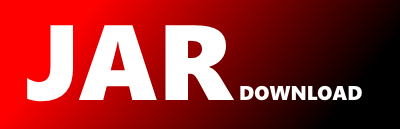
com.amazonaws.services.applicationinsights.model.Problem Maven / Gradle / Ivy
Show all versions of aws-java-sdk-applicationinsights Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.applicationinsights.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Describes a problem that is detected by correlating observations.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class Problem implements Serializable, Cloneable, StructuredPojo {
/**
*
* The ID of the problem.
*
*/
private String id;
/**
*
* The name of the problem.
*
*/
private String title;
/**
*
* A detailed analysis of the problem using machine learning.
*
*/
private String insights;
/**
*
* The status of the problem.
*
*/
private String status;
/**
*
* The resource affected by the problem.
*
*/
private String affectedResource;
/**
*
* The time when the problem started, in epoch seconds.
*
*/
private java.util.Date startTime;
/**
*
* The time when the problem ended, in epoch seconds.
*
*/
private java.util.Date endTime;
/**
*
* A measure of the level of impact of the problem.
*
*/
private String severityLevel;
/**
*
* The AWS account ID for the owner of the resource group affected by the problem.
*
*/
private String accountId;
/**
*
* The name of the resource group affected by the problem.
*
*/
private String resourceGroupName;
/**
*
* Feedback provided by the user about the problem.
*
*/
private java.util.Map feedback;
/**
*
* The number of times that the same problem reoccurred after the first time it was resolved.
*
*/
private Long recurringCount;
/**
*
* The last time that the problem reoccurred after its last resolution.
*
*/
private java.util.Date lastRecurrenceTime;
/**
*
* Specifies whether or not you can view the problem. Updates to ignored problems do not generate notifications.
*
*/
private String visibility;
/**
*
* Specifies how the problem was resolved. If the value is AUTOMATIC
, the system resolved the problem.
* If the value is MANUAL
, the user resolved the problem. If the value is UNRESOLVED
, then
* the problem is not resolved.
*
*/
private String resolutionMethod;
/**
*
* The ID of the problem.
*
*
* @param id
* The ID of the problem.
*/
public void setId(String id) {
this.id = id;
}
/**
*
* The ID of the problem.
*
*
* @return The ID of the problem.
*/
public String getId() {
return this.id;
}
/**
*
* The ID of the problem.
*
*
* @param id
* The ID of the problem.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Problem withId(String id) {
setId(id);
return this;
}
/**
*
* The name of the problem.
*
*
* @param title
* The name of the problem.
*/
public void setTitle(String title) {
this.title = title;
}
/**
*
* The name of the problem.
*
*
* @return The name of the problem.
*/
public String getTitle() {
return this.title;
}
/**
*
* The name of the problem.
*
*
* @param title
* The name of the problem.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Problem withTitle(String title) {
setTitle(title);
return this;
}
/**
*
* A detailed analysis of the problem using machine learning.
*
*
* @param insights
* A detailed analysis of the problem using machine learning.
*/
public void setInsights(String insights) {
this.insights = insights;
}
/**
*
* A detailed analysis of the problem using machine learning.
*
*
* @return A detailed analysis of the problem using machine learning.
*/
public String getInsights() {
return this.insights;
}
/**
*
* A detailed analysis of the problem using machine learning.
*
*
* @param insights
* A detailed analysis of the problem using machine learning.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Problem withInsights(String insights) {
setInsights(insights);
return this;
}
/**
*
* The status of the problem.
*
*
* @param status
* The status of the problem.
* @see Status
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The status of the problem.
*
*
* @return The status of the problem.
* @see Status
*/
public String getStatus() {
return this.status;
}
/**
*
* The status of the problem.
*
*
* @param status
* The status of the problem.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Status
*/
public Problem withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The status of the problem.
*
*
* @param status
* The status of the problem.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Status
*/
public Problem withStatus(Status status) {
this.status = status.toString();
return this;
}
/**
*
* The resource affected by the problem.
*
*
* @param affectedResource
* The resource affected by the problem.
*/
public void setAffectedResource(String affectedResource) {
this.affectedResource = affectedResource;
}
/**
*
* The resource affected by the problem.
*
*
* @return The resource affected by the problem.
*/
public String getAffectedResource() {
return this.affectedResource;
}
/**
*
* The resource affected by the problem.
*
*
* @param affectedResource
* The resource affected by the problem.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Problem withAffectedResource(String affectedResource) {
setAffectedResource(affectedResource);
return this;
}
/**
*
* The time when the problem started, in epoch seconds.
*
*
* @param startTime
* The time when the problem started, in epoch seconds.
*/
public void setStartTime(java.util.Date startTime) {
this.startTime = startTime;
}
/**
*
* The time when the problem started, in epoch seconds.
*
*
* @return The time when the problem started, in epoch seconds.
*/
public java.util.Date getStartTime() {
return this.startTime;
}
/**
*
* The time when the problem started, in epoch seconds.
*
*
* @param startTime
* The time when the problem started, in epoch seconds.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Problem withStartTime(java.util.Date startTime) {
setStartTime(startTime);
return this;
}
/**
*
* The time when the problem ended, in epoch seconds.
*
*
* @param endTime
* The time when the problem ended, in epoch seconds.
*/
public void setEndTime(java.util.Date endTime) {
this.endTime = endTime;
}
/**
*
* The time when the problem ended, in epoch seconds.
*
*
* @return The time when the problem ended, in epoch seconds.
*/
public java.util.Date getEndTime() {
return this.endTime;
}
/**
*
* The time when the problem ended, in epoch seconds.
*
*
* @param endTime
* The time when the problem ended, in epoch seconds.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Problem withEndTime(java.util.Date endTime) {
setEndTime(endTime);
return this;
}
/**
*
* A measure of the level of impact of the problem.
*
*
* @param severityLevel
* A measure of the level of impact of the problem.
* @see SeverityLevel
*/
public void setSeverityLevel(String severityLevel) {
this.severityLevel = severityLevel;
}
/**
*
* A measure of the level of impact of the problem.
*
*
* @return A measure of the level of impact of the problem.
* @see SeverityLevel
*/
public String getSeverityLevel() {
return this.severityLevel;
}
/**
*
* A measure of the level of impact of the problem.
*
*
* @param severityLevel
* A measure of the level of impact of the problem.
* @return Returns a reference to this object so that method calls can be chained together.
* @see SeverityLevel
*/
public Problem withSeverityLevel(String severityLevel) {
setSeverityLevel(severityLevel);
return this;
}
/**
*
* A measure of the level of impact of the problem.
*
*
* @param severityLevel
* A measure of the level of impact of the problem.
* @return Returns a reference to this object so that method calls can be chained together.
* @see SeverityLevel
*/
public Problem withSeverityLevel(SeverityLevel severityLevel) {
this.severityLevel = severityLevel.toString();
return this;
}
/**
*
* The AWS account ID for the owner of the resource group affected by the problem.
*
*
* @param accountId
* The AWS account ID for the owner of the resource group affected by the problem.
*/
public void setAccountId(String accountId) {
this.accountId = accountId;
}
/**
*
* The AWS account ID for the owner of the resource group affected by the problem.
*
*
* @return The AWS account ID for the owner of the resource group affected by the problem.
*/
public String getAccountId() {
return this.accountId;
}
/**
*
* The AWS account ID for the owner of the resource group affected by the problem.
*
*
* @param accountId
* The AWS account ID for the owner of the resource group affected by the problem.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Problem withAccountId(String accountId) {
setAccountId(accountId);
return this;
}
/**
*
* The name of the resource group affected by the problem.
*
*
* @param resourceGroupName
* The name of the resource group affected by the problem.
*/
public void setResourceGroupName(String resourceGroupName) {
this.resourceGroupName = resourceGroupName;
}
/**
*
* The name of the resource group affected by the problem.
*
*
* @return The name of the resource group affected by the problem.
*/
public String getResourceGroupName() {
return this.resourceGroupName;
}
/**
*
* The name of the resource group affected by the problem.
*
*
* @param resourceGroupName
* The name of the resource group affected by the problem.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Problem withResourceGroupName(String resourceGroupName) {
setResourceGroupName(resourceGroupName);
return this;
}
/**
*
* Feedback provided by the user about the problem.
*
*
* @return Feedback provided by the user about the problem.
*/
public java.util.Map getFeedback() {
return feedback;
}
/**
*
* Feedback provided by the user about the problem.
*
*
* @param feedback
* Feedback provided by the user about the problem.
*/
public void setFeedback(java.util.Map feedback) {
this.feedback = feedback;
}
/**
*
* Feedback provided by the user about the problem.
*
*
* @param feedback
* Feedback provided by the user about the problem.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Problem withFeedback(java.util.Map feedback) {
setFeedback(feedback);
return this;
}
/**
* Add a single Feedback entry
*
* @see Problem#withFeedback
* @returns a reference to this object so that method calls can be chained together.
*/
public Problem addFeedbackEntry(String key, String value) {
if (null == this.feedback) {
this.feedback = new java.util.HashMap();
}
if (this.feedback.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.feedback.put(key, value);
return this;
}
/**
* Removes all the entries added into Feedback.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Problem clearFeedbackEntries() {
this.feedback = null;
return this;
}
/**
*
* The number of times that the same problem reoccurred after the first time it was resolved.
*
*
* @param recurringCount
* The number of times that the same problem reoccurred after the first time it was resolved.
*/
public void setRecurringCount(Long recurringCount) {
this.recurringCount = recurringCount;
}
/**
*
* The number of times that the same problem reoccurred after the first time it was resolved.
*
*
* @return The number of times that the same problem reoccurred after the first time it was resolved.
*/
public Long getRecurringCount() {
return this.recurringCount;
}
/**
*
* The number of times that the same problem reoccurred after the first time it was resolved.
*
*
* @param recurringCount
* The number of times that the same problem reoccurred after the first time it was resolved.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Problem withRecurringCount(Long recurringCount) {
setRecurringCount(recurringCount);
return this;
}
/**
*
* The last time that the problem reoccurred after its last resolution.
*
*
* @param lastRecurrenceTime
* The last time that the problem reoccurred after its last resolution.
*/
public void setLastRecurrenceTime(java.util.Date lastRecurrenceTime) {
this.lastRecurrenceTime = lastRecurrenceTime;
}
/**
*
* The last time that the problem reoccurred after its last resolution.
*
*
* @return The last time that the problem reoccurred after its last resolution.
*/
public java.util.Date getLastRecurrenceTime() {
return this.lastRecurrenceTime;
}
/**
*
* The last time that the problem reoccurred after its last resolution.
*
*
* @param lastRecurrenceTime
* The last time that the problem reoccurred after its last resolution.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Problem withLastRecurrenceTime(java.util.Date lastRecurrenceTime) {
setLastRecurrenceTime(lastRecurrenceTime);
return this;
}
/**
*
* Specifies whether or not you can view the problem. Updates to ignored problems do not generate notifications.
*
*
* @param visibility
* Specifies whether or not you can view the problem. Updates to ignored problems do not generate
* notifications.
* @see Visibility
*/
public void setVisibility(String visibility) {
this.visibility = visibility;
}
/**
*
* Specifies whether or not you can view the problem. Updates to ignored problems do not generate notifications.
*
*
* @return Specifies whether or not you can view the problem. Updates to ignored problems do not generate
* notifications.
* @see Visibility
*/
public String getVisibility() {
return this.visibility;
}
/**
*
* Specifies whether or not you can view the problem. Updates to ignored problems do not generate notifications.
*
*
* @param visibility
* Specifies whether or not you can view the problem. Updates to ignored problems do not generate
* notifications.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Visibility
*/
public Problem withVisibility(String visibility) {
setVisibility(visibility);
return this;
}
/**
*
* Specifies whether or not you can view the problem. Updates to ignored problems do not generate notifications.
*
*
* @param visibility
* Specifies whether or not you can view the problem. Updates to ignored problems do not generate
* notifications.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Visibility
*/
public Problem withVisibility(Visibility visibility) {
this.visibility = visibility.toString();
return this;
}
/**
*
* Specifies how the problem was resolved. If the value is AUTOMATIC
, the system resolved the problem.
* If the value is MANUAL
, the user resolved the problem. If the value is UNRESOLVED
, then
* the problem is not resolved.
*
*
* @param resolutionMethod
* Specifies how the problem was resolved. If the value is AUTOMATIC
, the system resolved the
* problem. If the value is MANUAL
, the user resolved the problem. If the value is
* UNRESOLVED
, then the problem is not resolved.
* @see ResolutionMethod
*/
public void setResolutionMethod(String resolutionMethod) {
this.resolutionMethod = resolutionMethod;
}
/**
*
* Specifies how the problem was resolved. If the value is AUTOMATIC
, the system resolved the problem.
* If the value is MANUAL
, the user resolved the problem. If the value is UNRESOLVED
, then
* the problem is not resolved.
*
*
* @return Specifies how the problem was resolved. If the value is AUTOMATIC
, the system resolved the
* problem. If the value is MANUAL
, the user resolved the problem. If the value is
* UNRESOLVED
, then the problem is not resolved.
* @see ResolutionMethod
*/
public String getResolutionMethod() {
return this.resolutionMethod;
}
/**
*
* Specifies how the problem was resolved. If the value is AUTOMATIC
, the system resolved the problem.
* If the value is MANUAL
, the user resolved the problem. If the value is UNRESOLVED
, then
* the problem is not resolved.
*
*
* @param resolutionMethod
* Specifies how the problem was resolved. If the value is AUTOMATIC
, the system resolved the
* problem. If the value is MANUAL
, the user resolved the problem. If the value is
* UNRESOLVED
, then the problem is not resolved.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ResolutionMethod
*/
public Problem withResolutionMethod(String resolutionMethod) {
setResolutionMethod(resolutionMethod);
return this;
}
/**
*
* Specifies how the problem was resolved. If the value is AUTOMATIC
, the system resolved the problem.
* If the value is MANUAL
, the user resolved the problem. If the value is UNRESOLVED
, then
* the problem is not resolved.
*
*
* @param resolutionMethod
* Specifies how the problem was resolved. If the value is AUTOMATIC
, the system resolved the
* problem. If the value is MANUAL
, the user resolved the problem. If the value is
* UNRESOLVED
, then the problem is not resolved.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ResolutionMethod
*/
public Problem withResolutionMethod(ResolutionMethod resolutionMethod) {
this.resolutionMethod = resolutionMethod.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getId() != null)
sb.append("Id: ").append(getId()).append(",");
if (getTitle() != null)
sb.append("Title: ").append(getTitle()).append(",");
if (getInsights() != null)
sb.append("Insights: ").append(getInsights()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getAffectedResource() != null)
sb.append("AffectedResource: ").append(getAffectedResource()).append(",");
if (getStartTime() != null)
sb.append("StartTime: ").append(getStartTime()).append(",");
if (getEndTime() != null)
sb.append("EndTime: ").append(getEndTime()).append(",");
if (getSeverityLevel() != null)
sb.append("SeverityLevel: ").append(getSeverityLevel()).append(",");
if (getAccountId() != null)
sb.append("AccountId: ").append(getAccountId()).append(",");
if (getResourceGroupName() != null)
sb.append("ResourceGroupName: ").append(getResourceGroupName()).append(",");
if (getFeedback() != null)
sb.append("Feedback: ").append(getFeedback()).append(",");
if (getRecurringCount() != null)
sb.append("RecurringCount: ").append(getRecurringCount()).append(",");
if (getLastRecurrenceTime() != null)
sb.append("LastRecurrenceTime: ").append(getLastRecurrenceTime()).append(",");
if (getVisibility() != null)
sb.append("Visibility: ").append(getVisibility()).append(",");
if (getResolutionMethod() != null)
sb.append("ResolutionMethod: ").append(getResolutionMethod());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof Problem == false)
return false;
Problem other = (Problem) obj;
if (other.getId() == null ^ this.getId() == null)
return false;
if (other.getId() != null && other.getId().equals(this.getId()) == false)
return false;
if (other.getTitle() == null ^ this.getTitle() == null)
return false;
if (other.getTitle() != null && other.getTitle().equals(this.getTitle()) == false)
return false;
if (other.getInsights() == null ^ this.getInsights() == null)
return false;
if (other.getInsights() != null && other.getInsights().equals(this.getInsights()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getAffectedResource() == null ^ this.getAffectedResource() == null)
return false;
if (other.getAffectedResource() != null && other.getAffectedResource().equals(this.getAffectedResource()) == false)
return false;
if (other.getStartTime() == null ^ this.getStartTime() == null)
return false;
if (other.getStartTime() != null && other.getStartTime().equals(this.getStartTime()) == false)
return false;
if (other.getEndTime() == null ^ this.getEndTime() == null)
return false;
if (other.getEndTime() != null && other.getEndTime().equals(this.getEndTime()) == false)
return false;
if (other.getSeverityLevel() == null ^ this.getSeverityLevel() == null)
return false;
if (other.getSeverityLevel() != null && other.getSeverityLevel().equals(this.getSeverityLevel()) == false)
return false;
if (other.getAccountId() == null ^ this.getAccountId() == null)
return false;
if (other.getAccountId() != null && other.getAccountId().equals(this.getAccountId()) == false)
return false;
if (other.getResourceGroupName() == null ^ this.getResourceGroupName() == null)
return false;
if (other.getResourceGroupName() != null && other.getResourceGroupName().equals(this.getResourceGroupName()) == false)
return false;
if (other.getFeedback() == null ^ this.getFeedback() == null)
return false;
if (other.getFeedback() != null && other.getFeedback().equals(this.getFeedback()) == false)
return false;
if (other.getRecurringCount() == null ^ this.getRecurringCount() == null)
return false;
if (other.getRecurringCount() != null && other.getRecurringCount().equals(this.getRecurringCount()) == false)
return false;
if (other.getLastRecurrenceTime() == null ^ this.getLastRecurrenceTime() == null)
return false;
if (other.getLastRecurrenceTime() != null && other.getLastRecurrenceTime().equals(this.getLastRecurrenceTime()) == false)
return false;
if (other.getVisibility() == null ^ this.getVisibility() == null)
return false;
if (other.getVisibility() != null && other.getVisibility().equals(this.getVisibility()) == false)
return false;
if (other.getResolutionMethod() == null ^ this.getResolutionMethod() == null)
return false;
if (other.getResolutionMethod() != null && other.getResolutionMethod().equals(this.getResolutionMethod()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getId() == null) ? 0 : getId().hashCode());
hashCode = prime * hashCode + ((getTitle() == null) ? 0 : getTitle().hashCode());
hashCode = prime * hashCode + ((getInsights() == null) ? 0 : getInsights().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getAffectedResource() == null) ? 0 : getAffectedResource().hashCode());
hashCode = prime * hashCode + ((getStartTime() == null) ? 0 : getStartTime().hashCode());
hashCode = prime * hashCode + ((getEndTime() == null) ? 0 : getEndTime().hashCode());
hashCode = prime * hashCode + ((getSeverityLevel() == null) ? 0 : getSeverityLevel().hashCode());
hashCode = prime * hashCode + ((getAccountId() == null) ? 0 : getAccountId().hashCode());
hashCode = prime * hashCode + ((getResourceGroupName() == null) ? 0 : getResourceGroupName().hashCode());
hashCode = prime * hashCode + ((getFeedback() == null) ? 0 : getFeedback().hashCode());
hashCode = prime * hashCode + ((getRecurringCount() == null) ? 0 : getRecurringCount().hashCode());
hashCode = prime * hashCode + ((getLastRecurrenceTime() == null) ? 0 : getLastRecurrenceTime().hashCode());
hashCode = prime * hashCode + ((getVisibility() == null) ? 0 : getVisibility().hashCode());
hashCode = prime * hashCode + ((getResolutionMethod() == null) ? 0 : getResolutionMethod().hashCode());
return hashCode;
}
@Override
public Problem clone() {
try {
return (Problem) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.applicationinsights.model.transform.ProblemMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}