN()) == false)
return false;
if (other.getEventStatus() == null ^ this.getEventStatus() == null)
return false;
if (other.getEventStatus() != null && other.getEventStatus().equals(this.getEventStatus()) == false)
return false;
if (other.getEventResourceType() == null ^ this.getEventResourceType() == null)
return false;
if (other.getEventResourceType() != null && other.getEventResourceType().equals(this.getEventResourceType()) == false)
return false;
if (other.getEventTime() == null ^ this.getEventTime() == null)
return false;
if (other.getEventTime() != null && other.getEventTime().equals(this.getEventTime()) == false)
return false;
if (other.getEventDetail() == null ^ this.getEventDetail() == null)
return false;
if (other.getEventDetail() != null && other.getEventDetail().equals(this.getEventDetail()) == false)
return false;
if (other.getEventResourceName() == null ^ this.getEventResourceName() == null)
return false;
if (other.getEventResourceName() != null && other.getEventResourceName().equals(this.getEventResourceName()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getResourceGroupName() == null) ? 0 : getResourceGroupName().hashCode());
hashCode = prime * hashCode + ((getAccountId() == null) ? 0 : getAccountId().hashCode());
hashCode = prime * hashCode + ((getMonitoredResourceARN() == null) ? 0 : getMonitoredResourceARN().hashCode());
hashCode = prime * hashCode + ((getEventStatus() == null) ? 0 : getEventStatus().hashCode());
hashCode = prime * hashCode + ((getEventResourceType() == null) ? 0 : getEventResourceType().hashCode());
hashCode = prime * hashCode + ((getEventTime() == null) ? 0 : getEventTime().hashCode());
hashCode = prime * hashCode + ((getEventDetail() == null) ? 0 : getEventDetail().hashCode());
hashCode = prime * hashCode + ((getEventResourceName() == null) ? 0 : getEventResourceName().hashCode());
return hashCode;
}
@Override
public ConfigurationEvent clone() {
try {
return (ConfigurationEvent) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.applicationinsights.model.transform.ConfigurationEventMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}