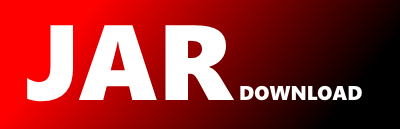
com.amazonaws.services.applicationinsights.model.ApplicationComponent Maven / Gradle / Ivy
Show all versions of aws-java-sdk-applicationinsights Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.applicationinsights.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Describes a standalone resource or similarly grouped resources that the application is made up of.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ApplicationComponent implements Serializable, Cloneable, StructuredPojo {
/**
*
* The name of the component.
*
*/
private String componentName;
/**
*
* If logging is supported for the resource type, indicates whether the component has configured logs to be
* monitored.
*
*/
private String componentRemarks;
/**
*
* The resource type. Supported resource types include EC2 instances, Auto Scaling group, Classic ELB, Application
* ELB, and SQS Queue.
*
*/
private String resourceType;
/**
*
* The operating system of the component.
*
*/
private String osType;
/**
*
* The stack tier of the application component.
*
*/
private String tier;
/**
*
* Indicates whether the application component is monitored.
*
*/
private Boolean monitor;
/**
*
* Workloads detected in the application component.
*
*/
private java.util.Map> detectedWorkload;
/**
*
* The name of the component.
*
*
* @param componentName
* The name of the component.
*/
public void setComponentName(String componentName) {
this.componentName = componentName;
}
/**
*
* The name of the component.
*
*
* @return The name of the component.
*/
public String getComponentName() {
return this.componentName;
}
/**
*
* The name of the component.
*
*
* @param componentName
* The name of the component.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ApplicationComponent withComponentName(String componentName) {
setComponentName(componentName);
return this;
}
/**
*
* If logging is supported for the resource type, indicates whether the component has configured logs to be
* monitored.
*
*
* @param componentRemarks
* If logging is supported for the resource type, indicates whether the component has configured logs to be
* monitored.
*/
public void setComponentRemarks(String componentRemarks) {
this.componentRemarks = componentRemarks;
}
/**
*
* If logging is supported for the resource type, indicates whether the component has configured logs to be
* monitored.
*
*
* @return If logging is supported for the resource type, indicates whether the component has configured logs to be
* monitored.
*/
public String getComponentRemarks() {
return this.componentRemarks;
}
/**
*
* If logging is supported for the resource type, indicates whether the component has configured logs to be
* monitored.
*
*
* @param componentRemarks
* If logging is supported for the resource type, indicates whether the component has configured logs to be
* monitored.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ApplicationComponent withComponentRemarks(String componentRemarks) {
setComponentRemarks(componentRemarks);
return this;
}
/**
*
* The resource type. Supported resource types include EC2 instances, Auto Scaling group, Classic ELB, Application
* ELB, and SQS Queue.
*
*
* @param resourceType
* The resource type. Supported resource types include EC2 instances, Auto Scaling group, Classic ELB,
* Application ELB, and SQS Queue.
*/
public void setResourceType(String resourceType) {
this.resourceType = resourceType;
}
/**
*
* The resource type. Supported resource types include EC2 instances, Auto Scaling group, Classic ELB, Application
* ELB, and SQS Queue.
*
*
* @return The resource type. Supported resource types include EC2 instances, Auto Scaling group, Classic ELB,
* Application ELB, and SQS Queue.
*/
public String getResourceType() {
return this.resourceType;
}
/**
*
* The resource type. Supported resource types include EC2 instances, Auto Scaling group, Classic ELB, Application
* ELB, and SQS Queue.
*
*
* @param resourceType
* The resource type. Supported resource types include EC2 instances, Auto Scaling group, Classic ELB,
* Application ELB, and SQS Queue.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ApplicationComponent withResourceType(String resourceType) {
setResourceType(resourceType);
return this;
}
/**
*
* The operating system of the component.
*
*
* @param osType
* The operating system of the component.
* @see OsType
*/
public void setOsType(String osType) {
this.osType = osType;
}
/**
*
* The operating system of the component.
*
*
* @return The operating system of the component.
* @see OsType
*/
public String getOsType() {
return this.osType;
}
/**
*
* The operating system of the component.
*
*
* @param osType
* The operating system of the component.
* @return Returns a reference to this object so that method calls can be chained together.
* @see OsType
*/
public ApplicationComponent withOsType(String osType) {
setOsType(osType);
return this;
}
/**
*
* The operating system of the component.
*
*
* @param osType
* The operating system of the component.
* @return Returns a reference to this object so that method calls can be chained together.
* @see OsType
*/
public ApplicationComponent withOsType(OsType osType) {
this.osType = osType.toString();
return this;
}
/**
*
* The stack tier of the application component.
*
*
* @param tier
* The stack tier of the application component.
* @see Tier
*/
public void setTier(String tier) {
this.tier = tier;
}
/**
*
* The stack tier of the application component.
*
*
* @return The stack tier of the application component.
* @see Tier
*/
public String getTier() {
return this.tier;
}
/**
*
* The stack tier of the application component.
*
*
* @param tier
* The stack tier of the application component.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Tier
*/
public ApplicationComponent withTier(String tier) {
setTier(tier);
return this;
}
/**
*
* The stack tier of the application component.
*
*
* @param tier
* The stack tier of the application component.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Tier
*/
public ApplicationComponent withTier(Tier tier) {
this.tier = tier.toString();
return this;
}
/**
*
* Indicates whether the application component is monitored.
*
*
* @param monitor
* Indicates whether the application component is monitored.
*/
public void setMonitor(Boolean monitor) {
this.monitor = monitor;
}
/**
*
* Indicates whether the application component is monitored.
*
*
* @return Indicates whether the application component is monitored.
*/
public Boolean getMonitor() {
return this.monitor;
}
/**
*
* Indicates whether the application component is monitored.
*
*
* @param monitor
* Indicates whether the application component is monitored.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ApplicationComponent withMonitor(Boolean monitor) {
setMonitor(monitor);
return this;
}
/**
*
* Indicates whether the application component is monitored.
*
*
* @return Indicates whether the application component is monitored.
*/
public Boolean isMonitor() {
return this.monitor;
}
/**
*
* Workloads detected in the application component.
*
*
* @return Workloads detected in the application component.
*/
public java.util.Map> getDetectedWorkload() {
return detectedWorkload;
}
/**
*
* Workloads detected in the application component.
*
*
* @param detectedWorkload
* Workloads detected in the application component.
*/
public void setDetectedWorkload(java.util.Map> detectedWorkload) {
this.detectedWorkload = detectedWorkload;
}
/**
*
* Workloads detected in the application component.
*
*
* @param detectedWorkload
* Workloads detected in the application component.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ApplicationComponent withDetectedWorkload(java.util.Map> detectedWorkload) {
setDetectedWorkload(detectedWorkload);
return this;
}
/**
* Add a single DetectedWorkload entry
*
* @see ApplicationComponent#withDetectedWorkload
* @returns a reference to this object so that method calls can be chained together.
*/
public ApplicationComponent addDetectedWorkloadEntry(String key, java.util.Map value) {
if (null == this.detectedWorkload) {
this.detectedWorkload = new java.util.HashMap>();
}
if (this.detectedWorkload.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.detectedWorkload.put(key, value);
return this;
}
/**
* Removes all the entries added into DetectedWorkload.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ApplicationComponent clearDetectedWorkloadEntries() {
this.detectedWorkload = null;
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getComponentName() != null)
sb.append("ComponentName: ").append(getComponentName()).append(",");
if (getComponentRemarks() != null)
sb.append("ComponentRemarks: ").append(getComponentRemarks()).append(",");
if (getResourceType() != null)
sb.append("ResourceType: ").append(getResourceType()).append(",");
if (getOsType() != null)
sb.append("OsType: ").append(getOsType()).append(",");
if (getTier() != null)
sb.append("Tier: ").append(getTier()).append(",");
if (getMonitor() != null)
sb.append("Monitor: ").append(getMonitor()).append(",");
if (getDetectedWorkload() != null)
sb.append("DetectedWorkload: ").append(getDetectedWorkload());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ApplicationComponent == false)
return false;
ApplicationComponent other = (ApplicationComponent) obj;
if (other.getComponentName() == null ^ this.getComponentName() == null)
return false;
if (other.getComponentName() != null && other.getComponentName().equals(this.getComponentName()) == false)
return false;
if (other.getComponentRemarks() == null ^ this.getComponentRemarks() == null)
return false;
if (other.getComponentRemarks() != null && other.getComponentRemarks().equals(this.getComponentRemarks()) == false)
return false;
if (other.getResourceType() == null ^ this.getResourceType() == null)
return false;
if (other.getResourceType() != null && other.getResourceType().equals(this.getResourceType()) == false)
return false;
if (other.getOsType() == null ^ this.getOsType() == null)
return false;
if (other.getOsType() != null && other.getOsType().equals(this.getOsType()) == false)
return false;
if (other.getTier() == null ^ this.getTier() == null)
return false;
if (other.getTier() != null && other.getTier().equals(this.getTier()) == false)
return false;
if (other.getMonitor() == null ^ this.getMonitor() == null)
return false;
if (other.getMonitor() != null && other.getMonitor().equals(this.getMonitor()) == false)
return false;
if (other.getDetectedWorkload() == null ^ this.getDetectedWorkload() == null)
return false;
if (other.getDetectedWorkload() != null && other.getDetectedWorkload().equals(this.getDetectedWorkload()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getComponentName() == null) ? 0 : getComponentName().hashCode());
hashCode = prime * hashCode + ((getComponentRemarks() == null) ? 0 : getComponentRemarks().hashCode());
hashCode = prime * hashCode + ((getResourceType() == null) ? 0 : getResourceType().hashCode());
hashCode = prime * hashCode + ((getOsType() == null) ? 0 : getOsType().hashCode());
hashCode = prime * hashCode + ((getTier() == null) ? 0 : getTier().hashCode());
hashCode = prime * hashCode + ((getMonitor() == null) ? 0 : getMonitor().hashCode());
hashCode = prime * hashCode + ((getDetectedWorkload() == null) ? 0 : getDetectedWorkload().hashCode());
return hashCode;
}
@Override
public ApplicationComponent clone() {
try {
return (ApplicationComponent) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.applicationinsights.model.transform.ApplicationComponentMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}