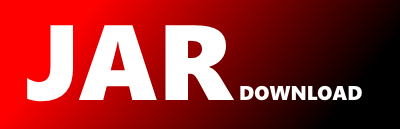
com.amazonaws.services.applicationsignals.AbstractAmazonApplicationSignalsAsync Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws-java-sdk-applicationsignals Show documentation
Show all versions of aws-java-sdk-applicationsignals Show documentation
The AWS Java SDK for Amazon CloudWatch Application Signals module holds the client classes that are used for communicating with Amazon CloudWatch Application Signals Service
The newest version!
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.applicationsignals;
import javax.annotation.Generated;
import com.amazonaws.services.applicationsignals.model.*;
/**
* Abstract implementation of {@code AmazonApplicationSignalsAsync}. Convenient method forms pass through to the
* corresponding overload that takes a request object and an {@code AsyncHandler}, which throws an
* {@code UnsupportedOperationException}.
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AbstractAmazonApplicationSignalsAsync extends AbstractAmazonApplicationSignals implements AmazonApplicationSignalsAsync {
protected AbstractAmazonApplicationSignalsAsync() {
}
@Override
public java.util.concurrent.Future batchGetServiceLevelObjectiveBudgetReportAsync(
BatchGetServiceLevelObjectiveBudgetReportRequest request) {
return batchGetServiceLevelObjectiveBudgetReportAsync(request, null);
}
@Override
public java.util.concurrent.Future batchGetServiceLevelObjectiveBudgetReportAsync(
BatchGetServiceLevelObjectiveBudgetReportRequest request,
com.amazonaws.handlers.AsyncHandler asyncHandler) {
throw new java.lang.UnsupportedOperationException();
}
@Override
public java.util.concurrent.Future createServiceLevelObjectiveAsync(CreateServiceLevelObjectiveRequest request) {
return createServiceLevelObjectiveAsync(request, null);
}
@Override
public java.util.concurrent.Future createServiceLevelObjectiveAsync(CreateServiceLevelObjectiveRequest request,
com.amazonaws.handlers.AsyncHandler asyncHandler) {
throw new java.lang.UnsupportedOperationException();
}
@Override
public java.util.concurrent.Future deleteServiceLevelObjectiveAsync(DeleteServiceLevelObjectiveRequest request) {
return deleteServiceLevelObjectiveAsync(request, null);
}
@Override
public java.util.concurrent.Future deleteServiceLevelObjectiveAsync(DeleteServiceLevelObjectiveRequest request,
com.amazonaws.handlers.AsyncHandler asyncHandler) {
throw new java.lang.UnsupportedOperationException();
}
@Override
public java.util.concurrent.Future getServiceAsync(GetServiceRequest request) {
return getServiceAsync(request, null);
}
@Override
public java.util.concurrent.Future getServiceAsync(GetServiceRequest request,
com.amazonaws.handlers.AsyncHandler asyncHandler) {
throw new java.lang.UnsupportedOperationException();
}
@Override
public java.util.concurrent.Future getServiceLevelObjectiveAsync(GetServiceLevelObjectiveRequest request) {
return getServiceLevelObjectiveAsync(request, null);
}
@Override
public java.util.concurrent.Future getServiceLevelObjectiveAsync(GetServiceLevelObjectiveRequest request,
com.amazonaws.handlers.AsyncHandler asyncHandler) {
throw new java.lang.UnsupportedOperationException();
}
@Override
public java.util.concurrent.Future listServiceDependenciesAsync(ListServiceDependenciesRequest request) {
return listServiceDependenciesAsync(request, null);
}
@Override
public java.util.concurrent.Future listServiceDependenciesAsync(ListServiceDependenciesRequest request,
com.amazonaws.handlers.AsyncHandler asyncHandler) {
throw new java.lang.UnsupportedOperationException();
}
@Override
public java.util.concurrent.Future listServiceDependentsAsync(ListServiceDependentsRequest request) {
return listServiceDependentsAsync(request, null);
}
@Override
public java.util.concurrent.Future listServiceDependentsAsync(ListServiceDependentsRequest request,
com.amazonaws.handlers.AsyncHandler asyncHandler) {
throw new java.lang.UnsupportedOperationException();
}
@Override
public java.util.concurrent.Future listServiceLevelObjectivesAsync(ListServiceLevelObjectivesRequest request) {
return listServiceLevelObjectivesAsync(request, null);
}
@Override
public java.util.concurrent.Future listServiceLevelObjectivesAsync(ListServiceLevelObjectivesRequest request,
com.amazonaws.handlers.AsyncHandler asyncHandler) {
throw new java.lang.UnsupportedOperationException();
}
@Override
public java.util.concurrent.Future listServiceOperationsAsync(ListServiceOperationsRequest request) {
return listServiceOperationsAsync(request, null);
}
@Override
public java.util.concurrent.Future listServiceOperationsAsync(ListServiceOperationsRequest request,
com.amazonaws.handlers.AsyncHandler asyncHandler) {
throw new java.lang.UnsupportedOperationException();
}
@Override
public java.util.concurrent.Future listServicesAsync(ListServicesRequest request) {
return listServicesAsync(request, null);
}
@Override
public java.util.concurrent.Future listServicesAsync(ListServicesRequest request,
com.amazonaws.handlers.AsyncHandler asyncHandler) {
throw new java.lang.UnsupportedOperationException();
}
@Override
public java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest request) {
return listTagsForResourceAsync(request, null);
}
@Override
public java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest request,
com.amazonaws.handlers.AsyncHandler asyncHandler) {
throw new java.lang.UnsupportedOperationException();
}
@Override
public java.util.concurrent.Future startDiscoveryAsync(StartDiscoveryRequest request) {
return startDiscoveryAsync(request, null);
}
@Override
public java.util.concurrent.Future startDiscoveryAsync(StartDiscoveryRequest request,
com.amazonaws.handlers.AsyncHandler asyncHandler) {
throw new java.lang.UnsupportedOperationException();
}
@Override
public java.util.concurrent.Future tagResourceAsync(TagResourceRequest request) {
return tagResourceAsync(request, null);
}
@Override
public java.util.concurrent.Future tagResourceAsync(TagResourceRequest request,
com.amazonaws.handlers.AsyncHandler asyncHandler) {
throw new java.lang.UnsupportedOperationException();
}
@Override
public java.util.concurrent.Future untagResourceAsync(UntagResourceRequest request) {
return untagResourceAsync(request, null);
}
@Override
public java.util.concurrent.Future untagResourceAsync(UntagResourceRequest request,
com.amazonaws.handlers.AsyncHandler asyncHandler) {
throw new java.lang.UnsupportedOperationException();
}
@Override
public java.util.concurrent.Future updateServiceLevelObjectiveAsync(UpdateServiceLevelObjectiveRequest request) {
return updateServiceLevelObjectiveAsync(request, null);
}
@Override
public java.util.concurrent.Future updateServiceLevelObjectiveAsync(UpdateServiceLevelObjectiveRequest request,
com.amazonaws.handlers.AsyncHandler asyncHandler) {
throw new java.lang.UnsupportedOperationException();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy